_BSP_User_Manual
|
stm3210c_eval_audio.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm3210c_eval_audio.c 00004 * @author MCD Application Team 00005 * @version $VERSION$ 00006 * @date $DATE$ 00007 * @brief This file provides the Audio driver for the STM3210C-Eval 00008 * board. 00009 @verbatim 00010 ============================================================================== 00011 ##### How to use this driver ##### 00012 ============================================================================== 00013 [..] 00014 (#) This driver supports STM32F107xC devices on STM3210C-Eval Kit: 00015 (++) to play an audio file (all functions names start by BSP_AUDIO_OUT_xxx) 00016 00017 [..] 00018 (#) PLAY A FILE: 00019 (++) Call the function BSP_AUDIO_OUT_Init( 00020 OutputDevice: physical output mode (OUTPUT_DEVICE_SPEAKER, 00021 OUTPUT_DEVICE_HEADPHONE, OUTPUT_DEVICE_AUTO or 00022 OUTPUT_DEVICE_BOTH) 00023 Volume: initial volume to be set (0 is min (mute), 100 is max (100%) 00024 AudioFreq: Audio frequency in Hz (8000, 16000, 22500, 32000 ...) 00025 this parameter is relative to the audio file/stream type. 00026 ) 00027 This function configures all the hardware required for the audio application 00028 (codec, I2C, I2S, GPIOs, DMA and interrupt if needed). This function returns 0 00029 if configuration is OK. 00030 If the returned value is different from 0 or the function is stuck then the 00031 communication with the codec (try to un-plug the power or reset device in this case). 00032 (+++) OUTPUT_DEVICE_SPEAKER: only speaker will be set as output for the 00033 audio stream. 00034 (+++) OUTPUT_DEVICE_HEADPHONE: only headphones will be set as output for 00035 the audio stream. 00036 (+++) OUTPUT_DEVICE_AUTO: Selection of output device is made through external 00037 switch (implemented into the audio jack on the evaluation board). 00038 When the Headphone is connected it is used as output. 00039 When the headphone is disconnected from the audio jack, the output is 00040 automatically switched to Speaker. 00041 (+++) OUTPUT_DEVICE_BOTH: both Speaker and Headphone are used as outputs 00042 for the audio stream at the same time. 00043 (++) Call the function BSP_AUDIO_OUT_Play( 00044 pBuffer: pointer to the audio data file address 00045 Size: size of the buffer to be sent in Bytes 00046 ) 00047 to start playing (for the first time) from the audio file/stream. 00048 (++) Call the function BSP_AUDIO_OUT_Pause() to pause playing 00049 (++) Call the function BSP_AUDIO_OUT_Resume() to resume playing. 00050 Note. After calling BSP_AUDIO_OUT_Pause() function for pause, 00051 only BSP_AUDIO_OUT_Resume() should be called for resume 00052 (it is not allowed to call BSP_AUDIO_OUT_Play() in this case). 00053 Note. This function should be called only when the audio file is played 00054 or paused (not stopped). 00055 (++) For each mode, you may need to implement the relative callback functions 00056 into your code. 00057 The Callback functions are named BSP_AUDIO_OUT_XXXCallBack() and only 00058 their prototypes are declared in the stm3210c_eval_audio.h file. 00059 (refer to the example for more details on the callbacks implementations) 00060 (++) To Stop playing, to modify the volume level, the frequency or to mute, 00061 use the functions BSP_AUDIO_OUT_Stop(), BSP_AUDIO_OUT_SetVolume(), 00062 AUDIO_OUT_SetFrequency() BSP_AUDIO_OUT_SetOutputMode and BSP_AUDIO_OUT_SetMute(). 00063 (++) The driver API and the callback functions are at the end of the 00064 stm3210c_eval_audio.h file. 00065 00066 (++) This driver provide the High Audio Layer: consists of the function API 00067 exported in the stm3210c_eval_audio.h file (BSP_AUDIO_OUT_Init(), 00068 BSP_AUDIO_OUT_Play() ...) 