include/codec.h File Reference
Function prototypes of codec lib. More...
#include <codec_type.h>
#include <codec_error.h>
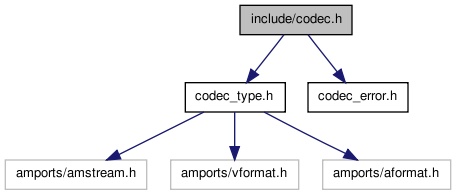

Go to the source code of this file.
Functions | |
int | codec_init (codec_para_t *) |
codec_init Initialize the codec device based on stream type | |
int | codec_close (codec_para_t *) |
codec_close Close codec device | |
void | codec_close_audio (codec_para_t *) |
codec_close_audio Close audio decoder | |
void | codec_resume_audio (codec_para_t *, unsigned int) |
codec_resume_audio Resume audio decoder to work (etc, after pause) | |
int | codec_reset (codec_para_t *) |
codec_reset Reset codec device | |
int | codec_init_sub (codec_para_t *) |
codec_init_sub Initialize subtile codec device | |
int | codec_open_sub_read (void) |
codec_open_sub_read Open read_subtitle device which is special for read subtile data | |
int | codec_close_sub (codec_para_t *) |
codec_close_sub Close subtile device | |
int | codec_close_sub_fd (CODEC_HANDLE) |
codec_close_sub_fd Close subtile device by fd | |
int | codec_reset_subtile (codec_para_t *pcodec) |
codec_reset_subtile Reset subtitle device, especially for subtitle swith | |
int | codec_poll_sub (codec_para_t *) |
codec_poll_sub Polling subtile device if subtitle data is ready | |
int | codec_poll_sub_fd (CODEC_HANDLE, int) |
codec_poll_sub_fd Polling subtile device if subtitle data is ready by fd | |
int | codec_get_sub_size (codec_para_t *) |
codec_get_sub_size Get the size of subtitle data which is ready | |
int | codec_get_sub_size_fd (CODEC_HANDLE) |
codec_get_sub_size_fd Get the size of subtitle data which is ready by fd | |
int | codec_read_sub_data (codec_para_t *, char *, unsigned int) |
codec_read_sub_data Read subtitle data from codec device | |
int | codec_read_sub_data_fd (CODEC_HANDLE, char *, unsigned int) |
codec_read_sub_data_fd Read subtitle data from codec device by fd | |
int | codec_write_sub_data (codec_para_t *, char *, unsigned int) |
codec_write_sub_data Write subtile data to subtitle device | |
int | codec_init_cntl (codec_para_t *) |
codec_init_cntl Initialize the video control device | |
int | codec_close_cntl (codec_para_t *) |
codec_close_cntl Close video control device | |
int | codec_poll_cntl (codec_para_t *) |
codec_poll_cntl Polling video control device | |
int | codec_get_cntl_state (codec_para_t *) |
codec_get_cntl_state Get the status of video control device, especially for trickmode | |
int | codec_set_cntl_mode (codec_para_t *, unsigned int) |
codec_set_cntl_mode Set the mode to video control device, especially for trickmode | |
int | codec_set_cntl_avthresh (codec_para_t *, unsigned int) |
codec_set_cntl_avthresh Set the AV sync threshold which defines the max time difference between A/V | |
int | codec_set_cntl_syncthresh (codec_para_t *pcodec, unsigned int syncthresh) |
codec_set_cntl_syncthresh Set sync threshold control which defines the starting system time (hold video or not) when playing | |
int | codec_reset_audio (codec_para_t *pcodec) |
codec_reset_audio Reset audio decoder, especially for audio switch | |
int | codec_set_audio_pid (codec_para_t *pcodec) |
codec_set_audio_id Set audio pid by codec device | |
int | codec_set_sub_id (codec_para_t *pcodec) |
codec_set_sub_id Set subtitle pid by codec device | |
int | codec_audio_reinit (codec_para_t *pcodec) |
codec_audio_reinit Re-initialize audio codec | |
int | codec_set_dec_reset (codec_para_t *pcodec) |
codec_set_dec_reset Set decoder reset flag when reset | |
