Ap4SampleEntry.h
Go to the documentation of this file.00001 /***************************************************************** 00002 | 00003 | AP4 - sample entries 00004 | 00005 | Copyright 2002-2008 Axiomatic Systems, LLC 00006 | 00007 | 00008 | This file is part of Bento4/AP4 (MP4 Atom Processing Library). 00009 | 00010 | Unless you have obtained Bento4 under a difference license, 00011 | this version of Bento4 is Bento4|GPL. 00012 | Bento4|GPL is free software; you can redistribute it and/or modify 00013 | it under the terms of the GNU General Public License as published by 00014 | the Free Software Foundation; either version 2, or (at your option) 00015 | any later version. 00016 | 00017 | Bento4|GPL is distributed in the hope that it will be useful, 00018 | but WITHOUT ANY WARRANTY; without even the implied warranty of 00019 | MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00020 | GNU General Public License for more details. 00021 | 00022 | You should have received a copy of the GNU General Public License 00023 | along with Bento4|GPL; see the file COPYING. If not, write to the 00024 | Free Software Foundation, 59 Temple Place - Suite 330, Boston, MA 00025 | 02111-1307, USA. 00026 | 00027 ****************************************************************/ 00028 00029 #ifndef _AP4_SAMPLE_ENTRY_H_ 00030 #define _AP4_SAMPLE_ENTRY_H_ 00031 00032 /*---------------------------------------------------------------------- 00033 | includes 00034 +---------------------------------------------------------------------*/ 00035 #include "Ap4Types.h" 00036 #include "Ap4List.h" 00037 #include "Ap4Atom.h" 00038 #include "Ap4EsdsAtom.h" 00039 #include "Ap4AtomFactory.h" 00040 #include "Ap4ContainerAtom.h" 00041 00042 /*---------------------------------------------------------------------- 00043 | class references 00044 +---------------------------------------------------------------------*/ 00045 class AP4_SampleDescription; 00046 class AP4_AvccAtom; 00047 00048 /*---------------------------------------------------------------------- 00049 | AP4_SampleEntry 00050 +---------------------------------------------------------------------*/ 00051 class AP4_SampleEntry : public AP4_ContainerAtom 00052 { 00053 public: 00054 AP4_IMPLEMENT_DYNAMIC_CAST_D(AP4_SampleEntry, AP4_ContainerAtom) 00055 00056 // methods 00057 AP4_SampleEntry(AP4_Atom::Type format, AP4_UI16 data_ref_index = 1); 00058 AP4_SampleEntry(AP4_Atom::Type format, 00059 AP4_Size size, 00060 AP4_ByteStream& stream, 00061 AP4_AtomFactory& atom_factory); 00062 virtual ~AP4_SampleEntry() {} 00063 00064 AP4_UI16 GetDataReferenceIndex() { return m_DataReferenceIndex; } 00065 virtual AP4_Result Write(AP4_ByteStream& stream); 00066 virtual AP4_Result Inspect(AP4_AtomInspector& inspector); 00067 virtual AP4_SampleDescription* ToSampleDescription(); 00068 00069 // AP4_AtomParent methods 00070 virtual void OnChildChanged(AP4_Atom* child); 00071 00072 protected: 00073 // constructor 00074 AP4_SampleEntry(AP4_Atom::Type format, AP4_Size size); 00075 00076 // methods 00077 virtual void Read(AP4_ByteStream& stream, AP4_AtomFactory& atom_factory); 00078 virtual AP4_Size GetFieldsSize(); 00079 virtual AP4_Result ReadFields(AP4_ByteStream& stream); 00080 virtual AP4_Result WriteFields(AP4_ByteStream& stream); 00081 virtual AP4_Result InspectFields(AP4_AtomInspector& inspector); 00082 00083 // members 