STM32L476G_EVAL BSP User Manual
|
stm32l476g_eval.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l476g_eval.c 00004 * @author MCD Application Team 00005 * @version $VERSION$ 00006 * @date $DATE$ 00007 * @brief This file provides a set of firmware functions to manage Leds, 00008 * push-button and COM ports for STM32L476G_EVAL 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* Includes ------------------------------------------------------------------*/ 00040 #include "stm32l476g_eval.h" 00041 #if defined(USE_IOEXPANDER) 00042 #include "stm32l476g_eval_io.h" 00043 #endif /* USE_IOEXPANDER */ 00044 00045 /** @addtogroup BSP 00046 * @{ 00047 */ 00048 00049 /** @defgroup STM32L476G_EVAL STM32L476G_EVAL 00050 * @{ 00051 */ 00052 00053 /** @defgroup STM32L476G_EVAL_Common STM32L476G_EVAL Common 00054 * @{ 00055 */ 00056 00057 /** @defgroup STM32L476G_EVAL_Private_TypesDefinitions Private Types Definitions 00058 * @brief This file provides firmware functions to manage Leds, push-buttons, 00059 * COM ports, SD card on SPI and temperature sensor (TS751) available on 00060 * STM32L476G-EVAL evaluation board from STMicroelectronics. 00061 * @{ 00062 */ 00063 00064 typedef struct 00065 { 00066 __IO uint16_t LCD_REG_R; /* Read Register */ 00067 __IO uint16_t LCD_RAM_R; /* Read RAM */ 00068 __IO uint16_t LCD_REG_W; /* Write Register */ 00069 __IO uint16_t LCD_RAM_W; /* Write RAM */ 00070 } TFT_LCD_TypeDef; 00071 00072 /** 00073 * @} 00074 */ 00075 00076 /** @defgroup STM32L476G_EVAL_Private_Defines Private Defines 00077 * @{ 00078 */ 00079 /* LINK LCD */ 00080 #define START_BYTE 0x70 00081 #define SET_INDEX 0x00 00082 #define READ_STATUS 0x01 00083 #define LCD_WRITE_REG 0x02 00084 #define LCD_READ_REG 0x03 00085 00086 /** 00087 * @brief STM32L476G EVAL BSP Driver version number $VERSION$ 00088 */ 00089 #define __STM32L476G_EVAL_BSP_VERSION_MAIN (0x01) /*!< [31:24] main version */ 00090 #define __STM32L476G_EVAL_BSP_VERSION_SUB1 (0x00) /*!< [23:16] sub1 version */ 00091 #define __STM32L476G_EVAL_BSP_VERSION_SUB2 (0x00) /*!< [15:8] sub2 version */ 00092 #define __STM32L476G_EVAL_BSP_VERSION_RC (0x00) /*!< [7:0] release candidate */ 00093 #define __STM32L476G_EVAL_BSP_VERSION ((__STM32L476G_EVAL_BSP_VERSION_MAIN << 24)\ 00094 |(__STM32L476G_EVAL_BSP_VERSION_SUB1 << 16)\ 00095 |(__STM32L476G_EVAL_BSP_VERSION_SUB2 << 8 )\ 00096 |(__STM32L476G_EVAL_BSP_VERSION_RC)) 00097 00098 /* Note: LCD /CS is NE3 - Bank 3 of NOR/SRAM Bank 1~4 */ 00099 #define TFT_LCD_BASE ((uint32_t)(0x60000000 | 0x08000000)) 00100 #define TFT_LCD ((TFT_LCD_TypeDef *) TFT_LCD_BASE) 00101 00102 /** 00103 * @} 00104 */ 00105 00106 00107 /** @defgroup STM32L476G_EVAL_Exported_Variables Exported Variables 00108 * @{ 00109 */ 00110 00111 /** 00112 * @brief LED variables 00113 */ 00114 #if defined(USE_STM32L476G_EVAL_REVA) 00115 /* 0xFFFF to mark as invalid port (LED2, LED3 and LED4 accessed thru IOExpander) */ 00116 GPIO_TypeDef* LED_PORT[LEDn] = {LED1_GPIO_PORT, 00117 (GPIO_TypeDef *)0xFFFF, 00118 (GPIO_TypeDef *)0xFFFF, 00119 (GPIO_TypeDef *)0xFFFF}; 00120 #elif defined(USE_STM32L476G_EVAL_REVB) 00121 /* 0xFFFF to mark as invalid port (LED2 and LED4 accessed thru IOExpander) */ 00122 GPIO_TypeDef* LED_PORT[LEDn] = {LED1_GPIO_PORT, 00123 (GPIO_TypeDef *)0xFFFF, 00124 LED3_GPIO_PORT, 00125 (GPIO_TypeDef *)0xFFFF}; 00126 #endif 00127 00128 #if defined(USE_IOEXPANDER) 00129 const uint16_t LED_PIN[LEDn] = {LED1_PIN, 00130 LED2_PIN, 00131 LED3_PIN, 00132 LED4_PIN}; 00133 #else 00134 #if defined(USE_STM32L476G_EVAL_REVA) 00135 const uint16_t LED_PIN[LEDn] = {LED1_PIN, 00136 (uint16_t)0x0, 00137 (uint16_t)0x0, 00138 (uint16_t)0x0}; 00139 #elif defined(USE_STM32L476G_EVAL_REVB) 00140 const uint16_t LED_PIN[LEDn] = {LED1_PIN, 00141 (uint16_t)0x0, 00142 LED3_PIN, 00143 (uint16_t)0x0}; 00144 #endif 00145 #endif /* USE_IOEXPANDER */ 00146 00147 /** 00148 * @brief BUTTON variables 00149 */ 00150 #if defined(USE_IOEXPANDER) 00151 GPIO_TypeDef* BUTTON_PORT[BUTTONn] = {TAMPER_BUTTON_GPIO_PORT, 00152 (GPIO_TypeDef *)0xFFFF, 00153 (GPIO_TypeDef *)0xFFFF, 00154 (GPIO_TypeDef *)0xFFFF, 00155 (GPIO_TypeDef *)0xFFFF, 00156 (GPIO_TypeDef *)0xFFFF}; 00157 /* 0xFFFF to mark as invalid port (Joystick buttons accessed thru IOExpander) */ 00158 #else 00159 GPIO_TypeDef* BUTTON_PORT[BUTTONn] = {TAMPER_BUTTON_GPIO_PORT}; 00160 #endif /* USE_IOEXPANDER */ 00161 00162 #if defined(USE_IOEXPANDER) 00163 const uint16_t BUTTON_PIN[BUTTONn] = {TAMPER_BUTTON_PIN, 00164 SEL_JOY_PIN, 00165 LEFT_JOY_PIN, 00166 RIGHT_JOY_PIN, 00167 DOWN_JOY_PIN, 00168 UP_JOY_PIN}; 00169 00170 const uint8_t BUTTON_IRQn[BUTTONn] = {TAMPER_BUTTON_EXTI_IRQn, 00171 SEL_JOY_EXTI_IRQn, 00172 LEFT_JOY_EXTI_IRQn, 00173 RIGHT_JOY_EXTI_IRQn, 00174 DOWN_JOY_EXTI_IRQn, 00175 UP_JOY_EXTI_IRQn}; 00176 #else 00177 const uint16_t BUTTON_PIN[BUTTONn] = {TAMPER_BUTTON_PIN}; 00178 00179 const uint8_t BUTTON_IRQn[BUTTONn] = {TAMPER_BUTTON_EXTI_IRQn}; 00180 #endif /* USE_IOEXPANDER */ 00181 00182 #if