Ap4Protection.h
Go to the documentation of this file.00001 /***************************************************************** 00002 | 00003 | AP4 - Protected Streams support 00004 | 00005 | Copyright 2002-2008 Axiomatic Systems, LLC 00006 | 00007 | 00008 | This file is part of Bento4/AP4 (MP4 Atom Processing Library). 00009 | 00010 | Unless you have obtained Bento4 under a difference license, 00011 | this version of Bento4 is Bento4|GPL. 00012 | Bento4|GPL is free software; you can redistribute it and/or modify 00013 | it under the terms of the GNU General Public License as published by 00014 | the Free Software Foundation; either version 2, or (at your option) 00015 | any later version. 00016 | 00017 | Bento4|GPL is distributed in the hope that it will be useful, 00018 | but WITHOUT ANY WARRANTY; without even the implied warranty of 00019 | MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00020 | GNU General Public License for more details. 00021 | 00022 | You should have received a copy of the GNU General Public License 00023 | along with Bento4|GPL; see the file COPYING. If not, write to the 00024 | Free Software Foundation, 59 Temple Place - Suite 330, Boston, MA 00025 | 02111-1307, USA. 00026 | 00027 ****************************************************************/ 00028 00029 #ifndef _AP4_PROTECTION_H_ 00030 #define _AP4_PROTECTION_H_ 00031 00032 /*---------------------------------------------------------------------- 00033 | includes 00034 +---------------------------------------------------------------------*/ 00035 #include "Ap4Types.h" 00036 #include "Ap4SampleEntry.h" 00037 #include "Ap4Atom.h" 00038 #include "Ap4AtomFactory.h" 00039 #include "Ap4SampleDescription.h" 00040 #include "Ap4Processor.h" 00041 00042 /*---------------------------------------------------------------------- 00043 | classes 00044 +---------------------------------------------------------------------*/ 00045 class AP4_StreamCipher; 00046 00047 /*---------------------------------------------------------------------- 00048 | constants 00049 +---------------------------------------------------------------------*/ 00050 // this is fixed for now 00051 const unsigned int AP4_PROTECTION_KEY_LENGTH = 16; 00052 00053 const AP4_UI32 AP4_PROTECTION_SCHEME_TYPE_ITUNES = AP4_ATOM_TYPE('i','t','u','n'); 00054 00055 /*---------------------------------------------------------------------- 00056 | AP4_EncaSampleEntry 00057 +---------------------------------------------------------------------*/ 00058 class AP4_EncaSampleEntry : public AP4_AudioSampleEntry 00059 { 00060 public: 00061 // methods 00062 AP4_EncaSampleEntry(AP4_Size size, 00063 AP4_ByteStream& stream, 00064 AP4_AtomFactory& atom_factory); 00065 AP4_EncaSampleEntry(AP4_UI32 type, 00066 AP4_Size size, 00067 AP4_ByteStream& stream, 00068 AP4_AtomFactory& atom_factory); 00069 00070 // methods 00071 AP4_SampleDescription* ToSampleDescription(); 00072 00073 // this method is used as a factory by the ISMACryp classes 00074 // NOTE: this should be named ToSampleDescription, but C++ has a 00075 // problem with that because the base class does not have this 00076 // overloaded method, but has another other one by that name 00077 virtual AP4_SampleDescription* ToTargetSampleDescription(AP4_UI32 format); 00078 }; 00079 00080 /*---------------------------------------------------------------------- 00081 | AP4_EncvSampleEntry 