00084 AP4_UI08 m_Reserved1[6]; // = 0 00085 AP4_UI16 m_DataReferenceIndex; 00086 }; 00087 00088 /*---------------------------------------------------------------------- 00089 | AP4_UnknownSampleEntry 00090 +---------------------------------------------------------------------*/ 00091 class AP4_UnknownSampleEntry : public AP4_SampleEntry 00092 { 00093 public: 00094 // this constructor takes ownership of the atom passed to it 00095 AP4_UnknownSampleEntry(AP4_Atom::Type type, AP4_Size size, AP4_ByteStream& stream); 00096 00097 // AP4_SampleEntry methods 00098 virtual AP4_SampleDescription* ToSampleDescription(); 00099 00100 // accessors 00101 const AP4_DataBuffer& GetPayload() { return m_Payload; } 00102 00103 protected: 00104 // methods 00105 virtual AP4_Size GetFieldsSize(); 00106 virtual AP4_Result ReadFields(AP4_ByteStream& stream); 00107 virtual AP4_Result WriteFields(AP4_ByteStream& stream); 00108 00109 // members 00110 AP4_DataBuffer m_Payload; 00111 }; 00112 00113 /*---------------------------------------------------------------------- 00114 | AP4_AudioSampleEntry 00115 +---------------------------------------------------------------------*/ 00116 class AP4_AudioSampleEntry : public AP4_SampleEntry 00117 { 00118 public: 00119 // methods 00120 AP4_AudioSampleEntry(AP4_Atom::Type format, 00121 AP4_UI32 sample_rate, 00122 AP4_UI16 sample_size, 00123 AP4_UI16 channel_count); 00124 AP4_AudioSampleEntry(AP4_Atom::Type format, 00125 AP4_Size size, 00126 AP4_ByteStream& stream, 00127 AP4_AtomFactory& atom_factory); 00128 00129 // accessors 00130 AP4_UI32 GetSampleRate(); 00131 AP4_UI16 GetSampleSize() { return m_SampleSize; } 00132 AP4_UI16 GetChannelCount(); 00133 00134 // methods 00135 AP4_SampleDescription* ToSampleDescription(); 00136 00137 protected: 00138 // methods 00139 virtual AP4_Size GetFieldsSize(); 00140 virtual AP4_Result ReadFields(AP4_ByteStream& stream); 00141 virtual AP4_Result WriteFields(AP4_ByteStream& stream); 00142 virtual AP4_Result InspectFields(AP4_AtomInspector& inspector); 00143 00144 // members 00145 AP4_UI16 m_QtVersion; // 0, 1 or 2 00146 AP4_UI16 m_QtRevision; // 0 00147 AP4_UI32 m_QtVendor; // 0 00148 AP4_UI16 m_ChannelCount; 00149 AP4_UI16 m_SampleSize; 00150 AP4_UI16 m_QtCompressionId; // 0 or -2 00151 AP4_UI16 m_QtPacketSize; // always 0 00152 AP4_UI32 m_SampleRate; // 16.16 fixed point 00153 00154 AP4_UI32 m_QtV1SamplesPerPacket; 00155 AP4_UI32 m_QtV1BytesPerPacket; 00156 AP4_UI32 m_QtV1BytesPerFrame; 00157 AP4_UI32 m_QtV1BytesPerSample; 00158 00159 AP4_UI32 m_QtV2StructSize; 00160 double m_QtV2SampleRate64; 00161 AP4_UI32 m_QtV2ChannelCount; 00162 AP4_UI32 m_QtV2Reserved; 00163 AP4_UI32 m_QtV2BitsPerChannel; 00164 AP4_UI32 m_QtV2FormatSpecificFlags; 00165 AP4_UI32 m_QtV2BytesPerAudioPacket; 00166 AP4_UI32 m_QtV2LPCMFramesPerAudioPacket; 00167 AP4_DataBuffer m_QtV2Extension; 00168 }; 00169 00170 /*---------------------------------------------------------------------- 00171 | AP4_VisualSampleEntry 00172 +---------------------------------------------------------------------*/ 00173 class AP4_VisualSampleEntry : public AP4_SampleEntry 00174 { 00175 public: 00176 // methods 00177 AP4_VisualSampleEntry(AP4_Atom::Type format, 00178 AP4_UI16 width, 00179 AP4_UI16 height, 00180 AP4_UI16 depth, 00181 const char* compressor_name); 00182 AP4_VisualSampleEntry(AP4_Atom::Type format, 00183 AP4_Size size, 00184 