Ap4OmaDcf.h
Go to the documentation of this file.00001 /***************************************************************** 00002 | 00003 | AP4 - OMA DCF support 00004 | 00005 | Copyright 2002-2008 Axiomatic Systems, LLC 00006 | 00007 | 00008 | This file is part of Bento4/AP4 (MP4 Atom Processing Library). 00009 | 00010 | Unless you have obtained Bento4 under a difference license, 00011 | this version of Bento4 is Bento4|GPL. 00012 | Bento4|GPL is free software; you can redistribute it and/or modify 00013 | it under the terms of the GNU General Public License as published by 00014 | the Free Software Foundation; either version 2, or (at your option) 00015 | any later version. 00016 | 00017 | Bento4|GPL is distributed in the hope that it will be useful, 00018 | but WITHOUT ANY WARRANTY; without even the implied warranty of 00019 | MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00020 | GNU General Public License for more details. 00021 | 00022 | You should have received a copy of the GNU General Public License 00023 | along with Bento4|GPL; see the file COPYING. If not, write to the 00024 | Free Software Foundation, 59 Temple Place - Suite 330, Boston, MA 00025 | 02111-1307, USA. 00026 | 00027 ****************************************************************/ 00028 00029 #ifndef _AP4_OMA_DCF_H_ 00030 #define _AP4_OMA_DCF_H_ 00031 00032 /*---------------------------------------------------------------------- 00033 | includes 00034 +---------------------------------------------------------------------*/ 00035 #include "Ap4Types.h" 00036 #include "Ap4SampleEntry.h" 00037 #include "Ap4Atom.h" 00038 #include "Ap4AtomFactory.h" 00039 #include "Ap4SampleDescription.h" 00040 #include "Ap4Processor.h" 00041 #include "Ap4Protection.h" 00042 #include "Ap4DynamicCast.h" 00043 00044 /*---------------------------------------------------------------------- 00045 | class references 00046 +---------------------------------------------------------------------*/ 00047 class AP4_OdafAtom; 00048 class AP4_StreamCipher; 00049 class AP4_CbcStreamCipher; 00050 class AP4_CtrStreamCipher; 00051 00052 /*---------------------------------------------------------------------- 00053 | constants 00054 +---------------------------------------------------------------------*/ 00055 const AP4_UI32 AP4_PROTECTION_SCHEME_TYPE_OMA = AP4_ATOM_TYPE('o','d','k','m'); 00056 const AP4_UI32 AP4_PROTECTION_SCHEME_VERSION_OMA_20 = 0x00000200; 00057 const AP4_UI32 AP4_OMA_DCF_BRAND_ODCF = AP4_ATOM_TYPE('o','d','c','f'); 00058 const AP4_UI32 AP4_OMA_DCF_BRAND_OPF2 = AP4_ATOM_TYPE('o','p','f','2'); 00059 00060 typedef enum { 00061 AP4_OMA_DCF_CIPHER_MODE_CTR, 00062 AP4_OMA_DCF_CIPHER_MODE_CBC 00063 } AP4_OmaDcfCipherMode; 00064 00065 /*---------------------------------------------------------------------- 00066 | AP4_OmaDcfAtomDecrypter 00067 +---------------------------------------------------------------------*/ 00068 class AP4_OmaDcfAtomDecrypter { 00069 public: 00070 // class methods 00071 static AP4_Result DecryptAtoms(AP4_AtomParent& atoms, 00072 AP4_Processor::ProgressListener* listener, 00073 AP4_BlockCipherFactory* block_cipher_factory, 00074 AP4_ProtectionKeyMap& key_map); 