API
For Arduino developers
|
Provide an easy-to-use way to manipulate ESP8266. More...
#include <ESP8266.h>
Public Member Functions | |
bool | kick (void) |
Verify ESP8266 whether live or not. More... | |
bool | restart (void) |
Restart ESP8266 by "AT+RST". More... | |
String | getVersion (void) |
Get the version of AT Command Set. More... | |
bool | deepSleep (uint32_t time) |
Start function of deep sleep. More... | |
bool | setEcho (uint8_t mode) |
Switch the echo function. More... | |
bool | restore (void) |
Restore factory. More... | |
bool | setUart (uint32_t baudrate, uint8_t pattern) |
Set up a serial port configuration. More... | |
bool | setOprToStation (uint8_t pattern1=3, uint8_t pattern2=3) |
Set operation mode to station. More... | |
String | getWifiModeList (void) |
Get the model values list. More... | |
bool | setOprToSoftAP (uint8_t pattern1=3, uint8_t pattern2=3) |
Set operation mode to softap. More... | |
bool | setOprToStationSoftAP (uint8_t pattern1=3, uint8_t pattern2=3) |
Set operation mode to station + softap. More... | |
String | getAPList (void) |
Search available AP list and return it. More... | |
String | getNowConecAp (uint8_t pattern=3) |
Search and returns the current connect AP. More... | |
bool | joinAP (String ssid, String pwd, uint8_t pattern=3) |
Join in AP. More... | |
bool | leaveAP (void) |
Leave AP joined before. More... | |
bool | setSoftAPParam (String ssid, String pwd, uint8_t chl=7, uint8_t ecn=4, uint8_t pattern=3) |
Set SoftAP parameters. More... | |
String | getSoftAPParam (uint8_t pattern=3) |
get SoftAP parameters. More... | |
String | getJoinedDeviceIP (void) |
Get the IP list of devices connected to SoftAP. More... | |
String | getDHCP (uint8_t pattern=3) |
Get the current state of DHCP. More... | |
bool | setDHCP (uint8_t mode, uint8_t en, uint8_t pattern=3) |
Set the state of DHCP. More... | |
bool | setAutoConnect (uint8_t en) |
make boot automatically connected. More... | |
String | getStationMac (uint8_t pattern=3) |
Get the station's MAC address. More... | |
bool | setStationMac (String mac, uint8_t pattern=3) |
Set the station's MAC address. More... | |
String | getStationIp (uint8_t pattern=3) |
Get the station's IP. More... | |
bool | setStationIp (String ip, String gateway, String netmask, uint8_t pattern=3) |
Set the station's IP. More... | |
String | getAPIp (uint8_t pattern=3) |
Get the AP's IP. More... | |
bool | setAPIp (String ip, uint8_t pattern=3) |
Set the AP IP. More... | |
bool | startSmartConfig (uint8_t type) |
start smartconfig. More... | |
bool | stopSmartConfig (void) |
stop smartconfig. More... | |
String | getIPStatus (void) |
Get the current status of connection(UDP and TCP). More... | |
String | getLocalIP (void) |
Get the IP address of ESP8266. More... | |
bool | enableMUX (void) |
Enable IP MUX(multiple connection mode). More... | |
bool | disableMUX (void) |
Disable IP MUX(single connection mode). More... | |
bool | createTCP (String addr, uint32_t port) |
Create TCP connection in single mode. More... | |
bool | releaseTCP (void) |
Release TCP connection in single mode. More... | |
bool | registerUDP (String addr, uint32_t port) |
Register UDP port number in single mode. More... | |
bool | unregisterUDP (void) |
Unregister UDP port number in single mode. More... | |
bool | createTCP (uint8_t mux_id, String addr, uint32_t port) |
Create TCP connection in multiple mode. More... | |
bool | releaseTCP (uint8_t mux_id) |
Release TCP connection in multiple mode. More... | |
bool | registerUDP (uint8_t mux_id, String addr, uint32_t port) |
Register UDP port number in multiple mode. More... | |
bool | unregisterUDP (uint8_t mux_id) |