AP4_ByteStream& stream, 00185 AP4_AtomFactory& atom_factory); 00186 00187 // accessors 00188 AP4_UI16 GetWidth() { return m_Width; } 00189 AP4_UI16 GetHeight() { return m_Height; } 00190 AP4_UI16 GetHorizResolution(){ return m_HorizResolution; } 00191 AP4_UI16 GetVertResolution() { return m_VertResolution; } 00192 AP4_UI16 GetDepth() { return m_Depth; } 00193 const char* GetCompressorName() { return m_CompressorName.GetChars(); } 00194 00195 // methods 00196 AP4_SampleDescription* ToSampleDescription(); 00197 00198 protected: 00199 // methods 00200 virtual AP4_Size GetFieldsSize(); 00201 virtual AP4_Result ReadFields(AP4_ByteStream& stream); 00202 virtual AP4_Result WriteFields(AP4_ByteStream& stream); 00203 virtual AP4_Result InspectFields(AP4_AtomInspector& inspector); 00204 00205 //members 00206 AP4_UI16 m_Predefined1; // = 0 00207 AP4_UI16 m_Reserved2; // = 0 00208 AP4_UI08 m_Predefined2[12]; // = 0 00209 AP4_UI16 m_Width; 00210 AP4_UI16 m_Height; 00211 AP4_UI32 m_HorizResolution; // = 0x00480000 (72 dpi) 00212 AP4_UI32 m_VertResolution; // = 0x00480000 (72 dpi) 00213 AP4_UI32 m_Reserved3; // = 0 00214 AP4_UI16 m_FrameCount; // = 1 00215 AP4_String m_CompressorName; 00216 AP4_UI16 m_Depth; // = 0x0018 00217 AP4_UI16 m_Predefined3; // = 0xFFFF 00218 }; 00219 00220 /*---------------------------------------------------------------------- 00221 | AP4_MpegSystemSampleEntry 00222 +---------------------------------------------------------------------*/ 00223 class AP4_MpegSystemSampleEntry : public AP4_SampleEntry 00224 { 00225 public: 00226 // constructors 00227 AP4_MpegSystemSampleEntry(AP4_UI32 type, 00228 AP4_EsDescriptor* descriptor); 00229 AP4_MpegSystemSampleEntry(AP4_UI32 type, 00230 AP4_Size size, 00231 AP4_ByteStream& stream, 00232 AP4_AtomFactory& atom_factory); 00233 00234 // methods 00235 AP4_SampleDescription* ToSampleDescription(); 00236 }; 00237 00238 /*---------------------------------------------------------------------- 00239 | AP4_MpegAudioSampleEntry 00240 +---------------------------------------------------------------------*/ 00241 class AP4_MpegAudioSampleEntry : public AP4_AudioSampleEntry 00242 { 00243 public: 00244 // constructors 00245 AP4_MpegAudioSampleEntry(AP4_UI32 type, 00246 AP4_UI32 sample_rate, 00247 AP4_UI16 sample_size, 00248 AP4_UI16 channel_count, 00249 AP4_EsDescriptor* descriptor); 00250 AP4_MpegAudioSampleEntry(AP4_UI32 type, 00251 AP4_Size size, 00252 AP4_ByteStream& stream, 00253 AP4_AtomFactory& atom_factory); 00254 00255 // methods 00256 AP4_SampleDescription* ToSampleDescription(); 00257 }; 00258 00259 /*---------------------------------------------------------------------- 00260 | AP4_MpegVideoSampleEntry 00261 +---------------------------------------------------------------------*/ 00262 class AP4_MpegVideoSampleEntry : public AP4_VisualSampleEntry 00263 { 00264 public: 00265 // constructors 00266 AP4_MpegVideoSampleEntry(AP4_UI32 type, 00267 AP4_UI16 width, 00268 AP4_UI16 height, 00269 AP4_UI16 depth, 00270 const char* compressor_name, 00271 AP4_EsDescriptor* descriptor); 00272 AP4_MpegVideoSampleEntry(AP4_UI32 type, 00273 AP4_Size size, 00274 AP4_ByteStream& stream, 00275 AP4_AtomFactory& atom_factory); 00276 00277 // methods 00278 AP4_SampleDescription* ToSampleDescription(); 00279 }; 00280 00281 /*---------------------------------------------------------------------- 00282 | AP4_Mp4sSampleEntry 