defined(USE_IOEXPANDER) 00183 /** 00184 * @brief JOYSTICK variables 00185 */ 00186 00187 const uint16_t JOY_PIN[JOYn] = {SEL_JOY_PIN, 00188 LEFT_JOY_PIN, 00189 RIGHT_JOY_PIN, 00190 DOWN_JOY_PIN, 00191 UP_JOY_PIN}; 00192 00193 const uint8_t JOY_IRQn[JOYn] = {SEL_JOY_EXTI_IRQn, 00194 LEFT_JOY_EXTI_IRQn, 00195 RIGHT_JOY_EXTI_IRQn, 00196 DOWN_JOY_EXTI_IRQn, 00197 UP_JOY_EXTI_IRQn}; 00198 #endif /* USE_IOEXPANDER */ 00199 00200 /** 00201 * @brief COM variables 00202 */ 00203 USART_TypeDef* COM_USART[COMn] = {EVAL_COM1}; 00204 00205 GPIO_TypeDef* COM_TX_PORT[COMn] = {EVAL_COM1_TX_GPIO_PORT}; 00206 00207 GPIO_TypeDef* COM_RX_PORT[COMn] = {EVAL_COM1_RX_GPIO_PORT}; 00208 00209 const uint16_t COM_TX_PIN[COMn] = {EVAL_COM1_TX_PIN}; 00210 00211 const uint16_t COM_RX_PIN[COMn] = {EVAL_COM1_RX_PIN}; 00212 00213 const uint16_t COM_TX_AF[COMn] = {EVAL_COM1_TX_AF}; 00214 00215 const uint16_t COM_RX_AF[COMn] = {EVAL_COM1_RX_AF}; 00216 00217 /** 00218 * @brief BUS variables 00219 */ 00220 00221 #ifdef HAL_I2C_MODULE_ENABLED 00222 uint32_t heval_I2cxTimeout = EVAL_I2Cx_TIMEOUT_MAX; /*<! Value of Timeout when I2C communication fails */ 00223 I2C_HandleTypeDef heval_I2c; 00224 #endif /* HAL_I2C_MODULE_ENABLED */ 00225 00226 /** 00227 * @} 00228 */ 00229 00230 /** @defgroup STM32L476G_EVAL_Private_FunctionPrototypes Private Functions 00231 * @{ 00232 */ 00233 /* FMC bus function */ 00234 #ifdef HAL_SRAM_MODULE_ENABLED 00235 static void FMC_BANK3_WriteData(uint16_t Data); 00236 static void FMC_BANK3_WriteReg(uint8_t Reg); 00237 static uint16_t FMC_BANK3_ReadData(uint8_t Reg); 00238 static void FMC_BANK3_Init(void); 00239 static void FMC_BANK3_MspInit(void); 00240 00241 /* LCD IO functions */ 00242 void LCD_IO_Init(void); 00243 void LCD_IO_WriteMultipleData(uint8_t *pData, uint32_t Size); 00244 void LCD_IO_WriteReg(uint8_t Reg); 00245 uint16_t LCD_IO_ReadData(uint8_t Reg); 00246 void LCD_Delay (uint32_t delay); 00247 #endif /* HAL_SRAM_MODULE_ENABLED */ 00248 00249 /* I2Cx bus function */ 00250 #ifdef HAL_I2C_MODULE_ENABLED 00251 /* Link function I2C for EEPROM, Audio Codec peripheral and IO expanders*/ 00252 static void I2Cx_Init(void); 00253 static void I2Cx_MspInit(I2C_HandleTypeDef *hi2c); 00254 static void I2Cx_DeInit(void); 00255 static void I2Cx_MspDeInit(I2C_HandleTypeDef *hi2c); 00256 static HAL_StatusTypeDef I2Cx_WriteBuffer(uint16_t Addr, uint16_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length); 00257 static HAL_StatusTypeDef I2Cx_ReadBuffer(uint16_t Addr, uint16_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length); 00258 static HAL_StatusTypeDef I2Cx_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00259 static void I2Cx_Error (void); 00260 00261 #if defined(USE_IOEXPANDER) 00262 /* Link function I2C for IO expanders */ 00263 static void I2Cx_WriteData(uint16_t Addr, uint16_t Reg, uint16_t RegSize, uint8_t Value); 00264 static uint8_t I2Cx_ReadData(uint16_t Addr, uint16_t Reg, uint16_t RegSize); 00265 00266 /* IOExpander IO functions */ 00267 void IOE_Init(void); 00268 void IOE_ITConfig(void); 00269 void IOE_Delay(uint32_t Delay); 00270 void IOE_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00271 uint8_t IOE_Read(uint8_t Addr, uint8_t Reg); 00272 uint16_t IOE_ReadMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length); 00273 #endif /* USE_IOEXPANDER */ 00274 00275 /* Link function for EEPROM peripheral over I2C */ 00276 void EEPROM_I2C_IO_Init(void); 00277 HAL_StatusTypeDef EEPROM_I2C_IO_WriteData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize); 00278 HAL_StatusTypeDef EEPROM_I2C_IO_ReadData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize); 00279 HAL_StatusTypeDef EEPROM_I2C_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00280 00281 /* Link function for Audio Codec peripheral */ 00282 void AUDIO_IO_Init(void); 00283 void AUDIO_IO_DeInit(void); 00284 void AUDIO_IO_Write(uint8_t Addr, uint16_t Reg, uint16_t Value); 00285 uint16_t AUDIO_IO_Read(uint8_t Addr, uint16_t Reg); 00286 void AUDIO_IO_Delay(uint32_t delay); 00287 #endif/* HAL_I2C_MODULE_ENABLED */ 00288 00289 /** 00290 * @} 00291 */ 00292 00293 /** @defgroup STM32L476G_EVAL_Exported_Functions Exported Functions 00294 * @{ 00295 */ 00296 00297 /** 00298 * @brief This method returns the STM32L476G EVAL BSP Driver revision 00299 * @retval version : 0xXYZR (8bits for each decimal, R for RC) 00300 */ 00301 uint32_t BSP_GetVersion(void) 00302 { 00303 return __STM32L476G_EVAL_BSP_VERSION; 00304 } 00305 00306 /** 00307 * @brief Configures LED GPIO. 00308 * @param Led: Specifies the Led to be configured. 