![]() |
RainbowBS Manual
v0.1.0
Written by QWQ([email protected])
|
Table of Contents
Dynamic memory management is an optional component for memory usage. It offers both memory block and memory pool mechanism.
DMM Features
- memory block offers fixed size memory management.
- memory pool offers variable size memory management.
- both mechanisms support register,byte-alignment,usage check and thread-safety.
Memory Block
register memory block
Depending on the
RBS_CFG_APP_MODEL
configuration whether or not using OS,one of two register interfaces should be used before using memory block.If
RBS_CFG_APP_MODEL
is configurated toRBS_APP_OS
,thread-safety version should be used.hDMM RBS_DMM_RegisterBlock(char *pName,void *pDM,USIZE Size,USIZE BlockSize,HMUTEX hMutex)
If
RBS_CFG_APP_MODEL
is configurated to others.hDMM RBS_DMM_RegisterBlock(char *pName,void *pDM,USIZE Size,USIZE BlockSize)
allocate and free block
hBLOCK RBS_DMM_AllocZeroBlock(hDMM hDmm)
block usage
Memory Pool
register memory pool
Depending on the
RBS_CFG_APP_MODEL
configuration whether or not using OS,one of two register interfaces should be used befor using memory pool.If
RBS_CFG_APP_MODEL
is configurated toRBS_APP_OS
,thread-safety version should be used.hDMM RBS_DMM_RegisterPool(char *pName,void *pDM,USIZE Size,BOOL bAntiFrag,U16 HCount,HMUTEX hMutex)
If
RBS_CFG_APP_MODEL
is configurated to others.hDMM RBS_DMM_RegisterPool(char *pName,void *pDM,USIZE Size,BOOL bAntiFrag,U16 HCount)
allocate and free pool
hMEM RBS_DMM_AllocZeroMem(hDMM hDmm,USIZE size)
hMEM RBS_DMM_AllocMem(hDMM hDmm,USIZE size)
pool usage
void* RBS_DMM_UseHMem(hMEM hMem)
Usage
In an embedded system,there are some memory types with different size such as internal SRAM integrated in micro-controller,external SDRAM,SRAM,etc... and those memory can be byte-addressed in the same address space.Some of them are used for stacks,while some used for global data and some used for dynamic memory allocation.So the specific usage of those memory are decided by the application.
Unlike traditional memory management like malloc
in C library,DMM can manage more than one continuous byte-addressed memory for dynamic memory allocation and can be sensitive to memory usage.
As an example,a micro-controller has 8K-bytes size internal RAM in and external 2M-bytes SDRAM.You want to use 2K-bytes internal RAM and 1M-bytes external SDRAM space for dynamic memory allocation.First of all,you just define two byte array,one for the 2K internal RAM and the other for the 1M-bytes external SDRAM,and the use the register interface to define the two memory space.After register,you can allocate memory from them by the DMM handle returned from register.
Allocated memory will return a memory handle and then you can use the memory handle to use,not use or free the allocated memory.
Using RBS_DMM_UseHBlock()
or RBS_DMM_UseHMem()
to refer to the allocated memory space.
Using RBS_DMM_UnuseHBlock()
or BS_DMM_UnuseHMem()
to notify the DMM to end the last memory referance.
Using RBS_DMM_FreeBlock()
or RBS_DMM_FreeMem()
to notify the DMM to release the memory.
RBS_DMM_UseHBloc()
and BRS_DMM_UnuseHBlock()
are used in pair,while RBS_DMM_UseHMem()
and RBS_DMM_UnuseHMem() are in pair.The reason is that each memory handle has a referance counter in internal,and the counter is increased by 1 per usage and decrease by 1 per no usage.So if the counter is not initial value,it will not be released successfully by RBS_DMM_FreeBlock()
or RBS_DMM_FreeMem()
.This is called usage check.Developers should keep in mind that after each reference to the allocated memory by RBS_DMM_UseHBlock()
or RBS_DMM_UseHMem()
,an RBS_DMM_UnuseHBlock()
or RBS_DMM_UnuseHMem()
should be called and if he wants to refer to the memory again,then RBS_DMM_UseHBlock()
or BS_DMM_UseHMem()
should be called again.With usage check,developers will find memory usage conflicts and bugs earlier.For example,there are two running tasks A and B that both using an allocated memory.If task A is using the memory without calling RBS_DMM_UnuseHBlock()
or RBS_DMM_UnuseHMem()
and task B call RBS_DMM_FreeBlock()
or RBS_DMM_FreeMem()
,then an error information will be printed by debug component,telling that it can not release memory handle because it is using.
Remember that usage check is only effective if RBS debug component is enabled,otherwise usage check is disabled.
Following is the example code for DMM usage.
Configuration
RBS_CFG_DMM_ALIGN
is defined for define byte-alignment.3 means 8 bytes,2 means 4 bytes,1 means 2 bytes,0 means 1 bytes.
Allocated memory started address and size are both alignment.So if RBS_CFG_DMM_ALIGN
is 2 and you allocated 25 bytes,then you get 28 bytes actually.
Generated by
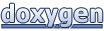