Unregister UDP port number in multiple mode. More... | |
bool | setTCPServerTimeout (uint32_t timeout=180) |
Set the timeout of TCP Server. More... | |
bool | startTCPServer (uint32_t port=333) |
Start TCP Server(Only in multiple mode). More... | |
bool | stopTCPServer (void) |
Stop TCP Server(Only in multiple mode). More... | |
bool | setCIPMODE (uint8_t mode) |
Set the module transfer mode. More... | |
bool | startServer (uint32_t port=333) |
Start Server(Only in multiple mode). More... | |
bool | stopServer (void) |
Stop Server(Only in multiple mode). More... | |
bool | saveTransLink (uint8_t mode, String ip, uint32_t port) |
Save the passthrough links. More... | |
bool | setPing (String ip) |
PING COMMAND. More... | |
bool | send (const uint8_t *buffer, uint32_t len) |
Send data based on TCP or UDP builded already in single mode. More... | |
bool | send (uint8_t mux_id, const uint8_t *buffer, uint32_t len) |
Send data based on one of TCP or UDP builded already in multiple mode. More... | |
uint32_t | recv (uint8_t *buffer, uint32_t buffer_size, uint32_t timeout=1000) |
Receive data from TCP or UDP builded already in single mode. More... | |
uint32_t | recv (uint8_t mux_id, uint8_t *buffer, uint32_t buffer_size, uint32_t timeout=1000) |
Receive data from one of TCP or UDP builded already in multiple mode. More... | |
uint32_t | recv (uint8_t *coming_mux_id, uint8_t *buffer, uint32_t buffer_size, uint32_t timeout=1000) |
Receive data from all of TCP or UDP builded already in multiple mode. More... | |
Detailed Description
Provide an easy-to-use way to manipulate ESP8266.
Member Function Documentation
bool ESP8266::createTCP | ( | String | addr, |
uint32_t | port | ||
) |
Create TCP connection in single mode.
- Parameters
-
addr - the IP or domain name of the target host. port - the port number of the target host.
- Return values
-
true - success. false - failure.
- Examples:
- HTTPGET.ino, TCPClientMultiple.ino, TCPClientSingle.ino, and TCPClientSingleUNO.ino.
Definition at line 298 of file ESP8266.cpp.
bool ESP8266::createTCP | ( | uint8_t | mux_id, |
String | addr, | ||
uint32_t | port | ||
) |
Create TCP connection in multiple mode.
- Parameters
-
mux_id - the identifier of this TCP(available value: 0 - 4). addr - the IP or domain name of the target host. port - the port number of the target host.
- Return values
-
true - success. false - failure.
Definition at line 318 of file ESP8266.cpp.
bool ESP8266::deepSleep | ( | uint32_t | time | ) |
Start function of deep sleep.
- Parameters
-
time - the sleep time.
- Return values
-
true - success. false - failure.
- Note
- the feature requires hardware support.
Definition at line 101 of file ESP8266.cpp.
bool ESP8266::disableMUX | ( | void | ) |
Disable IP MUX(single connection mode).
In single connection mode, only one TCP or UDP communication can be builded.
- Return values
-
true - success. false - failure.
- Examples:
- HTTPGET.ino, TCPClientSingle.ino, TCPClientSingleUNO.ino, and UDPClientSingle.ino.
Definition at line 293 of file ESP8266.cpp.
bool ESP8266::enableMUX | ( | void | ) |
Enable IP MUX(multiple connection mode).
In multiple connection mode, a couple of TCP and UDP communication can be builded. They can be distinguished by the identifier of TCP or UDP named mux_id.
- Return values
-
true - success. false - failure.
- Examples:
- TCPClientMultiple.ino, TCPServer.ino, and UDPClientMultiple.ino.
Definition at line 288 of file ESP8266.cpp.
String ESP8266::getAPIp | ( | uint8_t | pattern = 3 | ) |
Get the AP's IP.
- Parameters
-
pattern -1 send "AT+CIPAP_DEF?" -2 send "AT+CIPAP_CUR?" -3 send "AT+CIPAP?".
- Returns
- ap's ip.
