Ap4Array.h
Go to the documentation of this file.00001 /***************************************************************** 00002 | 00003 | AP4 - Arrays 00004 | 00005 | Copyright 2002-2008 Axiomatic Systems, LLC 00006 | 00007 | 00008 | This file is part of Bento4/AP4 (MP4 Atom Processing Library). 00009 | 00010 | Unless you have obtained Bento4 under a difference license, 00011 | this version of Bento4 is Bento4|GPL. 00012 | Bento4|GPL is free software; you can redistribute it and/or modify 00013 | it under the terms of the GNU General Public License as published by 00014 | the Free Software Foundation; either version 2, or (at your option) 00015 | any later version. 00016 | 00017 | Bento4|GPL is distributed in the hope that it will be useful, 00018 | but WITHOUT ANY WARRANTY; without even the implied warranty of 00019 | MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00020 | GNU General Public License for more details. 00021 | 00022 | You should have received a copy of the GNU General Public License 00023 | along with Bento4|GPL; see the file COPYING. If not, write to the 00024 | Free Software Foundation, 59 Temple Place - Suite 330, Boston, MA 00025 | 02111-1307, USA. 00026 | 00027 ****************************************************************/ 00033 #ifndef _AP4_ARRAY_H_ 00034 #define _AP4_ARRAY_H_ 00035 00036 /*---------------------------------------------------------------------- 00037 | includes 00038 +---------------------------------------------------------------------*/ 00039 #include "Ap4Config.h" 00040 #if defined(APT_CONFIG_HAVE_NEW_H) 00041 #include <new> 00042 #endif 00043 #include "Ap4Types.h" 00044 #include "Ap4Results.h" 00045 00046 /*---------------------------------------------------------------------- 00047 | constants 00048 +---------------------------------------------------------------------*/ 00049 const int AP4_ARRAY_INITIAL_COUNT = 64; 00050 00051 /*---------------------------------------------------------------------- 00052 | AP4_Array 00053 +---------------------------------------------------------------------*/ 00054 template <typename T> 00055 class AP4_Array 00056 { 00057 public: 00058 // methods 00059 AP4_Array(): m_AllocatedCount(0), m_ItemCount(0), m_Items(0) {} 00060 AP4_Array(const T* items, AP4_Size count); 00061 virtual ~AP4_Array(); 00062 AP4_Cardinal ItemCount() const { return m_ItemCount; } 00063 AP4_Result Append(const T& item); 00064 AP4_Result RemoveLast(); 00065 T& operator[](unsigned long idx) { return m_Items[idx]; } 00066 const T& operator[](unsigned long idx) const { return m_Items[idx]; } 00067 AP4_Result Clear(); 00068 AP4_Result EnsureCapacity(AP4_Cardinal count); 00069 AP4_Result SetItemCount(AP4_Cardinal item_count); 00070 00071 protected: 00072 // members 00073 AP4_Cardinal m_AllocatedCount; 00074 AP4_Cardinal m_ItemCount; 00075 T* m_Items; 00076 }; 00077 00078 /*---------------------------------------------------------------------- 00079 | AP4_Array<T>::AP4_Array<T> 00080 +---------------------------------------------------------------------*/ 00081 template <typename T> 00082 AP4_Array<T>::AP4_Array(const T* items, AP4_Size count) : 00083 m_AllocatedCount(count), 00084 m_ItemCount(count), 00085 m_Items((T*)::operator new(count*sizeof(T))) 00086 { 00087 for (unsigned int i=0; i<count; i++) { 00088 new ((void*)&m_Items[i]) T(items[i]); 00089 } 00090 } 00091 00092 /*---------------------------------------------------------------------- 00093 | AP4_Array<T>::~AP4_Array<T> 00094 +---------------------------------------------------------------------*/ 00095 template <typename T> 00096 AP4_Array<T>::~AP4_Array() 00097 { 00098 Clear(); 00099 ::operator delete((void*)m_Items); 00100 } 00101 00102 /*---------------------------------------------------------------------- 00103 | NPT_Array<T>::Clear 00104 +---------------------------------------------------------------------*/ 00105 template <typename T> 00106 AP4_Result 00107 AP4_Array<T>::Clear() 00108 { 00109 // destroy all items 00110 for (AP4_Ordinal i=0; i<m_ItemCount; i++) { 00111 m_Items[i].