STM32L4xx_Nucleo_144 BSP User Manual
|
stm32l4xx_nucleo_144.c
Go to the documentation of this file.
00001 00002 /** 00003 ****************************************************************************** 00004 * @file stm32l4xx_nucleo_144.c 00005 * @author MCD Application Team 00006 * @brief This file provides set of firmware functions to manage: 00007 * - LEDs and push-button available on STM32L4XX-Nucleo-144 Kit 00008 * from STMicroelectronics 00009 * - LCD, joystick and microSD available on Adafruit 1.8" TFT LCD 00010 * shield (reference ID 802) 00011 ****************************************************************************** 00012 * @attention 00013 * 00014 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00015 * 00016 * Redistribution and use in source and binary forms, with or without modification, 00017 * are permitted provided that the following conditions are met: 00018 * 1. Redistributions of source code must retain the above copyright notice, 00019 * this list of conditions and the following disclaimer. 00020 * 2. Redistributions in binary form must reproduce the above copyright notice, 00021 * this list of conditions and the following disclaimer in the documentation 00022 * and/or other materials provided with the distribution. 00023 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00024 * may be used to endorse or promote products derived from this software 00025 * without specific prior written permission. 00026 * 00027 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00028 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00029 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00030 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00031 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00032 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00033 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00034 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00035 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00036 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00037 * 00038 ****************************************************************************** 00039 */ 00040 00041 /* Includes ------------------------------------------------------------------*/ 00042 #include "stm32l4xx_nucleo_144.h" 00043 00044 00045 /** @addtogroup BSP 00046 * @{ 00047 */ 00048 00049 /** @addtogroup STM32L4XX_NUCLEO_144 00050 * @brief This file provides set of firmware functions to manage Leds and push-button 00051 * available on STM32L4xx-Nucleo Kit from STMicroelectronics. 00052 * @{ 00053 */ 00054 00055 /** @defgroup STM32L4XX_NUCLEO_144_Private_Constants Private Constants 00056 * @{ 00057 */ 00058 00059 /** 00060 * @brief STM32L4xx NUCLEO BSP Driver version number 00061 */ 00062 #define __STM32L4xx_NUCLEO_144_BSP_VERSION_MAIN (0x01) /*!< [31:24] main version */ 00063 #define __STM32L4xx_NUCLEO_144_BSP_VERSION_SUB1 (0x00) /*!< [23:16] sub1 version */ 00064 #define __STM32L4xx_NUCLEO_144_BSP_VERSION_SUB2 (0x01) /*!< [15:8] sub2 version */ 00065 #define __STM32L4xx_NUCLEO_144_BSP_VERSION_RC (0x00) /*!< [7:0] release candidate */ 00066 #define __STM32L4xx_NUCLEO_144_BSP_VERSION ((__STM32L4xx_NUCLEO_144_BSP_VERSION_MAIN << 24)\ 00067 |(__STM32L4xx_NUCLEO_144_BSP_VERSION_SUB1 << 16)\ 00068 |(__STM32L4xx_NUCLEO_144_BSP_VERSION_SUB2 << 8 )\ 00069 |(__STM32L4xx_NUCLEO_144_BSP_VERSION_RC)) 00070 00071 /** 00072 * @brief LINK SD Card 00073 */ 00074 #define SD_DUMMY_BYTE 0xFF 00075 #define SD_NO_RESPONSE_EXPECTED 0x80 00076 00077 00078 #ifdef USE_STM32L4XX_NUCLEO_144_SMPS 00079 00080 /** 00081 * @brief SMPS 00082 */ 00083 00084 00085 #ifdef USE_ADP5301ACBZ /* ADP5301ACBZ */ 00086 00087 /* ######################################################################## */ 00088 /* #define PORT_SMPS GPIOG */ 00089 /* #define PIN_SMPS_ENABLE GPIO_PIN_11 */ 00090 /* #define PIN_SMPS_POWERGOOD GPIO_PIN_12 */ 00091 /* #define PIN_SMPS_SWITCH_ENABLE GPIO_PIN_13 */ 00092 00093 /* IN CASE OF SMPS VOLTAGE RANGE SELECTION */ 00094 /* #define PIN_SMPS_V1 GPIO_PIN_10 */ 00095 /* ######################################################################## */ 00096 00097 #endif /* ADP5301ACBZ */ 00098 00099 /** 00100 * @} 00101 */ 00102 00103 #endif /* USE_STM32L4XX_NUCLEO_144_SMPS */ 00104 00105 00106 /** @defgroup STM32L4XX_NUCLEO_144_Private_Variables Private Variables 00107 * @{ 00108 */ 00109 GPIO_TypeDef *GPIO_PORT[LEDn] = {LED1_GPIO_PORT, LED2_GPIO_PORT, LED3_GPIO_PORT}; 00110 00111 const uint16_t GPIO_PIN[LEDn] = {LED1_PIN, LED2_PIN, LED3_PIN}; 00112 00113 GPIO_TypeDef *BUTTON_PORT[BUTTONn] = {USER_BUTTON_GPIO_PORT}; 00114 const uint16_t BUTTON_PIN[BUTTONn] = {USER_BUTTON_PIN}; 00115 const uint8_t BUTTON_IRQn[BUTTONn] = {USER_BUTTON_EXTI_IRQn}; 00116 00117 /** 00118 * @brief BUS variables 00119 */ 00120 