STM32L0xx_Nucleo_32 BSP User Manual
|
stm32l0xx_nucleo_32.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l0xx_nucleo_32.h 00004 * @author MCD Application Team 00005 * @brief This file contains definitions for: 00006 * - LEDs and push-button available on STM32L0XX-Nucleo Kit 00007 * from STMicroelectronics 00008 * - LCD, joystick and microSD available on Adafruit 1.8" TFT LCD 00009 * shield (reference ID 802) 00010 ****************************************************************************** 00011 * @attention 00012 * 00013 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00014 * 00015 * Redistribution and use in source and binary forms, with or without modification, 00016 * are permitted provided that the following conditions are met: 00017 * 1. Redistributions of source code must retain the above copyright notice, 00018 * this list of conditions and the following disclaimer. 00019 * 2. Redistributions in binary form must reproduce the above copyright notice, 00020 * this list of conditions and the following disclaimer in the documentation 00021 * and/or other materials provided with the distribution. 00022 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00023 * may be used to endorse or promote products derived from this software 00024 * without specific prior written permission. 00025 * 00026 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00027 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00028 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00029 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00030 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00031 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00032 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00033 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00034 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00035 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00036 * 00037 ****************************************************************************** 00038 */ 00039 00040 /* Define to prevent recursive inclusion -------------------------------------*/ 00041 #ifndef __STM32L0XX_NUCLEO_32_H 00042 #define __STM32L0XX_NUCLEO_32_H 00043 00044 #ifdef __cplusplus 00045 extern "C" { 00046 #endif 00047 00048 /* Includes ------------------------------------------------------------------*/ 00049 #include "stm32l0xx_hal.h" 00050 00051 00052 /** @addtogroup BSP 00053 * @{ 00054 */ 00055 00056 /** @addtogroup STM32L0XX_NUCLEO_32 NUCLEO 32 00057 * @{ 00058 */ 00059 00060 /** @defgroup STM32L0XX_NUCLEO_32_Exported_Types Exported Types 00061 * @{ 00062 */ 00063 typedef enum 00064 { 00065 LED3 = 0, 00066 LED_GREEN = LED3 00067 } Led_TypeDef; 00068 00069 typedef enum 00070 { 00071 JOY_NONE = 0, 00072 JOY_SEL = 1, 00073 JOY_DOWN = 2, 00074 JOY_LEFT = 3, 00075 JOY_RIGHT = 4, 00076 JOY_UP = 5 00077 } JOYState_TypeDef; 00078 00079 /** 00080 * @} 00081 */ 00082 00083 /** @defgroup STM32L0XX_NUCLEO_32_Exported_Constants Exported Constants 00084 * @{ 00085 */ 00086 00087 /** 00088 * @brief Define for STM32L0XX_NUCLEO_32 board 00089 */ 00090 #if !defined (USE_STM32L0XX_NUCLEO_32) 00091 #define USE_STM32L0XX_NUCLEO_32 00092 #endif 00093 00094 /** @addtogroup STM32L0XX_NUCLEO_32_LED 00095 * @{ 00096 */ 00097 #define LEDn 1 00098 00099 #define LED3_PIN GPIO_PIN_3 00100 #define LED3_GPIO_PORT GPIOB 00101 #define LED3_GPIO_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00102 #define LED3_GPIO_CLK_DISABLE() __HAL_RCC_GPIOB_CLK_DISABLE() 00103 00104 #define LEDx_GPIO_CLK_ENABLE(__INDEX__) do {LED3_GPIO_CLK_ENABLE(); } while(0) 00105 #define LEDx_GPIO_CLK_DISABLE(__INDEX__) LED3_GPIO_CLK_DISABLE()) 