00309 * This parameter can be one of following parameters: 00310 * @arg LED1 00311 * @arg LED2 00312 * @arg LED3 00313 * @arg LED4 00314 * @retval None 00315 */ 00316 void BSP_LED_Init(Led_TypeDef Led) 00317 { 00318 GPIO_InitTypeDef gpioinitstruct = {0}; 00319 00320 #if defined(USE_STM32L476G_EVAL_REVA) 00321 if (Led == LED1) 00322 #elif defined(USE_STM32L476G_EVAL_REVB) 00323 if ((Led == LED1) || (Led == LED3)) 00324 #endif 00325 { 00326 /* Enable the GPIO_LED clock */ 00327 LEDx_GPIO_CLK_ENABLE(Led); 00328 00329 /* Configure the GPIO_LED pin */ 00330 gpioinitstruct.Pin = LED_PIN[Led]; 00331 gpioinitstruct.Mode = GPIO_MODE_OUTPUT_PP; 00332 gpioinitstruct.Pull = GPIO_PULLUP; 00333 gpioinitstruct.Speed = GPIO_SPEED_HIGH; 00334 00335 HAL_GPIO_Init(LED_PORT[Led], &gpioinitstruct); 00336 00337 /* By default, turn off LED */ 00338 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_SET); 00339 } 00340 #if defined(USE_IOEXPANDER) 00341 else 00342 { 00343 /* Initialize the IO functionalities */ 00344 BSP_IO_Init(); 00345 00346 BSP_IO_ConfigPin(LED_PIN[Led],IO_MODE_OUTPUT); 00347 00348 /* By default, turn off LED */ 00349 BSP_IO_WritePin(LED_PIN[Led], GPIO_PIN_SET); 00350 } 00351 #endif /* USE_IOEXPANDER */ 00352 } 00353 00354 /** 00355 * @brief Turns selected LED On. 00356 * @param Led: Specifies the Led to be set on. 00357 * This parameter can be one of following parameters: 00358 * @arg LED1 00359 * @arg LED2 00360 * @arg LED3 00361 * @arg LED4 00362 * @retval None 00363 */ 00364 void BSP_LED_On(Led_TypeDef Led) 00365 { 00366 #if defined(USE_STM32L476G_EVAL_REVA) 00367 if (Led == LED1) 00368 #elif defined(USE_STM32L476G_EVAL_REVB) 00369 if ((Led == LED1) || (Led == LED3)) 00370 #endif 00371 { 00372 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_RESET); 00373 } 00374 #if defined(USE_IOEXPANDER) 00375 else 00376 { 00377 BSP_IO_WritePin(LED_PIN[Led], GPIO_PIN_RESET); 00378 } 00379 #endif /* USE_IOEXPANDER */ 00380 } 00381 00382 /** 00383 * @brief Turns selected LED Off. 00384 * @param Led: Specifies the Led to be set off. 00385 * This parameter can be one of following parameters: 00386 * @arg LED1 00387 * @arg LED2 00388 * @arg LED3 00389 * @arg LED4 00390 * @retval None 00391 */ 00392 void BSP_LED_Off(Led_TypeDef Led) 00393 { 00394 #if defined(USE_STM32L476G_EVAL_REVA) 00395 if (Led == LED1) 00396 #elif defined(USE_STM32L476G_EVAL_REVB) 00397 if ((Led == LED1) || (Led == LED3)) 00398 #endif 00399 { 00400 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_SET); 00401 } 00402 #if defined(USE_IOEXPANDER) 00403 else 00404 { 00405 BSP_IO_WritePin(LED_PIN[Led], GPIO_PIN_SET); 00406 } 00407 #endif /* USE_IOEXPANDER */ 00408 } 00409 00410 /** 00411 * @brief Toggles the selected LED. 00412 * @param Led: Specifies the Led to be toggled. 00413 * This parameter can be one of following parameters: 00414 * @arg LED1 00415 * @arg LED2 00416 * @arg LED3 00417 * @arg LED4 00418 * @retval None 00419 */ 00420 void BSP_LED_Toggle(Led_TypeDef Led) 00421 { 00422 #if defined(USE_STM32L476G_EVAL_REVA) 00423 if (Led == LED1) 00424 #elif defined(USE_STM32L476G_EVAL_REVB) 00425 if ((Led == LED1) || (Led == LED3)) 00426 #endif 00427 { 00428 HAL_GPIO_TogglePin(LED_PORT[Led], LED_PIN[Led]); 00429 } 00430 #if defined(USE_IOEXPANDER) 00431 else 00432 { 00433 BSP_IO_TogglePin(LED_PIN[Led]); 00434 } 00435 #endif /* USE_IOEXPANDER */ 00436 } 00437 00438 /** 00439 * @brief Configures push button GPIO and EXTI Line. 00440 * @param Button: Button to be configured. 00441 * This parameter can be one of the following values: 00442 * @arg BUTTON_TAMPER: Wakeup/Tamper Push Button 00443 * @param Button_Mode: Button mode requested. 00444 * This parameter can be one of the following values: 00445 * @arg BUTTON_MODE_GPIO: Button will be used as simple IO 00446 * @arg BUTTON_MODE_EXTI: Button will be connected to EXTI line 00447 * with interrupt generation capability 00448 * @retval None 00449 */ 00450 void BSP_PB_Init(Button_TypeDef Button, ButtonMode_TypeDef Button_Mode) 00451 { 00452 GPIO_InitTypeDef gpioinitstruct = {0}; 00453 00454 if (Button == BUTTON_TAMPER) 00455 { 00456 /* Enable the corresponding Push Button clock */ 00457 BUTTONx_GPIO_CLK_ENABLE(Button); 00458 00459 /* Configure Push Button pin as input */ 00460 gpioinitstruct.Pin = BUTTON_PIN[Button]; 00461 gpioinitstruct.Pull = GPIO_PULLDOWN; 00462 gpioinitstruct.Speed = GPIO_SPEED_HIGH; 00463 00464 if (Button_Mode == BUTTON_MODE_GPIO) 00465 { 00466 /* Configure Button pin as input */ 00467 gpioinitstruct.Mode = GPIO_MODE_INPUT; 00468 HAL_GPIO_Init(BUTTON_PORT[Button], &gpioinitstruct); 00469 } 00470 else if (Button_Mode == BUTTON_MODE_EXTI) 00471 { 00472 /* Configure Tamper/Wake-up Push Button pin as input with External interrupt, falling edge */ 00473 gpioinitstruct.Mode = GPIO_MODE_IT_FALLING; 00474 00475 HAL_GPIO_Init(BUTTON_PORT[Button], &gpioinitstruct); 00476 00477 /* Enable and set Button EXTI Interrupt to the lowest priority */ 00478 HAL_NVIC_SetPriority((IRQn_Type)(BUTTON_IRQn[Button]), 0x0F, 0); 00479 HAL_NVIC_EnableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00480 } 00481 } 00482 } 00483 00484 /** 00485 * @brief Returns the selected button state. 00486 * @param Button: Button to be checked. 