source/dvr_enc_ioctl.h
Go to the documentation of this file.
00001 /*! \mainpage GM8126 dvr encode, decode and display ioctl functions 00002 * 00003 * dvr_enc_ioctl.h describe dvr encode ioctl functions behavior 00004 * \n dvr_dec_ioctl.h describe dvr decode ioctl functions behavior 00005 * \n dvr_disp_ioctl.h describe dvr display ioctl functions behavior 00006 * \n dvr_common_api.h describe dvr common ioctl functions behavior 00007 */ 00008 00009 /** 00010 * \example main-bitstream-record.c 00011 * \example sub-bitstream-record.c 00012 * \example update-record-setting.c 00013 * \example mpeg4-record.c 00014 * \example mjpeg-record.c 00015 * \example snapshot.c 00016 * \example motion-detection.c 00017 * \example update-bitrate.c 00018 * \example playback.c 00019 * \example roi.c 00020 * \example capture_raw.c 00021 * \example liveview.c 00022 * \example 2ch_liveview.c 00023 * \example 2ch_playback.c 00024 * \example motion-detection-mpeg4.c 00025 * \example pip.c 00026 */ 00027 00028 #define DVR_ENC_IOC_MAGIC 'B' 00029 00030 /** 00031 * \b ioctl(enc_fd, DVR_ENC_SET_CHANNEL_PARAM, &ch_param) 00032 * 00033 * \arg explanation : get channel parameter from user space ,and set parameter to device driver 00034 * \arg parameter : 00035 * \n \b \e pointer \b \e ch_param : argument from user space ioctl parameter, it means structure dvr_enc_channel_param 00036 * 00037 */ 00038 #define DVR_ENC_SET_CHANNEL_PARAM _IOWR(DVR_ENC_IOC_MAGIC, 2, dvr_enc_channel_param) 00039 00040 /** 00041 * \b ioctl(enc_fd, DVR_ENC_GET_CHANNEL_PARAM, &ch_param) : 00042 * 00043 * \arg explanation : get channel parameter from user space 00044 * \arg parameter : 00045 * \n \b \e pointer \b \e ch_param : argument from user space ioctl parameter, it means structure dvr_enc_channel_param 00046 */ 00047 #define DVR_ENC_GET_CHANNEL_PARAM _IOWR(DVR_ENC_IOC_MAGIC, 3, dvr_enc_channel_param) 00048 00049 /** 00050 * \b ioctl(enc_fd, DVR_ENC_QUEUE_GET, &data) 00051 * 00052 * \arg explanation : Get buffer to user space. It includes the buffer length, offset. 00053 * \arg parameter : 00054 * \n \b \e pointer \b \e data : argument from user space ioctl parameter, it means structure dvr_enc_queue_get 00055 */ 00056 #define DVR_ENC_QUEUE_GET _IOWR(DVR_ENC_IOC_MAGIC, 5, dvr_enc_queue_get) 00057 00058 /** 00059 * \b ioctl(enc_fd, DVR_ENC_QUEUE_PUT, &data) 00060 * 00061 * \arg explanation : get buffer from user space, and release buffer at videograph layer 00062 * \arg parameter : 00063 * \n \b \e pointer \b \e data : argument from user space ioctl parameter, it means structure dvr_enc_queue_get 00064 */ 00065 #define DVR_ENC_QUEUE_PUT _IOWR(DVR_ENC_IOC_MAGIC, 6, dvr_enc_queue_get) 00066 00067 /** 00068 * \b ioctl(enc_fd, DVR_ENC_CONTROL, &enc_ctrl) 00069 * 00070 * \arg explanation : get