STM32L0538-Discovery BSP User Manual
|
stm32l0538_discovery.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l0538_discovery.c 00004 * @author MCD Application Team 00005 * @brief This file provides set of firmware functions to manage Leds and 00006 * push-button available on STM32L0538-DISCO Kit from STMicroelectronics. 00007 ****************************************************************************** 00008 * @attention 00009 * 00010 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00011 * 00012 * Redistribution and use in source and binary forms, with or without modification, 00013 * are permitted provided that the following conditions are met: 00014 * 1. Redistributions of source code must retain the above copyright notice, 00015 * this list of conditions and the following disclaimer. 00016 * 2. Redistributions in binary form must reproduce the above copyright notice, 00017 * this list of conditions and the following disclaimer in the documentation 00018 * and/or other materials provided with the distribution. 00019 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00020 * may be used to endorse or promote products derived from this software 00021 * without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00024 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00025 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00026 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00027 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00028 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00029 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00030 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00031 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00032 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 * 00034 ****************************************************************************** 00035 */ 00036 00037 /* Includes ------------------------------------------------------------------*/ 00038 #include "stm32l0538_discovery.h" 00039 00040 /** @addtogroup BSP 00041 * @{ 00042 */ 00043 00044 /** @addtogroup STM32L0538_DISCOVERY 00045 * @{ 00046 */ 00047 00048 /** @defgroup STM32L0538_DISCOVERY_LOW_LEVEL 00049 * @brief This file provides set of firmware functions to manage Leds and push-button 00050 * available on STM32L0538-Discovery Kit from STMicroelectronics. 00051 * @{ 00052 */ 00053 00054 /** @defgroup STM32L0538_DISCOVERY_LOW_LEVEL_Private_TypesDefinitions 00055 * @{ 00056 */ 00057 /** 00058 * @} 00059 */ 00060 00061 /** @defgroup STM32L0538_DISCOVERY_LOW_LEVEL_Private_Defines 00062 * @{ 00063 */ 00064 00065 /** 00066 * @brief STM32L0538 DISCOVERY BSP Driver version number 00067 */ 00068 #define __STM32L0538_DISCO_BSP_VERSION_MAIN (0x01) /*!< [31:24] main version */ 00069 #define __STM32L0538_DISCO_BSP_VERSION_SUB1 (0x02) /*!< [23:16] sub1 version */ 00070 #define __STM32L0538_DISCO_BSP_VERSION_SUB2 (0x03) /*!< [15:8] sub2 version */ 00071 #define __STM32L0538_DISCO_BSP_VERSION_RC (0x00) /*!