00487 * This parameter can be one of the following values: 00488 * @arg BUTTON_TAMPER: Wakeup/Tamper Push Button 00489 * @retval Button state 00490 */ 00491 uint32_t BSP_PB_GetState(Button_TypeDef Button) 00492 { 00493 return HAL_GPIO_ReadPin(BUTTON_PORT[Button], BUTTON_PIN[Button]); 00494 } 00495 00496 #if defined(USE_IOEXPANDER) 00497 /** 00498 * @brief Configures all buttons of the joystick in GPIO or EXTI modes. 00499 * @param Joy_Mode: Joystick mode. 00500 * This parameter can be one of the following values: 00501 * @arg JOY_MODE_GPIO: Joystick pins will be used as simple IOs 00502 * @arg JOY_MODE_EXTI: Joystick pins will be connected to EXTI line 00503 * with interrupt generation capability 00504 * @retval HAL_OK: if all initializations are OK. Other value if error. 00505 */ 00506 uint8_t BSP_JOY_Init(JOYMode_TypeDef Joy_Mode) 00507 { 00508 BSP_IO_Init(); 00509 00510 /* Configure joystick pins in IT mode */ 00511 if(Joy_Mode == JOY_MODE_EXTI) 00512 { 00513 BSP_IO_ConfigPin(JOY_ALL_PINS, IO_MODE_IT_FALLING_EDGE); 00514 00515 /* Configure IO Expander interrupt */ 00516 IOE_ITConfig(); 00517 } 00518 else 00519 { 00520 /* GPIO mode configuration */ 00521 BSP_IO_ConfigPin(JOY_ALL_PINS,IO_MODE_INPUT); 00522 } 00523 00524 return HAL_OK; 00525 } 00526 00527 /** 00528 * @brief Returns the current joystick status. 00529 * @retval Code of the joystick key pressed 00530 * This code can be one of the following values: 00531 * @arg JOY_NONE 00532 * @arg JOY_SEL 00533 * @arg JOY_DOWN 00534 * @arg JOY_LEFT 00535 * @arg JOY_RIGHT 00536 * @arg JOY_UP 00537 */ 00538 JOYState_TypeDef BSP_JOY_GetState(void) 00539 { 00540 uint32_t tmp = 0; 00541 00542 /* Read the status of all the joystick pins */ 00543 tmp = (uint32_t)BSP_IO_ReadPin(JOY_ALL_PINS); 00544 00545 /* Check the pressed joystick button */ 00546 if(!(tmp & SEL_JOY_PIN)) 00547 { 00548 return(JOYState_TypeDef) JOY_SEL; 00549 } 00550 else if(!(tmp & DOWN_JOY_PIN)) 00551 { 00552 return(JOYState_TypeDef) JOY_DOWN; 00553 } 00554 else if(!(tmp & LEFT_JOY_PIN)) 00555 { 00556 return(JOYState_TypeDef) JOY_LEFT; 00557 } 00558 else if(!(tmp & RIGHT_JOY_PIN)) 00559 { 00560 return(JOYState_TypeDef) JOY_RIGHT; 00561 } 00562 else if(!(tmp & UP_JOY_PIN)) 00563 { 00564 return(JOYState_TypeDef) JOY_UP; 00565 } 00566 else 00567 { 00568 return(JOYState_TypeDef) JOY_NONE; 00569 } 00570 } 00571 #endif /* USE_IOEXPANDER */ 00572 00573 #ifdef HAL_UART_MODULE_ENABLED 00574 /** 00575 * @brief Configures COM port. 00576 * @param COM: Specifies the COM port to be configured. 00577 * This parameter can be one of following parameters: 00578 * @arg COM1 00579 * @param huart: pointer to a UART_HandleTypeDef structure that 00580 * contains the configuration information for the specified UART peripheral. 00581 * @retval None 00582 */ 00583 void BSP_COM_Init(COM_TypeDef COM, UART_HandleTypeDef* huart) 00584 { 00585 GPIO_InitTypeDef gpioinitstruct = {0}; 00586 00587 /* Enable GPIO clock */ 00588 COMx_TX_GPIO_CLK_ENABLE(COM); 00589 COMx_RX_GPIO_CLK_ENABLE(COM); 00590 00591 /* Enable USART clock */ 00592 COMx_CLK_ENABLE(COM); 00593 00594 /* Configure USART Tx as alternate function push-pull */ 00595 gpioinitstruct.Pin = COM_TX_PIN[COM]; 00596 gpioinitstruct.Mode = GPIO_MODE_AF_PP; 00597 gpioinitstruct.Speed = GPIO_SPEED_HIGH; 00598 gpioinitstruct.Pull = GPIO_PULLUP; 00599 gpioinitstruct.Alternate = COM_TX_AF[COM]; 00600 HAL_GPIO_Init(COM_TX_PORT[COM], &gpioinitstruct); 00601 00602 /* Configure USART Rx as alternate function push-pull */ 00603 gpioinitstruct.Pin = COM_RX_PIN[COM]; 00604 gpioinitstruct.Alternate = COM_RX_AF[COM]; 00605 HAL_GPIO_Init(COM_RX_PORT[COM], &gpioinitstruct); 00606 00607 /* USART configuration */ 00608 huart->Instance = COM_USART[COM]; 00609 HAL_UART_Init(huart); 00610 } 00611 #endif /* HAL_UART_MODULE_ENABLED */ 00612 00613 /** 00614 * @} 00615 */ 00616 00617 /** @defgroup STM32L476G_EVAL_BusOperations_Functions Bus Operations Functions 00618 * @{ 00619 */ 00620 00621 /******************************************************************************* 00622 BUS OPERATIONS 00623 *******************************************************************************/ 00624 #ifdef HAL_SRAM_MODULE_ENABLED 00625 /********************************* LINK LCD ***********************************/ 00626 00627 /** 00628 * @brief Initializes LCD low level. 00629 * @retval None 00630 */ 00631 void LCD_IO_Init(void) 00632 { 00633 BSP_IO_Init(); 00634 00635 BSP_IO_ConfigPin(IO2_PIN_9, IO_MODE_OUTPUT); 00636 00637 /* By default, turn on Backlight */ 00638 BSP_IO_WritePin(IO2_PIN_9, GPIO_PIN_RESET); 00639 00640 FMC_BANK3_Init(); 00641 } 00642 00643 /** 00644 * @brief Writes data on LCD data register. 00645 * @param pData: Data to be written 00646 * @param Size: number of data to write 00647 * @retval None 00648 */ 00649 void LCD_IO_WriteMultipleData(uint8_t *pData, uint32_t Size) 00650 { 00651 uint32_t counter = 0; 00652 uint16_t regvalue; 00653 00654 regvalue = *pData | (*(pData+1) << 8); 00655 00656 for (counter = Size; counter != 0; counter--) 00657 { 00658 /* Write 16-bit Reg */ 00659 FMC_BANK3_WriteData(regvalue); 00660 counter--; 00661 pData += 2; 00662 regvalue = *pData | (*(pData+1) << 8); 00663 } 00664 } 00665 00666 /** 00667 * @brief Writes register on LCD register. 