encode contorl command from user space, and set command to videograph layer 00071 * \arg parameter : 00072 * \n \b \e pointer \b \e enc_ctrl : argument from user space ioctl parameter, it means structure dvr_enc_control 00073 */ 00074 #define DVR_ENC_CONTROL _IOW(DVR_ENC_IOC_MAGIC, 7, dvr_enc_control) 00075 00076 /** 00077 * \b ioctl(enc_fd, DVR_ENC_QUERY_OUTPUT_BUFFER_SIZE, &enc_buf_size) 00078 * 00079 * \arg explanation : get output buffer size for dvr encode to user space. 00080 buffer size = main bitstream + sub1 bitstream + sub2 bitstream + snapshot 00081 * \arg parameter : 00082 * \n \b \e pointer \b \e enc_buf_size : argument from user space ioctl parameter, it means request buffer size. 00083 */ 00084 #define DVR_ENC_QUERY_OUTPUT_BUFFER_SIZE _IOWR(DVR_ENC_IOC_MAGIC, 8, int) 00085 00086 /** 00087 * \b ioctl(enc_fd, DVR_ENC_QUERY_OUTPUT_BUFFER_SIZE, &enc_buf_size); 00088 * 00089 * \arg explanation : get snapshot buffer to user space 00090 * \arg parameter : 00091 * \n \b \e pointer \b \e queue_data : argument from user space ioctl parameter, it means structure dvr_enc_queue_get 00092 */ 00093 #define DVR_ENC_QUEUE_GET_SNAP _IOWR(DVR_ENC_IOC_MAGIC, 9, dvr_enc_queue_get) 00094 00095 /** 00096 * ioctl(enc_fd, DVR_ENC_QUERY_OUTPUT_BUFFER_SNAP_OFFSET, &bs_buf_snap_offset) 00097 * 00098 * \arg explanation : get output buffer offset for snapshot to user space 00099 * \arg parameter : 00100 * \n \b \e pointer \b \e bs_buf_snap_offset : argument from user space ioctl parameter, it means snapshot offset. 00101 * 00102 */ 00103 #define DVR_ENC_QUERY_OUTPUT_BUFFER_SNAP_OFFSET _IOR(DVR_ENC_IOC_MAGIC, 10, int) 00104 00105 /** 00106 * \b ioctl(enc_fd, DVR_ENC_QUEUE_GET_SUB1_BS, &data) : 00107 * 00108 * \arg explanation : get sub1-bitstream buffer to user space. 00109 * \arg parameter : 00110 * \n \b \e pointer \b \e data : argument from user space ioctl parameter, it means structure dvr_enc_queue_get 00111 */ 00112 #define DVR_ENC_QUEUE_GET_SUB1_BS _IOWR(DVR_ENC_IOC_MAGIC, 11, dvr_enc_queue_get) 00113 00114 /** 00115 * \b ioctl(enc_fd, DVR_ENC_QUEUE_GET_SUB2_BS, &data) 00116 * 00117 * \arg explanation : get sub2-bitstream buffer to user space. 00118 * \arg parameter : 00119 * \n \b \e pointer \b \e data : argument from user space ioctl parameter, it means structure dvr_enc_queue_get 00120 */ 00121 #define DVR_ENC_QUEUE_GET_SUB2_BS _IOWR(DVR_ENC_IOC_MAGIC, 12, dvr_enc_queue_get) 00122 00123 /** 00124 * \b ioctl(enc_fd, DVR_ENC_QUERY_OUTPUT_BUFFER_SUB1_BS_OFFSET, &sub1_bs_buf_offset) 00125 * 00126 * \arg explanation : get output buffer offset for Sub1-bitstream to user space 00127 * \arg parameter : 00128 * \n \b \e pointer \b \e sub1_bs_buf_offset : argument from user space ioctl parameter, it means sub1 bitstream buffer offset. 