STM32L476G_EVAL BSP User Manual
|
stm32l476g_eval_lcd.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l476g_eval_lcd.c 00004 * @author MCD Application Team 00005 * @version $VERSION$ 00006 * @date $DATE$ 00007 * @brief This file includes the driver for Liquid Crystal Display (LCD) module 00008 * mounted on STM32L476G-EVAL evaluation board. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* File Info : ----------------------------------------------------------------- 00040 User NOTES 00041 1. How To use this driver: 00042 -------------------------- 00043 - This driver is used to drive indirectly an LCD TFT. 00044 - This driver supports the SPFD5408 LCD mounted on MB895 daughter board 00045 - The SPFD5408 component driver MUST be included with this driver. 00046 00047 2. Driver description: 00048 --------------------- 00049 + Initialization steps: 00050 o Initialize the LCD using the BSP_LCD_Init() function. 00051 00052 + Display on LCD 00053 o Clear the hole LCD using yhe BSP_LCD_Clear() function or only one specified 00054 string line using the BSP_LCD_ClearStringLine() function. 00055 o Display a character on the specified line and column using the BSP_LCD_DisplayChar() 00056 function or a complete string line using the BSP_LCD_DisplayStringAtLine() function. 00057 o Display a string line on the specified position (x,y in pixel) and align mode 00058 using the BSP_LCD_DisplayStringAtLine() function. 00059 o Draw and fill a basic shapes (dot, line, rectangle, circle, ellipse, .. bitmap, raw picture) 00060 on LCD using a set of functions. 00061 00062 ------------------------------------------------------------------------------*/ 00063 00064 /* Includes ------------------------------------------------------------------*/ 00065 #include "stm32l476g_eval_lcd.h" 00066 #include "../../../Utilities/Fonts/fonts.h" 00067 #include "../../../Utilities/Fonts/font24.c" 00068 #include "../../../Utilities/Fonts/font20.c" 00069 #include "../../../Utilities/Fonts/font16.c" 00070 #include "../../../Utilities/Fonts/font12.c" 00071 #include "../../../Utilities/Fonts/font8.c" 00072 00073 /** @addtogroup BSP 00074 * @{ 00075 */ 00076 00077 /** @addtogroup STM32L476G_EVAL 00078 * @{ 00079 */ 00080 00081 /** @defgroup STM32L476G_EVAL_LCD STM32L476G_EVAL LCD 00082 * @{ 00083 */ 00084 00085 /** @defgroup STM32L476G_EVAL_LCD_Private_Types_Definitions Private Types Definitions 00086 * @{ 00087 */ 00088 00089 /** 00090 * @} 00091 */ 00092 00093 /** @defgroup STM32L476G_EVAL_LCD_Private_Constants Private Constants 00094 * @{ 00095 */ 00096 #define POLY_X(Z) ((int32_t)((pPoints + (Z))->X)) 00097 #define POLY_Y(Z) ((int32_t)((pPoints + (Z))->Y)) 00098 00099 #define MAX_HEIGHT_FONT 17 00100 #define MAX_WIDTH_FONT 24 00101 #define OFFSET_BITMAP 54 00102 /** 00103 * @} 00104 */ 00105 00106 /** @defgroup STM32L476G_EVAL_LCD_Private_Macros Private Macros 00107 * @{ 00108 */ 00109 #define ABS(X) ((X) > 0 ? (X) : -(X)) 00110 00111 /** 00112 * @} 00113 */ 00114 00115 /** @defgroup STM32L476G_EVAL_LCD_Private_Variables Private Variables 00116 * @{ 00117 */ 00118 LCD_DrawPropTypeDef DrawProp; 00119 00120 static LCD_DrvTypeDef *lcd_drv; 00121 00122 /* Max size of bitmap will based on a font24 (17x24) */ 00123 static uint8_t bitmap[MAX_HEIGHT_FONT*MAX_WIDTH_FONT*2+OFFSET_BITMAP] = {0}; 00124 00125 /** 00126 * @} 00127 */ 00128 00129 /** @defgroup STM32L476G_EVAL_LCD_Private_Functions Private Functions 00130 * @{ 00131 */ 00132 static void LCD_DrawPixel(uint16_t Xpos, uint16_t Ypos, uint16_t RGBCode); 00133 static void LCD_DrawChar(uint16_t Xpos, uint16_t Ypos, const uint8_t *pChar); 00134 static void LCD_SetDisplayWindow(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height); 00135 00136 /** 00137 * @} 00138 */ 00139 00140 /** @addtogroup STM32L476G_EVAL_LCD_Exported_Functions 00141 * @{ 00142 */ 00143 00144 /** 00145 * @brief Initializes the LCD. 00146 * @retval LCD state 00147 */ 00148 uint8_t BSP_LCD_Init(void) 00149 { 00150 uint8_t ret = LCD_ERROR; 00151 00152 /* Default value for draw propriety */ 00153 DrawProp.BackColor = 0xFFFF; 00154 DrawProp.pFont = &Font24; 00155 DrawProp.TextColor = 0x0000; 00156 00157 if(hx8347g_drv.ReadID() == HX8347G_ID) 00158 { 00159 lcd_drv = &hx8347g_drv; 00160 00161 /* LCD Init */ 00162 lcd_drv->Init(); 00163 00164 /* Initialize the font */ 00165 BSP_LCD_SetFont(&LCD_DEFAULT_FONT); 00166 00167 ret = LCD_OK; 00168 } 00169 00170 return ret; 00171 } 00172 00173 /** 00174 * @brief Gets the LCD X size. 00175 * @retval Used LCD X size 00176 */ 00177 uint32_t BSP_LCD_GetXSize(void) 00178 { 00179 return(lcd_drv->GetLcdPixelWidth()); 00180 } 00181 00182 /** 00183 * @brief Gets the LCD Y size. 00184 * @retval Used LCD Y size 00185 */ 00186 uint32_t BSP_LCD_GetYSize(void) 00187 { 00188 return(lcd_drv->GetLcdPixelHeight()); 00189 } 00190 00191 /** 00192 * @brief Gets the LCD text color. 00193 * @retval Used text color. 00194 */ 00195 uint16_t BSP_LCD_GetTextColor(void) 00196 { 00197 return DrawProp.TextColor; 00198 } 00199 00200 /** 00201 * @brief Gets the LCD background color. 00202 * @retval Used background color 00203 */ 00204 uint16_t BSP_LCD_GetBackColor(void) 00205 { 00206 return DrawProp.BackColor; 00207 } 00208 00209 /** 00210 * @brief Sets the LCD text color. 00211 * @param Color: Text color code RGB(5-6-5) 00212 * @retval None 00213 */ 00214 void BSP_LCD_SetTextColor(uint16_t Color) 00215 { 00216 DrawProp.TextColor = Color; 00217 } 00218 00219 /** 00220 * @brief Sets the LCD background color. 00221 * @param Color: Background color code RGB(5-6-5) 00222 * @retval None 00223 */ 00224 void BSP_LCD_SetBackColor(uint16_t Color) 00225 { 00226 DrawProp.BackColor = Color; 00227 } 00228 00229 /** 00230 * @brief Sets the LCD text font. 00231 * @param pFonts: Font to be used 00232 * @retval None 00233 */ 00234 void BSP_LCD_SetFont(sFONT *pFonts) 00235 { 00236 DrawProp.pFont = pFonts; 00237 } 00238 00239 /** 00240 * @brief Gets the LCD text font. 00241 * @retval Used font 00242 */ 00243 sFONT *BSP_LCD_GetFont(void) 00244 { 00245 return DrawProp.pFont; 00246 } 00247 00248 /** 00249 * @brief Clears the hole LCD. 00250 * @param Color: Color of the background 00251 * @retval None 00252 */ 00253 void BSP_LCD_Clear(uint16_t Color) 00254 { 00255 uint32_t counter = 0; 00256 00257 uint32_t color_backup = DrawProp.TextColor; 00258 DrawProp.TextColor = Color; 00259 00260 for(counter = 0; counter < BSP_LCD_GetYSize(); counter++) 00261 { 00262 BSP_LCD_DrawHLine(0, counter, BSP_LCD_GetXSize()); 00263 } 00264 00265 DrawProp.TextColor = color_backup; 00266 BSP_LCD_SetTextColor(DrawProp.TextColor); 00267 } 00268 00269 /** 00270 * @brief Clears the selected line. 00271 * @param Line: Line to be cleared 00272 * This parameter can be one of the following values: 00273 * @arg 0..9: if the Current fonts is Font16x24 00274 * @arg 0..19: if the Current fonts is Font12x12 or Font8x12 00275 * @arg 0..29: if the Current fonts is Font8x8 00276 * @retval None 00277 */ 00278 void BSP_LCD_ClearStringLine(uint16_t Line) 00279 { 00280 uint32_t colorbackup = DrawProp.TextColor; 00281 DrawProp.TextColor = DrawProp.BackColor;; 00282 00283 /* Draw a rectangle with background color */ 00284 BSP_LCD_FillRect(0, (Line * DrawProp.pFont->Height), BSP_LCD_GetXSize(), DrawProp.pFont->Height); 00285 00286 DrawProp.TextColor = colorbackup; 00287 BSP_LCD_SetTextColor(DrawProp.TextColor); 00288 } 00289 00290 /** 00291 * @brief Displays one character. 00292 * @param Xpos: Start column address 00293 * @param Ypos: Line where to display the character shape. 