STM32L476G_EVAL BSP User Manual
|
stm32l476g_eval_sd.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l476g_eval_sd.c 00004 * @author MCD Application Team 00005 * @version $VERSION$ 00006 * @date $DATE$ 00007 * @brief This file includes the uSD card driver. 00008 @verbatim 00009 ============================================================================== 00010 ##### How to use this driver ##### 00011 ============================================================================== 00012 (#) This driver is used to drive the micro SD external card mounted on STM32L476G-EVAL 00013 evaluation board. 00014 00015 (#) This driver does not need a specific component driver for the micro SD device 00016 to be included with. 00017 00018 (#) Initialization steps: 00019 (++) Initialize the micro SD card using the BSP_SD_Init() function. This 00020 function includes the MSP layer hardware resources initialization and the 00021 SDMMC1 interface configuration to interface with the external micro SD. It 00022 also includes the micro SD initialization sequence. 00023 (++) To check the SD card presence you can use the function BSP_SD_IsDetected() which 00024 returns the detection status 00025 (++) If SD presence detection interrupt mode is desired, you must configure the 00026 SD detection interrupt mode by calling the function BSP_SD_ITConfig(). The interrupt 00027 is generated as an external interrupt whenever the micro SD card is 00028 plugged/unplugged in/from the evaluation board. The SD detection interrupt 00029 is handeled by calling the function BSP_SD_DetectIT() which is called in the IRQ 00030 handler file, the user callback is implemented in the function BSP_SD_DetectCallback(). 00031 (++) The function BSP_SD_GetCardInfo() is used to get the micro SD card information 00032 which is stored in the structure "HAL_SD_CardInfoTypedef". 00033 00034 (#) Micro SD card operations 00035 (++) The micro SD card can be accessed with read/write block(s) operations once 00036 it is reay for access. The access cand be performed whether using the polling 00037 mode by calling the functions BSP_SD_ReadBlocks()/BSP_SD_WriteBlocks(), or by DMA 00038 transfer using the functions BSP_SD_ReadBlocks_DMA()/BSP_SD_WriteBlocks_DMA() 00039 (++) The DMA transfer complete is used with interrupt mode. Once the SD transfer 00040 is complete, the SD interrupt is handeled using the function BSP_SD_IRQHandler(), 00041 the DMA Tx/Rx transfer complete are handeled using the functions 00042 BSP_SD_DMA_Tx_IRQHandler()/BSP_SD_DMA_Rx_IRQHandler(). The corresponding user callbacks 00043 are implemented by the user at application level. 00044 (++) The SD erase block(s) is performed using the function BSP_SD_Erase() with specifying 00045 the number of blocks to erase. 00046 (++) The SD runtime status is returned when calling the function BSP_SD_GetStatus(). 00047 [..] 00048 @endverbatim 00049 ****************************************************************************** 00050 * @attention 00051 * 00052 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00053 * 00054 * Redistribution and use in source and binary forms, with or without modification, 00055 * are permitted provided that the following conditions are met: 00056 * 1. Redistributions of source code must retain the above copyright notice, 00057 * this list of conditions and the following disclaimer. 