STM32L0538-Discovery BSP User Manual
|
stm32l0538_discovery.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l0538_discovery.h 00004 * @author MCD Application Team 00005 * @brief This file contains definitions for STM32L0538-DISCO Kit Leds, push- 00006 * buttons hardware resources. 00007 ****************************************************************************** 00008 * @attention 00009 * 00010 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00011 * 00012 * Redistribution and use in source and binary forms, with or without modification, 00013 * are permitted provided that the following conditions are met: 00014 * 1. Redistributions of source code must retain the above copyright notice, 00015 * this list of conditions and the following disclaimer. 00016 * 2. Redistributions in binary form must reproduce the above copyright notice, 00017 * this list of conditions and the following disclaimer in the documentation 00018 * and/or other materials provided with the distribution. 00019 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00020 * may be used to endorse or promote products derived from this software 00021 * without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00024 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00025 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00026 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00027 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00028 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00029 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00030 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00031 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00032 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 * 00034 ****************************************************************************** 00035 */ 00036 00037 /* Define to prevent recursive inclusion -------------------------------------*/ 00038 #ifndef __STM32L0538_DISCOVERY_H 00039 #define __STM32L0538_DISCOVERY_H 00040 00041 #ifdef __cplusplus 00042 extern "C" { 00043 #endif 00044 00045 /* Includes ------------------------------------------------------------------*/ 00046 #include "stm32l0xx_hal.h" 00047 00048 /** @addtogroup BSP 00049 * @{ 00050 */ 00051 00052 /** @addtogroup STM32L0538_DISCOVERY 00053 * @{ 00054 */ 00055 00056 /** @addtogroup STM32L0538_DISCOVERY_LOW_LEVEL 00057 * @{ 00058 */ 00059 00060 /** @defgroup STM32L0538_DISCOVERY_LOW_LEVEL_Exported_Types 00061 * @{ 00062 */ 00063 typedef enum 00064 { 00065 LED3 = 0, 00066 LED4 = 1 00067 } Led_TypeDef; 00068 00069 typedef enum 00070 { 00071 BUTTON_KEY = 0 00072 } Button_TypeDef; 00073 00074 typedef enum 00075 { 00076 BUTTON_MODE_GPIO = 0, 00077 BUTTON_MODE_EXTI = 1 00078 } ButtonMode_TypeDef; 00079 00080 /** 00081 * @} 00082 */ 00083 00084 /** @defgroup STM32L0538_DISCOVERY_LOW_LEVEL_Exported_Constants 00085 * @{ 00086 */ 00087 00088 /** 00089 * @brief Define for STM32L0538_DISCO board 00090 */ 00091 #if !defined (USE_STM32L0538_DISCO) 00092 #define