00294 * @param Ascii: Character ascii code 00295 * This parameter must be a number between Min_Data = 0x20 and Max_Data = 0x7E 00296 * @retval None 00297 */ 00298 void BSP_LCD_DisplayChar(uint16_t Xpos, uint16_t Ypos, uint8_t Ascii) 00299 { 00300 LCD_DrawChar(Ypos, Xpos, &DrawProp.pFont->table[(Ascii-' ') *\ 00301 DrawProp.pFont->Height * ((DrawProp.pFont->Width + 7) / 8)]); 00302 } 00303 00304 /** 00305 * @brief Displays characters on the LCD. 00306 * @param Xpos: X position (in pixel) 00307 * @param Ypos: Y position (in pixel) 00308 * @param pText: Pointer to string to display on LCD 00309 * @param Mode: Display mode 00310 * This parameter can be one of the following values: 00311 * @arg CENTER_MODE 00312 * @arg RIGHT_MODE 00313 * @arg LEFT_MODE 00314 * @retval None 00315 */ 00316 void BSP_LCD_DisplayStringAt(uint16_t Xpos, uint16_t Ypos, uint8_t *pText, Line_ModeTypdef Mode) 00317 { 00318 uint16_t refcolumn = 1, counter = 0; 00319 uint32_t size = 0, ysize = 0; 00320 uint8_t *ptr = pText; 00321 00322 /* Get the text size */ 00323 while (*ptr++) size ++ ; 00324 00325 /* Characters number per line */ 00326 ysize = (BSP_LCD_GetXSize()/DrawProp.pFont->Width); 00327 00328 switch (Mode) 00329 { 00330 case CENTER_MODE: 00331 { 00332 refcolumn = Xpos + ((ysize - size)* DrawProp.pFont->Width) / 2; 00333 break; 00334 } 00335 case LEFT_MODE: 00336 { 00337 refcolumn = Xpos; 00338 break; 00339 } 00340 case RIGHT_MODE: 00341 { 00342 refcolumn = -Xpos + ((ysize - size)*DrawProp.pFont->Width); 00343 break; 00344 } 00345 default: 00346 { 00347 refcolumn = Xpos; 00348 break; 00349 } 00350 } 00351 00352 /* Send the string character by character on lCD */ 00353 while ((*pText != 0) & (((BSP_LCD_GetXSize() - (counter*DrawProp.pFont->Width)) & 0xFFFF) >= DrawProp.pFont->Width)) 00354 { 00355 /* Display one character on LCD */ 00356 BSP_LCD_DisplayChar(refcolumn, Ypos, *pText); 00357 /* Decrement the column position by 16 */ 00358 refcolumn += DrawProp.pFont->Width; 00359 /* Point on the next character */ 00360 pText++; 00361 counter++; 00362 } 00363 } 00364 00365 /** 00366 * @brief Displays a character on the LCD. 00367 * @param Line: Line where to display the character shape 00368 * This parameter can be one of the following values: 00369 * @arg 0..9: if the Current fonts is Font16x24 00370 * @arg 0..19: if the Current fonts is Font12x12 or Font8x12 00371 * @arg 0..29: if the Current fonts is Font8x8 00372 * @param pText: Pointer to string to display on LCD 00373 * @retval None 00374 */ 00375 void BSP_LCD_DisplayStringAtLine(uint16_t Line, uint8_t *pText) 00376 { 00377 BSP_LCD_DisplayStringAt(0, LINE(Line),pText, LEFT_MODE); 00378 } 00379 00380 /** 00381 * @brief Reads an LCD pixel. 00382 * @param Xpos: X position 00383 * @param Ypos: Y position 00384 * @retval RGB pixel color 00385 */ 00386 uint16_t BSP_LCD_ReadPixel(uint16_t Xpos, uint16_t Ypos) 00387 { 00388 uint16_t ret = 0; 00389 00390 if(lcd_drv->ReadPixel != NULL) 00391 { 00392 ret = lcd_drv->ReadPixel(Xpos, Ypos); 00393 } 00394 00395 return ret; 00396 } 00397 00398 /** 00399 * @brief Draws an horizontal line. 00400 * @param Xpos: X position 00401 * @param Ypos: Y position 00402 * @param Length: Line length 00403 * @retval None 00404 */ 00405 void BSP_LCD_DrawHLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length) 00406 { 00407 uint32_t index = 0; 00408 00409 if(lcd_drv->DrawHLine != NULL) 00410 { 00411 lcd_drv->DrawHLine(DrawProp.TextColor, Ypos, Xpos, Length); 00412 } 00413 else 00414 { 00415 for(index = 0; index < Length; index++) 00416 { 00417 LCD_DrawPixel((Ypos + index), Xpos, DrawProp.TextColor); 00418 } 00419 } 00420 } 00421 00422 /** 00423 * @brief Draws a vertical line. 00424 * @param Xpos: X position 00425 * @param Ypos: Y position 00426 * @param Length: Line length 00427 * @retval None 00428 */ 00429 void BSP_LCD_DrawVLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length) 00430 { 00431 uint32_t index = 0; 00432 00433 if(lcd_drv->DrawVLine != NULL) 00434 { 00435 LCD_SetDisplayWindow(Ypos, Xpos, 1, Length); 00436 lcd_drv->DrawVLine(DrawProp.TextColor, Ypos, Xpos, Length); 00437 LCD_SetDisplayWindow(0, 0, BSP_LCD_GetXSize(), BSP_LCD_GetYSize()); 00438 } 00439 else 00440 { 00441 for(index = 0; index < Length; index++) 00442 { 00443 LCD_DrawPixel(Ypos, Xpos + index, DrawProp.TextColor); 00444 } 00445 } 00446 } 00447 00448 /** 00449 * @brief Draws an uni-line (between two points). 00450 * @param X1: Point 1 X position 00451 * @param Y1: Point 1 Y position 00452 * @param X2: Point 2 X position 00453 * @param Y2: Point 2 Y position 00454 * @retval None 00455 */ 00456 void BSP_LCD_DrawLine(uint16_t X1, uint16_t Y1, uint16_t X2, uint16_t Y2) 00457 { 00458 int16_t deltax = 0, deltay = 0, x = 0, y = 0, xinc1 = 0, xinc2 = 0, 00459 yinc1 = 0, yinc2 = 0, den = 0, num = 0, numadd = 0, numpixels = 0, 00460 curpixel = 0; 00461 00462 deltax = ABS(Y2 - Y1); /* The difference between the x's */ 00463 deltay = ABS(X2 - X1); /* The difference between the y's */ 00464 x = Y1; /* Start x off at the first pixel */ 00465 y = X1; /* Start y off at the first pixel */ 00466 00467 if (Y2 >= Y1) /* The x-values are increasing */ 00468 { 00469 xinc1 = 1; 00470 xinc2 = 1; 00471 } 00472 else /* The x-values are decreasing */ 00473 { 00474 xinc1 = -1; 00475 xinc2 = -1; 00476 } 00477 00478 if (X2 >= X1) /* The y-values are increasing */ 00479 { 00480 yinc1 = 1; 00481 yinc2 = 1; 00482 } 00483 else /* The y-values are decreasing */ 00484 { 00485 yinc1 = -1; 00486 yinc2 = -1; 00487 } 00488 00489 if (deltax >= deltay) /* There is at least one x-value for every y-value */ 00490 { 00491 xinc1 = 0; /* Don't change the x when numerator >= denominator */ 00492 yinc2 = 0; /* Don't change the y for every iteration */ 00493 den = deltax; 00494 num = deltax / 2; 00495 numadd = deltay; 00496 numpixels = deltax; /* There are more x-values than y-values */ 00497 } 00498 else /* There is at least one y-value for every x-value */ 00499 { 00500 xinc2 = 0; /* Don't change the x for every iteration */ 00501 yinc1 = 0; /* Don't change the y when numerator >= denominator */ 00502 den = deltay; 00503 num = deltay / 2; 00504 numadd = deltax; 00505 numpixels = deltay; /* There are more y-values than x-values */ 00506 } 00507 00508 for (curpixel = 0; curpixel <= numpixels; curpixel++) 00509 { 00510 LCD_DrawPixel(x, y, DrawProp.TextColor); /* Draw the current pixel */ 00511 num += numadd; /* Increase the numerator by the top of the fraction */ 00512 if (num >= den) /* Check if numerator >= denominator */ 00513 { 00514 num -= den; /* Calculate the new numerator value */ 00515 x += xinc1; /* Change the x as appropriate */ 00516 y += yinc1; /* Change the y as appropriate */ 00517 } 00518 x += xinc2; /* Change the x as appropriate */ 00519 y += yinc2; /* Change the y as appropriate */ 00520 } 00521 } 00522 00523 /** 00524 * @brief Draws a rectangle. 00525 * @param Xpos: X position 00526 * @param Ypos: Y position 00527 * @param Width: Rectangle width 00528 * @param Height: Rectangle height 00529 * @retval None 00530 */ 00531 void BSP_LCD_DrawRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 00532 { 00533 /* Draw horizontal lines */ 00534 BSP_LCD_DrawHLine(Xpos, Ypos, Width); 00535 BSP_LCD_DrawHLine(Xpos, (Ypos+ Height), Width); 00536 00537 /* Draw vertical lines */ 00538 BSP_LCD_DrawVLine(Xpos, Ypos, Height); 00539 BSP_LCD_DrawVLine((Xpos + Width), Ypos, Height); 00540 } 00541 00542 /** 00543 * @brief Draws a circle. 