00058 * 2. Redistributions in binary form must reproduce the above copyright notice, 00059 * this list of conditions and the following disclaimer in the documentation 00060 * and/or other materials provided with the distribution. 00061 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00062 * may be used to endorse or promote products derived from this software 00063 * without specific prior written permission. 00064 * 00065 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00066 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00067 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00068 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00069 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00070 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00071 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00072 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00073 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00074 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00075 * 00076 ****************************************************************************** 00077 */ 00078 00079 /* Includes ------------------------------------------------------------------*/ 00080 #include "stm32l476g_eval_sd.h" 00081 00082 /** @addtogroup BSP 00083 * @{ 00084 */ 00085 00086 /** @addtogroup STM32L476G_EVAL 00087 * @{ 00088 */ 00089 00090 /** @defgroup STM32L476G_EVAL_SD STM32L476G_EVAL SD 00091 * @{ 00092 */ 00093 00094 /* Private variables ---------------------------------------------------------*/ 00095 00096 /** @defgroup STM32L476G_SD_Private_Variables Private Variables 00097 * @{ 00098 */ 00099 SD_HandleTypeDef uSdHandle; 00100 static SD_CardInfo uSdCardInfo; 00101 /** 00102 * @} 00103 */ 00104 00105 /* Private function prototypes -----------------------------------------------*/ 00106 00107 /** @defgroup STM32L476G_EVAL_SD_Private_Functions Private Functions 00108 * @{ 00109 */ 00110 static void SD_MspInit(void); 00111 HAL_SD_ErrorTypedef SD_DMAConfigRx(SD_HandleTypeDef *hsd); 00112 HAL_SD_ErrorTypedef SD_DMAConfigTx(SD_HandleTypeDef *hsd); 00113 00114 /** 00115 * @} 00116 */ 00117 00118 /* Exported functions ---------------------------------------------------------*/ 00119 00120 /** @addtogroup STM32L476G_EVAL_SD_Exported_Functions 00121 * @{ 00122 */ 00123 00124 /** 00125 * @brief Initializes the SD card device. 00126 * @retval SD status. 00127 */ 00128 uint8_t BSP_SD_Init(void) 00129 { 00130 uint8_t state = MSD_OK; 00131 00132 /* uSD device interface configuration */ 00133 uSdHandle.Instance = SDMMC1; 00134 00135 uSdHandle.Init.ClockEdge = SDMMC_CLOCK_EDGE_RISING; 00136 uSdHandle.Init.ClockBypass = SDMMC_CLOCK_BYPASS_DISABLE; 00137 uSdHandle.Init.ClockPowerSave = SDMMC_CLOCK_POWER_SAVE_DISABLE; 00138 uSdHandle.Init.BusWide = SDMMC_BUS_WIDE_1B; 00139 uSdHandle.Init.HardwareFlowControl = SDMMC_HARDWARE_FLOW_CONTROL_DISABLE; 00140 uSdHandle.Init.ClockDiv = 2; 00141 00142 /* Check if the SD card is plugged in the slot */ 00143 SD_MspInit(); 00144 if(BSP_SD_IsDetected() != SD_PRESENT) 00145 { 00146 return MSD_ERROR; 00147 } 00148 00149 /* HAL SD initialization */ 00150 