STM32L4xx_Nucleo_32 BSP User Manual
|
stm32l4xx_nucleo_32.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l4xx_nucleo_32.c 00004 * @author MCD Application Team 00005 * @version V1.0.0 00006 * @date 26-February-2016 00007 * @brief This file provides set of firmware functions to manage: 00008 * - LED available on STM32L4XX-Nucleo Kit 00009 * from STMicroelectronics. 00010 * - Gravitech 7segment shield available separatly. 00011 ****************************************************************************** 00012 * @attention 00013 * 00014 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00015 * 00016 * Redistribution and use in source and binary forms, with or without modification, 00017 * are permitted provided that the following conditions are met: 00018 * 1. Redistributions of source code must retain the above copyright notice, 00019 * this list of conditions and the following disclaimer. 00020 * 2. Redistributions in binary form must reproduce the above copyright notice, 00021 * this list of conditions and the following disclaimer in the documentation 00022 * and/or other materials provided with the distribution. 00023 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00024 * may be used to endorse or promote products derived from this software 00025 * without specific prior written permission. 00026 * 00027 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00028 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00029 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00030 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00031 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00032 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00033 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00034 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00035 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00036 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00037 * 00038 ****************************************************************************** 00039 */ 00040 00041 /* Includes ------------------------------------------------------------------*/ 00042 #include "stm32l4xx_nucleo_32.h" 00043 00044 /** @addtogroup BSP 00045 * @{ 00046 */ 00047 00048 /** @addtogroup STM32L4XX_NUCLEO_32 NUCLEO 32 00049 * @{ 00050 */ 00051 00052 /** @defgroup STM32L4XX_NUCLEO_32_Private_Constants Private Constants 00053 * @{ 00054 */ 00055 00056 /** 00057 * @brief STM32L4XX NUCLEO BSP Driver version number V1.0.0 00058 */ 00059 #define __STM32L4XX_NUCLEO_32_BSP_VERSION_MAIN (0x01) /*!< [31:24] main version */ 00060 #define __STM32L4XX_NUCLEO_32_BSP_VERSION_SUB1 (0x00) /*!< [23:16] sub1 version */ 00061 #define __STM32L4XX_NUCLEO_32_BSP_VERSION_SUB2 (0x00) /*!< [15:8] sub2 version */ 00062 #define __STM32L4XX_NUCLEO_32_BSP_VERSION_RC (0x00) /*!< [7:0] release candidate */ 00063 #define __STM32L4XX_NUCLEO_32_BSP_VERSION ((__STM32L4XX_NUCLEO_32_BSP_VERSION_MAIN << 24)\ 00064 |(__STM32L4XX_NUCLEO_32_BSP_VERSION_SUB1 << 16)\ 00065 |(__STM32L4XX_NUCLEO_32_BSP_VERSION_SUB2 << 8 )\ 00066 |(__STM32L4XX_NUCLEO_32_BSP_VERSION_RC)) 00067 00068 /** @defgroup STM32L4XX_NUCLEO_32_Private_Variables Private Variables 00069 * @{ 00070 */ 00071 GPIO_TypeDef* LED_PORT[LEDn] = {LED3_GPIO_PORT}; 00072 const uint16_t LED_PIN[LEDn] = {LED3_PIN}; 00073 00074 00075 /** 00076 * @brief BUS variables 00077 */ 00078 #if defined(HAL_I2C_MODULE_ENABLED) 00079 uint32_t I2c1Timeout = BSP_I2C1_TIMEOUT_MAX; /*<! Value of Timeout when I2C1 communication fails */ 00080 I2C_HandleTypeDef nucleo32_I2c1; 00081 #endif /* HAL_I2C_MODULE_ENABLED */ 00082 00083 /** 00084 * @} 00085 */ 00086 00087 /** @defgroup STM32L4XX_NUCLEO_32_Private_Function_Prototypes Private Function Prototypes 00088 * @{ 00089 */ 00090 00091 #if defined(HAL_I2C_MODULE_ENABLED) 00092 /* I2C1 bus function */ 00093 /* Link function for I2C