_BSP_User_Manual
|
stm3210c_eval_lcd.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm3210c_eval_lcd.c 00004 * @author MCD Application Team 00005 * @version $VERSION$ 00006 * @date $DATE$ 00007 * @brief This file includes the driver for Liquid Crystal Display (LCD) module 00008 * mounted on STM3210C-EVAL evaluation board. 00009 @verbatim 00010 ============================================================================== 00011 ##### How to use this driver ##### 00012 ============================================================================== 00013 [..] 00014 (#) This driver is used to drive indirectly an LCD TFT. 00015 00016 (#) This driver supports the AM-240320L8TNQW00H (LCD_ILI9320) and AM240320D5TOQW01H 00017 (LCD_ILI9325) LCD mounted on MB785 daughter board 00018 00019 (#) The ILI9320 and ILI9325 components driver MUST be included with this driver. 00020 00021 (#) Initialization steps: 00022 (++) Initialize the LCD using the BSP_LCD_Init() function. 00023 00024 (#) Display on LCD 00025 (++) Clear the hole LCD using yhe BSP_LCD_Clear() function or only one specified 00026 string line using the BSP_LCD_ClearStringLine() function. 00027 (++) Display a character on the specified line and column using the BSP_LCD_DisplayChar() 00028 function or a complete string line using the BSP_LCD_DisplayStringAtLine() function. 00029 (++) Display a string line on the specified position (x,y in pixel) and align mode 00030 using the BSP_LCD_DisplayStringAtLine() function. 00031 (++) Draw and fill a basic shapes (dot, line, rectangle, circle, ellipse, .. bitmap, raw picture) 00032 on LCD using a set of functions. 00033 @endverbatim 00034 ****************************************************************************** 00035 * @attention 00036 * 00037 * <h2><center>© COPYRIGHT(c) 2014 STMicroelectronics</center></h2> 00038 * 00039 * Redistribution and use in source and binary forms, with or without modification, 00040 * are permitted provided that the following conditions are met: 00041 * 1. Redistributions of source code must retain the above copyright notice, 00042 * this list of conditions and the following disclaimer. 00043 * 2. Redistributions in binary form must reproduce the above copyright notice, 00044 * this list of conditions and the following disclaimer in the documentation 00045 * and/or other materials provided with the distribution. 00046 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00047 * may be used to endorse or promote products derived from this software 00048 * without specific prior written permission. 00049 * 00050 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00051 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00052 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00053 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00054 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00055 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00056 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00057 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00058 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00059 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00060 * 00061 ****************************************************************************** 00062 */ 00063 00064 /* Includes ------------------------------------------------------------------*/ 00065 #include "stm3210c_eval_lcd.h" 00066 #include "../../../Utilities/Fonts/fonts.h" 00067 #include "../../../Utilities/Fonts/font24.c" 00068 #include "../../../Utilities/Fonts/font20.c" 00069 #include "../../../Utilities/Fonts/font16.c" 00070 #include "../../../Utilities/Fonts/font12.c" 00071 #include "../../../Utilities/Fonts/font8.c" 00072 00073 /** @addtogroup BSP 00074 * @{ 00075 */ 00076 00077 /** @addtogroup STM3210C_EVAL 00078 * @{ 00079 */ 00080 00081 /** @defgroup STM3210C_EVAL_LCD STM3210C_EVAL LCD 00082 * @{ 00083 */ 00084 00085 00086 /** @defgroup STM3210C_EVAL_LCD_Private_Defines Private Defines 00087 * @{ 00088 */ 00089 #define POLY_X(Z) ((int32_t)((Points + (Z))->X)) 00090 #define POLY_Y(Z) ((int32_t)((Points + (Z))->Y)) 00091 00092 #define MAX_HEIGHT_FONT 17 00093 #define MAX_WIDTH_FONT 24 00094 #define OFFSET_BITMAP 54 00095 /** 00096 * @} 00097 */ 00098 00099 /** @defgroup STM3210C_EVAL_LCD_Private_Macros Private Macros 00100 * @{ 00101 */ 00102 #define ABS(X) ((X) > 0 ? (X) : -(X)) 00103 00104 /** 00105 * @} 00106 */ 00107 00108 /** @defgroup STM3210C_EVAL_LCD_Private_Variables Private Variables 00109 * @{ 00110 */ 00111 LCD_DrawPropTypeDef DrawProp; 00112 00113 static LCD_DrvTypeDef *lcd_drv; 00114 00115 /* Max size of bitmap will based on a font24 (17x24) */ 00116 static uint8_t bitmap[MAX_HEIGHT_FONT*MAX_WIDTH_FONT*2+OFFSET_BITMAP] = {0}; 00117 00118 /** 00119 * @} 00120 */ 00121 00122 /** @defgroup STM3210C_EVAL_LCD_Private_Functions Private Functions 00123 * @{ 00124 */ 00125 static void LCD_DrawPixel(uint16_t Xpos, uint16_t Ypos, uint16_t RGBCode); 00126 static void LCD_DrawChar(uint16_t Xpos, uint16_t Ypos, const uint8_t *c); 00127 static void LCD_SetDisplayWindow(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height); 00128 /** 00129 * @} 00130 */ 00131 00132 00133 /** @defgroup STM3210C_EVAL_LCD_Exported_Functions Exported Functions 00134 * @{ 00135 */ 00136 00137 /** 00138 * @brief Initializes the LCD. 00139 * @retval LCD state 00140 */ 00141 uint8_t BSP_LCD_Init(void) 00142 { 00143 uint8_t ret = LCD_ERROR; 00144 00145 /* Default value for draw propriety */ 00146 DrawProp.BackColor = 0xFFFF; 00147 DrawProp.pFont = &Font24; 00148 DrawProp.TextColor = 0x0000; 00149 00150 if(ili9320_drv.ReadID() == ILI9320_ID) 00151 { 00152 lcd_drv = &ili9320_drv; 00153 00154 /* LCD Init */ 00155 lcd_drv->Init(); 00156 00157 /* Initialize the font */ 00158 BSP_LCD_SetFont(&LCD_DEFAULT_FONT); 00159 00160 ret = LCD_OK; 00161 } 00162 else if(ili9325_drv.ReadID() == ILI9325_ID) 00163 { 00164 lcd_drv = &ili9325_drv; 00165 00166 /* LCD Init */ 00167 lcd_drv->Init(); 00168 00169 /* Initialize the font */ 00170 BSP_LCD_SetFont(&LCD_DEFAULT_FONT); 00171 00172 ret = LCD_OK; 00173 } 00174 00175 return ret; 00176 } 00177 00178 /** 00179 * @brief Gets the LCD X size. 00180 * @retval Used LCD X size 00181 */ 00182 uint32_t BSP_LCD_GetXSize(void) 00183 { 00184 return(lcd_drv->GetLcdPixelWidth()); 00185 } 00186 00187 /** 00188 * @brief Gets the LCD Y size. 00189 * @retval Used LCD Y size 00190 */ 00191 uint32_t BSP_LCD_GetYSize(void) 00192 { 00193 return(lcd_drv->GetLcdPixelHeight()); 00194 } 00195 00196 /** 00197 * @brief Gets the LCD text color. 00198 * @retval Used text color. 00199 */ 00200 uint16_t BSP_LCD_GetTextColor(void) 00201 { 00202 return DrawProp.TextColor; 00203 } 00204 00205 /** 00206 * @brief Gets the LCD background color. 00207 * @retval Used background color 00208 */ 00209 uint16_t BSP_LCD_GetBackColor(void) 00210 { 00211 return DrawProp.BackColor; 00212 } 00213 00214 /** 00215 * @brief Sets the LCD text color. 