STM32L476G_EVAL BSP User Manual
|
stm32l476g_eval_sram.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l476g_eval_sram.c 00004 * @author MCD Application Team 00005 * @version $VERSION$ 00006 * @date $DATE$ 00007 * @brief This file provides a set of functions needed to drive the 00008 * IS61WV102416BLL SRAM memory mounted on STM32L476G-EVAL board. 00009 @verbatim 00010 ============================================================================== 00011 ##### How to use this driver ##### 00012 ============================================================================== 00013 [..] 00014 (#) This driver is used to drive the IS61WV102416BLL-10M SRAM external memory mounted 00015 on STM32L476G-EVAL evaluation board. 00016 00017 (#) This driver does not need a specific component driver for the SRAM device 00018 to be included with. 00019 00020 (#) Initialization steps: 00021 (++) Initialize the SRAM external memory using the BSP_SRAM_Init() function. This 00022 function includes the MSP layer hardware resources initialization and the 00023 FMC controller configuration to interface with the external SRAM memory. 00024 00025 (#) SRAM read/write operations 00026 (++) SRAM external memory can be accessed with read/write operations once it is 00027 initialized. 00028 Read/write operation can be performed with AHB access using the functions 00029 BSP_SRAM_ReadData()/BSP_SRAM_WriteData(), or by DMA transfer using the functions 00030 BSP_SRAM_ReadData_DMA()/BSP_SRAM_WriteData_DMA(). 00031 (++) The AHB access is performed with 16-bit width transaction, the DMA transfer 00032 configuration is fixed at single (no burst) halfword transfer 00033 (see the SRAM_MspInit() static function). 00034 (++) User can implement his own functions for read/write access with his desired 00035 configurations. 00036 (++) If interrupt mode is used for DMA transfer, the function BSP_SRAM_DMA_IRQHandler() 00037 is called in IRQ handler file, to serve the generated interrupt once the DMA 00038 transfer is complete. 00039 @endverbatim 00040 ****************************************************************************** 00041 * @attention 00042 * 00043 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00044 * 00045 * Redistribution and use in source and binary forms, with or without modification, 00046 * are permitted provided that the following conditions are met: 00047 * 1. Redistributions of source code must retain the above copyright notice, 00048 * this list of conditions and the following disclaimer. 00049 * 2. Redistributions in binary form must reproduce the above copyright notice, 00050 * this list of conditions and the following disclaimer in the documentation 00051 * and/or other materials provided with the distribution. 00052 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00053 * may be used to endorse or promote products derived from this software 00054 * without specific prior written permission. 