Ap4SampleDescription.h
Go to the documentation of this file.00001 /***************************************************************** 00002 | 00003 | AP4 - Sample Descriptions 00004 | 00005 | Copyright 2002-2008 Axiomatic Systems, LLC 00006 | 00007 | 00008 | This file is part of Bento4/AP4 (MP4 Atom Processing Library). 00009 | 00010 | Unless you have obtained Bento4 under a difference license, 00011 | this version of Bento4 is Bento4|GPL. 00012 | Bento4|GPL is free software; you can redistribute it and/or modify 00013 | it under the terms of the GNU General Public License as published by 00014 | the Free Software Foundation; either version 2, or (at your option) 00015 | any later version. 00016 | 00017 | Bento4|GPL is distributed in the hope that it will be useful, 00018 | but WITHOUT ANY WARRANTY; without even the implied warranty of 00019 | MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00020 | GNU General Public License for more details. 00021 | 00022 | You should have received a copy of the GNU General Public License 00023 | along with Bento4|GPL; see the file COPYING. If not, write to the 00024 | Free Software Foundation, 59 Temple Place - Suite 330, Boston, MA 00025 | 02111-1307, USA. 00026 | 00027 ****************************************************************/ 00028 00029 #ifndef _AP4_SAMPLE_DESCRIPTION_H_ 00030 #define _AP4_SAMPLE_DESCRIPTION_H_ 00031 00032 /*---------------------------------------------------------------------- 00033 | includes 00034 +---------------------------------------------------------------------*/ 00035 #include "Ap4Types.h" 00036 #include "Ap4Atom.h" 00037 #include "Ap4EsDescriptor.h" 00038 #include "Ap4EsdsAtom.h" 00039 #include "Ap4Array.h" 00040 #include "Ap4AvccAtom.h" 00041 #include "Ap4DynamicCast.h" 00042 00043 /*---------------------------------------------------------------------- 00044 | class references 00045 +---------------------------------------------------------------------*/ 00046 class AP4_SampleEntry; 00047 class AP4_DataBuffer; 00048 00049 /*---------------------------------------------------------------------- 00050 | constants 00051 +---------------------------------------------------------------------*/ 00052 #define AP4_SAMPLE_FORMAT_MP4A AP4_ATOM_TYPE('m','p','4','a') 00053 #define AP4_SAMPLE_FORMAT_MP4V AP4_ATOM_TYPE('m','p','4','v') 00054 #define AP4_SAMPLE_FORMAT_MP4S AP4_ATOM_TYPE('m','p','4','s') 00055 #define AP4_SAMPLE_FORMAT_AVC1 AP4_ATOM_TYPE('a','v','c','1') 00056 #define AP4_SAMPLE_FORMAT_ALAC AP4_ATOM_TYPE('a','l','a','c') 00057 #define AP4_SAMPLE_FORMAT_OWMA AP4_ATOM_TYPE('o','w','m','a') 00058 #define AP4_SAMPLE_FORMAT_OVC1 AP4_ATOM_TYPE('o','v','c','1') 00059 #define AP4_SAMPLE_FORMAT_AVCP AP4_ATOM_TYPE('a','v','c','p') 00060 #define AP4_SAMPLE_FORMAT_DRAC AP4_ATOM_TYPE('d','r','a','c') 00061 #define AP4_SAMPLE_FORMAT_DRA1 AP4_ATOM_TYPE('d','r','a','1') 00062 #define AP4_SAMPLE_FORMAT_AC_3 AP4_ATOM_TYPE('a','c','-','3') 00063 #define AP4_SAMPLE_FORMAT_EC_3 AP4_ATOM_TYPE('e','c','-','3') 00064 #define AP4_SAMPLE_FORMAT_G726 AP4_ATOM_TYPE('g','7','2','6') 