< [7:0] release candidate */ 00072 #define __STM32L0538_DISCO_BSP_VERSION ((__STM32L0538_DISCO_BSP_VERSION_MAIN << 24)\ 00073 |(__STM32L0538_DISCO_BSP_VERSION_SUB1 << 16)\ 00074 |(__STM32L0538_DISCO_BSP_VERSION_SUB2 << 8 )\ 00075 |(__STM32L0538_DISCO_BSP_VERSION_RC)) 00076 00077 /** 00078 * @} 00079 */ 00080 00081 /** @defgroup STM32L0538-DISCOVERY LOW_LEVEL_Private_Macros 00082 * @{ 00083 */ 00084 /** 00085 * @} 00086 */ 00087 00088 /** @defgroup STM32L0538_DISCOVERY LOW_LEVEL_Private_Variables 00089 * @{ 00090 */ 00091 GPIO_TypeDef* GPIO_PORT[LEDn] = {LED3_GPIO_PORT, 00092 LED4_GPIO_PORT}; 00093 00094 const uint16_t GPIO_PIN[LEDn] = {LED3_PIN, 00095 LED4_PIN}; 00096 00097 GPIO_TypeDef* BUTTON_PORT[BUTTONn] = {KEY_BUTTON_GPIO_PORT}; 00098 const uint16_t BUTTON_PIN[BUTTONn] = {KEY_BUTTON_PIN}; 00099 const uint8_t BUTTON_IRQn[BUTTONn] = {KEY_BUTTON_EXTI_IRQn}; 00100 00101 static SPI_HandleTypeDef SpiHandle; 00102 /** 00103 * @} 00104 */ 00105 00106 /** @defgroup STM32L0538_DISCOVERY LOW_LEVEL_Private_FunctionPrototypes 00107 * @{ 00108 */ 00109 static void SPIx_Init(void); 00110 static void SPIx_Write(uint8_t Value); 00111 static uint32_t SPIx_Read(void); 00112 static void SPIx_Error(void); 00113 static void SPIx_MspInit(SPI_HandleTypeDef *hspi); 00114 00115 /* Link functions for EPD peripheral */ 00116 void EPD_IO_Init(void); 00117 void EPD_IO_WriteData(uint16_t RegValue); 00118 void EPD_IO_WriteReg(uint8_t Reg); 00119 uint16_t EPD_IO_ReadData(void); 00120 void EPD_Delay(uint32_t delay); 00121 /** 00122 * @} 00123 */ 00124 00125 /** @defgroup STM32L0538_DISCOVERY LOW_LEVEL_Private_Functions 00126 * @{ 00127 */ 00128 00129 /** 00130 * @brief This method returns the STM32L0538 DISCO BSP Driver revision 00131 * @param None 00132 * @retval version: 0xXYZR (8bits for each decimal, R for RC) 00133 */ 00134 uint32_t BSP_GetVersion(void) 00135 { 00136 return __STM32L0538_DISCO_BSP_VERSION; 00137 } 00138 00139 /** 00140 * @brief Configures LED GPIO. 00141 * @param Led: Specifies the Led to be configured. 00142 * This parameter can be one of following parameters: 00143 * @arg LED3 00144 * @arg LED4 00145 * @retval None 00146 */ 00147 void BSP_LED_Init(Led_TypeDef Led) 00148 { 00149 GPIO_InitTypeDef GPIO_InitStruct; 00150 00151 /* Enable the GPIO_LED Clock */ 00152 LEDx_GPIO_CLK_ENABLE(Led); 00153 00154 /* Configure the GPIO_LED pin */ 00155 GPIO_InitStruct.Pin = GPIO_PIN[Led]; 00156 GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; 00157 GPIO_InitStruct.Pull = GPIO_PULLUP; 00158 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 00159 00160 HAL_GPIO_Init(GPIO_PORT[Led], &GPIO_InitStruct); 00161 00162 HAL_GPIO_WritePin(GPIO_PORT[Led], GPIO_PIN[Led], GPIO_PIN_RESET); 00163 } 00164 00165 /** 00166 * @brief Turns selected LED On. 00167 * @param Led: Specifies the Led to be set on. 00168 * This parameter can be one of following parameters: 00169 * @arg LED3 00170 * @arg LED4 00171 * @retval None 00172 */ 00173 void BSP_LED_On(Led_TypeDef Led) 00174 { 00175 HAL_GPIO_WritePin(GPIO_PORT[Led], GPIO_PIN[Led], GPIO_PIN_SET); 00176 } 00177 00178 /** 00179 * @brief Turns selected LED Off. 00180 * @param Led: Specifies the Led to be set off. 00181 * This parameter can be one of following parameters: 00182 * @arg LED3 00183 * @arg LED4 00184 * @retval None 00185 */ 00186 void BSP_LED_Off(Led_TypeDef Led) 00187 { 00188 HAL_GPIO_WritePin(GPIO_PORT[Led], GPIO_PIN[Led], GPIO_PIN_RESET); 00189 } 00190 00191 /** 00192 * @brief Toggles the selected LED. 00193 * @param Led: Specifies the Led to be toggled. 