source/dvr_disp_api.h
Go to the documentation of this file.
00001 #ifndef __DVR_DISP_API_H__ 00002 #define __DVR_DISP_API_H__ 00003 00004 #include <linux/ioctl.h> 00005 00006 #include "dvr_type_define.h" 00007 #include "dvr_disp_ioctl.h" 00008 00009 #define DVR_PLANE_ID(d,p) ((d<<8)|p) 00010 #define GET_DISP_NUM_FROM_PLANE_ID(h) (h>>8) 00011 #define GET_PLANE_NUM_FROM_PLANE_ID(h) (0xFF&h) 00012 00013 00014 enum dvr_disp_type { 00015 DISP_TYPE_LIVEVIEW, 00016 DISP_TYPE_CASCADE, 00017 DISP_TYPE_PLAYBACK, 00018 DISP_TYPE_ON_BUFFER, 00019 }; 00020 00021 /* TODO: the following should be replaced by including capture header file.... */ 00022 /* 00023 #define VIDEO_MODE_PAL 0 00024 #define VIDEO_MODE_NTSC 1 00025 #define VIDEO_MODE_SECAM 2 00026 #define VIDEO_MODE_AUTO 3 00027 */ 00028 /**/ 00029 00030 00031 //### Declaration for DVR_DISP_GET_DISP_PARAM and DVR_DISP_SET_DISP_PARAM ### 00032 typedef struct dvr_disp_color_attribute_tag { 00033 int brightness; 00034 int saturation; 00035 int contrast; 00036 int huesin; 00037 int huecos; 00038 int sharpnessk0; 00039 int sharpnessk1; 00040 int sharpness_thres0; 00041 int shaprness_thres1; 00042 int reserved[2]; 00043 }dvr_disp_color_attribute; 00044 00045 typedef struct dvr_disp_color_key_tag { 00046 int is_enable; 00047 int color; 00048 int reserved[2]; 00049 }dvr_disp_color_key; 00050 00051 typedef enum dvr_disp_plane_combination_tag { 00052 BG_ONLY =0, ///< only backgraound 00053 BG_AND_1PLANE =1, ///< background and another plane 00054 BG_AND_2PLANE =2 ///< background and another 2 planes 00055 }dvr_disp_plane_combination; 00056 00057 typedef struct dvr_disp_vbi_info_tag { 00058 int filler; ///< 0:driver do it, 1:ap can set values for it(need to fill the following fields) 00059 int lineno; 00060 int lineheight; 00061 int fb_offset; 00062 int fb_size; 00063 int reserved[2]; 00064 }dvr_disp_vbi_info; 00065 00066 typedef struct dvr_disp_scaler_info_tag { 00067 int is_enable; 00068 /*! #DIM_tag */ 00069 DIM dim; 00070 int reserved[2]; 00071 }dvr_disp_scaler_info; 00072 00073 typedef struct dvr_disp_resolution_tag { 00074 int input_res; 00075 int output_type; 00076 int reserved[2]; 00077 }dvr_disp_resolution; 00078 00079 00080 typedef struct dvr_disp_disp_param_tag { 00081 int disp_num; ///< input(write only) 00082 00083 int target_id; ///< where to output. 1:LCD1, 2:LCD2 00084 /*! #dvr_disp_plane_combination_tag */ 00085 int plane_comb; ///< the number of active plane for this LCD 00086 /*! #MCP_VIDEO_NTSC */ 00087 /*! #MCP_VIDEO_PAL */ 00088 /*! #MCP_VIDEO_VGA */ 00089 int output_system; 00090 /*! #LCDOutputModeTag */ 00091 int output_mode; 00092 /*! #dvr_disp_color_attribute_tag */ 00093 dvr_disp_color_attribute color_attrib; 00094 /*! #dvr_disp_color_key_tag */ 00095 dvr_disp_color_key transparent_color[2]; 00096 /*! #dvr_disp_vbi_info_tag */ 00097 dvr_disp_vbi_info vbi_info; 00098 /*! #dvr_disp_scaler_info_tag */ 00099 dvr_disp_scaler_info scl_info; 00100 /*! #DIM_tag */ 00101 DIM dim; ///< default background dimension 00102 /*! #dvr_disp_resolution_tag */ 00103 dvr_disp_resolution res; 00104 int reserved[8]; 00105 }dvr_disp_disp_param; 00106 00107 00108 00109 //### Declaration for DVR_DISP_UPDATE_DISP_PARAM ### 00110 typedef enum dvr_disp_disp_param_name_tag { 00111 DISP_PARAM_TARGET, 00112 DISP_PARAM_PLANE_COMBINATION, 00113 DISP_PARAM_OUTPUT_MODE, 00114 