00129 * 00130 */ 00131 #define DVR_ENC_QUERY_OUTPUT_BUFFER_SUB1_BS_OFFSET _IOR(DVR_ENC_IOC_MAGIC, 13, int) 00132 00133 /** 00134 * \b ioctl(enc_fd, DVR_ENC_QUERY_OUTPUT_BUFFER_SUB2_BS_OFFSET, &sub2_bs_buf_offset) 00135 * 00136 * \arg explanation : get output buffer offset for Sub2-bitstream to user space 00137 * \arg parameter : 00138 * \n \b \e pointer \b \e sub2_bs_buf_offset : argument from user space ioctl parameter, it means sub2 bitstream buffer offset. 00139 * 00140 */ 00141 #define DVR_ENC_QUERY_OUTPUT_BUFFER_SUB2_BS_OFFSET _IOR(DVR_ENC_IOC_MAGIC, 14, int) 00142 00143 /** 00144 * \b ioctl(enc_fd, DVR_ENC_RESET_INTRA, &stream_num) 00145 * 00146 * \arg explanation : get i-frame as possible 00147 * \arg parameter : 00148 * \n \b \e pointer \b \e stream_num : argument from user space ioctl parameter, it means structure main-bitstream or sub1-bitstream or sub2-bitstream 00149 */ 00150 #define DVR_ENC_RESET_INTRA _IOR(DVR_ENC_IOC_MAGIC, 15, int) 00151 00152 /** 00153 * \b ioctl(enc_fd, DVR_ENC_SET_SUB_BS_PARAM, &sub_bitstream) 00154 * 00155 * \arg explanation : get sub-bitstream parameter from user space, and set sub-bitstream parameter to device driver 00156 * \arg parameter : 00157 * \n \b \e pointer \b \e sub_bitstream : argument from user space ioctl parameter, it means structure ReproduceBitStream 00158 */ 00159 #define DVR_ENC_SET_SUB_BS_PARAM _IOW(DVR_ENC_IOC_MAGIC, 16, int) 00160 00161 /** 00162 * \b ioctl(enc_fd, DVR_ENC_SWAP_INTRA, &stream_num) 00163 * 00164 * \arg explanation : get i-frame definitely when next switch 00165 * \arg parameter : 00166 * \n \b \e pointer \b \e stream_num : argument from user space ioctl parameter, it means structure main-bitstream or sub1-bitstream or sub2-bitstream 00167 */ 00168 #define DVR_ENC_SWAP_INTRA _IOR(DVR_ENC_IOC_MAGIC, 18, int) 00169 00170 /** 00171 * \b ioctl(enc_fd, DVR_ENC_SUB_PATH_DENOISE_CTRL, &control) 00172 * 00173 * \arg explanation : get denoise option from user space, and set to device driver 00174 * \arg parameter : 00175 * \n \b \e pointer \b \e control : argument from user space ioctl parameter, it means denoise on/off 00176 */ 00177 #define DVR_ENC_SUB_PATH_DENOISE_CTRL _IOW(DVR_ENC_IOC_MAGIC, 19, int) 00178 00179 /** 00180 * \b ioctl(enc_fd, DVR_ENC_QUEUE_GET_SUB3_BS, &data) 00181 * 00182 * \arg explanation : get sub3-bitstream buffer to user space. 00183 * \arg parameter : 00184 * \n \b \e pointer \b \e data : argument from user space ioctl parameter, it means structure dvr_enc_queue_get 00185 */ 00186 #define DVR_ENC_QUEUE_GET_SUB3_BS _IOWR(DVR_ENC_IOC_MAGIC, 20, dvr_enc_queue_get) 00187 00188 /** 00189 * \b ioctl(enc_fd, DVR_ENC_QUEUE_GET_SUB4_BS, &data) 00190 * 00191 * \arg explanation : get sub4-bitstream buffer to user space. 