00216 * @param Color: Text color code RGB(5-6-5) 00217 * @retval None 00218 */ 00219 void BSP_LCD_SetTextColor(uint16_t Color) 00220 { 00221 DrawProp.TextColor = Color; 00222 } 00223 00224 /** 00225 * @brief Sets the LCD background color. 00226 * @param Color: Background color code RGB(5-6-5) 00227 * @retval None 00228 */ 00229 void BSP_LCD_SetBackColor(uint16_t Color) 00230 { 00231 DrawProp.BackColor = Color; 00232 } 00233 00234 /** 00235 * @brief Sets the LCD text font. 00236 * @param pFonts: Font to be used 00237 * @retval None 00238 */ 00239 void BSP_LCD_SetFont(sFONT *pFonts) 00240 { 00241 DrawProp.pFont = pFonts; 00242 } 00243 00244 /** 00245 * @brief Gets the LCD text font. 00246 * @retval Used font 00247 */ 00248 sFONT *BSP_LCD_GetFont(void) 00249 { 00250 return DrawProp.pFont; 00251 } 00252 00253 /** 00254 * @brief Clears the hole LCD. 00255 * @param Color: Color of the background 00256 * @retval None 00257 */ 00258 void BSP_LCD_Clear(uint16_t Color) 00259 { 00260 uint32_t counter = 0; 00261 00262 uint32_t color_backup = DrawProp.TextColor; 00263 DrawProp.TextColor = Color; 00264 00265 for(counter = 0; counter < BSP_LCD_GetYSize(); counter++) 00266 { 00267 BSP_LCD_DrawHLine(0, counter, BSP_LCD_GetXSize()); 00268 } 00269 00270 DrawProp.TextColor = color_backup; 00271 BSP_LCD_SetTextColor(DrawProp.TextColor); 00272 } 00273 00274 /** 00275 * @brief Clears the selected line. 00276 * @param Line: Line to be cleared 00277 * This parameter can be one of the following values: 00278 * @arg 0..9: if the Current fonts is Font16x24 00279 * @arg 0..19: if the Current fonts is Font12x12 or Font8x12 00280 * @arg 0..29: if the Current fonts is Font8x8 00281 * @retval None 00282 */ 00283 void BSP_LCD_ClearStringLine(uint16_t Line) 00284 { 00285 uint32_t colorbackup = DrawProp.TextColor; 00286 DrawProp.TextColor = DrawProp.BackColor;; 00287 00288 /* Draw a rectangle with background color */ 00289 BSP_LCD_FillRect(0, (Line * DrawProp.pFont->Height), BSP_LCD_GetXSize(), DrawProp.pFont->Height); 00290 00291 DrawProp.TextColor = colorbackup; 00292 BSP_LCD_SetTextColor(DrawProp.TextColor); 00293 } 00294 00295 /** 00296 * @brief Displays one character. 00297 * @param Xpos: Start column address 00298 * @param Ypos: Line where to display the character shape. 00299 * @param Ascii: Character ascii code 00300 * This parameter must be a number between Min_Data = 0x20 and Max_Data = 0x7E 00301 * @retval None 00302 */ 00303 void BSP_LCD_DisplayChar(uint16_t Xpos, uint16_t Ypos, uint8_t Ascii) 00304 { 00305 LCD_DrawChar(Xpos, Ypos, &DrawProp.pFont->table[(Ascii-' ') *\ 00306 DrawProp.pFont->Height * ((DrawProp.pFont->Width + 7) / 8)]); 00307 } 00308 00309 /** 00310 * @brief Displays characters on the LCD. 00311 * @param Xpos: X position (in pixel) 00312 * @param Ypos: Y position (in pixel) 00313 * @param pText: Pointer to string to display on LCD 00314 * @param Mode: Display mode 00315 * This parameter can be one of the following values: 00316 * @arg CENTER_MODE 00317 * @arg RIGHT_MODE 00318 * @arg LEFT_MODE 00319 * @retval None 00320 */ 00321 void BSP_LCD_DisplayStringAt(uint16_t Xpos, uint16_t Ypos, uint8_t *pText, Line_ModeTypdef Mode) 00322 { 00323 uint16_t refcolumn = 1, counter = 0; 00324 uint32_t size = 0, xsize = 0; 00325 uint8_t *ptr = pText; 00326 00327 /* Get the text size */ 00328 while (*ptr++) size ++ ; 00329 00330 /* Characters number per line */ 