00069 (++) This driver provide also the Media Access Layer (MAL): which consists 00070 of functions allowing to access the media containing/providing the 00071 audio file/stream. These functions are also included as local functions into 00072 the stm3210c_eval_audio.c file (I2SOUT_Init()...) 00073 00074 [..] 00075 ##### Known Limitations ##### 00076 ============================================================================== 00077 (#) When using the Speaker, if the audio file quality is not high enough, the 00078 speaker output may produce high and uncomfortable noise level. To avoid 00079 this issue, to use speaker output properly, try to increase audio file 00080 sampling rate (typically higher than 48KHz). 00081 This operation will lead to larger file size. 00082 00083 (#) Communication with the audio codec (through I2C) may be corrupted if it 00084 is interrupted by some user interrupt routines (in this case, interrupts 00085 could be disabled just before the start of communication then re-enabled 00086 when it is over). Note that this communication is only done at the 00087 configuration phase (BSP_AUDIO_OUT_Init() or BSP_AUDIO_OUT_Stop()) 00088 and when Volume control modification is performed (BSP_AUDIO_OUT_SetVolume() 00089 or BSP_AUDIO_OUT_SetMute()or BSP_AUDIO_OUT_SetOutputMode()). 00090 When the audio data is played, no communication is required with the audio codec. 00091 00092 (#) Parsing of audio file is not implemented (in order to determine audio file 00093 properties: Mono/Stereo, Data size, File size, Audio Frequency, Audio Data 00094 header size ...). The configuration is fixed for the given audio file. 00095 00096 (#) Mono audio streaming is not supported (in order to play mono audio streams, 00097 each data should be sent twice on the I2S or should be duplicated on the 00098 source buffer. Or convert the stream in stereo before playing). 00099 00100 (#) Supports only 16-bit audio data size. 00101 00102 @endverbatim 00103 ****************************************************************************** 00104 * @attention 00105 * 00106 * <h2><center>© COPYRIGHT(c) 2014 STMicroelectronics</center></h2> 00107 * 00108 * Redistribution and use in source and binary forms, with or without modification, 00109 * are permitted provided that the following conditions are met: 00110 * 1. Redistributions of source code must retain the above copyright notice, 00111 * this list of conditions and the following disclaimer. 00112 * 2. Redistributions in binary form must reproduce the above copyright notice, 00113 * this list of conditions and the following disclaimer in the documentation 00114 * and/or other materials provided with the distribution. 00115 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00116 * may be used to endorse or promote products derived from this software 00117 * without specific prior written permission. 00118 * 00119 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00120 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00121 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00122 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00123 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00124 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00125 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00126 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00127 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00128 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00129 * 00130 ****************************************************************************** 00131 */ 00132 00133 /* Includes ------------------------------------------------------------------*/ 00134 #include "stm3210c_eval_audio.h" 00135 00136 /** @addtogroup BSP 00137 * @{ 00138 */ 00139 00140 /** @addtogroup STM3210C_EVAL 00141 * @{ 00142 */ 00143 00144 /** @addtogroup STM3210C_EVAL_AUDIO 00145 * @brief This file includes the low layer audio driver available on STM3210C-Eval 00146 * eval board. 