amplayerMy Project
|
player_type.h
00001 #ifndef _PLAYER_TYPE_H_ 00002 #define _PLAYER_TYPE_H_ 00003 00004 #include <libavformat/avformat.h> 00005 #include <stream_format.h> 00006 00007 #define MSG_SIZE 64 00008 #define MAX_VIDEO_STREAMS 10 00009 #define MAX_AUDIO_STREAMS 16 00010 #define MAX_SUB_INTERNAL 64 00011 #define MAX_SUB_EXTERNAL 24 00012 #define MAX_SUB_STREAMS (MAX_SUB_INTERNAL + MAX_SUB_EXTERNAL) 00013 #define MAX_PLAYER_THREADS 32 00014 00015 #define CALLBACK_INTERVAL (300) 00016 00017 //#define DEBUG_VARIABLE_DUR 00018 00019 typedef enum 00020 { 00021 /****************************** 00022 * 0x1000x: 00023 * player do parse file 00024 * decoder not running 00025 ******************************/ 00026 PLAYER_INITING = 0x10001, 00027 PLAYER_TYPE_REDY = 0x10002, 00028 PLAYER_INITOK = 0x10003, 00029 00030 /****************************** 00031 * 0x2000x: 00032 * playback status 00033 * decoder is running 00034 ******************************/ 00035 PLAYER_RUNNING = 0x20001, 00036 PLAYER_BUFFERING = 0x20002, 00037 PLAYER_PAUSE = 0x20003, 00038 PLAYER_SEARCHING = 0x20004, 00039 00040 PLAYER_SEARCHOK = 0x20005, 00041 PLAYER_START = 0x20006, 00042 PLAYER_FF_END = 0x20007, 00043 PLAYER_FB_END = 0x20008, 00044 00045 PLAYER_PLAY_NEXT = 0x20009, 00046 PLAYER_BUFFER_OK = 0x2000a, 00047 PLAYER_FOUND_SUB = 0x2000b, 00048 00049 /****************************** 00050 * 0x3000x: 00051 * player will exit 00052 ******************************/ 00053 PLAYER_ERROR = 0x30001, 00054 PLAYER_PLAYEND = 0x30002, 00055 PLAYER_STOPED = 0x30003, 00056 PLAYER_EXIT = 0x30004, 00057 00058 /****************************** 00059 * 0x4000x: 00060 * divx drm 00061 * decoder will exit or give 00062 * a message dialog 00063 * ****************************/ 00064 PLAYER_DIVX_AUTHORERR = 0x40001, 00065 PLAYER_DIVX_RENTAL_EXPIRED = 0x40002, 00066 PLAYER_DIVX_RENTAL_VIEW = 0x40003, 00067 }player_status; 00068 00069 00070 typedef enum { 00071 DRM_LEVEL1 = 1, 00072 DRM_LEVEL2 = 2, 00073 DRM_LEVEL3 = 3, 00074 DRM_NONE = 4, 00075 } drm_level_t; 00076 00077 typedef struct drm_info { 00078 drm_level_t drm_level; 00079 int drm_flag; 00080 int drm_hasesdata; 00081 int drm_priv; 00082 unsigned int drm_pktsize; 00083 unsigned int drm_pktpts; 00084 unsigned int drm_phy; 00085 unsigned int drm_vir; 00086 unsigned int drm_remap; 00087 int data_offset; 00088 int extpad[8]; 00089 } drminfo_t; 00090 00091 00092 00093 typedef struct 00094 { 00095 int index; 00096 int id; 00097 int width; 00098 int height; 00099 int aspect_ratio_num; 00100 int aspect_ratio_den; 00101 int frame_rate_num; 00102 int frame_rate_den; 00103 int bit_rate; 00104 vformat_t format; 00105 int duartion; 00106 unsigned int video_rotation_degree; 00107 }mvideo_info_t; 00108 00109 typedef enum 00110 { 00111 ACOVER_NONE = 0, 00112 ACOVER_JPG , 00113 ACOVER_PNG , 00114 }audio_cover_type; 00115 00116 typedef struct 00117 { 00118 char title[512]; 00119 char author[512]; 00120 char album[512]; 00121 char comment[512]; 00122 char year[4]; 00123 int track; 00124 char genre[32]; 00125 char copyright[512]; 00126 audio_cover_type pic; 00127 }audio_tag_info; 00128 00129 typedef struct 00130 { 00131 int index; 