00331 xsize = (BSP_LCD_GetXSize()/DrawProp.pFont->Width); 00332 00333 switch (Mode) 00334 { 00335 case CENTER_MODE: 00336 { 00337 refcolumn = Xpos + ((xsize - size)* DrawProp.pFont->Width) / 2; 00338 break; 00339 } 00340 case LEFT_MODE: 00341 { 00342 refcolumn = Xpos; 00343 break; 00344 } 00345 case RIGHT_MODE: 00346 { 00347 refcolumn = - Xpos + ((xsize - size)*DrawProp.pFont->Width); 00348 break; 00349 } 00350 default: 00351 { 00352 refcolumn = Xpos; 00353 break; 00354 } 00355 } 00356 00357 /* Send the string character by character on lCD */ 00358 while ((*pText != 0) & (((BSP_LCD_GetXSize() - (counter*DrawProp.pFont->Width)) & 0xFFFF) >= DrawProp.pFont->Width)) 00359 { 00360 /* Display one character on LCD */ 00361 BSP_LCD_DisplayChar(refcolumn, Ypos, *pText); 00362 /* Decrement the column position by 16 */ 00363 refcolumn += DrawProp.pFont->Width; 00364 /* Point on the next character */ 00365 pText++; 00366 counter++; 00367 } 00368 } 00369 00370 /** 00371 * @brief Displays a character on the LCD. 00372 * @param Line: Line where to display the character shape 00373 * This parameter can be one of the following values: 00374 * @arg 0..9: if the Current fonts is Font16x24 00375 * @arg 0..19: if the Current fonts is Font12x12 or Font8x12 00376 * @arg 0..29: if the Current fonts is Font8x8 00377 * @param pText: Pointer to string to display on LCD 00378 * @retval None 00379 */ 00380 void BSP_LCD_DisplayStringAtLine(uint16_t Line, uint8_t *pText) 00381 { 00382 BSP_LCD_DisplayStringAt(0, LINE(Line),pText, LEFT_MODE); 00383 } 00384 00385 /** 00386 * @brief Reads an LCD pixel. 00387 * @param Xpos: X position 00388 * @param Ypos: Y position 00389 * @retval RGB pixel color 00390 */ 00391 uint16_t BSP_LCD_ReadPixel(uint16_t Xpos, uint16_t Ypos) 00392 { 00393 uint16_t ret = 0; 00394 00395 if(lcd_drv->ReadPixel != NULL) 00396 { 00397 ret = lcd_drv->ReadPixel(Xpos, Ypos); 00398 } 00399 00400 return ret; 00401 } 00402 00403 /** 00404 * @brief Draws an horizontal line. 00405 * @param Xpos: X position 00406 * @param Ypos: Y position 00407 * @param Length: Line length 00408 * @retval None 00409 */ 00410 void BSP_LCD_DrawHLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length) 00411 { 00412 uint32_t index = 0; 00413 00414 if(lcd_drv->DrawHLine != NULL) 00415 { 00416 lcd_drv->DrawHLine(DrawProp.TextColor, Xpos, Ypos, Length); 00417 } 00418 else 00419 { 00420 for(index = 0; index < Length; index++) 00421 { 00422 LCD_DrawPixel((Xpos + index), Ypos, DrawProp.TextColor); 00423 } 00424 } 00425 } 00426 00427 /** 00428 * @brief Draws a vertical line. 00429 * @param Xpos: X position 00430 * @param Ypos: Y position 00431 * @param Length: Line length 00432 * @retval None 00433 */ 00434 void BSP_LCD_DrawVLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length) 00435 { 00436 uint32_t index = 0; 00437 00438 if(lcd_drv->DrawVLine != NULL) 00439 { 00440 lcd_drv->DrawVLine(DrawProp.TextColor, Xpos, Ypos, Length); 00441 } 00442 else 00443 { 00444 for(index = 0; index < Length; index++) 00445 { 00446 LCD_DrawPixel(Xpos, Ypos + index, DrawProp.TextColor); 00447 } 00448 } 00449 } 00450 00451 /** 00452 * @brief Draws an uni-line (between two points). 