Ap4MetaData.h
Go to the documentation of this file.00001 /***************************************************************** 00002 | 00003 | AP4 - MetaData 00004 | 00005 | Copyright 2002-2008 Axiomatic Systems, LLC 00006 | 00007 | 00008 | This file is part of Bento4/AP4 (MP4 Atom Processing Library). 00009 | 00010 | Unless you have obtained Bento4 under a difference license, 00011 | this version of Bento4 is Bento4|GPL. 00012 | Bento4|GPL is free software; you can redistribute it and/or modify 00013 | it under the terms of the GNU General Public License as published by 00014 | the Free Software Foundation; either version 2, or (at your option) 00015 | any later version. 00016 | 00017 | Bento4|GPL is distributed in the hope that it will be useful, 00018 | but WITHOUT ANY WARRANTY; without even the implied warranty of 00019 | MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00020 | GNU General Public License for more details. 00021 | 00022 | You should have received a copy of the GNU General Public License 00023 | along with Bento4|GPL; see the file COPYING. If not, write to the 00024 | Free Software Foundation, 59 Temple Place - Suite 330, Boston, MA 00025 | 02111-1307, USA. 00026 | 00027 ****************************************************************/ 00028 00029 #ifndef _AP4_META_DATA_H_ 00030 #define _AP4_META_DATA_H_ 00031 00032 /*---------------------------------------------------------------------- 00033 | includes 00034 +---------------------------------------------------------------------*/ 00035 #include "Ap4Atom.h" 00036 #include "Ap4AtomFactory.h" 00037 #include "Ap4String.h" 00038 #include "Ap4Array.h" 00039 #include "Ap4List.h" 00040 00041 /*---------------------------------------------------------------------- 00042 | class references 00043 +---------------------------------------------------------------------*/ 00044 class AP4_File; 00045 class AP4_MoovAtom; 00046 class AP4_DataBuffer; 00047 class AP4_ContainerAtom; 00048 class AP4_DataAtom; 00049 class AP4_3GppLocalizedStringAtom; 00050 class AP4_DcfStringAtom; 00051 class AP4_DcfdAtom; 00052 00053 /*---------------------------------------------------------------------- 00054 | metadata keys 00055 +---------------------------------------------------------------------*/ 00056 const AP4_Atom::Type AP4_ATOM_TYPE_DATA = AP4_ATOM_TYPE('d','a','t','a'); // data 00057 const AP4_Atom::Type AP4_ATOM_TYPE_MEAN = AP4_ATOM_TYPE('m','e','a','n'); // namespace 00058 const AP4_Atom::Type AP4_ATOM_TYPE_NAME = AP4_ATOM_TYPE('n','a','m','e'); // name 00059 const AP4_Atom::Type AP4_ATOM_TYPE_dddd = AP4_ATOM_TYPE('-','-','-','-'); // free form 00060 const AP4_Atom::Type AP4_ATOM_TYPE_cNAM = AP4_ATOM_TYPE(0xA9,'n','a','m'); // name 00061 const AP4_Atom::Type AP4_ATOM_TYPE_cART = AP4_ATOM_TYPE(0xA9,'A','R','T'); // artist 00062 const AP4_Atom::Type AP4_ATOM_TYPE_cCOM = AP4_ATOM_TYPE(0xA9,'c','o','m'); // composer 00063 const AP4_Atom::Type AP4_ATOM_TYPE_cWRT = AP4_ATOM_TYPE(0xA9,'w','r','t'); // writer 00064 const AP4_Atom::Type AP4_ATOM_TYPE_cALB = AP4_ATOM_TYPE(0xA9,'a','l','b'); // album 00065 const AP4_Atom::Type AP4_ATOM_TYPE_cGEN = AP4_ATOM_TYPE(0xA9,'g','e','n'); // genre 00066 const AP4_Atom::Type AP4_ATOM_TYPE_cGRP = AP4_ATOM_TYPE(0xA9,'g','r','p'); // group 00067 const