STM32L1xx_Nucleo BSP User Manual
|
stm32l1xx_nucleo.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l1xx_nucleo.c 00004 * @author MCD Application Team 00005 * @version 21-April-2017 00006 * @date V1.1.1 00007 * @brief This file provides set of firmware functions to manage: 00008 * - LEDs and push-button available on STM32L1XX-Nucleo Kit 00009 * from STMicroelectronics 00010 * - LCD, joystick and microSD available on Adafruit 1.8" TFT LCD 00011 * shield (reference ID 802) 00012 ****************************************************************************** 00013 * @attention 00014 * 00015 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00016 * 00017 * Redistribution and use in source and binary forms, with or without modification, 00018 * are permitted provided that the following conditions are met: 00019 * 1. Redistributions of source code must retain the above copyright notice, 00020 * this list of conditions and the following disclaimer. 00021 * 2. Redistributions in binary form must reproduce the above copyright notice, 00022 * this list of conditions and the following disclaimer in the documentation 00023 * and/or other materials provided with the distribution. 00024 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00025 * may be used to endorse or promote products derived from this software 00026 * without specific prior written permission. 00027 * 00028 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00029 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00030 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00031 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00032 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00033 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00034 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00035 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00036 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00037 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00038 * 00039 ****************************************************************************** 00040 */ 00041 00042 /* Includes ------------------------------------------------------------------*/ 00043 #include "stm32l1xx_nucleo.h" 00044 00045 /** @addtogroup BSP 00046 * @{ 00047 */ 00048 00049 /** @defgroup STM32L1XX_NUCLEO STM32L152RE-Nucleo 00050 * @brief This file provides set of firmware functions to manage Leds and push-button 00051 * available on STM32L1XX-Nucleo Kit from STMicroelectronics. 00052 * It provides also LCD, joystick and uSD functions to communicate with 00053 * Adafruit 1.8" TFT LCD shield (reference ID 802) 00054 * @{ 00055 */ 00056 00057 00058 /** @defgroup STM32L1XX_NUCLEO_Private_Defines Private Defines 00059 * @{ 00060 */ 00061 00062 /** 00063 * @brief STM32L152RE NUCLEO BSP Driver version 00064 */ 00065 #define __STM32L1XX_NUCLEO_BSP_VERSION_MAIN (0x01) /*!< [31:24] main version */ 00066 #define __STM32L1XX_NUCLEO_BSP_VERSION_SUB1 (0x01) /*!< [23:16] sub1 version */ 00067 #define __STM32L1XX_NUCLEO_BSP_VERSION_SUB2 (0x01) /*!< [15:8] sub2 version */ 00068 #define __STM32L1XX_NUCLEO_BSP_VERSION_RC (0x00) /*!< [7:0] release candidate */ 00069 #define __STM32L1XX_NUCLEO_BSP_VERSION ((__STM32L1XX_NUCLEO_BSP_VERSION_MAIN << 24)\ 00070 |(__STM32L1XX_NUCLEO_BSP_VERSION_SUB1 << 16)\ 00071 |(__STM32L1XX_NUCLEO_BSP_VERSION_SUB2 << 8 )\ 00072 |(__STM32L1XX_NUCLEO_BSP_VERSION_RC)) 00073 00074 /** 00075 * @brief LINK SD Card 00076 */ 00077 #define SD_DUMMY_BYTE 0xFF 00078 #define SD_NO_RESPONSE_EXPECTED 0x80 00079 00080 /** 00081 * @} 00082 */ 00083 00084 00085 /** @defgroup STM32L1XX_NUCLEO_Private_Variables Private Variables 00086 * @{ 00087 */ 00088 