DISP_PARAM_OUTPUT_SYSTEM, 00115 DISP_PARAM_COLOR_ATTRIBUTE, 00116 DISP_PARAM_TRANSPARENT_COLOR, 00117 DISP_PARAM_RESOLUTION, 00118 DISP_PARAM_APPLY /* Use this to apply all parameters. */ 00119 }dvr_disp_disp_param_name; 00120 00121 00122 typedef struct dvr_disp_update_disp_param_tag { 00123 int disp_num; ///< input 00124 /*! #dvr_disp_disp_param_name_tag */ 00125 dvr_disp_disp_param_name param; 00126 // output 00127 struct val_t { 00128 int target_id; 00129 /*! #dvr_disp_plane_combination_tag */ 00130 int plane_comb; 00131 /*! #MCP_VIDEO_NTSC */ 00132 /*! #MCP_VIDEO_PAL */ 00133 /*! #MCP_VIDEO_VGA */ 00134 int output_system; 00135 /*! #LCDOutputModeTag */ 00136 int output_mode; 00137 int display_rate; ///< NTSC : 30, PAL : 25 00138 /*! #dvr_disp_color_attribute_tag */ 00139 dvr_disp_color_attribute color_attrib; 00140 /*! #dvr_disp_color_key_tag */ 00141 dvr_disp_color_key transparent_color[2]; 00142 /*! #dvr_disp_vbi_info_tag */ 00143 dvr_disp_vbi_info vbi_info; 00144 /*! #dvr_disp_scaler_info_tag */ 00145 dvr_disp_scaler_info scl_info; 00146 /*! #dvr_disp_resolution_tag */ 00147 dvr_disp_resolution res; 00148 int reserved[8]; 00149 }val; 00150 }dvr_disp_update_disp_param; 00151 00152 00153 00154 //### Declaration for DVR_DISP_GET_PLANE_PARAM and DVR_DISP_SET_PLANE_PARAM ### 00155 typedef struct dvr_disp_plane_param_tag { 00156 int plane_id; 00157 /*! #RECT_tag */ 00158 RECT win; 00159 /*! #LCDOutputModeTag */ 00160 int data_mode; 00161 /*! #LCDOutputColorTypeTag */ 00162 int color_mode; 00163 int reserved[2]; 00164 }dvr_disp_plane_param_st; 00165 00166 typedef struct dvr_disp_plane_param_st_tag { 00167 //input 00168 int disp_num; 00169 int plane_num; 00170 /*! #dvr_disp_plane_param_tag */ 00171 dvr_disp_plane_param_st param; 00172 int reserved[2]; 00173 }dvr_disp_plane_param; 00174 00175 00176 //### Declaration for DVR_DISP_UPDATE_PLANE_PARAM ### 00177 typedef enum dvr_disp_plane_param_name_tag { 00178 PLANE_PARAM_COLOR_MODE, 00179 PLANE_PARAM_WINDOW, 00180 PLANE_PARAM_DATA_MODE, 00181 PLANE_PARAM_APPLY 00182 }dvr_disp_plane_param_name; 00183 00184 typedef struct dvr_disp_update_plane_param_tag { 00185 //input 00186 int plane_id; 00187 /*! #dvr_disp_plane_param_name_tag */ 00188 dvr_disp_plane_param_name param; 00189 int reserved[8]; 00190 union { 00191 /*! #RECT_tag */ 00192 RECT win; 00193 /*! #LCDOutputModeTag */ 00194 int data_mode; 00195 /*! #LCDOutputColorTypeTag */ 00196 int color_mode; 00197 }val; 00198 int reserved1[8]; 00199 }dvr_disp_update_plane_param; 00200 00201 00202 //### Declaration for DVR_DISP_CONTROL ### 00203 typedef enum dvr_disp_ctrl_cmd_tag { 00204 DISP_START, 00205 DISP_STOP, 00206 DISP_UPDATE, 00207 DISP_RUN, 00208 }dvr_disp_ctrl_cmd; 00209 //+++ stanley add 00210 typedef enum dvr_disp_channel_type_tag { 00211 DISP_NORMAL_CHN, 00212 DISP_LAYER0_CHN, 00213 DISP_LAYER1_CHN, 00214 }dvr_disp_channel_type; 00215 00216 typedef struct DispParam_Ext1_tag { 00217 /*! #dvr_disp_channel_type_tag */ 00218 dvr_disp_channel_type chn_type; 00219 }DispParam_Ext1; 00220 //--- 00221 #define DVR_PARAM_MAGIC 0x1688 00222 #define DVR_PARAM_MAGIC_SHIFT 16 00223 #define CAP_BUF_ID(id) ((DVR_PARAM_MAGIC << DVR_PARAM_MAGIC_SHIFT)|(id)) 00224 //+++ stanley add 00225 #define DVR_DISP_MAGIC_ADD_VAL(val) ((DVR_PARAM_MAGIC << DVR_PARAM_MAGIC_SHIFT)|(val)) 00226 #define DVR_DISP_CHECK_MAGIC(val) (((val)>>DVR_PARAM_MAGIC_SHIFT)==DVR_PARAM_MAGIC) 