CLOCK_XMC4
|
Usage
Below shows typical usages of CLOCK_XMC4 APP.
Use case 1:
This example monitors the system clock frequency via EXTCLK pin.
Instantiate the required APPs
Drag an instance of CLOCK_XMC4. Update the fields in the GUI of this APP with the following configuration.
Configure the APP
CLOCK_XMC4:
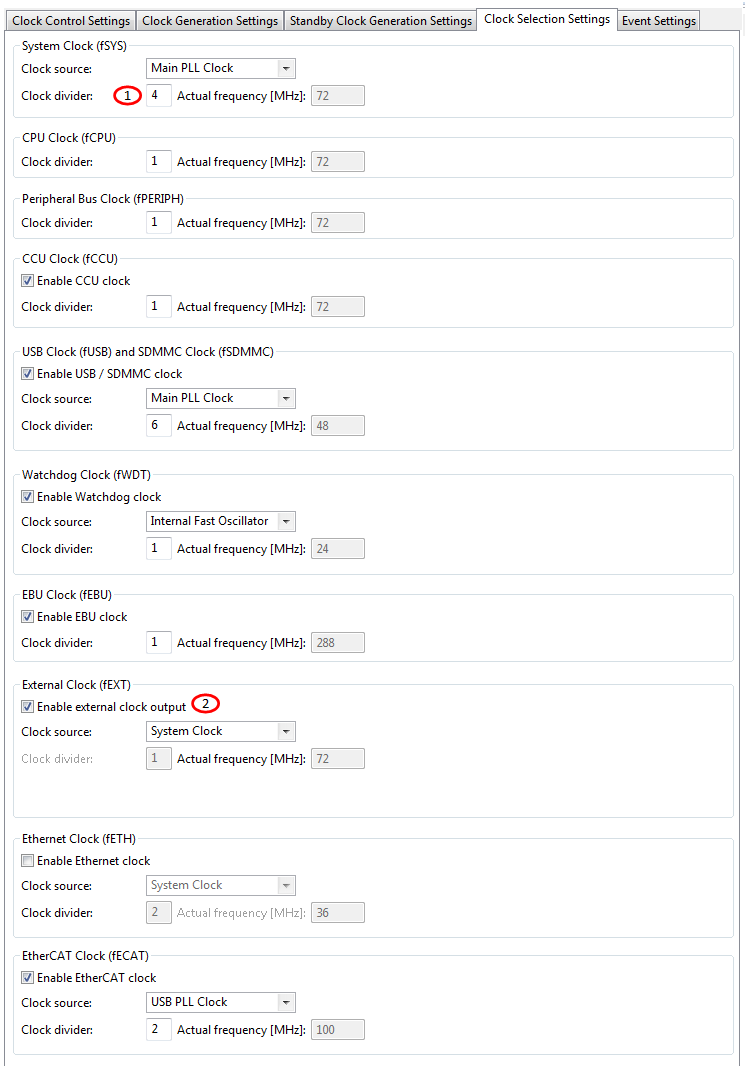
-
Configure System clock divider as 4
-
Select external clock output and System Clock as external clock output source
Manual pin allocation
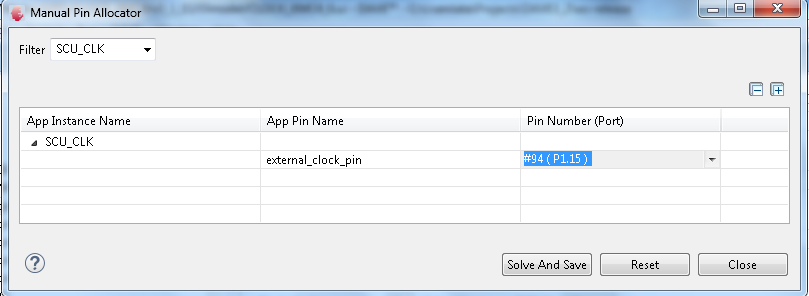
-
Select a pin to monitor System clock frequency
Note: The pin number is specific to the development board chosen to run this example. The pin shown in the image above may not be available on every XMC boot kit. Ensure that a proper pin is selected according to the board.
Generate code
Files are generated here: `<project_name>/Dave/Generated/' (`project_name' is the name chosen by the user during project creation). APP instance definitions and APIs are generated only after code generation.
Note: Code must be explicitly generated for every change in the GUI configuration.
Important: Any manual modification to APP specific files will be overwritten by a subsequent code generation operation.
Build and Run the Project
Observation
The configured system clock frequency (72MHz for XMC48/47/43 devices, 30MHz for XMC45/44 devices, 20MHz for XMC42 devices) must observes in the oscilloscope at pin P1.15.