00106 /** 00107 * @} 00108 */ 00109 00110 00111 00112 /** @addtogroup STM32L0XX_NUCLEO_32_BUS 00113 * @{ 00114 */ 00115 #if defined(HAL_I2C_MODULE_ENABLED) 00116 /*##################### I2C2 ###################################*/ 00117 /* User can use this section to tailor I2Cx instance used and associated resources */ 00118 /* Definition for I2C1 Pins */ 00119 #define BSP_I2C1 I2C1 00120 #define BSP_I2C1_CLK_ENABLE() __HAL_RCC_I2C1_CLK_ENABLE() 00121 #define BSP_I2C1_CLK_DISABLE() __HAL_RCC_I2C1_CLK_DISABLE() 00122 #define BSP_I2C1_FORCE_RESET() __HAL_RCC_I2C1_FORCE_RESET() 00123 #define BSP_I2C1_RELEASE_RESET() __HAL_RCC_I2C1_RELEASE_RESET() 00124 00125 #define BSP_I2C1_SCL_PIN GPIO_PIN_6 /* PB.6 add wire between D5 and A5 */ 00126 #define BSP_I2C1_SDA_PIN GPIO_PIN_7 /* PB.7 add wire between D4 and A4 */ 00127 00128 #define BSP_I2C1_GPIO_PORT GPIOB /* GPIOB */ 00129 #define BSP_I2C1_GPIO_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00130 #define BSP_I2C1_GPIO_CLK_DISABLE() __HAL_RCC_GPIOB_CLK_DISABLE() 00131 #define BSP_I2C1_SCL_SDA_AF GPIO_AF1_I2C1 00132 00133 /* Maximum Timeout values for flags waiting loops. These timeouts are not based 00134 on accurate values, they just guarantee that the application will not remain 00135 stuck if the I2C communication is corrupted. 00136 You may modify these timeout values depending on CPU frequency and application 00137 conditions (interrupts routines ...). */ 00138 #define BSP_I2C1_TIMEOUT_MAX 1000 00139 00140 /* I2C TIMING is calculated in case of the I2C Clock source is the SYSCLK = 32 MHz */ 00141 /* Set TIMING to 0x009080B5 to reach 100 KHz speed (Rise time = 50ns, Fall time = 10ns) */ 00142 #define I2C1_TIMING 0x009080B5 00143 00144 #endif /* HAL_I2C_MODULE_ENABLED */ 00145 00146 #if defined(HAL_SPI_MODULE_ENABLED) 00147 /*###################### SPI1 ###################################*/ 00148 #define NUCLEO_SPIx SPI1 00149 #define NUCLEO_SPIx_CLK_ENABLE() __HAL_RCC_SPI1_CLK_ENABLE() 00150 00151 #define NUCLEO_SPIx_SCK_AF GPIO_AF0_SPI1 00152 #define NUCLEO_SPIx_SCK_GPIO_PORT GPIOA 00153 #define NUCLEO_SPIx_SCK_PIN GPIO_PIN_11 00154 #define NUCLEO_SPIx_SCK_GPIO_CLK_ENABLE() __HAL_RCC_GPIOA_CLK_ENABLE() 00155 #define NUCLEO_SPIx_SCK_GPIO_CLK_DISABLE() __HAL_RCC_GPIOA_CLK_DISABLE() 00156 00157 #define NUCLEO_SPIx_MISO_MOSI_AF GPIO_AF0_SPI1 00158 #define NUCLEO_SPIx_MISO_MOSI_GPIO_PORT GPIOB 00159 #define NUCLEO_SPIx_MISO_MOSI_GPIO_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00160 #define NUCLEO_SPIx_MISO_MOSI_GPIO_CLK_DISABLE() __HAL_RCC_GPIOB_CLK_DISABLE() 00161 #define NUCLEO_SPIx_MISO_PIN GPIO_PIN_4 00162 #define NUCLEO_SPIx_MOSI_PIN GPIO_PIN_5 00163 /* Maximum Timeout values for flags waiting loops. These timeouts are not based 00164 on accurate values, they just guarantee that the application will not remain 00165 stuck if the SPI communication is corrupted. 00166 You may modify these timeout values depending on CPU frequency and application 00167 conditions (interrupts routines ...). */ 00168 #define NUCLEO_SPIx_TIMEOUT_MAX 1000 00169 #endif /* HAL_SPI_MODULE_ENABLED */ 00170 /** 00171 * @} 00172 */ 00173 00174 /** @addtogroup STM32L0XX_NUCLEO_32_COMPONENT 00175 * @{ 00176 */ 00177 00178 /** 00179 * @brief SD Control Lines management 00180 */ 00181 #define SD_CS_LOW() HAL_GPIO_WritePin(SD_CS_GPIO_PORT, SD_CS_PIN, GPIO_PIN_RESET) 00182 #define SD_CS_HIGH() HAL_GPIO_WritePin(SD_CS_GPIO_PORT, SD_CS_PIN, GPIO_PIN_SET) 00183 00184 /** 00185 * @brief LCD Control Lines management 00186 */ 00187 #define LCD_CS_LOW() HAL_GPIO_WritePin(LCD_CS_GPIO_PORT, LCD_CS_PIN, GPIO_PIN_RESET) 00188 #define