00453 * @param x1: Point 1 X position 00454 * @param y1: Point 1 Y position 00455 * @param x2: Point 2 X position 00456 * @param y2: Point 2 Y position 00457 * @retval None 00458 */ 00459 void BSP_LCD_DrawLine(uint16_t x1, uint16_t y1, uint16_t x2, uint16_t y2) 00460 { 00461 int16_t deltax = 0, deltay = 0, x = 0, y = 0, xinc1 = 0, xinc2 = 0, 00462 yinc1 = 0, yinc2 = 0, den = 0, num = 0, numadd = 0, numpixels = 0, 00463 curpixel = 0; 00464 00465 deltax = ABS(x2 - x1); /* The difference between the x's */ 00466 deltay = ABS(y2 - y1); /* The difference between the y's */ 00467 x = x1; /* Start x off at the first pixel */ 00468 y = y1; /* Start y off at the first pixel */ 00469 00470 if (x2 >= x1) /* The x-values are increasing */ 00471 { 00472 xinc1 = 1; 00473 xinc2 = 1; 00474 } 00475 else /* The x-values are decreasing */ 00476 { 00477 xinc1 = -1; 00478 xinc2 = -1; 00479 } 00480 00481 if (y2 >= y1) /* The y-values are increasing */ 00482 { 00483 yinc1 = 1; 00484 yinc2 = 1; 00485 } 00486 else /* The y-values are decreasing */ 00487 { 00488 yinc1 = -1; 00489 yinc2 = -1; 00490 } 00491 00492 if (deltax >= deltay) /* There is at least one x-value for every y-value */ 00493 { 00494 xinc1 = 0; /* Don't change the x when numerator >= denominator */ 00495 yinc2 = 0; /* Don't change the y for every iteration */ 00496 den = deltax; 00497 num = deltax / 2; 00498 numadd = deltay; 00499 numpixels = deltax; /* There are more x-values than y-values */ 00500 } 00501 else /* There is at least one y-value for every x-value */ 00502 { 00503 xinc2 = 0; /* Don't change the x for every iteration */ 00504 yinc1 = 0; /* Don't change the y when numerator >= denominator */ 00505 den = deltay; 00506 num = deltay / 2; 00507 numadd = deltax; 00508 numpixels = deltay; /* There are more y-values than x-values */ 00509 } 00510 00511 for (curpixel = 0; curpixel <= numpixels; curpixel++) 00512 { 00513 LCD_DrawPixel(x, y, DrawProp.TextColor); /* Draw the current pixel */ 00514 num += numadd; /* Increase the numerator by the top of the fraction */ 00515 if (num >= den) /* Check if numerator >= denominator */ 00516 { 00517 num -= den; /* Calculate the new numerator value */ 00518 x += xinc1; /* Change the x as appropriate */ 00519 y += yinc1; /* Change the y as appropriate */ 00520 } 00521 x += xinc2; /* Change the x as appropriate */ 00522 y += yinc2; /* Change the y as appropriate */ 00523 } 00524 } 00525 00526 /** 00527 * @brief Draws a rectangle. 00528 * @param Xpos: X position 00529 * @param Ypos: Y position 00530 * @param Width: Rectangle width 00531 * @param Height: Rectangle height 00532 * @retval None 00533 */ 00534 void BSP_LCD_DrawRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 00535 { 00536 /* Draw horizontal lines */ 00537 BSP_LCD_DrawHLine(Xpos, Ypos, Width); 00538 BSP_LCD_DrawHLine(Xpos, (Ypos+ Height), Width); 00539 00540 /* Draw vertical lines */ 00541 BSP_LCD_DrawVLine(Xpos, Ypos, Height); 00542 BSP_LCD_DrawVLine((Xpos + Width), Ypos, Height); 00543 } 00544 00545 /** 00546 * @brief Draws a circle. 00547 * @param Xpos: X position 00548 * @param Ypos: Y position 00549 * @param Radius: Circle radius 00550 * @retval None 00551 */ 00552 void BSP_LCD_DrawCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius) 00553 { 00554 int32_t D; /* Decision Variable */ 00555 uint32_t CurX; /* Current X Value */ 00556 uint32_t CurY; /* Current Y Value */ 00557 00558 D = 3 - (Radius << 1); 00559 CurX = 0; 00560 CurY = Radius; 00561 00562 while (CurX <= CurY) 00563 { 00564 LCD_DrawPixel((Xpos + CurX), (Ypos - CurY), DrawProp.TextColor); 00565 00566 LCD_DrawPixel((Xpos - CurX), (Ypos - CurY), DrawProp.TextColor); 00567 00568 LCD_DrawPixel((Xpos + CurY), (Ypos - CurX), DrawProp.TextColor); 