00668 * @param Reg: Register to be written 00669 * @retval None 00670 */ 00671 void LCD_IO_WriteReg(uint8_t Reg) 00672 { 00673 /* Write 16-bit Index, then Write Reg */ 00674 FMC_BANK3_WriteReg(Reg); 00675 } 00676 00677 /** 00678 * @brief Reads data from LCD data register. 00679 * @retval Read data. 00680 */ 00681 uint16_t LCD_IO_ReadData(uint8_t Reg) 00682 { 00683 return FMC_BANK3_ReadData(Reg); 00684 } 00685 00686 /** 00687 * @brief Wait for loop in ms. 00688 * @param Delay in ms. 00689 * @retval None 00690 */ 00691 void LCD_Delay (uint32_t Delay) 00692 { 00693 HAL_Delay(Delay); 00694 } 00695 00696 /*************************** FMC Routines ************************************/ 00697 /** 00698 * @brief Initializes FMC_BANK3 MSP. 00699 * @retval None 00700 */ 00701 static void FMC_BANK3_MspInit(void) 00702 { 00703 GPIO_InitTypeDef gpioinitstruct = {0}; 00704 00705 /* Enable FMC clock */ 00706 __HAL_RCC_FMC_CLK_ENABLE(); 00707 00708 /* Enable GPIOs clock */ 00709 __HAL_RCC_GPIOD_CLK_ENABLE(); 00710 __HAL_RCC_GPIOE_CLK_ENABLE(); 00711 __HAL_RCC_GPIOF_CLK_ENABLE(); 00712 __HAL_RCC_GPIOG_CLK_ENABLE(); 00713 __HAL_RCC_PWR_CLK_ENABLE(); 00714 HAL_PWREx_EnableVddIO2(); 00715 00716 /* Common GPIO configuration */ 00717 gpioinitstruct.Mode = GPIO_MODE_AF_PP; 00718 gpioinitstruct.Pull = GPIO_PULLDOWN; 00719 gpioinitstruct.Speed = GPIO_SPEED_HIGH; 00720 gpioinitstruct.Alternate = GPIO_AF12_FMC; 00721 00722 /*## Data Bus #######*/ 00723 /* GPIOD configuration */ 00724 gpioinitstruct.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_8 | GPIO_PIN_9 | 00725 GPIO_PIN_10 | GPIO_PIN_14 | GPIO_PIN_15; 00726 HAL_GPIO_Init(GPIOD, &gpioinitstruct); 00727 00728 /* GPIOE configuration */ 00729 gpioinitstruct.Pin = GPIO_PIN_7 | GPIO_PIN_8 | GPIO_PIN_9 | GPIO_PIN_10 | 00730 GPIO_PIN_11 | GPIO_PIN_12 | GPIO_PIN_13 | 00731 GPIO_PIN_14 | GPIO_PIN_15; 00732 HAL_GPIO_Init(GPIOE, &gpioinitstruct); 00733 00734 /*## Address Bus #######*/ 00735 /* GPIOF configuration */ 00736 gpioinitstruct.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2 | GPIO_PIN_3 | 00737 GPIO_PIN_4 | GPIO_PIN_5 | GPIO_PIN_12 | GPIO_PIN_13 | 00738 GPIO_PIN_14 | GPIO_PIN_15; 00739 HAL_GPIO_Init(GPIOF, &gpioinitstruct); 00740 00741 /* GPIOG configuration */ 00742 gpioinitstruct.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2 | 00743 GPIO_PIN_3 | GPIO_PIN_4 | GPIO_PIN_5; 00744 HAL_GPIO_Init(GPIOG, &gpioinitstruct); 00745 00746 /* GPIOD configuration */ 00747 gpioinitstruct.Pin = GPIO_PIN_11 | GPIO_PIN_12 | GPIO_PIN_13; 00748 HAL_GPIO_Init(GPIOD, &gpioinitstruct); 00749 00750 /* GPIOE configuration */ 00751 gpioinitstruct.Pin = GPIO_PIN_3 | GPIO_PIN_4 | GPIO_PIN_5 | 00752 GPIO_PIN_6 | GPIO_PIN_2; 00753 HAL_GPIO_Init(GPIOE, &gpioinitstruct); 00754 00755 /*## NOE and NWE configuration #######*/ 00756 gpioinitstruct.Pin = GPIO_PIN_4 |GPIO_PIN_5; 00757 HAL_GPIO_Init(GPIOD, &gpioinitstruct); 00758 00759 /*## NE3 configuration #######*/ 00760 gpioinitstruct.Pin = GPIO_PIN_10; 00761 HAL_GPIO_Init(GPIOG, &gpioinitstruct); 00762 00763 /*## LCD_DO, NBL1 configuration #######*/ 00764 gpioinitstruct.Pin = GPIO_PIN_6; 00765 HAL_GPIO_Init(GPIOA, &gpioinitstruct); 00766 } 00767 00768 /** 00769 * @brief Initializes LCD IO. 00770 * @retval None 00771 */ 00772 static void FMC_BANK3_Init(void) 00773 { 00774 SRAM_HandleTypeDef hsram; 00775 FSMC_NORSRAM_TimingTypeDef sramtiming = {0}; 00776 00777 /*** Configure the SRAM Bank 3 ***/ 00778 /* Configure IPs */ 00779 hsram.Instance = FMC_NORSRAM_DEVICE; 00780 hsram.Extended = FMC_NORSRAM_EXTENDED_DEVICE; 00781 00782 sramtiming.AddressSetupTime = 4; 00783 sramtiming.AddressHoldTime = 3; 00784 sramtiming.DataSetupTime = 7; 00785 sramtiming.BusTurnAroundDuration = 1; 00786 sramtiming.CLKDivision = 2; 00787 sramtiming.DataLatency = 2; 00788 sramtiming.AccessMode = FMC_ACCESS_MODE_A; 00789 00790 hsram.Init.NSBank = FMC_NORSRAM_BANK3; 00791 hsram.Init.DataAddressMux = FMC_DATA_ADDRESS_MUX_ENABLE; 00792 hsram.Init.MemoryType = FMC_MEMORY_TYPE_SRAM; 00793 hsram.Init.MemoryDataWidth = FMC_NORSRAM_MEM_BUS_WIDTH_16; 00794 hsram.Init.BurstAccessMode = FMC_BURST_ACCESS_MODE_DISABLE; 00795 hsram.Init.WaitSignalPolarity = FMC_WAIT_SIGNAL_POLARITY_LOW; 00796 hsram.Init.WaitSignalActive = FMC_WAIT_TIMING_BEFORE_WS; 00797 hsram.Init.WriteOperation = FMC_WRITE_OPERATION_ENABLE; 00798 hsram.Init.WaitSignal = FMC_WAIT_SIGNAL_DISABLE; 00799 hsram.Init.ExtendedMode = FMC_EXTENDED_MODE_DISABLE; 00800 hsram.Init.AsynchronousWait = FMC_ASYNCHRONOUS_WAIT_DISABLE; 00801 hsram.Init.WriteBurst = FMC_WRITE_BURST_DISABLE; 00802 hsram.Init.ContinuousClock = FMC_CONTINUOUS_CLOCK_SYNC_ASYNC; 00803 hsram.Init.WriteFifo = FMC_WRITE_FIFO_DISABLE; 00804 hsram.Init.PageSize = FMC_PAGE_SIZE_NONE; 00805 00806 /* Initialize the SRAM controller */ 00807 FMC_BANK3_MspInit(); 00808 HAL_SRAM_Init(&hsram, &sramtiming, &sramtiming); 00809 } 00810 00811 /** 00812 * @brief Writes register value. 