- Note
- This method should not be called when station mode.
- Examples:
- test.ino.
Definition at line 249 of file ESP8266.cpp.
String ESP8266::getAPList | ( | void | ) |
Search available AP list and return it.
- Returns
- the list of available APs.
- Note
- This method will occupy a lot of memeory(hundreds of Bytes to a couple of KBytes). Do not call this method unless you must and ensure that your board has enough memery left.
- Examples:
- test.ino.
Definition at line 171 of file ESP8266.cpp.
String ESP8266::getDHCP | ( | uint8_t | pattern = 3 | ) |
Get the current state of DHCP.
- Parameters
-
pattern -1 send "AT+CWDHCP_DEF?" -2 send "AT+CWDHCP_CUR?" -3 send "AT+CWDHCP?".
- Returns
- the state of DHCP.
- Examples:
- test.ino.
Definition at line 209 of file ESP8266.cpp.
String ESP8266::getIPStatus | ( | void | ) |
Get the current status of connection(UDP and TCP).
- Returns
- the status.
- Examples:
- TCPServer.ino, and test.ino.
Definition at line 274 of file ESP8266.cpp.
String ESP8266::getJoinedDeviceIP | ( | void | ) |
Get the IP list of devices connected to SoftAP.
- Returns
- the list of IP.
- Note
- This method should not be called when station mode.
- Examples:
- test.ino.
Definition at line 202 of file ESP8266.cpp.
String ESP8266::getLocalIP | ( | void | ) |
Get the IP address of ESP8266.
- Returns
- the IP list.
- Examples:
- ConnectWiFi.ino, HTTPGET.ino, TCPClientMultiple.ino, TCPClientSingle.ino, TCPClientSingleUNO.ino, TCPServer.ino, UDPClientMultiple.ino, and UDPClientSingle.ino.
Definition at line 281 of file ESP8266.cpp.
String ESP8266::getNowConecAp | ( | uint8_t | pattern = 3 | ) |
Search and returns the current connect AP.
- Parameters
-
pattern -1, send "AT+CWJAP_DEF?",-2,send "AT+CWJAP_CUR?",-3,send "AT+CWJAP?".
- Returns
- the ssid of AP connected now.
- Examples:
- test.ino.
Definition at line 163 of file ESP8266.cpp.
String ESP8266::getSoftAPParam | ( | uint8_t | pattern = 3 | ) |
get SoftAP parameters.
- Parameters
-
pattern -1 send "AT+CWSAP_DEF?" -2 send "AT+CWSAP_CUR?" -3 send "AT+CWSAP?".
- Note
- This method should not be called when station mode.
- Examples:
- test.ino.
Definition at line 188 of file ESP8266.cpp.
String ESP8266::getStationIp | ( | uint8_t | pattern = 3 | ) |
Get the station's IP.
- Parameters
-
pattern -1 send "AT+CIPSTA_DEF?" -2 send "AT+CIPSTA_CUR?" -3 send "AT+CIPSTA?".
- Returns
- the station's IP.
- Note
- This method should not be called when ap mode.
- Examples:
- test.ino.
Definition at line 237 of file ESP8266.cpp.
String ESP8266::getStationMac | ( | uint8_t | pattern = 3 | ) |
Get the station's MAC address.
- Parameters
-
pattern -1 send "AT+CIPSTAMAC_DEF?=" -2 send "AT+CIPSTAMAC_CUR?" -3 send "AT+CIPSTAMAC?".
- Returns
- mac address.
- Note
- This method should not be called when ap mode.
- Examples:
- test.ino.
Definition at line 224 of file ESP8266.cpp.
String ESP8266::getVersion | ( | void | ) |
Get the version of AT Command Set.
- Returns
- the string of version.
- Examples:
- ConnectWiFi.ino, HTTPGET.ino, TCPClientMultiple.ino, TCPClientSingle.ino, TCPClientSingleUNO.ino, TCPServer.ino, UDPClientMultiple.ino, and UDPClientSingle.ino.
Definition at line 80 of file ESP8266.cpp.
String ESP8266::getWifiModeList | ( | void | ) |
Get the model values list.
