Dong_Minh_Doxygen
|
EnemyAI.cs
Go to the documentation of this file.
113 transform.rotation = Quaternion.Slerp (transform.rotation, rotation, Time.deltaTime * rotationDamping);
129 GameObject BulletInstance = Instantiate(bulletPrefab, bulletEmitter.position, bulletEmitter.rotation) as GameObject;
void lookAtPlayer()
Extensive math problem here ti determine the player position and enemy position.
Definition: EnemyAI.cs:111
Definition: EnemyAI.cs:5
void Update()
Update every per frame. Only function in here is the DistanceToLookAt.
Definition: EnemyAI.cs:32
void OnCollisionEnter(Collision collision)
When there is a collision detected by the player bullet, the enemy's life will go down...
Definition: EnemyAI.cs:55
void DistanceToLookAt()
Determine a function of how far the enemy looks at the player (distance), and when to shoot at the pl...
Definition: EnemyAI.cs:82
Generated by
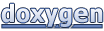