if(HAL_SD_Init(&uSdHandle, &uSdCardInfo) != SD_OK) 00151 { 00152 state = MSD_ERROR; 00153 } 00154 00155 /* Configure SD Bus width */ 00156 if(state == MSD_OK) 00157 { 00158 /* Enable wide operation */ 00159 if(HAL_SD_WideBusOperation_Config(&uSdHandle, SDMMC_BUS_WIDE_4B) != SD_OK) 00160 { 00161 state = MSD_ERROR; 00162 } 00163 else 00164 { 00165 state = MSD_OK; 00166 } 00167 } 00168 00169 return state; 00170 } 00171 00172 /** 00173 * @brief Configures Interrupt mode for SD detection pin. 00174 * @retval Returns 0 00175 */ 00176 uint8_t BSP_SD_ITConfig(void) 00177 { 00178 GPIO_InitTypeDef gpioinitstruct = {0}; 00179 00180 /* Configure Interrupt mode for SD detection pin */ 00181 gpioinitstruct.Mode = GPIO_MODE_IT_RISING_FALLING; 00182 gpioinitstruct.Pull = GPIO_PULLUP; 00183 gpioinitstruct.Speed = GPIO_SPEED_HIGH; 00184 gpioinitstruct.Pin = SD_DETECT_PIN; 00185 HAL_GPIO_Init(SD_DETECT_GPIO_PORT, &gpioinitstruct); 00186 00187 /* NVIC configuration for SD detection interrupts */ 00188 HAL_NVIC_SetPriority(SD_DETECT_IRQn, 5, 0); 00189 HAL_NVIC_EnableIRQ(SD_DETECT_IRQn); 00190 00191 return 0; 00192 } 00193 00194 /** 00195 * @brief Detects if SD card is correctly plugged in the memory slot or not. 00196 * @retval Returns if SD is detected or not 00197 */ 00198 uint8_t BSP_SD_IsDetected(void) 00199 { 00200 __IO uint8_t status = SD_PRESENT; 00201 00202 /* Check SD card detect pin */ 00203 if(HAL_GPIO_ReadPin(SD_DETECT_GPIO_PORT, SD_DETECT_PIN) != GPIO_PIN_RESET) 00204 { 00205 status = SD_NOT_PRESENT; 00206 } 00207 00208 return status; 00209 } 00210 00211 /** @brief SD detect IT treatment 00212 * @retval None 00213 */ 00214 void BSP_SD_DetectIT(void) 00215 { 00216 /* SD detect IT callback */ 00217 BSP_SD_DetectCallback(); 00218 00219 } 00220 00221 00222 /** @brief SD detect IT detection callback 00223 * @retval None 00224 */ 00225 __weak void BSP_SD_DetectCallback(void) 00226 { 00227 /* NOTE: This function Should not be modified, when the callback is needed, 00228 the BSP_SD_DetectCallback could be implemented in the user file 00229 */ 00230 00231 } 00232 00233 /** 00234 * @brief Reads block(s) from a specified address in an SD card, in polling mode. 00235 * @param pData: Pointer to the buffer that will contain the data to transmit 00236 * @param ReadAddr: Address from where data is to be read 00237 * @param BlockSize: SD card data block size, that should be 512 00238 * @param NumOfBlocks: Number of SD blocks to read 00239 * @retval SD status 00240 */ 00241 uint8_t BSP_SD_ReadBlocks(uint32_t *pData, uint64_t ReadAddr, uint32_t BlockSize, uint32_t NumOfBlocks) 00242 { 00243 if(HAL_SD_ReadBlocks(&uSdHandle, pData, ReadAddr, BlockSize, NumOfBlocks) != SD_OK) 00244 { 00245 return MSD_ERROR; 00246 } 00247 else 00248 { 00249 return MSD_OK; 00250 } 00251 } 00252 00253 /** 00254 * @brief Writes block(s) to a specified address in an SD card, in polling mode. 00255 * @param pData: Pointer to the buffer that will contain the data to transmit 00256 * @param WriteAddr: Address from where data is to be written 00257 * @param BlockSize: SD card data block size, that should be 512 00258 * @param NumOfBlocks: Number of SD blocks to write 00259 * @retval SD status 00260 */ 00261 uint8_t BSP_SD_WriteBlocks(uint32_t *pData, uint64_t WriteAddr, uint32_t