00147 * @{ 00148 */ 00149 00150 /** @defgroup STM3210C_EVAL_AUDIO_Private_Types AUDIO_Private_Types 00151 * @{ 00152 */ 00153 /** 00154 * @} 00155 */ 00156 00157 /** @defgroup STM3210C_EVAL_AUDIO_Private_Defines AUDIO_Private_Defines 00158 * @{ 00159 */ 00160 00161 /** 00162 * @} 00163 */ 00164 00165 /** @defgroup STM3210C_EVAL_AUDIO_Private_Macros AUDIO Private_Macros 00166 * @{ 00167 */ 00168 /** 00169 * @} 00170 */ 00171 00172 /** @defgroup STM3210C_EVAL_AUDIO_Private_Variables AUDIO_Private_Variables 00173 * @{ 00174 */ 00175 /*### PLAY ###*/ 00176 static AUDIO_DrvTypeDef *pAudioDrv; 00177 I2S_HandleTypeDef hAudioOutI2s; 00178 00179 /** 00180 * @} 00181 */ 00182 00183 /** @defgroup STM3210C_EVAL_AUDIO_Private_Function_Prototypes AUDIO_Private_Function_Prototypes 00184 * @{ 00185 */ 00186 static void I2SOUT_MspInit(void); 00187 static void I2SOUT_Init(uint32_t AudioFreq); 00188 00189 /** 00190 * @} 00191 */ 00192 00193 /** @defgroup STM3210C_EVAL_AUDIO_OUT_Exported_Functions AUDIO_OUT_Exported_Functions 00194 * @{ 00195 */ 00196 00197 /** 00198 * @brief Configure the audio peripherals. 00199 * @param OutputDevice: OUTPUT_DEVICE_SPEAKER, OUTPUT_DEVICE_HEADPHONE, 00200 * OUTPUT_DEVICE_BOTH or OUTPUT_DEVICE_AUTO . 00201 * @param Volume: Initial volume level (from 0 (Mute) to 100 (Max)) 00202 * @param AudioFreq: Audio frequency used to play the audio stream. 00203 * @retval 0 if correct communication, else wrong communication 00204 */ 00205 uint8_t BSP_AUDIO_OUT_Init(uint16_t OutputDevice, uint8_t Volume, uint32_t AudioFreq) 00206 { 00207 uint8_t ret = AUDIO_ERROR; 00208 uint32_t deviceid = 0x00; 00209 00210 deviceid = cs43l22_drv.ReadID(AUDIO_I2C_ADDRESS); 00211 00212 if((deviceid & CS43L22_ID_MASK) == CS43L22_ID) 00213 { 00214 /* Initialize the audio driver structure */ 00215 pAudioDrv = &cs43l22_drv; 00216 ret = AUDIO_OK; 00217 } 00218 else 00219 { 00220 ret = AUDIO_ERROR; 00221 } 00222 00223 if(ret == AUDIO_OK) 00224 { 00225 pAudioDrv->Init(AUDIO_I2C_ADDRESS, OutputDevice, Volume, AudioFreq); 00226 /* I2S data transfer preparation: 00227 Prepare the Media to be used for the audio transfer from memory to I2S peripheral */ 00228 /* Configure the I2S peripheral */ 00229 I2SOUT_Init(AudioFreq); 00230 } 00231 00232 return ret; 00233 } 00234 00235 /** 00236 * @brief Starts playing audio stream from a data buffer for a determined size. 00237 * @param pBuffer: Pointer to the buffer 00238 * @param Size: Number of audio data BYTES. 00239 * @retval AUDIO_OK if correct communication, else wrong communication 00240 */ 00241 uint8_t BSP_AUDIO_OUT_Play(uint16_t* pBuffer, uint32_t Size) 00242 { 00243 /* Call the audio Codec Play function */ 00244 if(pAudioDrv->Play(AUDIO_I2C_ADDRESS, pBuffer, Size) != 0) 00245 { 00246 return AUDIO_ERROR; 00247 } 00248 else 00249 { 00250 /* Update the Media layer and enable it for play */ 00251 HAL_I2S_Transmit_DMA(&hAudioOutI2s, pBuffer, DMA_MAX(Size)); 00252 00253 return AUDIO_OK; 00254 } 00255 } 00256 00257 /** 00258 * @brief Sends n-Bytes on the I2S interface. 