AP4_Atom::Type AP4_ATOM_TYPE_cDAY = AP4_ATOM_TYPE(0xA9,'d','a','y'); // date 00068 const AP4_Atom::Type AP4_ATOM_TYPE_cTOO = AP4_ATOM_TYPE(0xA9,'t','o','o'); // tool 00069 const AP4_Atom::Type AP4_ATOM_TYPE_cCMT = AP4_ATOM_TYPE(0xA9,'c','m','t'); // comment 00070 const AP4_Atom::Type AP4_ATOM_TYPE_cLYR = AP4_ATOM_TYPE(0xA9,'l','y','r'); // lyrics 00071 const AP4_Atom::Type AP4_ATOM_TYPE_TRKN = AP4_ATOM_TYPE('t','r','k','n'); // track# 00072 const AP4_Atom::Type AP4_ATOM_TYPE_DISK = AP4_ATOM_TYPE('d','i','s','k'); // disk# 00073 const AP4_Atom::Type AP4_ATOM_TYPE_COVR = AP4_ATOM_TYPE('c','o','v','r'); // cover art 00074 const AP4_Atom::Type AP4_ATOM_TYPE_DESC = AP4_ATOM_TYPE('d','e','s','c'); // description 00075 const AP4_Atom::Type AP4_ATOM_TYPE_CPIL = AP4_ATOM_TYPE('c','p','i','l'); // compilation? 00076 const AP4_Atom::Type AP4_ATOM_TYPE_TMPO = AP4_ATOM_TYPE('t','m','p','o'); // tempo 00077 const AP4_Atom::Type AP4_ATOM_TYPE_apID = AP4_ATOM_TYPE('a','p','I','D'); 00078 const AP4_Atom::Type AP4_ATOM_TYPE_cnID = AP4_ATOM_TYPE('c','n','I','D'); 00079 const AP4_Atom::Type AP4_ATOM_TYPE_cmID = AP4_ATOM_TYPE('c','m','I','D'); 00080 const AP4_Atom::Type AP4_ATOM_TYPE_atID = AP4_ATOM_TYPE('a','t','I','D'); 00081 const AP4_Atom::Type AP4_ATOM_TYPE_plID = AP4_ATOM_TYPE('p','l','I','D'); 00082 const AP4_Atom::Type AP4_ATOM_TYPE_geID = AP4_ATOM_TYPE('g','e','I','D'); 00083 const AP4_Atom::Type AP4_ATOM_TYPE_sfID = AP4_ATOM_TYPE('s','f','I','D'); 00084 const AP4_Atom::Type AP4_ATOM_TYPE_akID = AP4_ATOM_TYPE('a','k','I','D'); 00085 const AP4_Atom::Type AP4_ATOM_TYPE_aART = AP4_ATOM_TYPE('a','A','R','T'); 00086 const AP4_Atom::Type AP4_ATOM_TYPE_TVNN = AP4_ATOM_TYPE('t','v','n','n'); // TV network 00087 const AP4_Atom::Type AP4_ATOM_TYPE_TVSH = AP4_ATOM_TYPE('t','v','s','h'); // TV show 00088 const AP4_Atom::Type AP4_ATOM_TYPE_TVEN = AP4_ATOM_TYPE('t','v','e','n'); // TV episode name 00089 const AP4_Atom::Type AP4_ATOM_TYPE_TVSN = AP4_ATOM_TYPE('t','v','s','n'); // TV show season # 00090 const AP4_Atom::Type AP4_ATOM_TYPE_TVES = AP4_ATOM_TYPE('t','v','e','s'); // TV show episode # 00091 const AP4_Atom::Type AP4_ATOM_TYPE_STIK = AP4_ATOM_TYPE('s','t','i','k'); 00092 const AP4_Atom::Type AP4_ATOM_TYPE_PCST = AP4_ATOM_TYPE('p','c','s','t'); // Podcast? 00093 const AP4_Atom::Type AP4_ATOM_TYPE_PURD = AP4_ATOM_TYPE('p','u','r','d'); // 00094 const AP4_Atom::Type AP4_ATOM_TYPE_PURL = AP4_ATOM_TYPE('p','u','r','l'); // Podcast URL (binary) 00095 const AP4_Atom::Type AP4_ATOM_TYPE_EGID = AP4_ATOM_TYPE('e','g','i','d'); // 00096 const AP4_Atom::Type AP4_ATOM_TYPE_PGAP = AP4_ATOM_TYPE('p','g','a','p'); // Gapless Playback 00097 const AP4_Atom::Type AP4_ATOM_TYPE_CATG = AP4_ATOM_TYPE('c','a','t','g'); // Category 00098 const AP4_Atom::Type AP4_ATOM_TYPE_KEYW = AP4_ATOM_TYPE('k','e','y','w'); // Keywords 00099 const AP4_Atom::Type AP4_ATOM_TYPE_SONM = AP4_ATOM_TYPE('s','o','n','m'); // Sort-Order: Name 00100 const AP4_Atom::Type AP4_ATOM_TYPE_SOAL = AP4_ATOM_TYPE('s','o','a','l'); // Sort-Order: Album 00101 const AP4_Atom::Type AP4_ATOM_TYPE_SOAR = AP4_ATOM_TYPE('s','o','a','r'); // Sort-Order: Artist 00102 const AP4_Atom::Type AP4_ATOM_TYPE_SOAA = AP4_ATOM_TYPE('s','o','a','a'); // Sort-Order: Album Artist 00103 const AP4_Atom::Type AP4_ATOM_TYPE_SOCO = AP4_ATOM_TYPE('s','o','c','o'); // Sort-Order: Composer 00104 const AP4_Atom::Type AP4_ATOM_TYPE_SOSN = AP4_ATOM_TYPE('s','o','s','n'); // Sort-Order: Show 00105 00106 const AP4_Atom::Type AP4_ATOM_TYPE_TITL = AP4_ATOM_TYPE('t','i','t','l'); // 3GPP: title 00107 const AP4_Atom::Type AP4_ATOM_TYPE_DSCP = AP4_ATOM_TYPE('d','s','c','p'); // 3GPP: description 00108 const AP4_Atom::Type AP4_ATOM_TYPE_CPRT = AP4_ATOM_TYPE('c','p','r','t'); // 3GPP, ISO or ILST: copyright 00109 const AP4_Atom::Type AP4_ATOM_TYPE_PERF = AP4_ATOM_TYPE('p','e','r','f'); // 3GPP: performer 00110 const AP4_Atom::Type AP4_ATOM_TYPE_AUTH = AP4_ATOM_TYPE('a','u','t','h'); // 3GPP: author 00111 const AP4_Atom::Type AP4_ATOM_TYPE_GNRE = AP4_ATOM_TYPE('g','n','r','e'); // 3GPP or ILST: genre (in 3GPP -> string, in ILST -> ID3v1 index + 1) 00112 const AP4_Atom::Type AP4_ATOM_TYPE_RTNG = AP4_ATOM_TYPE('r','t','n','g'); // 3GPP or ILST: rating 00113 const AP4_Atom::Type AP4_ATOM_TYPE_CLSF = AP4_ATOM_TYPE('c','l','s','f'); // 3GPP: classification 00114 const AP4_Atom::Type AP4_ATOM_TYPE_KYWD = AP4_ATOM_TYPE('k','y','w','d'); // 3GPP: keywords 00115 const AP4_Atom::Type AP4_ATOM_TYPE_LOCI = AP4_ATOM_TYPE('l','o','c','i'); // 3GPP: location information 00116 const AP4_Atom::Type AP4_ATOM_TYPE_ALBM = AP4_ATOM_TYPE('a','l','b','m'); // 3GPP: album title and track number 00117 const AP4_Atom::Type AP4_ATOM_TYPE_YRRC = AP4_ATOM_TYPE('y','r','r','c'); // 3GPP: recording year 00118 const AP4_Atom::Type AP4_ATOM_TYPE_TSEL = AP4_ATOM_TYPE('t','s','e','l'); // 3GPP: track selection 00119 00120 const AP4_Atom::Type AP4_ATOM_TYPE_ICNU = AP4_ATOM_TYPE('i','c','n','u'); // DCF: icon URI (OMA DCF 2.1) 00121 const AP4_Atom::Type AP4_ATOM_TYPE_INFU = AP4_ATOM_TYPE('i','n','f','u'); // DCF: info URI (OMA DCF 2.1) 00122 const AP4_Atom::Type AP4_ATOM_TYPE_CVRU = AP4_ATOM_TYPE('c','v','r','u'); // DCF: cover art URI (OMA DCF 2.1) 00123 const AP4_Atom::Type AP4_ATOM_TYPE_LRCU = AP4_ATOM_TYPE('l','r','c','u'); // DCF: lyrics URI (OMA DCF 2.1) 00124 const AP4_Atom::Type AP4_ATOM_TYPE_DCFD = AP4_ATOM_TYPE('d','c','f','D'); // DCF: duration (OMarlin) 00125 00126 /*---------------------------------------------------------------------- 00127 | AP4_MetaData 00128 +---------------------------------------------------------------------*/ 00129 class AP4_MetaData { 00130 public: 00131 class Key { 00132 public: 00133 // constructors 00134 Key(const char* name, const char* ns) : 00135 m_Name(name), m_Namespace(ns) {} 00136 00137 // methods 00138 const AP4_String& GetNamespace() const { return m_Namespace; } 00139 const AP4_String& GetName() const { return m_Name; } 00140 00141 private: 00142 // members 00143 const AP4_String m_Name; 00144 const AP4_String m_Namespace; 00145 }; 00146 00147 class Value { 00148 public: 00149 // types 00150 typedef enum { 00151 TYPE_BINARY, 00152 TYPE_STRING_UTF_8, 00153 TYPE_STRING_UTF_16, 00154 TYPE_STRING_PASCAL, 00155 TYPE_GIF, 00156 TYPE_JPEG, 00157 TYPE_INT_08_BE, 00158 TYPE_INT_16_BE, 00159 TYPE_INT_32_BE, 00160 TYPE_FLOAT_32_BE, 00161 TYPE_FLOAT_64_BE 00162 } Type; 00163 00164 typedef enum { 00165 TYPE_CATEGORY_STRING, 00166 TYPE_CATEGORY_BINARY, 00167 TYPE_CATEGORY_INTEGER, 00168 TYPE_CATEGORY_FLOAT 00169 } TypeCategory; 00170 00171 typedef enum { 00172 MEANING_UNKNOWN, 00173 