00569 00570 LCD_DrawPixel((Xpos - CurY), (Ypos - CurX), DrawProp.TextColor); 00571 00572 LCD_DrawPixel((Xpos + CurX), (Ypos + CurY), DrawProp.TextColor); 00573 00574 LCD_DrawPixel((Xpos - CurX), (Ypos + CurY), DrawProp.TextColor); 00575 00576 LCD_DrawPixel((Xpos + CurY), (Ypos + CurX), DrawProp.TextColor); 00577 00578 LCD_DrawPixel((Xpos - CurY), (Ypos + CurX), DrawProp.TextColor); 00579 00580 /* Initialize the font */ 00581 BSP_LCD_SetFont(&LCD_DEFAULT_FONT); 00582 00583 if (D < 0) 00584 { 00585 D += (CurX << 2) + 6; 00586 } 00587 else 00588 { 00589 D += ((CurX - CurY) << 2) + 10; 00590 CurY--; 00591 } 00592 CurX++; 00593 } 00594 } 00595 00596 /** 00597 * @brief Draws an poly-line (between many points). 00598 * @param Points: Pointer to the points array 00599 * @param PointCount: Number of points 00600 * @retval None 00601 */ 00602 void BSP_LCD_DrawPolygon(pPoint Points, uint16_t PointCount) 00603 { 00604 int16_t X = 0, Y = 0; 00605 00606 if(PointCount < 2) 00607 { 00608 return; 00609 } 00610 00611 BSP_LCD_DrawLine(Points->X, Points->Y, (Points+PointCount-1)->X, (Points+PointCount-1)->Y); 00612 00613 while(--PointCount) 00614 { 00615 X = Points->X; 00616 Y = Points->Y; 00617 Points++; 00618 BSP_LCD_DrawLine(X, Y, Points->X, Points->Y); 00619 } 00620 00621 } 00622 00623 /** 00624 * @brief Draws an ellipse on LCD. 00625 * @param Xpos: X position 00626 * @param Ypos: Y position 00627 * @param XRadius: Ellipse X radius 00628 * @param YRadius: Ellipse Y radius 00629 * @retval None 00630 */ 00631 void BSP_LCD_DrawEllipse(int Xpos, int Ypos, int XRadius, int YRadius) 00632 { 00633 int x = 0, y = -YRadius, err = 2-2*XRadius, e2; 00634 float K = 0, rad1 = 0, rad2 = 0; 00635 00636 rad1 = XRadius; 00637 rad2 = YRadius; 00638 00639 K = (float)(rad2/rad1); 00640 00641 do { 00642 LCD_DrawPixel((Xpos-(uint16_t)(x/K)), (Ypos+y), DrawProp.TextColor); 00643 LCD_DrawPixel((Xpos+(uint16_t)(x/K)), (Ypos+y), DrawProp.TextColor); 00644 LCD_DrawPixel((Xpos+(uint16_t)(x/K)), (Ypos-y), DrawProp.TextColor); 00645 LCD_DrawPixel((Xpos-(uint16_t)(x/K)), (Ypos-y), DrawProp.TextColor); 00646 00647 e2 = err; 00648 if (e2 <= x) { 00649 err += ++x*2+1; 00650 if (-y == x && e2 <= y) e2 = 0; 00651 } 00652 if (e2 > y) err += ++y*2+1; 00653 } 00654 while (y <= 0); 00655 } 00656 00657 /** 00658 * @brief Draws a bitmap picture (16 bpp). 00659 * @param Xpos: Bmp X position in the LCD 00660 * @param Ypos: Bmp Y position in the LCD 00661 * @param pbmp: Pointer to Bmp picture address. 00662 * @retval None 00663 */ 00664 void BSP_LCD_DrawBitmap(uint16_t Xpos, uint16_t Ypos, uint8_t *pbmp) 00665 { 00666 uint32_t height = 0; 00667 uint32_t width = 0; 00668 00669 00670 /* Read bitmap width */ 00671 width = *(uint16_t *) (pbmp + 18); 00672 width |= (*(uint16_t *) (pbmp + 20)) << 16; 00673 00674 /* Read bitmap height */ 00675 height = *(uint16_t *) (pbmp + 22); 00676 height |= (*(uint16_t *) (pbmp + 24)) << 16; 00677 00678 LCD_SetDisplayWindow(Xpos, Ypos, width, height); 00679 00680 if(lcd_drv->DrawBitmap != NULL) 00681 { 00682 lcd_drv->DrawBitmap(Xpos, Ypos, pbmp); 00683 } 00684 LCD_SetDisplayWindow(0, 0, BSP_LCD_GetXSize(), BSP_LCD_GetYSize()); 00685 } 00686 00687 /** 00688 * @brief Draws RGB Image (16 bpp). 00689 * @param Xpos: X position in the LCD 00690 * @param Ypos: Y position in the LCD 00691 * @param Xsize: X size in the LCD 00692 * @param Ysize: Y size in the LCD 00693 * @param pdata: Pointer to the RGB Image address. 