int | codec_write (codec_para_t *pcodec, void *buffer, int len) |
codec_write Write data to codec device | |
int | codec_checkin_pts (codec_para_t *pcodec, unsigned long pts) |
codec_checkin_pts Checkin pts to codec device | |
int | codec_get_vbuf_state (codec_para_t *, struct buf_status *) |
codec_get_vbuf_state Get the state of video buffer by codec device | |
int | codec_get_abuf_state (codec_para_t *, struct buf_status *) |
codec_get_abuf_state Get the state of audio buffer by codec device | |
int | codec_get_vdec_state (codec_para_t *, struct vdec_status *) |
codec_get_vdec_state Get the state of video decoder by codec device | |
int | codec_get_adec_state (codec_para_t *, struct adec_status *) |
codec_get_adec_state Get the state of audio decoder by codec device | |
int | codec_pause (codec_para_t *) |
codec_pause Pause all playing(A/V) by codec device | |
int | codec_resume (codec_para_t *) |
codec_resume Resume playing(A/V) by codec device | |
int | codec_audio_search (codec_para_t *p) |
int | codec_set_mute (codec_para_t *p, int mute) |
codec_set_mute Set audio mute | |
int | codec_get_volume_range (codec_para_t *, int *min, int *max) |
codec_get_volume_range Get audio volume range | |
int | codec_set_volume (codec_para_t *, int val) |
codec_set_volume Set audio volume | |
int | codec_get_volume (codec_para_t *) |
codec_get_volume Get audio volume | |
int | codec_get_mutesta (codec_para_t *) |
codec_get_mutesta Get codec mute status | |
int | codec_set_volume_balance (codec_para_t *, int) |
codec_set_volume_balance Set volume balance | |
int | codec_swap_left_right (codec_para_t *) |
codec_swap_left_right Swap audio left and right channel | |
int | codec_left_mono (codec_para_t *p) |
codec_left_mono Set mono with left channel | |
int | codec_right_mono (codec_para_t *p) |
codec_right_mono Set mono with right channel | |
int | codec_stereo (codec_para_t *p) |
codec_stereo Set stereo | |
int | codec_audio_automute (int auto_mute) |
codec_audio_automute Set decoder to automute mode | |
int | codec_audio_spectrum_switch (codec_para_t *p, int isStart, int interval) |
codec_audio_spectrum_switch Switch audio spectrum |
Detailed Description
Function prototypes of codec lib.
- Author:
- Zhang Chen <[email protected]>
- Version:
- 1.0.0
- Date:
- 2011-02-24
Definition in file codec.h.
Function Documentation
int codec_audio_automute | ( | int | auto_mute | ) |
codec_audio_automute Set decoder to automute mode
- Parameters:
-
[in] auto_mute automute mode
- Returns:
- Command result
Definition at line 238 of file audio_ctrl.c.
int codec_audio_reinit | ( | codec_para_t * | pcodec | ) |
codec_audio_reinit Re-initialize audio codec
- Parameters:
-
[in] pcodec Pointer of codec parameter structure
- Returns:
- 0 for success, or fail type if < 0
Definition at line 1395 of file codec_ctrl.c.
int codec_audio_spectrum_switch | ( | codec_para_t * | p, | |
int | isStart, | |||
int | interval | |||
) |
codec_audio_spectrum_switch Switch audio spectrum
- Parameters:
-
[in] p Pointer of codec parameter structure [in] isStart Start(1) or stop(0) spectrum [in] interval Spectrum interval
- Returns:
- Command result
Definition at line 259 of file audio_ctrl.c.
int codec_checkin_pts | ( | codec_para_t * | pcodec, | |
unsigned long | pts | |||
) |
codec_checkin_pts Checkin pts to codec device
- Parameters:
-
[in] pcodec Pointer of codec parameter structure [in] pts Pts to be checked in
- Returns:
- 0 for success, or fail type
Definition at line 750 of file codec_ctrl.c.