00055 * 00056 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00057 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00058 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00059 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00060 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00061 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00062 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00063 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00064 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00065 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00066 * 00067 ****************************************************************************** 00068 */ 00069 00070 /* Includes ------------------------------------------------------------------*/ 00071 #include "stm32l476g_eval_sram.h" 00072 00073 /** @addtogroup BSP 00074 * @{ 00075 */ 00076 00077 /** @addtogroup STM32L476G_EVAL 00078 * @{ 00079 */ 00080 00081 /** @defgroup STM32L476G_EVAL_SRAM STM32L476G_EVAL SRAM 00082 * @{ 00083 */ 00084 00085 /* Private variables ---------------------------------------------------------*/ 00086 00087 /** @defgroup STM32L476G_EVAL_SRAM_Private_Variables Private Variables 00088 * @{ 00089 */ 00090 static SRAM_HandleTypeDef sramHandle; 00091 static FMC_NORSRAM_TimingTypeDef Timing; 00092 /** 00093 * @} 00094 */ 00095 00096 /* Private function prototypes -----------------------------------------------*/ 00097 00098 /** @defgroup STM32L476G_EVAL_SRAM_Private_Functions Private Functions 00099 * @{ 00100 */ 00101 static void SRAM_MspInit(void); 00102 00103 /** 00104 * @} 00105 */ 00106 00107 /* Exported functions ---------------------------------------------------------*/ 00108 00109 /** @addtogroup STM32L476G_EVAL_SRAM_Exported_Functions 00110 * @{ 00111 */ 00112 00113 /** 00114 * @brief Initializes the SRAM device. 00115 * @retval SRAM status 00116 */ 00117 uint8_t BSP_SRAM_Init(void) 00118 { 00119 sramHandle.Instance = FMC_NORSRAM_DEVICE; 00120 sramHandle.Extended = FMC_NORSRAM_EXTENDED_DEVICE; 00121 00122 /* SRAM device configuration */ 00123 Timing.AddressSetupTime = 2; 00124 Timing.AddressHoldTime = 1; 00125 Timing.DataSetupTime = 2; 00126 Timing.BusTurnAroundDuration = 1; 00127 Timing.CLKDivision = 2; 00128 Timing.DataLatency = 2; 00129 Timing.AccessMode = FMC_ACCESS_MODE_A; 00130 00131 sramHandle.Init.NSBank = FMC_NORSRAM_BANK1; 00132 sramHandle.Init.DataAddressMux = FMC_DATA_ADDRESS_MUX_DISABLE; 00133 sramHandle.Init.MemoryType = FMC_MEMORY_TYPE_SRAM; 00134 sramHandle.Init.MemoryDataWidth = SRAM_MEMORY_WIDTH; 00135 sramHandle.Init.BurstAccessMode = SRAM_BURSTACCESS; 00136 sramHandle.Init.WaitSignalPolarity = FMC_WAIT_SIGNAL_POLARITY_LOW; 00137 sramHandle.Init.WaitSignalActive = FMC_WAIT_TIMING_BEFORE_WS; 00138 sramHandle.Init.WriteOperation = FMC_WRITE_OPERATION_ENABLE; 00139 sramHandle.Init.WaitSignal = FMC_WAIT_SIGNAL_DISABLE; 00140 sramHandle.Init.ExtendedMode = FMC_EXTENDED_MODE_DISABLE; 00141 sramHandle.Init.AsynchronousWait = FMC_ASYNCHRONOUS_WAIT_DISABLE; 00142 sramHandle.Init.WriteBurst = SRAM_WRITEBURST; 00143 sramHandle.Init.ContinuousClock = FMC_CONTINUOUS_CLOCK_SYNC_ONLY; 00144 sramHandle.Init.WriteFifo = FMC_WRITE_FIFO_DISABLE; 00145 sramHandle.Init.PageSize = FMC_PAGE_SIZE_NONE; 00146 00147 /* SRAM controller initialization */ 00148 SRAM_MspInit(); 00149 if(HAL_SRAM_Init(&sramHandle, &Timing, &Timing) != HAL_OK) 00150 { 00151 return SRAM_ERROR; 00152 } 00153 else 00154 { 00155 return SRAM_OK; 00156 } 00157 } 00158 00159 /** 00160 * @brief Reads an amount of data from the SRAM device in polling mode. 00161 * @param uwStartAddress: Read start address 00162 * @param pData: Pointer to data to be read 00163 * @param uwDataSize: Size of read data from the memory 00164 * @retval SRAM status 00165 */ 00166 uint8_t BSP_SRAM_ReadData(uint32_t uwStartAddress, uint16_t *pData, uint32_t uwDataSize) 00167 { 00168 if(HAL_SRAM_Read_16b(&sramHandle, (uint32_t *)uwStartAddress, pData, uwDataSize) != HAL_OK) 00169 { 00170 return SRAM_ERROR; 00171 } 00172 else 00173 { 00174 return SRAM_OK; 00175 } 00176 } 00177 00178 /** 00179 * @brief Reads an amount of data from the SRAM device in DMA mode. 00180 * @param uwStartAddress: Read start address 00181 * @param pData: Pointer to data to be read 00182 * @param uwDataSize: Size of read data from the memory 00183 * @retval SRAM status 00184 */ 00185 uint8_t BSP_SRAM_ReadData_DMA(uint32_t uwStartAddress, uint16_t *pData, uint32_t uwDataSize) 00186 { 00187 if(HAL_SRAM_Read_DMA(&sramHandle, (uint32_t *)uwStartAddress, (uint32_t *)pData, uwDataSize) != HAL_OK) 00188 { 00189 return SRAM_ERROR; 00190 } 00191 else 00192 { 00193 return SRAM_OK; 00194 } 00195 } 00196 00197 /** 00198 * @brief Writes an amount of data from the SRAM device in polling mode. 00199 * @param uwStartAddress: Write start address 00200 * @param pData: Pointer to data to be written 00201 * @param uwDataSize: Size of written data from the memory 00202 * @retval SRAM status 00203 */ 00204 uint8_t BSP_SRAM_WriteData(uint32_t uwStartAddress, uint16_t *pData, uint32_t uwDataSize) 00205 { 00206 if(HAL_SRAM_Write_16b(&sramHandle, (uint32_t *)uwStartAddress, pData, uwDataSize) != HAL_OK) 00207 { 00208 return SRAM_ERROR; 00209 } 00210 else 00211 { 00212 return SRAM_OK; 00213 } 00214 } 00215 00216 /** 00217 * @brief Writes an amount of data from the SRAM device in DMA mode. 00218 * @param uwStartAddress: Write start address 00219 * @param pData: Pointer to data to be written 00220 * @param uwDataSize: Size of written data from the memory 00221 * @retval SRAM status 00222 */ 00223 uint8_t BSP_SRAM_WriteData_DMA(uint32_t uwStartAddress, uint16_t *pData, uint32_t uwDataSize) 00224 { 00225 if(HAL_SRAM_Write_DMA(&sramHandle, (uint32_t *)uwStartAddress, (uint32_t *)pData, uwDataSize) != HAL_OK) 00226 { 00227 return SRAM_ERROR; 00228 } 00229 else 00230 { 00231 return SRAM_OK; 00232 } 00233 } 00234 00235 /** 00236 * @brief Handles SRAM DMA transfer interrupt request. 00237 * @retval None 00238 */ 00239 void BSP_SRAM_DMA_IRQHandler(void) 00240 { 00241 HAL_DMA_IRQHandler(sramHandle.hdma); 00242 } 00243 00244 /** 00245 * @} 00246 */ 00247 00248 /** @addtogroup STM32L476G_EVAL_SRAM_Private_Functions 00249 * @{ 00250 */ 00251 00252 /** 00253 * @brief Initializes SRAM MSP. 00254 * @retval None 00255 */ 00256 static void SRAM_MspInit(void) 00257 { 00258 static DMA_HandleTypeDef dmaHandle; 00259 GPIO_InitTypeDef gpioinitstruct; 00260 SRAM_HandleTypeDef *hsram = &sramHandle; 00261 00262 /* Enable FMC clock */ 00263 __HAL_RCC_FMC_CLK_ENABLE(); 00264 00265 /* Enable chosen DMAx clock */ 00266 SRAM_DMAx_CLK_ENABLE(); 00267 00268 /* Enable GPIOs clock */ 00269 __HAL_RCC_GPIOD_CLK_ENABLE(); 00270 __HAL_RCC_GPIOE_CLK_ENABLE(); 00271 __HAL_RCC_GPIOF_CLK_ENABLE(); 00272 __HAL_RCC_GPIOG_CLK_ENABLE(); 00273 __HAL_RCC_PWR_CLK_ENABLE(); 00274 HAL_PWREx_EnableVddIO2(); 00275 00276 /* Common GPIO configuration */ 00277 gpioinitstruct.Mode = GPIO_MODE_AF_PP; 00278 gpioinitstruct.Pull = GPIO_PULLUP; 00279 gpioinitstruct.Speed = GPIO_SPEED_HIGH; 00280 gpioinitstruct.Alternate = GPIO_AF12_FMC; 00281 00282 /*## Data Bus #######*/ 00283 /* GPIOD configuration */ 00284 gpioinitstruct.