00813 * @param Data: 00814 * @retval None 00815 */ 00816 static void FMC_BANK3_WriteData(uint16_t Data) 00817 { 00818 /* Write 16-bit Data */ 00819 TFT_LCD->LCD_RAM_W = Data; 00820 } 00821 00822 /** 00823 * @brief Writes register address. 00824 * @param Reg: 00825 * @retval None 00826 */ 00827 static void FMC_BANK3_WriteReg(uint8_t Reg) 00828 { 00829 /* Write 16-bit Index, then Write Reg */ 00830 TFT_LCD->LCD_REG_W = Reg; 00831 } 00832 00833 /** 00834 * @brief Reads register value. 00835 * @retval Read value 00836 */ 00837 static uint16_t FMC_BANK3_ReadData(uint8_t Reg) 00838 { 00839 /* Read 16-bit Reg */ 00840 return (TFT_LCD->LCD_RAM_R); 00841 } 00842 #endif /* HAL_SRAM_MODULE_ENABLED */ 00843 00844 #ifdef HAL_I2C_MODULE_ENABLED 00845 /******************************* I2C Routines**********************************/ 00846 00847 /** 00848 * @brief Eval I2Cx MSP Initialization 00849 * @param hi2c: I2C handle 00850 * @retval None 00851 */ 00852 static void I2Cx_MspInit(I2C_HandleTypeDef *hi2c) 00853 { 00854 GPIO_InitTypeDef gpioinitstruct = {0}; 00855 RCC_PeriphCLKInitTypeDef RCC_PeriphCLKInitStruct; 00856 00857 /* IOSV bit MUST be set to access GPIO port G[2:15] */ 00858 __HAL_RCC_PWR_CLK_ENABLE(); 00859 SET_BIT(PWR->CR2, PWR_CR2_IOSV); 00860 00861 if (hi2c->Instance == EVAL_I2Cx) 00862 { 00863 /*##-1- Configure the Eval I2C clock source. The clock is derived from the SYSCLK #*/ 00864 RCC_PeriphCLKInitStruct.PeriphClockSelection = RCC_PERIPHCLK_I2C1; 00865 RCC_PeriphCLKInitStruct.I2c1ClockSelection = RCC_I2C1CLKSOURCE_SYSCLK; 00866 HAL_RCCEx_PeriphCLKConfig(&RCC_PeriphCLKInitStruct); 00867 00868 /*##-2- Configure the GPIOs ################################################*/ 00869 00870 /* Enable GPIO clock */ 00871 EVAL_I2Cx_SDA_GPIO_CLK_ENABLE(); 00872 EVAL_I2Cx_SCL_GPIO_CLK_ENABLE(); 00873 00874 /* Configure I2C Rx/Tx as alternate function */ 00875 gpioinitstruct.Pin = EVAL_I2Cx_SCL_PIN | EVAL_I2Cx_SDA_PIN; 00876 gpioinitstruct.Mode = GPIO_MODE_AF_OD; 00877 gpioinitstruct.Pull = GPIO_PULLUP; 00878 gpioinitstruct.Speed = GPIO_SPEED_HIGH; 00879 gpioinitstruct.Alternate = EVAL_I2Cx_SCL_SDA_AF; 00880 HAL_GPIO_Init(EVAL_I2Cx_SCL_GPIO_PORT, &gpioinitstruct); 00881 00882 /*##-3- Configure the Eval I2Cx peripheral #######################################*/ 00883 /* Enable Eval_I2Cx clock */ 00884 EVAL_I2Cx_CLK_ENABLE(); 00885 00886 /* Force the I2C Peripheral Clock Reset */ 00887 EVAL_I2Cx_FORCE_RESET(); 00888 00889 /* Release the I2C Peripheral Clock Reset */ 00890 EVAL_I2Cx_RELEASE_RESET(); 00891 00892 /* Enable and set Eval I2Cx Interrupt to the highest priority */ 00893 HAL_NVIC_SetPriority(EVAL_I2Cx_EV_IRQn, 0x00, 0); 00894 HAL_NVIC_EnableIRQ(EVAL_I2Cx_EV_IRQn); 00895 00896 /* Enable and set Eval I2Cx Interrupt to the highest priority */ 00897 HAL_NVIC_SetPriority(EVAL_I2Cx_ER_IRQn, 0x00, 0); 00898 HAL_NVIC_EnableIRQ(EVAL_I2Cx_ER_IRQn); 00899 } 00900 } 00901 00902 /** 00903 * @brief Eval I2Cx Bus initialization 00904 * @retval None 00905 */ 00906 static void I2Cx_Init(void) 00907 { 00908 if(HAL_I2C_GetState(&heval_I2c) == HAL_I2C_STATE_RESET) 00909 { 00910 heval_I2c.Instance = EVAL_I2Cx; 00911 heval_I2c.Init.Timing = EVAL_I2Cx_TIMING; 00912 heval_I2c.Init.OwnAddress1 = 0; 00913 heval_I2c.Init.AddressingMode = I2C_ADDRESSINGMODE_7BIT; 00914 heval_I2c.Init.DualAddressMode = I2C_DUALADDRESS_DISABLE; 00915 heval_I2c.Init.OwnAddress2 = 0; 00916 heval_I2c.Init.GeneralCallMode = I2C_GENERALCALL_DISABLE; 00917 heval_I2c.Init.NoStretchMode = I2C_NOSTRETCH_DISABLE; 00918 00919 HAL_I2C_DeInit(&heval_I2c); 00920 /* Init the I2C */ 00921 I2Cx_MspInit(&heval_I2c); 00922 HAL_I2C_Init(&heval_I2c); 00923 } 00924 } 00925 00926 /** 00927 * @brief Eval I2Cx MSP Deinitialization 00928 * @param hi2c: I2C handle 00929 * @retval None 00930 */ 00931 static void I2Cx_MspDeInit(I2C_HandleTypeDef *hi2c) 00932 { 00933 } 00934 00935 /** 00936 * @brief Eval I2Cx Bus deinitialization 00937 * @retval None 00938 */ 00939 static void I2Cx_DeInit(void) 00940 { 00941 if(HAL_I2C_GetState(&heval_I2c) != HAL_I2C_STATE_RESET) 00942 { 00943 /* Deinit the I2C */ 00944 HAL_I2C_DeInit(&heval_I2c); 00945 I2Cx_MspDeInit(&heval_I2c); 00946 } 00947 } 00948 00949 /** 00950 * @brief Write a value in a register of the device through BUS. 00951 * @param Addr: Device address on BUS Bus. 00952 * @param Reg: The target register address to write 00953 * @param RegSize: The target register size (can be 8BIT or 16BIT) 00954 * @param pBuffer: The target register value to be written 00955 * @param Length: buffer size to be written 00956 * @retval None 00957 */ 00958 static HAL_StatusTypeDef I2Cx_WriteBuffer(uint16_t Addr, uint16_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length) 00959 { 00960 HAL_StatusTypeDef status = HAL_OK; 00961 00962 status = HAL_I2C_Mem_Write(&heval_I2c, Addr, (uint16_t)Reg, RegSize, pBuffer, Length, heval_I2cxTimeout); 