int codec_close | ( | codec_para_t * | pcodec | ) |
codec_close Close codec device
- Parameters:
-
[in] pcodec Pointer of codec parameter structure
- Returns:
- 0 for success, or fail type if < 0
Definition at line 694 of file codec_ctrl.c.
Referenced by codec_reset().
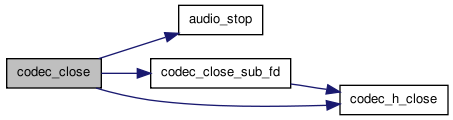
void codec_close_audio | ( | codec_para_t * | pcodec | ) |
codec_close_audio Close audio decoder
- Parameters:
-
[in] pcodec Pointer of codec parameter structure
Definition at line 714 of file codec_ctrl.c.

int codec_close_cntl | ( | codec_para_t * | pcodec | ) |
codec_close_cntl Close video control device
- Parameters:
-
[in] pcodec Pointer of codec parameter structure
- Returns:
- Success or fail error type
Definition at line 1227 of file codec_ctrl.c.

int codec_close_sub | ( | codec_para_t * | pcodec | ) |
codec_close_sub Close subtile device
- Parameters:
-
[in] pcodec Pointer of codec parameter structure
- Returns:
- Success or fail error type
Definition at line 987 of file codec_ctrl.c.

int codec_close_sub_fd | ( | CODEC_HANDLE | sub_fd | ) |
codec_close_sub_fd Close subtile device by fd
- Parameters:
-
[in] sub_fd subtile device fd
- Returns:
- 0 for success, or fail type if < 0
Definition at line 1004 of file codec_ctrl.c.
Referenced by codec_close().

