STM32H7xx_Nucleo_144 BSP User Manual
|
stm32h7xx_nucleo_144.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32h7xx_nucleo_144.h 00004 * @author MCD Application Team 00005 * @version V1.0.0 00006 * @date 21-April-2017 00007 * @brief This file contains definitions for: 00008 * - LEDs and push-button available on STM32H7xx-Nucleo-144 Kit 00009 * from STMicroelectronics 00010 * - LCD, joystick and microSD available on Adafruit 1.8" TFT LCD 00011 * shield (reference ID 802) 00012 ****************************************************************************** 00013 * @attention 00014 * 00015 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00016 * 00017 * Redistribution and use in source and binary forms, with or without modification, 00018 * are permitted provided that the following conditions are met: 00019 * 1. Redistributions of source code must retain the above copyright notice, 00020 * this list of conditions and the following disclaimer. 00021 * 2. Redistributions in binary form must reproduce the above copyright notice, 00022 * this list of conditions and the following disclaimer in the documentation 00023 * and/or other materials provided with the distribution. 00024 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00025 * may be used to endorse or promote products derived from this software 00026 * without specific prior written permission. 00027 * 00028 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00029 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00030 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00031 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00032 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00033 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00034 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00035 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00036 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00037 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00038 * 00039 ****************************************************************************** 00040 */ 00041 00042 /* Define to prevent recursive inclusion -------------------------------------*/ 00043 #ifndef __STM32H7xx_NUCLEO_144_H 00044 #define __STM32H7xx_NUCLEO_144_H 00045 00046 #ifdef __cplusplus 00047 extern "C" { 00048 #endif 00049 00050 /* Includes ------------------------------------------------------------------*/ 00051 #include "stm32h7xx_hal.h" 00052 00053 /* To be defined only if the board is provided with the related shield */ 00054 /* https://www.adafruit.com/products/802 */ 00055 #ifndef ADAFRUIT_TFT_JOY_SD_ID802 00056 #define ADAFRUIT_TFT_JOY_SD_ID802 00057 #endif 00058 00059 /** @defgroup BSP BSP Nucleo 144 00060 * @{ 00061 */ 00062 00063 /** @defgroup STM32H7xx_NUCLEO_144 STM32H7xx NUCLEO 144 00064 * @{ 00065 */ 00066 00067 /** @defgroup STM32H7xx_NUCLEO_144_Exported_Types Exported Types 00068 * @{ 00069 */ 00070 typedef enum 00071 { 00072 LED1 = 0, 00073 LED_GREEN = LED1, 00074 LED2 = 1, 00075 LED_BLUE = LED2, 00076 LED3 = 