STM32L100C-Discovery BSP User Manual
|
stm32l100c_discovery.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l100c_discovery.c 00004 * @author MCD Application Team 00005 * @brief This file provides 00006 * - set of firmware functions to manage Led and push-button 00007 * available on STM32L100C-Discovery board from STMicroelectronics. 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00012 * 00013 * Redistribution and use in source and binary forms, with or without modification, 00014 * are permitted provided that the following conditions are met: 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright notice, 00018 * this list of conditions and the following disclaimer in the documentation 00019 * and/or other materials provided with the distribution. 00020 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00021 * may be used to endorse or promote products derived from this software 00022 * without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00025 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00026 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00028 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00029 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 ****************************************************************************** 00036 */ 00037 /* Includes ------------------------------------------------------------------*/ 00038 #include "stm32l100c_discovery.h" 00039 00040 /** @addtogroup BSP 00041 * @{ 00042 */ 00043 00044 /** @defgroup STM32L100C_DISCOVERY STM32L100C-Discovery 00045 * @brief This file provides firmware functions to manage Leds and push-buttons 00046 * available on STM32L100C discovery board from STMicroelectronics. 00047 * @{ 00048 */ 00049 00050 /** @defgroup STM32L100C_DISCOVERY_Private_Defines Private Defines 00051 * @{ 00052 */ 00053 00054 /** 00055 * @brief STM32L100C-Discovery BSP Driver version number 00056 */ 00057 #define __STM32L100C_DISCO_BSP_VERSION_MAIN (0x01) /*!< [31:24] main version */ 00058 #define __STM32L100C_DISCO_BSP_VERSION_SUB1 (0x00) /*!< [23:16] sub1 version */ 00059 #define __STM32L100C_DISCO_BSP_VERSION_SUB2 (0x04) /*!< [15:8] sub2 version */ 00060 #define __STM32L100C_DISCO_BSP_VERSION_RC (0x00) /*!< [7:0] release candidate */ 00061 #define __STM32L100C_DISCO_BSP_VERSION ((__STM32L100C_DISCO_BSP_VERSION_MAIN << 24)\ 00062 |(__STM32L100C_DISCO_BSP_VERSION_SUB1 << 16)\ 00063 |(__STM32L100C_DISCO_BSP_VERSION_SUB2 << 8 )\ 00064 |(__STM32L100C_DISCO_BSP_VERSION_RC)) 00065 /** 00066 * @} 00067 */ 00068 00069 /** @defgroup STM32L100C_DISCOVERY_Private_Variables Private Variables 00070 * @{ 00071 */ 00072 GPIO_TypeDef* GPIO_PORT[LEDn] = {LED3_GPIO_PORT, 00073 LED4_GPIO_PORT}; 00074 00075 const uint16_t GPIO_PIN[LEDn] = {LED3_PIN, 00076 LED4_PIN}; 00077 00078 00079 GPIO_TypeDef* BUTTON_PORT[BUTTONn] = {USER_BUTTON_GPIO_PORT}; 00080 const uint16_t BUTTON_PIN[BUTTONn] = {USER_BUTTON_PIN}; 00081 const uint8_t BUTTON_IRQn[BUTTONn] = {USER_BUTTON_EXTI_IRQn}; 00082 00083 /** 00084 * @} 00085 */ 00086 00087 00088 /** @defgroup STM32L100C_DISCOVERY_Exported_Functions Exported Functions 00089 * @{ 00090 */ 00091 00092 /** 00093 * @brief This method returns the STM32L100C-Discovery BSP Driver version 00094 * @retval version : 0xXYZR (8bits for each decimal, R for RC) 00095 */ 00096 uint32_t BSP_GetVersion(void) 00097 { 00098 return __STM32L100C_DISCO_BSP_VERSION; 00099 } 00100 00101 /** 00102 * @brief Configures LED GPIO. 00103 * @param Led: Specifies the Led to be configured. 00104 * This parameter can be one of following parameters: 00105 * @arg LED3 00106 * @arg LED4 00107 * @retval None 00108 */ 00109 void BSP_LED_Init(Led_TypeDef Led) 00110 { 00111 GPIO_InitTypeDef gpioinitstruct; 00112 00113 /* Enable the GPIO_LED Clock */ 00114 LEDx_GPIO_CLK_ENABLE(Led); 00115 00116 /* Configure the GPIO_LED pin */ 00117 gpioinitstruct.Pin = GPIO_PIN[Led]; 00118 gpioinitstruct.Mode = GPIO_MODE_OUTPUT_PP; 00119 gpioinitstruct.Pull = GPIO_NOPULL; 00120 gpioinitstruct.