PWM
|
Usage
The PWM APP is typically used just for a simple Pulse Width Modulation output generation.
This example demonstrates the brightness control of a LED (Light Emitting Diode) using PWM duty cycle.
Instantiate the required APPs
Drag an instance of PWM APP and INTERRUPT APP. Update the fields in the GUI of these APPs with the following configuration.
Configure the APPs
PWM APP:
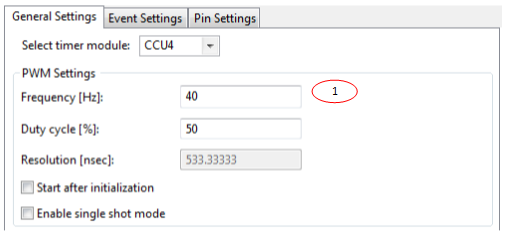
- Set frequency as 40Hz.
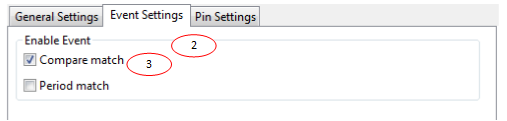
- Goto the Events Tab.
- Enable compare match event.
INTERRUPT APP:
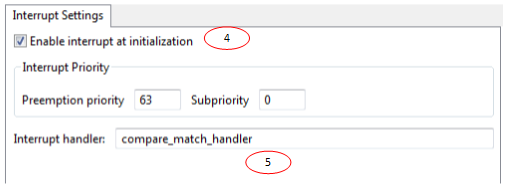
- Check the Enable interrupt at initialization.
- Provide the interrupt handler as "compare_match_handler".
Signal Connection
Establish a HW signal connection between the PWM and the INTERRUPT APP to ensure PWM events generates interrupts.
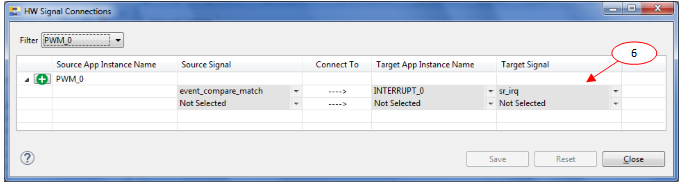
- Connect PWM_0/event_compare_match -> INTERRUPT_0/sr_irq to ensure assigning ISR node to the compare match event.
Manual pin allocation
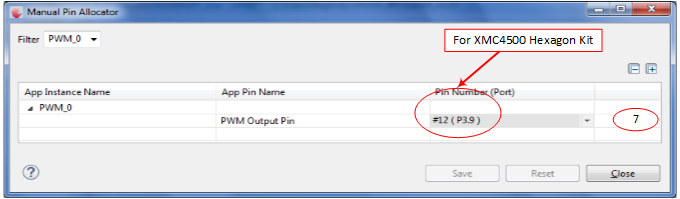
-
Select the LED Pin present in the boot kit
Note:The pin number is specific to the development board chosen to run this example. The pin shown in the image above may not be available on every XMC kit. Ensure that a proper pin is selected according to the board.
Generate code
Files are generated here: '<project_name>/Dave/Generated/' ('project_name' is the name chosen by the user during project creation). APP instance definitions and APIs are generated only after code generation.
-
Note: Code must be explicitly generated for every change in the GUI configuration.
Important: Any manual modification to APP specific files will be overwritten by a subsequent code generation operation.
Sample Application (main.c)
#include <DAVE.h> // Add the following function in main.c void compare_match_handler(void) { static uint32_t duty = (uint32_t)100; static bool decrement_duty = (bool)false; if(decrement_duty != false) { //Decrement the duty cycle until it reaches 1% duty -= (uint32_t)100; // Once the duty has reached 1% flag status is changed to Increment if (duty <= (uint32_t)100) { decrement_duty = false; } } else { // Increment the duty cycle until it reaches 100% duty += (uint32_t)100; // Once the duty has reached 100% flag status is changed to decrement if (duty >= (uint32_t)10000) { decrement_duty = true; } } // Sets the duty cycle of the PWM PWM_SetDutyCycle(&PWM_0,duty); // Clear the compare match interrupt. PWM_ClearEvent(&PWM_0,PWM_INTERRUPT_COMPAREMATCH ); } int main(void) { DAVE_STATUS_t status; status = DAVE_Init(); /* Initialization of DAVE Apps */ if(status == DAVE_STATUS_FAILURE) { /* Placeholder for error handler code. The while loop below can be replaced with an user error handler */ XMC_DEBUG(("DAVE Apps initialization failed with status %d\n", status)); while(1U) { } } PWM_Start(&PWM_0); while(1U); return 1; }
Build and Run the Project
Observation
The LED brightness will gradually decrease and then increase. The brightness variation cycle will occur repeatedly.