Ap4Marlin.h
Go to the documentation of this file.00001 /***************************************************************** 00002 | 00003 | AP4 - Marlin File Format support 00004 | 00005 | Copyright 2002-2008 Axiomatic Systems, LLC 00006 | 00007 | 00008 | This file is part of Bento4/AP4 (MP4 Atom Processing Library). 00009 | 00010 | Unless you have obtained Bento4 under a difference license, 00011 | this version of Bento4 is Bento4|GPL. 00012 | Bento4|GPL is free software; you can redistribute it and/or modify 00013 | it under the terms of the GNU General Public License as published by 00014 | the Free Software Foundation; either version 2, or (at your option) 00015 | any later version. 00016 | 00017 | Bento4|GPL is distributed in the hope that it will be useful, 00018 | but WITHOUT ANY WARRANTY; without even the implied warranty of 00019 | MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00020 | GNU General Public License for more details. 00021 | 00022 | You should have received a copy of the GNU General Public License 00023 | along with Bento4|GPL; see the file COPYING. If not, write to the 00024 | Free Software Foundation, 59 Temple Place - Suite 330, Boston, MA 00025 | 02111-1307, USA. 00026 | 00027 ****************************************************************/ 00028 00029 #ifndef _AP4_MARLIN_H_ 00030 #define _AP4_MARLIN_H_ 00031 00032 /*---------------------------------------------------------------------- 00033 | includes 00034 +---------------------------------------------------------------------*/ 00035 #include "Ap4Types.h" 00036 #include "Ap4SampleEntry.h" 00037 #include "Ap4Atom.h" 00038 #include "Ap4AtomFactory.h" 00039 #include "Ap4SampleDescription.h" 00040 #include "Ap4Processor.h" 00041 #include "Ap4Protection.h" 00042 #include "Ap4TrefTypeAtom.h" 00043 #include "Ap4ObjectDescriptor.h" 00044 #include "Ap4Command.h" 00045 #include "Ap4UuidAtom.h" 00046 #include "Ap4OmaDcf.h" 00047 00048 /*---------------------------------------------------------------------- 00049 | constants 00050 +---------------------------------------------------------------------*/ 00051 const AP4_UI32 AP4_MARLIN_BRAND_MGSV = AP4_ATOM_TYPE('M','G','S','V'); 00052 const AP4_UI16 AP4_MARLIN_IPMPS_TYPE_MGSV = 0xA551; 00053 const AP4_UI32 AP4_PROTECTION_SCHEME_TYPE_MARLIN_ACBC = AP4_ATOM_TYPE('A','C','B','C'); 00054 const AP4_UI32 AP4_PROTECTION_SCHEME_TYPE_MARLIN_ACGK = AP4_ATOM_TYPE('A','C','G','K'); 00055 00056 const AP4_Atom::Type AP4_ATOM_TYPE_SATR = AP4_ATOM_TYPE('s','a','t','r'); 00057 const AP4_Atom::Type AP4_ATOM_TYPE_STYP = AP4_ATOM_TYPE('s','t','y','p'); 00058 const AP4_Atom::Type AP4_ATOM_TYPE_HMAC = AP4_ATOM_TYPE('h','m','a','c'); 00059 const AP4_Atom::Type AP4_ATOM_TYPE_GKEY = AP4_ATOM_TYPE('g','k','e','y'); 00060 00061 const char* const AP4_MARLIN_IPMP_STYP_VIDEO = "urn:marlin:organization:sne:content-type:video"; 00062 const char* const AP4_MARLIN_IPMP_STYP_AUDIO = "urn:marlin:organization:sne:content-type:audio"; 00063 00064 /*---------------------------------------------------------------------- 00065 | AP4_MarlinIpmpParser 00066 +---------------------------------------------------------------------*/ 00067 class AP4_MarlinIpmpParser 00068 { 00069 public: 00070 // types 00071 struct SinfEntry { 00072 SinfEntry(AP4_UI32 track_id, AP4_ContainerAtom* sinf) : 00073 m_TrackId(track_id), m_Sinf(sinf) {} 00074 ~SinfEntry() { delete m_Sinf; } 00075 AP4_UI32 m_TrackId; 00076 AP4_ContainerAtom* m_Sinf; 00077 }; 00078 00079 // methods 00080 static AP4_Result Parse(AP4_AtomParent& top_level, 00081 AP4_ByteStream& stream, 00082 AP4_List<SinfEntry>& sinf_entries, 00083 bool remove_od_data=false); 00084 00085 private: 00086 AP4_MarlinIpmpParser() {} // this class can't be instantiated 00087 }; 00088 00089 /*---------------------------------------------------------------------- 00090 | AP4_MarlinIpmpDecryptingProcessor 00091 +---------------------------------------------------------------------*/ 00092 class AP4_MarlinIpmpDecryptingProcessor : public AP4_Processor 00093 { 00094 public: 00095 // constructor and destructor 00096 AP4_MarlinIpmpDecryptingProcessor(const AP4_ProtectionKeyMap* key_map = NULL, 00097 AP4_BlockCipherFactory* block_cipher_factory = NULL); 00098 ~AP4_MarlinIpmpDecryptingProcessor(); 00099 00100 // accessors 00101 AP4_ProtectionKeyMap& GetKeyMap() { return m_KeyMap; } 00102 00103 // methods 00104 virtual AP4_Result Initialize(AP4_AtomParent& top_level, 00105 AP4_ByteStream& stream, 00106 ProgressListener* listener); 00107 virtual AP4_Processor::TrackHandler* CreateTrackHandler(AP4_TrakAtom* trak); 00108 00109 private: 00110 00111 // members 00112 AP4_BlockCipherFactory* m_BlockCipherFactory; 00113 AP4_ProtectionKeyMap m_KeyMap; 00114 AP4_List<AP4_MarlinIpmpParser::SinfEntry> m_SinfEntries; 00115 }; 00116 00117 /*---------------------------------------------------------------------- 00118 | AP4_MarlinIpmpTrackDecrypter 00119 +---------------------------------------------------------------------*/ 00120 class AP4_MarlinIpmpTrackDecrypter : public AP4_Processor::TrackHandler 00121 { 00122 public: 00123 // class methods 00124 static AP4_Result Create(AP4_BlockCipherFactory& cipher_factory, 00125 const AP4_UI08* key, 00126 AP4_MarlinIpmpTrackDecrypter*& decrypter); 00127 00128 // destructor 00129 ~AP4_MarlinIpmpTrackDecrypter(); 00130 00131 // AP4_Processor::TrackHandler methods 00132 virtual AP4_Size GetProcessedSampleSize(AP4_Sample& sample); 00133 virtual AP4_Result ProcessSample(AP4_DataBuffer& data_in, 00134 AP4_DataBuffer& data_out); 00135 00136 00137 private: 00138 // constructor 00139 AP4_MarlinIpmpTrackDecrypter(AP4_StreamCipher* cipher) : m_Cipher(cipher) {} 00140 00141 // members 00142 AP4_StreamCipher* m_Cipher; 00143 }; 00144 00145 /*---------------------------------------------------------------------- 00146 | AP4_MarlinIpmpEncryptingProcessor 00147 +---------------------------------------------------------------------*/ 00148 class AP4_MarlinIpmpEncryptingProcessor : public AP4_Processor 00149 { 00150 public: 00151 // constructor 00152 AP4_MarlinIpmpEncryptingProcessor(bool use_group_key = false, 00153 const AP4_ProtectionKeyMap* key_map = NULL, 00154 AP4_BlockCipherFactory* block_cipher_factory = NULL); 00155 00156 // accessors 00157 AP4_ProtectionKeyMap& GetKeyMap() { return m_KeyMap; } 00158 AP4_TrackPropertyMap& GetPropertyMap() { return m_PropertyMap; } 00159 00160 // AP4_Processor methods 00161 virtual AP4_Result Initialize(AP4_AtomParent& top_level, 00162 AP4_ByteStream& stream, 00163 AP4_Processor::ProgressListener* listener = NULL); 00164 virtual AP4_Processor::TrackHandler* CreateTrackHandler(AP4_TrakAtom* trak); 00165 00166 private: 00167 // members 00168 AP4_BlockCipherFactory* m_BlockCipherFactory; 00169 bool m_UseGroupKey; 00170 AP4_ProtectionKeyMap m_KeyMap; 00171 AP4_TrackPropertyMap m_PropertyMap; 00172 }; 00173 00174 /*---------------------------------------------------------------------- 00175 | AP4_MarlinIpmpTrackEncrypter 00176 +---------------------------------------------------------------------*/ 00177 class AP4_MarlinIpmpTrackEncrypter : public AP4_Processor::TrackHandler 00178 { 00179 public: 00180 // class methods 00181 static AP4_Result Create(AP4_BlockCipherFactory& cipher_factory, 00182 const AP4_UI08* key, 00183 const AP4_UI08* iv, 00184 AP4_MarlinIpmpTrackEncrypter*& encrypter); 00185 00186 // destructor 00187 ~AP4_MarlinIpmpTrackEncrypter(); 00188 00189 // AP4_Processor::TrackHandler methods 00190 virtual AP4_Size GetProcessedSampleSize(AP4_Sample& sample); 00191 virtual AP4_Result ProcessSample(AP4_DataBuffer& data_in, 00192 AP4_DataBuffer& data_out); 00193 00194 00195 private: 00196 // constructor 00197 AP4_MarlinIpmpTrackEncrypter(AP4_StreamCipher* cipher, const AP4_UI08* iv); 00198 00199 // members 00200 AP4_UI08 m_IV[16]; 00201 AP4_StreamCipher* m_Cipher; 00202 }; 00203 00204 #endif // _AP4_MARLIN_H_
Generated on Thu May 13 16:36:32 2010 for Bento4 MP4 SDK by
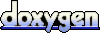