USE_STM32L0538_DISCO 00093 #endif 00094 00095 /** @addtogroup STM32L0538_DISCOVERY_LOW_LEVEL_LED 00096 * @{ 00097 */ 00098 #define LEDn 2 00099 00100 #define LED3_PIN GPIO_PIN_4 00101 #define LED3_GPIO_PORT GPIOB 00102 #define LED3_GPIO_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00103 #define LED3_GPIO_CLK_DISABLE() __HAL_RCC_GPIOB_CLK_DISABLE() 00104 00105 #define LED4_PIN GPIO_PIN_5 00106 #define LED4_GPIO_PORT GPIOA 00107 #define LED4_GPIO_CLK_ENABLE() __HAL_RCC_GPIOA_CLK_ENABLE() 00108 #define LED4_GPIO_CLK_DISABLE() __HAL_RCC_GPIOA_CLK_DISABLE() 00109 00110 #define LEDx_GPIO_CLK_ENABLE(__INDEX__) do {if(__INDEX__ == 0) LED3_GPIO_CLK_ENABLE(); else LED4_GPIO_CLK_ENABLE();} while (0) 00111 #define LEDx_GPIO_CLK_DISABLE(__INDEX__) (((__INDEX__) == 0) ? LED3_GPIO_CLK_DISABLE() : LED4_GPIO_CLK_DISABLE()) 00112 00113 /** 00114 * @} 00115 */ 00116 00117 /** @addtogroup STM32L0538_DISCOVERY_LOW_LEVEL_BUTTON 00118 * @{ 00119 */ 00120 #define BUTTONn 1 00121 00122 /** 00123 * @brief Wakeup push-button 00124 */ 00125 #define KEY_BUTTON_PIN GPIO_PIN_0 00126 #define KEY_BUTTON_GPIO_PORT GPIOA 00127 #define KEY_BUTTON_GPIO_CLK_ENABLE() __HAL_RCC_GPIOA_CLK_ENABLE() 00128 #define KEY_BUTTON_GPIO_CLK_DISABLE() __HAL_RCC_GPIOA_CLK_DISABLE() 00129 #define KEY_BUTTON_EXTI_LINE GPIO_PIN_0 00130 #define KEY_BUTTON_EXTI_IRQn EXTI0_1_IRQn 00131 00132 #define BUTTONx_GPIO_CLK_ENABLE(__INDEX__) do {KEY_BUTTON_GPIO_CLK_ENABLE();} while(0) 00133 #define BUTTONx_GPIO_CLK_DISABLE(__INDEX__) (KEY_BUTTON_GPIO_CLK_DISABLE()) 00134 /** 00135 * @} 00136 */ 00137 00138 /*############################### SPI1 #######################################*/ 00139 #define DISCOVERY_SPIx SPI1 00140 #define DISCOVERY_SPIx_CLK_ENABLE() __HAL_RCC_SPI1_CLK_ENABLE() 00141 #define DISCOVERY_SPIx_GPIO_PORT GPIOB /* GPIOB */ 00142 #define DISCOVERY_SPIx_AF GPIO_AF0_SPI1 00143 00144 #define DISCOVERY_SPIx_GPIO_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00145 #define DISCOVERY_SPIx_GPIO_CLK_DISABLE() __HAL_RCC_GPIOB_CLK_DISABLE() 00146 00147 #define DISCOVERY_SPIx_SCK_PIN GPIO_PIN_3 /* PB.03 */ 00148 #define DISCOVERY_SPIx_MISO_PIN GPIO_PIN_4 /* PB.04 */ 00149 #define DISCOVERY_SPIx_MOSI_PIN GPIO_PIN_5 /* PB.05 */ 00150 00151 /* Maximum Timeout values for flags waiting loops. These timeouts are not based 00152 on accurate values, they just guarantee that the application will not remain 00153 stuck if the SPI communication is corrupted. 00154 You may modify these timeout values depending on CPU frequency and application 00155 conditions (interrupts routines ...). */ 00156 #define SPIx_TIMEOUT_MAX ((uint32_t)0x1000) 00157 00158 /*################################ EPD #######################################*/ 00159 /* Chip Select macro definition */ 00160 #define EPD_CS_LOW() HAL_GPIO_WritePin(EPD_CS_GPIO_PORT, EPD_CS_PIN, GPIO_PIN_RESET) 00161 #define EPD_CS_HIGH() HAL_GPIO_WritePin(EPD_CS_GPIO_PORT, EPD_CS_PIN, GPIO_PIN_SET) 00162 00163 /* EPD Data/Command macro definition */ 00164 #define