00259 * @param pData: pointer on data address 00260 * @param Size: number of data to be written 00261 * @retval None 00262 */ 00263 void BSP_AUDIO_OUT_ChangeBuffer(uint16_t *pData, uint16_t Size) 00264 { 00265 HAL_I2S_Transmit_DMA(&hAudioOutI2s, pData, Size); 00266 } 00267 00268 /** 00269 * @brief This function Pauses the audio file stream. In case 00270 * of using DMA, the DMA Pause feature is used. 00271 * @note When calling BSP_AUDIO_OUT_Pause() function for pause, only 00272 * BSP_AUDIO_OUT_Resume() function should be called for resume (use of BSP_AUDIO_OUT_Play() 00273 * function for resume could lead to unexpected behavior). 00274 * @retval AUDIO_OK if correct communication, else wrong communication 00275 */ 00276 uint8_t BSP_AUDIO_OUT_Pause(void) 00277 { 00278 /* Call the Audio Codec Pause/Resume function */ 00279 if(pAudioDrv->Pause(AUDIO_I2C_ADDRESS) != 0) 00280 { 00281 return AUDIO_ERROR; 00282 } 00283 else 00284 { 00285 /* Call the Media layer pause function */ 00286 HAL_I2S_DMAPause(&hAudioOutI2s); 00287 00288 /* Return AUDIO_OK if all operations are OK */ 00289 return AUDIO_OK; 00290 } 00291 } 00292 00293 /** 00294 * @brief This function Resumes the audio file stream. 00295 * @note When calling BSP_AUDIO_OUT_Pause() function for pause, only 00296 * BSP_AUDIO_OUT_Resume() function should be called for resume (use of BSP_AUDIO_OUT_Play() 00297 * function for resume could lead to unexpected behavior). 00298 * @retval AUDIO_OK if correct communication, else wrong communication 00299 */ 00300 uint8_t BSP_AUDIO_OUT_Resume(void) 00301 { 00302 /* Call the Audio Codec Pause/Resume function */ 00303 if(pAudioDrv->Resume(AUDIO_I2C_ADDRESS) != 0) 00304 { 00305 return AUDIO_ERROR; 00306 } 00307 else 00308 { 00309 /* Call the Media layer resume function */ 00310 HAL_I2S_DMAResume(&hAudioOutI2s); 00311 /* Return AUDIO_OK if all operations are OK */ 00312 return AUDIO_OK; 00313 } 00314 } 00315 00316 /** 00317 * @brief Stops audio playing and Power down the Audio Codec. 00318 * @param Option: could be one of the following parameters 00319 * - CODEC_PDWN_HW: completely shut down the codec (physically). 00320 * Then need to reconfigure the Codec after power on. 00321 * @retval AUDIO_OK if correct communication, else wrong communication 00322 */ 00323 uint8_t BSP_AUDIO_OUT_Stop(uint32_t Option) 00324 { 00325 /* Call DMA Stop to disable DMA stream before stopping codec */ 00326 HAL_I2S_DMAStop(&hAudioOutI2s); 00327 00328 /* Call Audio Codec Stop function */ 00329 if(pAudioDrv->Stop(AUDIO_I2C_ADDRESS, Option) != 0) 00330 { 00331 return AUDIO_ERROR; 00332 } 00333 else 00334 { 00335 if(Option == CODEC_PDWN_HW) 00336 { 00337 /* Wait at least 100us */ 00338 HAL_Delay(1); 00339 00340 /* Power Down the codec */ 00341 BSP_IO_WritePin(AUDIO_RESET_PIN, GPIO_PIN_RESET); 00342 00343 } 00344 /* Return AUDIO_OK when all operations are correctly done */ 00345 return AUDIO_OK; 00346 } 00347 } 00348 00349 /** 00350 * @brief Controls the current audio volume level. 00351 * @param Volume: Volume level to be set in percentage from 0% to 100% (0 for 00352 * Mute and 100 for Max volume level). 00353 * @retval AUDIO_OK if correct communication, else wrong communication 00354 */ 00355 uint8_t BSP_AUDIO_OUT_SetVolume(uint8_t Volume) 00356 { 00357 /* Call the codec volume control function with converted volume value */ 00358 if(pAudioDrv->SetVolume(AUDIO_I2C_ADDRESS, Volume) != 0) 00359 { 00360 return AUDIO_ERROR; 00361 } 00362 else 00363 { 00364 /* Return AUDIO_OK when all operations are correctly done */ 00365 return AUDIO_OK; 00366 } 00367 } 00368 00369 /** 00370 * @brief Enables or disables the MUTE mode by software 00371 * @param Cmd: could be AUDIO_MUTE_ON to mute sound or AUDIO_MUTE_OFF to 00372 * unmute the codec and restore previous volume level. 