STM32H7xx_Nucleo_144 BSP User Manual
|
stm32h7xx_nucleo_144.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32h7xx_nucleo_144.c 00004 * @author MCD Application Team 00005 * @version V1.0.0 00006 * @date 21-April-2017 00007 * @brief This file provides set of firmware functions to manage: 00008 * - LEDs and push-button available on STM32H7XX-Nucleo-144 Kit 00009 * from STMicroelectronics 00010 * - LCD, joystick and microSD available on Adafruit 1.8" TFT LCD 00011 * shield (reference ID 802) 00012 ****************************************************************************** 00013 * @attention 00014 * 00015 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00016 * 00017 * Redistribution and use in source and binary forms, with or without modification, 00018 * are permitted provided that the following conditions are met: 00019 * 1. Redistributions of source code must retain the above copyright notice, 00020 * this list of conditions and the following disclaimer. 00021 * 2. Redistributions in binary form must reproduce the above copyright notice, 00022 * this list of conditions and the following disclaimer in the documentation 00023 * and/or other materials provided with the distribution. 00024 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00025 * may be used to endorse or promote products derived from this software 00026 * without specific prior written permission. 00027 * 00028 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00029 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00030 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00031 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00032 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00033 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00034 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00035 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00036 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00037 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00038 * 00039 ****************************************************************************** 00040 */ 00041 00042 /* Includes ------------------------------------------------------------------*/ 00043 #include "stm32h7xx_nucleo_144.h" 00044 00045 00046 /** @addtogroup BSP 00047 * @{ 00048 */ 00049 00050 /** @addtogroup STM32H7xx_NUCLEO_144 00051 * @{ 00052 */ 00053 00054 /** @defgroup STM32H7xx_NUCLEO_144_LOW_LEVEL_Private_TypesDefinitions LOW LEVEL Private Types 00055 * @{ 00056 */ 00057 /** 00058 * @} 00059 */ 00060 00061 00062 /** @defgroup STM32H7xx_NUCLEO_144_LOW_LEVEL_Private_Defines LOW LEVEL Private Defines 00063 * @{ 00064 */ 00065 00066 /** 00067 * @brief STM32H7xx NUCLEO BSP Driver version number V1.0.0 00068 */ 00069 #define __STM32H7xx_NUCLEO_BSP_VERSION_MAIN (0x01) /*!< [31:24] main version */ 00070 #define __STM32H7xx_NUCLEO_BSP_VERSION_SUB1 (0x00) /*!< [23:16] sub1 version */ 00071 #define __STM32H7xx_NUCLEO_BSP_VERSION_SUB2 (0x00) /*!< [15:8] sub2 version */ 00072 #define __STM32H7xx_NUCLEO_BSP_VERSION_RC (0x00) /*!