peripherals */ 00094 void I2C1_Init(void); 00095 void I2C1_Error (void); 00096 void I2C1_MspInit(I2C_HandleTypeDef *hi2c); 00097 void I2C1_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00098 uint8_t I2C1_Read(uint8_t Addr, uint8_t Reg); 00099 HAL_StatusTypeDef I2C1_WriteBuffer(uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length); 00100 HAL_StatusTypeDef I2C1_ReadBuffer(uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length); 00101 HAL_StatusTypeDef I2C1_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00102 #endif /* HAL_I2C_MODULE_ENABLED */ 00103 00104 /** 00105 * @} 00106 */ 00107 00108 /** @addtogroup STM32L4XX_NUCLEO_32_Exported_Functions 00109 * @{ 00110 */ 00111 00112 /** 00113 * @brief This method returns the STM32L4XX NUCLEO BSP Driver revision. 00114 * @retval version : 0xXYZR (8bits for each decimal, R for RC) 00115 */ 00116 uint32_t BSP_GetVersion(void) 00117 { 00118 return __STM32L4XX_NUCLEO_32_BSP_VERSION; 00119 } 00120 00121 /** 00122 * @brief Configures LED GPIO. 00123 * @param Led: Specifies the Led to be configured. 00124 * This parameter can be one of following parameters: 00125 * @arg LED3 00126 * @retval None 00127 */ 00128 void BSP_LED_Init(Led_TypeDef Led) 00129 { 00130 GPIO_InitTypeDef GPIO_InitStruct; 00131 00132 /* Enable the GPIO_LED Clock */ 00133 LEDx_GPIO_CLK_ENABLE(Led); 00134 00135 /* Configure the GPIO_LED pin */ 00136 GPIO_InitStruct.Pin = LED_PIN[Led]; 00137 GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; 00138 GPIO_InitStruct.Pull = GPIO_NOPULL; 00139 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 00140 00141 HAL_GPIO_Init(LED_PORT[Led], &GPIO_InitStruct); 00142 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_RESET); 00143 } 00144 00145 /** 00146 * @brief Turns selected LED On. 00147 * @param Led: Specifies the Led to be set on. 00148 * This parameter can be one of following parameters: 00149 * @arg LED3 00150 * @retval None 00151 */ 00152 void BSP_LED_On(Led_TypeDef Led) 00153 { 00154 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_SET); 00155 } 00156 00157 /** 00158 * @brief Turns selected LED Off. 00159 * @param Led: Specifies the Led to be set off. 00160 * This parameter can be one of following parameters: 00161 * @arg LED3 00162 * @retval None 00163 */ 00164 void BSP_LED_Off(Led_TypeDef Led) 00165 { 00166 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_RESET); 00167 } 00168 00169 /** 00170 * @brief Toggles the selected LED. 00171 * @param Led: Specifies the Led to be toggled. 00172 * This parameter can be one of following parameters: 00173 * @arg LED3 00174 * @retval None 00175 */ 00176 void BSP_LED_Toggle(Led_TypeDef Led) 00177 { 00178 HAL_GPIO_TogglePin(LED_PORT[Led], LED_PIN[Led]); 00179 } 00180 00181 /** 00182 * @} 00183 */ 00184 00185 /** @defgroup STM32L4XX_NUCLEO_32_Private_Functions Private Functions 00186 * @{ 00187 */ 00188 00189 /****************************************************************************** 00190 BUS OPERATIONS 00191 *******************************************************************************/ 00192 #if defined(HAL_I2C_MODULE_ENABLED) 00193 /******************************* I2C Routines *********************************/ 00194 00195 /** 00196 * @brief I2C Bus initialization 00197 * @retval None 00198 */ 00199 void I2C1_Init(void) 00200 { 00201 if(HAL_I2C_GetState(&nucleo32_I2c1) == HAL_I2C_STATE_RESET) 00202 { 00203 nucleo32_I2c1.Instance = BSP_I2C1; 00204 nucleo32_I2c1.Init.Timing = I2C1_TIMING; 00205 nucleo32_I2c1.Init.OwnAddress1 = 0; 00206 nucleo32_I2c1.Init.AddressingMode = I2C_ADDRESSINGMODE_7BIT; 00207 nucleo32_I2c1.Init.DualAddressMode = I2C_DUALADDRESS_DISABLE; 00208 nucleo32_I2c1.Init.OwnAddress2 = 0; 00209 nucleo32_I2c1.Init.OwnAddress2Masks = I2C_OA2_NOMASK; 00210 nucleo32_I2c1.Init.GeneralCallMode = I2C_GENERALCALL_DISABLE; 00211 nucleo32_I2c1.Init.NoStretchMode = I2C_NOSTRETCH_DISABLE; 00212 00213 /* Init the I2C */ 00214 I2C1_MspInit(&nucleo32_I2c1); 00215 HAL_I2C_Init(&nucleo32_I2c1); 00216 } 00217 } 00218 00219 /** 00220 * @brief Writes a single data. 00221 * @param Addr: I2C address 00222 * @param Reg: Register address 00223 * @param Value: Data to be written 00224 * @retval None 00225 */ 00226 void I2C1_Write(uint8_t Addr, uint8_t Reg, uint8_t Value) 00227 { 00228 HAL_StatusTypeDef status = HAL_OK; 00229 00230 status = HAL_I2C_Mem_Write(&nucleo32_I2c1, Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, &Value, 1, I2c1Timeout); 00231 00232 /* Check the communication status */ 00233 if(status != HAL_OK) 00234 { 00235 /* Execute user timeout callback */ 00236 I2C1_Error(); 00237 } 00238 } 00239 00240 /** 00241 * @brief Reads a single data. 00242 * @param Addr: I2C address 00243 * @param Reg: Register address 00244 * @retval Read data 00245 */ 00246 uint8_t I2C1_Read(uint8_t Addr, uint8_t Reg) 00247 { 00248 HAL_StatusTypeDef status = HAL_OK; 00249 uint8_t Value = 0; 00250 00251 status = HAL_I2C_Mem_Read(&nucleo32_I2c1, Addr, Reg, I2C_MEMADD_SIZE_8BIT, &Value, 1, I2c1Timeout); 00252 00253 /* Check the communication status */ 00254 if(status != HAL_OK) 00255 { 00256 /* Execute user timeout callback */ 00257 I2C1_Error(); 00258 } 00259 return Value; 00260 } 00261 00262 /** 00263 * @brief Reads multiple data on the BUS. 00264 * @param Addr : I2C Address 00265 * @param Reg : Reg Address 00266 * @param RegSize : The target register size (can be 8BIT or 16BIT) 00267 * @param pBuffer : pointer to read data buffer 00268 * @param Length : length of the data 00269 * @retval 0 if no problems to read multiple data 00270 */ 00271 HAL_StatusTypeDef I2C1_ReadBuffer(uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length) 00272 { 00273 HAL_StatusTypeDef status = HAL_OK; 00274 00275 status = HAL_I2C_Mem_Read(&nucleo32_I2c1, Addr, Reg, RegSize, pBuffer, Length, I2c1Timeout); 00276 00277 /* Check the communication status */ 00278 if(status != HAL_OK) 00279 { 00280 /* Re-Initiaize the BUS */ 00281 I2C1_Error(); 00282 } 00283 return status; 00284 } 00285 00286 /** 00287 * @brief Checks if target device is ready for communication. 00288 * @note This function is used with Memory devices 00289 * @param DevAddress: Target device address 00290 * @param Trials: Number of trials 00291 * @retval HAL status 00292 */ 00293 HAL_StatusTypeDef I2C1_IsDeviceReady(uint16_t DevAddress, uint32_t Trials) 00294 { 00295 return (HAL_I2C_IsDeviceReady(&nucleo32_I2c1, DevAddress, Trials, I2c1Timeout)); 00296 } 00297 00298 /** 00299 * @brief Write a value in a register of the device through BUS. 00300 * @param Addr: Device address on BUS Bus. 00301 * @param Reg: The target register address to write 00302 * @param RegSize: The target register size (can be 8BIT or 16BIT) 00303 * @param pBuffer: The target register value to be written 00304 * @param Length: buffer size to be written 00305 * @retval None 00306 */ 00307 HAL_StatusTypeDef I2C1_WriteBuffer(uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length) 00308 { 00309 HAL_StatusTypeDef status = HAL_OK; 00310 00311 status = HAL_I2C_Mem_Write(&nucleo32_I2c1, Addr, Reg, RegSize, pBuffer, Length, I2c1Timeout); 00312 00313 /* Check the communication status */ 00314 if(status != HAL_OK) 00315 { 00316 /* Re-Initiaize the BUS */ 00317 I2C1_Error(); 00318 } 00319 return status; 00320 } 00321 00322 /** 00323 * @brief Manages error callback to re-initialize I2C. 00324 * @retval None 00325 */ 00326 void I2C1_Error(void) 00327 { 00328 /* De-initialize the I2C communication BUS */ 00329 HAL_I2C_DeInit(&nucleo32_I2c1); 00330 00331 /* Re-Initiaize the I2C communication BUS */ 00332 I2C1_Init(); 00333 } 00334 00335 /** 00336 * @brief I2C MSP Initialization 00337 * @param hi2c: I2C handle 00338 * @retval None 00339 */ 00340 void