00373 * @retval AUDIO_OK if correct communication, else wrong communication 00374 */ 00375 uint8_t BSP_AUDIO_OUT_SetMute(uint32_t Cmd) 00376 { 00377 /* Call the Codec Mute function */ 00378 if(pAudioDrv->SetMute(AUDIO_I2C_ADDRESS, Cmd) != 0) 00379 { 00380 return AUDIO_ERROR; 00381 } 00382 else 00383 { 00384 /* Return AUDIO_OK when all operations are correctly done */ 00385 return AUDIO_OK; 00386 } 00387 } 00388 00389 /** 00390 * @brief Switch dynamically (while audio file is played) the output target 00391 * (speaker or headphone). 00392 * @note This function modifies a global variable of the audio codec driver: OutputDev. 00393 * @param Output: specifies the audio output target: OUTPUT_DEVICE_SPEAKER, 00394 * OUTPUT_DEVICE_HEADPHONE, OUTPUT_DEVICE_BOTH or OUTPUT_DEVICE_AUTO 00395 * @retval AUDIO_OK if correct communication, else wrong communication 00396 */ 00397 uint8_t BSP_AUDIO_OUT_SetOutputMode(uint8_t Output) 00398 { 00399 /* Call the Codec output Device function */ 00400 if(pAudioDrv->SetOutputMode(AUDIO_I2C_ADDRESS, Output) != 0) 00401 { 00402 return AUDIO_ERROR; 00403 } 00404 else 00405 { 00406 /* Return AUDIO_OK when all operations are correctly done */ 00407 return AUDIO_OK; 00408 } 00409 } 00410 00411 /** 00412 * @brief Update the audio frequency. 00413 * @param AudioFreq: Audio frequency used to play the audio stream. 00414 * @retval None 00415 * @note This API should be called after the BSP_AUDIO_OUT_Init() to adjust the 00416 * audio frequency. 00417 */ 00418 void BSP_AUDIO_OUT_SetFrequency(uint32_t AudioFreq) 00419 { 00420 /* Update the I2S audio frequency configuration */ 00421 I2SOUT_Init(AudioFreq); 00422 } 00423 00424 /** 00425 * @brief Tx Transfer completed callbacks 00426 * @param hi2s: I2S handle 00427 * @retval None 00428 */ 00429 void HAL_I2S_TxCpltCallback(I2S_HandleTypeDef *hi2s) 00430 { 00431 if(hi2s->Instance == I2SOUT) 00432 { 00433 /* Call the user function which will manage directly transfer complete*/ 00434 BSP_AUDIO_OUT_TransferComplete_CallBack(); 00435 } 00436 } 00437 00438 /** 00439 * @brief Tx Transfer Half completed callbacks 00440 * @param hi2s: I2S handle 00441 * @retval None 00442 */ 00443 void HAL_I2S_TxHalfCpltCallback(I2S_HandleTypeDef *hi2s) 00444 { 00445 if(hi2s->Instance == I2SOUT) 00446 { 00447 /* Manage the remaining file size and new address offset: This function 00448 should be coded by user (its prototype is already declared in stm3210c_eval_audio.h) */ 00449 BSP_AUDIO_OUT_HalfTransfer_CallBack(); 00450 } 00451 } 00452 00453 /** 00454 * @brief I2S error callbacks 00455 * @param hi2s: I2S handle 00456 * @retval None 00457 */ 00458 void HAL_I2S_ErrorCallback(I2S_HandleTypeDef *hi2s) 00459 { 00460 /* Manage the error generated on DMA FIFO: This function 00461 should be coded by user (its prototype is already declared in stm3210c_eval_audio.h) */ 00462 if(hi2s->Instance == I2SOUT) 00463 { 00464 BSP_AUDIO_OUT_Error_CallBack(); 00465 } 00466 } 00467 00468 /** 00469 * @brief Manages the DMA full Transfer complete event. 00470 * @retval None 00471 */ 00472 __weak void BSP_AUDIO_OUT_TransferComplete_CallBack(void) 00473 { 00474 } 00475 00476 /** 00477 * @brief Manages the DMA Half Transfer complete event. 00478 * @retval None 00479 */ 00480 __weak void BSP_AUDIO_OUT_HalfTransfer_CallBack(void) 00481 { 00482 } 00483 00484 /** 00485 * @brief Manages the DMA FIFO error event. 