GPIO_TypeDef* LED_PORT[LEDn] = {LED2_GPIO_PORT}; 00089 00090 const uint16_t LED_PIN[LEDn] = {LED2_PIN}; 00091 00092 GPIO_TypeDef* BUTTON_PORT[BUTTONn] = {USER_BUTTON_GPIO_PORT}; 00093 const uint16_t BUTTON_PIN[BUTTONn] = {USER_BUTTON_PIN}; 00094 const uint8_t BUTTON_IRQn[BUTTONn] = {USER_BUTTON_EXTI_IRQn }; 00095 00096 /** 00097 * @brief BUS variables 00098 */ 00099 00100 #ifdef HAL_SPI_MODULE_ENABLED 00101 uint32_t SpixTimeout = NUCLEO_SPIx_TIMEOUT_MAX; /*<! Value of Timeout when SPI communication fails */ 00102 static SPI_HandleTypeDef hnucleo_Spi; 00103 #endif /* HAL_SPI_MODULE_ENABLED */ 00104 00105 #ifdef HAL_ADC_MODULE_ENABLED 00106 static ADC_HandleTypeDef hnucleo_Adc; 00107 /* ADC channel configuration structure declaration */ 00108 static ADC_ChannelConfTypeDef sConfig; 00109 #endif /* HAL_ADC_MODULE_ENABLED */ 00110 00111 /** 00112 * @} 00113 */ 00114 00115 /** @defgroup STM32L1XX_NUCLEO_Private_Functions Private Functions 00116 * @{ 00117 */ 00118 #ifdef HAL_SPI_MODULE_ENABLED 00119 static void SPIx_Init(void); 00120 static void SPIx_Write(uint8_t Value); 00121 static void SPIx_WriteData(uint8_t *DataIn, uint16_t DataLength); 00122 static void SPIx_WriteReadData(const uint8_t *DataIn, uint8_t *DataOut, uint16_t DataLegnth); 00123 static void SPIx_Error (void); 00124 static void SPIx_MspInit(void); 00125 00126 /* SD IO functions */ 00127 void SD_IO_Init(void); 00128 void SD_IO_CSState(uint8_t state); 00129 void SD_IO_WriteReadData(const uint8_t *DataIn, uint8_t *DataOut, uint16_t DataLength); 00130 void SD_IO_ReadData(uint8_t *DataOut, uint16_t DataLength); 00131 void SD_IO_WriteData(const uint8_t *Data, uint16_t DataLength); 00132 uint8_t SD_IO_WriteByte(uint8_t Data); 00133 uint8_t SD_IO_ReadByte(void); 00134 00135 /* LCD IO functions */ 00136 void LCD_IO_Init(void); 00137 void LCD_IO_WriteData(uint8_t Data); 00138 void LCD_IO_WriteMultipleData(uint8_t *pData, uint32_t Size); 00139 void LCD_IO_WriteReg(uint8_t LCDReg); 00140 void LCD_Delay(uint32_t delay); 00141 #endif /* HAL_SPI_MODULE_ENABLED */ 00142 00143 #ifdef HAL_ADC_MODULE_ENABLED 00144 static HAL_StatusTypeDef ADCx_Init(void); 00145 static void ADCx_DeInit(void); 00146 static void ADCx_MspInit(ADC_HandleTypeDef *hadc); 00147 static void ADCx_MspDeInit(ADC_HandleTypeDef *hadc); 00148 #endif /* HAL_ADC_MODULE_ENABLED */ 00149 /** 00150 * @} 00151 */ 00152 00153 /** @defgroup STM32L1XX_NUCLEO_Exported_Functions Exported Functions 00154 * @{ 00155 */ 00156 00157 /** 00158 * @brief This method returns the STM32L1XX NUCLEO BSP Driver revision 00159 * @retval version : 0xXYZR (8bits for each decimal, R for RC) 00160 */ 00161 uint32_t BSP_GetVersion(void) 00162 { 00163 return __STM32L1XX_NUCLEO_BSP_VERSION; 00164 } 00165 00166 /** @defgroup STM32L1XX_NUCLEO_LED_Functions LED Functions 00167 * @{ 00168 */ 00169 00170 /** 00171 * @brief Configures LED GPIO. 00172 * @param Led: Led to be configured. 00173 * This parameter can be one of the following values: 00174 * @arg LED2 00175 * @retval None 00176 */ 00177 void BSP_LED_Init(Led_TypeDef Led) 00178 { 00179 GPIO_InitTypeDef gpioinitstruct; 00180 00181 /* Enable the GPIO_LED Clock */ 00182 LEDx_GPIO_CLK_ENABLE(Led); 00183 00184 /* Configure the GPIO_LED pin */ 00185 gpioinitstruct.Pin = LED_PIN[Led]; 00186 gpioinitstruct.Mode = GPIO_MODE_OUTPUT_PP; 00187 gpioinitstruct.Pull = GPIO_NOPULL; 00188 gpioinitstruct.Speed = GPIO_SPEED_FREQ_HIGH; 00189 00190 HAL_GPIO_Init(LED_PORT[Led], &gpioinitstruct); 00191 00192 /* Reset PIN to switch off the LED */ 00193 HAL_GPIO_WritePin(LED_PORT[Led],LED_PIN[Led], GPIO_PIN_RESET); 00194 } 00195 00196 /** 00197 * @brief DeInit LEDs. 00198 * @param Led: LED to be de-init. 00199 * This parameter can be one of