STM32L0xx_Nucleo_32 BSP User Manual
|
stm32l0xx_nucleo_32.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l0xx_nucleo_32.c 00004 * @author MCD Application Team 00005 * @brief This file provides set of firmware functions to manage: 00006 * - LEDs and push-button available on STM32L0XX-Nucleo Kit 00007 * from STMicroelectronics 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00012 * 00013 * Redistribution and use in source and binary forms, with or without modification, 00014 * are permitted provided that the following conditions are met: 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright notice, 00018 * this list of conditions and the following disclaimer in the documentation 00019 * and/or other materials provided with the distribution. 00020 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00021 * may be used to endorse or promote products derived from this software 00022 * without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00025 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00026 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00028 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00029 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 ****************************************************************************** 00036 */ 00037 00038 /* Includes ------------------------------------------------------------------*/ 00039 #include "stm32l0xx_nucleo_32.h" 00040 00041 /** @addtogroup BSP 00042 * @{ 00043 */ 00044 00045 /** @addtogroup STM32L0XX_NUCLEO_32 NUCLEO 32 00046 * @brief This file provides set of firmware functions to manage Leds and push-button 00047 * available on STM32L0XX-Nucleo Kit from STMicroelectronics. 00048 * @{ 00049 */ 00050 00051 /** @defgroup STM32L0XX_NUCLEO_32_Private_Defines Private Defines 00052 * @{ 00053 */ 00054 00055 /** 00056 * @brief STM32L0XX NUCLEO BSP Driver version number 00057 */ 00058 #define __STM32L0XX_NUCLEO_32_BSP_VERSION_MAIN (0x01) /*!< [31:24] main version */ 00059 #define __STM32L0XX_NUCLEO_32_BSP_VERSION_SUB1 (0x00) /*!< [23:16] sub1 version */ 00060 #define __STM32L0XX_NUCLEO_32_BSP_VERSION_SUB2 (0x03) /*!< [15:8] sub2 version */ 00061 #define __STM32L0XX_NUCLEO_32_BSP_VERSION_RC (0x00) /*!< [7:0] release candidate */ 00062 #define __STM32L0XX_NUCLEO_32_BSP_VERSION ((__STM32L0XX_NUCLEO_32_BSP_VERSION_MAIN << 24)\ 00063 |(__STM32L0XX_NUCLEO_32_BSP_VERSION_SUB1 << 16)\ 00064 |(__STM32L0XX_NUCLEO_32_BSP_VERSION_SUB2 << 8 )\ 00065 |(__STM32L0XX_NUCLEO_32_BSP_VERSION_RC)) 00066 00067 /** 00068 * @brief LINK SD Card 00069 */ 00070 #define SD_DUMMY_BYTE 0xFF 00071 #define SD_NO_RESPONSE_EXPECTED 0x80 00072 00073 /** 00074 * @} 00075 */ 00076 00077 /** @defgroup STM32L0XX_NUCLEO_32_Private_Variables Private Variables 00078 * @{ 00079 */ 00080 GPIO_TypeDef* LED_PORT[LEDn] = {LED3_GPIO_PORT}; 00081 const uint16_t LED_PIN[LEDn] = {LED3_PIN}; 00082 00083 /** 00084 * @brief BUS variables 00085 */ 00086 #if defined(HAL_I2C_MODULE_ENABLED) 00087 uint32_t I2c1Timeout = BSP_I2C1_TIMEOUT_MAX; /*<! Value of Timeout when I2C1 communication fails */ 00088 I2C_HandleTypeDef heval_I2c1; 00089 #endif /* HAL_I2C_MODULE_ENABLED */ 00090 00091 #ifdef HAL_SPI_MODULE_ENABLED 00092 uint32_t SpixTimeout = NUCLEO_SPIx_TIMEOUT_MAX; /*<! Value of Timeout when SPI communication fails */ 00093 static SPI_HandleTypeDef hnucleo_Spi; 00094 #endif /* HAL_SPI_MODULE_ENABLED */ 00095 00096 #ifdef HAL_ADC_MODULE_ENABLED 00097 static ADC_HandleTypeDef hnucleo_Adc; 00098 /* ADC channel configuration structure declaration */ 00099 static ADC_ChannelConfTypeDef sConfig; 00100 #endif /* HAL_ADC_MODULE_ENABLED */ 00101 /** 00102 * @} 00103 */ 00104 00105 /** @defgroup