Ap4Mpeg2Ts.h
Go to the documentation of this file.00001 /***************************************************************** 00002 | 00003 | AP4 - MPEG2 Transport Streams 00004 | 00005 | Copyright 2002-2009 Axiomatic Systems, LLC 00006 | 00007 | 00008 | This file is part of Bento4/AP4 (MP4 Atom Processing Library). 00009 | 00010 | Unless you have obtained Bento4 under a difference license, 00011 | this version of Bento4 is Bento4|GPL. 00012 | Bento4|GPL is free software; you can redistribute it and/or modify 00013 | it under the terms of the GNU General Public License as published by 00014 | the Free Software Foundation; either version 2, or (at your option) 00015 | any later version. 00016 | 00017 | Bento4|GPL is distributed in the hope that it will be useful, 00018 | but WITHOUT ANY WARRANTY; without even the implied warranty of 00019 | MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00020 | GNU General Public License for more details. 00021 | 00022 | You should have received a copy of the GNU General Public License 00023 | along with Bento4|GPL; see the file COPYING. If not, write to the 00024 | Free Software Foundation, 59 Temple Place - Suite 330, Boston, MA 00025 | 02111-1307, USA. 00026 | 00027 ****************************************************************/ 00028 00029 #ifndef _AP4_MPEG2_TS_H_ 00030 #define _AP4_MPEG2_TS_H_ 00031 00032 /*---------------------------------------------------------------------- 00033 | includes 00034 +---------------------------------------------------------------------*/ 00035 #include "Ap4Types.h" 00036 00037 /*---------------------------------------------------------------------- 00038 | classes 00039 +---------------------------------------------------------------------*/ 00040 class AP4_ByteStream; 00041 class AP4_Sample; 00042 class AP4_SampleDescription; 00043 00044 /*---------------------------------------------------------------------- 00045 | constants 00046 +---------------------------------------------------------------------*/ 00047 const AP4_UI16 AP4_MPEG2_TS_DEFAULT_PID_PMT = 0x100; 00048 const AP4_UI16 AP4_MPEG2_TS_DEFAULT_PID_AUDIO = 0x101; 00049 const AP4_UI16 AP4_MPEG2_TS_DEFAULT_PID_VIDEO = 0x102; 00050 const AP4_UI16 AP4_MPEG2_TS_DEFAULT_STREAM_ID_AUDIO = 0xc0; 00051 const AP4_UI16 AP4_MPEG2_TS_DEFAULT_STREAM_ID_VIDEO = 0xe0; 00052 00053 const AP4_UI08 AP4_MPEG2_STREAM_TYPE_ISO_IEC_13818_7 = 0x0F; 00054 const AP4_UI08 AP4_MPEG2_STREAM_TYPE_AVC = 0x1B; 00055 00056 /*---------------------------------------------------------------------- 00057 | AP4_Mpeg2TsWriter 00058 +---------------------------------------------------------------------*/ 00066 class AP4_Mpeg2TsWriter 00067 { 00068 public: 00069 // classes 00070 class Stream { 00071 public: 00072 Stream(AP4_UI16 pid) : m_PID(pid), m_ContinuityCounter(0) {} 00073 virtual ~Stream() {} 00074 00075 AP4_UI16 GetPID() { return m_PID; } 00076 void WritePacketHeader(bool payload_start, 00077 unsigned int& payload_size, 00078 bool with_pcr, 00079 AP4_UI64 pcr, 00080 AP4_ByteStream& output); 00081 00082 private: 00083 unsigned int m_PID; 00084 unsigned int m_ContinuityCounter; 00085 }; 00086 00087 class SampleStream : public Stream { 00088 public: 00089 SampleStream(AP4_UI16 pid, AP4_UI16 stream_id, AP4_UI08 stream_type, AP4_UI32 timescale) : 00090 Stream(pid), 00091 m_StreamId(stream_id), 00092 m_StreamType(stream_type), 00093 m_TimeScale(timescale) {} 00094 00095 virtual AP4_Result WritePES(const unsigned char* data, 00096 unsigned int data_size, 00097 AP4_UI64 dts, 00098 bool with_dts, 00099 AP4_UI64 pts, 00100 bool with_pcr, 00101 AP4_ByteStream& output); 00102 virtual AP4_Result WriteSample(AP4_Sample& sample, 00103 AP4_SampleDescription* sample_description, 00104 bool with_pcr, 00105 AP4_ByteStream& output) = 0; 00106 00107 unsigned int m_StreamId; 00108 AP4_UI08 m_StreamType; 00109 AP4_UI32 m_TimeScale; 00110 }; 00111 00112 // constructor 00113 AP4_Mpeg2TsWriter(); 00114 ~AP4_Mpeg2TsWriter(); 00115 00116 Stream* GetPAT() { return m_PAT; } 00117 Stream* GetPMT() { return m_PMT; } 00118 AP4_Result WritePAT(AP4_ByteStream& output); 00119 AP4_Result WritePMT(AP4_ByteStream& output); 00120 AP4_Result SetAudioStream(AP4_UI32 timescale, SampleStream*& stream); 00121 AP4_Result SetVideoStream(AP4_UI32 timescale, SampleStream*& stream); 00122 00123 private: 00124 Stream* m_PAT; 00125 Stream* m_PMT; 00126 SampleStream* m_Audio; 00127 SampleStream* m_Video; 00128 }; 00129 00130 #endif // _AP4_MPEG2_TS_H_
Generated on Thu May 13 16:36:33 2010 for Bento4 MP4 SDK by
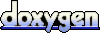