the following values: 00200 * @arg LED2 00201 * @note Led DeInit does not disable the GPIO clock nor disable the Mfx 00202 * @retval None 00203 */ 00204 void BSP_LED_DeInit(Led_TypeDef Led) 00205 { 00206 GPIO_InitTypeDef gpio_init_structure; 00207 00208 /* Turn off LED */ 00209 HAL_GPIO_WritePin(LED_PORT[Led],LED_PIN[Led], GPIO_PIN_RESET); 00210 /* DeInit the GPIO_LED pin */ 00211 gpio_init_structure.Pin = LED_PIN[Led]; 00212 HAL_GPIO_DeInit(LED_PORT[Led], gpio_init_structure.Pin); 00213 } 00214 00215 /** 00216 * @brief Turns selected LED On. 00217 * @param Led: Specifies the Led to be set on. 00218 * This parameter can be one of following parameters: 00219 * @arg LED2 00220 * @retval None 00221 */ 00222 void BSP_LED_On(Led_TypeDef Led) 00223 { 00224 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_SET); 00225 } 00226 00227 /** 00228 * @brief Turns selected LED Off. 00229 * @param Led: Specifies the Led to be set off. 00230 * This parameter can be one of following parameters: 00231 * @arg LED2 00232 * @retval None 00233 */ 00234 void BSP_LED_Off(Led_TypeDef Led) 00235 { 00236 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_RESET); 00237 } 00238 00239 /** 00240 * @brief Toggles the selected LED. 00241 * @param Led: Specifies the Led to be toggled. 00242 * This parameter can be one of following parameters: 00243 * @arg LED2 00244 * @retval None 00245 */ 00246 void BSP_LED_Toggle(Led_TypeDef Led) 00247 { 00248 HAL_GPIO_TogglePin(LED_PORT[Led], LED_PIN[Led]); 00249 } 00250 00251 /** 00252 * @} 00253 */ 00254 00255 /** @defgroup STM32L1XX_NUCLEO_BUTTON_Functions BUTTON Functions 00256 * @{ 00257 */ 00258 00259 /** 00260 * @brief Configures Button GPIO and EXTI Line. 00261 * @param Button: Specifies the Button to be configured. 00262 * This parameter should be: BUTTON_USER 00263 * @param ButtonMode: Specifies Button mode. 00264 * This parameter can be one of following parameters: 00265 * @arg BUTTON_MODE_GPIO: Button will be used as simple IO 00266 * @arg BUTTON_MODE_EXTI: Button will be connected to EXTI line with interrupt 00267 * generation capability 00268 * @retval None 00269 */ 00270 void BSP_PB_Init(Button_TypeDef Button, ButtonMode_TypeDef ButtonMode) 00271 { 00272 GPIO_InitTypeDef gpioinitstruct; 00273 00274 /* Enable the BUTTON Clock */ 00275 BUTTONx_GPIO_CLK_ENABLE(Button); 00276 00277 gpioinitstruct.Pin = BUTTON_PIN[Button]; 00278 gpioinitstruct.Pull = GPIO_NOPULL; 00279 gpioinitstruct.Speed = GPIO_SPEED_FREQ_MEDIUM; 00280 00281 if (ButtonMode == BUTTON_MODE_GPIO) 00282 { 00283 /* Configure Button pin as input */ 00284 gpioinitstruct.Mode = GPIO_MODE_INPUT; 00285 00286 HAL_GPIO_Init(BUTTON_PORT[Button], &gpioinitstruct); 00287 } 00288 00289 if (ButtonMode == BUTTON_MODE_EXTI) 00290 { 00291 /* Configure Button pin as input with External interrupt */ 00292 gpioinitstruct.Mode = GPIO_MODE_IT_FALLING; 00293 HAL_GPIO_Init(BUTTON_PORT[Button], &gpioinitstruct); 00294 00295 /* Enable and set Button EXTI Interrupt to the lowest priority */ 00296 HAL_NVIC_SetPriority((IRQn_Type)(BUTTON_IRQn[Button]), 0x0F, 0); 00297 HAL_NVIC_EnableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00298 } 00299 } 00300 00301 /** 00302 * @brief Push Button DeInit. 00303 * @param Button: Button to be configured 00304 * This parameter should be: BUTTON_USER 00305 * @note PB DeInit does not disable the GPIO clock 00306 * @retval None 00307 */ 00308 void BSP_PB_DeInit(Button_TypeDef Button) 00309 { 00310 GPIO_InitTypeDef gpio_init_structure; 00311 00312 gpio_init_structure.Pin = BUTTON_PIN[Button]; 00313 HAL_NVIC_DisableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00314 HAL_GPIO_DeInit(BUTTON_PORT[Button], gpio_init_structure.Pin); 00315 } 00316 00317 /** 00318 * @brief Returns the selected Button state. 