- Returns
- the list of model.
- Examples:
- test.ino.
Definition at line 123 of file ESP8266.cpp.
bool ESP8266::joinAP | ( | String | ssid, |
String | pwd, | ||
uint8_t | pattern = 3 |
||
) |
Join in AP.
- Parameters
-
pattern -1 send "AT+CWJAP_DEF=" -2 send "AT+CWJAP_CUR=" -3 send "AT+CWJAP=". ssid - SSID of AP to join in. pwd - Password of AP to join in.
- Return values
-
true - success. false - failure.
- Note
- This method will take a couple of seconds.
- Examples:
- ConnectWiFi.ino, HTTPGET.ino, TCPClientMultiple.ino, TCPClientSingle.ino, TCPClientSingleUNO.ino, TCPServer.ino, test.ino, UDPClientMultiple.ino, and UDPClientSingle.ino.
Definition at line 178 of file ESP8266.cpp.
bool ESP8266::kick | ( | void | ) |
Verify ESP8266 whether live or not.
Actually, this method will send command "AT" to ESP8266 and waiting for "OK".
- Return values
-
true - alive. false - dead.
Definition at line 58 of file ESP8266.cpp.
bool ESP8266::leaveAP | ( | void | ) |
Leave AP joined before.
- Return values
-
true - success. false - failure.
- Examples:
- test.ino.
Definition at line 183 of file ESP8266.cpp.
uint32_t ESP8266::recv | ( | uint8_t * | buffer, |
uint32_t | buffer_size, | ||
uint32_t | timeout = 1000 |
||
) |
Receive data from TCP or UDP builded already in single mode.
- Parameters
-
buffer - the buffer for storing data. buffer_size - the length of the buffer. timeout - the time waiting data.
- Returns
- the length of data received actually.
- Examples:
- HTTPGET.ino, TCPClientMultiple.ino, TCPClientSingle.ino, TCPClientSingleUNO.ino, TCPServer.ino, UDPClientMultiple.ino, and UDPClientSingle.ino.
Definition at line 396 of file ESP8266.cpp.
uint32_t ESP8266::recv | ( | uint8_t | mux_id, |
uint8_t * | buffer, | ||
uint32_t | buffer_size, | ||
uint32_t | timeout = 1000 |
||
) |
Receive data from one of TCP or UDP builded already in multiple mode.
- Parameters
-
mux_id - the identifier of this TCP(available value: 0 - 4). buffer - the buffer for storing data. buffer_size - the length of the buffer. timeout - the time waiting data.
- Returns
- the length of data received actually.
Definition at line 401 of file ESP8266.cpp.
uint32_t ESP8266::recv | ( | uint8_t * | coming_mux_id, |
uint8_t * | buffer, | ||
uint32_t | buffer_size, | ||
uint32_t | timeout = 1000 |
||
) |
Receive data from all of TCP or UDP builded already in multiple mode.
After return, coming_mux_id store the id of TCP or UDP from which data coming. User should read the value of coming_mux_id and decide what next to do.
- Parameters
-
coming_mux_id - the identifier of TCP or UDP. buffer - the buffer for storing data. buffer_size - the length of the buffer. timeout - the time waiting data.
- Returns
- the length of data received actually.
Definition at line 412 of file ESP8266.cpp.
bool ESP8266::registerUDP | ( | String | addr, |
uint32_t | port | ||
) |
Register UDP port number in single mode.
- Parameters
-
addr - the IP or domain name of the target host. port - the port number of the target host.
- Return values
-
true - success. false - failure.
- Examples:
- UDPClientMultiple.ino, and UDPClientSingle.ino.
Definition at line 308 of file ESP8266.cpp.
bool ESP8266::registerUDP | ( | uint8_t | mux_id, |
String | addr, | ||
uint32_t | port | ||
) |
Register UDP port number in multiple mode.
- Parameters
-
mux_id - the identifier of this TCP(available value: 0 - 4). addr - the IP or domain name of the target host. port - the port number of the target host.
- Return values
-
true - success. false - failure.
