Ap4Track.h
Go to the documentation of this file.00001 /***************************************************************** 00002 | 00003 | AP4 - Track Objects 00004 | 00005 | Copyright 2002-2008 Axiomatic Systems, LLC 00006 | 00007 | 00008 | This file is part of Bento4/AP4 (MP4 Atom Processing Library). 00009 | 00010 | Unless you have obtained Bento4 under a difference license, 00011 | this version of Bento4 is Bento4|GPL. 00012 | Bento4|GPL is free software; you can redistribute it and/or modify 00013 | it under the terms of the GNU General Public License as published by 00014 | the Free Software Foundation; either version 2, or (at your option) 00015 | any later version. 00016 | 00017 | Bento4|GPL is distributed in the hope that it will be useful, 00018 | but WITHOUT ANY WARRANTY; without even the implied warranty of 00019 | MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00020 | GNU General Public License for more details. 00021 | 00022 | You should have received a copy of the GNU General Public License 00023 | along with Bento4|GPL; see the file COPYING. If not, write to the 00024 | Free Software Foundation, 59 Temple Place - Suite 330, Boston, MA 00025 | 02111-1307, USA. 00026 | 00027 ****************************************************************/ 00028 00029 #ifndef _AP4_TRAK_H_ 00030 #define _AP4_TRAK_H_ 00031 00032 /*---------------------------------------------------------------------- 00033 | includes 00034 +---------------------------------------------------------------------*/ 00035 #include "Ap4Types.h" 00036 #include "Ap4Array.h" 00037 00038 /*---------------------------------------------------------------------- 00039 | forward declarations 00040 +---------------------------------------------------------------------*/ 00041 class AP4_StblAtom; 00042 class AP4_ByteStream; 00043 class AP4_Sample; 00044 class AP4_DataBuffer; 00045 class AP4_TrakAtom; 00046 class AP4_MoovAtom; 00047 class AP4_SampleDescription; 00048 class AP4_SampleTable; 00049 00050 /*---------------------------------------------------------------------- 00051 | constants 00052 +---------------------------------------------------------------------*/ 00053 const AP4_UI32 AP4_TRACK_DEFAULT_MOVIE_TIMESCALE = 1000; 00054 00055 const AP4_UI32 AP4_TRACK_FLAG_ENABLED = 0x0001; 00056 const AP4_UI32 AP4_TRACK_FLAG_IN_MOVIE = 0x0002; 00057 const AP4_UI32 AP4_TRACK_FLAG_IN_PREVIEW = 0x0004; 00058 00059 /*---------------------------------------------------------------------- 00060 | AP4_Track 00061 +---------------------------------------------------------------------*/ 00062 class AP4_Track { 00063 public: 00064 // types 00065 typedef enum { 00066 TYPE_UNKNOWN = 0, 00067 TYPE_AUDIO = 1, 00068 TYPE_VIDEO = 2, 00069 TYPE_SYSTEM = 3, 00070 TYPE_HINT = 4, 00071 TYPE_TEXT = 5, 00072 TYPE_JPEG = 6, 00073 TYPE_RTP = 7 00074 } Type; 00075 00076 // methods 00077 AP4_Track(Type type, 00078 AP4_SampleTable* sample_table, // ownership is transfered to the AP4_Track object 00079 AP4_UI32 track_id, 00080 AP4_UI32 movie_time_scale, // 0 = use default 00081 AP4_UI64 track_duration, // in the movie timescale 00082 AP4_UI32 media_time_scale, 00083 AP4_UI64 media_duration, // in the media timescale 00084 const char* language, 00085 AP4_UI32 width, // in 16.16 fixed point 00086 AP4_UI32 height); // in 16.16 fixed point 00087 AP4_Track(AP4_TrakAtom& atom, 00088 AP4_ByteStream& sample_stream, 00089 AP4_UI32 movie_time_scale); 00090 virtual ~AP4_Track(); 00091 00097 AP4_Track* Clone(AP4_Result* result = NULL); 00098 00099 AP4_UI32 GetFlags(); 00100 AP4_Result SetFlags(AP4_UI32 flags); 00101 AP4_Track::Type GetType() { return m_Type; } 00102 AP4_UI32 GetHandlerType(); 00103 AP4_UI64 GetDuration(); // in the timescale of the movie 00104 AP4_UI32 GetDurationMs(); // in milliseconds 00105 AP4_UI32 GetWidth(); // in 16.16 fixed point 00106 AP4_UI32 GetHeight(); // in 16.16 fixed point 00107 AP4_Cardinal GetSampleCount(); 00108 AP4_Result GetSample(AP4_Ordinal index, AP4_Sample& sample); 00109 AP4_Result ReadSample(AP4_Ordinal index, 00110 AP4_Sample& sample, 00111 AP4_DataBuffer& data); 00112 AP4_Result GetSampleIndexForTimeStampMs(AP4_UI32 ts_ms, 00113 AP4_Ordinal& index); 00114 AP4_Ordinal GetNearestSyncSampleIndex(AP4_Ordinal index, bool before=true); 00115 AP4_SampleDescription* GetSampleDescription(AP4_Ordinal index); 00116 AP4_SampleTable* GetSampleTable() { return m_SampleTable; } 00117 AP4_UI32 GetId(); 00118 AP4_Result SetId(AP4_UI32 track_id); 00119 AP4_TrakAtom* GetTrakAtom() { return m_TrakAtom; } 00120 AP4_Result SetMovieTimeScale(AP4_UI32 time_scale); 00121 AP4_UI32 GetMovieTimeScale() { return m_MovieTimeScale; } 00122 AP4_UI32 GetMediaTimeScale(); 00123 AP4_UI64 GetMediaDuration(); // in the timescale of the media 00124 const char* GetTrackName(); 00125 const char* GetTrackLanguage(); 00126 AP4_Result Attach(AP4_MoovAtom* moov); 00127 00128 protected: 00129 // members 00130 AP4_TrakAtom* m_TrakAtom; 00131 bool m_TrakAtomIsOwned; 00132 Type m_Type; 00133 AP4_SampleTable* m_SampleTable; 00134 bool m_SampleTableIsOwned; 00135 AP4_UI32 m_MovieTimeScale; 00136 }; 00137 00138 #endif // _AP4_TRAK_H_
Generated on Thu May 13 16:36:34 2010 for Bento4 MP4 SDK by
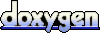