2, 00077 LED_RED = LED3 00078 }Led_TypeDef; 00079 00080 typedef enum 00081 { 00082 BUTTON_USER = 0, 00083 /* Alias */ 00084 BUTTON_KEY = BUTTON_USER 00085 }Button_TypeDef; 00086 00087 typedef enum 00088 { 00089 BUTTON_MODE_GPIO = 0, 00090 BUTTON_MODE_EXTI = 1 00091 }ButtonMode_TypeDef; 00092 00093 typedef enum 00094 { 00095 JOY_NONE = 0, 00096 JOY_SEL = 1, 00097 JOY_DOWN = 2, 00098 JOY_LEFT = 3, 00099 JOY_RIGHT = 4, 00100 JOY_UP = 5 00101 }JOYState_TypeDef; 00102 00103 /** 00104 * @} 00105 */ 00106 00107 /** @defgroup STM32H7xx_NUCLEO_144_Exported_Constants Exported Constants 00108 * @{ 00109 */ 00110 00111 /** 00112 * @brief Define for STM32H7xx_NUCLEO_144 board 00113 */ 00114 #if !defined (USE_STM32H7xx_NUCLEO_144) 00115 #define USE_STM32H7xx_NUCLEO_144 00116 #endif 00117 00118 /** @defgroup STM32H7xx_NUCLEO_144_LED NUCLEO_144 LED 00119 * @{ 00120 */ 00121 #define LEDn 3 00122 00123 #define LED1_PIN GPIO_PIN_0 00124 #define LED1_GPIO_PORT GPIOB 00125 #define LED1_GPIO_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00126 #define LED1_GPIO_CLK_DISABLE() __HAL_RCC_GPIOB_CLK_DISABLE() 00127 00128 #define LED2_PIN GPIO_PIN_7 00129 #define LED2_GPIO_PORT GPIOB 00130 #define LED2_GPIO_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00131 #define LED2_GPIO_CLK_DISABLE() __HAL_RCC_GPIOB_CLK_DISABLE() 00132 00133 #define LED3_PIN GPIO_PIN_14 00134 #define LED3_GPIO_PORT GPIOB 00135 #define LED3_GPIO_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00136 #define LED3_GPIO_CLK_DISABLE() __HAL_RCC_GPIOB_CLK_DISABLE() 00137 00138 #define LEDx_GPIO_CLK_ENABLE(__INDEX__) do { if((__INDEX__) == 0) {__HAL_RCC_GPIOB_CLK_ENABLE();} else\ 00139 {__HAL_RCC_GPIOB_CLK_ENABLE(); }} while(0) 00140 #define LEDx_GPIO_CLK_DISABLE(__INDEX__) do { if((__INDEX__) == 0) {__HAL_RCC_GPIOB_CLK_DISABLE();} else\ 00141 {__HAL_RCC_GPIOB_CLK_DISABLE(); }} while(0) 00142 /** 00143 * @} 00144 */ 00145 00146 /** @defgroup STM32H7xx_NUCLEO_144_BUTTON NUCLEO_144 BUTTON 00147 * @{ 00148 */ 00149 #define BUTTONn 1 00150 00151 /** 00152 * @brief Key push-button 00153 */ 00154 #define USER_BUTTON_PIN GPIO_PIN_13 00155 #define USER_BUTTON_GPIO_PORT GPIOC 00156 #define USER_BUTTON_GPIO_CLK_ENABLE() __HAL_RCC_GPIOC_CLK_ENABLE() 00157 #define USER_BUTTON_GPIO_CLK_DISABLE() __HAL_RCC_GPIOC_CLK_DISABLE() 00158 #define USER_BUTTON_EXTI_LINE GPIO_PIN_13 00159 #define USER_BUTTON_EXTI_IRQn EXTI15_10_IRQn 00160 00161 #define BUTTONx_GPIO_CLK_ENABLE(__INDEX__) USER_BUTTON_GPIO_CLK_ENABLE() 00162 #define BUTTONx_GPIO_CLK_DISABLE(__INDEX__) USER_BUTTON_GPIO_CLK_DISABLE() 00163 00164 /* Aliases */ 00165 #define KEY_BUTTON_PIN USER_BUTTON_PIN 00166 #define KEY_BUTTON_GPIO_PORT USER_BUTTON_GPIO_PORT 00167 #define KEY_BUTTON_GPIO_CLK_ENABLE() USER_BUTTON_GPIO_CLK_ENABLE() 00168 #define KEY_BUTTON_GPIO_CLK_DISABLE() USER_BUTTON_GPIO_CLK_DISABLE() 00169 #define KEY_BUTTON_EXTI_LINE USER_BUTTON_EXTI_LINE 00170 #define KEY_BUTTON_EXTI_IRQn USER_BUTTON_EXTI_IRQn 00171 /** 00172 * @} 00173 */ 00174 00175 /** @defgroup STM32H7xx_NUCLEO_144_USB_PINS