Definition at line 328 of file ESP8266.cpp.
bool ESP8266::releaseTCP | ( | void | ) |
Release TCP connection in single mode.
- Return values
-
true - success. false - failure.
- Examples:
- HTTPGET.ino, TCPClientMultiple.ino, TCPClientSingle.ino, TCPClientSingleUNO.ino, and TCPServer.ino.
Definition at line 303 of file ESP8266.cpp.
bool ESP8266::releaseTCP | ( | uint8_t | mux_id | ) |
Release TCP connection in multiple mode.
- Parameters
-
mux_id - the identifier of this TCP(available value: 0 - 4).
- Return values
-
true - success. false - failure.
Definition at line 323 of file ESP8266.cpp.
bool ESP8266::restart | ( | void | ) |
Restart ESP8266 by "AT+RST".
This method will take 3 seconds or more.
- Return values
-
true - success. false - failure.
Definition at line 63 of file ESP8266.cpp.
bool ESP8266::restore | ( | void | ) |
Restore factory.
- Return values
-
true - success. false - failure.
- Note
- The operation can lead to restart the machine.
Definition at line 92 of file ESP8266.cpp.
bool ESP8266::saveTransLink | ( | uint8_t | mode, |
String | ip, | ||
uint32_t | port | ||
) |
Save the passthrough links.
- Return values
-
true - success. false - failure.
- Examples:
- test.ino.
Definition at line 363 of file ESP8266.cpp.
bool ESP8266::send | ( | const uint8_t * | buffer, |
uint32_t | len | ||
) |
Send data based on TCP or UDP builded already in single mode.
- Parameters
-
buffer - the buffer of data to send. len - the length of data to send.
- Return values
-
true - success. false - failure.
- Examples:
- HTTPGET.ino, TCPClientMultiple.ino, TCPClientSingle.ino, TCPClientSingleUNO.ino, TCPServer.ino, UDPClientMultiple.ino, and UDPClientSingle.ino.
Definition at line 386 of file ESP8266.cpp.
bool ESP8266::send | ( | uint8_t | mux_id, |
const uint8_t * | buffer, | ||
uint32_t | len | ||
) |
Send data based on one of TCP or UDP builded already in multiple mode.
- Parameters
-
mux_id - the identifier of this TCP(available value: 0 - 4). buffer - the buffer of data to send. len - the length of data to send.
- Return values
-
true - success. false - failure.
Definition at line 391 of file ESP8266.cpp.
bool ESP8266::setAPIp | ( | String | ip, |
uint8_t | pattern = 3 |
||
) |
Set the AP IP.
- Parameters
-
pattern -1 send "AT+CIPAP_DEF=" -2 send "AT+CIPAP_CUR=" -3 send "AT+CIPAP=". ip - the ip of AP.
- Return values
-
true - success. false - failure.
- Note
- This method should not be called when station mode.
- Examples:
- test.ino.
Definition at line 256 of file ESP8266.cpp.
bool ESP8266::setAutoConnect | ( | uint8_t | en | ) |
make boot automatically connected.
- Parameters
-
en -1 enable -0 disable.
- Return values
-
true - success. false - failure.
- Examples:
- test.ino.
Definition at line 220 of file ESP8266.cpp.
bool ESP8266::setCIPMODE | ( | uint8_t | mode | ) |
Set the module transfer mode.
- Return values
-
true - success. false - failure.
Definition at line 358 of file ESP8266.cpp.
bool ESP8266::setDHCP | ( | uint8_t | mode, |
uint8_t | en, | ||
uint8_t | pattern = 3 |
||
) |
Set the state of DHCP.
- Parameters
-
pattern -1 send "AT+CWDHCP_DEF=" -2 send "AT+CWDHCP_CUR=" -3 send "AT+CWDHCP=". mode - set ap or set station or set ap + station. en - 0 disable DHCP - 1 enable DHCP.
- Return values
-
true - success. false - failure.
- Examples:
- test.ino.
Definition at line 215 of file ESP8266.cpp.
bool ESP8266::setEcho | ( | uint8_t | mode | ) |
Switch the echo function.
