STM32L100C-Discovery BSP User Manual
|
stm32l100c_discovery.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l100c_discovery.h 00004 * @author MCD Application Team 00005 * @brief This file contains definitions for STM32L100C-Discovery's Leds, push-buttons 00006 ****************************************************************************** 00007 * @attention 00008 * 00009 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00010 * 00011 * Redistribution and use in source and binary forms, with or without modification, 00012 * are permitted provided that the following conditions are met: 00013 * 1. Redistributions of source code must retain the above copyright notice, 00014 * this list of conditions and the following disclaimer. 00015 * 2. Redistributions in binary form must reproduce the above copyright notice, 00016 * this list of conditions and the following disclaimer in the documentation 00017 * and/or other materials provided with the distribution. 00018 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00019 * may be used to endorse or promote products derived from this software 00020 * without specific prior written permission. 00021 * 00022 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00023 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00024 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00025 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00026 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00027 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00028 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00029 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00030 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00031 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00032 * 00033 ****************************************************************************** 00034 */ 00035 00036 /* Define to prevent recursive inclusion -------------------------------------*/ 00037 #ifndef __STM32L100C_DISCOVERY_H 00038 #define __STM32L100C_DISCOVERY_H 00039 00040 #ifdef __cplusplus 00041 extern "C" { 00042 #endif 00043 00044 /* Includes ------------------------------------------------------------------*/ 00045 #include "stm32l1xx_hal.h" 00046 00047 /** @addtogroup BSP 00048 * @{ 00049 */ 00050 00051 /** @addtogroup STM32L100C_DISCOVERY 00052 * @{ 00053 */ 00054 00055 /** @defgroup STM32L100C_DISCOVERY_Exported_Types Exported Types 00056 * @{ 00057 */ 00058 typedef enum 00059 { 00060 LED3 = 0, 00061 LED4 = 1, 00062 00063 LED_GREEN = LED3, 00064 LED_BLUE = LED4 00065 00066 } Led_TypeDef; 00067 00068 typedef enum 00069 { 00070 BUTTON_USER = 0, 00071 } Button_TypeDef; 00072 00073 typedef enum 00074 { 00075 BUTTON_MODE_GPIO = 0, 00076 BUTTON_MODE_EXTI = 1 00077 } ButtonMode_TypeDef; 00078 /** 00079 * @} 00080 */ 00081 00082 /** @defgroup STM32L100C_DISCOVERY_Exported_Constants Exported Constants 00083 * @{ 00084 */ 00085 00086 /** 00087 * @brief Define for STM32L100C-Discovery board 00088 */ 00089 #if !defined (USE_STM32L100C_DISCO) 00090 #define USE_STM32L100C_DISCO 00091 #endif 00092 00093 /** @defgroup STM32L100C_DISCOVERY_LED LED Constants 00094 * @{ 00095 */ 00096 #define LEDn 2 00097 00098 #define LED3_PIN GPIO_PIN_9 /* PC.09 */ 00099 #define LED3_GPIO_PORT GPIOC 00100 #define LED3_GPIO_CLK_ENABLE() __HAL_RCC_GPIOC_CLK_ENABLE() 00101 #define LED3_GPIO_CLK_DISABLE() __HAL_RCC_GPIOC_CLK_DISABLE() 00102 00103 #define LED4_PIN GPIO_PIN_8 /* PC.08 */ 00104 #define LED4_GPIO_PORT GPIOC 00105 #define LED4_GPIO_CLK_ENABLE() __HAL_RCC_GPIOC_CLK_ENABLE() 00106 #define LED4_GPIO_CLK_DISABLE() __HAL_RCC_GPIOC_CLK_DISABLE() 00107 00108 #define LEDx_GPIO_CLK_ENABLE(__INDEX__) do { if ((__INDEX__) == 0) LED3_GPIO_CLK_ENABLE(); else LED4_GPIO_CLK_ENABLE();} while(0) 00109 00110 #define LEDx_GPIO_CLK_DISABLE(__INDEX__) (((__INDEX__) == 0) ? LED3_GPIO_CLK_DISABLE() : LED4_GPIO_CLK_DISABLE()) 00111 /** 00112 * @} 00113 */ 00114 00115 /** @defgroup STM32L100C_DISCOVERY_BUTTON BUTTON Constants 00116 * @{ 00117 */ 00118 #define BUTTONn 1 00119 /** 00120 * @brief Key push-button 00121 */ 00122 #define USER_BUTTON_PIN GPIO_PIN_0 /* PA.00 */ 00123 #define USER_BUTTON_GPIO_PORT GPIOA 00124 #define USER_BUTTON_GPIO_CLK_ENABLE() __HAL_RCC_GPIOA_CLK_ENABLE() 00125 #define USER_BUTTON_GPIO_CLK_DISABLE() __HAL_RCC_GPIOA_CLK_DISABLE() 00126 #define USER_BUTTON_EXTI_IRQn EXTI0_IRQn 00127 00128 #define BUTTONx_GPIO_CLK_ENABLE(__INDEX__) do { USER_BUTTON_GPIO_CLK_ENABLE();} while(0) 00129 00130 #define BUTTONx_GPIO_CLK_DISABLE(__INDEX__) (USER_BUTTON_GPIO_CLK_DISABLE()) 00131 00132 /** 00133 * @} 00134 */ 00135 00136 /** 00137 * @} 00138 */ 00139 00140 /** @addtogroup STM32L100C_DISCOVERY_Exported_Functions 00141 * @{ 00142 */ 00143 uint32_t BSP_GetVersion(void); 00144 void BSP_LED_Init(Led_TypeDef Led); 00145 void BSP_LED_On(Led_TypeDef Led); 00146 void BSP_LED_Off(Led_TypeDef Led); 00147 void BSP_LED_Toggle(Led_TypeDef Led); 00148 void BSP_PB_Init(Button_TypeDef Button, ButtonMode_TypeDef Mode); 00149 uint32_t BSP_PB_GetState(Button_TypeDef Button); 00150 /** 00151 * @} 00152 */ 00153 #ifdef __cplusplus 00154 } 00155 #endif 00156 00157 #endif /* __STM32L100C_DISCOVERY_H */ 00158 /** 00159 * @} 00160 */ 00161 00162 /** 00163 * @} 00164 */ 00165 00166 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Thu Aug 24 2017 17:53:00 for STM32L100C-Discovery BSP User Manual by
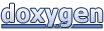