I2C1_MspInit(I2C_HandleTypeDef *hi2c) 00341 { 00342 GPIO_InitTypeDef GPIO_InitStruct; 00343 RCC_PeriphCLKInitTypeDef RCC_PeriphCLKInitStruct; 00344 00345 /*##-1- Set source clock to SYSCLK for I2C1 ################################################*/ 00346 RCC_PeriphCLKInitStruct.PeriphClockSelection = RCC_PERIPHCLK_I2C1; 00347 RCC_PeriphCLKInitStruct.I2c1ClockSelection = RCC_I2C1CLKSOURCE_SYSCLK; 00348 HAL_RCCEx_PeriphCLKConfig(&RCC_PeriphCLKInitStruct); 00349 00350 /*##-2- Configure the GPIOs ################################################*/ 00351 00352 /* Enable GPIO clock */ 00353 BSP_I2C1_GPIO_CLK_ENABLE(); 00354 00355 /* Configure I2C SCL & SDA as alternate function */ 00356 GPIO_InitStruct.Pin = (BSP_I2C1_SCL_PIN| BSP_I2C1_SDA_PIN); 00357 GPIO_InitStruct.Mode = GPIO_MODE_AF_OD; 00358 GPIO_InitStruct.Pull = GPIO_PULLUP; 00359 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 00360 GPIO_InitStruct.Alternate = BSP_I2C1_SCL_SDA_AF; 00361 HAL_GPIO_Init(BSP_I2C1_GPIO_PORT, &GPIO_InitStruct); 00362 00363 /*##-3- Configure the Eval I2C peripheral #######################################*/ 00364 /* Enable I2C clock */ 00365 BSP_I2C1_CLK_ENABLE(); 00366 00367 /* Force the I2C peripheral clock reset */ 00368 BSP_I2C1_FORCE_RESET(); 00369 00370 /* Release the I2C peripheral clock reset */ 00371 BSP_I2C1_RELEASE_RESET(); 00372 } 00373 00374 #endif /*HAL_I2C_MODULE_ENABLED*/ 00375 00376 /** 00377 * @} 00378 */ 00379 00380 /** 00381 * @} 00382 */ 00383 00384 /** 00385 * @} 00386 */ 00387 00388 /** @addtogroup STM32L4XX_NUCLEO_32_GRAVITECH_4DIGITS 00389 * @{ 00390 */ 00391 00392 /** @addtogroup STM32_GRAVITECH_4DIGITS_Exported_Functions 00393 * @{ 00394 */ 00395 00396 #if defined(HAL_I2C_MODULE_ENABLED) 00397 00398 /** 00399 * @brief BSP init of the 4 digits 7 Segment Display shield for Arduino Nano - Gravitech. 00400 * @retval HAL_StatusTypeDef 00401 */ 00402 HAL_StatusTypeDef BSP_DIGIT4_SEG7_Init(void) 00403 { 00404 uint8_t control[1] = {0x47}; 00405 00406 /* Init I2C */ 00407 I2C1_Init(); 00408 00409 /* Configure the SAA1064 component */ 00410 return I2C1_WriteBuffer(0x70, 0, 1, control, sizeof(control)); 00411 } 00412 00413 /** 00414 * @brief BSP of the 4 digits 7 Segment Display shield for Arduino Nano - Gravitech. 00415 Display the value if value belong to [0-9999] 00416 * @param Value A number between 0 and 9999 will be displayed on the screen. 00417 * DIGIT4_SEG7_RESET will reset the screen (any value above 9999 will reset the screen also) 00418 * @retval HAL_StatusTypeDef 00419 */ 00420 HAL_StatusTypeDef BSP_DIGIT4_SEG7_Display(uint32_t Value) 00421 { 00422 const uint8_t lookup[10] = {0x3F,0x06,0x5B,0x4F,0x66, 00423 0x6D,0x7D,0x07,0x7F,0x6F}; 00424 00425 uint32_t thousands, hundreds, tens, base; 00426 HAL_StatusTypeDef status = HAL_ERROR; 00427 uint8_t d1d2d3d4[4]; 00428 00429 if (Value < 10000) 00430 { 00431 thousands = Value / 1000; 00432 hundreds = (Value - (thousands * 1000)) / 100; 00433 tens = (Value - ((thousands * 1000) + (hundreds * 100))) / 10; 00434 base = Value - ((thousands * 1000) + (hundreds * 100) + (tens * 10)); 00435 00436 d1d2d3d4[3] = lookup[thousands]; 00437 d1d2d3d4[2] = lookup[hundreds]; 00438 d1d2d3d4[1] = lookup[tens]; 00439 d1d2d3d4[0] = lookup[base]; 00440 00441 } 00442 else 00443 { 00444 d1d2d3d4[3] = 0; 00445 d1d2d3d4[2] = 0; 00446 d1d2d3d4[1] = 0; 00447 d1d2d3d4[0] = 0; 00448 } 00449 00450 /* Send the four digits to the SAA1064 component */ 00451 status = I2C1_WriteBuffer(0x70, 1, 1, d1d2d3d4, sizeof(d1d2d3d4)); 00452 00453 return status; 00454 } 00455 00456 #endif /*HAL_I2C_MODULE_ENABLED*/ 00457 00458 /** 00459 * @} 00460 */ 00461 00462 /** 00463 * @} 00464 */ 00465 00466 /** 00467 * @} 00468 */ 00469 00470 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Tue Feb 23 2016 16:08:48 for STM32L4xx_Nucleo_32 BSP User Manual by
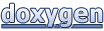