BlockSize, uint32_t NumOfBlocks) 00262 { 00263 if(HAL_SD_WriteBlocks(&uSdHandle, pData, WriteAddr, BlockSize, NumOfBlocks) != SD_OK) 00264 { 00265 return MSD_ERROR; 00266 } 00267 else 00268 { 00269 return MSD_OK; 00270 } 00271 } 00272 00273 /** 00274 * @brief Reads block(s) from a specified address in an SD card, in DMA mode. 00275 * @param pData: Pointer to the buffer that will contain the data to transmit 00276 * @param ReadAddr: Address from where data is to be read 00277 * @param BlockSize: SD card data block size, that should be 512 00278 * @param NumOfBlocks: Number of SD blocks to read 00279 * @retval SD status 00280 */ 00281 uint8_t BSP_SD_ReadBlocks_DMA(uint32_t *pData, uint64_t ReadAddr, uint32_t BlockSize, uint32_t NumOfBlocks) 00282 { 00283 uint8_t state = MSD_OK; 00284 00285 /* Invalidate the dma tx handle*/ 00286 uSdHandle.hdmatx = NULL; 00287 00288 /* Prepare the dma channel for a read operation */ 00289 state = ((SD_DMAConfigRx(&uSdHandle) == SD_OK) ? MSD_OK : MSD_ERROR); 00290 00291 if(state == MSD_OK) 00292 { 00293 /* Read block(s) in DMA transfer mode */ 00294 state = ((HAL_SD_ReadBlocks_DMA(&uSdHandle, pData, ReadAddr, BlockSize, NumOfBlocks) == SD_OK) ? MSD_OK : MSD_ERROR); 00295 00296 /* Wait until transfer is complete */ 00297 if(state == MSD_OK) 00298 { 00299 state = ((HAL_SD_CheckReadOperation(&uSdHandle, (uint32_t)SD_DATATIMEOUT) == SD_OK) ? MSD_OK : MSD_ERROR); 00300 } 00301 } 00302 00303 return state; 00304 } 00305 00306 /** 00307 * @brief Writes block(s) to a specified address in an SD card, in DMA mode. 00308 * @param pData: Pointer to the buffer that will contain the data to transmit 00309 * @param WriteAddr: Address from where data is to be written 00310 * @param BlockSize: SD card data block size, that should be 512 00311 * @param NumOfBlocks: Number of SD blocks to write 00312 * @retval SD status 00313 */ 00314 uint8_t BSP_SD_WriteBlocks_DMA(uint32_t *pData, uint64_t WriteAddr, uint32_t BlockSize, uint32_t NumOfBlocks) 00315 { 00316 uint8_t state = MSD_OK; 00317 00318 /* Invalidate the dma rx handle*/ 00319 uSdHandle.hdmarx = NULL; 00320 00321 /* Prepare the dma channel for a read operation */ 00322 state = ((SD_DMAConfigTx(&uSdHandle) == SD_OK) ? MSD_OK : MSD_ERROR); 00323 00324 if(state == MSD_OK) 00325 { 00326 /* Write block(s) in DMA transfer mode */ 00327 state = ((HAL_SD_WriteBlocks_DMA(&uSdHandle, pData, WriteAddr, BlockSize, NumOfBlocks) == SD_OK) ? MSD_OK : MSD_ERROR); 00328 00329 /* Wait until transfer is complete */ 00330 if(state == MSD_OK) 00331 { 00332 state = ((HAL_SD_CheckWriteOperation(&uSdHandle, (uint32_t)SD_DATATIMEOUT) == SD_OK) ? MSD_OK : MSD_ERROR); 00333 } 00334 } 00335 00336 return state; 00337 } 00338 00339 /** 00340 * @brief Erases the specified memory area of the given SD card. 00341 * @param StartAddr: Start byte address 00342 * @param EndAddr: End byte address 00343 * @retval SD status 00344 */ 00345 uint8_t BSP_SD_Erase(uint64_t StartAddr, uint64_t EndAddr) 00346 { 00347 if(HAL_SD_Erase(&uSdHandle, StartAddr, EndAddr) != SD_OK) 00348 { 00349 return MSD_ERROR; 00350 } 00351 else 00352 { 00353 return MSD_OK; 00354 } 00355 } 00356 00357 /** 00358 * @brief Handles SD card interrupt request. 