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_8 | GPIO_PIN_9 | 00285 GPIO_PIN_10 | GPIO_PIN_14 | GPIO_PIN_15; 00286 HAL_GPIO_Init(GPIOD, &gpioinitstruct); 00287 00288 /* GPIOE configuration */ 00289 gpioinitstruct.Pin = GPIO_PIN_7 | GPIO_PIN_8 | GPIO_PIN_9 | GPIO_PIN_10 | 00290 GPIO_PIN_11 | GPIO_PIN_12 | GPIO_PIN_13 | 00291 GPIO_PIN_14 | GPIO_PIN_15; 00292 HAL_GPIO_Init(GPIOE, &gpioinitstruct); 00293 00294 /*## Address Bus #######*/ 00295 /* GPIOF configuration */ 00296 gpioinitstruct.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2 | GPIO_PIN_3 | 00297 GPIO_PIN_4 | GPIO_PIN_5 | GPIO_PIN_12 | GPIO_PIN_13 | 00298 GPIO_PIN_14 | GPIO_PIN_15; 00299 HAL_GPIO_Init(GPIOF, &gpioinitstruct); 00300 00301 00302 /* GPIOG configuration */ 00303 gpioinitstruct.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2 | 00304 GPIO_PIN_3 | GPIO_PIN_4 | GPIO_PIN_5; 00305 HAL_GPIO_Init(GPIOG, &gpioinitstruct); 00306 00307 /* GPIOD configuration */ 00308 gpioinitstruct.Pin = GPIO_PIN_11 | GPIO_PIN_12 | GPIO_PIN_13; 00309 HAL_GPIO_Init(GPIOD, &gpioinitstruct); 00310 00311 /* GPIOE configuration */ 00312 gpioinitstruct.Pin = GPIO_PIN_3 | GPIO_PIN_4 | GPIO_PIN_5; 00313 HAL_GPIO_Init(GPIOE, &gpioinitstruct); 00314 00315 /*## NOE and NWE configuration #######*/ 00316 gpioinitstruct.Pin = GPIO_PIN_4 |GPIO_PIN_5; 00317 HAL_GPIO_Init(GPIOD, &gpioinitstruct); 00318 00319 /*## NE1 configuration #######*/ 00320 gpioinitstruct.Pin = GPIO_PIN_7; 00321 HAL_GPIO_Init(GPIOD, &gpioinitstruct); 00322 00323 #if defined(USE_STM32L476G_EVAL_REVB) 00324 /*## LCD NE3 configuration #######*/ 00325 gpioinitstruct.Pin = GPIO_PIN_10; 00326 HAL_GPIO_Init(GPIOG, &gpioinitstruct); 00327 #endif /* USE_STM32L476G_EVAL_REVB */ 00328 00329 /*## NBL0, NBL1 configuration #######*/ 00330 gpioinitstruct.Pin = GPIO_PIN_0 | GPIO_PIN_1; 00331 HAL_GPIO_Init(GPIOE, &gpioinitstruct); 00332 00333 /* Configure common DMA parameters */ 00334 dmaHandle.Init.Direction = DMA_MEMORY_TO_MEMORY; 00335 dmaHandle.Init.PeriphInc = DMA_PINC_ENABLE; 00336 dmaHandle.Init.MemInc = DMA_MINC_ENABLE; 00337 dmaHandle.Init.PeriphDataAlignment = DMA_PDATAALIGN_HALFWORD; 00338 dmaHandle.Init.MemDataAlignment = DMA_MDATAALIGN_HALFWORD; 00339 dmaHandle.Init.Mode = DMA_NORMAL; 00340 dmaHandle.Init.Priority = DMA_PRIORITY_HIGH; 00341 00342 dmaHandle.Instance = SRAM_DMAx_CHANNEL; 00343 00344 /* Associate the DMA handle */ 00345 __HAL_LINKDMA(hsram, hdma, dmaHandle); 00346 00347 /* Deinitialize the Stream for new transfer */ 00348 HAL_DMA_DeInit(&dmaHandle); 00349 00350 /* Configure the DMA Stream */ 00351 HAL_DMA_Init(&dmaHandle); 00352 00353 /* NVIC configuration for DMA transfer complete interrupt */ 00354 HAL_NVIC_SetPriority(SRAM_DMAx_IRQn, 5, 0); 00355 HAL_NVIC_EnableIRQ(SRAM_DMAx_IRQn); 00356 } 00357 00358 /** 00359 * @} 00360 */ 00361 00362 /** 00363 * @} 00364 */ 00365 00366 /** 00367 * @} 00368 */ 00369 00370 /** 00371 * @} 00372 */ 00373 00374 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Sun Jun 21 2015 23:46:41 for STM32L476G_EVAL BSP User Manual by
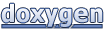