STM32L476G_EVAL BSP User Manual
|
stm32l476g_eval_ts.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l476g_eval_ts.c 00004 * @author MCD Application Team 00005 * @version $VERSION$ 00006 * @date $DATE$ 00007 * @brief This file provides a set of functions needed to manage the touch 00008 * screen on STM32L476G-EVAL evaluation board. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* File Info : ----------------------------------------------------------------- 00040 User NOTES 00041 1. How To use this driver: 00042 -------------------------- 00043 - This driver is used to drive the touch screen module of the STM32L476G-EVAL 00044 evaluation board on the HX8347G LCD mounted on MB989 rev B daughter board . 00045 - The STMPE811 IO expander device component driver must be included with this 00046 driver in order to run the TS module commanded by the IO expander device 00047 mounted on the evaluation board. 00048 00049 2. Driver description: 00050 --------------------- 00051 + Initialization steps: 00052 o Initialize the TS module using the BSP_TS_Init() function. This 00053 function includes the MSP layer hardware resources initialization and the 00054 communication layer configuration to start the TS use. The LCD size properties 00055 (x and y) are passed as parameters. 00056 o If TS interrupt mode is desired, you must configure the TS interrupt mode 00057 by calling the function BSP_TS_ITConfig(). The TS interrupt mode is generated 00058 as an external interrupt whenever a touch is detected. 00059 00060 + Touch screen use 00061 o The touch screen state is captured whenever the function BSP_TS_GetState() is 00062 used. This function returns information about the last LCD touch occurred 00063 in the TS_StateTypeDef structure. 00064 o If TS interrupt mode is used, the function BSP_TS_ITGetStatus() is needed to get 00065 the interrupt status. To clear the IT pending bits, you should call the 00066 function BSP_TS_ITClear(). 00067 o The IT is handled using the corresponding external interrupt IRQ handler, 00068 the user IT callback treatment is implemented on the same external interrupt 00069 callback. 00070 00071 ------------------------------------------------------------------------------*/ 00072 00073 /* Includes ------------------------------------------------------------------*/ 00074 #include "stm32l476g_eval_ts.h" 00075 00076 /** @addtogroup BSP 00077 * @{ 00078 */ 00079 00080 /** @addtogroup STM32L476G_EVAL 00081 * @{ 00082 */ 00083 00084 /** @defgroup STM32L476G_EVAL_TS STM32L476G_EVAL TS 00085 * @{ 00086 */ 00087 00088 /* Private types ---------------------------------------------------------*/ 00089 00090 /** @defgroup STM32L476G_EVAL_TS_Private_Types_Definitions Private Types 00091 * @{ 00092 */ 00093 /** 00094 * @} 00095 */ 00096 00097 /* Private constants ---------------------------------------------------------*/ 00098 00099 /** @defgroup STM32L476G_EVAL_TS_Private_Constants Private Constants 00100 * @{ 00101 */ 00102 /** 00103 * @} 00104 */ 00105 00106 /** @defgroup STM32L476G_EVAL_TS_Private_Macros Private Macros 00107 * @{ 00108 */ 00109 /** 00110 * @} 00111 */ 00112 00113 /* Private variables ---------------------------------------------------------*/ 00114 00115 /** @defgroup STM32L476G_EVAL_TS_Private_Variables Private Variables 00116 * @{ 00117 */ 00118 static TS_DrvTypeDef *ts_driver; 00119 static uint16_t ts_x_boundary, ts_y_boundary; 00120 static uint8_t ts_orientation; 00121 /** 00122 * @} 00123 */ 00124 00125 /* Private function prototypes -----------------------------------------------*/ 00126 00127 /** @defgroup STM32L476G_EVAL_TS_Private_Functions Private Functions 00128 * @{ 00129 */ 00130 /** 00131 * @} 00132 */ 00133 00134 /* Exported functions ---------------------------------------------------------*/ 00135 00136 /** @addtogroup STM32L476G_EVAL_TS_Exported_Functions 00137 * @{ 00138 */ 00139 00140 /** 00141 * @brief Initializes and configures the touch screen functionalities and 00142 * configures all necessary hardware resources (GPIOs, clocks..). 