00694 * @retval None 00695 */ 00696 void BSP_LCD_DrawRGBImage(uint16_t Xpos, uint16_t Ypos, uint16_t Xsize, uint16_t Ysize, uint8_t *pdata) 00697 { 00698 00699 LCD_SetDisplayWindow(Xpos, Ypos, Xsize, Ysize); 00700 00701 if(lcd_drv->DrawRGBImage != NULL) 00702 { 00703 lcd_drv->DrawRGBImage(Xpos, Ypos, Xsize, Ysize, pdata); 00704 } 00705 LCD_SetDisplayWindow(0, 0, BSP_LCD_GetXSize(), BSP_LCD_GetYSize()); 00706 } 00707 00708 /** 00709 * @brief Draws a full rectangle. 00710 * @param Xpos: X position 00711 * @param Ypos: Y position 00712 * @param Width: Rectangle width 00713 * @param Height: Rectangle height 00714 * @retval None 00715 */ 00716 void BSP_LCD_FillRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 00717 { 00718 BSP_LCD_SetTextColor(DrawProp.TextColor); 00719 do 00720 { 00721 BSP_LCD_DrawHLine(Xpos, Ypos++, Width); 00722 } 00723 while(Height--); 00724 } 00725 00726 /** 00727 * @brief Draws a full circle. 00728 * @param Xpos: X position 00729 * @param Ypos: Y position 00730 * @param Radius: Circle radius 00731 * @retval None 00732 */ 00733 void BSP_LCD_FillCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius) 00734 { 00735 int32_t D; /* Decision Variable */ 00736 uint32_t CurX; /* Current X Value */ 00737 uint32_t CurY; /* Current Y Value */ 00738 00739 D = 3 - (Radius << 1); 00740 00741 CurX = 0; 00742 CurY = Radius; 00743 00744 BSP_LCD_SetTextColor(DrawProp.TextColor); 00745 00746 while (CurX <= CurY) 00747 { 00748 if(CurY > 0) 00749 { 00750 BSP_LCD_DrawHLine(Xpos - CurY, Ypos + CurX, 2*CurY); 00751 BSP_LCD_DrawHLine(Xpos - CurY, Ypos - CurX, 2*CurY); 00752 } 00753 00754 if(CurX > 0) 00755 { 00756 BSP_LCD_DrawHLine(Xpos - CurX, Ypos - CurY, 2*CurX); 00757 BSP_LCD_DrawHLine(Xpos - CurX, Ypos + CurY, 2*CurX); 00758 } 00759 if (D < 0) 00760 { 00761 D += (CurX << 2) + 6; 00762 } 00763 else 00764 { 00765 D += ((CurX - CurY) << 2) + 10; 00766 CurY--; 00767 } 00768 CurX++; 00769 } 00770 00771 BSP_LCD_SetTextColor(DrawProp.TextColor); 00772 BSP_LCD_DrawCircle(Xpos, Ypos, Radius); 00773 } 00774 00775 /** 00776 * @brief Draws a full ellipse. 00777 * @param Xpos: X position 00778 * @param Ypos: Y position 00779 * @param XRadius: Ellipse X radius 00780 * @param YRadius: Ellipse Y radius 00781 * @retval None 00782 */ 00783 void BSP_LCD_FillEllipse(int Xpos, int Ypos, int XRadius, int YRadius) 00784 { 00785 int x = 0, y = -YRadius, err = 2-2*XRadius, e2; 00786 float K = 0, rad1 = 0, rad2 = 0; 00787 00788 rad1 = XRadius; 00789 rad2 = YRadius; 00790 00791 K = (float)(rad2/rad1); 00792 00793 do 00794 { 00795 BSP_LCD_DrawHLine((Xpos-(uint16_t)(x/K)), (Ypos+y), (2*(uint16_t)(x/K) + 1)); 00796 BSP_LCD_DrawHLine((Xpos-(uint16_t)(x/K)), (Ypos-y), (2*(uint16_t)(x/K) + 1)); 00797 00798 e2 = err; 00799 if (e2 <= x) 00800 { 00801 err += ++x*2+1; 00802 if (-y == x && e2 <= y) e2 = 0; 00803 } 00804 if (e2 > y) err += ++y*2+1; 00805 } 00806 while (y <= 0); 00807 } 00808 00809 /** 00810 * @brief Enables the display. 00811 * @retval None 00812 */ 00813 void BSP_LCD_DisplayOn(void) 00814 { 00815 lcd_drv->DisplayOn(); 00816 } 00817 00818 /** 00819 * @brief Disables the display. 