00121 #ifdef HAL_SPI_MODULE_ENABLED 00122 uint32_t SpixTimeout = NUCLEO_SPIx_TIMEOUT_MAX; /*<! Value of Timeout when SPI communication fails */ 00123 static SPI_HandleTypeDef hnucleo_Spi; 00124 #endif /* HAL_SPI_MODULE_ENABLED */ 00125 00126 #ifdef HAL_ADC_MODULE_ENABLED 00127 static ADC_HandleTypeDef hnucleo_Adc; 00128 /* ADC channel configuration structure declaration */ 00129 static ADC_ChannelConfTypeDef sConfig; 00130 #endif /* HAL_ADC_MODULE_ENABLED */ 00131 /** 00132 * @} 00133 */ 00134 00135 /* Private Function Prototypes */ 00136 #ifdef HAL_SPI_MODULE_ENABLED 00137 static void SPIx_Init(void); 00138 static void SPIx_MspInit(SPI_HandleTypeDef *hspi); 00139 static void SPIx_Write(uint8_t Value); 00140 static void SPIx_WriteReadData(const uint8_t *DataIn, uint8_t *DataOut, uint16_t DataLength); 00141 static void SPIx_Error(void); 00142 00143 /* SD IO functions */ 00144 void SD_IO_Init(void); 00145 void SD_IO_CSState(uint8_t state); 00146 void SD_IO_WriteReadData(const uint8_t *DataIn, uint8_t *DataOut, uint16_t DataLength); 00147 uint8_t SD_IO_WriteByte(uint8_t Data); 00148 00149 /* LCD IO functions */ 00150 void LCD_IO_Init(void); 00151 void LCD_IO_WriteData(uint8_t Data); 00152 void LCD_IO_WriteMultipleData(uint8_t *pData, uint32_t Size); 00153 void LCD_IO_WriteReg(uint8_t LCDReg); 00154 void LCD_Delay(uint32_t delay); 00155 #endif /* HAL_SPI_MODULE_ENABLED */ 00156 00157 #ifdef HAL_ADC_MODULE_ENABLED 00158 static void ADCx_Init(void); 00159 static void ADCx_DeInit(void); 00160 static void ADCx_MspInit(ADC_HandleTypeDef *hadc); 00161 static void ADCx_MspDeInit(ADC_HandleTypeDef *hadc); 00162 #endif /* HAL_ADC_MODULE_ENABLED */ 00163 00164 /** @defgroup STM32L4XX_NUCLEO_144_Exported_Functions Exported Functions 00165 * @{ 00166 */ 00167 00168 /** 00169 * @brief This method returns the STM32L4xx NUCLEO BSP Driver revision 00170 * @retval version : 0xXYZR (8bits for each decimal, R for RC) 00171 */ 00172 uint32_t BSP_GetVersion(void) 00173 { 00174 return __STM32L4xx_NUCLEO_144_BSP_VERSION; 00175 } 00176 00177 /** @defgroup STM32L4XX_NUCLEO_144_LED_Functions LED Functions 00178 * @{ 00179 */ 00180 00181 /** 00182 * @brief Configure LED GPIO. 00183 * @param Led: Specifies the Led to be configured. 00184 * This parameter can be one of following parameters: 00185 * @arg LED1 00186 * @arg LED2 00187 * @arg LED3 00188 * @retval None 00189 */ 00190 void BSP_LED_Init(Led_TypeDef Led) 00191 { 00192 GPIO_InitTypeDef GPIO_InitStruct; 00193 00194 /* Enable the GPIO_LED Clock */ 00195 LEDx_GPIO_CLK_ENABLE(Led); 00196 00197 /* Configure the GPIO_LED pin */ 00198 GPIO_InitStruct.Pin = GPIO_PIN[Led]; 00199 GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; 00200 GPIO_InitStruct.Pull = GPIO_NOPULL; 00201 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 00202 00203 HAL_GPIO_Init(GPIO_PORT[Led], &GPIO_InitStruct); 00204 HAL_GPIO_WritePin(GPIO_PORT[Led], GPIO_PIN[Led], GPIO_PIN_RESET); 00205 } 00206 00207 /** 00208 * @brief DeInitialize LEDs. 00209 * @param Led: LED to be de-init. 00210 * This parameter can be one of the following values: 00211 * @arg LED1 00212 * @arg LED2 00213 * @arg LED3 00214 * @note BSP_LED_DeInit() does not disable the GPIO clock 00215 * @retval None 00216 */ 00217 void BSP_LED_DeInit(Led_TypeDef Led) 00218 { 00219 GPIO_InitTypeDef GPIO_InitStruct; 00220 00221 /* Turn off LED */ 00222 HAL_GPIO_WritePin(GPIO_PORT[Led], GPIO_PIN[Led], GPIO_PIN_RESET); 00223 /* DeInit the GPIO_LED pin */ 00224 GPIO_InitStruct.Pin = GPIO_PIN[Led]; 00225 HAL_GPIO_DeInit(GPIO_PORT[Led], GPIO_InitStruct.Pin); 00226 } 00227 00228 /** 00229 * @brief Turn selected LED On. 00230 * @param Led: Specifies the Led to be set on. 00231 * This parameter can be one of following parameters: 00232 * @arg LED1 00233 * @arg LED2 00234 * @arg LED3 00235 * @retval None 00236 */ 00237 void BSP_LED_On(Led_TypeDef Led) 00238 { 00239 HAL_GPIO_WritePin(GPIO_PORT[Led], GPIO_PIN[Led], GPIO_PIN_SET); 00240 } 00241 00242 /** 00243 * @brief Turn selected LED Off. 00244 * @param Led: Specifies the Led to be set off. 00245 * This parameter can be one of following parameters: 00246 * @arg LED1 00247 * @arg LED2 00248 * @arg LED3 00249 * @retval None 00250 */ 00251 void BSP_LED_Off(Led_TypeDef Led) 00252 { 00253 HAL_GPIO_WritePin(GPIO_PORT[Led], GPIO_PIN[Led], GPIO_PIN_RESET); 00254 } 00255 00256 /** 00257 * @brief Toggle the selected LED. 00258 * @param Led: Specifies the Led to be toggled. 00259 * This parameter can be one of following parameters: 00260 * @arg LED1 00261 * @arg LED2 00262 * @arg LED3 00263 * @retval None 00264 */ 00265 void BSP_LED_Toggle(Led_TypeDef Led) 00266 { 00267 HAL_GPIO_TogglePin(GPIO_PORT[Led], GPIO_PIN[Led]); 00268 } 00269 00270 /** 00271 * @} 00272 */ 00273 00274 /** @defgroup STM32L4XX_NUCLEO_144_BUTTON_Functions BUTTON Functions 00275 * @{ 00276 */ 00277 00278 /** 00279 * @brief Configure Button GPIO and EXTI Line. 00280 * @param Button: Specifies the Button to be configured. 