STM32L0XX_NUCLEO_32_Private_Function_Prototypes Private Function Prototypes 00106 * @{ 00107 */ 00108 00109 #ifdef HAL_SPI_MODULE_ENABLED 00110 static void SPIx_Init(void); 00111 static void SPIx_Write(uint8_t Value); 00112 static uint32_t SPIx_Read(void); 00113 static void SPIx_Error (void); 00114 static void SPIx_MspInit(SPI_HandleTypeDef *hspi); 00115 00116 /* SD IO functions */ 00117 void SD_IO_Init(void); 00118 HAL_StatusTypeDef SD_IO_WriteCmd(uint8_t Cmd, uint32_t Arg, uint8_t Crc, uint8_t Response); 00119 HAL_StatusTypeDef SD_IO_WaitResponse(uint8_t Response); 00120 void SD_IO_WriteDummy(void); 00121 void SD_IO_WriteByte(uint8_t Data); 00122 uint8_t SD_IO_ReadByte(void); 00123 00124 /* LCD IO functions */ 00125 void LCD_IO_Init(void); 00126 void LCD_IO_WriteData(uint8_t Data); 00127 void LCD_IO_WriteMultipleData(uint8_t *pData, uint32_t Size); 00128 void LCD_IO_WriteReg(uint8_t LCDReg); 00129 void LCD_Delay(uint32_t delay); 00130 #endif /* HAL_SPI_MODULE_ENABLED */ 00131 00132 #ifdef HAL_ADC_MODULE_ENABLED 00133 static void ADCx_Init(void); 00134 static void ADCx_MspInit(ADC_HandleTypeDef *hadc); 00135 #endif /* HAL_ADC_MODULE_ENABLED */ 00136 /** 00137 * @} 00138 */ 00139 00140 /** @defgroup STM32L0XX_NUCLEO_32_Private_Functions Private Functions 00141 * @{ 00142 */ 00143 00144 /** 00145 * @brief This method returns the STM32L0XX NUCLEO BSP Driver revision 00146 * @retval version : 0xXYZR (8bits for each decimal, R for RC) 00147 */ 00148 uint32_t BSP_GetVersion(void) 00149 { 00150 return __STM32L0XX_NUCLEO_32_BSP_VERSION; 00151 } 00152 00153 /** 00154 * @brief Configures LED GPIO. 00155 * @param Led: Specifies the Led to be configured. 00156 * This parameter can be one of following parameters: 00157 * @arg LED3 00158 * @retval None 00159 */ 00160 void BSP_LED_Init(Led_TypeDef Led) 00161 { 00162 GPIO_InitTypeDef GPIO_InitStruct; 00163 00164 /* Enable the GPIO_LED Clock */ 00165 LEDx_GPIO_CLK_ENABLE(Led); 00166 00167 /* Configure the GPIO_LED pin */ 00168 GPIO_InitStruct.Pin = LED_PIN[Led]; 00169 GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; 00170 GPIO_InitStruct.Pull = GPIO_NOPULL; 00171 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00172 00173 HAL_GPIO_Init(LED_PORT[Led], &GPIO_InitStruct); 00174 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_RESET); 00175 } 00176 00177 /** 00178 * @brief Turns selected LED On. 00179 * @param Led: Specifies the Led to be set on. 00180 * This parameter can be one of following parameters: 00181 * @arg LED3 00182 * @retval None 00183 */ 00184 void BSP_LED_On(Led_TypeDef Led) 00185 { 00186 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_SET); 00187 } 00188 00189 /** 00190 * @brief Turns selected LED Off. 00191 * @param Led: Specifies the Led to be set off. 00192 * This parameter can be one of following parameters: 00193 * @arg LED3 00194 * @retval None 00195 */ 00196 void BSP_LED_Off(Led_TypeDef Led) 00197 { 00198 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_RESET); 00199 } 00200 00201 /** 00202 * @brief Toggles the selected LED. 00203 * @param Led: Specifies the Led to be toggled. 00204 * This parameter can be one of following parameters: 00205 * @arg LED3 00206 * @retval None 00207 */ 00208 void BSP_LED_Toggle(Led_TypeDef Led) 00209 { 00210 HAL_GPIO_TogglePin(LED_PORT[Led], LED_PIN[Led]); 00211 } 00212 00213 /****************************************************************************** 00214 BUS OPERATIONS 00215 *******************************************************************************/ 00216 #if defined(HAL_I2C_MODULE_ENABLED) 00217 /******************************* I2C Routines *********************************/ 00218 00219 /** 00220 * @brief I2C