00065 #define AP4_SAMPLE_FORMAT_MJP2 AP4_ATOM_TYPE('m','j','p','2') 00066 #define AP4_SAMPLE_FORMAT_OKSD AP4_ATOM_TYPE('o','k','s','d') 00067 #define AP4_SAMPLE_FORMAT_RAW_ AP4_ATOM_TYPE('r','a','w',' ') 00068 #define AP4_SAMPLE_FORMAT_RTP_ AP4_ATOM_TYPE('r','t','p',' ') 00069 #define AP4_SAMPLE_FORMAT_S263 AP4_ATOM_TYPE('s','2','6','3') 00070 #define AP4_SAMPLE_FORMAT_SAMR AP4_ATOM_TYPE('s','a','m','r') 00071 #define AP4_SAMPLE_FORMAT_SAWB AP4_ATOM_TYPE('s','a','w','b') 00072 #define AP4_SAMPLE_FORMAT_SAWP AP4_ATOM_TYPE('s','a','w','p') 00073 #define AP4_SAMPLE_FORMAT_SEVC AP4_ATOM_TYPE('s','e','v','c') 00074 #define AP4_SAMPLE_FORMAT_SQCP AP4_ATOM_TYPE('s','q','c','p') 00075 #define AP4_SAMPLE_FORMAT_SRTP AP4_ATOM_TYPE('s','r','t','p') 00076 #define AP4_SAMPLE_FORMAT_SSMV AP4_ATOM_TYPE('s','s','m','v') 00077 #define AP4_SAMPLE_FORMAT_TEXT AP4_ATOM_TYPE('t','e','t','x') 00078 #define AP4_SAMPLE_FORMAT_TWOS AP4_ATOM_TYPE('t','w','o','s') 00079 #define AP4_SAMPLE_FORMAT_TX3G AP4_ATOM_TYPE('t','x','3','g') 00080 #define AP4_SAMPLE_FORMAT_VC_1 AP4_ATOM_TYPE('v','c','-','1') 00081 #define AP4_SAMPLE_FORMAT_XML_ AP4_ATOM_TYPE('x','m','l',' ') 00082 00083 const char* 00084 AP4_GetFormatName(AP4_UI32 format); 00085 00086 /*---------------------------------------------------------------------- 00087 | AP4_SampleDescription 00088 +---------------------------------------------------------------------*/ 00089 class AP4_SampleDescription 00090 { 00091 public: 00092 AP4_IMPLEMENT_DYNAMIC_CAST(AP4_SampleDescription) 00093 00094 // type constants of the sample description 00095 enum Type { 00096 TYPE_UNKNOWN = 0x00, 00097 TYPE_MPEG = 0x01, 00098 TYPE_PROTECTED = 0x02, 00099 TYPE_AVC = 0x03 00100 }; 00101 00102 // constructors & destructor 00103 AP4_SampleDescription(Type type, 00104 AP4_UI32 format, 00105 AP4_AtomParent* details); 00106 virtual ~AP4_SampleDescription() {} 00107 virtual AP4_SampleDescription* Clone(AP4_Result* result = NULL); 00108 00109 // accessors 00110 Type GetType() const { return m_Type; } 00111 AP4_UI32 GetFormat() const { return m_Format; } 00112 AP4_AtomParent& GetDetails() { return m_Details; } 00113 00114 // factories 00115 virtual AP4_Atom* ToAtom() const; 00116 00117 protected: 00118 Type m_Type; 00119 AP4_UI32 m_Format; 00120 AP4_AtomParent m_Details; 00121 }; 00122 00123 /*---------------------------------------------------------------------- 00124 | AP4_UnknownSampleDescription 00125 +---------------------------------------------------------------------*/ 00126 class AP4_UnknownSampleDescription : public AP4_SampleDescription 00127 { 00128 public: 00129 AP4_IMPLEMENT_DYNAMIC_CAST_D(AP4_UnknownSampleDescription, AP4_SampleDescription) 00130 00131 // this constructor takes makes a copy of the atom passed as an argument 00132 AP4_UnknownSampleDescription(AP4_Atom* atom); 00133 ~AP4_UnknownSampleDescription(); 00134 00135 virtual AP4_SampleDescription* Clone(AP4_Result* result); 00136 virtual AP4_Atom* ToAtom() const; 00137 00138 // accessor 00139 const AP4_Atom* GetAtom() { return m_Atom; } 00140 00141 private: 00142 