00281 * This parameter should be: BUTTON_USER 00282 * @param ButtonMode: Specifies Button mode. 00283 * This parameter can be one of following parameters: 00284 * @arg BUTTON_MODE_GPIO: Button will be used as simple IO 00285 * @arg BUTTON_MODE_EXTI: Button will be connected to EXTI line with interrupt 00286 * generation capability 00287 * @retval None 00288 */ 00289 void BSP_PB_Init(Button_TypeDef Button, ButtonMode_TypeDef ButtonMode) 00290 { 00291 GPIO_InitTypeDef GPIO_InitStruct; 00292 00293 /* Enable the BUTTON Clock */ 00294 BUTTONx_GPIO_CLK_ENABLE(Button); 00295 00296 if (ButtonMode == BUTTON_MODE_GPIO) 00297 { 00298 /* Configure Button pin as input */ 00299 GPIO_InitStruct.Pin = BUTTON_PIN[Button]; 00300 GPIO_InitStruct.Mode = GPIO_MODE_INPUT; 00301 GPIO_InitStruct.Pull = GPIO_PULLDOWN; 00302 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 00303 HAL_GPIO_Init(BUTTON_PORT[Button], &GPIO_InitStruct); 00304 } 00305 else if (ButtonMode == BUTTON_MODE_EXTI) 00306 { 00307 /* Configure Button pin as input with External interrupt */ 00308 GPIO_InitStruct.Pin = BUTTON_PIN[Button]; 00309 GPIO_InitStruct.Pull = GPIO_NOPULL; 00310 GPIO_InitStruct.Mode = GPIO_MODE_IT_FALLING; 00311 HAL_GPIO_Init(BUTTON_PORT[Button], &GPIO_InitStruct); 00312 00313 /* Enable and set Button EXTI Interrupt to the lowest priority */ 00314 HAL_NVIC_SetPriority((IRQn_Type)(BUTTON_IRQn[Button]), 0x0F, 0x00); 00315 HAL_NVIC_EnableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00316 } 00317 } 00318 00319 /** 00320 * @brief DeInitialize Push Button. 00321 * @param Button: Button to be configured 00322 * This parameter should be: BUTTON_USER 00323 * @note BSP_PB_DeInit() does not disable the GPIO clock 00324 * @retval None 00325 */ 00326 void BSP_PB_DeInit(Button_TypeDef Button) 00327 { 00328 GPIO_InitTypeDef GPIO_InitStruct; 00329 00330 GPIO_InitStruct.Pin = BUTTON_PIN[Button]; 00331 HAL_NVIC_DisableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00332 HAL_GPIO_DeInit(BUTTON_PORT[Button], GPIO_InitStruct.Pin); 00333 } 00334 00335 /** 00336 * @brief Return the selected Button state. 00337 * @param Button: Specifies the Button to be checked. 00338 * This parameter should be: BUTTON_USER 00339 * @retval The Button GPIO pin value. 00340 */ 00341 uint32_t BSP_PB_GetState(Button_TypeDef Button) 00342 { 00343 return HAL_GPIO_ReadPin(BUTTON_PORT[Button], BUTTON_PIN[Button]); 00344 } 00345 00346 #ifdef HAL_ADC_MODULE_ENABLED 00347 /** 00348 * @brief Configure joystick available on adafruit 1.8" TFT shield 00349 * managed through ADC to detect motion. 00350 * @retval Joystickstatus (0=> success, 1=> fail) 00351 */ 00352 uint8_t BSP_JOY_Init(void) 00353 { 00354 ADCx_Init(); 00355 00356 /* Select the ADC Channel to be converted */ 00357 sConfig.Channel = NUCLEO_ADCx_CHANNEL; 00358 sConfig.Rank = ADC_REGULAR_RANK_1; 00359 sConfig.SamplingTime = NUCLEO_ADCx_SAMPLETIME; 00360 sConfig.SingleDiff = ADC_SINGLE_ENDED; 00361 sConfig.OffsetNumber = ADC_OFFSET_NONE; 00362 sConfig.Offset = 0; 00363 00364 /* Return Joystick initialization status */ 00365 return (uint8_t)HAL_ADC_ConfigChannel(&hnucleo_Adc, &sConfig); 00366 } 00367 00368 /** 00369 * @brief DeInitialize joystick GPIOs. 00370 * @retval None. 00371 */ 00372 void BSP_JOY_DeInit(void) 00373 { 00374 ADCx_DeInit(); 00375 } 00376 00377 /** 00378 * @brief Return the Joystick key pressed. 00379 * @note To know which Joystick key is pressed we need to detect the voltage 00380 * level on each key output 00381 * - None : 3.3 V / 4095 00382 * - SEL : 1.055 V / 1308 00383 * - DOWN : 0.71 V / 88 00384 * - LEFT : 3.0 V / 3720 00385 * - RIGHT : 0.595 V / 737 00386 * - UP : 1.65 V / 2046 00387 * @retval JOYState_TypeDef: Code of the Joystick key pressed. 00388 */ 00389 JOYState_TypeDef BSP_JOY_GetState(void) 00390 { 00391 JOYState_TypeDef state; 00392 uint16_t keyconvertedvalue = 0; 00393 00394 /* Start the conversion process */ 00395 HAL_ADC_Start(&hnucleo_Adc); 00396 00397 /* Wait for the end of conversion */ 00398 if (HAL_ADC_PollForConversion(&hnucleo_Adc, 10) != HAL_TIMEOUT) 00399 { 00400 /* Get the converted value of regular channel */ 00401 keyconvertedvalue = HAL_ADC_GetValue(&hnucleo_Adc); 00402 } 00403 00404 if ((keyconvertedvalue > 2010) && (keyconvertedvalue < 2090)) 00405 { 00406 state = JOY_UP; 00407 } 00408 else if ((keyconvertedvalue > 680) && (keyconvertedvalue < 780)) 00409 { 00410 state = JOY_RIGHT; 00411 } 00412 else if ((keyconvertedvalue > 1270) && (keyconvertedvalue < 1350)) 00413 { 00414 state = JOY_SEL; 00415 } 00416 else if ((keyconvertedvalue > 50) && (keyconvertedvalue < 130)) 00417 { 00418 state = JOY_DOWN; 