00194 * This parameter can be one of following parameters: 00195 * @arg LED3 00196 * @arg LED4 00197 * @retval None 00198 */ 00199 void BSP_LED_Toggle(Led_TypeDef Led) 00200 { 00201 HAL_GPIO_TogglePin(GPIO_PORT[Led], GPIO_PIN[Led]); 00202 } 00203 00204 /** 00205 * @brief Configures Button GPIO and EXTI Line. 00206 * @param Button: Specifies the Button to be configured. 00207 * This parameter should be: BUTTON_KEY 00208 * @param Button_Mode: Specifies Button mode. 00209 * This parameter can be one of following parameters: 00210 * @arg BUTTON_MODE_GPIO: Button will be used as simple IO 00211 * @arg BUTTON_MODE_EXTI: Button will be connected to EXTI line with interrupt 00212 * generation capability 00213 * @retval None 00214 */ 00215 void BSP_PB_Init(Button_TypeDef Button, ButtonMode_TypeDef ButtonMode) 00216 { 00217 GPIO_InitTypeDef GPIO_InitStruct; 00218 00219 /* Enable the BUTTON Clock */ 00220 BUTTONx_GPIO_CLK_ENABLE(Button); 00221 00222 if (ButtonMode == BUTTON_MODE_GPIO) 00223 { 00224 /* Configure Button pin as input */ 00225 GPIO_InitStruct.Pin = BUTTON_PIN[Button]; 00226 GPIO_InitStruct.Mode = GPIO_MODE_INPUT; 00227 GPIO_InitStruct.Pull = GPIO_PULLDOWN; 00228 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 00229 HAL_GPIO_Init(BUTTON_PORT[Button], &GPIO_InitStruct); 00230 } 00231 00232 if (ButtonMode == BUTTON_MODE_EXTI) 00233 { 00234 /* Configure Button pin as input with External interrupt */ 00235 GPIO_InitStruct.Pin = BUTTON_PIN[Button]; 00236 GPIO_InitStruct.Pull = GPIO_NOPULL; 00237 GPIO_InitStruct.Mode = GPIO_MODE_IT_FALLING; 00238 HAL_GPIO_Init(BUTTON_PORT[Button], &GPIO_InitStruct); 00239 00240 /* Enable and set Button EXTI Interrupt to the lowest priority */ 00241 HAL_NVIC_SetPriority((IRQn_Type)(BUTTON_IRQn[Button]), 3, 0); 00242 HAL_NVIC_EnableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00243 } 00244 } 00245 00246 /** 00247 * @brief Returns the selected Button state. 00248 * @param Button: Specifies the Button to be checked. 00249 * This parameter should be: BUTTON_KEY 00250 * @retval The Button GPIO pin value. 00251 */ 00252 uint32_t BSP_PB_GetState(Button_TypeDef Button) 00253 { 00254 return HAL_GPIO_ReadPin(BUTTON_PORT[Button], BUTTON_PIN[Button]); 00255 } 00256 00257 /******************************************************************************* 00258 BUS OPERATIONS 00259 *******************************************************************************/ 00260 00261 /******************************* SPI Routines *********************************/ 00262 /** 00263 * @brief SPIx Bus initialization 00264 * @param None 00265 * @retval None 00266 */ 00267 static void SPIx_Init(void) 00268 { 00269 if(HAL_SPI_GetState(&SpiHandle) == HAL_SPI_STATE_RESET) 00270 { 00271 /* SPI Config */ 00272 SpiHandle.Instance = DISCOVERY_SPIx; 00273 00274 /* On STM32L0538-DISCO, EPD ID cannot be read then keep a common configuration */ 00275 /* for EPD (SPI_DIRECTION_2LINES) */ 00276 /* Note: To read a register a EPD, SPI_DIRECTION_1LINE should be set */ 00277 SpiHandle.Init.Mode = SPI_MODE_MASTER; 00278 SpiHandle.Init.Direction = SPI_DIRECTION_2LINES; 00279 SpiHandle.Init.BaudRatePrescaler = SPI_BAUDRATEPRESCALER_8; 00280 SpiHandle.Init.DataSize = SPI_DATASIZE_8BIT; 00281 SpiHandle.Init.CLKPhase = SPI_PHASE_2EDGE; 00282 SpiHandle.Init.CLKPolarity = SPI_POLARITY_HIGH; 00283 SpiHandle.Init.FirstBit = SPI_FIRSTBIT_MSB; 00284 SpiHandle.Init.NSS = SPI_NSS_SOFT; 00285 SpiHandle.Init.CRCCalculation = SPI_CRCCALCULATION_DISABLE; 00286 SpiHandle.Init.CRCPolynomial = 7; 00287 SpiHandle.Init.TIMode = SPI_TIMODE_DISABLE; 00288 00289 SPIx_MspInit(&SpiHandle); 00290 HAL_SPI_Init(&SpiHandle); 00291 } 00292 } 00293 00294 /** 00295 * @brief SPI Read 4 bytes from device. 00296 * @param ReadSize Number of bytes to read (max 4 bytes) 00297 * @retval Value read on the SPI 00298 */ 00299 static uint32_t SPIx_Read(void) 00300 { 00301 HAL_StatusTypeDef status = HAL_OK; 00302 uint32_t readvalue = 0; 00303 uint32_t writevalue = 0xFFFFFFFF; 00304 00305 status = HAL_SPI_TransmitReceive(&SpiHandle, (uint8_t*) &writevalue, (uint8_t*) &readvalue, 1, SPIx_TIMEOUT_MAX); 00306 00307 /* Check the communication status */ 00308 if(status != HAL_OK) 00309 { 00310 /* Re-Initiaize the BUS */ 00311 SPIx_Error(); 00312 } 00313 00314 return readvalue; 00315 } 00316 00317 /** 00318 * @brief SPI Write a byte to device. 00319 * @param Value: value to be written 00320 * @retval None 00321 */ 00322 static void SPIx_Write(uint8_t Value) 00323 { 00324 HAL_StatusTypeDef status = HAL_OK; 00325 00326 status = HAL_SPI_Transmit(&SpiHandle, (uint8_t*) &Value, 1, SPIx_TIMEOUT_MAX); 00327 00328 /* Check the communication status */ 00329 if(status != HAL_OK) 00330 { 00331 /* Re-Initiaize the BUS */ 00332 SPIx_Error(); 00333 } 00334 } 00335 00336 /** 00337 * @brief SPI error treatment function. 00338 * @param None 00339 * @retval None 00340 */ 00341 static void SPIx_Error (void) 00342 { 00343 /* De-Initialize the SPI comunication BUS */ 00344 HAL_SPI_DeInit(&SpiHandle); 00345 00346 /* Re-Initiaize the SPI comunication BUS */ 00347 SPIx_Init(); 00348 } 00349 00350 /** 00351 * @brief SPI MSP Init 00352 * @param hspi: SPI handle 00353 * @retval None 00354 */ 00355 static void SPIx_MspInit(SPI_HandleTypeDef *hspi) 00356 { 00357 GPIO_InitTypeDef GPIO_InitStruct; 00358 00359 /*** Configure the GPIOs ***/ 00360 /* Enable GPIO clock */ 00361 DISCOVERY_SPIx_GPIO_CLK_ENABLE(); 00362 00363 /* Configure SPI SCK */ 00364 GPIO_InitStruct.Pin = DISCOVERY_SPIx_SCK_PIN; 00365 GPIO_InitStruct.Mode = GPIO_MODE_AF_PP; 00366 GPIO_InitStruct.Pull = GPIO_PULLUP; 00367 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00368 GPIO_InitStruct.Alternate = DISCOVERY_SPIx_AF; 00369 HAL_GPIO_Init(DISCOVERY_SPIx_GPIO_PORT, &GPIO_InitStruct); 00370 00371 /* Configure SPI MOSI */ 00372 GPIO_InitStruct.Pin = DISCOVERY_SPIx_MOSI_PIN; 00373 GPIO_InitStruct.Alternate = DISCOVERY_SPIx_AF; 00374 GPIO_InitStruct.Pull = GPIO_PULLDOWN; 00375 HAL_GPIO_Init(DISCOVERY_SPIx_GPIO_PORT, &GPIO_InitStruct); 00376 00377 /*** Configure the SPI peripheral ***/ 00378 /* Enable SPI clock */ 00379 DISCOVERY_SPIx_CLK_ENABLE(); 00380 } 00381 00382 /********************************* LINK EPD ***********************************/ 00383 00384 /** 00385 * @brief Configures the EPD SPI interface. 