NUCLEO_144 USB Pins definition 00176 * @{ 00177 */ 00178 /** 00179 * @brief USB Pins definition 00180 */ 00181 #define OTG_FS1_OVER_CURRENT_PIN GPIO_PIN_7 00182 #define OTG_FS1_OVER_CURRENT_PORT GPIOG 00183 #define OTG_FS1_OVER_CURRENT_PORT_CLK_ENABLE() __HAL_RCC_GPIOG_CLK_ENABLE() 00184 00185 #define OTG_FS1_POWER_SWITCH_PIN GPIO_PIN_6 00186 #define OTG_FS1_POWER_SWITCH_PORT GPIOG 00187 #define OTG_FS1_POWER_SWITCH_PORT_CLK_ENABLE() __HAL_RCC_GPIOG_CLK_ENABLE() 00188 /** 00189 * @} 00190 */ 00191 00192 /** @defgroup STM32H7xx_NUCLEO_144_LOW_LEVEL_BUS Low Level Bus 00193 * @{ 00194 */ 00195 /*############################### SPI_A #######################################*/ 00196 #ifdef HAL_SPI_MODULE_ENABLED 00197 00198 #define NUCLEO_SPIx SPI1 00199 #define NUCLEO_SPIx_CLK_ENABLE() __HAL_RCC_SPI1_CLK_ENABLE() 00200 00201 #define NUCLEO_SPIx_SCK_AF GPIO_AF5_SPI1 00202 #define NUCLEO_SPIx_SCK_GPIO_PORT GPIOA 00203 #define NUCLEO_SPIx_SCK_PIN GPIO_PIN_5 00204 #define NUCLEO_SPIx_SCK_GPIO_CLK_ENABLE() __HAL_RCC_GPIOA_CLK_ENABLE() 00205 #define NUCLEO_SPIx_SCK_GPIO_CLK_DISABLE() __HAL_RCC_GPIOA_CLK_DISABLE() 00206 00207 #define NUCLEO_SPIx_MISO_MOSI_AF GPIO_AF5_SPI1 00208 #define NUCLEO_SPIx_MISO_MOSI_GPIO_PORT GPIOA 00209 #define NUCLEO_SPIx_MISO_MOSI_GPIO_CLK_ENABLE() __HAL_RCC_GPIOA_CLK_ENABLE() 00210 #define NUCLEO_SPIx_MISO_MOSI_GPIO_CLK_DISABLE() __HAL_RCC_GPIOA_CLK_DISABLE() 00211 #define NUCLEO_SPIx_MISO_PIN GPIO_PIN_6 00212 #define NUCLEO_SPIx_MOSI_PIN GPIO_PIN_7 00213 /* Maximum Timeout values for flags waiting loops. These timeouts are not based 00214 on accurate values, they just guarantee that the application will not remain 00215 stuck if the SPI communication is corrupted. 00216 You may modify these timeout values depending on CPU frequency and application 00217 conditions (interrupts routines ...). */ 00218 #define NUCLEO_SPIx_TIMEOUT_MAX 1000 00219 00220 #define NUCLEO_SPIx_CS_GPIO_PORT GPIOD 00221 #define NUCLEO_SPIx_CS_PIN GPIO_PIN_14 00222 #define NUCLEO_SPIx_CS_GPIO_CLK_ENABLE() __HAL_RCC_GPIOD_CLK_ENABLE() 00223 #define NUCLEO_SPIx_CS_GPIO_CLK_DISABLE() __HAL_RCC_GPIOD_CLK_DISABLE() 00224 00225 #define SPIx__CS_LOW() HAL_GPIO_WritePin(NUCLEO_SPIx_CS_GPIO_PORT, NUCLEO_SPIx_CS_PIN, GPIO_PIN_RESET) 00226 #define SPIx__CS_HIGH() HAL_GPIO_WritePin(NUCLEO_SPIx_CS_GPIO_PORT, NUCLEO_SPIx_CS_PIN, GPIO_PIN_SET) 00227 00228 /** 00229 * @brief SD Control Lines management 00230 */ 00231 #define SD_CS_LOW() HAL_GPIO_WritePin(SD_CS_GPIO_PORT, SD_CS_PIN, GPIO_PIN_RESET) 00232 #define SD_CS_HIGH() HAL_GPIO_WritePin(SD_CS_GPIO_PORT, SD_CS_PIN, GPIO_PIN_SET) 00233 00234 /** 00235 * @brief LCD Control Lines management 00236 */ 00237 #define LCD_CS_LOW() HAL_GPIO_WritePin(LCD_CS_GPIO_PORT, LCD_CS_PIN, GPIO_PIN_RESET) 00238 #define LCD_CS_HIGH() HAL_GPIO_WritePin(LCD_CS_GPIO_PORT, LCD_CS_PIN, GPIO_PIN_SET) 00239 #define LCD_DC_LOW() HAL_GPIO_WritePin(LCD_DC_GPIO_PORT, LCD_DC_PIN, GPIO_PIN_RESET) 00240 #define LCD_DC_HIGH() HAL_GPIO_WritePin(LCD_DC_GPIO_PORT, LCD_DC_PIN, GPIO_PIN_SET) 