00419 } 00420 else if ((keyconvertedvalue > 3680) && (keyconvertedvalue < 3760)) 00421 { 00422 state = JOY_LEFT; 00423 } 00424 else 00425 { 00426 state = JOY_NONE; 00427 } 00428 00429 /* Loop while a key is pressed */ 00430 if (state != JOY_NONE) 00431 { 00432 keyconvertedvalue = HAL_ADC_GetValue(&hnucleo_Adc); 00433 } 00434 /* Return the code of the Joystick key pressed */ 00435 return state; 00436 } 00437 #endif /* HAL_ADC_MODULE_ENABLED */ 00438 00439 /** 00440 * @} 00441 */ 00442 00443 #ifdef USE_STM32L4XX_NUCLEO_144_SMPS 00444 #ifdef USE_ADP5301ACBZ /* ADP5301ACBZ */ 00445 00446 /** @defgroup STM32L4XX_NUCLEO_144_SMPS_Functions SMPS Functions 00447 * @{ 00448 */ 00449 00450 /****************************************************************************** 00451 SMPS OPERATIONS 00452 *******************************************************************************/ 00453 00454 /** 00455 * @brief DeInitialize the external SMPS component 00456 * @note Low power consumption GPIO settings 00457 * @retval SMPS status 00458 */ 00459 uint32_t BSP_SMPS_DeInit(void) 00460 { 00461 GPIO_InitTypeDef GPIO_InitStruct; 00462 00463 PWR_AND_CLK_SMPS(); 00464 00465 /* -------------------------------------------------------------------------------------- */ 00466 /* Added for Deinit if No PIN_SMPS_ENABLE & PIN_SMPS_SWITCH_ENABLE are not disabled before */ 00467 00468 /* Disable SMPS SWITCH */ 00469 HAL_GPIO_WritePin(PORT_SMPS, PIN_SMPS_SWITCH_ENABLE, GPIO_PIN_RESET); 00470 00471 HAL_Delay(1); 00472 00473 /* Disable SMPS */ 00474 HAL_GPIO_WritePin(PORT_SMPS, PIN_SMPS_ENABLE, GPIO_PIN_RESET); 00475 00476 /* -------------------------------------------------------------------------------------- */ 00477 00478 /* To be updated */ 00479 /* Set all GPIO in analog state to reduce power consumption, */ 00480 GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; 00481 00482 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_LOW; 00483 GPIO_InitStruct.Pull = GPIO_NOPULL; 00484 00485 GPIO_InitStruct.Pin = PIN_SMPS_SWITCH_ENABLE; 00486 00487 /* SWITCH */ 00488 HAL_GPIO_Init(GPIOG, &GPIO_InitStruct); 00489 00490 GPIO_InitStruct.Pin = PIN_SMPS_ENABLE | PIN_SMPS_SWITCH_ENABLE; 00491 /* --------- SMPS VOLTAGE RANGE SELECTION ----------------------------------*/ 00492 /* ######################################################################## */ 00493 /* GPIO_InitStruct.Pin = PIN_SMPS_ENABLE | PIN_SMPS_SWITCH_ENABLE | PIN_SMPS_V1; */ 00494 00495 /* ENABLE = OFF */ 00496 HAL_GPIO_Init(GPIOG, &GPIO_InitStruct); 00497 00498 return SMPS_OK; 00499 } 00500 00501 /** 00502 * @brief Initialize the external SMPS component 00503 * @param VoltageRange: Select operating SMPS supply 00504 * @arg DCDC_AND_BOARD_DEPENDENT 00505 * @note VoltageRange is not used with all boards 00506 * VoltageRange is not used with MB11312A/S 00507 * i.e. SMPS only PWR_REGULATOR_VOLTAGE_SCALE2. 00508 * @retval SMPS status 00509 */ 00510 uint32_t BSP_SMPS_Init(uint32_t VoltageRange) 00511 { 00512 PWR_AND_CLK_SMPS(); 00513 00514 GPIO_InitTypeDef GPIO_InitStruct; 00515 00516 /* Upon wake UP (standby case) */ 00517 /* IF PIN_SMPS_ENABLE was pulled up */ 00518 /* Then maintain PIN_SMPS_ENABLE = high */ 00519 /* Needed to keep ENABLE HIGH */ 00520 if (READ_BIT(PWR->PUCRG, PWR_GPIO_ENABLE)) 00521 { 00522 HAL_GPIO_WritePin(PORT_SMPS, PIN_SMPS_ENABLE, GPIO_PIN_SET); 00523 } 00524 else 00525 { 00526 HAL_PWREx_EnableGPIOPullDown(PWR_GPIO_SMPS, PWR_GPIO_SWITCH_ENABLE); 00527 HAL_PWREx_EnablePullUpPullDownConfig(); 00528 /* Level shifter consumes because of missing pull up/down, so pull it up (only one autorized PA13) */ 00529 HAL_PWREx_EnableGPIOPullUp(PWR_GPIO_A, GPIO_PIN_13); /* SWD/TMS */ 00530 HAL_PWREx_EnableGPIOPullUp(PWR_GPIO_B, GPIO_PIN_3); /* SWO */ 00531 } 00532 /* ------------------------------------------------------------------------ */ 00533 GPIO_InitStruct.Mode = GPIO_MODE_INPUT; 00534 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_LOW; 00535 GPIO_InitStruct.Pull = GPIO_PULLUP; 00536 00537 GPIO_InitStruct.Pin = PIN_SMPS_POWERGOOD; 00538 00539 HAL_GPIO_Init(GPIOG, &GPIO_InitStruct); 00540 00541 /* ------------------------------------------------------------------------ */ 00542 00543 GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; 00544 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_LOW; 00545 GPIO_InitStruct.Pull = GPIO_NOPULL; 00546 00547 GPIO_InitStruct.Pin = PIN_SMPS_ENABLE | PIN_SMPS_SWITCH_ENABLE; 00548 /* --------- ADD SMPS VOLTAGE RANGE SELECTION -----------------------------*/ 00549 /* - > Applicable to ST1PS02D1QTR on MB1312A/S */ 00550 /* GPIO_InitStruct.Pin = PIN_SMPS_ENABLE | PIN_SMPS_SWITCH_ENABLE | PIN_SMPS_V1; */ 00551 00552 HAL_GPIO_Init(GPIOG, &GPIO_InitStruct); 00553 00554 /* --------- SMPS VOLTAGE RANGE SELECTION ----------------------------------*/ 00555 /* ######################################################################## */ 00556 /* - > Not applicable to ADP5301A on MB1312A/S */ 00557 /* ######################################################################## */ 00558 /* - > Applicable to ST1PS02D1QTR on MB1312A/S */ 00559 /* Control to be added */ 00560 00561 /* ST1PS02D1QTR on MB1312 */ 00562 /* if (VoltageRange == ST1PS02D1QTR_VOUT_1_25) */ 00563 /* HAL_GPIO_WritePin(PORT_SMPS, PIN_SMPS_V1, GPIO_PIN_SET); */ 00564 /* 1.25V */ 00565 /* D0/D1/D2 = H/L/L */ 00566 /* else */ 00567 00568 /* */ 00569 /* ST1PS02D1QTR on MB1312 */ 00570 /* ST1PS02D1QTR_VOUT_1_05 */ 00571 /* 1.05V */ 00572 /* D0/D1/D2 = L/L/L */ 00573 /* HAL_GPIO_WritePin(PORT_SMPS, PIN_SMPS_V1, GPIO_PIN_RESET); */ 00574 /* ######################################################################## */ 00575 return SMPS_OK; 00576 } 00577 00578 /** 00579 * @brief Enable the external SMPS component 00580 * @param Delay: delay in ms after enable 00581 * @param Power_Good_Check: Enable Power good check 00582 * @note Power_Good_Check 00583 * is not used with all boards 00584 * VoltageRange is not used with MB11312A/S 00585 * i.e. SMPS only PWR_REGULATOR_VOLTAGE_SCALE2 by board. 00586 * @retval SMPS status 00587 * @arg SMPS_OK: SMPS ENABLE OK 00588 * @arg SMPS_KO: POWER GOOD CHECK FAILS 00589 * 00590 */ 00591 uint32_t BSP_SMPS_Enable(uint32_t Delay, uint32_t Power_Good_Check) 00592 { 00593 PWR_AND_CLK_SMPS(); 00594 00595 HAL_GPIO_WritePin(PORT_SMPS, PIN_SMPS_ENABLE, GPIO_PIN_SET); 00596 00597 HAL_PWREx_EnableGPIOPullUp(PWR_GPIO_SMPS, PWR_GPIO_BIT_11); 00598 HAL_PWREx_EnablePullUpPullDownConfig(); 00599 00600 /* Delay upon request */ 00601 if (Delay != 0) 00602 { 00603 HAL_Delay(Delay); 00604 } 00605 00606 /* CHECK POWER GOOD or NOT */ 00607 if (Power_Good_Check != 0) 00608 { 00609 if (GPIO_PIN_RESET == (HAL_GPIO_ReadPin(PORT_SMPS, PIN_SMPS_POWERGOOD))) 00610 { 00611 /* POWER GOOD KO */ 00612 return SMPS_KO; 00613 } 00614 } 00615 00616 /* SMPS ENABLED */ 00617 return SMPS_OK; 00618 } 00619 00620 /** 00621 * @brief Disable the external SMPS component 00622 * @note SMPS SWITCH should be disabled first ! 00623 * @retval SMPS status 00624 * @arg SMPS_OK: SMPS DISABLE OK - DONE 00625 * @arg SMPS_KO: POWER GOOD CHECK FAILS 00626 * 00627 */ 00628 uint32_t BSP_SMPS_Disable(void) 00629 { 00630 00631 PWR_AND_CLK_SMPS(); 00632 00633 /* Check if SWITCH is DISABLE */ 00634 if (HAL_GPIO_ReadPin(PORT_SMPS, PIN_SMPS_SWITCH_ENABLE) != GPIO_PIN_RESET) 00635 { 00636 /* ERROR AS SWITCH SHOULD BE DISABLED */ 00637 return SMPS_KO; 00638 } 00639 00640 /* Disable SMPS */ 00641 HAL_GPIO_WritePin(PORT_SMPS, PIN_SMPS_ENABLE, GPIO_PIN_RESET); 00642 00643 HAL_PWREx_EnableGPIOPullDown(PWR_GPIO_SMPS, PWR_GPIO_ENABLE); 00644 HAL_PWREx_EnablePullUpPullDownConfig(); 00645 00646 /* SMPS DISABLED */ 00647 return SMPS_OK; 00648 } 00649 00650 /** 00651 * @brief Enable the external SMPS SWITCH component 00652 * @param Delay: delay in ms before SMPS SWITCH ENABLE 00653 * @param Power_Good_Check: Enable Power good check 00654 * @note Power_Good_Check 00655 * is not used with all boards 00656 * VoltageRange is not used with MB11312A/S 00657 * i.e. SMPS only PWR_REGULATOR_VOLTAGE_SCALE2 by board. 00658 * @retval SMPS status 00659 * @arg SMPS_OK: SMPS ENABLE OK 00660 * @arg SMPS_KO: POWER GOOD CHECK FAILS 00661 * 00662 */ 00663 uint32_t BSP_SMPS_Supply_Enable(uint32_t Delay, uint32_t Power_Good_Check) 00664 { 00665 PWR_AND_CLK_SMPS(); 00666 00667 if (Delay != 0) 00668 { 00669 HAL_Delay(Delay); 00670 } 00671 /* CHECK POWER GOOD or NOT */ 00672 if (Power_Good_Check != 0) 00673 { 00674 if (GPIO_PIN_RESET == (HAL_GPIO_ReadPin(PORT_SMPS, PIN_SMPS_POWERGOOD))) 00675 { 00676 /* POWER GOOD KO */ 00677 return SMPS_KO; 00678 } 00679 } 00680 00681 /* SMPS SWITCH ENABLE */ 00682 HAL_GPIO_WritePin(PORT_SMPS, PIN_SMPS_SWITCH_ENABLE, GPIO_PIN_SET); 00683 00684 return SMPS_OK; 00685 } 00686 00687 /** 00688 * @brief Disable the external SMPS component 00689 * @retval SMPS status 00690 * @arg SMPS_OK: SMPS SWITCH DISABLE OK 00691 * 00692 */ 00693 uint32_t BSP_SMPS_Supply_Disable(void) 00694 { 00695 PWR_AND_CLK_SMPS(); 00696 00697 /* SMPS SWITCH DISABLED */ 00698 HAL_GPIO_WritePin(PORT_SMPS, PIN_SMPS_SWITCH_ENABLE, GPIO_PIN_RESET); 00699 00700 return SMPS_OK; 00701 } 00702 00703 #endif /* ADP5301ACBZ */ 00704 00705 #endif /* USE_STM32L4XX_NUCLEO_144_SMPS */ 00706 00707 /** 00708 * @} 00709 */ 00710 00711 /** 00712 * @} 00713 */ 00714 00715 /** @defgroup STM32L4XX_NUCLEO_144_Private_Functions Private Functions 00716 * @{ 00717 */ 00718 00719 /****************************************************************************** 00720 BUS OPERATIONS 00721 *******************************************************************************/ 00722 