00319 * @param Button: Specifies the Button to be checked. 00320 * This parameter should be: BUTTON_USER 00321 * @retval Button state. 00322 */ 00323 uint32_t BSP_PB_GetState(Button_TypeDef Button) 00324 { 00325 return HAL_GPIO_ReadPin(BUTTON_PORT[Button], BUTTON_PIN[Button]); 00326 } 00327 /** 00328 * @} 00329 */ 00330 00331 /** 00332 * @} 00333 */ 00334 00335 /** @addtogroup STM32L1XX_NUCLEO_Private_Functions 00336 * @{ 00337 */ 00338 00339 #ifdef HAL_SPI_MODULE_ENABLED 00340 /****************************************************************************** 00341 BUS OPERATIONS 00342 *******************************************************************************/ 00343 /** 00344 * @brief Initialize SPI MSP. 00345 * @retval None 00346 */ 00347 static void SPIx_MspInit(void) 00348 { 00349 GPIO_InitTypeDef gpioinitstruct = {0}; 00350 00351 /*** Configure the GPIOs ***/ 00352 /* Enable GPIO clock */ 00353 NUCLEO_SPIx_SCK_GPIO_CLK_ENABLE(); 00354 NUCLEO_SPIx_MISO_MOSI_GPIO_CLK_ENABLE(); 00355 00356 /* Configure SPI SCK */ 00357 gpioinitstruct.Pin = NUCLEO_SPIx_SCK_PIN; 00358 gpioinitstruct.Mode = GPIO_MODE_AF_PP; 00359 gpioinitstruct.Pull = GPIO_PULLUP; 00360 gpioinitstruct.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00361 gpioinitstruct.Alternate = NUCLEO_SPIx_SCK_AF; 00362 HAL_GPIO_Init(NUCLEO_SPIx_SCK_GPIO_PORT, &gpioinitstruct); 00363 00364 /* Configure SPI MISO and MOSI */ 00365 gpioinitstruct.Pin = NUCLEO_SPIx_MOSI_PIN; 00366 gpioinitstruct.Alternate = NUCLEO_SPIx_MISO_MOSI_AF; 00367 gpioinitstruct.Pull = GPIO_PULLDOWN; 00368 HAL_GPIO_Init(NUCLEO_SPIx_MISO_MOSI_GPIO_PORT, &gpioinitstruct); 00369 00370 gpioinitstruct.Pin = NUCLEO_SPIx_MISO_PIN; 00371 HAL_GPIO_Init(NUCLEO_SPIx_MISO_MOSI_GPIO_PORT, &gpioinitstruct); 00372 00373 /*** Configure the SPI peripheral ***/ 00374 /* Enable SPI clock */ 00375 NUCLEO_SPIx_CLK_ENABLE(); 00376 } 00377 00378 /** 00379 * @brief Initialize SPI HAL. 00380 * @retval None 00381 */ 00382 static void SPIx_Init(void) 00383 { 00384 if(HAL_SPI_GetState(&hnucleo_Spi) == HAL_SPI_STATE_RESET) 00385 { 00386 /* SPI Config */ 00387 hnucleo_Spi.Instance = NUCLEO_SPIx; 00388 /* SPI baudrate is set to 8 MHz maximum (PCLK2/SPI_BaudRatePrescaler = 64/8 = 8 MHz) 00389 to verify these constraints: 00390 - ST7735 LCD SPI interface max baudrate is 15MHz for write and 6.66MHz for read 00391 Since the provided driver doesn't use read capability from LCD, only constraint 00392 on write baudrate is considered. 00393 - SD card SPI interface max baudrate is 25MHz for write/read 00394 - PCLK2 max frequency is 32 MHz 00395 */ 00396 hnucleo_Spi.Init.BaudRatePrescaler = SPI_BAUDRATEPRESCALER_8; 00397 hnucleo_Spi.Init.Direction = SPI_DIRECTION_2LINES; 00398 hnucleo_Spi.Init.CLKPhase = SPI_PHASE_1EDGE; 00399 hnucleo_Spi.Init.CLKPolarity = SPI_POLARITY_LOW; 00400 hnucleo_Spi.Init.CRCCalculation = SPI_CRCCALCULATION_DISABLE; 00401 hnucleo_Spi.Init.CRCPolynomial = 7; 00402 hnucleo_Spi.Init.DataSize = SPI_DATASIZE_8BIT; 00403 hnucleo_Spi.Init.FirstBit = SPI_FIRSTBIT_MSB; 00404 hnucleo_Spi.Init.NSS = SPI_NSS_SOFT; 00405 hnucleo_Spi.Init.TIMode = SPI_TIMODE_DISABLE; 00406 hnucleo_Spi.Init.Mode = SPI_MODE_MASTER; 00407 00408 SPIx_MspInit(); 00409 HAL_SPI_Init(&hnucleo_Spi); 00410 } 00411 } 00412 00413 /** 00414 * @brief SPI Write a byte to device 00415 * @param DataIn value to be written 00416 * @param DataLength number of bytes to write 00417 * @param DataOut read value 00418 * @retval None 00419 */ 00420 static void SPIx_WriteReadData(const uint8_t *DataIn, uint8_t *DataOut, uint16_t DataLength) 00421 { 00422 HAL_StatusTypeDef status = HAL_OK; 00423 