int codec_get_abuf_state | ( | codec_para_t * | p, | |
struct buf_status * | buf | |||
) |
codec_get_abuf_state Get the state of audio buffer by codec device
- Parameters:
-
[in] p Pointer of codec parameter structure [out] buf Pointer of buffer status structure to get audio buffer state
- Returns:
- Success or fail type
Definition at line 784 of file codec_ctrl.c.
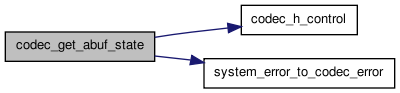
int codec_get_adec_state | ( | codec_para_t * | p, | |
struct adec_status * | adec | |||
) |
codec_get_adec_state Get the state of audio decoder by codec device
- Parameters:
-
[in] p Pointer of codec parameter structure [out] adec Pointer of audio decoder status structure
- Returns:
- Success or fail type
Definition at line 825 of file codec_ctrl.c.
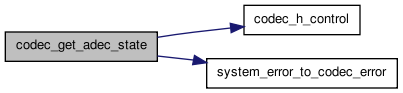
int codec_get_cntl_state | ( | codec_para_t * | pcodec | ) |
codec_get_cntl_state Get the status of video control device, especially for trickmode
- Parameters:
-
[in] pcodec Pointer of codec parameter structure
- Returns:
- Video control device status or fail error type
Definition at line 1267 of file codec_ctrl.c.
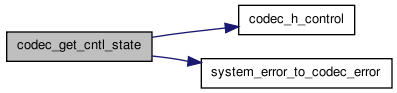
int codec_get_mutesta | ( | codec_para_t * | p | ) |
codec_get_mutesta Get codec mute status
- Parameters:
-
[in] p Pointer of codec parameter structure
- Returns:
- audio command result
Definition at line 72 of file audio_ctrl.c.
int codec_get_sub_size | ( | codec_para_t * | pcodec | ) |
codec_get_sub_size Get the size of subtitle data which is ready
- Parameters:
-
[in] pcodec Pointer of codec parameter structure
- Returns:
- Subtile ready data size, or fail error type if < 0
Definition at line 1065 of file codec_ctrl.c.
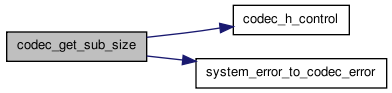
int codec_get_sub_size_fd | ( | CODEC_HANDLE | sub_fd | ) |
codec_get_sub_size_fd Get the size of subtitle data which is ready by fd
- Parameters:
-
[in] sub_fd Subtitle device fd
- Returns:
- Subtile ready data size, or fail error type if < 0
Definition at line 1091 of file codec_ctrl.c.
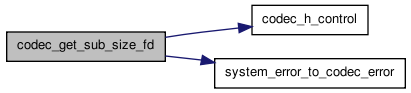
int codec_get_vbuf_state | ( | codec_para_t * | p, | |
struct buf_status * | buf | |||
) |
codec_get_vbuf_state Get the state of video buffer by codec device
- Parameters:
-
[in] p Pointer of codec parameter structure [out] buf Pointer of buffer status structure to get video buffer state
- Returns:
- Success or fail type
Definition at line 766 of file codec_ctrl.c.
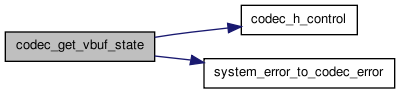
int codec_get_vdec_state | ( | codec_para_t * | p, | |
struct vdec_status * | vdec | |||
) |
codec_get_vdec_state Get the state of video decoder by codec device
- Parameters:
-
[in] p Pointer of codec parameter structure [out] vdec Pointer of video decoder status structure
- Returns:
- Success or fail type
Definition at line 803 of file codec_ctrl.c.
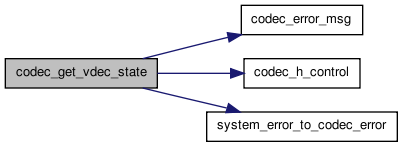
int codec_get_volume | ( | codec_para_t * | p | ) |
codec_get_volume Get audio volume
- Parameters:
-
[in] p Pointer of codec parameter structure
- Returns:
- command result
Definition at line 143 of file audio_ctrl.c.
int codec_get_volume_range | ( | codec_para_t * | p, | |
int * | min, | |||
int * | max | |||
) |
codec_get_volume_range Get audio volume range
- Parameters:
-
[in] p Pointer of codec parameter structure [out] min Data to save the min volume [out] max Data to save the max volume
- Returns:
- not used, read failed
Definition at line 110 of file audio_ctrl.c.
int codec_init | ( | codec_para_t * | pcodec | ) |
codec_init Initialize the codec device based on stream type
- Parameters:
-
[in] pcodec Pointer of codec parameter structure
- Returns:
- Success or fail error type
Definition at line 607 of file codec_ctrl.c.
Referenced by codec_reset().
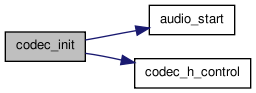
int codec_init_cntl | ( | codec_para_t * | pcodec | ) |
codec_init_cntl Initialize the video control device
- Parameters:
-
[in] pcodec Pointer of codec parameter structure
- Returns:
- Success or fail error type
Definition at line 1204 of file codec_ctrl.c.
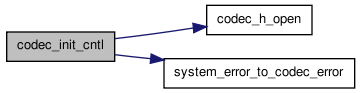
int codec_init_sub | ( | codec_para_t * | pcodec | ) |
codec_init_sub Initialize subtile codec device
- Parameters:
-
[in] pcodec Pointer of codec parameter structure
- Returns:
- Success or fail error type
Definition at line 943 of file codec_ctrl.c.
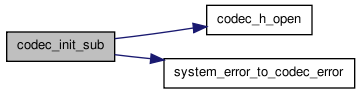
int codec_left_mono | ( | codec_para_t * | p | ) |
codec_left_mono Set mono with left channel
- Parameters:
-
[in] p Pointer of codec parameter structure
- Returns:
- Command result
Definition at line 190 of file audio_ctrl.c.
int codec_open_sub_read | ( | void | ) |
codec_open_sub_read Open read_subtitle device which is special for read subtile data
- Returns:
- Device handler, or error type if < 0
Definition at line 965 of file codec_ctrl.c.
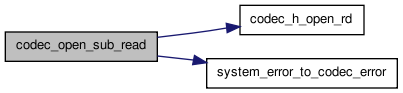
int codec_pause | ( | codec_para_t * | p | ) |
codec_pause Pause all playing(A/V) by codec device
- Parameters:
-
[in] p Pointer of codec parameter structure
- Returns:
- Success or fail error type
Definition at line 873 of file codec_ctrl.c.