00486 * @retval None 00487 */ 00488 __weak void BSP_AUDIO_OUT_Error_CallBack(void) 00489 { 00490 } 00491 00492 /****************************************************************************** 00493 Static Function 00494 *******************************************************************************/ 00495 00496 /** 00497 * @brief AUDIO OUT I2S MSP Init 00498 * @retval None 00499 */ 00500 static void I2SOUT_MspInit(void) 00501 { 00502 static DMA_HandleTypeDef hdma_i2stx; 00503 GPIO_InitTypeDef gpioinitstruct = {0}; 00504 I2S_HandleTypeDef *hi2s = &hAudioOutI2s; 00505 00506 /* Enable I2SOUT clock */ 00507 I2SOUT_CLK_ENABLE(); 00508 00509 /*** Configure the GPIOs ***/ 00510 /* Enable I2S GPIO clocks */ 00511 I2SOUT_SCK_SD_CLK_ENABLE(); 00512 I2SOUT_WS_CLK_ENABLE(); 00513 00514 /* I2SOUT pins configuration: WS, SCK and SD pins -----------------------------*/ 00515 gpioinitstruct.Pin = I2SOUT_SCK_PIN | I2SOUT_SD_PIN; 00516 gpioinitstruct.Mode = GPIO_MODE_AF_PP; 00517 gpioinitstruct.Pull = GPIO_NOPULL; 00518 gpioinitstruct.Speed = GPIO_SPEED_MEDIUM; 00519 HAL_GPIO_Init(I2SOUT_SCK_SD_GPIO_PORT, &gpioinitstruct); 00520 00521 gpioinitstruct.Pin = I2SOUT_WS_PIN ; 00522 HAL_GPIO_Init(I2SOUT_WS_GPIO_PORT, &gpioinitstruct); 00523 00524 /* I2SOUT pins configuration: MCK pin */ 00525 I2SOUT_MCK_CLK_ENABLE(); 00526 gpioinitstruct.Pin = I2SOUT_MCK_PIN; 00527 HAL_GPIO_Init(I2SOUT_MCK_GPIO_PORT, &gpioinitstruct); 00528 00529 /* Enable the I2S DMA clock */ 00530 I2SOUT_DMAx_CLK_ENABLE(); 00531 00532 if(hi2s->Instance == I2SOUT) 00533 { 00534 /* Configure the hdma_i2stx handle parameters */ 00535 hdma_i2stx.Init.Direction = DMA_MEMORY_TO_PERIPH; 00536 hdma_i2stx.Init.PeriphInc = DMA_PINC_DISABLE; 00537 hdma_i2stx.Init.MemInc = DMA_MINC_ENABLE; 00538 hdma_i2stx.Init.PeriphDataAlignment = I2SOUT_DMAx_PERIPH_DATA_SIZE; 00539 hdma_i2stx.Init.MemDataAlignment = I2SOUT_DMAx_MEM_DATA_SIZE; 00540 hdma_i2stx.Init.Mode = DMA_NORMAL; 00541 hdma_i2stx.Init.Priority = DMA_PRIORITY_HIGH; 00542 00543 hdma_i2stx.Instance = I2SOUT_DMAx_CHANNEL; 00544 00545 /* Associate the DMA handle */ 00546 __HAL_LINKDMA(hi2s, hdmatx, hdma_i2stx); 00547 00548 /* Deinitialize the Channel for new transfer */ 00549 HAL_DMA_DeInit(&hdma_i2stx); 00550 00551 /* Configure the DMA Channel */ 00552 HAL_DMA_Init(&hdma_i2stx); 00553 } 00554 00555 /* I2S DMA IRQ Channel configuration */ 00556 HAL_NVIC_SetPriority(I2SOUT_DMAx_IRQ, AUDIO_OUT_IRQ_PREPRIO, 0); 00557 HAL_NVIC_EnableIRQ(I2SOUT_DMAx_IRQ); 00558 } 00559 00560 /** 00561 * @brief Initializes the Audio Codec audio interface (I2S) 00562 * @param AudioFreq: Audio frequency to be configured for the I2S peripheral. 00563 */ 00564 static void I2SOUT_Init(uint32_t AudioFreq) 00565 { 00566 /* Initialize the hAudioOutI2s Instance parameter */ 00567 hAudioOutI2s.Instance = I2SOUT; 00568 00569 /* Disable I2S block */ 00570 __HAL_I2S_DISABLE(&hAudioOutI2s); 00571 00572 /* Perform MSP initialization at first function call */ 00573 if(HAL_I2S_GetState(&hAudioOutI2s) == HAL_I2S_STATE_RESET) 00574 { 00575 I2SOUT_MspInit(); 00576 } 00577 00578 /* I2SOUT peripheral configuration */ 00579 hAudioOutI2s.Init.AudioFreq = AudioFreq; 00580 hAudioOutI2s.Init.CPOL = I2S_CPOL_LOW; 00581 hAudioOutI2s.Init.DataFormat = I2S_DATAFORMAT_16B; 00582 hAudioOutI2s.Init.MCLKOutput = I2S_MCLKOUTPUT_ENABLE; 00583 hAudioOutI2s.Init.Mode = I2S_MODE_MASTER_TX; 00584 hAudioOutI2s.Init.Standard = I2S_STANDARD; 00585 00586 /* Initialize the I2S peripheral with the structure above */ 00587 HAL_I2S_Init(&hAudioOutI2s); 00588 } 00589 00590 /** 00591 * @} 00592 */ 00593 00594 /** 00595 * @} 00596 */ 00597 00598 /** 00599 * @} 00600 */ 00601 00602 /** 00603 * @} 00604 */ 00605 00606 /** 00607 * @} 00608 */ 00609 00610 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Thu Dec 11 2014 15:38:28 for _BSP_User_Manual by
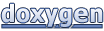