00424 status = HAL_SPI_TransmitReceive(&hnucleo_Spi, (uint8_t*) DataIn, DataOut, DataLength, SpixTimeout); 00425 00426 /* Check the communication status */ 00427 if(status != HAL_OK) 00428 { 00429 /* Execute user timeout callback */ 00430 SPIx_Error(); 00431 } 00432 } 00433 00434 /** 00435 * @brief SPI Write an amount of data to device 00436 * @param DataIn value to be written 00437 * @param DataLength number of bytes to write 00438 * @retval None 00439 */ 00440 static void SPIx_WriteData(uint8_t *DataIn, uint16_t DataLength) 00441 { 00442 HAL_StatusTypeDef status = HAL_OK; 00443 00444 status = HAL_SPI_Transmit(&hnucleo_Spi, DataIn, DataLength, SpixTimeout); 00445 00446 /* Check the communication status */ 00447 if(status != HAL_OK) 00448 { 00449 /* Execute user timeout callback */ 00450 SPIx_Error(); 00451 } 00452 } 00453 00454 /** 00455 * @brief SPI Write a byte to device 00456 * @param Value: value to be written 00457 * @retval None 00458 */ 00459 static void SPIx_Write(uint8_t Value) 00460 { 00461 HAL_StatusTypeDef status = HAL_OK; 00462 uint8_t data; 00463 00464 status = HAL_SPI_TransmitReceive(&hnucleo_Spi, (uint8_t*) &Value, &data, 1, SpixTimeout); 00465 00466 /* Check the communication status */ 00467 if(status != HAL_OK) 00468 { 00469 /* Execute user timeout callback */ 00470 SPIx_Error(); 00471 } 00472 } 00473 00474 /** 00475 * @brief SPI error treatment function 00476 * @retval None 00477 */ 00478 static void SPIx_Error (void) 00479 { 00480 /* De-initialize the SPI communication BUS */ 00481 HAL_SPI_DeInit(&hnucleo_Spi); 00482 00483 /* Re-Initiaize the SPI communication BUS */ 00484 SPIx_Init(); 00485 } 00486 00487 /****************************************************************************** 00488 LINK OPERATIONS 00489 *******************************************************************************/ 00490 00491 /********************************* LINK SD ************************************/ 00492 /** 00493 * @brief Initialize the SD Card and put it into StandBy State (Ready for 00494 * data transfer). 00495 * @retval None 00496 */ 00497 void SD_IO_Init(void) 00498 { 00499 GPIO_InitTypeDef gpioinitstruct = {0}; 00500 uint8_t counter = 0; 00501 00502 /* SD_CS_GPIO Periph clock enable */ 00503 SD_CS_GPIO_CLK_ENABLE(); 00504 00505 /* Configure SD_CS_PIN pin: SD Card CS pin */ 00506 gpioinitstruct.Pin = SD_CS_PIN; 00507 gpioinitstruct.Mode = GPIO_MODE_OUTPUT_PP; 00508 gpioinitstruct.Pull = GPIO_PULLUP; 00509 gpioinitstruct.Speed = GPIO_SPEED_FREQ_HIGH; 00510 HAL_GPIO_Init(SD_CS_GPIO_PORT, &gpioinitstruct); 00511 00512 /* Configure LCD_CS_PIN pin: LCD Card CS pin */ 00513 gpioinitstruct.Pin = LCD_CS_PIN; 00514 gpioinitstruct.Mode = GPIO_MODE_OUTPUT_PP; 00515 gpioinitstruct.Pull = GPIO_NOPULL; 00516 gpioinitstruct.Speed = GPIO_SPEED_FREQ_HIGH; 00517 HAL_GPIO_Init(SD_CS_GPIO_PORT, &gpioinitstruct); 00518 LCD_CS_HIGH(); 00519 /*------------Put SD in SPI mode--------------*/ 00520 /* SD SPI Config */ 00521 SPIx_Init(); 00522 00523 /* SD chip select high */ 00524 SD_CS_HIGH(); 00525 00526 /* Send dummy byte 0xFF, 10 times with CS high */ 00527 /* Rise CS and MOSI for 80 clocks cycles */ 00528 for (counter = 0; counter <= 9; counter++) 00529 { 00530 /* Send dummy byte 0xFF */ 00531 SD_IO_WriteByte(SD_DUMMY_BYTE); 00532 } 00533 } 00534 00535 /** 00536 * @brief Set the SD_CS pin. 00537 * @param val pin value. 