Ap4HintTrackReader.h
Go to the documentation of this file.00001 /***************************************************************** 00002 | 00003 | AP4 - Hint Track Reader 00004 | 00005 | Copyright 2002-2008 Axiomatic Systems, LLC 00006 | 00007 | 00008 | This file is part of Bento4/AP4 (MP4 Atom Processing Library). 00009 | 00010 | Unless you have obtained Bento4 under a difference license, 00011 | this version of Bento4 is Bento4|GPL. 00012 | Bento4|GPL is free software; you can redistribute it and/or modify 00013 | it under the terms of the GNU General Public License as published by 00014 | the Free Software Foundation; either version 2, or (at your option) 00015 | any later version. 00016 | 00017 | Bento4|GPL is distributed in the hope that it will be useful, 00018 | but WITHOUT ANY WARRANTY; without even the implied warranty of 00019 | MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00020 | GNU General Public License for more details. 00021 | 00022 | You should have received a copy of the GNU General Public License 00023 | along with Bento4|GPL; see the file COPYING. If not, write to the 00024 | Free Software Foundation, 59 Temple Place - Suite 330, Boston, MA 00025 | 02111-1307, USA. 00026 | 00027 ****************************************************************/ 00028 00029 #ifndef _AP4_HINT_TRACK_READER_H_ 00030 #define _AP4_HINT_TRACK_READER_H_ 00031 00032 /*---------------------------------------------------------------------- 00033 | includes 00034 +---------------------------------------------------------------------*/ 00035 #include "Ap4Types.h" 00036 #include "Ap4Sample.h" 00037 00038 /*---------------------------------------------------------------------- 00039 | class declarations 00040 +---------------------------------------------------------------------*/ 00041 class AP4_DataBuffer; 00042 class AP4_Movie; 00043 class AP4_Track; 00044 class AP4_RtpSampleData; 00045 class AP4_RtpPacket; 00046 class AP4_ImmediateRtpConstructor; 00047 class AP4_SampleRtpConstructor; 00048 class AP4_String; 00049 00050 /*---------------------------------------------------------------------- 00051 | AP4_HintTrackReader 00052 +---------------------------------------------------------------------*/ 00053 class AP4_HintTrackReader 00054 { 00055 public: 00056 // constructor and destructor 00057 static AP4_Result Create(AP4_Track& hint_track, 00058 AP4_Movie& movie, 00059 AP4_UI32 ssrc, // if 0, a random value is chosen 00060 AP4_HintTrackReader*& reader); 00061 ~AP4_HintTrackReader(); 00062 00063 // methods 00064 AP4_Result GetNextPacket(AP4_DataBuffer& packet, 00065 AP4_UI32& ts_ms); 00066 AP4_Result SeekToTimeStampMs(AP4_UI32 desired_ts_ms, 00067 AP4_UI32& actual_ts_ms); 00068 AP4_UI32 GetCurrentTimeStampMs(); 00069 AP4_Result Rewind(); 00070 AP4_Result GetSdpText(AP4_String& sdp); 00071 AP4_Track* GetMediaTrack() { return m_MediaTrack; } 00072 00073 private: 00074 // use the factory instead of the constructor 00075 AP4_HintTrackReader(AP4_Track& hint_track, 00076 AP4_Movie& movie, 00077 AP4_UI32 ssrc); 00078 00079 // methods 00080 AP4_Result GetRtpSample(AP4_Ordinal index); 00081 AP4_Result BuildRtpPacket(AP4_RtpPacket* packet, 00082 AP4_DataBuffer& packet_data); 00083 AP4_Result WriteImmediateRtpData(AP4_ImmediateRtpConstructor* constructor, 00084 AP4_ByteStream* data_stream); 00085 AP4_Result WriteSampleRtpData(AP4_SampleRtpConstructor* constructor, 00086 AP4_ByteStream* data_stream); 00087 00088 // members 00089 AP4_Track& m_HintTrack; 00090 AP4_Track* m_MediaTrack; 00091 AP4_UI32 m_MediaTimeScale; 00092 AP4_Sample m_CurrentHintSample; 00093 AP4_RtpSampleData* m_RtpSampleData; 00094 AP4_UI32 m_Ssrc; 00095 AP4_Ordinal m_SampleIndex; 00096 AP4_Ordinal m_PacketIndex; 00097 AP4_UI16 m_RtpSequenceStart; 00098 AP4_UI32 m_RtpTimeStampStart; 00099 AP4_UI32 m_RtpTimeScale; 00100 }; 00101 00102 #endif // _AP4_HINT_TRACK_READER_H_
Generated on Thu May 13 16:36:32 2010 for Bento4 MP4 SDK by
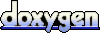