STM32L4xx_Nucleo_32 BSP User Manual
|
stm32l4xx_nucleo_32.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l4xx_nucleo_32.h 00004 * @author MCD Application Team 00005 * @version V1.0.0 00006 * @date 26-February-2016 00007 * @brief This file contains definitions for: 00008 * - LED available on STM32L4xx-Nucleo_32 Kit from STMicroelectronics 00009 * - 7 segment display from Gravitech 00010 ****************************************************************************** 00011 * @attention 00012 * 00013 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00014 * 00015 * Redistribution and use in source and binary forms, with or without modification, 00016 * are permitted provided that the following conditions are met: 00017 * 1. Redistributions of source code must retain the above copyright notice, 00018 * this list of conditions and the following disclaimer. 00019 * 2. Redistributions in binary form must reproduce the above copyright notice, 00020 * this list of conditions and the following disclaimer in the documentation 00021 * and/or other materials provided with the distribution. 00022 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00023 * may be used to endorse or promote products derived from this software 00024 * without specific prior written permission. 00025 * 00026 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00027 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00028 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00029 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00030 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00031 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00032 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00033 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00034 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00035 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00036 * 00037 ****************************************************************************** 00038 */ 00039 00040 /* Define to prevent recursive inclusion -------------------------------------*/ 00041 #ifndef __STM32L4XX_NUCLEO_32_H 00042 #define __STM32L4XX_NUCLEO_32_H 00043 00044 #ifdef __cplusplus 00045 extern "C" { 00046 #endif 00047 00048 /* Includes ------------------------------------------------------------------*/ 00049 #include "stm32l4xx_hal.h" 00050 00051 00052 /** @addtogroup BSP 00053 * @{ 00054 */ 00055 00056 /** @defgroup STM32L4XX_NUCLEO_32 NUCLEO 32 00057 * @brief This section contains the exported types, contants and functions 00058 * required to use the Nucleo 32 board. 00059 * @{ 00060 */ 00061 00062 /** @defgroup STM32L4XX_NUCLEO_32_Exported_Types Exported Types 00063 * @{ 00064 */ 00065 00066 typedef enum 00067 { 00068 LED3 = 0, 00069 LED_GREEN = LED3 00070 } Led_TypeDef; 00071 00072 /** 00073 * @} 00074 */ 00075 00076 /** @defgroup STM32L4XX_NUCLEO_32_Exported_Constants Exported Constants 00077 * @brief Define for STM32L4XX_NUCLEO_32 board 00078 * @{ 00079 */ 00080 00081 #if !defined (USE_STM32L4XX_NUCLEO_32) 00082 #define USE_STM32L4XX_NUCLEO_32 00083 #endif 00084 00085 /** @defgroup STM32L4XX_NUCLEO_LED LED Constants 00086 * @{ 00087 */ 00088 00089 #define LEDn 1 00090 00091 #define LED3_PIN GPIO_PIN_3 00092 #define LED3_GPIO_PORT GPIOB 00093 #define LED3_GPIO_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00094 #define LED3_GPIO_CLK_DISABLE() __HAL_RCC_GPIOB_CLK_DISABLE() 