00359 * @retval None 00360 */ 00361 void BSP_SD_IRQHandler(void) 00362 { 00363 HAL_SD_IRQHandler(&uSdHandle); 00364 } 00365 00366 /** 00367 * @brief Handles SD DMA Tx transfer interrupt request. 00368 * @retval None 00369 */ 00370 void BSP_SD_DMA_Tx_IRQHandler(void) 00371 { 00372 HAL_DMA_IRQHandler(uSdHandle.hdmatx); 00373 } 00374 00375 /** 00376 * @brief Handles SD DMA Rx transfer interrupt request. 00377 * @retval None 00378 */ 00379 void BSP_SD_DMA_Rx_IRQHandler(void) 00380 { 00381 HAL_DMA_IRQHandler(uSdHandle.hdmarx); 00382 } 00383 00384 /** 00385 * @brief Gets the current SD card data status. 00386 * @retval Data transfer state. 00387 * This value can be one of the following values: 00388 * @arg SD_TRANSFER_OK: No data transfer is acting 00389 * @arg SD_TRANSFER_BUSY: Data transfer is acting 00390 * @arg SD_TRANSFER_ERROR: Data transfer error 00391 */ 00392 HAL_SD_TransferStateTypedef BSP_SD_GetStatus(void) 00393 { 00394 return(HAL_SD_GetStatus(&uSdHandle)); 00395 } 00396 00397 /** 00398 * @brief Get SD information about specific SD card. 00399 * @param CardInfo: Pointer to HAL_SD_CardInfoTypedef structure 00400 * @retval None 00401 */ 00402 void BSP_SD_GetCardInfo(HAL_SD_CardInfoTypedef *CardInfo) 00403 { 00404 /* Get SD card Information */ 00405 HAL_SD_Get_CardInfo(&uSdHandle, CardInfo); 00406 } 00407 00408 /** 00409 * @} 00410 */ 00411 00412 00413 /** @addtogroup STM32L476G_EVAL_SD_Private_Functions 00414 * @{ 00415 */ 00416 00417 /** 00418 * @brief Initializes the SD MSP. 00419 * @retval None 00420 */ 00421 static void SD_MspInit(void) 00422 { 00423 GPIO_InitTypeDef gpioinitstruct = {0}; 00424 RCC_PeriphCLKInitTypeDef RCC_PeriphClkInit; 00425 00426 /* Configure the Eval SDMMC1 clock source. The clock is derived from the PLLSAI1 */ 00427 RCC_PeriphClkInit.PeriphClockSelection = RCC_PERIPHCLK_SDMMC1; 00428 RCC_PeriphClkInit.PLLSAI1.PLLSAI1N = 24; 00429 RCC_PeriphClkInit.PLLSAI1.PLLSAI1Q = 4; 00430 RCC_PeriphClkInit.PLLSAI1.PLLSAI1ClockOut = RCC_PLLSAI1_48M2CLK; 00431 RCC_PeriphClkInit.Sdmmc1ClockSelection = RCC_SDMMC1CLKSOURCE_PLLSAI1; 00432 if(HAL_RCCEx_PeriphCLKConfig(&RCC_PeriphClkInit) != HAL_OK) 00433 { 00434 while(1) {} 00435 } 00436 00437 /* Enable SDMMC1 clock */ 00438 __HAL_RCC_SDMMC1_CLK_ENABLE(); 00439 00440 /* Enable DMA2 clocks */ 00441 __DMAx_TxRx_CLK_ENABLE(); 00442 00443 /* Enable GPIOs clock */ 00444 __HAL_RCC_GPIOC_CLK_ENABLE(); 00445 __HAL_RCC_GPIOD_CLK_ENABLE(); 00446 __SD_DETECT_GPIO_CLK_ENABLE(); 00447 00448 /* Common GPIO configuration */ 00449 gpioinitstruct.Mode = GPIO_MODE_AF_PP; 00450 gpioinitstruct.Pull = GPIO_PULLUP; 00451 gpioinitstruct.Speed = GPIO_SPEED_HIGH; 00452 gpioinitstruct.Alternate = GPIO_AF12_SDMMC1; 00453 00454 /* GPIOC configuration */ 00455 gpioinitstruct.Pin = GPIO_PIN_8 | GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_11 | GPIO_PIN_12; 00456 00457 HAL_GPIO_Init(GPIOC, &gpioinitstruct); 00458 00459 /* GPIOD configuration */ 00460 gpioinitstruct.Pin = GPIO_PIN_2; 00461 HAL_GPIO_Init(GPIOD, &gpioinitstruct); 00462 00463 /* SD Card detect pin configuration */ 00464 gpioinitstruct.Mode = GPIO_MODE_INPUT; 00465 gpioinitstruct.Pull = GPIO_PULLUP; 00466 gpioinitstruct.Speed = GPIO_SPEED_HIGH; 00467 gpioinitstruct.Pin = SD_DETECT_PIN; 00468 HAL_GPIO_Init(SD_DETECT_GPIO_PORT, &gpioinitstruct); 00469 00470 /* NVIC