00082 +---------------------------------------------------------------------*/ 00083 class AP4_EncvSampleEntry : public AP4_VisualSampleEntry 00084 { 00085 public: 00086 // constructors 00087 AP4_EncvSampleEntry(AP4_Size size, 00088 AP4_ByteStream& stream, 00089 AP4_AtomFactory& atom_factory); 00090 AP4_EncvSampleEntry(AP4_UI32 type, 00091 AP4_Size size, 00092 AP4_ByteStream& stream, 00093 AP4_AtomFactory& atom_factory); 00094 00095 // methods 00096 AP4_SampleDescription* ToSampleDescription(); 00097 00098 // this method is used as a factory by the ISMACryp classes 00099 // NOTE: this should be named ToSampleDescription, but C++ has a 00100 // problem with that because the base class does not have this 00101 // overloaded method, but has another other one by that name 00102 virtual AP4_SampleDescription* ToTargetSampleDescription(AP4_UI32 format); 00103 }; 00104 00105 /*---------------------------------------------------------------------- 00106 | AP4_DrmsSampleEntry 00107 +---------------------------------------------------------------------*/ 00108 class AP4_DrmsSampleEntry : public AP4_EncaSampleEntry 00109 { 00110 public: 00111 // methods 00112 AP4_DrmsSampleEntry(AP4_Size size, 00113 AP4_ByteStream& stream, 00114 AP4_AtomFactory& atom_factory); 00115 }; 00116 00117 /*---------------------------------------------------------------------- 00118 | AP4_DrmiSampleEntry 00119 +---------------------------------------------------------------------*/ 00120 class AP4_DrmiSampleEntry : public AP4_EncvSampleEntry 00121 { 00122 public: 00123 // methods 00124 AP4_DrmiSampleEntry(AP4_Size size, 00125 AP4_ByteStream& stream, 00126 AP4_AtomFactory& atom_factory); 00127 }; 00128 00129 /*---------------------------------------------------------------------- 00130 | AP4_ProtectionKeyMap 00131 +---------------------------------------------------------------------*/ 00132 class AP4_ProtectionKeyMap 00133 { 00134 public: 00135 // constructors and destructor 00136 AP4_ProtectionKeyMap(); 00137 ~AP4_ProtectionKeyMap(); 00138 00139 // methods 00140 AP4_Result SetKey(AP4_UI32 track_id, const AP4_UI08* key, const AP4_UI08* iv = NULL); 00141 AP4_Result SetKeys(const AP4_ProtectionKeyMap& key_map); 00142 AP4_Result GetKeyAndIv(AP4_UI32 track_id, const AP4_UI08*& key, const AP4_UI08*& iv); 00143 const AP4_UI08* GetKey(AP4_UI32 track_id) const; 00144 00145 private: 00146 // types 00147 class KeyEntry { 00148 public: 00149 KeyEntry(AP4_UI32 track_id, const AP4_UI08* key, const AP4_UI08* iv = NULL); 00150 void SetKey(const AP4_UI08* key, const AP4_UI08* iv); 00151 AP4_Ordinal m_TrackId; 00152 AP4_UI08 m_Key[AP4_PROTECTION_KEY_LENGTH]; 00153 AP4_UI08 m_IV[AP4_PROTECTION_KEY_LENGTH]; 00154 }; 00155 00156 // methods 00157 KeyEntry* GetEntry(AP4_UI32 track_id) const; 00158 00159 // members 00160 AP4_List<KeyEntry> m_KeyEntries; 00161 }; 00162 00163 /*---------------------------------------------------------------------- 00164 | AP4_TrackPropertyMap 00165 +---------------------------------------------------------------------*/ 00166 class AP4_TrackPropertyMap 00167 { 00168 public: 00169 // methods 00170 AP4_Result SetProperty(AP4_UI32 track_id, const char* name, const char* value); 00171 AP4_Result SetProperties(const AP4_TrackPropertyMap& properties); 00172 const char* GetProperty(AP4_UI32 track_id, const char* name); 00173 AP4_Result GetTextualHeaders(AP4_UI32 