00192 * \arg parameter : 00193 * \n \b \e pointer \b \e data : argument from user space ioctl parameter, it means structure dvr_enc_queue_get 00194 */ 00195 #define DVR_ENC_QUEUE_GET_SUB4_BS _IOWR(DVR_ENC_IOC_MAGIC, 21, dvr_enc_queue_get) 00196 00197 /** 00198 * \b ioctl(enc_fd, DVR_ENC_QUEUE_GET_SUB5_BS, &data) 00199 * 00200 * \arg explanation : get sub5-bitstream buffer to user space. 00201 * \arg parameter : 00202 * \n \b \e pointer \b \e data : argument from user space ioctl parameter, it means structure dvr_enc_queue_get 00203 */ 00204 #define DVR_ENC_QUEUE_GET_SUB5_BS _IOWR(DVR_ENC_IOC_MAGIC, 22, dvr_enc_queue_get) 00205 00206 /** 00207 * \b ioctl(enc_fd, DVR_ENC_QUEUE_GET_SUB6_BS, &data) 00208 * 00209 * \arg explanation : get sub6-bitstream buffer to user space. 00210 * \arg parameter : 00211 * \n \b \e pointer \b \e data : argument from user space ioctl parameter, it means structure dvr_enc_queue_get 00212 */ 00213 #define DVR_ENC_QUEUE_GET_SUB6_BS _IOWR(DVR_ENC_IOC_MAGIC, 23, dvr_enc_queue_get) 00214 00215 /** 00216 * \b ioctl(enc_fd, DVR_ENC_QUEUE_GET_SUB7_BS, &data) 00217 * 00218 * \arg explanation : get sub7-bitstream buffer to user space. 00219 * \arg parameter : 00220 * \n \b \e pointer \b \e data : argument from user space ioctl parameter, it means structure dvr_enc_queue_get 00221 */ 00222 #define DVR_ENC_QUEUE_GET_SUB7_BS _IOWR(DVR_ENC_IOC_MAGIC, 24, dvr_enc_queue_get) 00223 00224 /** 00225 * \b ioctl(enc_fd, DVR_ENC_QUEUE_GET_SUB8_BS, &data) 00226 * 00227 * \arg explanation : get sub8-bitstream buffer to user space. 00228 * \arg parameter : 00229 * \n \b \e pointer \b \e data : argument from user space ioctl parameter, it means structure dvr_enc_queue_get 00230 */ 00231 #define DVR_ENC_QUEUE_GET_SUB8_BS _IOWR(DVR_ENC_IOC_MAGIC, 25, dvr_enc_queue_get) 00232 00233 /** 00234 * \b ioctl(enc_fd, DVR_ENC_QUERY_OUTPUT_BUFFER_SUB3_BS_OFFSET, &sub3_bs_buf_offset) 00235 * 00236 * \arg explanation : get output buffer offset for Sub3-bitstream to user space 00237 * \arg parameter : 00238 * \n \b \e pointer \b \e sub3_bs_buf_offset : argument from user space ioctl parameter, it means sub3 bitstream buffer offset. 00239 * 00240 */ 00241 #define DVR_ENC_QUERY_OUTPUT_BUFFER_SUB3_BS_OFFSET _IOR(DVR_ENC_IOC_MAGIC, 26, int) 00242 00243 /** 00244 * \b ioctl(enc_fd, DVR_ENC_QUERY_OUTPUT_BUFFER_SUB4_BS_OFFSET, &sub4_bs_buf_offset) 00245 * 00246 * \arg explanation : get output buffer offset for Sub4-bitstream to user space 00247 * \arg parameter : 00248 * \n \b \e pointer \b \e sub4_bs_buf_offset : argument from user space ioctl parameter, it means sub4 bitstream buffer offset. 