00386 * @param None 00387 * @retval None 00388 */ 00389 void EPD_IO_Init(void) 00390 { 00391 GPIO_InitTypeDef GPIO_InitStruct; 00392 00393 /* EPD_CS_GPIO and EPD_DC_GPIO Periph clock enable */ 00394 EPD_CS_GPIO_CLK_ENABLE(); 00395 EPD_DC_GPIO_CLK_ENABLE(); 00396 EPD_RESET_GPIO_CLK_ENABLE(); 00397 EPD_BUSY_GPIO_CLK_ENABLE(); 00398 EPD_PWR_GPIO_CLK_ENABLE(); 00399 00400 /* Configure EPD_CS_PIN pin: EPD Card CS pin */ 00401 GPIO_InitStruct.Pin = EPD_CS_PIN; 00402 GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; 00403 GPIO_InitStruct.Pull = GPIO_NOPULL; 00404 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00405 HAL_GPIO_Init(EPD_CS_GPIO_PORT, &GPIO_InitStruct); 00406 00407 /* Configure EPD_DC_PIN pin: EPD Card DC pin */ 00408 GPIO_InitStruct.Pin = EPD_DC_PIN; 00409 HAL_GPIO_Init(EPD_DC_GPIO_PORT, &GPIO_InitStruct); 00410 00411 /* Configure EPD_RESET_PIN pin */ 00412 GPIO_InitStruct.Pin = EPD_RESET_PIN; 00413 HAL_GPIO_Init(EPD_PWR_GPIO_PORT, &GPIO_InitStruct); 00414 00415 /* Configure EPD_RESET_PIN pin */ 00416 GPIO_InitStruct.Pin = EPD_PWR_PIN; 00417 HAL_GPIO_Init(EPD_RESET_GPIO_PORT, &GPIO_InitStruct); 00418 00419 /* Configure EPD_BUSY_PIN pin */ 00420 GPIO_InitStruct.Pin = EPD_BUSY_PIN; 00421 GPIO_InitStruct.Mode = GPIO_MODE_INPUT; 00422 GPIO_InitStruct.Pull = GPIO_PULLDOWN; 00423 HAL_GPIO_Init(EPD_BUSY_GPIO_PORT, &GPIO_InitStruct); 00424 00425 /* Enbale Display */ 00426 EPD_PWR_LOW(); 00427 00428 /* Set or Reset the control line */ 00429 EPD_CS_LOW(); 00430 EPD_CS_HIGH(); 00431 00432 /* EPD reset pin mamagement */ 00433 EPD_RESET_HIGH(); 00434 EPD_Delay(10); 00435 00436 /* SPI Configuration */ 00437 SPIx_Init(); 00438 } 00439 00440 /** 00441 * @brief Write register value. 00442 * @param None 00443 * @retval None 00444 */ 00445 void EPD_IO_WriteData(uint16_t RegValue) 00446 { 00447 /* Reset EPD control line CS */ 00448 EPD_CS_LOW(); 00449 00450 /* Set EPD data/command line DC to High */ 00451 EPD_DC_HIGH(); 00452 00453 /* Send Data */ 00454 SPIx_Write(RegValue); 00455 00456 /* Deselect: Chip Select high */ 00457 EPD_CS_HIGH(); 00458 } 00459 00460 /** 00461 * @brief Writes command to selected EPD register. 00462 * @param Reg: Address of the selected register. 00463 * @retval None 00464 */ 00465 void EPD_IO_WriteReg(uint8_t Reg) 00466 { 00467 /* Reset EPD control line CS */ 00468 EPD_CS_LOW(); 00469 00470 /* Set EPD data/command line DC to Low */ 00471 EPD_DC_LOW(); 00472 00473 /* Send Command */ 00474 SPIx_Write(Reg); 00475 00476 /* Deselect: Chip Select high */ 00477 EPD_CS_HIGH(); 00478 } 00479 00480 /** 00481 * @brief Reads data from EPD data register. 00482 * @param None 00483 * @retval Read data. 00484 */ 00485 uint16_t EPD_IO_ReadData(void) 00486 { 00487 /* Reset EPD control line CS */ 00488 EPD_CS_LOW(); 00489 00490 /* Deselect: Chip Select high */ 00491 EPD_CS_HIGH(); 00492 00493 /* Send Data */ 00494 return SPIx_Read(); 00495 } 00496 00497 /** 00498 * @brief Wait for loop in ms. 00499 * @param Delay in ms. 00500 * @retval None 00501 */ 00502 void EPD_Delay (uint32_t Delay) 00503 { 00504 HAL_Delay (Delay); 00505 } 00506 00507 /** 00508 * @} 00509 */ 00510 00511 /** 00512 * @} 00513 */ 00514 00515 /** 00516 * @} 00517 */ 00518 00519 /** 00520 * @} 00521 */ 00522 00523 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Mon Aug 28 2017 14:51:54 for STM32L0538-Discovery BSP User Manual by
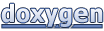