00075 00076 // Returns a byte stream that will produce the decrypted data found 00077 // in the 'odda' child atom of an 'odrm' atom 00078 static AP4_Result CreateDecryptingStream(AP4_ContainerAtom& odrm_atom, 00079 const AP4_UI08* key, 00080 AP4_Size key_size, 00081 AP4_BlockCipherFactory* block_cipher_factory, 00082 AP4_ByteStream*& stream); 00083 00084 // Returns a byte stream that will produce the decrypted data from 00085 // an encrypted stream where the IV follows the encrypted bytes. 00086 // This method is normally not called directly: most callers will call 00087 // the stream factory that takes an 'odrm' atom as an input parameter 00088 static AP4_Result CreateDecryptingStream(AP4_OmaDcfCipherMode mode, 00089 AP4_ByteStream& encrypted_stream, 00090 AP4_LargeSize cleartext_size, 00091 const AP4_UI08* key, 00092 AP4_Size key_size, 00093 AP4_BlockCipherFactory* block_cipher_factory, 00094 AP4_ByteStream*& stream); 00095 }; 00096 00097 /*---------------------------------------------------------------------- 00098 | AP4_OmaDcfSampleDecrypter 00099 +---------------------------------------------------------------------*/ 00100 class AP4_OmaDcfSampleDecrypter : public AP4_SampleDecrypter 00101 { 00102 public: 00103 // factory 00104 static AP4_Result Create(AP4_ProtectedSampleDescription* sample_description, 00105 const AP4_UI08* key, 00106 AP4_Size key_size, 00107 AP4_BlockCipherFactory* block_cipher_factory, 00108 AP4_OmaDcfSampleDecrypter*& cipher); 00109 00110 // constructor and destructor 00111 AP4_OmaDcfSampleDecrypter(AP4_Size iv_length, 00112 bool selective_encryption) : 00113 m_IvLength(iv_length), 00114 m_SelectiveEncryption(selective_encryption) {} 00115 00116 protected: 00117 AP4_Size m_IvLength; 00118 AP4_Size m_KeyIndicatorLength; 00119 bool m_SelectiveEncryption; 00120 }; 00121 00122 /*---------------------------------------------------------------------- 00123 | AP4_OmaDcfCtrSampleDecrypter 00124 +---------------------------------------------------------------------*/ 00125 class AP4_OmaDcfCtrSampleDecrypter : public AP4_OmaDcfSampleDecrypter 00126 { 00127 public: 00128 // constructor and destructor 00129 AP4_OmaDcfCtrSampleDecrypter(AP4_BlockCipher* block_cipher, 00130 AP4_Size iv_length, 00131 bool selective_encryption); 00132 ~AP4_OmaDcfCtrSampleDecrypter(); 00133 00134 // methods 00135 virtual AP4_Result DecryptSampleData(AP4_DataBuffer& data_in, 00136 AP4_DataBuffer& data_out, 00137 const AP4_UI08* iv = NULL); 00138 virtual AP4_Size GetDecryptedSampleSize(AP4_Sample& sample); 00139 00140 private: 00141 // members 00142 AP4_CtrStreamCipher* m_Cipher; 00143 }; 00144 00145 /*---------------------------------------------------------------------- 00146 | AP4_OmaDcfCbcSampleDecrypter 00147 +---------------------------------------------------------------------*/ 00148 class AP4_OmaDcfCbcSampleDecrypter : public AP4_OmaDcfSampleDecrypter 00149 { 00150 public: 00151 // constructor and destructor 00152 AP4_OmaDcfCbcSampleDecrypter(AP4_BlockCipher* block_cipher, 00153 bool selective_encryption); 00154 ~AP4_OmaDcfCbcSampleDecrypter(); 00155 00156 // methods 00157 virtual AP4_Result