00241 00242 /** 00243 * @brief SD Control Interface pins (shield D4) 00244 */ 00245 #define SD_CS_PIN GPIO_PIN_14 00246 #define SD_CS_GPIO_PORT GPIOF 00247 #define SD_CS_GPIO_CLK_ENABLE() __HAL_RCC_GPIOF_CLK_ENABLE() 00248 #define SD_CS_GPIO_CLK_DISABLE() __HAL_RCC_GPIOF_CLK_DISABLE() 00249 00250 /** 00251 * @brief LCD Control Interface pins (shield D10) 00252 */ 00253 #define LCD_CS_PIN GPIO_PIN_14 00254 #define LCD_CS_GPIO_PORT GPIOD 00255 #define LCD_CS_GPIO_CLK_ENABLE() __HAL_RCC_GPIOD_CLK_ENABLE() 00256 #define LCD_CS_GPIO_CLK_DISABLE() __HAL_RCC_GPIOD_CLK_DISABLE() 00257 00258 /** 00259 * @brief LCD Data/Command Interface pins (shield D8) 00260 */ 00261 #define LCD_DC_PIN GPIO_PIN_12 00262 #define LCD_DC_GPIO_PORT GPIOF 00263 #define LCD_DC_GPIO_CLK_ENABLE() __HAL_RCC_GPIOF_CLK_ENABLE() 00264 #define LCD_DC_GPIO_CLK_DISABLE() __HAL_RCC_GPIOF_CLK_DISABLE() 00265 00266 #endif /* HAL_SPI_MODULE_ENABLED */ 00267 00268 /*################################ ADC3 ######################################*/ 00269 /** 00270 * @brief ADC Interface pins 00271 * used to detect motion of Joystick available on Adafruit 1.8" TFT shield 00272 */ 00273 00274 #ifdef HAL_ADC_MODULE_ENABLED 00275 00276 #define NUCLEO_ADCx ADC3 00277 #define NUCLEO_ADCx_CLK_ENABLE() __HAL_RCC_ADC3_CLK_ENABLE() 00278 #define NUCLEO_ADCx_CLK_DISABLE() __HAL_RCC_ADC3_CLK_DISABLE() 00279 00280 #define NUCLEO_ADCx_CHANNEL ADC_CHANNEL_5 00281 00282 #define NUCLEO_ADCx_GPIO_PORT GPIOF 00283 #define NUCLEO_ADCx_GPIO_PIN GPIO_PIN_3 00284 #define NUCLEO_ADCx_GPIO_CLK_ENABLE() __HAL_RCC_GPIOF_CLK_ENABLE() 00285 #define NUCLEO_ADCx_GPIO_CLK_DISABLE() __HAL_RCC_GPIOF_CLK_DISABLE() 00286 00287 #endif /* HAL_ADC_MODULE_ENABLED */ 00288 00289 /** 00290 * @} 00291 */ 00292 00293 /** 00294 * @} 00295 */ 00296 00297 /** @defgroup STM32H7xx_NUCLEO_144_LOW_LEVEL_Exported_Macros LOW LEVEL Exported Macros 00298 * @{ 00299 */ 00300 /** 00301 * @} 00302 */ 00303 00304 /** @defgroup STM32H7xx_NUCLEO_144_Exported_Functions Exported Functions 00305 * @{ 00306 */ 00307 uint32_t BSP_GetVersion(void); 00308 void BSP_LED_Init(Led_TypeDef Led); 00309 void BSP_LED_DeInit(Led_TypeDef Led); 00310 void BSP_LED_On(Led_TypeDef Led); 00311 void BSP_LED_Off(Led_TypeDef Led); 00312 void BSP_LED_Toggle(Led_TypeDef Led); 00313 void BSP_PB_Init(Button_TypeDef Button, ButtonMode_TypeDef ButtonMode); 00314 void BSP_PB_DeInit(Button_TypeDef Button); 00315 uint32_t BSP_PB_GetState(Button_TypeDef Button); 00316 #ifdef HAL_ADC_MODULE_ENABLED 00317 uint8_t BSP_JOY_Init(void); 00318 JOYState_TypeDef BSP_JOY_GetState(void); 00319 void BSP_JOY_DeInit(void); 00320 #endif /* HAL_ADC_MODULE_ENABLED */ 00321 /** 00322 * @} 00323 */ 00324 00325 /** 00326 * @} 00327 */ 00328 00329 /** 00330 * @} 00331 */ 00332 00333 #ifdef __cplusplus 00334 } 00335 #endif 00336 00337 #endif /* __STM32H7xx_NUCLEO_144_H */ 00338 00339 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri Apr 21 2017 17:27:08 for STM32H7xx_Nucleo_144 BSP User Manual by
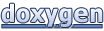