AP4_Atom* m_Atom; 00143 }; 00144 00145 /*---------------------------------------------------------------------- 00146 | AP4_AudioSampleDescription // MIXIN class 00147 +---------------------------------------------------------------------*/ 00148 class AP4_AudioSampleDescription 00149 { 00150 public: 00151 AP4_IMPLEMENT_DYNAMIC_CAST(AP4_AudioSampleDescription) 00152 00153 // constructor 00154 AP4_AudioSampleDescription(unsigned int sample_rate, 00155 unsigned int sample_size, 00156 unsigned int channel_count) : 00157 m_SampleRate(sample_rate), 00158 m_SampleSize(sample_size), 00159 m_ChannelCount(channel_count) {} 00160 00161 // accessors 00162 AP4_UI32 GetSampleRate() { return m_SampleRate; } 00163 AP4_UI16 GetSampleSize() { return m_SampleSize; } 00164 AP4_UI16 GetChannelCount() { return m_ChannelCount; } 00165 00166 protected: 00167 // members 00168 AP4_UI32 m_SampleRate; 00169 AP4_UI16 m_SampleSize; 00170 AP4_UI16 m_ChannelCount; 00171 }; 00172 00173 /*---------------------------------------------------------------------- 00174 | AP4_VideoSampleDescription // MIXIN class 00175 +---------------------------------------------------------------------*/ 00176 class AP4_VideoSampleDescription 00177 { 00178 public: 00179 AP4_IMPLEMENT_DYNAMIC_CAST(AP4_VideoSampleDescription) 00180 00181 // constructor 00182 AP4_VideoSampleDescription(AP4_UI16 width, 00183 AP4_UI16 height, 00184 AP4_UI16 depth, 00185 const char* compressor_name) : 00186 m_Width(width), 00187 m_Height(height), 00188 m_Depth(depth), 00189 m_CompressorName(compressor_name) {} 00190 00191 // accessors 00192 AP4_UI16 GetWidth() { return m_Width; } 00193 AP4_UI16 GetHeight() { return m_Height; } 00194 AP4_UI16 GetDepth() { return m_Depth; } 00195 const char* GetCompressorName() { return m_CompressorName.GetChars(); } 00196 00197 protected: 00198 // members 00199 AP4_UI16 m_Width; 00200 AP4_UI16 m_Height; 00201 AP4_UI16 m_Depth; 00202 AP4_String m_CompressorName; 00203 }; 00204 00205 /*---------------------------------------------------------------------- 00206 | AP4_GenericAudioSampleDescription 00207 +---------------------------------------------------------------------*/ 00208 class AP4_GenericAudioSampleDescription : public AP4_SampleDescription, 00209 public AP4_AudioSampleDescription 00210 { 00211 public: 00212 AP4_IMPLEMENT_DYNAMIC_CAST_D2(AP4_GenericAudioSampleDescription, AP4_SampleDescription, AP4_AudioSampleDescription) 00213 00214 // constructors 00215 AP4_GenericAudioSampleDescription(AP4_UI32 format, 00216 unsigned int sample_rate, 00217 unsigned int sample_size, 00218 unsigned int channel_count, 00219 AP4_AtomParent* details) : 00220 AP4_SampleDescription(TYPE_UNKNOWN, format, details), 00221 AP4_AudioSampleDescription(sample_rate, sample_size, channel_count) {} 00222 }; 00223 00224 /*---------------------------------------------------------------------- 00225 | AP4_GenericVideoSampleDescription 00226 +---------------------------------------------------------------------*/ 00227 class AP4_GenericVideoSampleDescription : public AP4_SampleDescription, 00228 