track_id, AP4_DataBuffer& buffer); 00174 00175 00176 // destructor 00177 virtual ~AP4_TrackPropertyMap(); 00178 00179 private: 00180 // types 00181 class Entry { 00182 public: 00183 Entry(AP4_UI32 track_id, const char* name, const char* value) : 00184 m_TrackId(track_id), m_Name(name), m_Value(value) {} 00185 AP4_UI32 m_TrackId; 00186 AP4_String m_Name; 00187 AP4_String m_Value; 00188 }; 00189 00190 // members 00191 AP4_List<Entry> m_Entries; 00192 }; 00193 00194 /*---------------------------------------------------------------------- 00195 | AP4_ProtectionSchemeInfo 00196 +---------------------------------------------------------------------*/ 00197 class AP4_ProtectionSchemeInfo 00198 { 00199 public: 00200 // constructors and destructor 00201 AP4_ProtectionSchemeInfo(AP4_ContainerAtom* schi); 00202 virtual ~AP4_ProtectionSchemeInfo(); 00203 00204 // accessors 00205 AP4_ContainerAtom* GetSchiAtom() { return m_SchiAtom; } 00206 00207 protected: 00208 AP4_ContainerAtom* m_SchiAtom; 00209 }; 00210 00211 /*---------------------------------------------------------------------- 00212 | AP4_ProtectedSampleDescription 00213 +---------------------------------------------------------------------*/ 00214 class AP4_ProtectedSampleDescription : public AP4_SampleDescription 00215 { 00216 public: 00217 AP4_IMPLEMENT_DYNAMIC_CAST_D(AP4_ProtectedSampleDescription, AP4_SampleDescription) 00218 00219 // constructor and destructor 00220 AP4_ProtectedSampleDescription(AP4_UI32 format, 00221 AP4_SampleDescription* original_sample_description, 00222 AP4_UI32 original_format, 00223 AP4_UI32 scheme_type, 00224 AP4_UI32 scheme_version, 00225 const char* scheme_uri, 00226 AP4_ContainerAtom* schi_atom, // will be cloned 00227 bool transfer_ownership_of_original=true); 00228 ~AP4_ProtectedSampleDescription(); 00229 00230 // accessors 00231 AP4_SampleDescription* GetOriginalSampleDescription() { 00232 return m_OriginalSampleDescription; 00233 } 00234 AP4_UI32 GetOriginalFormat() const { return m_OriginalFormat; } 00235 AP4_UI32 GetSchemeType() const { return m_SchemeType; } 00236 AP4_UI32 GetSchemeVersion() const { return m_SchemeVersion; } 00237 const AP4_String& GetSchemeUri() const { return m_SchemeUri; } 00238 AP4_ProtectionSchemeInfo* GetSchemeInfo() const { 00239 return m_SchemeInfo; 00240 } 00241 00242 // implementation of abstract base class methods 00243 virtual AP4_Atom* ToAtom() const; 00244 00245 private: 00246 // members 00247 AP4_SampleDescription* m_OriginalSampleDescription; 00248 bool m_OriginalSampleDescriptionIsOwned; 00249 AP4_UI32 m_OriginalFormat; 00250 AP4_UI32 m_SchemeType; 00251 AP4_UI32 m_SchemeVersion; 00252 AP4_String m_SchemeUri; 00253 AP4_ProtectionSchemeInfo* m_SchemeInfo; 00254 }; 00255 00256 /*---------------------------------------------------------------------- 00257 | AP4_BlockCipher 00258 +---------------------------------------------------------------------*/ 00259 class AP4_BlockCipher 00260 { 00261 public: 00262 // types 00263 typedef enum { 00264 ENCRYPT, 00265 DECRYPT 00266 } CipherDirection; 00267 00268 typedef enum { 00269 AES_128 00270 } CipherType; 00271 00272 // constructor and destructor 00273 virtual ~AP4_BlockCipher() {} 00274 00275 // methods 00276 virtual AP4_Result ProcessBlock(const AP4_UI08* block_in, AP4_UI08* block_out) = 0; 00277 }; 00278 00279 /*---------------------------------------------------------------------- 00280 | AP4_BlockCipherFactory 00281 +---------------------------------------------------------------------*/ 00282 class AP4_BlockCipherFactory 00283 { 00284 public: 00285 // methods 00286 virtual ~AP4_BlockCipherFactory() {} 00287 virtual AP4_Result Create(AP4_BlockCipher::CipherType type, 00288 AP4_BlockCipher::CipherDirection direction, 00289 const AP4_UI08* key, 00290 AP4_Size key_size, 00291 AP4_BlockCipher*& cipher) = 0; 00292 }; 00293 00294 /*---------------------------------------------------------------------- 00295 | AP4_DefaultBlockCipherFactory 00296 +---------------------------------------------------------------------*/ 00297 class AP4_DefaultBlockCipherFactory : public AP4_BlockCipherFactory 00298 { 00299 public: 00300 // class variables 00301 static AP4_DefaultBlockCipherFactory Instance; 00302 00303 // methods 00304 virtual AP4_Result Create(AP4_BlockCipher::CipherType type, 00305 AP4_BlockCipher::CipherDirection direction, 00306 const AP4_UI08* key, 00307 AP4_Size key_size, 00308 AP4_BlockCipher*& cipher); 00309 }; 00310 00311 /*---------------------------------------------------------------------- 00312 | AP4_SampleDecrypter 00313 +---------------------------------------------------------------------*/ 00314 class AP4_SampleDecrypter 00315 { 00316 public: 00320 static AP4_SampleDecrypter* Create(AP4_ProtectedSampleDescription* sample_description, 00321 const AP4_UI08* key, 00322 AP4_Size key_size, 00323 AP4_BlockCipherFactory* block_cipher_factory = NULL); 00324 00328 static AP4_SampleDecrypter* Create(AP4_ProtectedSampleDescription* sample_description, 00329 AP4_ContainerAtom* traf, 00330 const AP4_UI08* key, 00331 AP4_Size key_size, 00332 AP4_BlockCipherFactory* block_cipher_factory = NULL); 00333 00334 // destructor 00335 virtual ~AP4_SampleDecrypter() {} 00336 00337 // methods 00338 virtual AP4_Size GetDecryptedSampleSize(AP4_Sample& sample) { return sample.GetSize(); } 00339 virtual AP4_Result SetSampleIndex(AP4_Ordinal /*index*/) { return AP4_SUCCESS; } 00340 virtual AP4_Result DecryptSampleData(AP4_DataBuffer& data_in, 00341 AP4_DataBuffer& data_out, 00342 const AP4_UI08* iv = NULL) = 0; 00343 }; 00344 00345 /*---------------------------------------------------------------------- 00346 | AP4_StandardDecryptingProcessor 00347 +---------------------------------------------------------------------*/ 00348 class AP4_StandardDecryptingProcessor : public AP4_Processor 00349 { 00350 public: 00351 // constructor 00352 AP4_StandardDecryptingProcessor(const AP4_ProtectionKeyMap* key_map = NULL, 00353 AP4_BlockCipherFactory* block_cipher_factory = NULL); 00354 00355 // accessors 00356 AP4_ProtectionKeyMap& GetKeyMap() { return m_KeyMap; } 00357 00358 // methods 00359 virtual AP4_Result Initialize(AP4_AtomParent& top_level, 00360 AP4_ByteStream& stream, 00361 ProgressListener* listener); 00362 virtual AP4_Processor::TrackHandler* CreateTrackHandler(AP4_TrakAtom* trak); 00363 00364 private: 00365 // members 00366 AP4_BlockCipherFactory* m_BlockCipherFactory; 00367 AP4_ProtectionKeyMap m_KeyMap; 00368 }; 00369 00370 /*---------------------------------------------------------------------- 00371 | AP4_DecryptingStream 00372 +---------------------------------------------------------------------*/ 00373 class AP4_DecryptingStream : public AP4_ByteStream 00374 { 00375 public: 00376 typedef enum { 00377 CIPHER_MODE_CTR, 00378 CIPHER_MODE_CBC 