< [7:0] release candidate */ 00073 #define __STM32H7xx_NUCLEO_BSP_VERSION ((__STM32H7xx_NUCLEO_BSP_VERSION_MAIN << 24)\ 00074 |(__STM32H7xx_NUCLEO_BSP_VERSION_SUB1 << 16)\ 00075 |(__STM32H7xx_NUCLEO_BSP_VERSION_SUB2 << 8 )\ 00076 |(__STM32H7xx_NUCLEO_BSP_VERSION_RC)) 00077 00078 /** 00079 * @brief LINK SD Card 00080 */ 00081 #define SD_DUMMY_BYTE 0xFF 00082 #define SD_NO_RESPONSE_EXPECTED 0x80 00083 00084 /** 00085 * @} 00086 */ 00087 00088 /** @defgroup STM32H7xx_NUCLEO_144_LOW_LEVEL_Private_Macros LOW LEVEL Private Macros 00089 * @{ 00090 */ 00091 /** 00092 * @} 00093 */ 00094 00095 /** @defgroup STM32H7xx_NUCLEO_144_LOW_LEVEL_Private_Variables LOW LEVEL Private Variables 00096 * @{ 00097 */ 00098 GPIO_TypeDef* GPIO_PORT[LEDn] = {LED1_GPIO_PORT, LED2_GPIO_PORT, LED3_GPIO_PORT}; 00099 00100 const uint16_t GPIO_PIN[LEDn] = {LED1_PIN, LED2_PIN, LED3_PIN}; 00101 00102 GPIO_TypeDef* BUTTON_PORT[BUTTONn] = {USER_BUTTON_GPIO_PORT}; 00103 const uint16_t BUTTON_PIN[BUTTONn] = {USER_BUTTON_PIN}; 00104 const uint8_t BUTTON_IRQn[BUTTONn] = {USER_BUTTON_EXTI_IRQn}; 00105 00106 /** 00107 * @brief BUS variables 00108 */ 00109 00110 #ifdef ADAFRUIT_TFT_JOY_SD_ID802 00111 #ifdef HAL_SPI_MODULE_ENABLED 00112 uint32_t SpixTimeout = NUCLEO_SPIx_TIMEOUT_MAX; /*<! Value of Timeout when SPI communication fails */ 00113 static SPI_HandleTypeDef hnucleo_Spi; 00114 #endif /* HAL_SPI_MODULE_ENABLED */ 00115 00116 #ifdef HAL_ADC_MODULE_ENABLED 00117 static ADC_HandleTypeDef hnucleo_Adc; 00118 /* ADC channel configuration structure declaration */ 00119 static ADC_ChannelConfTypeDef sConfig; 00120 #endif /* HAL_ADC_MODULE_ENABLED */ 00121 #endif /* ADAFRUIT_TFT_JOY_SD_ID802 */ 00122 00123 /** 00124 * @} 00125 */ 00126 00127 /** @defgroup STM32H7xx_NUCLEO_144_LOW_LEVEL_Private_Functions LOW LEVEL Private Functions 00128 * @{ 00129 */ 00130 #ifdef ADAFRUIT_TFT_JOY_SD_ID802 00131 00132 #ifdef HAL_SPI_MODULE_ENABLED 00133 static void SPIx_Init(void); 00134 static void SPIx_MspInit(SPI_HandleTypeDef *hspi); 00135 static void SPIx_Write(uint8_t Value); 00136 static void SPIx_WriteReadData(const uint8_t *DataIn, uint8_t *DataOut, uint16_t DataLength); 00137 static void SPIx_Error(void); 00138 00139 /* SD IO functions */ 00140 void SD_IO_Init(void); 00141 void SD_IO_CSState(uint8_t state); 00142 void SD_IO_WriteReadData(const uint8_t *DataIn, uint8_t *DataOut, uint16_t DataLength); 00143 uint8_t SD_IO_WriteByte(uint8_t Data); 00144 00145 /* LCD IO functions */ 00146 void LCD_IO_Init(void); 00147 void LCD_IO_WriteData(uint8_t Data); 00148 void LCD_IO_WriteMultipleData(uint8_t *pData, uint32_t Size); 00149 void LCD_IO_WriteReg(uint8_t LCDReg); 00150 void LCD_Delay(uint32_t delay); 00151 #endif /* HAL_SPI_MODULE_ENABLED */ 00152 00153 #ifdef HAL_ADC_MODULE_ENABLED 00154 static void ADCx_Init(void); 00155 static void ADCx_DeInit(void); 00156 static void ADCx_MspInit(ADC_HandleTypeDef *hadc); 00157 static void ADCx_MspDeInit(ADC_HandleTypeDef *hadc); 00158 #endif /* HAL_ADC_MODULE_ENABLED */ 00159 00160 #endif /* ADAFRUIT_TFT_JOY_SD_ID802 */ 00161 00162 /** 00163 * @} 00164 */ 00165 00166 /** @addtogroup STM32H7xx_NUCLEO_144_Exported_Functions 00167 * @{ 00168 */ 00169 00170 /** 00171 * @brief This method returns the STM32H7xx NUCLEO BSP Driver revision 00172 * @retval version: 0xXYZR (8bits for each decimal, R for RC) 00173 */ 00174 uint32_t BSP_GetVersion(void) 00175 { 00176 return __STM32H7xx_NUCLEO_BSP_VERSION; 00177 } 00178 00179 /** 00180 * @brief Configures LED GPIO. 00181 * @param Led: Specifies the Led to be configured. 