00249 * 00250 */ 00251 #define DVR_ENC_QUERY_OUTPUT_BUFFER_SUB4_BS_OFFSET _IOR(DVR_ENC_IOC_MAGIC, 27, int) 00252 00253 /** 00254 * \b ioctl(enc_fd, DVR_ENC_QUERY_OUTPUT_BUFFER_SUB5_BS_OFFSET, &sub5_bs_buf_offset) 00255 * 00256 * \arg explanation : get output buffer offset for Sub5-bitstream to user space 00257 * \arg parameter : 00258 * \n \b \e pointer \b \e sub5_bs_buf_offset : argument from user space ioctl parameter, it means sub5 bitstream buffer offset. 00259 * 00260 */ 00261 #define DVR_ENC_QUERY_OUTPUT_BUFFER_SUB5_BS_OFFSET _IOR(DVR_ENC_IOC_MAGIC, 28, int) 00262 00263 /** 00264 * \b ioctl(enc_fd, DVR_ENC_QUERY_OUTPUT_BUFFER_SUB6_BS_OFFSET, &sub6_bs_buf_offset) 00265 * 00266 * \arg explanation : get output buffer offset for Sub6-bitstream to user space 00267 * \arg parameter : 00268 * \n \b \e pointer \b \e sub6_bs_buf_offset : argument from user space ioctl parameter, it means sub6 bitstream buffer offset. 00269 * 00270 */ 00271 #define DVR_ENC_QUERY_OUTPUT_BUFFER_SUB6_BS_OFFSET _IOR(DVR_ENC_IOC_MAGIC, 29, int) 00272 00273 /** 00274 * \b ioctl(enc_fd, DVR_ENC_QUERY_OUTPUT_BUFFER_SUB7_BS_OFFSET, &sub7_bs_buf_offset) 00275 * 00276 * \arg explanation : get output buffer offset for Sub7-bitstream to user space 00277 * \arg parameter : 00278 * \n \b \e pointer \b \e sub7_bs_buf_offset : argument from user space ioctl parameter, it means sub7 bitstream buffer offset. 00279 * 00280 */ 00281 #define DVR_ENC_QUERY_OUTPUT_BUFFER_SUB7_BS_OFFSET _IOR(DVR_ENC_IOC_MAGIC, 30, int) 00282 00283 /** 00284 * \b ioctl(enc_fd, DVR_ENC_QUERY_OUTPUT_BUFFER_SUB8_BS_OFFSET, &sub8_bs_buf_offset) 00285 * 00286 * \arg explanation : get output buffer offset for Sub8-bitstream to user space 00287 * \arg parameter : 00288 * \n \b \e pointer \b \e sub8_bs_buf_offset : argument from user space ioctl parameter, it means sub8 bitstream buffer offset. 00289 * 00290 */ 00291 #define DVR_ENC_QUERY_OUTPUT_BUFFER_SUB8_BS_OFFSET _IOR(DVR_ENC_IOC_MAGIC, 31, int) 00292 00293 /** 00294 * \b ioctl(enc_fd, DVR_ENC_QUEUE_GET_COPY, &data) 00295 * 00296 * \arg explanation : Get buffer and copy to user space. It includes the bitstream length. 00297 * \arg parameter : 00298 * \n \b \e pointer \b \e data : argument from user space ioctl parameter, it means structure dvr_enc_copy_buf 00299 */ 00300 #define DVR_ENC_QUEUE_GET_COPY _IOWR(DVR_ENC_IOC_MAGIC, 35, dvr_enc_copy_buf) 00301 00302 /** 00303 * \b ioctl(enc_fd, DVR_ENC_QUEUE_GET_SUB1_BS_COPY, &data) 00304 * 00305 * \arg explanation : get and copy sub8-bitstream to user space buffer. 00306 * \arg parameter : 00307 * \n \b \e pointer \b \e data : argument from user space ioctl parameter, it means structure dvr_enc_copy_buf 00308 */ 00309 #define DVR_ENC_QUEUE_GET_SUB1_BS_COPY _IOWR(DVR_ENC_IOC_MAGIC, 36, dvr_enc_copy_buf) 00310 00311 /** 00312 * \b ioctl(enc_fd, DVR_ENC_QUEUE_GET_SUB2_BS_COPY, &data) 00313 * 00314 * \arg explanation : get and copy sub8-bitstream to user space buffer. 