configuration for SDMMC1 interrupts */ 00471 HAL_NVIC_SetPriority(SDMMC1_IRQn, 5, 0); 00472 HAL_NVIC_EnableIRQ(SDMMC1_IRQn); 00473 00474 /* DMA initialization should be done here but , as there is only one channel for RX and TX it is configured and done directly when required*/ 00475 } 00476 00477 /** 00478 * @brief Configure the DMA to receive data from the SD card 00479 * @retval 00480 * SD_ERROR or SD_OK 00481 */ 00482 HAL_SD_ErrorTypedef SD_DMAConfigRx(SD_HandleTypeDef *hsd) 00483 { 00484 static DMA_HandleTypeDef hdma_rx; 00485 HAL_StatusTypeDef status = HAL_ERROR; 00486 00487 /* Configure DMA Rx parameters */ 00488 hdma_rx.Init.Request = DMA_REQUEST_7; 00489 hdma_rx.Init.Direction = DMA_PERIPH_TO_MEMORY; 00490 hdma_rx.Init.PeriphInc = DMA_PINC_DISABLE; 00491 hdma_rx.Init.MemInc = DMA_MINC_ENABLE; 00492 hdma_rx.Init.PeriphDataAlignment = DMA_PDATAALIGN_WORD; 00493 hdma_rx.Init.MemDataAlignment = DMA_MDATAALIGN_WORD; 00494 hdma_rx.Init.Priority = DMA_PRIORITY_VERY_HIGH; 00495 00496 hdma_rx.Instance = DMA2_Channel4; 00497 00498 /* Associate the DMA handle */ 00499 __HAL_LINKDMA(hsd, hdmarx, hdma_rx); 00500 00501 /* Stop any ongoing transfer and reset the state*/ 00502 HAL_DMA_Abort(&hdma_rx); 00503 00504 /* Deinitialize the Channel for new transfer */ 00505 HAL_DMA_DeInit(&hdma_rx); 00506 00507 /* Configure the DMA Channel */ 00508 status = HAL_DMA_Init(&hdma_rx); 00509 00510 /* NVIC configuration for DMA transfer complete interrupt */ 00511 HAL_NVIC_SetPriority(DMA2_Channel4_IRQn, 6, 0); 00512 HAL_NVIC_EnableIRQ(DMA2_Channel4_IRQn); 00513 00514 return (status != HAL_OK? SD_ERROR : SD_OK); 00515 } 00516 00517 /** 00518 * @brief Configure the DMA to transmit data to the SD card 00519 * @retval 00520 * SD_ERROR or SD_OK 00521 */ 00522 HAL_SD_ErrorTypedef SD_DMAConfigTx(SD_HandleTypeDef *hsd) 00523 { 00524 static DMA_HandleTypeDef hdma_tx; 00525 HAL_StatusTypeDef status; 00526 00527 /* Configure DMA Tx parameters */ 00528 hdma_tx.Init.Request = DMA_REQUEST_7; 00529 hdma_tx.Init.Direction = DMA_MEMORY_TO_PERIPH; 00530 hdma_tx.Init.PeriphInc = DMA_PINC_DISABLE; 00531 hdma_tx.Init.MemInc = DMA_MINC_ENABLE; 00532 hdma_tx.Init.PeriphDataAlignment = DMA_PDATAALIGN_WORD; 00533 hdma_tx.Init.MemDataAlignment = DMA_MDATAALIGN_WORD; 00534 hdma_tx.Init.Priority = DMA_PRIORITY_VERY_HIGH; 00535 00536 hdma_tx.Instance = DMA2_Channel4; 00537 00538 /* Associate the DMA handle */ 00539 __HAL_LINKDMA(hsd, hdmatx, hdma_tx); 00540 00541 /* Stop any ongoing transfer and reset the state*/ 00542 HAL_DMA_Abort(&hdma_tx); 00543 00544 /* Deinitialize the Channel for new transfer */ 00545 HAL_DMA_DeInit(&hdma_tx); 00546 00547 /* Configure the DMA Channel */ 00548 status = HAL_DMA_Init(&hdma_tx); 00549 00550 /* NVIC configuration for DMA transfer complete interrupt */ 00551 HAL_NVIC_SetPriority(DMA2_Channel4_IRQn, 6, 0); 00552 HAL_NVIC_EnableIRQ(DMA2_Channel4_IRQn); 00553 00554 return (status != HAL_OK? SD_ERROR : SD_OK); 00555 } 00556 00557 /** 00558 * @} 00559 */ 00560 00561 /** 00562 * @} 00563 */ 00564 00565 /** 00566 * @} 00567 */ 00568 00569 /** 00570 * @} 00571 */ 00572 00573 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Sun Jun 21 2015 23:46:41 for STM32L476G_EVAL BSP User Manual by
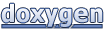