Ap4StscAtom.h
Go to the documentation of this file.00001 /***************************************************************** 00002 | 00003 | AP4 - stsc Atoms 00004 | 00005 | Copyright 2002-2008 Axiomatic Systems, LLC 00006 | 00007 | 00008 | This file is part of Bento4/AP4 (MP4 Atom Processing Library). 00009 | 00010 | Unless you have obtained Bento4 under a difference license, 00011 | this version of Bento4 is Bento4|GPL. 00012 | Bento4|GPL is free software; you can redistribute it and/or modify 00013 | it under the terms of the GNU General Public License as published by 00014 | the Free Software Foundation; either version 2, or (at your option) 00015 | any later version. 00016 | 00017 | Bento4|GPL is distributed in the hope that it will be useful, 00018 | but WITHOUT ANY WARRANTY; without even the implied warranty of 00019 | MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00020 | GNU General Public License for more details. 00021 | 00022 | You should have received a copy of the GNU General Public License 00023 | along with Bento4|GPL; see the file COPYING. If not, write to the 00024 | Free Software Foundation, 59 Temple Place - Suite 330, Boston, MA 00025 | 02111-1307, USA. 00026 | 00027 ****************************************************************/ 00028 00029 #ifndef _AP4_STSC_ATOM_H_ 00030 #define _AP4_STSC_ATOM_H_ 00031 00032 /*---------------------------------------------------------------------- 00033 | includes 00034 +---------------------------------------------------------------------*/ 00035 #include "Ap4Types.h" 00036 #include "Ap4Atom.h" 00037 #include "Ap4Array.h" 00038 00039 /*---------------------------------------------------------------------- 00040 | AP4_StscTableEntry 00041 +---------------------------------------------------------------------*/ 00042 class AP4_StscTableEntry { 00043 public: 00044 AP4_StscTableEntry() : 00045 m_FirstChunk(0), 00046 m_FirstSample(0), 00047 m_ChunkCount(0), 00048 m_SamplesPerChunk(0), 00049 m_SampleDescriptionIndex(0) {} 00050 AP4_StscTableEntry(AP4_Ordinal first_chunk, 00051 AP4_Ordinal first_sample, 00052 AP4_Cardinal samples_per_chunk, 00053 AP4_Ordinal sample_description_index) : 00054 m_FirstChunk(first_chunk), 00055 m_FirstSample(first_sample), 00056 m_ChunkCount(0), 00057 m_SamplesPerChunk(samples_per_chunk), 00058 m_SampleDescriptionIndex(sample_description_index) {} 00059 AP4_StscTableEntry(AP4_Ordinal first_chunk, 00060 AP4_Ordinal first_sample, 00061 AP4_Cardinal chunk_count, 00062 AP4_Cardinal samples_per_chunk, 00063 AP4_Ordinal sample_description_index) : 00064 m_FirstChunk(first_chunk), 00065 m_FirstSample(first_sample), 00066 m_ChunkCount(chunk_count), 00067 m_SamplesPerChunk(samples_per_chunk), 00068 m_SampleDescriptionIndex(sample_description_index) {} 00069 AP4_Ordinal m_FirstChunk; 00070 AP4_Ordinal m_FirstSample; // computed (not in file) 00071 AP4_Cardinal m_ChunkCount; // computed (not in file) 00072 AP4_Cardinal m_SamplesPerChunk; 00073 AP4_Ordinal m_SampleDescriptionIndex; 00074 }; 00075 00076 /*---------------------------------------------------------------------- 00077 | AP4_StscAtom 00078 +---------------------------------------------------------------------*/ 00079 class AP4_StscAtom : public AP4_Atom 00080 { 00081 public: 00082 AP4_IMPLEMENT_DYNAMIC_CAST_D(AP4_StscAtom, AP4_Atom) 00083 00084 // class methods 00085 static AP4_StscAtom* Create(AP4_Size size, AP4_ByteStream& stream); 00086 00087 // methods 00088 AP4_StscAtom(); 00089 virtual AP4_Result InspectFields(AP4_AtomInspector& inspector); 00090 virtual AP4_Result GetChunkForSample(AP4_Ordinal sample, 00091 AP4_Ordinal& chunk, 00092 AP4_Ordinal& skip, 00093 AP4_Ordinal& sample_description_index); 00094 virtual AP4_Result AddEntry(AP4_Cardinal chunk_count, 00095 AP4_Cardinal samples_per_chunk, 00096 AP4_Ordinal sample_description_index); 00097 virtual AP4_Result WriteFields(AP4_ByteStream& stream); 00098 00099 private: 00100 // methods 00101 AP4_StscAtom(AP4_UI32 size, 00102 AP4_UI32 version, 00103 AP4_UI32 flags, 00104 AP4_ByteStream& stream); 00105 00106 // members 00107 AP4_Array<AP4_StscTableEntry> m_Entries; 00108 AP4_Ordinal m_CachedChunkGroup; 00109 }; 00110 00111 #endif // _AP4_STSC_ATOM_H_
Generated on Thu May 13 16:36:33 2010 for Bento4 MP4 SDK by
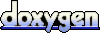