DecryptSampleData(AP4_DataBuffer& data_in, 00158 AP4_DataBuffer& data_out, 00159 const AP4_UI08* iv = NULL); 00160 virtual AP4_Size GetDecryptedSampleSize(AP4_Sample& sample); 00161 00162 private: 00163 // members 00164 AP4_CbcStreamCipher* m_Cipher; 00165 }; 00166 00167 /*---------------------------------------------------------------------- 00168 | AP4_OmaDcfTrackDecrypter 00169 +---------------------------------------------------------------------*/ 00170 class AP4_OmaDcfTrackDecrypter : public AP4_Processor::TrackHandler { 00171 public: 00172 // constructor 00173 static AP4_Result Create(const AP4_UI08* key, 00174 AP4_Size key_size, 00175 AP4_ProtectedSampleDescription* sample_description, 00176 AP4_SampleEntry* sample_entry, 00177 AP4_BlockCipherFactory* block_cipher_factory, 00178 AP4_OmaDcfTrackDecrypter*& decrypter); 00179 virtual ~AP4_OmaDcfTrackDecrypter(); 00180 00181 // methods 00182 virtual AP4_Size GetProcessedSampleSize(AP4_Sample& sample); 00183 virtual AP4_Result ProcessTrack(); 00184 virtual AP4_Result ProcessSample(AP4_DataBuffer& data_in, 00185 AP4_DataBuffer& data_out); 00186 00187 private: 00188 // constructor 00189 AP4_OmaDcfTrackDecrypter(AP4_OmaDcfSampleDecrypter* cipher, 00190 AP4_SampleEntry* sample_entry, 00191 AP4_UI32 original_format); 00192 00193 // members 00194 AP4_OmaDcfSampleDecrypter* m_Cipher; 00195 AP4_SampleEntry* m_SampleEntry; 00196 AP4_UI32 m_OriginalFormat; 00197 }; 00198 00199 /*---------------------------------------------------------------------- 00200 | AP4_OmaDcfSampleEncrypter 00201 +---------------------------------------------------------------------*/ 00202 class AP4_OmaDcfSampleEncrypter 00203 { 00204 public: 00205 // constructor and destructor 00206 AP4_OmaDcfSampleEncrypter(const AP4_UI08* salt); // salt is only 8 bytes 00207 virtual ~AP4_OmaDcfSampleEncrypter() {} 00208 00209 // methods 00210 virtual AP4_Result EncryptSampleData(AP4_DataBuffer& data_in, 00211 AP4_DataBuffer& data_out, 00212 AP4_UI64 bso, 00213 bool skip_encryption) = 0; 00214 virtual AP4_Size GetEncryptedSampleSize(AP4_Sample& sample) = 0; 00215 00216 protected: 00217 // members 00218 AP4_UI08 m_Salt[16]; 00219 }; 00220 00221 /*---------------------------------------------------------------------- 00222 | AP4_OmaDcfCtrSampleEncrypter 00223 +---------------------------------------------------------------------*/ 00224 class AP4_OmaDcfCtrSampleEncrypter : public AP4_OmaDcfSampleEncrypter 00225 { 00226 public: 00227 // constructor and destructor 00228 AP4_OmaDcfCtrSampleEncrypter(AP4_BlockCipher* block_cipher, 00229 const AP4_UI08* salt); // salt is only 8 bytes 00230 ~AP4_OmaDcfCtrSampleEncrypter(); 00231 00232 // methods 00233 virtual AP4_Result EncryptSampleData(AP4_DataBuffer& data_in, 00234 AP4_DataBuffer& data_out, 00235 AP4_UI64 bso, 00236 bool skip_encryption); 00237 virtual AP4_Size GetEncryptedSampleSize(AP4_Sample& sample); 00238 00239 private: 00240 // members 00241 AP4_CtrStreamCipher* m_Cipher; 00242 }; 00243 00244 /*---------------------------------------------------------------------- 00245 | AP4_OmaDcfCbcSampleEncrypter 00246 +---------------------------------------------------------------------*/ 00247 