00227 #define DVR_DISP_GET_VALUE(val) ((val)&((1<<DVR_PARAM_MAGIC_SHIFT)-1)) 00228 //--- 00229 00230 typedef struct dvr_disp_control_tag { 00231 /*! #dvr_disp_type */ 00232 int type; 00233 int channel; 00234 /*! #dvr_disp_ctrl_cmd_tag */ 00235 dvr_disp_ctrl_cmd command; 00236 00237 int reserved[8]; 00238 00239 struct 00240 { 00241 struct { 00242 int cap_path; 00243 /*! #LiveviewFrameTypeTag */ 00244 int di_mode; 00245 /*! #LiveviewFrameModeTag */ 00246 int mode; 00247 /*! #LiveviewDMAOrderTag */ 00248 int dma_order; 00249 /*! #LiveviewScalerRatioTag */ 00250 int scale_indep; ///< 0:fixed aspect-ratio 00251 /*! #MCP_VIDEO_NTSC */ 00252 /*! #MCP_VIDEO_PAL */ 00253 /*! #MCP_VIDEO_VGA */ 00254 int input_system; 00255 int cap_rate; 00256 /*! #CaptureColorModeTag */ 00257 int color_mode; 00258 int is_use_scaler; //if TRUE, capture as "src_para.lv.dim" and scale it to "des_param.lv.win" 00259 //if FALSE, capture as "des_param.lv.win" and display it directly 00260 /*! #DIM_tag */ 00261 DIM dim; 00262 /*! #RECT_tag */ 00263 RECT win; 00264 /*! #video_process_tag */ 00265 video_process vp_param; 00266 /*! #ScalerParamtag */ 00267 ScalerParam scl_param; //if is_use_scaler is TRUE, user needs to fill this structure 00268 int cap_buf_id; 00269 /*! display parameter extension size */ 00270 int ext_size; 00271 /*! point to display parameter extension structure */ 00272 void *pext_data; 00273 int reserved[2]; 00274 }lv; 00275 struct { 00276 /*! #RECT_tag */ 00277 RECT win; 00278 int rate; 00279 /*! #LiveviewFrameTypeTag */ 00280 int di_mode; 00281 /*! #LiveviewFrameModeTag */ 00282 int mode; 00283 /*! #LiveviewDMAOrderTag */ 00284 int dma_order; 00285 /*! #LiveviewScalerRatioTag */ 00286 int scale_indep; 00287 /*! #MCP_VIDEO_NTSC */ 00288 /*! #MCP_VIDEO_PAL */ 00289 /*! #MCP_VIDEO_VGA */ 00290 int input_system; 00291 int cap_rate; 00292 /*! #CaptureColorModeTag */ 00293 int color_mode; 00294 int is_use_scaler; //if TRUE, capture as "src_para.lv.dim" and scale it to "des_param.lv.win" 00295 //if FALSE, capture as "des_param.lv.win" and display it directly 00296 /*! #DIM_tag */ 00297 DIM dim; 00298 /*! #video_process_tag */ 00299 video_process vp_param; 00300 /*! #ScalerParamtag */ 00301 ScalerParam scl_param; ///< if is_use_scaler is TRUE, user needs to fill this structure 00302 int reserved[5]; 00303 }cas; 00304 int reserved[5]; 00305 }src_param; 00306 00307 struct 00308 { 00309 struct { 00310 /*! #RECT_tag */ 00311 RECT win; 00312 int plane_id; 00313 int reserved[5]; 00314 }lv; 00315 00316 struct { 00317 int path; 00318 /*! #RECT_tag */ 00319 RECT win; 00320 /*! #RECT_tag */ 00321 RECT rect0; 00322 /*! #RECT_tag */ 00323 RECT rect1; 00324 /*! #RECT_tag */ 00325 RECT swc_rect0; 00326 /*! #RECT_tag */ 00327 RECT swc_rect1; 00328 /*! #RECT_tag */ 00329 RECT bg_rect0; 00330 /*! #RECT_tag */ 00331 RECT bg_rect1; 00332 int plane_id; 00333 int reserved[5]; 00334 }cas; 00335 int reserved[5]; 00336 }dst_param; 00337 }dvr_disp_control; 00338 00339 00340 typedef struct dvr_disp_clear_param_tag 00341 { 00342 int plane_id; 00343 /*! #RECT_tag */ 00344 RECT win; 00345 unsigned int pattern; 00346 int reserved[2]; 00347 }dvr_disp_clear_param; 00348 00349 #endif /* __DVR_DISP_API_H__ */ 00350 00351
Generated on Wed Jun 15 2011 15:51:00 for This describe GM8126 ioctl functions by
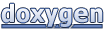