public AP4_VideoSampleDescription 00229 { 00230 public: 00231 AP4_IMPLEMENT_DYNAMIC_CAST_D2(AP4_GenericVideoSampleDescription, AP4_SampleDescription, AP4_VideoSampleDescription) 00232 00233 // constructor 00234 AP4_GenericVideoSampleDescription(AP4_UI32 format, 00235 AP4_UI16 width, 00236 AP4_UI16 height, 00237 AP4_UI16 depth, 00238 const char* compressor_name, 00239 AP4_AtomParent* details) : 00240 AP4_SampleDescription(TYPE_UNKNOWN, format, details), 00241 AP4_VideoSampleDescription(width, height, depth, compressor_name) {} 00242 }; 00243 00244 /*---------------------------------------------------------------------- 00245 | AP4_AvcSampleDescription 00246 +---------------------------------------------------------------------*/ 00247 class AP4_AvcSampleDescription : public AP4_SampleDescription, 00248 public AP4_VideoSampleDescription 00249 { 00250 public: 00251 AP4_IMPLEMENT_DYNAMIC_CAST_D2(AP4_AvcSampleDescription, AP4_SampleDescription, AP4_VideoSampleDescription) 00252 00253 // constructors 00254 AP4_AvcSampleDescription(AP4_UI16 width, 00255 AP4_UI16 height, 00256 AP4_UI16 depth, 00257 const char* compressor_name, 00258 const AP4_AvccAtom* avcc); 00259 00260 AP4_AvcSampleDescription(AP4_UI16 width, 00261 AP4_UI16 height, 00262 AP4_UI16 depth, 00263 const char* compressor_name, 00264 AP4_AtomParent* details); 00265 00266 AP4_AvcSampleDescription(AP4_UI16 width, 00267 AP4_UI16 height, 00268 AP4_UI16 depth, 00269 const char* compressor_name, 00270 AP4_UI08 profile, 00271 AP4_UI08 level, 00272 AP4_UI08 profile_compatibility, 00273 AP4_UI08 nalu_length_size, 00274 const AP4_Array<AP4_DataBuffer>& sequence_parameters, 00275 const AP4_Array<AP4_DataBuffer>& picture_parameters); 00276 00277 // accessors 00278 AP4_UI08 GetConfigurationVersion() const { return m_AvccAtom->GetConfigurationVersion(); } 00279 AP4_UI08 GetProfile() const { return m_AvccAtom->GetProfile(); } 00280 AP4_UI08 GetLevel() const { return m_AvccAtom->GetLevel(); } 00281 AP4_UI08 GetProfileCompatibility() const { return m_AvccAtom->GetProfileCompatibility(); } 00282 AP4_UI08 GetNaluLengthSize() const { return m_AvccAtom->GetNaluLengthSize(); } 00283 AP4_Array<AP4_DataBuffer>& GetSequenceParameters() {return m_AvccAtom->GetSequenceParameters(); } 00284 AP4_Array<AP4_DataBuffer>& GetPictureParameters() { return m_AvccAtom->GetPictureParameters(); } 00285 const AP4_DataBuffer& GetRawBytes() const { return m_AvccAtom->GetRawBytes(); } 00286 00287 // inherited from AP4_SampleDescription 00288 virtual AP4_Atom* ToAtom() const; 00289 00290 // static methods 00291 static const char* GetProfileName(AP4_UI08 profile) { 00292 return AP4_AvccAtom::GetProfileName(profile); 00293 } 00294 00295 private: 00296 AP4_AvccAtom* m_AvccAtom; 00297 }; 00298 00299 /*---------------------------------------------------------------------- 00300 | AP4_MpegSampleDescription 00301 +---------------------------------------------------------------------*/ 00302 class AP4_MpegSampleDescription : public AP4_SampleDescription 00303 { 00304 public: 00305 AP4_IMPLEMENT_DYNAMIC_CAST_D(AP4_MpegSampleDescription, AP4_SampleDescription) 