Use case 2:
This example enables user to monitor OSC_HP frequency (fOSC) whether it is usable for the VCO as a part of the PLL or not. And also allow user to take appropriate action when NMI TRAP occurs.
Instantiate the required APPs
Drag an instance of CLOCK_XMC4 APP and CPU_CTRL_XMC4 APP. Update the fields in the GUI of this APP with the following configuration.
Configure the APP
CLOCK_XMC4:
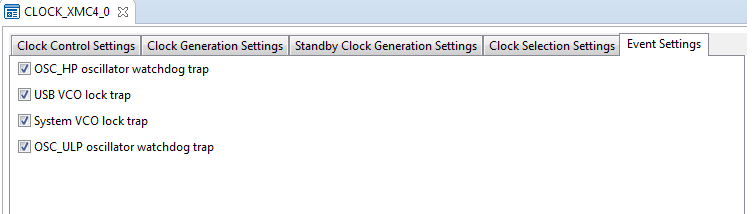
-
Enable OSC_HP oscillator watchdog trap
-
Enable USB VCO lock trap
-
Enable System VCO lock trap
-
Enable OSC_ULP oscillator watchdog trap
Note: User must define the NMI TRAP handler as void NMI_Handler(void) for handling trap.
Generate code
Files are generated here: `<project_name>/Dave/Generated/' (`project_name' is the name chosen by the user during project creation). APP instance definitions and APIs are generated only after code generation.
Note: Code must be explicitly generated for every change in the GUI configuration.
Important: Any manual modification to APP specific files will be overwritten by a subsequent code generation operation.
Sample Application (main.c)
#include <DAVE.h> //Declarations from DAVE Code Generation (includes SFR declaration) volatile uint32_t TRAP_OSC_WDG_FLAG=0; volatile uint32_t TRAP_VCO_LOCK_FLAG=0; volatile uint32_t TRAP_USB_VCO_FLAG=0; volatile uint32_t TRAP_ULP_WDG_FLAG=0; void NMI_Handler(void) { uint32_t TRAP_FLAG=0; TRAP_FLAG = XMC_SCU_TRAP_GetStatus(); if((TRAP_FLAG & XMC_SCU_TRAP_OSC_WDG) >> SCU_TRAP_TRAPSTAT_SOSCWDGT_Pos) { TRAP_OSC_WDG_FLAG++; XMC_SCU_TRAP_ClearStatus(XMC_SCU_TRAP_OSC_WDG); // Add application code here for handling OSC_HP oscillator watchdog trap } if((TRAP_FLAG & XMC_SCU_TRAP_VCO_LOCK) >> SCU_TRAP_TRAPSTAT_SVCOLCKT_Pos) { TRAP_VCO_LOCK_FLAG++; XMC_SCU_TRAP_ClearStatus(XMC_SCU_TRAP_VCO_LOCK); // Add application code here for handling system VCO lock trap } if((TRAP_FLAG & XMC_SCU_TRAP_USB_VCO_LOCK) >> SCU_TRAP_TRAPSTAT_UVCOLCKT_Pos) { TRAP_USB_VCO_FLAG++; XMC_SCU_TRAP_ClearStatus(XMC_SCU_TRAP_USB_VCO_LOCK); // Add application code here for handling USB VCO lock trap } if((TRAP_FLAG & XMC_SCU_TRAP_ULP_WDG) >> SCU_TRAP_TRAPSTAT_ULPWDGT_Pos) { TRAP_ULP_WDG_FLAG++; XMC_SCU_TRAP_ClearStatus(XMC_SCU_TRAP_ULP_WDG); // Add application code here for handling OSC_ULP oscillator watchdog trap } } // // @brief main() - Application entry point // // <b>Details of function</b><br> // This routine is the application entry point. It is invoked by the device startup code. It is responsible for // invoking the App initialization dispatcher routine - DAVE_Init() and hosting the place-holder for user application // code. // int main(void) { DAVE_STATUS_t status; status = DAVE_Init(); // CLOCK_XMC4_Init() is called from within DAVE_Init(). if(status == DAVE_STATUS_FAILURE) { // Placeholder for error handler code. The while loop below can be replaced with an user error handler XMC_DEBUG(("DAVE Apps initialization failed with status %d\n", status)); while(1U) { } } // Placeholder for user application code. The while loop below can be replaced with user application code. while(1U) { } return (1); }
Build and Run the Project
Note: User must add application code for ensuring the safety operation.
Observation
TRAP_OSC_WDG_FLAG, TRAP_VCO_LOCK_FLAG, TRAP_USB_VCO_FLAG and TRAP_ULP_WDG_FLAG flags can be monitored for ensuring the safety operation.