Ap4StreamCipher.h
Go to the documentation of this file.00001 /***************************************************************** 00002 | 00003 | AP4 - Stream Cipher 00004 | 00005 | Copyright 2002-2008 Axiomatic Systems, LLC 00006 | 00007 | 00008 | This file is part of Bento4/AP4 (MP4 Atom Processing Library). 00009 | 00010 | Unless you have obtained Bento4 under a difference license, 00011 | this version of Bento4 is Bento4|GPL. 00012 | Bento4|GPL is free software; you can redistribute it and/or modify 00013 | it under the terms of the GNU General Public License as published by 00014 | the Free Software Foundation; either version 2, or (at your option) 00015 | any later version. 00016 | 00017 | Bento4|GPL is distributed in the hope that it will be useful, 00018 | but WITHOUT ANY WARRANTY; without even the implied warranty of 00019 | MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00020 | GNU General Public License for more details. 00021 | 00022 | You should have received a copy of the GNU General Public License 00023 | along with Bento4|GPL; see the file COPYING. If not, write to the 00024 | Free Software Foundation, 59 Temple Place - Suite 330, Boston, MA 00025 | 02111-1307, USA. 00026 | 00027 ****************************************************************/ 00028 00029 #ifndef _AP4_STREAM_CIPHER_H_ 00030 #define _AP4_STREAM_CIPHER_H_ 00031 00032 /*---------------------------------------------------------------------- 00033 | includes 00034 +---------------------------------------------------------------------*/ 00035 #include "Ap4Protection.h" 00036 #include "Ap4Results.h" 00037 #include "Ap4Types.h" 00038 00039 /*---------------------------------------------------------------------- 00040 | constants 00041 +---------------------------------------------------------------------*/ 00042 // we only support this for now 00043 const unsigned int AP4_CIPHER_BLOCK_SIZE = 16; 00044 00045 /*---------------------------------------------------------------------- 00046 | AP4_StreamCipher interface 00047 +---------------------------------------------------------------------*/ 00048 class AP4_StreamCipher 00049 { 00050 public: 00051 // types 00052 typedef enum { 00053 ENCRYPT, 00054 DECRYPT 00055 } CipherDirection; 00056 00057 // methods 00058 virtual ~AP4_StreamCipher() {} 00059 00060 virtual AP4_UI64 GetStreamOffset() = 0; 00061 00062 virtual AP4_Result ProcessBuffer(const AP4_UI08* in, 00063 AP4_Size in_size, 00064 AP4_UI08* out, 00065 AP4_Size* out_size, 00066 bool is_last_buffer = false) = 0; 00067 00068 // preroll gives the number of bytes you have to preroll your input and feed 00069 // it through ProcessBuffer (in one shot) in order to be able to spit out 00070 // the output at the given offset 00071 virtual AP4_Result SetStreamOffset(AP4_UI64 offset, 00072 AP4_Cardinal* preroll) = 0; 00073 00074 virtual AP4_Result SetIV(const AP4_UI08* iv) = 0; 00075 virtual const AP4_UI08* GetIV() = 0; 00076 }; 00077 00078 00079 /*---------------------------------------------------------------------- 00080 | AP4_CtrStreamCipher 00081 +---------------------------------------------------------------------*/ 00082 class AP4_CtrStreamCipher : public AP4_StreamCipher 00083 { 00084 public: 00085 // methods 00086 00091 AP4_CtrStreamCipher(AP4_BlockCipher* block_cipher, 00092 const AP4_UI08* salt, 00093 AP4_Size counter_size); 00094 ~AP4_CtrStreamCipher(); 00095 00096 // AP4_StreamCipher implementation 00097 virtual AP4_Result SetStreamOffset(AP4_UI64 offset, 00098 AP4_Cardinal* preroll = NULL); 00099 virtual AP4_UI64 GetStreamOffset() { return m_StreamOffset; } 00100 virtual AP4_Result ProcessBuffer(const AP4_UI08* in, 00101 AP4_Size in_size, 00102 AP4_UI08* out, 00103 AP4_Size* out_size = NULL, 00104 bool is_last_buffer = false); 00105 00106 virtual AP4_Result SetIV(const AP4_UI08* iv); 00107 virtual const AP4_UI08* GetIV() { return m_BaseCounter; } 00108 00109 private: 00110 // methods 00111 void SetCounterOffset(AP4_UI32 offset); 00112 void UpdateKeyStream(); 00113 00114 // members 00115 AP4_UI64 m_StreamOffset; 00116 AP4_Size m_CounterSize; 00117 AP4_UI08 m_BaseCounter[AP4_CIPHER_BLOCK_SIZE]; 00118 AP4_UI08 m_XBlock[AP4_CIPHER_BLOCK_SIZE]; 00119 AP4_BlockCipher* m_BlockCipher; 00120 }; 00121 00122 /*---------------------------------------------------------------------- 00123 | AP4_CbcStreamCipher 00124 +---------------------------------------------------------------------*/ 00125 class AP4_CbcStreamCipher : public AP4_StreamCipher 00126 { 00127 public: 00128 // methods 00129 00134 AP4_CbcStreamCipher(AP4_BlockCipher* block_cipher, CipherDirection direction); 00135 ~AP4_CbcStreamCipher(); 00136 00137 // AP4_StreamCipher implementation 00138 virtual AP4_Result SetStreamOffset(AP4_UI64 offset, 00139 AP4_Cardinal* preroll); 00140 virtual AP4_UI64 GetStreamOffset() { return m_StreamOffset; } 00141 virtual AP4_Result ProcessBuffer(const AP4_UI08* in, 00142 AP4_Size in_size, 00143 AP4_UI08* out, 00144 AP4_Size* out_size, 00145 bool is_last_buffer = false); 00146 virtual AP4_Result SetIV(const AP4_UI08* iv); 00147 virtual const AP4_UI08* GetIV() { return m_Iv; }; 00148 00149 private: 00150 // members 00151 CipherDirection m_Direction; 00152 AP4_UI64 m_StreamOffset; 00153 AP4_Size m_OutputSkip; 00154 AP4_UI08 m_InBlockCache[AP4_CIPHER_BLOCK_SIZE]; 00155 AP4_UI08 m_OutBlockCache[AP4_CIPHER_BLOCK_SIZE]; 00156 AP4_UI08 m_Iv[AP4_CIPHER_BLOCK_SIZE]; 00157 AP4_BlockCipher* m_BlockCipher; 00158 bool m_Eos; 00159 AP4_Cardinal m_PrerollByteCount; 00160 }; 00161 00162 #endif // _AP4_STREAM_CIPHER_H_
Generated on Thu May 13 16:36:33 2010 for Bento4 MP4 SDK by
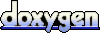