Ap4SyntheticSampleTable.h
Go to the documentation of this file.00001 /***************************************************************** 00002 | 00003 | AP4 - Synthetic Sample Table 00004 | 00005 | Copyright 2002-2008 Axiomatic Systems, LLC 00006 | 00007 | 00008 | This file is part of Bento4/AP4 (MP4 Atom Processing Library). 00009 | 00010 | Unless you have obtained Bento4 under a difference license, 00011 | this version of Bento4 is Bento4|GPL. 00012 | Bento4|GPL is free software; you can redistribute it and/or modify 00013 | it under the terms of the GNU General Public License as published by 00014 | the Free Software Foundation; either version 2, or (at your option) 00015 | any later version. 00016 | 00017 | Bento4|GPL is distributed in the hope that it will be useful, 00018 | but WITHOUT ANY WARRANTY; without even the implied warranty of 00019 | MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00020 | GNU General Public License for more details. 00021 | 00022 | You should have received a copy of the GNU General Public License 00023 | along with Bento4|GPL; see the file COPYING. If not, write to the 00024 | Free Software Foundation, 59 Temple Place - Suite 330, Boston, MA 00025 | 02111-1307, USA. 00026 | 00027 ****************************************************************/ 00028 00029 #ifndef _AP4_SYNTHETIC_SAMPLE_TABLE_H_ 00030 #define _AP4_SYNTHETIC_SAMPLE_TABLE_H_ 00031 00032 /*---------------------------------------------------------------------- 00033 | includes 00034 +---------------------------------------------------------------------*/ 00035 #include "Ap4Array.h" 00036 #include "Ap4List.h" 00037 #include "Ap4Sample.h" 00038 #include "Ap4SampleTable.h" 00039 #include "Ap4SampleDescription.h" 00040 00041 /*---------------------------------------------------------------------- 00042 | forward declarations 00043 +---------------------------------------------------------------------*/ 00044 class AP4_ByteStream; 00045 00046 /*---------------------------------------------------------------------- 00047 | constants 00048 +---------------------------------------------------------------------*/ 00049 const AP4_Cardinal AP4_SYNTHETIC_SAMPLE_TABLE_DEFAULT_CHUNK_SIZE = 10; 00050 00051 /*---------------------------------------------------------------------- 00052 | AP4_SyntheticSampleTable 00053 +---------------------------------------------------------------------*/ 00054 class AP4_SyntheticSampleTable : public AP4_SampleTable 00055 { 00056 public: 00057 // methods 00058 AP4_SyntheticSampleTable(AP4_Cardinal chunk_size 00059 = AP4_SYNTHETIC_SAMPLE_TABLE_DEFAULT_CHUNK_SIZE); 00060 virtual ~AP4_SyntheticSampleTable(); 00061 00062 // AP4_SampleTable methods 00063 virtual AP4_Result GetSample(AP4_Ordinal index, AP4_Sample& sample); 00064 virtual AP4_Cardinal GetSampleCount(); 00065 virtual AP4_Result GetSampleChunkPosition(AP4_Ordinal sample_index, 00066 AP4_Ordinal& chunk_index, 00067 AP4_Ordinal& position_in_chunk); 00068 virtual AP4_Cardinal GetSampleDescriptionCount(); 00069 virtual AP4_SampleDescription* GetSampleDescription(AP4_Ordinal index); 00070 virtual AP4_Result GetSampleIndexForTimeStamp(AP4_UI64 ts, AP4_Ordinal& index); 00071 virtual AP4_Ordinal GetNearestSyncSampleIndex(AP4_Ordinal index, bool before); 00072 00073 // methods 00086 virtual AP4_Result AddSampleDescription(AP4_SampleDescription* description, 00087 bool transfer_ownership=true); 00088 00113 virtual AP4_Result AddSample(AP4_ByteStream& data_stream, 00114 AP4_Position offset, 00115 AP4_Size size, 00116 AP4_UI32 duration, 00117 AP4_Ordinal description_index, 00118 AP4_UI64 dts, 00119 AP4_UI32 cts_delta, 00120 bool sync); 00121 00122 private: 00123 // classes 00124 class SampleDescriptionHolder { 00125 public: 00126 SampleDescriptionHolder(AP4_SampleDescription* description, bool is_owned) : 00127 m_SampleDescription(description), m_IsOwned(is_owned) {} 00128 ~SampleDescriptionHolder() { if (m_IsOwned) delete m_SampleDescription; } 00129 AP4_SampleDescription* m_SampleDescription; 00130 bool m_IsOwned; 00131 }; 00132 00133 // members 00134 AP4_Array<AP4_Sample> m_Samples; 00135 AP4_List<SampleDescriptionHolder> m_SampleDescriptions; 00136 AP4_Cardinal m_ChunkSize; 00137 AP4_Array<AP4_UI32> m_SamplesInChunk; 00138 struct { 00139 AP4_Ordinal m_Sample; 00140 AP4_Ordinal m_Chunk; 00141 } m_LookupCache; 00142 }; 00143 00144 #endif // _AP4_SYNTHETIC_SAMPLE_TABLE_H_
Generated on Thu May 13 16:36:33 2010 for Bento4 MP4 SDK by
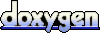