00132 int id; 00133 int channel; 00134 int sample_rate; 00135 int bit_rate; 00136 aformat_t aformat; 00137 int duration; 00138 audio_tag_info *audio_tag; 00139 }maudio_info_t; 00140 00141 typedef struct 00142 { 00143 int index; 00144 char id; 00145 char internal_external; //0:internal_sub 1:external_sub 00146 unsigned short width; 00147 unsigned short height; 00148 unsigned int sub_type; 00149 char resolution; 00150 long long subtitle_size; 00151 char *sub_language; 00152 }msub_info_t; 00153 00154 typedef struct 00155 { 00156 char *filename; 00157 int duration; 00158 long long file_size; 00159 pfile_type type; 00160 int bitrate; 00161 int has_video; 00162 int has_audio; 00163 int has_sub; 00164 int nb_streams; 00165 int total_video_num; 00166 int cur_video_index; 00167 int total_audio_num; 00168 int cur_audio_index; 00169 int total_sub_num; 00170 int cur_sub_index; 00171 int seekable; 00172 int drm_check; 00173 int adif_file_flag; 00174 }mstream_info_t; 00175 00176 typedef struct 00177 { 00178 mstream_info_t stream_info; 00179 mvideo_info_t *video_info[MAX_VIDEO_STREAMS]; 00180 maudio_info_t *audio_info[MAX_AUDIO_STREAMS]; 00181 msub_info_t *sub_info[MAX_SUB_STREAMS]; 00182 }media_info_t; 00183 00184 typedef struct player_info 00185 { 00186 char *name; 00187 player_status last_sta; 00188 player_status status; /*stop,pause */ 00189 int full_time; /*Seconds */ 00190 int full_time_ms; /* mSeconds */ 00191 int current_time; /*Seconds */ 00192 int current_ms; /*ms*/ 00193 int last_time; 00194 int error_no; 00195 int64_t start_time; 00196 int64_t first_time; 00197 int pts_video; 00198 //int pts_pcrscr; 00199 unsigned int current_pts; 00200 long curtime_old_time; 00201 unsigned int video_error_cnt; 00202 unsigned int audio_error_cnt; 00203 float audio_bufferlevel; // relative value 00204 float video_bufferlevel; // relative value 00205 int64_t bufed_pos; 00206 int bufed_time;/* Second*/ 00207 unsigned int drm_rental; 00208 int64_t download_speed; //download speed 00209 unsigned int last_pts; 00210 int seek_point; 00211 int seek_delay; 00212 }player_info_t; 00213 00214 typedef struct pid_info 00215 { 00216 int num; 00217 int pid[MAX_PLAYER_THREADS]; 00218 }pid_info_t; 00219 00220 typedef struct player_file_type 00221 { 00222 const char *fmt_string; 00223 int video_tracks; 00224 int audio_tracks; 00225 int subtitle_tracks; 00226 00227 }player_file_type_t; 00228 00229 00230 #define STATE_PRE(sta) (sta>>16) 00231 #define PLAYER_THREAD_IS_INITING(sta) (STATE_PRE(sta)==0x1) 00232 #define PLAYER_THREAD_IS_RUNNING(sta) (STATE_PRE(sta)==0x2) 00233 #define PLAYER_THREAD_IS_STOPPED(sta) (sta==PLAYER_EXIT) 00234 00235 typedef int (*update_state_fun_t)(int pid,player_info_t *) ; 00236 typedef int (*notify_callback)(int pid,int msg,unsigned long ext1,unsigned long ext2); 00237 typedef enum 00238 { 00239 PLAYER_EVENTS_PLAYER_INFO=1, 00240 PLAYER_EVENTS_STATE_CHANGED, 00241 PLAYER_EVENTS_ERROR, 00242 PLAYER_EVENTS_BUFFERING, 00243 PLAYER_EVENTS_FILE_TYPE, 00244 PLAYER_EVENTS_HTTP_WV, 00245 PLAYER_EVENTS_HWBUF_DATA_SIZE_CHANGED, 00246 PLAYER_EVENTS_NOT_SUPPORT_SEEKABLE, //not support seek; 00247 PLAYER_EVENTS_VIDEO_SIZE_CHANGED, 00248 