00182 * This parameter can be one of following parameters: 00183 * @arg LED1 00184 * @arg LED2 00185 * @arg LED3 00186 * @retval None 00187 */ 00188 void BSP_LED_Init(Led_TypeDef Led) 00189 { 00190 GPIO_InitTypeDef GPIO_InitStruct; 00191 00192 /* Enable the GPIO_LED Clock */ 00193 LEDx_GPIO_CLK_ENABLE(Led); 00194 00195 /* Configure the GPIO_LED pin */ 00196 GPIO_InitStruct.Pin = GPIO_PIN[Led]; 00197 GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; 00198 GPIO_InitStruct.Pull = GPIO_NOPULL; 00199 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00200 00201 HAL_GPIO_Init(GPIO_PORT[Led], &GPIO_InitStruct); 00202 HAL_GPIO_WritePin(GPIO_PORT[Led], GPIO_PIN[Led], GPIO_PIN_RESET); 00203 } 00204 00205 /** 00206 * @brief DeInit LEDs. 00207 * @param Led: LED to be de-init. 00208 * This parameter can be one of the following values: 00209 * @arg LED1 00210 * @arg LED2 00211 * @arg LED3 00212 * @note Led DeInit does not disable the GPIO clock nor disable the Mfx 00213 * @retval None 00214 */ 00215 void BSP_LED_DeInit(Led_TypeDef Led) 00216 { 00217 GPIO_InitTypeDef gpio_init_structure; 00218 00219 /* Turn off LED */ 00220 HAL_GPIO_WritePin(GPIO_PORT[Led], GPIO_PIN[Led], GPIO_PIN_RESET); 00221 /* DeInit the GPIO_LED pin */ 00222 gpio_init_structure.Pin = GPIO_PIN[Led]; 00223 HAL_GPIO_DeInit(GPIO_PORT[Led], gpio_init_structure.Pin); 00224 } 00225 00226 /** 00227 * @brief Turns selected LED On. 00228 * @param Led: Specifies the Led to be set on. 00229 * This parameter can be one of following parameters: 00230 * @arg LED2 00231 * @retval None 00232 */ 00233 void BSP_LED_On(Led_TypeDef Led) 00234 { 00235 HAL_GPIO_WritePin(GPIO_PORT[Led], GPIO_PIN[Led], GPIO_PIN_SET); 00236 } 00237 00238 /** 00239 * @brief Turns selected LED Off. 00240 * @param Led: Specifies the Led to be set off. 00241 * This parameter can be one of following parameters: 00242 * @arg LED1 00243 * @arg LED2 00244 * @arg LED3 00245 * @retval None 00246 */ 00247 void BSP_LED_Off(Led_TypeDef Led) 00248 { 00249 HAL_GPIO_WritePin(GPIO_PORT[Led], GPIO_PIN[Led], GPIO_PIN_RESET); 00250 } 00251 00252 /** 00253 * @brief Toggles the selected LED. 00254 * @param Led: Specifies the Led to be toggled. 00255 * This parameter can be one of following parameters: 00256 * @arg LED1 00257 * @arg LED2 00258 * @arg LED3 00259 * @retval None 00260 */ 00261 void BSP_LED_Toggle(Led_TypeDef Led) 00262 { 00263 HAL_GPIO_TogglePin(GPIO_PORT[Led], GPIO_PIN[Led]); 00264 } 00265 00266 /** 00267 * @brief Configures Button GPIO and EXTI Line. 00268 * @param Button: Specifies the Button to be configured. 00269 * This parameter should be: BUTTON_USER 00270 * @param ButtonMode: Specifies Button mode. 00271 * This parameter can be one of following parameters: 00272 * @arg BUTTON_MODE_GPIO: Button will be used as simple IO 00273 * @arg BUTTON_MODE_EXTI: Button will be connected to EXTI line with interrupt 00274 * generation capability 00275 * @retval None 00276 */ 00277 void BSP_PB_Init(Button_TypeDef Button, ButtonMode_TypeDef ButtonMode) 00278 { 00279 GPIO_InitTypeDef GPIO_InitStruct; 00280 00281 /* Enable the BUTTON Clock */ 00282 