00544 * @param Xpos: X position 00545 * @param Ypos: Y position 00546 * @param Radius: Circle radius 00547 * @retval None 00548 */ 00549 void BSP_LCD_DrawCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius) 00550 { 00551 int32_t decision; /* Decision Variable */ 00552 uint32_t curx; /* Current X Value */ 00553 uint32_t cury; /* Current Y Value */ 00554 00555 decision = 3 - (Radius << 1); 00556 curx = 0; 00557 cury = Radius; 00558 00559 while (curx <= cury) 00560 { 00561 LCD_DrawPixel((Ypos + curx), (Xpos - cury), DrawProp.TextColor); 00562 00563 LCD_DrawPixel((Ypos - curx), (Xpos - cury), DrawProp.TextColor); 00564 00565 LCD_DrawPixel((Ypos + cury), (Xpos - curx), DrawProp.TextColor); 00566 00567 LCD_DrawPixel((Ypos - cury), (Xpos - curx), DrawProp.TextColor); 00568 00569 LCD_DrawPixel((Ypos + curx), (Xpos + cury), DrawProp.TextColor); 00570 00571 LCD_DrawPixel((Ypos - curx), (Xpos + cury), DrawProp.TextColor); 00572 00573 LCD_DrawPixel((Ypos + cury), (Xpos + curx), DrawProp.TextColor); 00574 00575 LCD_DrawPixel((Ypos - cury), (Xpos + curx), DrawProp.TextColor); 00576 00577 /* Initialize the font */ 00578 BSP_LCD_SetFont(&LCD_DEFAULT_FONT); 00579 00580 if (decision < 0) 00581 { 00582 decision += (curx << 2) + 6; 00583 } 00584 else 00585 { 00586 decision += ((curx - cury) << 2) + 10; 00587 cury--; 00588 } 00589 curx++; 00590 } 00591 } 00592 00593 /** 00594 * @brief Draws an poly-line (between many points). 00595 * @param pPoints: Pointer to the points array 00596 * @param PointCount: Number of points 00597 * @retval None 00598 */ 00599 void BSP_LCD_DrawPolygon(pPoint pPoints, uint16_t PointCount) 00600 { 00601 int16_t x = 0, y = 0; 00602 00603 if(PointCount < 2) 00604 { 00605 return; 00606 } 00607 00608 BSP_LCD_DrawLine(pPoints->X, pPoints->Y, (pPoints+PointCount-1)->X, (pPoints+PointCount-1)->Y); 00609 00610 while(--PointCount) 00611 { 00612 x = pPoints->X; 00613 y = pPoints->Y; 00614 pPoints++; 00615 BSP_LCD_DrawLine(x, y, pPoints->X, pPoints->Y); 00616 } 00617 00618 } 00619 00620 /** 00621 * @brief Draws an ellipse on LCD. 00622 * @param Xpos: X position 00623 * @param Ypos: Y position 00624 * @param XRadius: Ellipse X radius 00625 * @param YRadius: Ellipse Y radius 00626 * @retval None 00627 */ 00628 void BSP_LCD_DrawEllipse(int Xpos, int Ypos, int XRadius, int YRadius) 00629 { 00630 int x = 0, y = -XRadius, err = 2-2*YRadius, e2; 00631 float k = 0, rad1 = 0, rad2 = 0; 00632 00633 rad1 = YRadius; 00634 rad2 = XRadius; 00635 00636 k = (float)(rad2/rad1); 00637 00638 do { 00639 LCD_DrawPixel((Ypos-(uint16_t)(x/k)), (Xpos+y), DrawProp.TextColor); 00640 LCD_DrawPixel((Ypos+(uint16_t)(x/k)), (Xpos+y), DrawProp.TextColor); 00641 LCD_DrawPixel((Ypos+(uint16_t)(x/k)), (Xpos-y), DrawProp.TextColor); 00642 LCD_DrawPixel((Ypos-(uint16_t)(x/k)), (Xpos-y), DrawProp.TextColor); 00643 00644 e2 = err; 00645 if (e2 <= x) { 00646 err += ++x*2+1; 00647 if (-y == x && e2 <= y) e2 = 0; 00648 } 00649 if (e2 > y) err += ++y*2+1; 00650 } 00651 while (y <= 0); 00652 } 00653 00654 /** 00655 * @brief Draws a bitmap picture loaded in the internal Flash (32 bpp). 00656 * @param Xpos: Bmp X position in the LCD 00657 * @param Ypos: Bmp Y position in the LCD 00658 * @param pBmp: Pointer to Bmp picture address in the internal Flash 00659 * @retval None 00660 */ 00661 void BSP_LCD_DrawBitmap(uint16_t Xpos, uint16_t Ypos, uint8_t *pBmp) 00662 { 00663 uint32_t height = 0, width = 0; 00664 00665 /* Read bitmap width */ 00666 width = *(uint16_t *) (pBmp + 18); 00667 width |= (*(uint16_t *) (pBmp + 20)) << 16; 00668 00669 /* Read bitmap height */ 00670 height = *(uint16_t *) (pBmp + 22); 00671 height |= (*(uint16_t *) (pBmp + 24)) << 16; 00672 00673 /* Remap Ypos, hx8347g works with inverted X in case of bitmap */ 00674 /* X = 0, cursor is on Bottom corner */ 00675 if(lcd_drv == &hx8347g_drv) 00676 { 00677 Ypos = BSP_LCD_GetYSize() - Ypos - height; 00678 } 00679 00680 LCD_SetDisplayWindow(Ypos, Xpos, width, height); 00681 00682 if(lcd_drv->DrawBitmap != NULL) 00683 { 00684 lcd_drv->DrawBitmap(Ypos, Xpos, pBmp); 00685 } 00686 LCD_SetDisplayWindow(0, 0, BSP_LCD_GetXSize(), BSP_LCD_GetYSize()); 00687 } 00688 00689 /** 00690 * @brief Draws a full rectangle. 