00963 00964 /* Check the communication status */ 00965 if(status != HAL_OK) 00966 { 00967 /* Re-Initiaize the BUS */ 00968 I2Cx_Error(); 00969 } 00970 return status; 00971 } 00972 00973 #if defined(USE_IOEXPANDER) 00974 /** 00975 * @brief Write a value in a register of the device through BUS. 00976 * @param Addr: Device address on BUS Bus. 00977 * @param Reg: The target register address to write 00978 * @param RegSize: The target register size (can be 8BIT or 16BIT) 00979 * @param Value: The target register value to be written 00980 * @retval None 00981 */ 00982 static void I2Cx_WriteData(uint16_t Addr, uint16_t Reg, uint16_t RegSize, uint8_t Value) 00983 { 00984 HAL_StatusTypeDef status = HAL_OK; 00985 00986 status = HAL_I2C_Mem_Write(&heval_I2c, Addr, (uint16_t)Reg, RegSize, &Value, 1, heval_I2cxTimeout); 00987 00988 /* Check the communication status */ 00989 if(status != HAL_OK) 00990 { 00991 /* Re-Initiaize the BUS */ 00992 I2Cx_Error(); 00993 } 00994 } 00995 00996 /** 00997 * @brief Read a register of the device through BUS 00998 * @param Addr: Device address on BUS 00999 * @param Reg: The target register address to read 01000 * @param RegSize: The target register size (can be 8BIT or 16BIT) 01001 * @retval read register value 01002 */ 01003 static uint8_t I2Cx_ReadData(uint16_t Addr, uint16_t Reg, uint16_t RegSize) 01004 { 01005 HAL_StatusTypeDef status = HAL_OK; 01006 uint8_t value = 0x0; 01007 01008 status = HAL_I2C_Mem_Read(&heval_I2c, Addr, Reg, RegSize, &value, 1, heval_I2cxTimeout); 01009 01010 /* Check the communication status */ 01011 if(status != HAL_OK) 01012 { 01013 /* Execute user timeout callback */ 01014 I2Cx_Error(); 01015 01016 } 01017 return value; 01018 } 01019 #endif /* USE_IOEXPANDER */ 01020 01021 /** 01022 * @brief Reads multiple data on the BUS. 01023 * @param Addr: I2C Address 01024 * @param Reg: Reg Address 01025 * @param RegSize : The target register size (can be 8BIT or 16BIT) 01026 * @param pBuffer: pointer to read data buffer 01027 * @param Length: length of the data 01028 * @retval 0 if no problems to read multiple data 01029 */ 01030 static HAL_StatusTypeDef I2Cx_ReadBuffer(uint16_t Addr, uint16_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length) 01031 { 01032 HAL_StatusTypeDef status = HAL_OK; 01033 01034 status = HAL_I2C_Mem_Read(&heval_I2c, Addr, (uint16_t)Reg, RegSize, pBuffer, Length, heval_I2cxTimeout); 01035 01036 /* Check the communication status */ 01037 if(status != HAL_OK) 01038 { 01039 /* Re-Initiaize the BUS */ 01040 I2Cx_Error(); 01041 } 01042 return status; 01043 } 01044 01045 /** 01046 * @brief Checks if target device is ready for communication. 01047 * @note This function is used with Memory devices 01048 * @param DevAddress: Target device address 01049 * @param Trials: Number of trials 01050 * @retval HAL status 01051 */ 01052 static HAL_StatusTypeDef I2Cx_IsDeviceReady(uint16_t DevAddress, uint32_t Trials) 01053 { 01054 return (HAL_I2C_IsDeviceReady(&heval_I2c, DevAddress, Trials, heval_I2cxTimeout)); 01055 } 01056 01057 01058 /** 01059 * @brief Eval I2Cx error treatment function 01060 * @retval None 01061 */ 01062 static void I2Cx_Error (void) 01063 { 01064 /* De-initialize the I2C communication BUS */ 01065 HAL_I2C_DeInit(&heval_I2c); 01066 01067 /* Re- Initiaize the I2C communication BUS */ 01068 I2Cx_Init(); 01069 } 01070 01071 /******************************************************************************* 01072 LINK OPERATIONS 01073 *******************************************************************************/ 01074 #if defined(USE_IOEXPANDER) 01075 /***************************** LINK IOE ***************************************/ 01076 01077 /** 01078 * @brief Initializes IOE low level. 01079 * @retval None 01080 */ 01081 void IOE_Init(void) 01082 { 01083 I2Cx_Init(); 01084 } 01085 01086 /** 01087 * @brief Configures IOE low level Interrupt. 01088 * @retval None 01089 */ 01090 void IOE_ITConfig(void) 01091 { 01092 static uint8_t IOE_IT_Enabled = 0; 01093 GPIO_InitTypeDef gpioinitstruct = {0}; 01094 01095 if(IOE_IT_Enabled == 0) 01096 { 01097 IOE_IT_Enabled = 1; 01098 01099 /* Configure IOExpander Interrupt GPIO */ 01100 gpioinitstruct.Pin = GPIO_PIN_15; 01101 gpioinitstruct.Pull = GPIO_NOPULL; 01102 gpioinitstruct.Speed = GPIO_SPEED_FAST; 01103 gpioinitstruct.Mode = GPIO_MODE_IT_FALLING; 01104 HAL_GPIO_Init(GPIOG, &gpioinitstruct); 01105 01106 /* All joystick buttons share the same IRQ Handler */ 01107 HAL_NVIC_SetPriority(RIGHT_JOY_EXTI_IRQn, 0x0F, 0); 01108 HAL_NVIC_EnableIRQ(RIGHT_JOY_EXTI_IRQn); 01109 } 01110 } 01111 01112 /** 01113 * @brief IOE writes single data. 01114 * @param Addr: I2C address 01115 * @param Reg: Reg address 01116 * @param Value: Data to be written 01117 * @retval None 01118 */ 01119 void IOE_Write(uint8_t Addr, uint8_t Reg, uint8_t Value) 01120 { 01121 I2Cx_WriteData(Addr, Reg, I2C_MEMADD_SIZE_8BIT, Value); 01122 } 01123 01124 /** 01125 * @brief IOE reads single