BUTTONx_GPIO_CLK_ENABLE(Button); 00283 00284 if(ButtonMode == BUTTON_MODE_GPIO) 00285 { 00286 /* Configure Button pin as input */ 00287 GPIO_InitStruct.Pin = BUTTON_PIN[Button]; 00288 GPIO_InitStruct.Mode = GPIO_MODE_INPUT; 00289 GPIO_InitStruct.Pull = GPIO_PULLDOWN; 00290 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00291 HAL_GPIO_Init(BUTTON_PORT[Button], &GPIO_InitStruct); 00292 } 00293 00294 if(ButtonMode == BUTTON_MODE_EXTI) 00295 { 00296 /* Configure Button pin as input with External interrupt */ 00297 GPIO_InitStruct.Pin = BUTTON_PIN[Button]; 00298 GPIO_InitStruct.Pull = GPIO_NOPULL; 00299 GPIO_InitStruct.Mode = GPIO_MODE_IT_FALLING; 00300 HAL_GPIO_Init(BUTTON_PORT[Button], &GPIO_InitStruct); 00301 00302 /* Enable and set Button EXTI Interrupt to the lowest priority */ 00303 HAL_NVIC_SetPriority((IRQn_Type)(BUTTON_IRQn[Button]), 0x0F, 0x00); 00304 HAL_NVIC_EnableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00305 } 00306 } 00307 00308 /** 00309 * @brief Push Button DeInit. 00310 * @param Button: Button to be configured 00311 * This parameter should be: BUTTON_USER 00312 * @note PB DeInit does not disable the GPIO clock 00313 * @retval None 00314 */ 00315 void BSP_PB_DeInit(Button_TypeDef Button) 00316 { 00317 GPIO_InitTypeDef gpio_init_structure; 00318 00319 gpio_init_structure.Pin = BUTTON_PIN[Button]; 00320 HAL_NVIC_DisableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00321 HAL_GPIO_DeInit(BUTTON_PORT[Button], gpio_init_structure.Pin); 00322 } 00323 00324 /** 00325 * @brief Returns the selected Button state. 00326 * @param Button: Specifies the Button to be checked. 00327 * This parameter should be: BUTTON_USER 00328 * @retval The Button GPIO pin value. 00329 */ 00330 uint32_t BSP_PB_GetState(Button_TypeDef Button) 00331 { 00332 return HAL_GPIO_ReadPin(BUTTON_PORT[Button], BUTTON_PIN[Button]); 00333 } 00334 00335 /** 00336 * @} 00337 */ 00338 00339 /** @addtogroup STM32H7xx_NUCLEO_144_LOW_LEVEL_Private_Functions 00340 * @{ 00341 */ 00342 00343 /****************************************************************************** 00344 BUS OPERATIONS 00345 *******************************************************************************/ 00346 #ifdef ADAFRUIT_TFT_JOY_SD_ID802 00347 00348 /******************************* SPI ********************************/ 00349 #ifdef HAL_SPI_MODULE_ENABLED 00350 00351 /** 00352 * @brief Initializes SPI MSP. 00353 * @retval None 00354 */ 00355 static void SPIx_MspInit(SPI_HandleTypeDef *hspi) 00356 { 00357 GPIO_InitTypeDef GPIO_InitStruct; 00358 RCC_PeriphCLKInitTypeDef SPI_PeriphClkInit; 00359 00360 /*** Configure the GPIOs ***/ 00361 /* Enable GPIO clock */ 00362 NUCLEO_SPIx_SCK_GPIO_CLK_ENABLE(); 00363 NUCLEO_SPIx_MISO_MOSI_GPIO_CLK_ENABLE(); 00364 00365 /* Configure SPI SCK */ 00366 GPIO_InitStruct.Pin = NUCLEO_SPIx_SCK_PIN; 00367 GPIO_InitStruct.Mode = GPIO_MODE_AF_PP; 00368 GPIO_InitStruct.Pull = GPIO_PULLUP; 00369 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00370 GPIO_InitStruct.Alternate = NUCLEO_SPIx_SCK_AF; 00371 HAL_GPIO_Init(NUCLEO_SPIx_SCK_GPIO_PORT, &GPIO_InitStruct); 00372 00373 /* Configure SPI MISO and MOSI */ 00374 GPIO_InitStruct.Pin = NUCLEO_SPIx_MOSI_PIN; 00375 GPIO_InitStruct.Alternate = NUCLEO_SPIx_MISO_MOSI_AF; 00376 GPIO_InitStruct.Pull = GPIO_NOPULL; 00377 HAL_GPIO_Init(NUCLEO_SPIx_MISO_MOSI_GPIO_PORT, &GPIO_InitStruct); 00378 00379 GPIO_InitStruct.Pin = NUCLEO_SPIx_MISO_PIN; 00380 GPIO_InitStruct.Pull = GPIO_NOPULL; 00381 HAL_GPIO_Init(NUCLEO_SPIx_MISO_MOSI_GPIO_PORT, &GPIO_InitStruct); 00382 00383 SPI_PeriphClkInit.PeriphClockSelection = RCC_PERIPHCLK_SPI1; 00384 SPI_PeriphClkInit.Spi1ClockSelection = RCC_SPI2CLKSOURCE_PLL; 00385 HAL_RCCEx_PeriphCLKConfig(&SPI_PeriphClkInit); 00386 00387 /*** Configure the SPI peripheral ***/ 00388 /* Enable SPI clock */ 00389 NUCLEO_SPIx_CLK_ENABLE(); 00390 } 00391 00392 00393 /** 00394 * @brief Initializes SPI HAL. 00395 * @retval None 00396 */ 00397 static void SPIx_Init(void) 00398 { 00399 if(HAL_SPI_GetState(&hnucleo_Spi) == HAL_SPI_STATE_RESET) 00400 { 00401 /* SPI Config */ 00402 hnucleo_Spi.Instance = NUCLEO_SPIx; 00403 /* SPI baudrate is set to 12,5 MHz maximum (PLL1_Q/SPI_BaudRatePrescaler = 200/16 = 12,5 MHz) 00404 to verify these constraints: 00405 - ST7735 LCD SPI interface max baudrate is 15MHz for write and 6.66MHz for read 00406 Since the provided driver doesn't use read capability from LCD, only constraint 00407 on write baudrate is considered. 00408 - SD card SPI interface max baudrate is 25MHz for write/read 00409 */ 00410 hnucleo_Spi.Init.BaudRatePrescaler = SPI_BAUDRATEPRESCALER_16; 00411 hnucleo_Spi.Init.Direction = SPI_DIRECTION_2LINES; 00412 hnucleo_Spi.Init.CLKPhase = SPI_PHASE_2EDGE; 00413 hnucleo_Spi.Init.CLKPolarity = SPI_POLARITY_HIGH; 00414 hnucleo_Spi.Init.CRCCalculation = SPI_CRCCALCULATION_DISABLE; 00415 hnucleo_Spi.Init.CRCPolynomial = 7; 00416 hnucleo_Spi.Init.DataSize = SPI_DATASIZE_8BIT; 00417 hnucleo_Spi.Init.FirstBit = SPI_FIRSTBIT_MSB; 00418 hnucleo_Spi.Init.NSS = SPI_NSS_SOFT; 00419 hnucleo_Spi.Init.TIMode = SPI_TIMODE_DISABLE; 00420 hnucleo_Spi.Init.Mode = SPI_MODE_MASTER; 00421 00422 hnucleo_Spi.Init.FifoThreshold = SPI_FIFO_THRESHOLD_01DATA; 00423 hnucleo_Spi.Init.CRCLength = SPI_CRC_LENGTH_8BIT; 00424 hnucleo_Spi.Init.TxCRCInitializationPattern = SPI_CRC_INITIALIZATION_ALL_ZERO_PATTERN; 00425 hnucleo_Spi.Init.RxCRCInitializationPattern = SPI_CRC_INITIALIZATION_ALL_ZERO_PATTERN; 00426 hnucleo_Spi.Init.NSSPolarity = SPI_NSS_POLARITY_LOW; 00427 hnucleo_Spi.Init.NSSPMode = SPI_NSS_PULSE_DISABLE; 00428 hnucleo_Spi.Init.MasterSSIdleness = 0x00000000; 00429 hnucleo_Spi.Init.MasterInterDataIdleness = 0x00000000; 00430 hnucleo_Spi.Init.MasterReceiverAutoSusp = 0x00000000; 00431 00432 SPIx_MspInit(&hnucleo_Spi); 00433 HAL_SPI_Init(&hnucleo_Spi); 00434 } 00435 } 00436 00437 /** 00438 * @brief SPI Write and Read data 00439 * @param DataIn: Data to write 00440 * @param DataOut: Data to read 00441 * @param DataLength: Data size 00442 * @retval None 00443 */ 00444 static void SPIx_WriteReadData(const uint8_t *DataIn, uint8_t *DataOut, uint16_t DataLength) 00445 { 00446 HAL_StatusTypeDef status = HAL_OK; 00447 00448 status = HAL_SPI_TransmitReceive(&hnucleo_Spi, (uint8_t*) DataIn, DataOut, DataLength, SpixTimeout); 00449 00450 /* Check the communication status */ 00451 if(status != HAL_OK) 00452 { 00453 /* Execute user timeout callback */ 00454 SPIx_Error(); 00455 } 00456 } 00457 00458 /** 00459 * @brief SPI Write a byte to device. 