00379 } CipherMode; 00380 00381 static AP4_Result Create(CipherMode mode, 00382 AP4_ByteStream& encrypted_stream, 00383 AP4_LargeSize cleartext_size, 00384 const AP4_UI08* iv, 00385 AP4_Size iv_size, 00386 const AP4_UI08* key, 00387 AP4_Size key_size, 00388 AP4_BlockCipherFactory* block_cipher_factory, 00389 AP4_ByteStream*& stream); 00390 00391 // AP4_ByteStream methods 00392 virtual AP4_Result ReadPartial(void* buffer, 00393 AP4_Size bytes_to_read, 00394 AP4_Size& bytes_read); 00395 virtual AP4_Result WritePartial(const void* buffer, 00396 AP4_Size bytes_to_write, 00397 AP4_Size& bytes_written); 00398 virtual AP4_Result Seek(AP4_Position position); 00399 virtual AP4_Result Tell(AP4_Position& position); 00400 virtual AP4_Result GetSize(AP4_LargeSize& size); 00401 00402 // AP4_Referenceable methods 00403 virtual void AddReference(); 00404 virtual void Release(); 00405 00406 private: 00407 // methods 00408 AP4_DecryptingStream() {} // private constructor, use the factory instead 00409 ~AP4_DecryptingStream(); 00410 00411 // members 00412 CipherMode m_Mode; 00413 AP4_LargeSize m_CleartextSize; 00414 AP4_Position m_CleartextPosition; 00415 AP4_ByteStream* m_EncryptedStream; 00416 AP4_LargeSize m_EncryptedSize; 00417 AP4_Position m_EncryptedPosition; 00418 AP4_StreamCipher* m_StreamCipher; 00419 AP4_UI08 m_Buffer[16]; 00420 AP4_Size m_BufferFullness; 00421 AP4_Size m_BufferOffset; 00422 AP4_Cardinal m_ReferenceCount; 00423 }; 00424 00425 /*---------------------------------------------------------------------- 00426 | AP4_EncryptingStream 00427 +---------------------------------------------------------------------*/ 00428 class AP4_EncryptingStream : public AP4_ByteStream 00429 { 00430 public: 00431 typedef enum { 00432 CIPHER_MODE_CTR, 00433 CIPHER_MODE_CBC 00434 } CipherMode; 00435 00436 static AP4_Result Create(CipherMode mode, 00437 AP4_ByteStream& cleartext_stream, 00438 const AP4_UI08* iv, 00439 AP4_Size iv_size, 00440 const AP4_UI08* key, 00441 AP4_Size key_size, 00442 bool prepend_iv, 00443 AP4_BlockCipherFactory* block_cipher_factory, 00444 AP4_ByteStream*& stream); 00445 00446 // AP4_ByteStream methods 00447 virtual AP4_Result ReadPartial(void* buffer, 00448 AP4_Size bytes_to_read, 00449 AP4_Size& bytes_read); 00450 virtual AP4_Result WritePartial(const void* buffer, 00451 AP4_Size bytes_to_write, 00452 AP4_Size& bytes_written); 00453 virtual AP4_Result Seek(AP4_Position position); 00454 virtual AP4_Result Tell(AP4_Position& position); 00455 virtual AP4_Result GetSize(AP4_LargeSize& size); 00456 00457 // AP4_Referenceable methods 00458 virtual void AddReference(); 00459 virtual void Release(); 00460 00461 private: 00462 // methods 00463 AP4_EncryptingStream() {} // private constructor, use the factory instead 00464 ~AP4_EncryptingStream(); 00465 00466 // members 00467 CipherMode m_Mode; 00468 AP4_LargeSize m_CleartextSize; 00469 AP4_Position m_CleartextPosition; 00470 AP4_ByteStream* m_CleartextStream; 00471 AP4_LargeSize m_EncryptedSize; 00472 AP4_Position m_EncryptedPosition; 00473 AP4_StreamCipher* m_StreamCipher; 00474 AP4_UI08 m_Buffer[32]; // one cipher block plus one block padding 00475 AP4_Size m_BufferFullness; 00476 AP4_Size m_BufferOffset; 00477 AP4_Cardinal m_ReferenceCount; 00478 }; 00479 00480 #endif // _AP4_PROTECTION_H_
Generated on Thu May 13 16:36:33 2010 for Bento4 MP4 SDK by
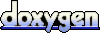