~T(); 00112 } 00113 00114 m_ItemCount = 0; 00115 00116 return AP4_SUCCESS; 00117 } 00118 00119 /*---------------------------------------------------------------------- 00120 | AP4_Array<T>::EnsureCapacity 00121 +---------------------------------------------------------------------*/ 00122 template <typename T> 00123 AP4_Result 00124 AP4_Array<T>::EnsureCapacity(AP4_Cardinal count) 00125 { 00126 // check if we already have enough 00127 if (count <= m_AllocatedCount) return AP4_SUCCESS; 00128 00129 // (re)allocate the items 00130 T* new_items = (T*) ::operator new (count*sizeof(T)); 00131 if (new_items == NULL) { 00132 return AP4_ERROR_OUT_OF_MEMORY; 00133 } 00134 if (m_ItemCount && m_Items) { 00135 for (unsigned int i=0; i<m_ItemCount; i++) { 00136 new ((void*)&new_items[i]) T(m_Items[i]); 00137 m_Items[i].~T(); 00138 } 00139 ::operator delete((void*)m_Items); 00140 } 00141 m_Items = new_items; 00142 m_AllocatedCount = count; 00143 00144 return AP4_SUCCESS; 00145 } 00146 00147 /*---------------------------------------------------------------------- 00148 | AP4_Array<T>::SetItemCount 00149 +---------------------------------------------------------------------*/ 00150 template <typename T> 00151 AP4_Result 00152 AP4_Array<T>::SetItemCount(AP4_Cardinal item_count) 00153 { 00154 // shortcut 00155 if (item_count == m_ItemCount) return AP4_SUCCESS; 00156 00157 // check for a reduction in the number of items 00158 if (item_count < m_ItemCount) { 00159 // destruct the items that are no longer needed 00160 for (unsigned int i=item_count; i<m_ItemCount; i++) { 00161 m_Items[i].~T(); 00162 } 00163 m_ItemCount = item_count; 00164 return AP4_SUCCESS; 00165 } 00166 00167 // grow the list 00168 AP4_Result result = EnsureCapacity(item_count); 00169 if (AP4_FAILED(result)) return result; 00170 00171 // construct the new items 00172 for (unsigned int i=m_ItemCount; i<item_count; i++) { 00173 new ((void*)&m_Items[i]) T(); 00174 } 00175 m_ItemCount = item_count; 00176 return AP4_SUCCESS; 00177 } 00178 00179 /*---------------------------------------------------------------------- 00180 | AP4_Array<T>::RemoveLast 00181 +---------------------------------------------------------------------*/ 00182 template <typename T> 00183 AP4_Result 00184 AP4_Array<T>::RemoveLast() 00185 { 00186 if (m_ItemCount) { 00187 m_Items[--m_ItemCount].~T(); 00188 return AP4_SUCCESS; 00189 } else { 00190 return AP4_ERROR_OUT_OF_RANGE; 00191 } 00192 } 00193 00194 /*---------------------------------------------------------------------- 00195 | AP4_Array<T>::Append 00196 +---------------------------------------------------------------------*/ 00197 template <typename T> 00198 AP4_Result 00199 AP4_Array<T>::Append(const T& item) 00200 { 00201 // ensure that we have enough space 00202 if (m_AllocatedCount < m_ItemCount+1) { 00203 // try double the size, with a minimum 00204 unsigned long new_count = m_AllocatedCount?2*m_AllocatedCount:AP4_ARRAY_INITIAL_COUNT; 00205 00206 // if that's still not enough, just ask for what we need 00207 if (new_count < m_ItemCount+1) new_count = m_ItemCount+1; 00208 00209 // reserve the space 00210 AP4_Result result = EnsureCapacity(new_count); 00211 if (result != AP4_SUCCESS) return result; 00212 } 00213 00214 // store the item 00215 new ((void*)&m_Items[m_ItemCount++]) T(item); 00216 00217 return AP4_SUCCESS; 00218 } 00219 00220 #endif // _AP4_ARRAY_H_ 00221 00222 00223 00224 00225 00226 00227 00228 00229 00230 00231 00232 00233
Generated on Thu May 13 16:36:31 2010 for Bento4 MP4 SDK by
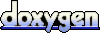