00143 * @param xSize: Maximum X size of the TS area on LCD 00144 * @param ySize: Maximum Y size of the TS area on LCD 00145 * @retval TS_OK if all initializations are OK. Other value if error. 00146 */ 00147 uint8_t BSP_TS_Init(uint16_t xSize, uint16_t ySize) 00148 { 00149 uint8_t ret = TS_ERROR; 00150 00151 if(stmpe811_ts_drv.ReadID(TS_I2C_ADDRESS) == STMPE811_ID) 00152 { 00153 /* Initialize the TS driver structure */ 00154 ts_driver = &stmpe811_ts_drv; 00155 00156 /* Initialize x and y positions boundaries */ 00157 ts_x_boundary = xSize; 00158 ts_y_boundary = ySize; 00159 ts_orientation = TS_SWAP_Y | TS_SWAP_XY; 00160 ret = TS_OK; 00161 } 00162 00163 if(ret == TS_OK) 00164 { 00165 /* Initialize the LL TS Driver */ 00166 ts_driver->Init(TS_I2C_ADDRESS); 00167 ts_driver->Start(TS_I2C_ADDRESS); 00168 } 00169 00170 return ret; 00171 } 00172 00173 /** 00174 * @brief Configures and enables the touch screen interrupts. 00175 * @retval TS_OK if all initializations are OK. Other value if error. 00176 */ 00177 uint8_t BSP_TS_ITConfig(void) 00178 { 00179 /* Call component driver to enable TS ITs */ 00180 ts_driver->EnableIT(TS_I2C_ADDRESS); 00181 00182 return TS_OK; 00183 } 00184 00185 /** 00186 * @brief Gets the touch screen interrupt status. 00187 * @retval TS_OK if all initializations are OK. Other value if error. 00188 */ 00189 uint8_t BSP_TS_ITGetStatus(void) 00190 { 00191 /* Call component driver to enable TS ITs */ 00192 return (ts_driver->GetITStatus(TS_I2C_ADDRESS)); 00193 } 00194 00195 /** 00196 * @brief Returns status and positions of the touch screen. 00197 * @param TS_State: Pointer to touch screen current state structure 00198 * @retval TS_OK if all initializations are OK. Other value if error. 00199 */ 00200 uint8_t BSP_TS_GetState(TS_StateTypeDef *TS_State) 00201 { 00202 static uint32_t _x = 0, _y = 0; 00203 uint16_t xDiff, yDiff , x , y; 00204 uint16_t swap; 00205 00206 TS_State->TouchDetected = ts_driver->DetectTouch(TS_I2C_ADDRESS); 00207 00208 if(TS_State->TouchDetected) 00209 { 00210 ts_driver->GetXY(TS_I2C_ADDRESS, &x, &y); 00211 00212 if(ts_orientation & TS_SWAP_X) 00213 { 00214 x = 4096 - x; 00215 } 00216 00217 if(ts_orientation & TS_SWAP_Y) 00218 { 00219 y = 4096 - y; 00220 } 00221 00222 if(ts_orientation & TS_SWAP_XY) 00223 { 00224 swap = y; 00225 y = x; 00226 x = swap; 00227 } 00228 00229 xDiff = x > _x? (x - _x): (_x - x); 00230 yDiff = y > _y? (y - _y): (_y - y); 00231 00232 if (xDiff + yDiff > 5) 00233 { 00234 _x = x; 00235 _y = y; 00236 } 00237 00238 TS_State->x = (ts_x_boundary * _x) >> 12; 00239 TS_State->y = (ts_y_boundary * _y) >> 12; 00240 } 00241 00242 return TS_OK; 00243 } 00244 00245 /** 00246 * @brief Clears all touch screen interrupts. 00247 * @retval None 00248 */ 00249 void BSP_TS_ITClear(void) 00250 { 00251 ts_driver->ClearIT(TS_I2C_ADDRESS); 00252 } 00253 00254 /** 00255 * @} 00256 */ 00257 00258 /** 00259 * @} 00260 */ 00261 00262 /** 00263 * @} 00264 */ 00265 00266 /** 00267 * @} 00268 */ 00269 00270 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Sun Jun 21 2015 23:46:41 for STM32L476G_EVAL BSP User Manual by
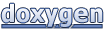