00723 /******************************* SPI ********************************/ 00724 #ifdef HAL_SPI_MODULE_ENABLED 00725 00726 /** 00727 * @brief Initialize SPI MSP. 00728 * @retval None 00729 */ 00730 static void SPIx_MspInit(SPI_HandleTypeDef *hspi) 00731 { 00732 GPIO_InitTypeDef GPIO_InitStruct; 00733 00734 /*** Configure the GPIOs ***/ 00735 /* Enable GPIO clock */ 00736 NUCLEO_SPIx_SCK_GPIO_CLK_ENABLE(); 00737 NUCLEO_SPIx_MISO_MOSI_GPIO_CLK_ENABLE(); 00738 00739 /* Configure SPI SCK */ 00740 GPIO_InitStruct.Pin = NUCLEO_SPIx_SCK_PIN; 00741 GPIO_InitStruct.Mode = GPIO_MODE_AF_PP; 00742 GPIO_InitStruct.Pull = GPIO_PULLUP; 00743 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 00744 GPIO_InitStruct.Alternate = NUCLEO_SPIx_SCK_AF; 00745 HAL_GPIO_Init(NUCLEO_SPIx_SCK_GPIO_PORT, &GPIO_InitStruct); 00746 00747 /* Configure SPI MISO and MOSI */ 00748 GPIO_InitStruct.Pin = NUCLEO_SPIx_MOSI_PIN; 00749 GPIO_InitStruct.Alternate = NUCLEO_SPIx_MISO_MOSI_AF; 00750 GPIO_InitStruct.Pull = GPIO_PULLDOWN; 00751 HAL_GPIO_Init(NUCLEO_SPIx_MISO_MOSI_GPIO_PORT, &GPIO_InitStruct); 00752 00753 GPIO_InitStruct.Pin = NUCLEO_SPIx_MISO_PIN; 00754 GPIO_InitStruct.Pull = GPIO_PULLDOWN; 00755 HAL_GPIO_Init(NUCLEO_SPIx_MISO_MOSI_GPIO_PORT, &GPIO_InitStruct); 00756 00757 /*** Configure the SPI peripheral ***/ 00758 /* Enable SPI clock */ 00759 NUCLEO_SPIx_CLK_ENABLE(); 00760 } 00761 00762 00763 /** 00764 * @brief Initialize SPI HAL. 00765 * @retval None 00766 */ 00767 static void SPIx_Init(void) 00768 { 00769 if (HAL_SPI_GetState(&hnucleo_Spi) == HAL_SPI_STATE_RESET) 00770 { 00771 /* SPI Config */ 00772 hnucleo_Spi.Instance = NUCLEO_SPIx; 00773 /* SPI baudrate is set to 9 MHz maximum (APB1/SPI_BaudRatePrescaler = 72/8 = 9 MHz) 00774 to verify these constraints: 00775 - ST7735 LCD SPI interface max baudrate is 15MHz for write and 6.66MHz for read 00776 Since the provided driver doesn't use read capability from LCD, only constraint 00777 on write baudrate is considered. 00778 - SD card SPI interface max baudrate is 25MHz for write/read 00779 - PCLK2 max frequency is 72 MHz 00780 */ 00781 hnucleo_Spi.Init.BaudRatePrescaler = SPI_BAUDRATEPRESCALER_8; 00782 hnucleo_Spi.Init.Direction = SPI_DIRECTION_2LINES; 00783 hnucleo_Spi.Init.CLKPhase = SPI_PHASE_2EDGE; 00784 hnucleo_Spi.Init.CLKPolarity = SPI_POLARITY_HIGH; 00785 hnucleo_Spi.Init.CRCCalculation = SPI_CRCCALCULATION_DISABLE; 00786 hnucleo_Spi.Init.CRCPolynomial = 7; 00787 hnucleo_Spi.Init.DataSize = SPI_DATASIZE_8BIT; 00788 hnucleo_Spi.Init.FirstBit = SPI_FIRSTBIT_MSB; 00789 hnucleo_Spi.Init.NSS = SPI_NSS_SOFT; 00790 hnucleo_Spi.Init.TIMode = SPI_TIMODE_DISABLE; 00791 hnucleo_Spi.Init.Mode = SPI_MODE_MASTER; 00792 00793 SPIx_MspInit(&hnucleo_Spi); 00794 HAL_SPI_Init(&hnucleo_Spi); 00795 } 00796 } 00797 00798 /** 00799 * @brief SPI Write byte(s) to device 00800 * @param DataIn: Pointer to data buffer to write 00801 * @param DataOut: Pointer to data buffer for read data 00802 * @param DataLength: number of bytes to write 00803 * @retval None 00804 */ 00805 static void SPIx_WriteReadData(const uint8_t *DataIn, uint8_t *DataOut, uint16_t DataLength) 00806 { 00807 HAL_StatusTypeDef status = HAL_OK; 00808 00809 status = HAL_SPI_TransmitReceive(&hnucleo_Spi, (uint8_t *) DataIn, DataOut, DataLength, SpixTimeout); 00810 00811 /* Check the communication status */ 00812 if (status != HAL_OK) 00813 { 00814 /* Execute user timeout callback */ 00815 SPIx_Error(); 00816 } 00817 } 00818 00819 /** 00820 * @brief SPI Write a byte to device. 00821 * @param Value: value to be written 00822 * @retval None 00823 */ 00824 static void SPIx_Write(uint8_t Value) 00825 { 00826 HAL_StatusTypeDef status = HAL_OK; 00827 uint8_t data; 00828 00829 status = HAL_SPI_TransmitReceive(&hnucleo_Spi, (uint8_t *) &Value, &data, 1, SpixTimeout); 00830 00831 /* Check the communication status */ 00832 if (status != HAL_OK) 00833 { 00834 /* Execute user timeout callback */ 00835 SPIx_Error(); 00836 } 00837 } 00838 00839 /** 00840 * @brief SPI error treatment function 00841 * @retval None 00842 */ 00843 static void SPIx_Error(void) 00844 { 00845 /* De-initialize the SPI communication BUS */ 00846 HAL_SPI_DeInit(&hnucleo_Spi); 00847 00848 /* Re-Initiaize the SPI communication BUS */ 00849 SPIx_Init(); 00850 } 00851 00852 /****************************************************************************** 00853 LINK OPERATIONS 00854 *******************************************************************************/ 00855 00856 /********************************* LINK SD ************************************/ 00857 /** 00858 * @brief Initialize the SD Card and put it into StandBy State (Ready for 00859 * data transfer). 