00283 +---------------------------------------------------------------------*/ 00284 class AP4_Mp4sSampleEntry : public AP4_MpegSystemSampleEntry 00285 { 00286 public: 00287 // constructors 00288 AP4_Mp4sSampleEntry(AP4_Size size, 00289 AP4_ByteStream& stream, 00290 AP4_AtomFactory& atom_factory); 00291 AP4_Mp4sSampleEntry(AP4_EsDescriptor* descriptor); 00292 00293 // methods 00294 AP4_SampleDescription* ToSampleDescription(); 00295 }; 00296 00297 /*---------------------------------------------------------------------- 00298 | AP4_Mp4aSampleEntry 00299 +---------------------------------------------------------------------*/ 00300 class AP4_Mp4aSampleEntry : public AP4_MpegAudioSampleEntry 00301 { 00302 public: 00303 // constructors 00304 AP4_Mp4aSampleEntry(AP4_Size size, 00305 AP4_ByteStream& stream, 00306 AP4_AtomFactory& atom_factory); 00307 AP4_Mp4aSampleEntry(AP4_UI32 sample_rate, 00308 AP4_UI16 sample_size, 00309 AP4_UI16 channel_count, 00310 AP4_EsDescriptor* descriptor); 00311 }; 00312 00313 /*---------------------------------------------------------------------- 00314 | AP4_Mp4vSampleEntry 00315 +---------------------------------------------------------------------*/ 00316 class AP4_Mp4vSampleEntry : public AP4_MpegVideoSampleEntry 00317 { 00318 public: 00319 // constructors 00320 AP4_Mp4vSampleEntry(AP4_Size size, 00321 AP4_ByteStream& stream, 00322 AP4_AtomFactory& atom_factory); 00323 AP4_Mp4vSampleEntry(AP4_UI16 width, 00324 AP4_UI16 height, 00325 AP4_UI16 depth, 00326 const char* compressor_name, 00327 AP4_EsDescriptor* descriptor); 00328 }; 00329 00330 /*---------------------------------------------------------------------- 00331 | AP4_Avc1SampleEntry 00332 +---------------------------------------------------------------------*/ 00333 class AP4_Avc1SampleEntry : public AP4_VisualSampleEntry 00334 { 00335 public: 00336 // constructors 00337 AP4_Avc1SampleEntry(AP4_Size size, 00338 AP4_ByteStream& stream, 00339 AP4_AtomFactory& atom_factory); 00340 AP4_Avc1SampleEntry(AP4_UI16 width, 00341 AP4_UI16 height, 00342 AP4_UI16 depth, 00343 const char* compressor_name, 00344 const AP4_AvccAtom& avcc); 00345 00346 // inherited from AP4_SampleEntry 00347 virtual AP4_SampleDescription* ToSampleDescription(); 00348 }; 00349 00350 /*---------------------------------------------------------------------- 00351 | AP4_RtpHintSampleEntry 00352 +---------------------------------------------------------------------*/ 00353 class AP4_RtpHintSampleEntry : public AP4_SampleEntry 00354 { 00355 public: 00356 // methods 00357 AP4_RtpHintSampleEntry(AP4_UI16 hint_track_version, 00358 AP4_UI16 highest_compatible_version, 00359 AP4_UI32 max_packet_size, 00360 AP4_UI32 timescale); 00361 AP4_RtpHintSampleEntry(AP4_Size size, 00362 AP4_ByteStream& stream, 00363 AP4_AtomFactory& atom_factory); 00364 00365 protected: 00366 // methods 00367 virtual AP4_Size GetFieldsSize(); 00368 virtual AP4_Result ReadFields(AP4_ByteStream& stream); 00369 virtual AP4_Result WriteFields(AP4_ByteStream& stream); 00370 virtual AP4_Result InspectFields(AP4_AtomInspector& inspector); 00371 00372 // members 00373 AP4_UI16 m_HintTrackVersion; 00374 AP4_UI16 m_HighestCompatibleVersion; 00375 AP4_UI32 m_MaxPacketSize; 00376 }; 00377 00378 #endif // _AP4_SAMPLE_ENTRY_H_
Generated on Thu May 13 16:36:33 2010 for Bento4 MP4 SDK by
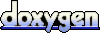