MEANING_ID3_GENRE, 00174 MEANING_BOOLEAN, 00175 MEANING_FILE_KIND, 00176 MEANING_BINARY_ENCODED_CHARS 00177 } Meaning; 00178 00179 // destructor 00180 virtual ~Value() {} 00181 00182 // methods 00183 Type GetType() const { return m_Type; } 00184 TypeCategory GetTypeCategory() const; 00185 Meaning GetMeaning() const { return m_Meaning; } 00186 const AP4_String& GetLanguage() const { return m_Language; } 00187 void SetLanguage(const char* language) { m_Language = language; } 00188 virtual AP4_String ToString() const = 0; 00189 virtual AP4_Result ToBytes(AP4_DataBuffer& bytes) const = 0; 00190 virtual long ToInteger() const = 0; 00191 00192 protected: 00193 // class methods 00194 static TypeCategory MapTypeToCategory(Type type); 00195 00196 // constructor 00197 Value(Type type, 00198 Meaning meaning = MEANING_UNKNOWN, 00199 const char* language = NULL) : 00200 m_Type(type), m_Meaning(meaning), m_Language(language) {} 00201 00202 // members 00203 Type m_Type; 00204 Meaning m_Meaning; 00205 AP4_String m_Language; 00206 }; 00207 00208 class KeyInfo { 00209 public: 00210 // members 00211 const char* name; 00212 const char* description; 00213 AP4_UI32 four_cc; 00214 Value::Type value_type; 00215 }; 00216 00217 class Entry { 00218 public: 00219 // constructor 00220 Entry(const char* name, const char* ns, Value* value) : 00221 m_Key(name, ns), m_Value(value) {} 00222 00223 // destructor 00224 ~Entry() { delete m_Value; } 00225 00226 // methods 00227 AP4_Result AddToFile(AP4_File& file, AP4_Ordinal index = 0); 00228 AP4_Result AddToFileIlst(AP4_File& file, AP4_Ordinal index = 0); 00229 AP4_Result AddToFileDcf(AP4_File& file, AP4_Ordinal index = 0); 00230 AP4_Result RemoveFromFile(AP4_File& file, AP4_Ordinal index); 00231 AP4_Result RemoveFromFileIlst(AP4_File& file, AP4_Ordinal index); 00232 AP4_Result RemoveFromFileDcf(AP4_File& file, AP4_Ordinal index); 00233 AP4_ContainerAtom* FindInIlst(AP4_ContainerAtom* ilst) const; 00234 AP4_Result ToAtom(AP4_Atom*& atom) const; 00235 00236 // members 00237 Key m_Key; 00238 Value* m_Value; 00239 }; 00240 00241 // class members 00242 static AP4_Array<KeyInfo> KeyInfos; 00243 00244 // constructor 00245 AP4_MetaData(AP4_File* file); 00246 00247 // methods 00248 AP4_Result ParseMoov(AP4_MoovAtom* moov); 00249 AP4_Result ParseUdta(AP4_ContainerAtom* udta, const char* namespc); 00250 00251 // destructor 00252 ~AP4_MetaData(); 00253 00254 // accessors 00255 const AP4_List<Entry>& GetEntries() const { return m_Entries; } 00256 00257 // methods 00258 AP4_Result ResolveKeyName(AP4_Atom::Type atom_type, AP4_String& value); 00259 AP4_Result AddIlstEntries(AP4_ContainerAtom* atom, const char* namespc); 00260 AP4_Result Add3GppEntry(AP4_3GppLocalizedStringAtom* atom, const char* namespc); 00261 AP4_Result AddDcfStringEntry(AP4_DcfStringAtom* atom, const char* namespc); 00262 AP4_Result AddDcfdEntry(AP4_DcfdAtom* atom, const char* namespc); 00263 00264 private: 00265 // members 00266 AP4_List<Entry> m_Entries; 00267 }; 00268 00269 /*---------------------------------------------------------------------- 00270 | AP4_MetaDataAtomTypeHandler 00271 +---------------------------------------------------------------------*/ 00272 class AP4_MetaDataAtomTypeHandler : public AP4_AtomFactory::TypeHandler 00273 { 00274 public: 00275 // constructor 00276 AP4_MetaDataAtomTypeHandler(AP4_AtomFactory* atom_factory) : 00277 m_AtomFactory(atom_factory) {} 00278 virtual AP4_Result CreateAtom(AP4_Atom::Type type, 00279 AP4_UI32 size, 00280 AP4_ByteStream& stream, 00281 AP4_Atom::Type context, 00282 AP4_Atom*& atom); 00283 00284 // types 00285 struct TypeList { 00286 const AP4_Atom::Type* m_Types; 00287 AP4_Size m_Size; 00288 }; 00289 00290 // class constants 00291 static const AP4_Atom::Type IlstTypes[]; 00292 static const TypeList IlstTypeList; 00293 static const AP4_Atom::Type _3gppLocalizedStringTypes[]; 00294 static const TypeList _3gppLocalizedStringTypeList; 00295 static const AP4_Atom::Type _3gppOtherTypes[]; 00296 static const TypeList _3gppOtherTypeList; 00297 static const AP4_Atom::Type DcfStringTypes[]; 00298 static const TypeList DcfStringTypeList; 00299 00300 // class methods 00301 static bool IsTypeInList(AP4_Atom::Type type, const TypeList& list); 00302 00303 private: 00304 // members 00305 AP4_AtomFactory* m_AtomFactory; 00306 }; 00307 00308 /*---------------------------------------------------------------------- 00309 | AP4_MetaDataTag 00310 +---------------------------------------------------------------------*/ 00311 class AP4_MetaDataTag 00312 { 00313 public: 00314 00315 // destructor 00316 virtual ~AP4_MetaDataTag() {} 00317 00318 // methods 00319 virtual AP4_Result Write(AP4_ByteStream& stream); 00320 virtual AP4_Result Inspect(AP4_AtomInspector& inspector); 00321 00322 protected: 00323 // constructor 00324 AP4_MetaDataTag(AP4_UI32 data_type, 00325 AP4_UI32 data_lang, 00326 AP4_Size size, 00327 AP4_ByteStream& stream); 00328 00329 }; 00330 00331 /*---------------------------------------------------------------------- 00332 | AP4_3GppLocalizedStringAtom 00333 +---------------------------------------------------------------------*/ 00334 class AP4_3GppLocalizedStringAtom : public AP4_Atom 00335 { 00336 public: 00337 AP4_IMPLEMENT_DYNAMIC_CAST_D(AP4_3GppLocalizedStringAtom, AP4_Atom) 00338 00339 // factory method 00340 static AP4_3GppLocalizedStringAtom* Create(Type type, AP4_UI32 size, AP4_ByteStream& stream); 00341 00342 // constructor 00343 AP4_3GppLocalizedStringAtom(Type type, const char* language, const char* value); 00344 AP4_3GppLocalizedStringAtom(Type type, 00345 AP4_UI32 size, 00346 AP4_UI32 version, 00347 AP4_UI32 flags, 00348 AP4_ByteStream& stream); 00349 00350 // AP4_Atom methods 00351 virtual AP4_Result InspectFields(AP4_AtomInspector& inspector); 00352 virtual AP4_Result WriteFields(AP4_ByteStream& stream); 00353 00354 // methods 00355 const char* GetLanguage() const { return m_Language; } 00356 const AP4_String& GetValue() const { return m_Value; } 00357 00358 private: 00359 // members 00360 char m_Language[4]; 00361 AP4_String m_Value; 00362 }; 00363 00364 /*---------------------------------------------------------------------- 00365 | AP4_DcfStringAtom 00366 +---------------------------------------------------------------------*/ 00367 class AP4_DcfStringAtom : public AP4_Atom 00368 { 00369 public: 00370 AP4_IMPLEMENT_DYNAMIC_CAST_D(AP4_DcfStringAtom, AP4_Atom) 00371 00372 // factory method 00373 static AP4_DcfStringAtom* Create(Type type, AP4_UI32 size, AP4_ByteStream& stream); 00374 00375 // constructor 00376 AP4_DcfStringAtom(Type