data. 01126 * @param Addr: I2C address 01127 * @param Reg: Reg address 01128 * @retval Read data 01129 */ 01130 uint8_t IOE_Read(uint8_t Addr, uint8_t Reg) 01131 { 01132 return I2Cx_ReadData(Addr, Reg, I2C_MEMADD_SIZE_8BIT); 01133 } 01134 01135 /** 01136 * @brief IOE reads multiple data. 01137 * @param Addr: I2C address 01138 * @param Reg: Reg address 01139 * @param Buffer: Pointer to data buffer 01140 * @param Length: Length of the data 01141 */ 01142 uint16_t IOE_ReadMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) 01143 { 01144 return I2Cx_ReadBuffer(Addr, Reg, I2C_MEMADD_SIZE_8BIT, Buffer, Length); 01145 } 01146 01147 01148 /** 01149 * @brief IOE Write multiple data. 01150 * @param Addr: I2C address 01151 * @param Reg: Reg address 01152 * @param Buffer: Pointer to data buffer 01153 * @param Length: Length of the data 01154 */ 01155 void IOE_WriteMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) 01156 { 01157 I2Cx_WriteBuffer(Addr, Reg, I2C_MEMADD_SIZE_8BIT, Buffer, Length); 01158 } 01159 01160 /** 01161 * @brief IOE delay. 01162 * @param Delay: Delay in ms 01163 * @retval None 01164 */ 01165 void IOE_Delay(uint32_t Delay) 01166 { 01167 HAL_Delay(Delay); 01168 } 01169 #endif /* USE_IOEXPANDER */ 01170 01171 /********************************* LINK AUDIO *********************************/ 01172 01173 /** 01174 * @brief Initializes Audio low level. 01175 * @retval None 01176 */ 01177 void AUDIO_IO_Init(void) 01178 { 01179 I2Cx_Init(); 01180 } 01181 01182 /** 01183 * @brief Deinitializes Audio low level. 01184 * @retval None 01185 */ 01186 void AUDIO_IO_DeInit(void) 01187 { 01188 I2Cx_DeInit(); 01189 } 01190 01191 /** 01192 * @brief Writes a single data. 01193 * @param Addr: I2C address 01194 * @param Reg: Reg address 01195 * @param Value: Data to be written 01196 * @retval None 01197 */ 01198 void AUDIO_IO_Write(uint8_t Addr, uint16_t Reg, uint16_t Value) 01199 { 01200 uint16_t tmp = Value; 01201 01202 Value = ((uint16_t)(tmp >> 8) & 0x00FF); 01203 Value |= ((uint16_t)(tmp << 8)& 0xFF00); 01204 01205 I2Cx_WriteBuffer(Addr, Reg, I2C_MEMADD_SIZE_16BIT,(uint8_t*)&Value, 2); 01206 } 01207 01208 /** 01209 * @brief Reads a single data. 01210 * @param Addr: I2C address 01211 * @param Reg: Reg address 01212 * @retval Data to be read 01213 */ 01214 uint16_t AUDIO_IO_Read(uint8_t Addr, uint16_t Reg) 01215 { 01216 uint16_t Read_Value = 0, tmp = 0; 01217 01218 I2Cx_ReadBuffer(Addr, Reg, I2C_MEMADD_SIZE_16BIT, (uint8_t*)&Read_Value, 2); 01219 01220 tmp = ((uint16_t)(Read_Value >> 8) & 0x00FF); 01221 tmp |= ((uint16_t)(Read_Value << 8)& 0xFF00); 01222 01223 Read_Value = tmp; 01224 01225 return Read_Value; 01226 } 01227 01228 /** 01229 * @brief AUDIO Codec delay 01230 * @param Delay: Delay in ms 01231 * @retval None 01232 */ 01233 void AUDIO_IO_Delay(uint32_t Delay) 01234 { 01235 HAL_Delay(Delay); 01236 } 01237 01238 /********************************* LINK I2C EEPROM *****************************/ 01239 /** 01240 * @brief Initializes peripherals used by the I2C EEPROM driver. 01241 * @retval None 01242 */ 01243 void EEPROM_I2C_IO_Init(void) 01244 { 01245 I2Cx_Init(); 01246 } 01247 01248 /** 01249 * @brief Write data to I2C EEPROM driver 01250 * @param DevAddress: Target device address 01251 * @param MemAddress: Internal memory address 01252 * @param pBuffer: Pointer to data buffer 01253 * @param BufferSize: Amount of data to be sent 01254 * @retval HAL status 01255 */ 01256 HAL_StatusTypeDef EEPROM_I2C_IO_WriteData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize) 01257 { 01258 return (I2Cx_WriteBuffer(DevAddress, MemAddress, I2C_MEMADD_SIZE_16BIT, pBuffer, BufferSize)); 01259 } 01260 01261 /** 01262 * @brief Read data from I2C EEPROM driver 01263 * @param DevAddress: Target device address 01264 * @param MemAddress: Internal memory address 01265 * @param pBuffer: Pointer to data buffer 01266 * @param BufferSize: Amount of data to be read 01267 * @retval HAL status 01268 */ 01269 HAL_StatusTypeDef EEPROM_I2C_IO_ReadData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize) 01270 { 01271 return (I2Cx_ReadBuffer(DevAddress, MemAddress, I2C_MEMADD_SIZE_16BIT, pBuffer, BufferSize)); 01272 } 01273 01274 /** 01275 * @brief Checks if target device is ready for communication. 01276 * @note This function is used with Memory devices 01277 * @param DevAddress: Target device address 01278 * @param Trials: Number of trials 01279 * @retval HAL status 01280 */ 01281 HAL_StatusTypeDef EEPROM_I2C_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials) 01282 { 01283 return (I2Cx_IsDeviceReady(DevAddress, Trials)); 01284 } 01285 01286 #endif /*HAL_I2C_MODULE_ENABLED*/ 01287 01288 01289 /** 01290 * @} 01291 */ 01292 01293 /** 01294 * @} 01295 */ 01296 01297 /** 01298 * @} 01299 */ 01300 01301 /** 01302 * @} 01303 */ 01304 01305 01306 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Sun Jun 21 2015 23:46:41 for STM32L476G_EVAL BSP User Manual by
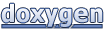