00691 * @param Xpos: X position 00692 * @param Ypos: Y position 00693 * @param Width: Rectangle width 00694 * @param Height: Rectangle height 00695 * @retval None 00696 */ 00697 void BSP_LCD_FillRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 00698 { 00699 BSP_LCD_SetTextColor(DrawProp.TextColor); 00700 do 00701 { 00702 BSP_LCD_DrawHLine(Xpos, Ypos++, Width); 00703 } 00704 while(Height--); 00705 } 00706 00707 /** 00708 * @brief Draws a full circle. 00709 * @param Xpos: X position 00710 * @param Ypos: Y position 00711 * @param Radius: Circle radius 00712 * @retval None 00713 */ 00714 void BSP_LCD_FillCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius) 00715 { 00716 int32_t decision; /* Decision Variable */ 00717 uint32_t curx; /* Current X Value */ 00718 uint32_t cury; /* Current Y Value */ 00719 00720 decision = 3 - (Radius << 1); 00721 00722 curx = 0; 00723 cury = Radius; 00724 00725 BSP_LCD_SetTextColor(DrawProp.TextColor); 00726 00727 while (curx <= cury) 00728 { 00729 if(cury > 0) 00730 { 00731 BSP_LCD_DrawVLine(Xpos + curx, Ypos - cury, 2*cury); 00732 BSP_LCD_DrawVLine(Xpos - curx, Ypos - cury, 2*cury); 00733 } 00734 00735 if(curx > 0) 00736 { 00737 BSP_LCD_DrawVLine(Xpos - cury, Ypos - curx, 2*curx); 00738 BSP_LCD_DrawVLine(Xpos + cury, Ypos - curx, 2*curx); 00739 } 00740 if (decision < 0) 00741 { 00742 decision += (curx << 2) + 6; 00743 } 00744 else 00745 { 00746 decision += ((curx - cury) << 2) + 10; 00747 cury--; 00748 } 00749 curx++; 00750 } 00751 00752 BSP_LCD_SetTextColor(DrawProp.TextColor); 00753 BSP_LCD_DrawCircle(Xpos, Ypos, Radius); 00754 } 00755 00756 /** 00757 * @brief Fill triangle. 00758 * @param X1: specifies the point 1 x position. 00759 * @param X2: specifies the point 2 x position. 00760 * @param X3: specifies the point 3 x position. 00761 * @param Y1: specifies the point 1 y position. 00762 * @param Y2: specifies the point 2 y position. 00763 * @param Y3: specifies the point 3 y position. 00764 * @retval None 00765 */ 00766 void BSP_LCD_FillTriangle(uint16_t X1, uint16_t X2, uint16_t X3, uint16_t Y1, uint16_t Y2, uint16_t Y3) 00767 { 00768 int16_t deltax = 0, deltay = 0, x = 0, y = 0, xinc1 = 0, xinc2 = 0, 00769 yinc1 = 0, yinc2 = 0, den = 0, num = 0, numadd = 0, numpixels = 0, 00770 curpixel = 0; 00771 00772 deltax = ABS(X2 - X1); /* The difference between the x's */ 00773 deltay = ABS(Y2 - Y1); /* The difference between the y's */ 00774 x = X1; /* Start x off at the first pixel */ 00775 y = Y1; /* Start y off at the first pixel */ 00776 00777 if (X2 >= X1) /* The x-values are increasing */ 00778 { 00779 xinc1 = 1; 00780 xinc2 = 1; 00781 } 00782 else /* The x-values are decreasing */ 00783 { 00784 xinc1 = -1; 00785 xinc2 = -1; 00786 } 00787 00788 if (Y2 >= Y1) /* The y-values are increasing */ 00789 { 00790 yinc1 = 1; 00791 yinc2 = 1; 00792 } 00793 else /* The y-values are decreasing */ 00794 { 00795 yinc1 = -1; 00796 yinc2 = -1; 00797 } 00798 00799 if (deltax >= deltay) /* There is at least one x-value for every y-value */ 00800 { 00801 xinc1 = 0; /* Don't change the x when numerator >= denominator */ 00802 yinc2 = 0; /* Don't change the y for every iteration */ 00803 den = deltax; 00804 num = deltax / 2; 00805 numadd = deltay; 00806 numpixels = deltax; /* There are more x-values