00095 00096 #define LEDx_GPIO_CLK_ENABLE(__INDEX__) do {LED3_GPIO_CLK_ENABLE(); } while(0) 00097 #define LEDx_GPIO_CLK_DISABLE(__INDEX__) LED3_GPIO_CLK_DISABLE()) 00098 00099 /** 00100 * @} 00101 */ 00102 00103 /** @defgroup STM32L4XX_NUCLEO_32_BUS BUS Constants 00104 * @{ 00105 */ 00106 00107 #if defined(HAL_I2C_MODULE_ENABLED) 00108 /*##################### I2C1 ###################################*/ 00109 /* User can use this section to tailor I2Cx instance used and associated resources */ 00110 /* Definition for I2C1 Pins */ 00111 #define BSP_I2C1 I2C1 00112 #define BSP_I2C1_CLK_ENABLE() __HAL_RCC_I2C1_CLK_ENABLE() 00113 #define BSP_I2C1_CLK_DISABLE() __HAL_RCC_I2C1_CLK_DISABLE() 00114 #define BSP_I2C1_FORCE_RESET() __HAL_RCC_I2C1_FORCE_RESET() 00115 #define BSP_I2C1_RELEASE_RESET() __HAL_RCC_I2C1_RELEASE_RESET() 00116 00117 #define BSP_I2C1_SCL_PIN GPIO_PIN_6 /* PB.6 add wire between D5 and A5 */ 00118 #define BSP_I2C1_SDA_PIN GPIO_PIN_7 /* PB.7 add wire between D4 and A4 */ 00119 00120 #define BSP_I2C1_GPIO_PORT GPIOB /* GPIOB */ 00121 #define BSP_I2C1_GPIO_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00122 #define BSP_I2C1_GPIO_CLK_DISABLE() __HAL_RCC_GPIOB_CLK_DISABLE() 00123 #define BSP_I2C1_SCL_SDA_AF GPIO_AF4_I2C1 00124 00125 /* Maximum Timeout values for flags waiting loops. These timeouts are not based 00126 on accurate values, they just guarantee that the application will not remain 00127 stuck if the I2C communication is corrupted. 00128 You may modify these timeout values depending on CPU frequency and application 00129 conditions (interrupts routines ...). */ 00130 #define BSP_I2C1_TIMEOUT_MAX 1000 00131 00132 /* I2C TIMING is calculated in case of the I2C Clock source is the SYSCLK = 80 MHz */ 00133 /* Set 0x40E03E53 value to reach 100 KHz speed (Rise time = 640ns, Fall time = 20ns) */ 00134 #define I2C1_TIMING 0x40E03E53 00135 00136 #endif /* HAL_I2C_MODULE_ENABLED */ 00137 00138 /** 00139 * @} 00140 */ 00141 00142 /** 00143 * @} 00144 */ 00145 00146 /** @defgroup STM32L4XX_NUCLEO_32_Exported_Functions Exported Functions 00147 * @{ 00148 */ 00149 00150 uint32_t BSP_GetVersion(void); 00151 void BSP_LED_Init(Led_TypeDef Led); 00152 void BSP_LED_On(Led_TypeDef Led); 00153 void BSP_LED_Off(Led_TypeDef Led); 00154 void BSP_LED_Toggle(Led_TypeDef Led); 00155 00156 /** 00157 * @} 00158 */ 00159 00160 /** 00161 * @} 00162 */ 00163 00164 /** @defgroup STM32L4XX_NUCLEO_32_GRAVITECH_4DIGITS GRAVITECH 4 DIGITS 00165 * @brief This section contains the exported functions 00166 * required to use Gravitech shield 7 Segment Display 00167 * @{ 00168 */ 00169 00170 /** @defgroup STM32_GRAVITECH_4DIGITS_Exported_Constants Exported Constants 00171 * @{ 00172 */ 00173 00174 #define DIGIT4_SEG7_RESET 10000 00175 /** 00176 * @} 00177 */ 00178 00179 /** @defgroup STM32_GRAVITECH_4DIGITS_Exported_Functions Exported Functions 00180 * @{ 00181 */ 00182 00183 HAL_StatusTypeDef BSP_DIGIT4_SEG7_Init(void); 00184 HAL_StatusTypeDef BSP_DIGIT4_SEG7_Display(uint32_t Value); 00185 00186 /** 00187 * @} 00188 */ 00189 00190 /** 00191 * @} 00192 */ 00193 00194 /** 00195 * @} 00196 */ 00197 00198 #ifdef __cplusplus 00199 } 00200 #endif 00201 00202 #endif /* __STM32L4XX_NUCLEO_32_H */ 00203 00204 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 00205
Generated on Tue Feb 23 2016 16:08:48 for STM32L4xx_Nucleo_32 BSP User Manual by
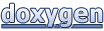