00860 * @retval None 00861 */ 00862 void SD_IO_Init(void) 00863 { 00864 GPIO_InitTypeDef GPIO_InitStruct; 00865 uint8_t counter; 00866 00867 /* SD_CS_GPIO Periph clock enable */ 00868 SD_CS_GPIO_CLK_ENABLE(); 00869 00870 /* Configure SD_CS_PIN pin: SD Card CS pin */ 00871 GPIO_InitStruct.Pin = SD_CS_PIN; 00872 GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; 00873 GPIO_InitStruct.Pull = GPIO_PULLUP; 00874 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 00875 HAL_GPIO_Init(SD_CS_GPIO_PORT, &GPIO_InitStruct); 00876 00877 /*------------Put SD in SPI mode--------------*/ 00878 /* SD SPI Config */ 00879 SPIx_Init(); 00880 00881 /* SD chip select high */ 00882 SD_CS_HIGH(); 00883 00884 /* Send dummy byte 0xFF, 10 times with CS high */ 00885 /* Rise CS and MOSI for 80 clocks cycles */ 00886 for (counter = 0; counter <= 9; counter++) 00887 { 00888 /* Send dummy byte 0xFF */ 00889 SD_IO_WriteByte(SD_DUMMY_BYTE); 00890 } 00891 } 00892 00893 /** 00894 * @brief Set the SD_CS pin. 00895 * @param val pin value. 00896 * @retval None 00897 */ 00898 void SD_IO_CSState(uint8_t val) 00899 { 00900 if (val == 1) 00901 { 00902 SD_CS_HIGH(); 00903 } 00904 else 00905 { 00906 SD_CS_LOW(); 00907 } 00908 } 00909 00910 /** 00911 * @brief Write bytes on the SD. 00912 * @param DataIn Input Data buffer pointer. 00913 * @param DataOut Output Data buffer pointer. 00914 * @param DataLength Number of byte to send. 00915 * @retval None 00916 */ 00917 void SD_IO_WriteReadData(const uint8_t *DataIn, uint8_t *DataOut, uint16_t DataLength) 00918 { 00919 // /* SD chip select low */ 00920 // SD_CS_LOW(); 00921 /* Send the byte */ 00922 SPIx_WriteReadData(DataIn, DataOut, DataLength); 00923 } 00924 00925 /** 00926 * @brief Write a byte on the SD. 00927 * @param Data byte to send. 00928 * @retval None 00929 */ 00930 uint8_t SD_IO_WriteByte(uint8_t Data) 00931 { 00932 uint8_t tmp; 00933 /* Send the byte */ 00934 SPIx_WriteReadData(&Data, &tmp, 1); 00935 return tmp; 00936 } 00937 00938 /********************************* LINK LCD ***********************************/ 00939 /** 00940 * @brief Initialize the LCD 00941 * @retval None 00942 */ 00943 void LCD_IO_Init(void) 00944 { 00945 GPIO_InitTypeDef GPIO_InitStruct; 00946 00947 /* LCD_CS_GPIO and LCD_DC_GPIO Periph clock enable */ 00948 LCD_CS_GPIO_CLK_ENABLE(); 00949 LCD_DC_GPIO_CLK_ENABLE(); 00950 00951 /* Configure LCD_CS_PIN pin: LCD Card CS pin */ 00952 GPIO_InitStruct.Pin = LCD_CS_PIN; 00953 GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; 00954 GPIO_InitStruct.Pull = GPIO_NOPULL; 00955 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 00956 HAL_GPIO_Init(LCD_CS_GPIO_PORT, &GPIO_InitStruct); 00957 00958 /* Configure LCD_DC_PIN pin: LCD Card DC pin */ 00959 GPIO_InitStruct.Pin = LCD_DC_PIN; 00960 HAL_GPIO_Init(LCD_DC_GPIO_PORT, &GPIO_InitStruct); 00961 00962 /* LCD chip select high */ 00963 LCD_CS_HIGH(); 00964 00965 /* LCD SPI Config */ 00966 SPIx_Init(); 00967 } 00968 00969 /** 00970 * @brief Write command to select the LCD register. 00971 * @param LCDReg Address of the selected register. 00972 * @retval None 00973 */ 00974 void LCD_IO_WriteReg(uint8_t LCDReg) 00975 { 00976 /* Reset LCD control line CS */ 00977 LCD_CS_LOW(); 00978 00979 /* Set LCD data/command line DC to Low */ 00980 LCD_DC_LOW(); 00981 00982 /* Send Command */ 00983 SPIx_Write(LCDReg); 00984 00985 /* Deselect : Chip Select high */ 00986 LCD_CS_HIGH(); 00987 } 00988 00989 /** 00990 * @brief Write data to select the LCD register. 00991 * This function must be used after st7735_WriteReg() function 00992 * @param Data data to write to the selected register. 00993 * @retval None 00994 */ 00995 void LCD_IO_WriteData(uint8_t Data) 00996 { 00997 /* Reset LCD control line CS */ 00998 LCD_CS_LOW(); 00999 01000 /* Set LCD data/command line DC to High */ 01001 LCD_DC_HIGH(); 01002 01003 /* Send Data */ 01004 SPIx_Write(Data); 01005 01006 /* Deselect : Chip Select high */ 01007 LCD_CS_HIGH(); 01008 } 01009 01010 /** 01011 * @brief Write register value. 01012 * @param pData Pointer on the register value 01013 * @param Size Size of byte to transmit to the register 01014 * @retval None 01015 */ 01016 void LCD_IO_WriteMultipleData(uint8_t *pData, uint32_t Size) 01017 { 01018 uint32_t counter = 0; 01019 __IO uint32_t data = 0; 01020 01021 /* Reset LCD control line CS */ 01022 LCD_CS_LOW(); 01023 01024 /* Set LCD data/command line DC to High */ 01025 LCD_DC_HIGH(); 01026 01027 if (Size == 1) 01028 { 01029 /* Only 1 byte to be sent to LCD - general interface can be used */ 01030 /* Send Data */ 01031 SPIx_Write(*pData); 01032 } 01033 else 01034 { 01035 /* Several data should be sent in a raw */ 01036 /* Direct SPI accesses for optimization */ 01037 for (counter = Size; counter != 0; counter--) 01038 { 01039 while (((hnucleo_Spi.Instance->SR) & SPI_FLAG_TXE) != SPI_FLAG_TXE) 01040 { 01041 } 01042 /* Need to invert bytes for LCD*/ 01043 *((__IO