than y-values */ 00807 } 00808 else /* There is at least one y-value for every x-value */ 00809 { 00810 xinc2 = 0; /* Don't change the x for every iteration */ 00811 yinc1 = 0; /* Don't change the y when numerator >= denominator */ 00812 den = deltay; 00813 num = deltay / 2; 00814 numadd = deltax; 00815 numpixels = deltay; /* There are more y-values than x-values */ 00816 } 00817 00818 for (curpixel = 0; curpixel <= numpixels; curpixel++) 00819 { 00820 BSP_LCD_DrawLine(x, y, X3, Y3); 00821 00822 num += numadd; /* Increase the numerator by the top of the fraction */ 00823 if (num >= den) /* Check if numerator >= denominator */ 00824 { 00825 num -= den; /* Calculate the new numerator value */ 00826 x += xinc1; /* Change the x as appropriate */ 00827 y += yinc1; /* Change the y as appropriate */ 00828 } 00829 x += xinc2; /* Change the x as appropriate */ 00830 y += yinc2; /* Change the y as appropriate */ 00831 } 00832 } 00833 00834 /** 00835 * @brief Displays a full poly-line (between many points). 00836 * @param pPoints: pointer to the points array. 00837 * @param PointCount: Number of points. 00838 * @retval None 00839 */ 00840 void BSP_LCD_FillPolygon(pPoint pPoints, uint16_t PointCount) 00841 { 00842 00843 int16_t x = 0, y = 0, x2 = 0, y2 = 0, xcenter = 0, ycenter = 0, xfirst = 0, yfirst = 0, pixelx = 0, pixely = 0, counter = 0; 00844 uint16_t imageleft = 0, imageright = 0, imagetop = 0, imagebottom = 0; 00845 00846 imageleft = imageright = pPoints->X; 00847 imagetop= imagebottom = pPoints->Y; 00848 00849 for(counter = 1; counter < PointCount; counter++) 00850 { 00851 pixelx = POLY_X(counter); 00852 if(pixelx < imageleft) 00853 { 00854 imageleft = pixelx; 00855 } 00856 if(pixelx > imageright) 00857 { 00858 imageright = pixelx; 00859 } 00860 00861 pixely = POLY_Y(counter); 00862 if(pixely < imagetop) 00863 { 00864 imagetop = pixely; 00865 } 00866 if(pixely > imagebottom) 00867 { 00868 imagebottom = pixely; 00869 } 00870 } 00871 00872 if(PointCount < 2) 00873 { 00874 return; 00875 } 00876 00877 xcenter = (imageleft + imageright)/2; 00878 ycenter = (imagebottom + imagetop)/2; 00879 00880 xfirst = pPoints->X; 00881 yfirst = pPoints->Y; 00882 00883 while(--PointCount) 00884 { 00885 x = pPoints->X; 00886 y = pPoints->Y; 00887 pPoints++; 00888 x2 = pPoints->X; 00889 y2 = pPoints->Y; 00890 00891 BSP_LCD_FillTriangle(x, x2, xcenter, y, y2, ycenter); 00892 BSP_LCD_FillTriangle(x, xcenter, x2, y, ycenter, y2); 00893 BSP_LCD_FillTriangle(xcenter, x2, x, ycenter, y2, y); 00894 } 00895 00896 BSP_LCD_FillTriangle(xfirst, x2, xcenter, yfirst, y2, ycenter); 00897 BSP_LCD_FillTriangle(xfirst, xcenter, x2, yfirst, ycenter, y2); 00898 BSP_LCD_FillTriangle(xcenter, x2, xfirst, ycenter, y2, yfirst); 00899 } 00900 00901 /** 00902 * @brief Draws a full ellipse. 00903 * @param Xpos: X position 00904 * @param Ypos: Y position 00905 * @param XRadius: Ellipse X radius 00906 * @param YRadius: Ellipse Y radius 00907 * @retval None 00908 */ 00909 void BSP_LCD_FillEllipse(int Xpos, int Ypos, int XRadius, int YRadius) 00910 { 00911 int x = 0, y = -XRadius, err = 2-2*YRadius, e2; 00912 float k = 0, rad1 = 0, rad2 = 0; 00913 00914 rad1 = YRadius; 00915 rad2 = XRadius; 00916 00917 k = (float)(rad2/rad1); 00918 00919 do 00920 { 00921 BSP_LCD_DrawVLine((Xpos+y), (Ypos-(uint16_t)(x/k)), (2*(uint16_t)(x/k) + 1)); 00922 BSP_LCD_DrawVLine((Xpos-y), (Ypos-(uint16_t)(x/k)), (2*(uint16_t)(x/k) + 1)); 00923 00924 e2 = err; 00925 if (e2 <= x) 00926 { 00927 err += ++x*2+1; 00928 if (-y == x && e2 <= y) e2 = 0; 00929 } 00930 if (e2 > y) err += ++y*2+1; 00931 } 00932 while (y <= 0); 00933 } 00934 00935 /** 00936 * @brief Enables the display. 00937 * @retval None 00938 */ 00939 void BSP_LCD_DisplayOn(void) 00940 { 00941 lcd_drv->DisplayOn(); 00942 } 00943 00944 /** 00945 * @brief Disables the display. 