00538 * @retval None 00539 */ 00540 void SD_IO_CSState(uint8_t val) 00541 { 00542 if(val == 1) 00543 { 00544 SD_CS_HIGH(); 00545 } 00546 else 00547 { 00548 SD_CS_LOW(); 00549 } 00550 } 00551 00552 /** 00553 * @brief Write byte(s) on the SD 00554 * @param DataIn: Pointer to data buffer to write 00555 * @param DataOut: Pointer to data buffer for read data 00556 * @param DataLength: number of bytes to write 00557 * @retval None 00558 */ 00559 void SD_IO_WriteReadData(const uint8_t *DataIn, uint8_t *DataOut, uint16_t DataLength) 00560 { 00561 /* Send the byte */ 00562 SPIx_WriteReadData(DataIn, DataOut, DataLength); 00563 } 00564 00565 /** 00566 * @brief Write a byte on the SD. 00567 * @param Data byte to send. 00568 * @retval Data written 00569 */ 00570 uint8_t SD_IO_WriteByte(uint8_t Data) 00571 { 00572 uint8_t tmp; 00573 00574 /* Send the byte */ 00575 SPIx_WriteReadData(&Data,&tmp,1); 00576 return tmp; 00577 } 00578 00579 /** 00580 * @brief Write an amount of data on the SD. 00581 * @param DataOut byte to send. 00582 * @param DataLength number of bytes to write 00583 * @retval none 00584 */ 00585 void SD_IO_ReadData(uint8_t *DataOut, uint16_t DataLength) 00586 { 00587 /* Send the byte */ 00588 SD_IO_WriteReadData(DataOut, DataOut, DataLength); 00589 } 00590 00591 /** 00592 * @brief Write an amount of data on the SD. 00593 * @param Data byte to send. 00594 * @param DataLength number of bytes to write 00595 * @retval none 00596 */ 00597 void SD_IO_WriteData(const uint8_t *Data, uint16_t DataLength) 00598 { 00599 /* Send the byte */ 00600 SPIx_WriteData((uint8_t *)Data, DataLength); 00601 } 00602 00603 /********************************* LINK LCD ***********************************/ 00604 /** 00605 * @brief Initialize the LCD 00606 * @retval None 00607 */ 00608 void LCD_IO_Init(void) 00609 { 00610 GPIO_InitTypeDef gpioinitstruct = {0}; 00611 00612 /* LCD_CS_GPIO and LCD_DC_GPIO Periph clock enable */ 00613 LCD_CS_GPIO_CLK_ENABLE(); 00614 LCD_DC_GPIO_CLK_ENABLE(); 00615 00616 /* Configure LCD_CS_PIN pin: LCD Card CS pin */ 00617 gpioinitstruct.Pin = LCD_CS_PIN; 00618 gpioinitstruct.Mode = GPIO_MODE_OUTPUT_PP; 00619 gpioinitstruct.Pull = GPIO_NOPULL; 00620 gpioinitstruct.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00621 HAL_GPIO_Init(SD_CS_GPIO_PORT, &gpioinitstruct); 00622 00623 /* Configure LCD_DC_PIN pin: LCD Card DC pin */ 00624 gpioinitstruct.Pin = LCD_DC_PIN; 00625 HAL_GPIO_Init(LCD_DC_GPIO_PORT, &gpioinitstruct); 00626 00627 /* LCD chip select high */ 00628 LCD_CS_HIGH(); 00629 00630 /* LCD SPI Config */ 00631 SPIx_Init(); 00632 } 00633 00634 /** 00635 * @brief Write command to select the LCD register. 00636 * @param LCDReg: Address of the selected register. 00637 * @retval None 00638 */ 00639 void LCD_IO_WriteReg(uint8_t LCDReg) 00640 { 00641 /* Reset LCD control line CS */ 00642 LCD_CS_LOW(); 00643 00644 /* Set LCD data/command line DC to Low */ 00645 LCD_DC_LOW(); 00646 00647 /* Send Command */ 00648 SPIx_Write(LCDReg); 00649 00650 /* Deselect : Chip Select high */ 00651 LCD_CS_HIGH(); 00652 } 00653 00654 /** 00655 * @brief Write register value. 00656 * @param pData Pointer on the register value 00657 * @param Size Size of byte to transmit to the register 00658 * @retval None 00659 */ 00660 void LCD_IO_WriteMultipleData(uint8_t *pData, uint32_t Size) 00661 { 00662 uint32_t counter = 0; 00663 00664 /* Reset LCD control line CS */ 00665 LCD_CS_LOW(); 00666 00667 /* Set LCD data/command line DC to High */ 00668 LCD_DC_HIGH(); 00669 00670 if (Size == 1) 00671 { 00672 /* Only 1 byte to be sent to LCD - general interface can be used */ 00673 /* Send Data */ 00674 SPIx_Write(*pData); 00675 } 00676 else 00677 { 00678 /* Several data should be sent in a raw */ 00679 /* Direct SPI accesses for optimization */ 00680 for (counter = Size; counter != 0; counter--) 00681 { 00682 while(((hnucleo_Spi.Instance->SR) & SPI_FLAG_TXE) != SPI_FLAG_TXE) 00683 { 00684 } 00685 /* Need to invert bytes for LCD*/ 00686 *((__IO uint8_t*)&hnucleo_Spi.Instance->DR) = *(pData+1); 