Bus initialization 00221 * @retval None 00222 */ 00223 void I2C1_Init(void) 00224 { 00225 if(HAL_I2C_GetState(&heval_I2c1) == HAL_I2C_STATE_RESET) 00226 { 00227 heval_I2c1.Instance = BSP_I2C1; 00228 heval_I2c1.Init.Timing = I2C1_TIMING; 00229 heval_I2c1.Init.OwnAddress1 = 0; 00230 heval_I2c1.Init.AddressingMode = I2C_ADDRESSINGMODE_7BIT; 00231 heval_I2c1.Init.DualAddressMode = I2C_DUALADDRESS_DISABLE; 00232 heval_I2c1.Init.OwnAddress2 = 0; 00233 heval_I2c1.Init.OwnAddress2Masks = I2C_OA2_NOMASK; 00234 heval_I2c1.Init.GeneralCallMode = I2C_GENERALCALL_DISABLE; 00235 heval_I2c1.Init.NoStretchMode = I2C_NOSTRETCH_DISABLE; 00236 00237 /* Init the I2C */ 00238 I2C1_MspInit(&heval_I2c1); 00239 HAL_I2C_Init(&heval_I2c1); 00240 } 00241 } 00242 00243 /** 00244 * @brief Writes a single data. 00245 * @param Addr: I2C address 00246 * @param Reg: Register address 00247 * @param Value: Data to be written 00248 * @retval None 00249 */ 00250 void I2C1_Write(uint8_t Addr, uint8_t Reg, uint8_t Value) 00251 { 00252 HAL_StatusTypeDef status = HAL_OK; 00253 00254 status = HAL_I2C_Mem_Write(&heval_I2c1, Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, &Value, 1, 100); 00255 00256 /* Check the communication status */ 00257 if(status != HAL_OK) 00258 { 00259 /* Execute user timeout callback */ 00260 I2C1_Error(); 00261 } 00262 } 00263 00264 /** 00265 * @brief Reads a single data. 00266 * @param Addr: I2C address 00267 * @param Reg: Register address 00268 * @retval Read data 00269 */ 00270 uint8_t I2C1_Read(uint8_t Addr, uint8_t Reg) 00271 { 00272 HAL_StatusTypeDef status = HAL_OK; 00273 uint8_t Value = 0; 00274 00275 status = HAL_I2C_Mem_Read(&heval_I2c1, Addr, Reg, I2C_MEMADD_SIZE_8BIT, &Value, 1, 1000); 00276 00277 /* Check the communication status */ 00278 if(status != HAL_OK) 00279 { 00280 /* Execute user timeout callback */ 00281 I2C1_Error(); 00282 } 00283 return Value; 00284 } 00285 00286 00287 00288 /** 00289 * @brief Reads multiple data on the BUS. 00290 * @param Addr : I2C Address 00291 * @param Reg : Reg Address 00292 * @param RegSize : The target register size (can be 8BIT or 16BIT) 00293 * @param pBuffer : pointer to read data buffer 00294 * @param Length : length of the data 00295 * @retval 0 if no problems to read multiple data 00296 */ 00297 HAL_StatusTypeDef I2C1_ReadBuffer(uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length) 00298 { 00299 HAL_StatusTypeDef status = HAL_OK; 00300 00301 status = HAL_I2C_Mem_Read(&heval_I2c1, Addr, Reg, RegSize, pBuffer, Length, I2c1Timeout); 00302 00303 /* Check the communication status */ 00304 if(status != HAL_OK) 00305 { 00306 /* Re-Initiaize the BUS */ 00307 I2C1_Error(); 00308 } 00309 return status; 00310 } 00311 00312 /** 00313 * @brief Checks if target device is ready for communication. 00314 * @note This function is used with Memory devices 00315 * @param DevAddress: Target device address 00316 * @param Trials: Number of trials 00317 * @retval HAL status 00318 */ 00319 HAL_StatusTypeDef I2C1_IsDeviceReady(uint16_t DevAddress, uint32_t Trials) 00320 { 00321 return (HAL_I2C_IsDeviceReady(&heval_I2c1, DevAddress, Trials, I2c1Timeout)); 00322 } 00323 00324 /** 00325 * @brief Write a value in a register of the device through BUS. 00326 * @param Addr: Device address on BUS Bus. 00327 * @param Reg: The target register address to write 00328 * @param RegSize: The target register size (can be 8BIT or 16BIT) 00329 * @param pBuffer: The target register value to be written 00330 * @param Length: buffer size to be written 00331 * @retval None 00332 */ 00333 HAL_StatusTypeDef I2C1_WriteBuffer(uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length) 00334 { 00335 HAL_StatusTypeDef status = HAL_OK; 00336 00337 status = HAL_I2C_Mem_Write(&heval_I2c1, Addr, Reg, RegSize, pBuffer, Length, I2c1Timeout); 00338 00339 /* Check the communication status */ 00340 if(status != HAL_OK) 00341 { 00342 /* Re-Initiaize the BUS */ 00343 I2C1_Error(); 00344 } 00345 return status; 00346 } 00347 00348 /** 00349 * @brief Manages error callback by re-initializing I2C. 00350 * @retval None 00351 */ 00352 void I2C1_Error(void) 00353 { 00354 /* De-initialize the I2C communication BUS */ 00355 HAL_I2C_DeInit(&heval_I2c1); 00356 00357 /* Re-Initiaize the I2C communication BUS */ 00358 I2C1_Init(); 00359 } 00360 00361 /** 00362 * @brief I2C MSP Initialization 00363 * @param hi2c: I2C handle 00364 * @retval None 00365 */ 00366 void I2C1_MspInit(I2C_HandleTypeDef *hi2c) 00367 { 00368 GPIO_InitTypeDef GPIO_InitStruct; 00369 RCC_PeriphCLKInitTypeDef RCC_PeriphCLKInitStruct; 00370 00371 /*##-1- Set source clock to SYSCLK for I2C1 ################################################*/ 00372 RCC_PeriphCLKInitStruct.PeriphClockSelection = RCC_PERIPHCLK_I2C1; 00373 RCC_PeriphCLKInitStruct.I2c1ClockSelection = RCC_I2C1CLKSOURCE_SYSCLK; 00374 HAL_RCCEx_PeriphCLKConfig(&RCC_PeriphCLKInitStruct); 00375 00376 /*##-2- Configure the GPIOs ################################################*/ 00377 00378 /* Enable GPIO clock */ 00379 BSP_I2C1_GPIO_CLK_ENABLE(); 00380 00381 /* Configure I2C SCL & SDA as alternate function */ 00382 GPIO_InitStruct.Pin = (BSP_I2C1_SCL_PIN| BSP_I2C1_SDA_PIN); 00383 GPIO_InitStruct.Mode = GPIO_MODE_AF_OD; 00384 GPIO_InitStruct.Pull = GPIO_NOPULL; 00385 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00386 GPIO_InitStruct.Alternate = BSP_I2C1_SCL_SDA_AF; 00387 HAL_GPIO_Init(BSP_I2C1_GPIO_PORT, &GPIO_InitStruct); 00388 00389 /*##-3- Configure the Eval I2C peripheral #######################################*/ 00390 /* Enable I2C clock */ 00391 BSP_I2C1_CLK_ENABLE(); 00392 00393 /* Force the I2C peripheral clock reset */ 00394 BSP_I2C1_FORCE_RESET(); 00395 00396 /* Release the I2C peripheral clock reset */ 00397 BSP_I2C1_RELEASE_RESET(); 00398 } 00399 00400 #endif /*HAL_I2C_MODULE_ENABLED*/ 00401 00402 #ifdef HAL_SPI_MODULE_ENABLED 00403 /** 00404 * @brief Initializes SPI MSP. 00405 * @param hspi: SPI handle 00406 * @retval None 00407 */ 00408 static void SPIx_MspInit(SPI_HandleTypeDef *hspi) 00409 { 00410 GPIO_InitTypeDef GPIO_InitStruct; 00411 00412 /*** Configure the GPIOs ***/ 00413 /* Enable GPIO clock */ 00414 NUCLEO_SPIx_SCK_GPIO_CLK_ENABLE(); 00415 NUCLEO_SPIx_MISO_MOSI_GPIO_CLK_ENABLE(); 00416 00417 /* Configure SPI SCK */ 00418 GPIO_InitStruct.Pin = NUCLEO_SPIx_SCK_PIN; 00419 GPIO_InitStruct.Mode = GPIO_MODE_AF_PP; 00420 GPIO_InitStruct.Pull = GPIO_PULLUP; 00421 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00422 GPIO_InitStruct.Alternate = NUCLEO_SPIx_SCK_AF; 00423 HAL_GPIO_Init(NUCLEO_SPIx_SCK_GPIO_PORT, &GPIO_InitStruct); 00424 00425 /* Configure SPI MISO and MOSI */ 00426 GPIO_InitStruct.Pin = NUCLEO_SPIx_MOSI_PIN; 00427 GPIO_InitStruct.Alternate = NUCLEO_SPIx_MISO_MOSI_AF; 00428 GPIO_InitStruct.Pull = GPIO_PULLDOWN; 00429 HAL_GPIO_Init(NUCLEO_SPIx_MISO_MOSI_GPIO_PORT, &GPIO_InitStruct); 00430 00431 GPIO_InitStruct.Pin = NUCLEO_SPIx_MISO_PIN; 00432 HAL_GPIO_Init(NUCLEO_SPIx_MISO_MOSI_GPIO_PORT, &GPIO_InitStruct); 00433 00434 /*** Configure the SPI peripheral ***/ 00435 /* Enable SPI clock */ 00436 NUCLEO_SPIx_CLK_ENABLE(); 00437 } 00438 00439 /** 00440 * @brief Initializes SPI HAL. 00441 * @retval None 00442 */ 00443 static void SPIx_Init(void) 00444 { 00445 if(HAL_SPI_GetState(&hnucleo_Spi) == HAL_SPI_STATE_RESET) 00446 { 00447 /* SPI Config */ 00448 hnucleo_Spi.Instance = NUCLEO_SPIx; 00449 /* SPI baudrate is set to 8 MHz maximum (PCLK2/SPI_BaudRatePrescaler = 32/4 = 8 MHz) 00450 to verify these constraints: 00451 - ST7735 LCD SPI interface max baudrate is 15MHz for write and 6.66MHz for read 00452 Since the provided driver doesn't use read capability from LCD, only constraint 00453 on write baudrate is considered. 