00306 00307 // types 00308 typedef AP4_UI08 StreamType; 00309 typedef AP4_UI08 OTI; 00310 00311 // class methods 00312 static const char* GetStreamTypeString(StreamType type); 00313 static const char* GetObjectTypeString(OTI oti); 00314 00315 // constructors & destructor 00316 AP4_MpegSampleDescription(AP4_UI32 format, 00317 AP4_EsdsAtom* esds); 00318 AP4_MpegSampleDescription(AP4_UI32 format, 00319 StreamType stream_type, 00320 OTI oti, 00321 const AP4_DataBuffer* decoder_info, 00322 AP4_UI32 buffer_size, 00323 AP4_UI32 max_bitrate, 00324 AP4_UI32 avg_bitrate); 00325 00326 // accessors 00327 AP4_Byte GetStreamType() const { return m_StreamType; } 00328 AP4_Byte GetObjectTypeId() const { return m_ObjectTypeId; } 00329 AP4_UI32 GetBufferSize() const { return m_BufferSize; } 00330 AP4_UI32 GetMaxBitrate() const { return m_MaxBitrate; } 00331 AP4_UI32 GetAvgBitrate() const { return m_AvgBitrate; } 00332 const AP4_DataBuffer& GetDecoderInfo() const { return m_DecoderInfo; } 00333 00334 // methods 00335 AP4_EsDescriptor* CreateEsDescriptor() const; 00336 00337 protected: 00338 // members 00339 AP4_UI32 m_Format; 00340 StreamType m_StreamType; 00341 OTI m_ObjectTypeId; 00342 AP4_UI32 m_BufferSize; 00343 AP4_UI32 m_MaxBitrate; 00344 AP4_UI32 m_AvgBitrate; 00345 AP4_DataBuffer m_DecoderInfo; 00346 }; 00347 00348 /*---------------------------------------------------------------------- 00349 | AP4_MpegSystemSampleDescription 00350 +---------------------------------------------------------------------*/ 00351 class AP4_MpegSystemSampleDescription : public AP4_MpegSampleDescription 00352 { 00353 public: 00354 AP4_IMPLEMENT_DYNAMIC_CAST_D(AP4_MpegSystemSampleDescription, AP4_MpegSampleDescription) 00355 00356 // constructor 00357 AP4_MpegSystemSampleDescription(AP4_EsdsAtom* esds); 00358 AP4_MpegSystemSampleDescription(StreamType stream_type, 00359 OTI oti, 00360 const AP4_DataBuffer* decoder_info, 00361 AP4_UI32 buffer_size, 00362 AP4_UI32 max_bitrate, 00363 AP4_UI32 avg_bitrate); 00364 00365 // methods 00366 AP4_Atom* ToAtom() const; 00367 }; 00368 00369 /*---------------------------------------------------------------------- 00370 | AP4_MpegAudioSampleDescription 00371 +---------------------------------------------------------------------*/ 00372 class AP4_MpegAudioSampleDescription : public AP4_MpegSampleDescription, 00373 public AP4_AudioSampleDescription 00374 { 00375 public: 00376 AP4_IMPLEMENT_DYNAMIC_CAST_D2(AP4_MpegAudioSampleDescription, AP4_MpegSampleDescription, AP4_AudioSampleDescription) 00377 00378 // types 00379 typedef AP4_UI08 Mpeg4AudioObjectType; 00380 00381 // class methods 00382 static const char* GetMpeg4AudioObjectTypeString(Mpeg4AudioObjectType type); 00383 00384 // constructor 00385 AP4_MpegAudioSampleDescription(unsigned int sample_rate, 00386 unsigned int sample_size, 00387 unsigned int channel_count, 00388 AP4_EsdsAtom* esds); 00389 00390 AP4_MpegAudioSampleDescription(OTI oti, 00391 unsigned int sample_rate, 00392 unsigned int sample_size, 00393 unsigned int channel_count, 00394 const