00315 * \arg parameter : 00316 * \n \b \e pointer \b \e data : argument from user space ioctl parameter, it means structure dvr_enc_copy_buf 00317 */ 00318 #define DVR_ENC_QUEUE_GET_SUB2_BS_COPY _IOWR(DVR_ENC_IOC_MAGIC, 37, dvr_enc_copy_buf) 00319 00320 /** 00321 * \b ioctl(enc_fd, DVR_ENC_QUEUE_GET_SUB3_BS_COPY, &data) 00322 * 00323 * \arg explanation : get and copy sub8-bitstream to user space buffer. 00324 * \arg parameter : 00325 * \n \b \e pointer \b \e data : argument from user space ioctl parameter, it means structure dvr_enc_copy_buf 00326 */ 00327 #define DVR_ENC_QUEUE_GET_SUB3_BS_COPY _IOWR(DVR_ENC_IOC_MAGIC, 38, dvr_enc_copy_buf) 00328 00329 /** 00330 * \b ioctl(enc_fd, DVR_ENC_QUEUE_GET_SUB4_BS_COPY, &data) 00331 * 00332 * \arg explanation : get and copy sub8-bitstream to user space buffer. 00333 * \arg parameter : 00334 * \n \b \e pointer \b \e data : argument from user space ioctl parameter, it means structure dvr_enc_copy_buf 00335 */ 00336 #define DVR_ENC_QUEUE_GET_SUB4_BS_COPY _IOWR(DVR_ENC_IOC_MAGIC, 39, dvr_enc_copy_buf) 00337 00338 /** 00339 * \b ioctl(enc_fd, DVR_ENC_QUEUE_GET_SUB5_BS_COPY, &data) 00340 * 00341 * \arg explanation : get and copy sub8-bitstream to user space buffer. 00342 * \arg parameter : 00343 * \n \b \e pointer \b \e data : argument from user space ioctl parameter, it means structure dvr_enc_copy_buf 00344 */ 00345 #define DVR_ENC_QUEUE_GET_SUB5_BS_COPY _IOWR(DVR_ENC_IOC_MAGIC, 40, dvr_enc_copy_buf) 00346 00347 /** 00348 * \b ioctl(enc_fd, DVR_ENC_QUEUE_GET_SUB6_BS_COPY, &data) 00349 * 00350 * \arg explanation : get and copy sub8-bitstream to user space buffer. 00351 * \arg parameter : 00352 * \n \b \e pointer \b \e data : argument from user space ioctl parameter, it means structure dvr_enc_copy_buf 00353 */ 00354 #define DVR_ENC_QUEUE_GET_SUB6_BS_COPY _IOWR(DVR_ENC_IOC_MAGIC, 41, dvr_enc_copy_buf) 00355 00356 /** 00357 * \b ioctl(enc_fd, DVR_ENC_QUEUE_GET_SUB7_BS_COPY, &data) 00358 * 00359 * \arg explanation : get and copy sub8-bitstream to user space buffer. 00360 * \arg parameter : 00361 * \n \b \e pointer \b \e data : argument from user space ioctl parameter, it means structure dvr_enc_copy_buf 00362 */ 00363 #define DVR_ENC_QUEUE_GET_SUB7_BS_COPY _IOWR(DVR_ENC_IOC_MAGIC, 42, dvr_enc_copy_buf) 00364 00365 00366 /** 00367 * \b ioctl(enc_fd, DVR_ENC_QUEUE_GET_SUB8_BS_COPY, &data) 00368 * 00369 * \arg explanation : get and copy sub8-bitstream to user space buffer. 00370 * \arg parameter : 00371 * \n \b \e pointer \b \e data : argument from user space ioctl parameter, it means structure dvr_enc_copy_buf 00372 */ 00373 #define DVR_ENC_QUEUE_GET_SUB8_BS_COPY _IOWR(DVR_ENC_IOC_MAGIC, 43, dvr_enc_copy_buf) 00374 00375 00376
Generated on Wed Jun 15 2011 15:51:00 for This describe GM8126 ioctl functions by
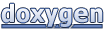