- Parameters
-
mode - 1 start echo -0 stop echo
- Return values
-
true - success. false - failure.
Definition at line 87 of file ESP8266.cpp.
bool ESP8266::setOprToSoftAP | ( | uint8_t | pattern1 = 3 , |
uint8_t | pattern2 = 3 |
||
) |
Set operation mode to softap.
- Parameters
-
pattern1 -1, send "AT+CWMODE_DEF?",-2,send "AT+CWMODE_CUR?",-3,send "AT+CWMODE?". pattern2 -1, send "AT+CWMODE_DEF=",-2,send "AT+CWMODE_CUR=",-3,send "AT+CWMODE=".
- Return values
-
true - success. false - failure.
- Examples:
- test.ino.
Definition at line 129 of file ESP8266.cpp.
bool ESP8266::setOprToStation | ( | uint8_t | pattern1 = 3 , |
uint8_t | pattern2 = 3 |
||
) |
Set operation mode to station.
- Parameters
-
pattern1 -1, send "AT+CWMODE_DEF?",-2,send "AT+CWMODE_CUR?",-3,send "AT+CWMODE?". pattern2 -1, send "AT+CWMODE_DEF=",-2,send "AT+CWMODE_CUR=",-3,send "AT+CWMODE=".
- Return values
-
true - success. false - failure.
- Examples:
- ConnectWiFi.ino, and test.ino.
Definition at line 107 of file ESP8266.cpp.
bool ESP8266::setOprToStationSoftAP | ( | uint8_t | pattern1 = 3 , |
uint8_t | pattern2 = 3 |
||
) |
Set operation mode to station + softap.
- Parameters
-
pattern1 -1, send "AT+CWMODE_DEF?",-2,send "AT+CWMODE_CUR?",-3,send "AT+CWMODE?". pattern2 -1, send "AT+CWMODE_DEF=",-2,send "AT+CWMODE_CUR=",-3,send "AT+CWMODE=".
- Return values
-
true - success. false - failure.
- Examples:
- HTTPGET.ino, TCPClientMultiple.ino, TCPClientSingle.ino, TCPClientSingleUNO.ino, TCPServer.ino, test.ino, UDPClientMultiple.ino, and UDPClientSingle.ino.
Definition at line 146 of file ESP8266.cpp.
bool ESP8266::setPing | ( | String | ip | ) |
PING COMMAND.
- Return values
-
true - success. false - failure.
Definition at line 368 of file ESP8266.cpp.
bool ESP8266::setSoftAPParam | ( | String | ssid, |
String | pwd, | ||
uint8_t | chl = 7 , |
||
uint8_t | ecn = 4 , |
||
uint8_t | pattern = 3 |
||
) |
Set SoftAP parameters.
- Parameters
-
pattern -1 send "AT+CWSAP_DEF=" -2 send "AT+CWSAP_CUR=" -3 send "AT+CWSAP=". ssid - SSID of SoftAP. pwd - PASSWORD of SoftAP. chl - the channel (1 - 13, default: 7). ecn - the way of encrypstion (0 - OPEN, 1 - WEP, 2 - WPA_PSK, 3 - WPA2_PSK, 4 - WPA_WPA2_PSK, default: 4).
- Return values
-
true - success. false - failure.
- Note
- This method should not be called when station mode.
- Examples:
- test.ino.
Definition at line 197 of file ESP8266.cpp.
bool ESP8266::setStationIp | ( | String | ip, |
String | gateway, | ||
String | netmask, | ||
uint8_t | pattern = 3 |
||
) |
Set the station's IP.
- Parameters
-
pattern -1 send "AT+CIPSTA_DEF=" -2 send "AT+CIPSTA_CUR=" -3 send "AT+CIPSTA=". ip - the ip of station. gateway -the gateway of station. netmask -the netmask of station.
- Return values
-
true - success. false - failure.
- Note
- This method should not be called when ap mode.
- Examples:
- test.ino.
Definition at line 244 of file ESP8266.cpp.
bool ESP8266::setStationMac | ( | String | mac, |
uint8_t | pattern = 3 |
||
) |
Set the station's MAC address.