type, const char* value); 00377 AP4_DcfStringAtom(Type type, 00378 AP4_UI32 size, 00379 AP4_UI32 version, 00380 AP4_UI32 flags, 00381 AP4_ByteStream& stream); 00382 00383 // AP4_Atom methods 00384 virtual AP4_Result InspectFields(AP4_AtomInspector& inspector); 00385 virtual AP4_Result WriteFields(AP4_ByteStream& stream); 00386 00387 // methods 00388 const AP4_String& GetValue() const { return m_Value; } 00389 00390 private: 00391 // members 00392 AP4_String m_Value; 00393 }; 00394 00395 /*---------------------------------------------------------------------- 00396 | AP4_DcfdAtom 00397 +---------------------------------------------------------------------*/ 00398 class AP4_DcfdAtom : public AP4_Atom 00399 { 00400 public: 00401 AP4_IMPLEMENT_DYNAMIC_CAST_D(AP4_DcfdAtom, AP4_Atom) 00402 00403 // factory method 00404 static AP4_DcfdAtom* Create(AP4_UI32 size, AP4_ByteStream& stream); 00405 00406 // constructors 00407 AP4_DcfdAtom(AP4_UI32 version, 00408 AP4_UI32 flags, 00409 AP4_ByteStream& stream); 00410 AP4_DcfdAtom(AP4_UI32 duration); 00411 00412 // AP4_Atom methods 00413 virtual AP4_Result InspectFields(AP4_AtomInspector& inspector); 00414 virtual AP4_Result WriteFields(AP4_ByteStream& stream); 00415 00416 // methods 00417 AP4_UI32 GetDuration() const { return m_Duration; } 00418 00419 private: 00420 // members 00421 AP4_UI32 m_Duration; 00422 }; 00423 00424 /*---------------------------------------------------------------------- 00425 | AP4_MetaDataStringAtom 00426 +---------------------------------------------------------------------*/ 00427 class AP4_MetaDataStringAtom : public AP4_Atom 00428 { 00429 public: 00430 // constructors 00431 AP4_MetaDataStringAtom(Type type, const char* value); 00432 AP4_MetaDataStringAtom(Type type, AP4_UI32 size, AP4_ByteStream& stream); 00433 00434 // AP4_Atom methods 00435 virtual AP4_Result InspectFields(AP4_AtomInspector& inspector); 00436 virtual AP4_Result WriteFields(AP4_ByteStream& stream); 00437 00438 // methods 00439 const AP4_String& GetValue() { return m_Value; } 00440 00441 private: 00442 // members 00443 AP4_UI32 m_Reserved; 00444 AP4_String m_Value; 00445 }; 00446 00447 /*---------------------------------------------------------------------- 00448 | AP4_DataAtom 00449 +---------------------------------------------------------------------*/ 00450 class AP4_DataAtom : public AP4_Atom 00451 { 00452 public: 00453 AP4_IMPLEMENT_DYNAMIC_CAST_D(AP4_DataAtom, AP4_Atom) 00454 00455 typedef enum { 00456 DATA_TYPE_BINARY = 0, 00457 DATA_TYPE_STRING_UTF_8 = 1, 00458 DATA_TYPE_STRING_UTF_16 = 2, 00459 DATA_TYPE_STRING_PASCAL = 3, 00460 DATA_TYPE_GIF = 13, 00461 DATA_TYPE_JPEG = 14, 00462 DATA_TYPE_SIGNED_INT_BE = 21, /* the size of the integer is derived from the container size */ 00463 DATA_TYPE_FLOAT_32_BE = 22, 00464 DATA_TYPE_FLOAT_64_BE = 23 00465 } DataType; 00466 00467 typedef enum { 00468 LANGUAGE_ENGLISH = 0 00469 } DataLang; 00470 00471 // constructors 00472 AP4_DataAtom(const AP4_MetaData::Value& value); 00473 AP4_DataAtom(AP4_UI32 size, AP4_ByteStream& stream); 00474 00475 // destructor 00476 ~AP4_DataAtom(); 00477 00478 // AP4_Atom methods 00479 virtual AP4_Result InspectFields(AP4_AtomInspector& inspector); 00480 virtual AP4_Result WriteFields(AP4_ByteStream& stream); 00481 00482 // accessors 00483 DataType GetDataType() { return m_DataType; } 00484 DataLang GetDataLang() { return m_DataLang; } 00485 AP4_MetaData::Value::Type GetValueType(); 00486 00487 // methods 00488 AP4_Result LoadString(AP4_String*& string); 00489 AP4_Result LoadBytes(AP4_DataBuffer& bytes); 00490 AP4_Result LoadInteger(long& value); 00491 00492 private: 00493 // members 00494 DataType m_DataType; 00495 DataLang m_DataLang; 00496 AP4_ByteStream* m_Source; 00497 }; 00498 00499 /*---------------------------------------------------------------------- 00500 | AP4_StringMetaDataValue 00501 +---------------------------------------------------------------------*/ 00502 class AP4_StringMetaDataValue : public AP4_MetaData::Value { 00503 public: 00504 // constructor 00505 AP4_StringMetaDataValue(const char* value, const char* language=NULL) : 00506 Value(TYPE_STRING_UTF_8, MEANING_UNKNOWN, language), 00507 m_Value(value) {} 00508 00509 // AP4_MetaData::Value methods 00510 virtual AP4_String ToString() const; 00511 virtual AP4_Result ToBytes(AP4_DataBuffer& bytes) const; 00512 virtual long ToInteger() const; 00513 00514 private: 00515 // members 00516 AP4_String m_Value; 00517 }; 00518 00519 /*---------------------------------------------------------------------- 00520 | AP4_IntegerMetaDataValue 00521 +---------------------------------------------------------------------*/ 00522 class AP4_IntegerMetaDataValue : public AP4_MetaData::Value { 00523 public: 00524 // constructor 00525 AP4_IntegerMetaDataValue(Type type, long value) : 00526 Value(type), m_Value(value) {} 00527 00528 // AP4_MetaData::Value methods 00529 virtual AP4_String ToString() const; 00530 virtual AP4_Result ToBytes(AP4_DataBuffer& bytes) const; 00531 virtual long ToInteger() const; 00532 00533 private: 00534 // members 00535 long m_Value; 00536 }; 00537 00538 /*---------------------------------------------------------------------- 00539 | AP4_BinaryMetaDataValue 00540 +---------------------------------------------------------------------*/ 00541 class AP4_BinaryMetaDataValue : public AP4_MetaData::Value { 00542 public: 00543 // constructor 00544 AP4_BinaryMetaDataValue(Type type, const AP4_UI08* data, AP4_Size size) : 00545 Value(type), m_Value(data, size) {} 00546 00547 // AP4_MetaData::Value methods 00548 virtual AP4_String ToString() const; 00549 virtual AP4_Result ToBytes(AP4_DataBuffer& bytes) const; 00550 virtual long ToInteger() const; 00551 00552 private: 00553 // members 00554 AP4_DataBuffer m_Value; 00555 }; 00556 00557 /*---------------------------------------------------------------------- 00558 | AP4_AtomMetaDataValue 00559 +---------------------------------------------------------------------*/ 00560 class AP4_AtomMetaDataValue : public AP4_MetaData::Value 00561 { 00562 public: 00563 // constructor 00564 AP4_AtomMetaDataValue(AP4_DataAtom* data_atom, AP4_UI32 parent_type); 00565 00566 // AP4_MetaData::Value methods 00567 virtual AP4_String ToString() const; 00568 virtual AP4_Result ToBytes(AP4_DataBuffer& bytes) const; 00569 virtual long ToInteger() const; 00570 00571 private: 00572 // members 00573 AP4_DataAtom* m_DataAtom; 00574 }; 00575 00576 #endif // _AP4_META_DATA_H_
Generated on Thu May 13 16:36:32 2010 for Bento4 MP4 SDK by
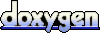