00820 * @retval None 00821 */ 00822 void BSP_LCD_DisplayOff(void) 00823 { 00824 lcd_drv->DisplayOff(); 00825 } 00826 00827 /** 00828 * @} 00829 */ 00830 00831 /** @addtogroup STM3210C_EVAL_LCD_Private_Functions 00832 * @{ 00833 */ 00834 00835 /****************************************************************************** 00836 Static Function 00837 *******************************************************************************/ 00838 /** 00839 * @brief Draws a pixel on LCD. 00840 * @param Xpos: X position 00841 * @param Ypos: Y position 00842 * @param RGBCode: Pixel color in RGB mode (5-6-5) 00843 * @retval None 00844 */ 00845 static void LCD_DrawPixel(uint16_t Xpos, uint16_t Ypos, uint16_t RGBCode) 00846 { 00847 if(lcd_drv->WritePixel != NULL) 00848 { 00849 lcd_drv->WritePixel(Xpos, Ypos, RGBCode); 00850 } 00851 } 00852 00853 /** 00854 * @brief Draws a character on LCD. 00855 * @param Xpos: Line where to display the character shape 00856 * @param Ypos: Start column address 00857 * @param pChar: Pointer to the character data 00858 * @retval None 00859 */ 00860 static void LCD_DrawChar(uint16_t Xpos, uint16_t Ypos, const uint8_t *pChar) 00861 { 00862 uint32_t counterh = 0, counterw = 0, index = 0; 00863 uint16_t height = 0, width = 0; 00864 uint8_t offset = 0; 00865 uint8_t *pchar = NULL; 00866 uint32_t line = 0; 00867 00868 00869 height = DrawProp.pFont->Height; 00870 width = DrawProp.pFont->Width; 00871 00872 /* Fill bitmap header*/ 00873 *(uint16_t *) (bitmap + 2) = (uint16_t)(height*width*2+OFFSET_BITMAP); 00874 *(uint16_t *) (bitmap + 4) = (uint16_t)((height*width*2+OFFSET_BITMAP)>>16); 00875 *(uint16_t *) (bitmap + 10) = OFFSET_BITMAP; 00876 *(uint16_t *) (bitmap + 18) = (uint16_t)(width); 00877 *(uint16_t *) (bitmap + 20) = (uint16_t)((width)>>16); 00878 *(uint16_t *) (bitmap + 22) = (uint16_t)(height); 00879 *(uint16_t *) (bitmap + 24) = (uint16_t)((height)>>16); 00880 00881 offset = 8 *((width + 7)/8) - width ; 00882 00883 for(counterh = 0; counterh < height; counterh++) 00884 { 00885 pchar = ((uint8_t *)pChar + (width + 7)/8 * counterh); 00886 00887 if(((width + 7)/8) == 3) 00888 { 00889 line = (pchar[0]<< 16) | (pchar[1]<< 8) | pchar[2]; 00890 } 00891 00892 if(((width + 7)/8) == 2) 00893 { 00894 line = (pchar[0]<< 8) | pchar[1]; 00895 } 00896 00897 if(((width + 7)/8) == 1) 00898 { 00899 line = pchar[0]; 00900 } 00901 00902 for (counterw = 0; counterw < width; counterw++) 00903 { 00904 /* Image in the bitmap is written from the bottom to the top */ 00905 /* Need to invert image in the bitmap */ 00906 index = (((height-counterh-1)*width)+(counterw))*2+OFFSET_BITMAP; 00907 if(line & (1 << (width- counterw + offset- 1))) 00908 { 00909 bitmap[index] = (uint8_t)DrawProp.TextColor; 00910 bitmap[index+1] = (uint8_t)(DrawProp.TextColor >> 8); 00911 } 00912 else 00913 { 00914 bitmap[index] = (uint8_t)DrawProp.BackColor; 00915 bitmap[index+1] = (uint8_t)(DrawProp.BackColor >> 8); 00916 } 00917 } 00918 } 00919 00920 BSP_LCD_DrawBitmap(Xpos, Ypos, bitmap); 00921 } 00922 00923 /** 00924 * @brief Sets display window. 00925 * @param Xpos: LCD X position 00926 * @param Ypos: LCD Y position 00927 * @param Width: LCD window width 00928 * @param Height: LCD window height 00929 * @retval None 00930 */ 00931 static void LCD_SetDisplayWindow(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 00932 { 00933 if(lcd_drv->SetDisplayWindow != NULL) 00934 { 00935 lcd_drv->SetDisplayWindow(Xpos, Ypos, Width, Height); 00936 } 00937 } 00938 00939 /** 00940 * @} 00941 */ 00942 00943 /** 00944 * @} 00945 */ 00946 00947 /** 00948 * @} 00949 */ 00950 00951 /** 00952 * @} 00953 */ 00954 00955 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 00956
Generated on Thu Dec 11 2014 15:38:29 for _BSP_User_Manual by
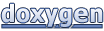