Ap4LinearReader.h
Go to the documentation of this file.00001 /***************************************************************** 00002 | 00003 | AP4 - File Writer 00004 | 00005 | Copyright 2002-2008 Axiomatic Systems, LLC 00006 | 00007 | 00008 | This file is part of Bento4/AP4 (MP4 Atom Processing Library). 00009 | 00010 | Unless you have obtained Bento4 under a difference license, 00011 | this version of Bento4 is Bento4|GPL. 00012 | Bento4|GPL is free software; you can redistribute it and/or modify 00013 | it under the terms of the GNU General Public License as published by 00014 | the Free Software Foundation; either version 2, or (at your option) 00015 | any later version. 00016 | 00017 | Bento4|GPL is distributed in the hope that it will be useful, 00018 | but WITHOUT ANY WARRANTY; without even the implied warranty of 00019 | MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00020 | GNU General Public License for more details. 00021 | 00022 | You should have received a copy of the GNU General Public License 00023 | along with Bento4|GPL; see the file COPYING. If not, write to the 00024 | Free Software Foundation, 59 Temple Place - Suite 330, Boston, MA 00025 | 02111-1307, USA. 00026 | 00027 ****************************************************************/ 00028 00029 #ifndef _AP4_LINEAR_READER_H_ 00030 #define _AP4_LINEAR_READER_H_ 00031 00032 /*---------------------------------------------------------------------- 00033 | includes 00034 +---------------------------------------------------------------------*/ 00035 #include "Ap4Types.h" 00036 #include "Ap4Array.h" 00037 #include "Ap4Movie.h" 00038 #include "Ap4Sample.h" 00039 00040 /*---------------------------------------------------------------------- 00041 | class references 00042 +---------------------------------------------------------------------*/ 00043 class AP4_Track; 00044 00045 /*---------------------------------------------------------------------- 00046 | constants 00047 +---------------------------------------------------------------------*/ 00048 const unsigned int AP4_LINEAR_READER_INITIALIZED = 1; 00049 const unsigned int AP4_LINEAR_READER_FLAG_EOS = 2; 00050 00051 const unsigned int AP4_LINEAR_READER_DEFAULT_BUFFER_SIZE = 4096*1024; 00052 00053 /*---------------------------------------------------------------------- 00054 | AP4_LinearReader 00055 +---------------------------------------------------------------------*/ 00056 class AP4_LinearReader { 00057 public: 00058 AP4_LinearReader(AP4_Movie& movie, AP4_Size max_buffer=AP4_LINEAR_READER_DEFAULT_BUFFER_SIZE); 00059 ~AP4_LinearReader(); 00060 00061 AP4_Result EnableTrack(AP4_UI32 track_id); 00066 AP4_Result ReadNextSample(AP4_Sample& sample, 00067 AP4_DataBuffer& sample_data, 00068 AP4_UI32& track_id); 00072 AP4_Result ReadNextSample(AP4_UI32 track_id, 00073 AP4_Sample& sample, 00074 AP4_DataBuffer& sample_data); 00075 00076 AP4_Result SetSampleIndex(AP4_UI32 track_id, AP4_UI32 sample_index); 00077 00078 // accessors 00079 AP4_Size GetBufferFullness() { return m_BufferFullness; } 00080 00081 private: 00082 class SampleBuffer { 00083 public: 00084 SampleBuffer(AP4_Sample* sample) : m_Sample(sample) {} 00085 ~SampleBuffer() { delete m_Sample; } 00086 AP4_Sample* m_Sample; 00087 AP4_DataBuffer m_Data; 00088 }; 00089 class Tracker { 00090 public: 00091 Tracker(AP4_Track* track) : 00092 m_Eos(false), 00093 m_Track(track), 00094 m_NextSample(NULL), 00095 m_NextSampleIndex(0) {} 00096 Tracker(const Tracker& other) : 00097 m_Eos(other.m_Eos), 00098 m_Track(other.m_Track), 00099 m_NextSample(NULL), 00100 m_NextSampleIndex(other.m_NextSampleIndex) {} // don't copy samples 00101 ~Tracker() { m_Samples.DeleteReferences(); } 00102 bool m_Eos; 00103 AP4_Track* m_Track; 00104 AP4_Sample* m_NextSample; 00105 AP4_Ordinal m_NextSampleIndex; 00106 AP4_List<SampleBuffer> m_Samples; 00107 }; 00108 00109 // methods 00110 Tracker* FindTracker(AP4_UI32 track_id); 00111 AP4_Result Advance(); 00112 bool PopSample(Tracker* tracker, AP4_Sample& sample, AP4_DataBuffer& sample_data); 00113 00114 // members 00115 AP4_Movie& m_Movie; 00116 AP4_Array<Tracker*> m_Trackers; 00117 AP4_Size m_BufferFullness; 00118 AP4_Size m_BufferFullnessPeak; 00119 AP4_Size m_MaxBufferFullness; 00120 }; 00121 00122 #endif // _AP4_LINEAR_READER_H_
Generated on Thu May 13 16:36:32 2010 for Bento4 MP4 SDK by
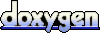