00454 - SD card SPI interface max baudrate is 25MHz for write/read 00455 - PCLK2 max frequency is 32 MHz 00456 */ 00457 hnucleo_Spi.Init.BaudRatePrescaler = SPI_BAUDRATEPRESCALER_4; 00458 hnucleo_Spi.Init.Direction = SPI_DIRECTION_2LINES; 00459 hnucleo_Spi.Init.CLKPhase = SPI_PHASE_2EDGE; 00460 hnucleo_Spi.Init.CLKPolarity = SPI_POLARITY_HIGH; 00461 hnucleo_Spi.Init.CRCCalculation = SPI_CRCCALCULATION_DISABLE; 00462 hnucleo_Spi.Init.CRCPolynomial = 7; 00463 hnucleo_Spi.Init.DataSize = SPI_DATASIZE_8BIT; 00464 hnucleo_Spi.Init.FirstBit = SPI_FIRSTBIT_MSB; 00465 hnucleo_Spi.Init.NSS = SPI_NSS_SOFT; 00466 hnucleo_Spi.Init.TIMode = SPI_TIMODE_DISABLE; 00467 hnucleo_Spi.Init.Mode = SPI_MODE_MASTER; 00468 00469 SPIx_MspInit(&hnucleo_Spi); 00470 HAL_SPI_Init(&hnucleo_Spi); 00471 } 00472 } 00473 00474 /** 00475 * @brief SPI Read 4 bytes from device 00476 * @retval Read data 00477 */ 00478 static uint32_t SPIx_Read(void) 00479 { 00480 HAL_StatusTypeDef status = HAL_OK; 00481 uint32_t readvalue = 0; 00482 uint32_t writevalue = 0xFFFFFFFF; 00483 00484 status = HAL_SPI_TransmitReceive(&hnucleo_Spi, (uint8_t*) &writevalue, (uint8_t*) &readvalue, 1, SpixTimeout); 00485 00486 /* Check the communication status */ 00487 if(status != HAL_OK) 00488 { 00489 /* Execute user timeout callback */ 00490 SPIx_Error(); 00491 } 00492 00493 return readvalue; 00494 } 00495 00496 /** 00497 * @brief SPI Write a byte to device 00498 * @param Value: value to be written 00499 * @retval None 00500 */ 00501 static void SPIx_Write(uint8_t Value) 00502 { 00503 HAL_StatusTypeDef status = HAL_OK; 00504 00505 status = HAL_SPI_Transmit(&hnucleo_Spi, (uint8_t*) &Value, 1, SpixTimeout); 00506 00507 /* Check the communication status */ 00508 if(status != HAL_OK) 00509 { 00510 /* Execute user timeout callback */ 00511 SPIx_Error(); 00512 } 00513 } 00514 00515 /** 00516 * @brief SPI error treatment function 00517 * @retval None 00518 */ 00519 static void SPIx_Error (void) 00520 { 00521 /* De-initialize the SPI communication BUS */ 00522 HAL_SPI_DeInit(&hnucleo_Spi); 00523 00524 /* Re-Initiaize the SPI communication BUS */ 00525 SPIx_Init(); 00526 } 00527 00528 /****************************************************************************** 00529 LINK OPERATIONS 00530 *******************************************************************************/ 00531 00532 /********************************* LINK SD ************************************/ 00533 /** 00534 * @brief Initializes the SD Card and put it into StandBy State (Ready for 00535 * data transfer). 00536 * @retval None 00537 */ 00538 void SD_IO_Init(void) 00539 { 00540 GPIO_InitTypeDef GPIO_InitStruct; 00541 uint8_t counter; 00542 00543 /* SD_CS_GPIO Periph clock enable */ 00544 SD_CS_GPIO_CLK_ENABLE(); 00545 00546 /* Configure SD_CS_PIN pin: SD Card CS pin */ 00547 GPIO_InitStruct.Pin = SD_CS_PIN; 00548 GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; 00549 GPIO_InitStruct.Pull = GPIO_PULLUP; 00550 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00551 HAL_GPIO_Init(SD_CS_GPIO_PORT, &GPIO_InitStruct); 00552 00553 /*------------Put SD in SPI mode--------------*/ 00554 /* SD SPI Config */ 00555 SPIx_Init(); 00556 00557 /* SD chip select high */ 00558 SD_CS_HIGH(); 00559 00560 /* Send dummy byte 0xFF, 10 times with CS high */ 00561 /* Rise CS and MOSI for 80 clocks cycles */ 00562 for (counter = 0; counter <= 9; counter++) 00563 { 00564 /* Send dummy byte 0xFF */ 00565 SD_IO_WriteByte(SD_DUMMY_BYTE); 00566 } 00567 } 00568 00569 /** 00570 * @brief Writes a byte on the SD. 00571 * @param Data: byte to send. 00572 * @retval None 00573 */ 00574 void SD_IO_WriteByte(uint8_t Data) 00575 { 00576 /* Send the byte */ 00577 SPIx_Write(Data); 00578 } 00579 00580 /** 00581 * @brief Reads a byte from the SD. 00582 * @retval The received byte. 00583 */ 00584 uint8_t SD_IO_ReadByte(void) 00585 { 00586 uint8_t data = 0; 00587 00588 /* Get the received data */ 00589 data = SPIx_Read(); 00590 00591 /* Return the shifted data */ 00592 return data; 00593 } 00594 00595 /** 00596 * @brief Sends 5 bytes command to the SD card and get response 00597 * @param Cmd: The user expected command to send to SD card. 00598 * @param Arg: The command argument. 00599 * @param Crc: The CRC. 00600 * @param Response: Expected response from the SD card 00601 * @retval HAL_StatusTypeDef HAL Status 00602 */ 00603 HAL_StatusTypeDef SD_IO_WriteCmd(uint8_t Cmd, uint32_t Arg, uint8_t Crc, uint8_t Response) 00604 { 00605 uint32_t counter = 0x00; 00606 uint8_t frame[6]; 00607 00608 /* Prepare Frame to send */ 00609 frame[0] = (Cmd | 0x40); /* Construct byte 1 */ 00610 frame[1] = (uint8_t)(Arg >> 24); /* Construct byte 2 */ 00611 frame[2] = (uint8_t)(Arg >> 16); /* Construct byte 3 */ 00612 frame[3] = (uint8_t)(Arg >> 8); /* Construct byte 4 */ 00613 frame[4] = (uint8_t)(Arg); /* Construct byte 5 */ 00614 frame[5] = (Crc); /* Construct byte 6 */ 00615 00616 /* SD chip select low */ 00617 SD_CS_LOW(); 00618 00619 /* Send Frame */ 00620 for (counter = 0; counter < 6; counter++) 00621 { 00622 SD_IO_WriteByte(frame[counter]); /* Send the Cmd bytes */ 00623 } 00624 00625 if(Response != SD_NO_RESPONSE_EXPECTED) 00626 { 00627 return SD_IO_WaitResponse(Response); 00628 } 00629 00630 return HAL_OK; 00631 } 00632 00633 /** 00634 * @brief Waits response from the SD card 00635 * @param Response: Expected response from the SD card 00636 * @retval HAL_StatusTypeDef HAL Status 00637 */ 00638 HAL_StatusTypeDef SD_IO_WaitResponse(uint8_t Response) 00639 { 00640 uint32_t timeout = 0xFFFF; 00641 00642 /* Check if response is got or a timeout is happen */ 00643 while ((SD_IO_ReadByte() != Response) && timeout) 00644 { 00645 timeout--; 00646 } 00647 00648 if (timeout == 0) 00649 { 00650 /* After time out */ 00651 return HAL_TIMEOUT; 00652 } 00653 else 00654 { 00655 /* Right response got */ 00656 return HAL_OK; 00657 } 00658 } 00659 00660 /** 00661 * @brief Sends dummy byte with CS High 00662 * @retval None 00663 */ 00664 void SD_IO_WriteDummy(void) 00665 { 00666 /* SD chip select high */ 00667 SD_CS_HIGH(); 00668 00669 /* Send Dummy byte 0xFF */ 00670 SD_IO_WriteByte(SD_DUMMY_BYTE); 00671 } 00672 00673 /********************************* LINK LCD ***********************************/ 00674 /** 00675 * @brief Initializes the LCD 00676 * @retval None 00677 */ 00678 void LCD_IO_Init(void) 00679 { 00680 GPIO_InitTypeDef GPIO_InitStruct; 00681 00682 /* LCD_CS_GPIO and LCD_DC_GPIO Periph clock enable */ 00683 LCD_CS_GPIO_CLK_ENABLE(); 00684 LCD_DC_GPIO_CLK_ENABLE(); 00685 00686 /* Configure LCD_CS_PIN pin: LCD Card CS pin */ 00687 GPIO_InitStruct.Pin = LCD_CS_PIN; 00688 GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; 00689 GPIO_InitStruct.Pull = GPIO_NOPULL; 00690 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00691 HAL_GPIO_Init(LCD_CS_GPIO_PORT, &GPIO_InitStruct); 00692 00693 /* Configure LCD_DC_PIN pin: LCD Card DC pin */ 00694 GPIO_InitStruct.Pin = LCD_DC_PIN; 00695 HAL_GPIO_Init(LCD_DC_GPIO_PORT, &GPIO_InitStruct); 00696 00697 /* LCD chip select high */ 00698 LCD_CS_HIGH(); 00699 00700 /* LCD SPI Config */ 00701 SPIx_Init(); 00702 } 00703 00704 /** 00705 * @brief Writes command to select the LCD register. 