AP4_DataBuffer* decoder_info, 00395 AP4_UI32 buffer_size, 00396 AP4_UI32 max_bitrate, 00397 AP4_UI32 avg_bitrate); 00398 00399 // methods 00400 AP4_Atom* ToAtom() const; 00401 00407 Mpeg4AudioObjectType GetMpeg4AudioObjectType() const; 00408 }; 00409 00410 /*---------------------------------------------------------------------- 00411 | AP4_MpegVideoSampleDescription 00412 +---------------------------------------------------------------------*/ 00413 class AP4_MpegVideoSampleDescription : public AP4_MpegSampleDescription, 00414 public AP4_VideoSampleDescription 00415 { 00416 public: 00417 AP4_IMPLEMENT_DYNAMIC_CAST_D2(AP4_MpegVideoSampleDescription, AP4_MpegSampleDescription, AP4_VideoSampleDescription) 00418 00419 // constructor 00420 AP4_MpegVideoSampleDescription(AP4_UI16 width, 00421 AP4_UI16 height, 00422 AP4_UI16 depth, 00423 const char* compressor_name, 00424 AP4_EsdsAtom* esds); 00425 00426 AP4_MpegVideoSampleDescription(OTI oti, 00427 AP4_UI16 width, 00428 AP4_UI16 height, 00429 AP4_UI16 depth, 00430 const char* compressor_name, 00431 const AP4_DataBuffer* decoder_info, 00432 AP4_UI32 buffer_size, 00433 AP4_UI32 max_bitrate, 00434 AP4_UI32 avg_bitrate); 00435 00436 // methods 00437 AP4_Atom* ToAtom() const; 00438 }; 00439 00440 /*---------------------------------------------------------------------- 00441 | constants 00442 +---------------------------------------------------------------------*/ 00443 const AP4_MpegSampleDescription::StreamType AP4_STREAM_TYPE_FORBIDDEN = 0x00; 00444 const AP4_MpegSampleDescription::StreamType AP4_STREAM_TYPE_OD = 0x01; 00445 const AP4_MpegSampleDescription::StreamType AP4_STREAM_TYPE_CR = 0x02; 00446 const AP4_MpegSampleDescription::StreamType AP4_STREAM_TYPE_BIFS = 0x03; 00447 const AP4_MpegSampleDescription::StreamType AP4_STREAM_TYPE_VISUAL = 0x04; 00448 const AP4_MpegSampleDescription::StreamType AP4_STREAM_TYPE_AUDIO = 0x05; 00449 const AP4_MpegSampleDescription::StreamType AP4_STREAM_TYPE_MPEG7 = 0x06; 00450 const AP4_MpegSampleDescription::StreamType AP4_STREAM_TYPE_IPMP = 0x07; 00451 const AP4_MpegSampleDescription::StreamType AP4_STREAM_TYPE_OCI = 0x08; 00452 const AP4_MpegSampleDescription::StreamType AP4_STREAM_TYPE_MPEGJ = 0x09; 00453 const AP4_MpegSampleDescription::StreamType AP4_STREAM_TYPE_TEXT = 0x0D; 00454 00455 const AP4_MpegSampleDescription::OTI AP4_OTI_MPEG4_SYSTEM = 0x01; 00456 const AP4_MpegSampleDescription::OTI AP4_OTI_MPEG4_SYSTEM_COR = 0x02; 00457 const AP4_MpegSampleDescription::OTI AP4_OTI_MPEG4_TEXT = 0x08; 00458 const AP4_MpegSampleDescription::OTI AP4_OTI_MPEG4_VISUAL = 0x20; 00459 const AP4_MpegSampleDescription::OTI AP4_OTI_MPEG4_AUDIO = 0x40; 00460 const AP4_MpegSampleDescription::OTI AP4_OTI_MPEG2_VISUAL_SIMPLE = 0x60; 00461 const AP4_MpegSampleDescription::OTI AP4_OTI_MPEG2_VISUAL_MAIN = 0x61; 00462 const AP4_MpegSampleDescription::OTI AP4_OTI_MPEG2_VISUAL_SNR = 0x62; 00463 const AP4_MpegSampleDescription::OTI AP4_OTI_MPEG2_VISUAL_SPATIAL = 0x63; 00464 const AP4_MpegSampleDescription::OTI AP4_OTI_MPEG2_VISUAL_HIGH = 0x64; 00465 const AP4_MpegSampleDescription::OTI