uint8_t *)&hnucleo_Spi.Instance->DR) = *(pData + 1); 01044 01045 while (((hnucleo_Spi.Instance->SR) & SPI_FLAG_TXE) != SPI_FLAG_TXE) 01046 { 01047 } 01048 *((__IO uint8_t *)&hnucleo_Spi.Instance->DR) = *pData; 01049 counter--; 01050 pData += 2; 01051 } 01052 01053 /* Wait until the bus is ready before releasing Chip select */ 01054 while (((hnucleo_Spi.Instance->SR) & SPI_FLAG_BSY) != RESET) 01055 { 01056 } 01057 } 01058 01059 /* Empty the Rx fifo */ 01060 data = *(&hnucleo_Spi.Instance->DR); 01061 UNUSED(data); 01062 01063 /* Deselect : Chip Select high */ 01064 LCD_CS_HIGH(); 01065 } 01066 01067 /** 01068 * @brief Wait for loop in ms. 01069 * @param Delay in ms. 01070 * @retval None 01071 */ 01072 void LCD_Delay(uint32_t Delay) 01073 { 01074 HAL_Delay(Delay); 01075 } 01076 #endif /* HAL_SPI_MODULE_ENABLED */ 01077 01078 #ifdef HAL_ADC_MODULE_ENABLED 01079 /******************************* LINK JOYSTICK ********************************/ 01080 /** 01081 * @brief Initialize ADC MSP. 01082 * @param hadc ADC handle 01083 * @retval None 01084 */ 01085 static void ADCx_MspInit(ADC_HandleTypeDef *hadc) 01086 { 01087 GPIO_InitTypeDef GPIO_InitStruct; 01088 01089 /*** Configure the GPIOs ***/ 01090 /* Enable GPIO clock */ 01091 NUCLEO_ADCx_GPIO_CLK_ENABLE(); 01092 01093 /* Configure the selected ADC Channel as analog input */ 01094 GPIO_InitStruct.Pin = NUCLEO_ADCx_GPIO_PIN ; 01095 GPIO_InitStruct.Mode = GPIO_MODE_ANALOG; 01096 GPIO_InitStruct.Pull = GPIO_NOPULL; 01097 HAL_GPIO_Init(NUCLEO_ADCx_GPIO_PORT, &GPIO_InitStruct); 01098 01099 /*** Configure the ADC peripheral ***/ 01100 /* Enable ADC clock */ 01101 NUCLEO_ADCx_CLK_ENABLE(); 01102 } 01103 01104 /** 01105 * @brief DeInitialize ADC MSP. 01106 * @param hadc ADC handle 01107 * @note ADCx_MspDeInit does not disable the GPIO clock 01108 * @retval None 01109 */ 01110 static void ADCx_MspDeInit(ADC_HandleTypeDef *hadc) 01111 { 01112 GPIO_InitTypeDef GPIO_InitStruct; 01113 01114 /*** DeInit the ADC peripheral ***/ 01115 /* Disable ADC clock */ 01116 NUCLEO_ADCx_CLK_DISABLE(); 01117 01118 /* Configure the selected ADC Channel as analog input */ 01119 GPIO_InitStruct.Pin = NUCLEO_ADCx_GPIO_PIN ; 01120 HAL_GPIO_DeInit(NUCLEO_ADCx_GPIO_PORT, GPIO_InitStruct.Pin); 01121 01122 /* Disable GPIO clock has to be done by the application*/ 01123 /* NUCLEO_ADCx_GPIO_CLK_DISABLE(); */ 01124 } 01125 01126 /** 01127 * @brief Initialize ADC HAL. 01128 * @retval None 01129 */ 01130 static void ADCx_Init(void) 01131 { 01132 /* Set ADC instance */ 01133 hnucleo_Adc.Instance = NUCLEO_ADCx; 01134 01135 if (HAL_ADC_GetState(&hnucleo_Adc) == HAL_ADC_STATE_RESET) 01136 { 01137 /* ADC Config */ 01138 hnucleo_Adc.Init.ClockPrescaler = ADC_CLOCK_SYNC_PCLK_DIV4; /* ADC clock */ 01139 hnucleo_Adc.Init.Resolution = ADC_RESOLUTION_12B; 01140 hnucleo_Adc.Init.DataAlign = ADC_DATAALIGN_RIGHT; 01141 hnucleo_Adc.Init.ScanConvMode = ADC_SCAN_DISABLE; /* Sequencer disabled (ADC conversion on only 1 channel: channel set on rank 1) */ 01142 hnucleo_Adc.Init.EOCSelection = ADC_EOC_SINGLE_CONV; 01143 hnucleo_Adc.Init.LowPowerAutoWait = DISABLE; 01144 hnucleo_Adc.Init.ContinuousConvMode = DISABLE; /* Continuous mode disabled to have only 1 conversion at each conversion trig */ 01145 hnucleo_Adc.Init.NbrOfConversion = 1; /* Parameter discarded because sequencer is disabled */ 01146 hnucleo_Adc.Init.DiscontinuousConvMode = DISABLE; /* Parameter discarded because sequencer is disabled */ 01147 hnucleo_Adc.Init.NbrOfDiscConversion = 1; /* Parameter discarded because sequencer is disabled */ 01148 hnucleo_Adc.Init.ExternalTrigConv = ADC_SOFTWARE_START; /* Software start to trig the 1st conversion manually, without external event */ 01149 hnucleo_Adc.Init.ExternalTrigConvEdge = ADC_EXTERNALTRIGCONVEDGE_NONE; /* Parameter discarded because trig by software start */ 01150 hnucleo_Adc.Init.DMAContinuousRequests = DISABLE; 01151 hnucleo_Adc.Init.Overrun = ADC_OVR_DATA_OVERWRITTEN; 01152 01153 /* Initialize MSP related to ADC */ 01154 ADCx_MspInit(&hnucleo_Adc); 01155 01156 /* Initialize ADC */ 01157 HAL_ADC_Init(&hnucleo_Adc); 01158 01159 /* Run ADC calibration */ 01160 HAL_ADCEx_Calibration_Start(&hnucleo_Adc, ADC_SINGLE_ENDED); 01161 } 01162 } 01163 01164 /** 01165 * @brief DeInitialize ADC HAL. 01166 * @retval None 01167 */ 01168 static void ADCx_DeInit(void) 01169 { 01170 hnucleo_Adc.Instance = NUCLEO_ADCx; 01171 01172 HAL_ADC_DeInit(&hnucleo_Adc); 01173 ADCx_MspDeInit(&hnucleo_Adc); 01174 } 01175 01176 #endif /* HAL_ADC_MODULE_ENABLED */ 01177 01178 /** 01179 * @} 01180 */ 01181 01182 /** 01183 * @} 01184 */ 01185 01186 /** 01187 * @} 01188 */ 01189 01190 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Mon Oct 9 2017 01:35:13 for STM32L4xx_Nucleo_144 BSP User Manual by
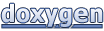