00706 * @param LCDReg: Address of the selected register. 00707 * @retval None 00708 */ 00709 void LCD_IO_WriteReg(uint8_t LCDReg) 00710 { 00711 /* Reset LCD control line CS */ 00712 LCD_CS_LOW(); 00713 00714 /* Set LCD data/command line DC to Low */ 00715 LCD_DC_LOW(); 00716 00717 /* Send Command */ 00718 SPIx_Write(LCDReg); 00719 00720 /* Deselect : Chip Select high */ 00721 LCD_CS_HIGH(); 00722 } 00723 00724 /** 00725 * @brief Writes data to select the LCD register. 00726 * This function must be used after st7735_WriteReg() function 00727 * @param Data: data to write to the selected register. 00728 * @retval None 00729 */ 00730 void LCD_IO_WriteData(uint8_t Data) 00731 { 00732 /* Reset LCD control line CS */ 00733 LCD_CS_LOW(); 00734 00735 /* Set LCD data/command line DC to High */ 00736 LCD_DC_HIGH(); 00737 00738 /* Send Data */ 00739 SPIx_Write(Data); 00740 00741 /* Deselect : Chip Select high */ 00742 LCD_CS_HIGH(); 00743 } 00744 00745 /** 00746 * @brief Write register value. 00747 * @param pData Pointer on the register value 00748 * @param Size Size of byte to transmit to the register 00749 * @retval None 00750 */ 00751 void LCD_IO_WriteMultipleData(uint8_t *pData, uint32_t Size) 00752 { 00753 uint32_t counter = 0; 00754 00755 /* Reset LCD control line CS */ 00756 LCD_CS_LOW(); 00757 00758 /* Set LCD data/command line DC to High */ 00759 LCD_DC_HIGH(); 00760 00761 if (Size == 1) 00762 { 00763 /* Only 1 byte to be sent to LCD - general interface can be used */ 00764 /* Send Data */ 00765 SPIx_Write(*pData); 00766 } 00767 else 00768 { 00769 /* Several data should be sent in a raw */ 00770 /* Direct SPI accesses for optimization */ 00771 for (counter = Size; counter != 0; counter--) 00772 { 00773 while(((hnucleo_Spi.Instance->SR) & SPI_FLAG_TXE) != SPI_FLAG_TXE) 00774 { 00775 } 00776 /* Need to invert bytes for LCD*/ 00777 *((__IO uint8_t*)&hnucleo_Spi.Instance->DR) = *(pData+1); 00778 00779 while(((hnucleo_Spi.Instance->SR) & SPI_FLAG_TXE) != SPI_FLAG_TXE) 00780 { 00781 } 00782 *((__IO uint8_t*)&hnucleo_Spi.Instance->DR) = *pData; 00783 counter--; 00784 pData += 2; 00785 } 00786 00787 /* Wait until the bus is ready before releasing Chip select */ 00788 while(((hnucleo_Spi.Instance->SR) & SPI_FLAG_BSY) != RESET) 00789 { 00790 } 00791 } 00792 /* Deselect : Chip Select high */ 00793 LCD_CS_HIGH(); 00794 } 00795 00796 /** 00797 * @brief Wait for loop in ms. 00798 * @param Delay in ms. 00799 * @retval None 00800 */ 00801 void LCD_Delay(uint32_t Delay) 00802 { 00803 HAL_Delay(Delay); 00804 } 00805 #endif /* HAL_SPI_MODULE_ENABLED */ 00806 00807 /******************************* LINK JOYSTICK ********************************/ 00808 #ifdef HAL_ADC_MODULE_ENABLED 00809 /** 00810 * @brief Initializes ADC MSP. 00811 * @param hadc: ADC peripheral 00812 * @retval None 00813 */ 00814 static void ADCx_MspInit(ADC_HandleTypeDef *hadc) 00815 { 00816 GPIO_InitTypeDef GPIO_InitStruct; 00817 00818 /*** Configure the GPIOs ***/ 00819 /* Enable GPIO clock */ 00820 NUCLEO_ADCx_GPIO_CLK_ENABLE(); 00821 00822 /* Configure ADC1 Channel8 as analog input */ 00823 GPIO_InitStruct.Pin = NUCLEO_ADCx_GPIO_PIN ; 00824 GPIO_InitStruct.Mode = GPIO_MODE_ANALOG; 00825 HAL_GPIO_Init(NUCLEO_ADCx_GPIO_PORT, &GPIO_InitStruct); 00826 00827 /*** Configure the ADC peripheral ***/ 00828 /* Enable ADC clock */ 00829 NUCLEO_ADCx_CLK_ENABLE(); 00830 } 00831 00832 /** 00833 * @brief Initializes ADC HAL. 00834 * @retval None 00835 */ 00836 static void ADCx_Init(void) 00837 { 00838 if(HAL_ADC_GetState(&hnucleo_Adc) == HAL_ADC_STATE_RESET) 00839 { 00840 /* ADC Config */ 00841 hnucleo_Adc.Instance = NUCLEO_ADCx; 00842 hnucleo_Adc.Init.OversamplingMode = DISABLE; 00843 hnucleo_Adc.Init.ClockPrescaler = ADC_CLOCK_SYNC_PCLK_DIV2; /* (must not exceed 16MHz) */ 00844 hnucleo_Adc.Init.LowPowerAutoPowerOff = DISABLE; 00845 hnucleo_Adc.Init.LowPowerFrequencyMode = ENABLE; 00846 hnucleo_Adc.Init.LowPowerAutoWait = ENABLE; 00847 hnucleo_Adc.Init.Resolution = ADC_RESOLUTION_12B; 00848 hnucleo_Adc.Init.SamplingTime = ADC_SAMPLETIME_1CYCLE_5; 00849 hnucleo_Adc.Init.ScanConvMode = ADC_SCAN_DIRECTION_FORWARD; 00850 hnucleo_Adc.Init.DataAlign = ADC_DATAALIGN_RIGHT; 00851 hnucleo_Adc.Init.ContinuousConvMode = DISABLE; 00852 hnucleo_Adc.Init.DiscontinuousConvMode = DISABLE; 00853 hnucleo_Adc.Init.ExternalTrigConvEdge = ADC_EXTERNALTRIGCONVEDGE_NONE; 00854 hnucleo_Adc.Init.EOCSelection = ADC_EOC_SINGLE_CONV; 00855 hnucleo_Adc.Init.DMAContinuousRequests = DISABLE; 00856 00857 ADCx_MspInit(&hnucleo_Adc); 00858 HAL_ADC_Init(&hnucleo_Adc); 00859 } 00860 } 00861 00862 /** 00863 * @brief Configures joystick available on adafruit 1.8" TFT shield 00864 * managed through ADC to detect motion. 00865 * @retval Joystickstatus (0=> success, 1=> fail) 00866 */ 00867 uint8_t BSP_JOY_Init(void) 00868 { 00869 uint8_t status = 1; 00870 00871 ADCx_Init(); 00872 00873 /* Start ADC calibration */ 00874 HAL_ADCEx_Calibration_Start(&hnucleo_Adc, ADC_SINGLE_ENDED); 00875 00876 /* Select Channel 0 to be converted */ 00877 sConfig.Channel = ADC_CHANNEL_8; 00878 status = HAL_ADC_ConfigChannel(&hnucleo_Adc, &sConfig); 00879 00880 /* Return Joystick initialization status */ 00881 return status; 00882 } 00883 00884 /** 00885 * @brief Returns the Joystick key pressed. 00886 * @note To know which Joystick key is pressed we need to detect the voltage 00887 * level on each key output 00888 * - None : 3.3 V / 4095 00889 * - SEL : 1.055 V / 1308 00890 * - DOWN : 0.71 V / 88 00891 * - LEFT : 3.0 V / 3720 00892 * - RIGHT : 0.595 V / 737 00893 * - UP : 1.65 V / 2046 00894 * @retval JOYState_TypeDef: Code of the Joystick key pressed. 00895 */ 00896 JOYState_TypeDef BSP_JOY_GetState(void) 00897 { 00898 JOYState_TypeDef state; 00899 uint16_t KeyConvertedValue = 0; 00900 00901 /* Start the conversion process */ 00902 HAL_ADC_Start(&hnucleo_Adc); 00903 00904 /* Wait for the end of conversion */ 00905 if (HAL_ADC_PollForConversion(&hnucleo_Adc, 10) != HAL_TIMEOUT) 00906 { 00907 /* Get the converted value of regular channel */ 00908 KeyConvertedValue = HAL_ADC_GetValue(&hnucleo_Adc); 00909 } 00910 00911 if((KeyConvertedValue > 2010) && (KeyConvertedValue < 2090)) 00912 { 00913 state = JOY_UP; 00914 } 00915 else if((KeyConvertedValue > 680) && (KeyConvertedValue < 780)) 00916 { 00917 state = JOY_RIGHT; 00918 } 00919 else if((KeyConvertedValue > 1270) && (KeyConvertedValue < 1350)) 00920 { 00921 state = JOY_SEL; 00922 } 00923 else if((KeyConvertedValue > 50) && (KeyConvertedValue < 130)) 00924 { 00925 state = JOY_DOWN; 00926 } 00927 else if((KeyConvertedValue > 3570) && (KeyConvertedValue < 3800)) 00928 { 00929 state = JOY_LEFT; 00930 } 00931 else 00932 { 00933 state = JOY_NONE; 00934 } 00935 00936 /* Loop while a key is pressed */ 00937 if(state != JOY_NONE) 00938 { 00939 KeyConvertedValue = HAL_ADC_GetValue(&hnucleo_Adc); 00940 } 00941 /* Return the code of the Joystick key pressed */ 00942 return state; 00943 } 00944 #endif /* HAL_ADC_MODULE_ENABLED */ 00945 00946 /** 00947 * @} 00948 */ 00949 00950 /** 00951 * @} 00952 */ 00953 00954 /** 00955 * @} 00956 */ 00957 00958 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Mon Aug 28 2017 14:48:51 for STM32L0xx_Nucleo_32 BSP User Manual by
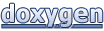