AP4_OTI_MPEG2_VISUAL_422 = 0x65; 00466 const AP4_MpegSampleDescription::OTI AP4_OTI_MPEG2_AAC_AUDIO_MAIN = 0x66; 00467 const AP4_MpegSampleDescription::OTI AP4_OTI_MPEG2_AAC_AUDIO_LC = 0x67; 00468 const AP4_MpegSampleDescription::OTI AP4_OTI_MPEG2_AAC_AUDIO_SSRP = 0x68; 00469 const AP4_MpegSampleDescription::OTI AP4_OTI_MPEG2_PART3_AUDIO = 0x69; 00470 const AP4_MpegSampleDescription::OTI AP4_OTI_MPEG1_VISUAL = 0x6A; 00471 const AP4_MpegSampleDescription::OTI AP4_OTI_MPEG1_AUDIO = 0x6B; 00472 const AP4_MpegSampleDescription::OTI AP4_OTI_JPEG = 0x6C; 00473 00474 const AP4_UI08 AP4_MPEG4_AUDIO_OBJECT_TYPE_AAC_MAIN = 1; 00475 const AP4_UI08 AP4_MPEG4_AUDIO_OBJECT_TYPE_AAC_LC = 2; 00476 const AP4_UI08 AP4_MPEG4_AUDIO_OBJECT_TYPE_AAC_SSR = 3; 00477 const AP4_UI08 AP4_MPEG4_AUDIO_OBJECT_TYPE_AAC_LTP = 4; 00478 const AP4_UI08 AP4_MPEG4_AUDIO_OBJECT_TYPE_SBR = 5; 00479 const AP4_UI08 AP4_MPEG4_AUDIO_OBJECT_TYPE_AAC_SCALABLE = 6; 00480 const AP4_UI08 AP4_MPEG4_AUDIO_OBJECT_TYPE_TWINVQ = 7; 00481 const AP4_UI08 AP4_MPEG4_AUDIO_OBJECT_TYPE_CELP = 8; 00482 const AP4_UI08 AP4_MPEG4_AUDIO_OBJECT_TYPE_HVXC = 9; 00483 const AP4_UI08 AP4_MPEG4_AUDIO_OBJECT_TYPE_TTSI = 12; 00484 const AP4_UI08 AP4_MPEG4_AUDIO_OBJECT_TYPE_MAIN_SYNTHETIC = 13; 00485 const AP4_UI08 AP4_MPEG4_AUDIO_OBJECT_TYPE_WAVETABLE_SYNTHESIS = 14; 00486 const AP4_UI08 AP4_MPEG4_AUDIO_OBJECT_TYPE_GENERAL_MIDI = 15; 00487 const AP4_UI08 AP4_MPEG4_AUDIO_OBJECT_TYPE_ALGORITHMIC_SYNTHESIS = 16; 00488 const AP4_UI08 AP4_MPEG4_AUDIO_OBJECT_TYPE_ER_AAC_LC = 17; 00489 const AP4_UI08 AP4_MPEG4_AUDIO_OBJECT_TYPE_ER_AAC_LTP = 19; 00490 const AP4_UI08 AP4_MPEG4_AUDIO_OBJECT_TYPE_ER_AAC_SCALABLE = 20; 00491 const AP4_UI08 AP4_MPEG4_AUDIO_OBJECT_TYPE_ER_TWINVQ = 21; 00492 const AP4_UI08 AP4_MPEG4_AUDIO_OBJECT_TYPE_ER_BSAC = 22; 00493 const AP4_UI08 AP4_MPEG4_AUDIO_OBJECT_TYPE_ER_AAC_LD = 23; 00494 const AP4_UI08 AP4_MPEG4_AUDIO_OBJECT_TYPE_ER_CELP = 24; 00495 const AP4_UI08 AP4_MPEG4_AUDIO_OBJECT_TYPE_ER_HVXC = 25; 00496 const AP4_UI08 AP4_MPEG4_AUDIO_OBJECT_TYPE_ER_HILN = 26; 00497 const AP4_UI08 AP4_MPEG4_AUDIO_OBJECT_TYPE_ER_PARAMETRIC = 27; 00498 const AP4_UI08 AP4_MPEG4_AUDIO_OBJECT_TYPE_SSC = 28; 00499 const AP4_UI08 AP4_MPEG4_AUDIO_OBJECT_TYPE_PS = 29; 00500 const AP4_UI08 AP4_MPEG4_AUDIO_OBJECT_TYPE_MPEG_SURROUND = 30; 00501 const AP4_UI08 AP4_MPEG4_AUDIO_OBJECT_TYPE_LAYER_1 = 32; 00502 const AP4_UI08 AP4_MPEG4_AUDIO_OBJECT_TYPE_LAYER_2 = 33; 00503 const AP4_UI08 AP4_MPEG4_AUDIO_OBJECT_TYPE_LAYER_3 = 34; 00504 const AP4_UI08 AP4_MPEG4_AUDIO_OBJECT_TYPE_DST = 35; 00505 const AP4_UI08 AP4_MPEG4_AUDIO_OBJECT_TYPE_ALS = 36; 00506 const AP4_UI08 AP4_MPEG4_AUDIO_OBJECT_TYPE_SLS = 37; 00507 const AP4_UI08 AP4_MPEG4_AUDIO_OBJECT_TYPE_SLS_NON_CORE = 38; 00508 const AP4_UI08 AP4_MPEG4_AUDIO_OBJECT_TYPE_ER_AAC_ELD = 39; 00509 const AP4_UI08 AP4_MPEG4_AUDIO_OBJECT_TYPE_SMR_SIMPLE = 40; 00510 const AP4_UI08 AP4_MPEG4_AUDIO_OBJECT_TYPE_SMR_MAIN = 41; 00512 #endif // _AP4_SAMPLE_DESCRIPTION_H_ 00513
Generated on Thu May 13 16:36:33 2010 for Bento4 MP4 SDK by
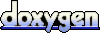