Ap4File.h
Go to the documentation of this file.00001 /***************************************************************** 00002 | 00003 | AP4 - File 00004 | 00005 | Copyright 2002-2008 Axiomatic Systems, LLC 00006 | 00007 | 00008 | This file is part of Bento4/AP4 (MP4 Atom Processing Library). 00009 | 00010 | Unless you have obtained Bento4 under a difference license, 00011 | this version of Bento4 is Bento4|GPL. 00012 | Bento4|GPL is free software; you can redistribute it and/or modify 00013 | it under the terms of the GNU General Public License as published by 00014 | the Free Software Foundation; either version 2, or (at your option) 00015 | any later version. 00016 | 00017 | Bento4|GPL is distributed in the hope that it will be useful, 00018 | but WITHOUT ANY WARRANTY; without even the implied warranty of 00019 | MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00020 | GNU General Public License for more details. 00021 | 00022 | You should have received a copy of the GNU General Public License 00023 | along with Bento4|GPL; see the file COPYING. If not, write to the 00024 | Free Software Foundation, 59 Temple Place - Suite 330, Boston, MA 00025 | 02111-1307, USA. 00026 | 00027 ****************************************************************/ 00028 00029 #ifndef _AP4_FILE_H_ 00030 #define _AP4_FILE_H_ 00031 00032 /*---------------------------------------------------------------------- 00033 | includes 00034 +---------------------------------------------------------------------*/ 00035 #include "Ap4Types.h" 00036 #include "Ap4List.h" 00037 #include "Ap4Atom.h" 00038 #include "Ap4AtomFactory.h" 00039 00040 /*---------------------------------------------------------------------- 00041 | class references 00042 +---------------------------------------------------------------------*/ 00043 class AP4_ByteStream; 00044 class AP4_Movie; 00045 class AP4_FtypAtom; 00046 class AP4_MetaData; 00047 00048 /*---------------------------------------------------------------------- 00049 | file type/brands 00050 +---------------------------------------------------------------------*/ 00051 const AP4_UI32 AP4_FILE_BRAND_QT__ = AP4_ATOM_TYPE('q','t',' ',' '); 00052 const AP4_UI32 AP4_FILE_BRAND_ISOM = AP4_ATOM_TYPE('i','s','o','m'); 00053 const AP4_UI32 AP4_FILE_BRAND_MP41 = AP4_ATOM_TYPE('m','p','4','1'); 00054 const AP4_UI32 AP4_FILE_BRAND_MP42 = AP4_ATOM_TYPE('m','p','4','2'); 00055 const AP4_UI32 AP4_FILE_BRAND_3GP1 = AP4_ATOM_TYPE('3','g','p','1'); 00056 const AP4_UI32 AP4_FILE_BRAND_3GP2 = AP4_ATOM_TYPE('3','g','p','2'); 00057 const AP4_UI32 AP4_FILE_BRAND_3GP3 = AP4_ATOM_TYPE('3','g','p','3'); 00058 const AP4_UI32 AP4_FILE_BRAND_3GP4 = AP4_ATOM_TYPE('3','g','p','4'); 00059 const AP4_UI32 AP4_FILE_BRAND_3GP5 = AP4_ATOM_TYPE('3','g','p','5'); 00060 const AP4_UI32 AP4_FILE_BRAND_3G2A = AP4_ATOM_TYPE('3','g','2','a'); 00061 const AP4_UI32 AP4_FILE_BRAND_MMP4 = AP4_ATOM_TYPE('m','m','p','4'); 00062 const AP4_UI32 AP4_FILE_BRAND_M4A_ = AP4_ATOM_TYPE('M','4','A',' '); 00063 const AP4_UI32 AP4_FILE_BRAND_M4P_ = AP4_ATOM_TYPE('M','4','P',' '); 00064 const AP4_UI32 AP4_FILE_BRAND_MJP2 = AP4_ATOM_TYPE('m','j','p','2'); 00065 00066 /*---------------------------------------------------------------------- 00067 | AP4_File 00068 +---------------------------------------------------------------------*/ 00069 00074 class AP4_File : public AP4_AtomParent { 00075 public: 00076 // constructors and destructor 00081 AP4_File(AP4_Movie* movie = NULL); 00082 00091 AP4_File(AP4_ByteStream& stream, 00092 AP4_AtomFactory& atom_factory = AP4_DefaultAtomFactory::Instance, 00093 bool moov_only = false); 00094 00098 virtual ~AP4_File(); 00099 00103 AP4_List<AP4_Atom>& GetTopLevelAtoms() { return m_Children; } 00104 00108 AP4_Movie* GetMovie() { return m_Movie; } 00109 00110 00114 AP4_FtypAtom* GetFileType() { return m_FileType; } 00115 00120 AP4_Result SetFileType(AP4_UI32 major_brand, 00121 AP4_UI32 minor_version, 00122 AP4_UI32* compatible_brands = NULL, 00123 AP4_Cardinal compatible_brand_count = 0); 00124 00128 bool IsMoovBeforeMdat() const { return m_MoovIsBeforeMdat; } 00129 00133 const AP4_MetaData* GetMetaData(); 00134 00138 virtual AP4_Result Inspect(AP4_AtomInspector& inspector); 00139 00140 private: 00141 // members 00142 AP4_Movie* m_Movie; 00143 AP4_FtypAtom* m_FileType; 00144 AP4_MetaData* m_MetaData; 00145 bool m_MoovIsBeforeMdat; 00146 }; 00147 00148 #endif // _AP4_FILE_H_
Generated on Thu May 13 16:36:32 2010 for Bento4 MP4 SDK by
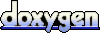