- Parameters
-
pattern -1 send "AT+CIPSTAMAC_DEF=" -2 send "AT+CIPSTAMAC_CUR=" -3 send "AT+CIPSTAMAC=". mac - the mac address of station.
- Return values
-
true - success. false - failure.
- Examples:
- test.ino.
Definition at line 232 of file ESP8266.cpp.
bool ESP8266::setTCPServerTimeout | ( | uint32_t | timeout = 180 | ) |
Set the timeout of TCP Server.
- Parameters
-
timeout - the duration for timeout by second(0 ~ 28800, default:180).
- Return values
-
true - success. false - failure.
- Examples:
- TCPServer.ino.
Definition at line 338 of file ESP8266.cpp.
bool ESP8266::setUart | ( | uint32_t | baudrate, |
uint8_t | pattern | ||
) |
Set up a serial port configuration.
- Parameters
-
pattern -1 send "AT+UART=", -2 send "AT+UART_CUR=", -3 send "AT+UART_DEF=". baudrate - the uart baudrate.
- Return values
-
true - success. false - failure.
- Note
- Only allows baud rate design, for the other parameters:databits- 8,stopbits -1,parity -0,flow control -0 .
- Examples:
- test.ino.
Definition at line 96 of file ESP8266.cpp.
bool ESP8266::startServer | ( | uint32_t | port = 333 | ) |
Start Server(Only in multiple mode).
- Parameters
-
port - the port number to listen(default: 333).
- Return values
-
true - success. false - failure.
- See also
- String getIPStatus(void);
- uint32_t recv(uint8_t *coming_mux_id, uint8_t *buffer, uint32_t len, uint32_t timeout);
Definition at line 376 of file ESP8266.cpp.
bool ESP8266::startSmartConfig | ( | uint8_t | type | ) |
start smartconfig.
- Parameters
-
type -1:ESP_TOUCH -2:AirKiss.
- Return values
-
true - success. false - failure.
- Examples:
- test.ino.
Definition at line 261 of file ESP8266.cpp.
bool ESP8266::startTCPServer | ( | uint32_t | port = 333 | ) |
Start TCP Server(Only in multiple mode).
After started, user should call method: getIPStatus to know the status of TCP connections. The methods of receiving data can be called for user's any purpose. After communication, release the TCP connection is needed by calling method: releaseTCP with mux_id.
- Parameters
-
port - the port number to listen(default: 333).
- Return values
-
true - success. false - failure.
- See also
- String getIPStatus(void);
- uint32_t recv(uint8_t *coming_mux_id, uint8_t *buffer, uint32_t len, uint32_t timeout);
- bool releaseTCP(uint8_t mux_id);
- Examples:
- TCPServer.ino.
Definition at line 343 of file ESP8266.cpp.
bool ESP8266::stopServer | ( | void | ) |
Stop Server(Only in multiple mode).
- Return values
-
true - success. false - failure.
Definition at line 381 of file ESP8266.cpp.
bool ESP8266::stopSmartConfig | ( | void | ) |
stop smartconfig.
- Return values
-
true - success. false - failure.
- Examples:
- test.ino.
Definition at line 266 of file ESP8266.cpp.
bool ESP8266::stopTCPServer | ( | void | ) |
Stop TCP Server(Only in multiple mode).
- Return values
-
true - success. false - failure.
Definition at line 351 of file ESP8266.cpp.
bool ESP8266::unregisterUDP | ( | void | ) |
Unregister UDP port number in single mode.
- Return values
-
true - success. false - failure.
- Examples:
- UDPClientMultiple.ino, and UDPClientSingle.ino.
Definition at line 313 of file ESP8266.cpp.
bool ESP8266::unregisterUDP | ( | uint8_t | mux_id | ) |
Unregister UDP port number in multiple mode.
- Parameters
-
mux_id - the identifier of this TCP(available value: 0 - 4).
- Return values
-
true - success. false - failure.
Definition at line 333 of file ESP8266.cpp.
The documentation for this class was generated from the following files:
Generated on Thu Apr 9 2015 13:57:59 for API by
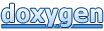