00687 00688 while(((hnucleo_Spi.Instance->SR) & SPI_FLAG_TXE) != SPI_FLAG_TXE) 00689 { 00690 } 00691 *((__IO uint8_t*)&hnucleo_Spi.Instance->DR) = *pData; 00692 counter--; 00693 pData += 2; 00694 } 00695 00696 /* Wait until the bus is ready before releasing Chip select */ 00697 while(((hnucleo_Spi.Instance->SR) & SPI_FLAG_BSY) != RESET) 00698 { 00699 } 00700 } 00701 00702 /* Deselect : Chip Select high */ 00703 LCD_CS_HIGH(); 00704 } 00705 00706 /** 00707 * @brief Wait for loop in ms. 00708 * @param Delay in ms. 00709 * @retval None 00710 */ 00711 void LCD_Delay(uint32_t Delay) 00712 { 00713 HAL_Delay(Delay); 00714 } 00715 00716 #endif /* HAL_SPI_MODULE_ENABLED */ 00717 00718 #ifdef HAL_ADC_MODULE_ENABLED 00719 /******************************* LINK JOYSTICK ********************************/ 00720 /** 00721 * @brief Initialize ADC MSP. 00722 * @param hadc ADC peripheral 00723 * @retval None 00724 */ 00725 static void ADCx_MspInit(ADC_HandleTypeDef *hadc) 00726 { 00727 GPIO_InitTypeDef gpioinitstruct = {0}; 00728 00729 /*** Configure the GPIOs ***/ 00730 /* Enable GPIO clock */ 00731 NUCLEO_ADCx_GPIO_CLK_ENABLE(); 00732 00733 /* Configure ADC1 Channel8 as analog input */ 00734 gpioinitstruct.Pin = NUCLEO_ADCx_GPIO_PIN ; 00735 gpioinitstruct.Mode = GPIO_MODE_ANALOG; 00736 gpioinitstruct.Pull = GPIO_NOPULL; 00737 HAL_GPIO_Init(NUCLEO_ADCx_GPIO_PORT, &gpioinitstruct); 00738 00739 /*** Configure the ADC peripheral ***/ 00740 /* Enable ADC clock */ 00741 NUCLEO_ADCx_CLK_ENABLE(); 00742 } 00743 00744 /** 00745 * @brief DeInitializes ADC MSP. 00746 * @param hadc ADC peripheral 00747 * @note ADC DeInit does not disable the GPIO clock 00748 * @retval None 00749 */ 00750 static void ADCx_MspDeInit(ADC_HandleTypeDef *hadc) 00751 { 00752 GPIO_InitTypeDef gpioinitstruct; 00753 00754 /*** DeInit the ADC peripheral ***/ 00755 /* Disable ADC clock */ 00756 NUCLEO_ADCx_CLK_DISABLE(); 00757 00758 /* Configure the selected ADC Channel as analog input */ 00759 gpioinitstruct.Pin = NUCLEO_ADCx_GPIO_PIN ; 00760 HAL_GPIO_DeInit(NUCLEO_ADCx_GPIO_PORT, gpioinitstruct.Pin); 00761 00762 /* Disable GPIO clock has to be done by the application*/ 00763 /* NUCLEO_ADCx_GPIO_CLK_DISABLE(); */ 00764 } 00765 00766 /** 00767 * @brief Initializes ADC HAL. 00768 * @retval None 00769 */ 00770 static HAL_StatusTypeDef ADCx_Init(void) 00771 { 00772 /* Set ADC instance */ 00773 hnucleo_Adc.Instance = NUCLEO_ADCx; 00774 00775 if(HAL_ADC_GetState(&hnucleo_Adc) == HAL_ADC_STATE_RESET) 00776 { 00777 /* ADC Config */ 00778 hnucleo_Adc.Instance = NUCLEO_ADCx; 00779 00780 hnucleo_Adc.Init.ClockPrescaler = ADC_CLOCK_ASYNC_DIV2; /* ADC clock must not exceed 16MHz */ 00781 hnucleo_Adc.Init.Resolution = ADC_RESOLUTION_12B; 00782 hnucleo_Adc.Init.DataAlign = ADC_DATAALIGN_RIGHT; 00783 hnucleo_Adc.Init.ScanConvMode = ADC_SCAN_DISABLE; /* Sequencer disabled (ADC conversion on only 1 channel: channel set on rank 1) */ 00784 hnucleo_Adc.Init.EOCSelection = ADC_EOC_SEQ_CONV; 00785 hnucleo_Adc.Init.LowPowerAutoWait = ADC_AUTOWAIT_UNTIL_DATA_READ; 00786 hnucleo_Adc.Init.LowPowerAutoPowerOff = ADC_AUTOPOWEROFF_DISABLE; 00787 hnucleo_Adc.Init.ChannelsBank = ADC_CHANNELS_BANK_A; 00788 hnucleo_Adc.Init.ContinuousConvMode = DISABLE; /* Continuous mode disabled to have only 1 conversion at each conversion trig */ 00789 hnucleo_Adc.Init.NbrOfConversion = 1; /* Parameter discarded because sequencer is disabled */ 00790 hnucleo_Adc.Init.DiscontinuousConvMode = DISABLE; /* Parameter discarded because sequencer is disabled */ 00791 hnucleo_Adc.Init.NbrOfDiscConversion = 1; /* Parameter discarded because sequencer is disabled */ 00792 hnucleo_Adc.Init.ExternalTrigConv = ADC_SOFTWARE_START; /* Trig