int codec_poll_cntl | ( | codec_para_t * | pcodec | ) |
codec_poll_cntl Polling video control device
- Parameters:
-
[in] pcodec Pointer of codec parameter structure
- Returns:
- Polling results
Definition at line 1244 of file codec_ctrl.c.
int codec_poll_sub | ( | codec_para_t * | pcodec | ) |
codec_poll_sub Polling subtile device if subtitle data is ready
- Parameters:
-
[in] pcodec Pointer of codec parameter structure
- Returns:
- Polling result
Definition at line 1018 of file codec_ctrl.c.
int codec_poll_sub_fd | ( | CODEC_HANDLE | sub_fd, | |
int | timeout | |||
) |
codec_poll_sub_fd Polling subtile device if subtitle data is ready by fd
- Parameters:
-
[in] sub_fd Subtitle device fd [in] timeout Timeout for polling
- Returns:
- Polling result
Definition at line 1042 of file codec_ctrl.c.
int codec_read_sub_data | ( | codec_para_t * | pcodec, | |
char * | buf, | |||
unsigned int | length | |||
) |
codec_read_sub_data Read subtitle data from codec device
- Parameters:
-
[in] pcodec Pointer of codec parameter structure [out] buf Buffer for data read from subtitle codec device [in] length Data length to be read from subtitle codec device
- Returns:
- 0 for success, or fail type if < 0
Definition at line 1119 of file codec_ctrl.c.
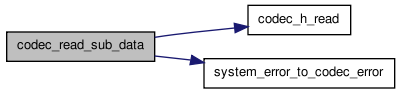
int codec_read_sub_data_fd | ( | CODEC_HANDLE | sub_fd, | |
char * | buf, | |||
unsigned int | length | |||
) |
codec_read_sub_data_fd Read subtitle data from codec device by fd
- Parameters:
-
[in] sub_fd Subtitle device fd [out] buf Buffer for data read from subtitle codec device [in] length Data length to be read from subtile codec device
- Returns:
- 0 for success, or fail type if < 0
Definition at line 1152 of file codec_ctrl.c.
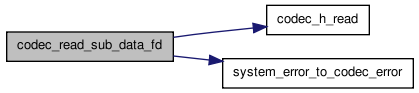
int codec_reset | ( | codec_para_t * | p | ) |
codec_reset Reset codec device
- Parameters:
-
[in] p Pointer of codec parameter structure
- Returns:
- Success or fail error type
Definition at line 922 of file codec_ctrl.c.
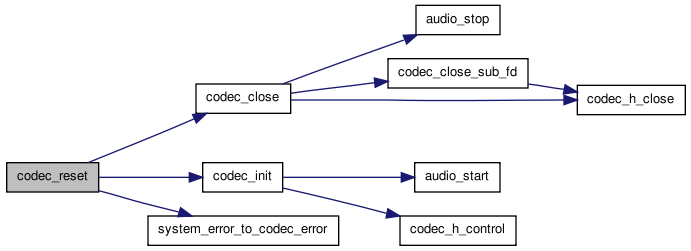
int codec_reset_audio | ( | codec_para_t * | pcodec | ) |
codec_reset_audio Reset audio decoder, especially for audio switch
- Parameters:
-
[in] pcodec Pointer of codec parameter structure
- Returns:
- 0 for success, or fail type if < 0
Definition at line 1339 of file codec_ctrl.c.

int codec_reset_subtile | ( | codec_para_t * | pcodec | ) |
codec_reset_subtile Reset subtitle device, especially for subtitle swith
- Parameters:
-
[in] pcodec Pointer of codec parameter structure
- Returns:
- 0 for success, or fail type if < 0
Definition at line 1353 of file codec_ctrl.c.

int codec_resume | ( | codec_para_t * | p | ) |
codec_resume Resume playing(A/V) by codec device
- Parameters:
-
[in] p Pointer of codec parameter structure
- Returns:
- Success or fail error type
Definition at line 897 of file codec_ctrl.c.

void codec_resume_audio | ( | codec_para_t * | pcodec, | |
unsigned int | orig | |||
) |
codec_resume_audio Resume audio decoder to work (etc, after pause)
- Parameters:
-
[in] pcodec Pointer of codec parameter structure [in] orig Original audio status (has audio or not)
Definition at line 731 of file codec_ctrl.c.

int codec_right_mono | ( | codec_para_t * | p | ) |
codec_right_mono Set mono with right channel
- Parameters:
-
[in] p Pointer of codec parameter structure
- Returns:
- Command result
Definition at line 206 of file audio_ctrl.c.
int codec_set_audio_pid | ( | codec_para_t * | pcodec | ) |
codec_set_audio_id Set audio pid by codec device
- Parameters:
-
[in] pcodec Pointer of codec parameter structure
- Returns:
- 0 for success, or fail type if < 0
Definition at line 1367 of file codec_ctrl.c.