LCD_CS_HIGH() HAL_GPIO_WritePin(LCD_CS_GPIO_PORT, LCD_CS_PIN, GPIO_PIN_SET) 00189 #define LCD_DC_LOW() HAL_GPIO_WritePin(LCD_DC_GPIO_PORT, LCD_DC_PIN, GPIO_PIN_RESET) 00190 #define LCD_DC_HIGH() HAL_GPIO_WritePin(LCD_DC_GPIO_PORT, LCD_DC_PIN, GPIO_PIN_SET) 00191 00192 /** 00193 * @brief SD Control Interface pins 00194 */ 00195 #define SD_CS_PIN GPIO_PIN_5 00196 #define SD_CS_GPIO_PORT GPIOB 00197 #define SD_CS_GPIO_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00198 #define SD_CS_GPIO_CLK_DISABLE() __HAL_RCC_GPIOB_CLK_DISABLE() 00199 00200 /** 00201 * @brief LCD Control Interface pins 00202 */ 00203 #define LCD_CS_PIN GPIO_PIN_6 00204 #define LCD_CS_GPIO_PORT GPIOB 00205 #define LCD_CS_GPIO_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00206 #define LCD_CS_GPIO_CLK_DISABLE() __HAL_RCC_GPIOB_CLK_DISABLE() 00207 00208 /** 00209 * @brief LCD Data/Command Interface pins 00210 */ 00211 #define LCD_DC_PIN GPIO_PIN_9 00212 #define LCD_DC_GPIO_PORT GPIOA 00213 #define LCD_DC_GPIO_CLK_ENABLE() __HAL_RCC_GPIOA_CLK_ENABLE() 00214 #define LCD_DC_GPIO_CLK_DISABLE() __HAL_RCC_GPIOA_CLK_DISABLE() 00215 00216 #if defined(HAL_ADC_MODULE_ENABLED) 00217 /*##################### ADC1 ###################################*/ 00218 /** 00219 * @brief ADC Interface pins 00220 * used to detect motion of Joystick available on Adafruit 1.8" TFT shield 00221 */ 00222 #define NUCLEO_ADCx ADC1 00223 #define NUCLEO_ADCx_CLK_ENABLE() __HAL_RCC_ADC1_CLK_ENABLE() 00224 00225 #define NUCLEO_ADCx_GPIO_PORT GPIOB 00226 #define NUCLEO_ADCx_GPIO_PIN GPIO_PIN_0 00227 #define NUCLEO_ADCx_GPIO_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00228 #define NUCLEO_ADCx_GPIO_CLK_DISABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00229 00230 #endif /* HAL_ADC_MODULE_ENABLED */ 00231 /** 00232 * @} 00233 */ 00234 00235 /** @defgroup STM32L0XX_NUCLEO_32_Exported_Macros Exported Macros 00236 * @{ 00237 */ 00238 /** 00239 * @} 00240 */ 00241 00242 /** @defgroup STM32L0XX_NUCLEO_32_Internal_Functions Internal Functions 00243 * @{ 00244 */ 00245 #if defined(HAL_I2C_MODULE_ENABLED) 00246 /* I2C1 bus function */ 00247 /* Link function for I2C peripherals */ 00248 void I2C1_Init(void); 00249 void I2C1_Error (void); 00250 void I2C1_MspInit(I2C_HandleTypeDef *hi2c); 00251 void I2C1_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00252 uint8_t I2C1_Read(uint8_t Addr, uint8_t Reg); 00253 HAL_StatusTypeDef I2C1_WriteBuffer(uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length); 00254 HAL_StatusTypeDef I2C1_ReadBuffer(uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length); 00255 HAL_StatusTypeDef I2C1_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00256 #endif /* HAL_I2C_MODULE_ENABLED */ 00257 /** 00258 * @} 00259 */ 00260 00261 /** @defgroup STM32L0XX_NUCLEO_32_Exported_Functions Exported Functions 00262 * @{ 00263 */ 00264 uint32_t BSP_GetVersion(void); 00265 void BSP_LED_Init(Led_TypeDef Led); 00266 void BSP_LED_On(Led_TypeDef Led); 00267 void BSP_LED_Off(Led_TypeDef Led); 00268 void BSP_LED_Toggle(Led_TypeDef Led); 00269 #if defined(HAL_ADC_MODULE_ENABLED) 00270 uint8_t BSP_JOY_Init(void); 00271 JOYState_TypeDef BSP_JOY_GetState(void); 00272 #endif /* HAL_ADC_MODULE_ENABLED */ 00273 /** 00274 * @} 00275 */ 00276 00277 /** 00278 * @} 00279 */ 00280 00281 /** 00282 * @} 00283 */ 00284 00285 /** 00286 * @} 00287 */ 00288 00289 #ifdef __cplusplus 00290 } 00291 #endif 00292 00293 #endif /* __STM32L0XX_NUCLEO_32_H */ 00294 00295 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 00296
Generated on Mon Aug 28 2017 14:48:51 for STM32L0xx_Nucleo_32 BSP User Manual by
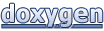