of conversion start done manually by software, without external event */ 00793 hnucleo_Adc.Init.ExternalTrigConvEdge = ADC_EXTERNALTRIGCONVEDGE_NONE; /* Parameter discarded because trig by software start */ 00794 hnucleo_Adc.Init.DMAContinuousRequests = DISABLE; 00795 00796 /* Initialize MSP related to ADC */ 00797 ADCx_MspInit(&hnucleo_Adc); 00798 00799 /* Initialize ADC */ 00800 if (HAL_ADC_Init(&hnucleo_Adc) != HAL_OK) 00801 { 00802 return HAL_ERROR; 00803 } 00804 } 00805 00806 return HAL_OK; 00807 } 00808 00809 /** 00810 * @brief Initializes ADC HAL. 00811 * @retval None 00812 */ 00813 static void ADCx_DeInit(void) 00814 { 00815 hnucleo_Adc.Instance = NUCLEO_ADCx; 00816 00817 HAL_ADC_DeInit(&hnucleo_Adc); 00818 ADCx_MspDeInit(&hnucleo_Adc); 00819 } 00820 00821 /******************************* LINK JOYSTICK ********************************/ 00822 00823 /** 00824 * @brief Configures joystick available on adafruit 1.8" TFT shield 00825 * managed through ADC to detect motion. 00826 * @retval Joystickstatus (0=> success, 1=> fail) 00827 */ 00828 uint8_t BSP_JOY_Init(void) 00829 { 00830 if (ADCx_Init() != HAL_OK) 00831 { 00832 return (uint8_t) HAL_ERROR; 00833 } 00834 00835 /* Select Channel 8 to be converted */ 00836 sConfig.Channel = ADC_CHANNEL_8; 00837 sConfig.SamplingTime = ADC_SAMPLETIME_16CYCLES; 00838 sConfig.Rank = ADC_REGULAR_RANK_1; 00839 00840 /* Return Joystick initialization status */ 00841 return (uint8_t)HAL_ADC_ConfigChannel(&hnucleo_Adc, &sConfig); 00842 } 00843 00844 /** 00845 * @brief DeInit joystick GPIOs. 00846 * @note JOY DeInit does not disable the Mfx, just set the Mfx pins in Off mode 00847 * @retval None. 00848 */ 00849 void BSP_JOY_DeInit(void) 00850 { 00851 ADCx_DeInit(); 00852 } 00853 00854 /** 00855 * @brief Returns the Joystick key pressed. 00856 * @note To know which Joystick key is pressed we need to detect the voltage 00857 * level on each key output 00858 * - None : 3.3 V / 4095 00859 * - SEL : 1.055 V / 1308 00860 * - DOWN : 0.71 V / 88 00861 * - LEFT : 3.0 V / 3720 00862 * - RIGHT : 0.595 V / 737 00863 * - UP : 1.65 V / 2046 00864 * @retval JOYState_TypeDef: Code of the Joystick key pressed. 00865 */ 00866 JOYState_TypeDef BSP_JOY_GetState(void) 00867 { 00868 JOYState_TypeDef state = JOY_NONE; 00869 uint16_t keyconvertedvalue = 0; 00870 00871 /* Start the conversion process */ 00872 HAL_ADC_Start(&hnucleo_Adc); 00873 00874 /* Wait for the end of conversion */ 00875 if (HAL_ADC_PollForConversion(&hnucleo_Adc, 10) != HAL_TIMEOUT) 00876 { 00877 /* Get the converted value of regular channel */ 00878 keyconvertedvalue = HAL_ADC_GetValue(&hnucleo_Adc); 00879 } 00880 00881 if((keyconvertedvalue > 2010) && (keyconvertedvalue < 2090)) 00882 { 00883 state = JOY_UP; 00884 } 00885 else if((keyconvertedvalue > 680) && (keyconvertedvalue < 780)) 00886 { 00887 state = JOY_RIGHT; 00888 } 00889 else if((keyconvertedvalue > 1270) && (keyconvertedvalue < 1350)) 00890 { 00891 state = JOY_SEL; 00892 } 00893 else if((keyconvertedvalue > 50) && (keyconvertedvalue < 130)) 00894 { 00895 state = JOY_DOWN; 00896 } 00897 else if((keyconvertedvalue > 3680) && (keyconvertedvalue < 3760)) 00898 { 00899 state = JOY_LEFT; 00900 } 00901 else 00902 { 00903 state = JOY_NONE; 00904 } 00905 00906 /* Return the code of the Joystick key pressed*/ 00907 return state; 00908 } 00909 #endif /* HAL_ADC_MODULE_ENABLED */ 00910 00911 /** 00912 * @} 00913 */ 00914 00915 /** 00916 * @} 00917 */ 00918 00919 /** 00920 * @} 00921 */ 00922 00923 /** 00924 * @} 00925 */ 00926 00927 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Sun Apr 16 2017 21:55:49 for STM32L1xx_Nucleo BSP User Manual by
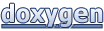