int codec_set_cntl_avthresh | ( | codec_para_t * | pcodec, | |
unsigned int | avthresh | |||
) |
codec_set_cntl_avthresh Set the AV sync threshold which defines the max time difference between A/V
- Parameters:
-
[in] pcodec Pointer of codec parameter structure [in] avthresh Threshold to be set
- Returns:
- 0 for success, or fail type if < 0
Definition at line 1309 of file codec_ctrl.c.

int codec_set_cntl_mode | ( | codec_para_t * | pcodec, | |
unsigned int | mode | |||
) |
codec_set_cntl_mode Set the mode to video control device, especially for trickmode
- Parameters:
-
[in] pcodec Pointer of codec parameter structure [in] mode Trick mode to be set
- Returns:
- 0 for success, or fail type if < 0
Definition at line 1294 of file codec_ctrl.c.

int codec_set_cntl_syncthresh | ( | codec_para_t * | pcodec, | |
unsigned int | syncthresh | |||
) |
codec_set_cntl_syncthresh Set sync threshold control which defines the starting system time (hold video or not) when playing
- Parameters:
-
[in] pcodec Pointer of codec parameter structure [in] syncthresh Sync threshold control
- Returns:
- 0 for success, or fail type if < 0
Definition at line 1325 of file codec_ctrl.c.

int codec_set_dec_reset | ( | codec_para_t * | pcodec | ) |
codec_set_dec_reset Set decoder reset flag when reset
- Parameters:
-
[in] pcodec Pointer of codec parameter structure
- Returns:
- 0 for success, or fail type if < 0
Definition at line 1409 of file codec_ctrl.c.

int codec_set_mute | ( | codec_para_t * | p, | |
int | mute | |||
) |
codec_set_mute Set audio mute
- Parameters:
-
[in] p Pointer of codec parameter structure [in] mute mute command, 1 for mute, 0 for unmute
- Returns:
- audio command result
Definition at line 89 of file audio_ctrl.c.
int codec_set_sub_id | ( | codec_para_t * | pcodec | ) |
codec_set_sub_id Set subtitle pid by codec device
- Parameters:
-
[in] pcodec Pointer of codec parameter structure
- Returns:
- 0 for success, or fail type if < 0
Definition at line 1381 of file codec_ctrl.c.

int codec_set_volume | ( | codec_para_t * | p, | |
int | val | |||
) |
codec_set_volume Set audio volume
- Parameters:
-
[in] p Pointer of codec parameter structure [in] val Volume to be set
- Returns:
- command result
Definition at line 125 of file audio_ctrl.c.
int codec_set_volume_balance | ( | codec_para_t * | p, | |
int | balance | |||
) |
codec_set_volume_balance Set volume balance
- Parameters:
-
[in] p Pointer of codec parameter structure [in] balance Balance to be set
- Returns:
- not used, return failed
Definition at line 160 of file audio_ctrl.c.
int codec_stereo | ( | codec_para_t * | p | ) |
codec_stereo Set stereo
- Parameters:
-
[in] p Pointer of codec parameter structure
- Returns:
- Command result
Definition at line 222 of file audio_ctrl.c.
int codec_swap_left_right | ( | codec_para_t * | p | ) |
codec_swap_left_right Swap audio left and right channel
- Parameters:
-
[in] p Pointer of codec parameter structure
- Returns:
- Command result
Definition at line 174 of file audio_ctrl.c.
int codec_write | ( | codec_para_t * | pcodec, | |
void * | buffer, | |||
int | len | |||
) |
codec_write Write data to codec device
- Parameters:
-
[in] pcodec Pointer of codec parameter structure [in] buffer Buffer for data to be written [in] len Length of the data to be written
- Returns:
- Length of the written data, or fail if < 0
Definition at line 664 of file codec_ctrl.c.

int codec_write_sub_data | ( | codec_para_t * | pcodec, | |
char * | buf, | |||
unsigned int | length | |||
) |
codec_write_sub_data Write subtile data to subtitle device
- Parameters:
-
[in] pcodec Pointer of codec parameter structure [in] buf Buffer for data to be written [in] length Length of the dat to be written
- Returns:
- Write length, or fail type if < 0
Definition at line 1185 of file codec_ctrl.c.

Generated on Fri Feb 25 15:30:32 2011 for Amcodec by
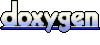