Ap4Utils.h
Go to the documentation of this file.00001 /***************************************************************** 00002 | 00003 | AP4 - Utilities 00004 | 00005 | Copyright 2002-2008 Axiomatic Systems, LLC 00006 | 00007 | 00008 | This file is part of Bento4/AP4 (MP4 Atom Processing Library). 00009 | 00010 | Unless you have obtained Bento4 under a difference license, 00011 | this version of Bento4 is Bento4|GPL. 00012 | Bento4|GPL is free software; you can redistribute it and/or modify 00013 | it under the terms of the GNU General Public License as published by 00014 | the Free Software Foundation; either version 2, or (at your option) 00015 | any later version. 00016 | 00017 | Bento4|GPL is distributed in the hope that it will be useful, 00018 | but WITHOUT ANY WARRANTY; without even the implied warranty of 00019 | MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00020 | GNU General Public License for more details. 00021 | 00022 | You should have received a copy of the GNU General Public License 00023 | along with Bento4|GPL; see the file COPYING. If not, write to the 00024 | Free Software Foundation, 59 Temple Place - Suite 330, Boston, MA 00025 | 02111-1307, USA. 00026 | 00027 ****************************************************************/ 00028 00029 #ifndef _AP4_UTILS_H_ 00030 #define _AP4_UTILS_H_ 00031 00032 /*---------------------------------------------------------------------- 00033 | includes 00034 +---------------------------------------------------------------------*/ 00035 #include "Ap4Config.h" 00036 #include "Ap4Types.h" 00037 #include "Ap4Results.h" 00038 #include "Ap4Config.h" 00039 00040 /*---------------------------------------------------------------------- 00041 | non-inline functions 00042 +---------------------------------------------------------------------*/ 00043 double AP4_BytesToDoubleBE(const unsigned char* bytes); 00044 AP4_UI64 AP4_BytesToUInt64BE(const unsigned char* bytes); 00045 void AP4_BytesFromDoubleBE(unsigned char* bytes, double value); 00046 void AP4_BytesFromUInt64BE(unsigned char* bytes, AP4_UI64 value); 00047 00048 /*---------------------------------------------------------------------- 00049 | AP4_BytesToUInt32BE 00050 +---------------------------------------------------------------------*/ 00051 inline AP4_UI32 00052 AP4_BytesToUInt32BE(const unsigned char* bytes) 00053 { 00054 return 00055 ( ((AP4_UI32)bytes[0])<<24 ) | 00056 ( ((AP4_UI32)bytes[1])<<16 ) | 00057 ( ((AP4_UI32)bytes[2])<<8 ) | 00058 ( ((AP4_UI32)bytes[3]) ); 00059 } 00060 00061 /*---------------------------------------------------------------------- 00062 | AP4_BytesToInt32BE 00063 +---------------------------------------------------------------------*/ 00064 inline AP4_SI32 00065 AP4_BytesToInt32BE(const unsigned char* bytes) 00066 { 00067 return AP4_BytesToUInt32BE(bytes); 00068 } 00069 00070 /*---------------------------------------------------------------------- 00071 | AP4_BytesToUInt24BE 00072 +---------------------------------------------------------------------*/ 00073 inline AP4_UI32 00074 AP4_BytesToUInt24BE(const unsigned char* bytes) 00075 { 00076 return 00077 ( ((AP4_UI32)bytes[0])<<16 ) | 00078 ( ((AP4_UI32)bytes[1])<<8 ) | 00079 ( ((AP4_UI32)bytes[2]) ); 00080 } 00081 00082 /*---------------------------------------------------------------------- 00083 | AP4_BytesToInt16BE 00084 +---------------------------------------------------------------------*/ 00085 inline AP4_UI16 00086 AP4_BytesToUInt16BE(const unsigned char* bytes) 00087 { 00088 return 00089 ( ((AP4_UI16)bytes[0])<<8 ) | 00090 ( ((AP4_UI16)bytes[1]) ); 00091 } 00092 00093 /*---------------------------------------------------------------------- 00094 | AP4_BytesToInt16BE 00095 +---------------------------------------------------------------------*/ 00096 inline AP4_SI16 00097 AP4_BytesToInt16BE(const unsigned char* bytes) 00098 { 00099 return (AP4_SI16)AP4_BytesToUInt16BE(bytes); 00100 } 00101 00102 /*---------------------------------------------------------------------- 00103 | AP4_BytesFromUInt32BE 00104 +---------------------------------------------------------------------*/ 00105 inline void 00106 AP4_BytesFromUInt32BE(unsigned char* bytes, AP4_UI32 value) 00107 { 00108 bytes[0] = (unsigned char)(value >> 24); 00109 bytes[1] = (unsigned char)(value >> 16); 00110 bytes[2] = (unsigned char)(value >> 8); 00111 bytes[3] = (unsigned char)(value ); 00112 } 00113 00114 /*---------------------------------------------------------------------- 00115 | AP4_BytesFromUInt24BE 00116 +---------------------------------------------------------------------*/ 00117 inline void 00118 AP4_BytesFromUInt24BE(unsigned char* bytes, AP4_UI32 value) 00119 { 00120 bytes[0] = (unsigned char)(value >> 16); 00121 bytes[1] = (unsigned char)(value >> 8); 00122 bytes[2] = (unsigned char)(value ); 00123 } 00124 00125 /*---------------------------------------------------------------------- 00126 | AP4_BytesFromUInt16BE 00127 +---------------------------------------------------------------------*/ 00128 inline void 00129 AP4_BytesFromUInt16BE(unsigned char* bytes, AP4_UI16 value) 00130 { 00131 bytes[0] = (unsigned char)(value >> 8); 00132 bytes[1] = (unsigned char)(value ); 00133 } 00134 00135 /*---------------------------------------------------------------------- 00136 | time functions 00137 +---------------------------------------------------------------------*/ 00138 AP4_UI32 AP4_DurationMsFromUnits(AP4_UI64 units, 00139 AP4_UI32 units_per_second); 00140 AP4_UI64 AP4_ConvertTime(AP4_UI64 time_value, 00141 AP4_UI32 from_time_scale, 00142 AP4_UI32 to_time_scale); 00143 00144 /*---------------------------------------------------------------------- 00145 | string utils 00146 +---------------------------------------------------------------------*/ 00147 #if defined (AP4_CONFIG_HAVE_STDIO_H) 00148 #include <stdio.h> 00149 #endif 00150 00151 #if defined (AP4_CONFIG_HAVE_SNPRINTF) 00152 #define AP4_FormatString AP4_snprintf 00153 #else 00154 int AP4_FormatString(char* str, AP4_Size size, const char* format, ...); 00155 #endif 00156 #if defined(AP4_CONFIG_HAVE_VSNPRINTF) 00157 #define AP4_FormatStringVN(s,c,f,a) AP4_vsnprintf(s,c,f,a) 00158 #else 00159 extern int AP4_FormatStringVN(char *buffer, size_t count, const char *format, va_list argptr); 00160 #endif 00161 00162 #if defined (AP4_CONFIG_HAVE_STRING_H) 00163 #include <string.h> 00164 #define AP4_StringLength(x) strlen(x) 00165 #define AP4_CopyMemory(x,y,z) memcpy(x,y,z) 00166 #define AP4_CompareMemory(x, y, z) memcmp(x, y, z) 00167 #define AP4_SetMemory(x,y,z) memset(x,y,z) 00168 #define AP4_CompareStrings(x,y) strcmp(x,y) 00169 #endif 00170 00171 unsigned char AP4_HexNibble(char c); 00172 char AP4_NibbleHex(unsigned int nibble); 00173 void AP4_FormatFourChars(char* str, AP4_UI32 value); 00174 void AP4_FormatFourCharsPrintable(char* str, AP4_UI32 value); 00175 AP4_Result 00176 AP4_ParseHex(const char* hex, unsigned char* bytes, unsigned int count); 00177 AP4_Result 00178 AP4_FormatHex(const AP4_UI08* data, unsigned int data_size, char* hex); 00179 AP4_Result 00180 AP4_SplitArgs(char* arg, char*& arg0, char*& arg1, char*& arg2); 00181 AP4_Result 00182 AP4_SplitArgs(char* arg, char*& arg0, char*& arg1); 00183 00184 /*---------------------------------------------------------------------- 00185 | AP4_BitWriter 00186 +---------------------------------------------------------------------*/ 00187 class AP4_BitWriter 00188 { 00189 public: 00190 AP4_BitWriter(AP4_Size size) : m_DataSize(size), m_BitCount(0) { 00191 if (size) { 00192 m_Data = new unsigned char[size]; 00193 AP4_SetMemory(m_Data, 0, size); 00194 } else { 00195 m_Data = NULL; 00196 } 00197 } 00198 ~AP4_BitWriter() { delete m_Data; } 00199 00200 void Write(AP4_UI32 bits, unsigned int bit_count); 00201 00202 unsigned int GetBitCount() { return m_BitCount; } 00203 const unsigned char* GetData() { return m_Data; } 00204 00205 private: 00206 unsigned char* m_Data; 00207 unsigned int m_DataSize; 00208 unsigned int m_BitCount; 00209 }; 00210 00211 00212 #endif // _AP4_UTILS_H_
Generated on Thu May 13 16:36:34 2010 for Bento4 MP4 SDK by
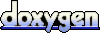