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00121 HAL_GPIO_Init(GPIO_PORT[Led], &gpioinitstruct); 00122 00123 /* Reset PIN to switch off the LED */ 00124 HAL_GPIO_WritePin(GPIO_PORT[Led],GPIO_PIN[Led], GPIO_PIN_RESET); 00125 } 00126 00127 /** 00128 * @brief Turns selected LED On. 00129 * @param Led: Specifies the Led to be set on. 00130 * This parameter can be one of following parameters: 00131 * @arg LED3 00132 * @arg LED4 00133 * @retval None 00134 */ 00135 void BSP_LED_On(Led_TypeDef Led) 00136 { 00137 HAL_GPIO_WritePin(GPIO_PORT[Led], GPIO_PIN[Led], GPIO_PIN_SET); 00138 } 00139 00140 /** 00141 * @brief Turns selected LED Off. 00142 * @param Led: Specifies the Led to be set off. 00143 * This parameter can be one of following parameters: 00144 * @arg LED3 00145 * @arg LED4 00146 * @retval None 00147 */ 00148 void BSP_LED_Off(Led_TypeDef Led) 00149 { 00150 HAL_GPIO_WritePin(GPIO_PORT[Led], GPIO_PIN[Led], GPIO_PIN_RESET); 00151 } 00152 00153 /** 00154 * @brief Toggles the selected LED. 00155 * @param Led: Specifies the Led to be toggled. 00156 * This parameter can be one of following parameters: 00157 * @arg LED3 00158 * @arg LED4 00159 * @retval None 00160 */ 00161 void BSP_LED_Toggle(Led_TypeDef Led) 00162 { 00163 HAL_GPIO_TogglePin(GPIO_PORT[Led], GPIO_PIN[Led]); 00164 } 00165 00166 /** 00167 * @brief Configures Button GPIO and EXTI Line. 00168 * @param Button: Specifies the Button to be configured. 00169 * This parameter should be: BUTTON_USER 00170 * @param Mode: Specifies Button mode. 00171 * This parameter can be one of following parameters: 00172 * @arg BUTTON_MODE_GPIO: Button will be used as simple IO 00173 * @arg BUTTON_MODE_EXTI: Button will be connected to EXTI line with interrupt 00174 * generation capability 00175 * @retval None 00176 */ 00177 void BSP_PB_Init(Button_TypeDef Button, ButtonMode_TypeDef Mode) 00178 { 00179 GPIO_InitTypeDef gpioinitstruct; 00180 00181 /* Enable the BUTTON Clock */ 00182 BUTTONx_GPIO_CLK_ENABLE(Button); 00183 00184 if (Mode == BUTTON_MODE_GPIO) 00185 { 00186 /* Configure Button pin as input */ 00187 gpioinitstruct.Pin = BUTTON_PIN[Button]; 00188 gpioinitstruct.Mode = GPIO_MODE_INPUT; 00189 gpioinitstruct.Pull = GPIO_NOPULL; 00190 gpioinitstruct.Speed = GPIO_SPEED_FREQ_HIGH; 00191 00192 HAL_GPIO_Init(BUTTON_PORT[Button], &gpioinitstruct); 00193 } 00194 else if (Mode == BUTTON_MODE_EXTI) 00195 { 00196 /* Configure Button pin as input with External interrupt */ 00197 gpioinitstruct.Pin = BUTTON_PIN[Button]; 00198 gpioinitstruct.Pull = GPIO_NOPULL; 00199 gpioinitstruct.Speed = GPIO_SPEED_FREQ_HIGH; 00200 gpioinitstruct.Mode = GPIO_MODE_IT_RISING; 00201 HAL_GPIO_Init(BUTTON_PORT[Button], &gpioinitstruct); 00202 00203 /* Enable and set Button EXTI Interrupt to the lowest priority */ 00204 HAL_NVIC_SetPriority((IRQn_Type)(BUTTON_IRQn[Button]), 0x0F, 0); 00205 HAL_NVIC_EnableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00206 } 00207 } 00208 00209 /** 00210 * @brief Returns the selected Button state. 00211 * @param Button: Specifies the Button to be checked. 00212 * This parameter should be: BUTTON_USER 00213 * @retval Button state. 00214 */ 00215 uint32_t BSP_PB_GetState(Button_TypeDef Button) 00216 { 00217 return HAL_GPIO_ReadPin(BUTTON_PORT[Button], BUTTON_PIN[Button]); 00218 } 00219 00220 /** 00221 * @} 00222 */ 00223 00224 /** 00225 * @} 00226 */ 00227 00228 /** 00229 * @} 00230 */ 00231 00232 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Thu Aug 24 2017 17:53:00 for STM32L100C-Discovery BSP User Manual by
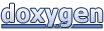