EPD_DC_LOW() HAL_GPIO_WritePin(EPD_DC_GPIO_PORT, EPD_DC_PIN, GPIO_PIN_RESET) 00165 #define EPD_DC_HIGH() HAL_GPIO_WritePin(EPD_DC_GPIO_PORT, EPD_DC_PIN, GPIO_PIN_SET) 00166 00167 /* EPD Reset macro definition */ 00168 #define EPD_RESET_LOW() HAL_GPIO_WritePin(EPD_RESET_GPIO_PORT, EPD_RESET_PIN, GPIO_PIN_RESET) 00169 #define EPD_RESET_HIGH() HAL_GPIO_WritePin(EPD_RESET_GPIO_PORT, EPD_RESET_PIN, GPIO_PIN_SET) 00170 00171 /* EPD PWR macro definition */ 00172 #define EPD_PWR_LOW() HAL_GPIO_WritePin(EPD_PWR_GPIO_PORT, EPD_PWR_PIN, GPIO_PIN_RESET) 00173 #define EPD_PWR_HIGH() HAL_GPIO_WritePin(EPD_PWR_GPIO_PORT, EPD_PWR_PIN, GPIO_PIN_SET) 00174 00175 /** 00176 * @brief EPD Control pin 00177 */ 00178 #define EPD_CS_PIN GPIO_PIN_15 00179 #define EPD_CS_GPIO_PORT GPIOA 00180 #define EPD_CS_GPIO_CLK_ENABLE() __HAL_RCC_GPIOA_CLK_ENABLE() 00181 #define EPD_CS_GPIO_CLK_DISABLE() __HAL_RCC_GPIOA_CLK_DISABLE() 00182 00183 /** 00184 * @brief EPD Command/data pin 00185 */ 00186 #define EPD_DC_PIN GPIO_PIN_11 00187 #define EPD_DC_GPIO_PORT GPIOB 00188 #define EPD_DC_GPIO_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00189 #define EPD_DC_GPIO_CLK_DISABLE() __HAL_RCC_GPIOB_CLK_DISABLE() 00190 00191 /** 00192 * @brief EPD Reset pin 00193 */ 00194 #define EPD_RESET_PIN GPIO_PIN_2 00195 #define EPD_RESET_GPIO_PORT GPIOB 00196 #define EPD_RESET_GPIO_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00197 #define EPD_RESET_GPIO_CLK_DISABLE() __HAL_RCC_GPIOB_CLK_DISABLE() 00198 00199 /** 00200 * @brief EPD Busy pin 00201 */ 00202 #define EPD_BUSY_PIN GPIO_PIN_8 00203 #define EPD_BUSY_GPIO_PORT GPIOA 00204 #define EPD_BUSY_GPIO_CLK_ENABLE() __HAL_RCC_GPIOA_CLK_ENABLE() 00205 #define EPD_BUSY_GPIO_CLK_DISABLE() __HAL_RCC_GPIOA_CLK_DISABLE() 00206 00207 /** 00208 * @brief EPD PWR pin 00209 */ 00210 #define EPD_PWR_PIN GPIO_PIN_10 00211 #define EPD_PWR_GPIO_PORT GPIOB 00212 #define EPD_PWR_GPIO_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00213 #define EPD_PWR_GPIO_CLK_DISABLE() __HAL_RCC_GPIOB_CLK_DISABLE() 00214 /** 00215 * @} 00216 */ 00217 00218 /** @defgroup STM32L0538_DISCOVERY LOW_LEVEL_Exported_Macros 00219 * @{ 00220 */ 00221 /** 00222 * @} 00223 */ 00224 00225 /** @defgroup STM32L0538_DISCOVERY LOW_LEVEL_Exported_Functions 00226 * @{ 00227 */ 00228 uint32_t BSP_GetVersion(void); 00229 void BSP_LED_Init(Led_TypeDef Led); 00230 void BSP_LED_On(Led_TypeDef Led); 00231 void BSP_LED_Off(Led_TypeDef Led); 00232 void BSP_LED_Toggle(Led_TypeDef Led); 00233 void BSP_PB_Init(Button_TypeDef Button, ButtonMode_TypeDef ButtonMode); 00234 uint32_t BSP_PB_GetState(Button_TypeDef Button); 00235 00236 #ifdef __cplusplus 00237 } 00238 #endif 00239 00240 #endif /* __STM32L0538_DISCOVERY_H */ 00241 00242 /** 00243 * @} 00244 */ 00245 00246 /** 00247 * @} 00248 */ 00249 00250 /** 00251 * @} 00252 */ 00253 00254 /** 00255 * @} 00256 */ 00257 00258 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Mon Aug 28 2017 14:51:54 for STM32L0538-Discovery BSP User Manual by
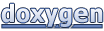