PLAYER_EVENTS_SUBTITLE_DATA, // sub data ext1 refers to subtitledata struct 00249 }player_events; 00250 00251 typedef struct 00252 { 00253 int vbufused; 00254 int vbufsize; 00255 int vdatasize; 00256 int abufused; 00257 int abufsize; 00258 int adatasize; 00259 int sbufused; 00260 int sbufsize; 00261 int sdatasize; 00262 }hwbufstats_t; 00263 00264 00265 typedef struct 00266 { 00267 update_state_fun_t update_statue_callback; 00268 int update_interval; 00269 long callback_old_time; 00270 notify_callback notify_fn; 00271 }callback_t; 00272 00273 typedef struct 00274 { 00275 char *file_name; //file url 00276 char *headers; //file name's authentication information,maybe used in network streaming 00277 //List *play_list; 00278 int video_index; //video track, no assigned, please set to -1 00279 int audio_index; //audio track, no assigned, please set to -1 00280 int sub_index; //subtitle track, no assigned, please set to -1 00281 float t_pos; //start postion, use second as unit 00282 int read_max_cnt; //read retry maxium counts, if exceed it, return error 00283 int avsync_threshold; //for adec av sync threshold in ms 00284 union 00285 { 00286 struct{ 00287 unsigned int loop_mode:1; //file loop mode 0:loop 1:not loop 00288 unsigned int nosound:1; //0:play with audio 1:play without audio 00289 unsigned int novideo:1; //0:play with video 1:play without video 00290 unsigned int hassub:1; //0:ignore subtitle 1:extract subtitle if have 00291 unsigned int need_start:1;/*If set need_start, we need call player_start_play to playback*/ 00292 #ifdef DEBUG_VARIABLE_DUR 00293 unsigned int is_variable:1; //0:extrack duration from header 1:update duration during playback 00294 #endif 00295 unsigned int displast_frame : 1;//0:black out when player exit 1:keep last frame when player exit 00296 }; 00297 int mode; //no use 00298 }; 00299 callback_t callback_fn; //callback function 00300 callback_t subdata_fn; // subtitle data notify function 00301 void *subhd; // sub handle 00302 int subdatasource; // sub data source 00303 int byteiobufsize; //byteio buffer size used in ffmpeg 00304 int loopbufsize; //loop buffer size used in ffmpeg 00305 int enable_rw_on_pause; //no use 00306 /* 00307 data%<min && data% <max enter buffering; 00308 data% >middle exit buffering; 00309 */ 00310 int auto_buffing_enable; //auto buffering switch 00311 float buffing_min; //auto buffering low limit 00312 float buffing_middle; //auto buffering middle limit 00313 float buffing_max; //auto buffering high limit 00314 int is_playlist; //no use 00315 int is_type_parser; //is try to get file type 00316 int is_livemode; // support timeshift for chinamobile 00317 int buffing_starttime_s; //for rest buffing_middle,buffering seconds data to start. 00318 int buffing_force_delay_s; 00319 int lowbuffermode_flag; 00320 int lowbuffermode_limited_ms; 00321 int is_ts_soft_demux; 00322 int reserved [56]; //reserved for furthur used,some one add more ,can del reserved num 00323 int SessionID; 00324 int t_duration_ms; //duration parsed from url 00325 }play_control_t; 00326 00327 #endif
Generated on Tue Dec 2 2014 21:55:13 for amplayerMy Project by
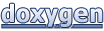