class AP4_OmaDcfCbcSampleEncrypter : public AP4_OmaDcfSampleEncrypter 00248 { 00249 public: 00250 // constructor and destructor 00251 AP4_OmaDcfCbcSampleEncrypter(AP4_BlockCipher* block_cipher, 00252 const AP4_UI08* salt); // salt is only 8 bytes 00253 ~AP4_OmaDcfCbcSampleEncrypter(); 00254 00255 // methods 00256 virtual AP4_Result EncryptSampleData(AP4_DataBuffer& data_in, 00257 AP4_DataBuffer& data_out, 00258 AP4_UI64 bso, 00259 bool skip_encryption); 00260 virtual AP4_Size GetEncryptedSampleSize(AP4_Sample& sample); 00261 00262 private: 00263 // members 00264 AP4_CbcStreamCipher* m_Cipher; 00265 }; 00266 00267 /*---------------------------------------------------------------------- 00268 | AP4_OmaDcfDecryptingProcessor 00269 +---------------------------------------------------------------------*/ 00274 class AP4_OmaDcfDecryptingProcessor : public AP4_Processor 00275 { 00276 public: 00277 // constructor 00278 AP4_OmaDcfDecryptingProcessor(const AP4_ProtectionKeyMap* key_map = NULL, 00279 AP4_BlockCipherFactory* block_cipher_factory = NULL); 00280 00281 // accessors 00282 AP4_ProtectionKeyMap& GetKeyMap() { return m_KeyMap; } 00283 00284 // methods 00285 virtual AP4_Result Initialize(AP4_AtomParent& top_level, 00286 AP4_ByteStream& stream, 00287 ProgressListener* listener); 00288 00289 private: 00290 // members 00291 AP4_BlockCipherFactory* m_BlockCipherFactory; 00292 AP4_ProtectionKeyMap m_KeyMap; 00293 }; 00294 00295 /*---------------------------------------------------------------------- 00296 | AP4_OmaDcfEncryptingProcessor 00297 +---------------------------------------------------------------------*/ 00298 class AP4_OmaDcfEncryptingProcessor : public AP4_Processor 00299 { 00300 public: 00301 // constructor 00302 AP4_OmaDcfEncryptingProcessor(AP4_OmaDcfCipherMode cipher_mode, 00303 AP4_BlockCipherFactory* block_cipher_factory = NULL); 00304 00305 // accessors 00306 AP4_ProtectionKeyMap& GetKeyMap() { return m_KeyMap; } 00307 AP4_TrackPropertyMap& GetPropertyMap() { return m_PropertyMap; } 00308 00309 // AP4_Processor methods 00310 virtual AP4_Result Initialize(AP4_AtomParent& top_level, 00311 AP4_ByteStream& stream, 00312 AP4_Processor::ProgressListener* listener = NULL); 00313 virtual AP4_Processor::TrackHandler* CreateTrackHandler(AP4_TrakAtom* trak); 00314 00315 private: 00316 // members 00317 AP4_OmaDcfCipherMode m_CipherMode; 00318 AP4_BlockCipherFactory* m_BlockCipherFactory; 00319 AP4_ProtectionKeyMap m_KeyMap; 00320 AP4_TrackPropertyMap m_PropertyMap; 00321 }; 00322 00323 /*---------------------------------------------------------------------- 00324 | AP4_OmaDrmInfo 00325 +---------------------------------------------------------------------*/ 00326 class AP4_OmaDrmInfo 00327 { 00328 public: 00329 AP4_IMPLEMENT_DYNAMIC_CAST(AP4_OmaDrmInfo) 00330 virtual ~AP4_OmaDrmInfo() {} 00331 virtual const AP4_String& GetContentId() const = 0; 00332 virtual const AP4_String& GetRightsIssuerUrl() const = 0; 00333 virtual const AP4_DataBuffer& GetTextualHeaders() const = 0; 00334 }; 00335 00336 00337 #endif // _AP4_OMA_DCF_H_
Generated on Thu May 13 16:36:33 2010 for Bento4 MP4 SDK by
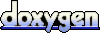