00946 * @retval None 00947 */ 00948 void BSP_LCD_DisplayOff(void) 00949 { 00950 lcd_drv->DisplayOff(); 00951 } 00952 00953 /****************************************************************************** 00954 Static Function 00955 *******************************************************************************/ 00956 /** 00957 * @brief Draws a pixel on LCD. 00958 * @param Xpos: X position 00959 * @param Ypos: Y position 00960 * @param RGBCode: Pixel color in RGB mode (5-6-5) 00961 * @retval None 00962 */ 00963 static void LCD_DrawPixel(uint16_t Xpos, uint16_t Ypos, uint16_t RGBCode) 00964 { 00965 if(lcd_drv->WritePixel != NULL) 00966 { 00967 lcd_drv->WritePixel(Xpos, Ypos, RGBCode); 00968 } 00969 } 00970 00971 /** 00972 * @brief Draws a character on LCD. 00973 * @param Xpos: Line where to display the character shape 00974 * @param Ypos: Start column address 00975 * @param pChar: Pointer to the character data 00976 * @retval None 00977 */ 00978 static void LCD_DrawChar(uint16_t Xpos, uint16_t Ypos, const uint8_t *pChar) 00979 { 00980 uint32_t counterh = 0, counterw = 0, index = 0; 00981 uint16_t height = 0, width = 0; 00982 uint8_t offset = 0; 00983 uint8_t *pchar = NULL; 00984 uint32_t line = 0; 00985 00986 height = DrawProp.pFont->Height; 00987 width = DrawProp.pFont->Width; 00988 00989 /* Fill bitmap header*/ 00990 *(uint16_t *) (bitmap + 2) = (uint16_t)(height*width*2+OFFSET_BITMAP); 00991 *(uint16_t *) (bitmap + 4) = (uint16_t)((height*width*2+OFFSET_BITMAP)>>16); 00992 *(uint16_t *) (bitmap + 10) = OFFSET_BITMAP; 00993 *(uint16_t *) (bitmap + 18) = (uint16_t)(width); 00994 *(uint16_t *) (bitmap + 20) = (uint16_t)((width)>>16); 00995 *(uint16_t *) (bitmap + 22) = (uint16_t)(height); 00996 *(uint16_t *) (bitmap + 24) = (uint16_t)((height)>>16); 00997 00998 offset = 8 *((width + 7)/8) - width ; 00999 01000 for(counterh = 0; counterh < height; counterh++) 01001 { 01002 pchar = ((uint8_t *)pChar + (width + 7)/8 * counterh); 01003 01004 if(((width + 7)/8) == 3) 01005 { 01006 line = (pchar[0]<< 16) | (pchar[1]<< 8) | pchar[2]; 01007 } 01008 01009 if(((width + 7)/8) == 2) 01010 { 01011 line = (pchar[0]<< 8) | pchar[1]; 01012 } 01013 01014 if(((width + 7)/8) == 1) 01015 { 01016 line = pchar[0]; 01017 } 01018 01019 for (counterw = 0; counterw < width; counterw++) 01020 { 01021 /* Image in the bitmap is written from the bottom to the top */ 01022 /* Need to invert image in the bitmap */ 01023 index = (((height-counterh-1)*width)+(counterw))*2+OFFSET_BITMAP; 01024 if(line & (1 << (width- counterw + offset- 1))) 01025 { 01026 bitmap[index] = (uint8_t)DrawProp.TextColor; 01027 bitmap[index+1] = (uint8_t)(DrawProp.TextColor >> 8); 01028 } 01029 else 01030 { 01031 bitmap[index] = (uint8_t)DrawProp.BackColor; 01032 bitmap[index+1] = (uint8_t)(DrawProp.BackColor >> 8); 01033 } 01034 } 01035 } 01036 01037 BSP_LCD_DrawBitmap(Ypos, Xpos, bitmap); 01038 } 01039 01040 /** 01041 * @brief Sets display window. 01042 * @param Xpos: LCD X position 01043 * @param Ypos: LCD Y position 01044 * @param Width: LCD window width 01045 * @param Height: LCD window height 01046 * @retval None 01047 */ 01048 static void LCD_SetDisplayWindow(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 01049 { 01050 if(lcd_drv->SetDisplayWindow != NULL) 01051 { 01052 lcd_drv->SetDisplayWindow(Xpos, Ypos, Width, Height); 01053 } 01054 } 01055 01056 /** 01057 * @} 01058 */ 01059 01060 /** 01061 * @} 01062 */ 01063 01064 /** 01065 * @} 01066 */ 01067 01068 /** 01069 * @} 01070 */ 01071 01072 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Sun Jun 21 2015 23:46:41 for STM32L476G_EVAL BSP User Manual by
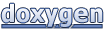