00460 * @param Value: value to be written 00461 * @retval None 00462 */ 00463 static void SPIx_Write(uint8_t Value) 00464 { 00465 HAL_StatusTypeDef status = HAL_OK; 00466 uint8_t data; 00467 00468 status = HAL_SPI_TransmitReceive(&hnucleo_Spi, (uint8_t*) &Value, &data, 1, SpixTimeout); 00469 00470 /* Check the communication status */ 00471 if(status != HAL_OK) 00472 { 00473 /* Execute user timeout callback */ 00474 SPIx_Error(); 00475 } 00476 } 00477 00478 /** 00479 * @brief SPI error treatment function 00480 * @retval None 00481 */ 00482 static void SPIx_Error (void) 00483 { 00484 /* De-initialize the SPI communication BUS */ 00485 HAL_SPI_DeInit(&hnucleo_Spi); 00486 00487 /* Re-Initiaize the SPI communication BUS */ 00488 SPIx_Init(); 00489 } 00490 00491 /****************************************************************************** 00492 LINK OPERATIONS 00493 *******************************************************************************/ 00494 00495 /********************************* LINK SD ************************************/ 00496 /** 00497 * @brief Initializes the SD Card and put it into StandBy State (Ready for 00498 * data transfer). 00499 * @retval None 00500 */ 00501 void SD_IO_Init(void) 00502 { 00503 GPIO_InitTypeDef GPIO_InitStruct; 00504 uint8_t counter; 00505 00506 /* SD_CS_GPIO Periph clock enable */ 00507 SD_CS_GPIO_CLK_ENABLE(); 00508 00509 /* Configure SD_CS_PIN pin: SD Card CS pin */ 00510 GPIO_InitStruct.Pin = SD_CS_PIN; 00511 GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; 00512 GPIO_InitStruct.Pull = GPIO_PULLUP; 00513 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 00514 HAL_GPIO_Init(SD_CS_GPIO_PORT, &GPIO_InitStruct); 00515 00516 00517 /* LCD chip select line perturbs SD also when the LCD is not used */ 00518 /* this is a workaround to avoid sporadic failures during r/w operations */ 00519 LCD_CS_GPIO_CLK_ENABLE(); 00520 GPIO_InitStruct.Pin = LCD_CS_PIN; 00521 GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; 00522 GPIO_InitStruct.Pull = GPIO_NOPULL; 00523 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 00524 HAL_GPIO_Init(LCD_CS_GPIO_PORT, &GPIO_InitStruct); 00525 LCD_CS_HIGH(); 00526 00527 00528 /*------------Put SD in SPI mode--------------*/ 00529 /* SD SPI Config */ 00530 SPIx_Init(); 00531 00532 /* SD chip select high */ 00533 SD_CS_HIGH(); 00534 00535 /* Send dummy byte 0xFF, 10 times with CS high */ 00536 /* Rise CS and MOSI for 80 clocks cycles */ 00537 for (counter = 0; counter <= 9; counter++) 00538 { 00539 /* Send dummy byte 0xFF */ 00540 SD_IO_WriteByte(SD_DUMMY_BYTE); 00541 } 00542 } 00543 00544 /** 00545 * @brief Set the SD_CS pin. 00546 * @param val: SD CS value. 00547 * @retval None 00548 */ 00549 void SD_IO_CSState(uint8_t val) 00550 { 00551 if(val == 1) 00552 { 00553 SD_CS_HIGH(); 00554 } 00555 else 00556 { 00557 SD_CS_LOW(); 00558 } 00559 } 00560 00561 /** 00562 * @brief SD Write and Read data 00563 * @param DataIn: Data to write 00564 * @param DataOut: Data to read 00565 * @param DataLength: Data size 00566 * @retval None 00567 */ 00568 void SD_IO_WriteReadData(const uint8_t *DataIn, uint8_t *DataOut, uint16_t DataLength) 00569 { 00570 /* Send the byte */ 00571 SPIx_WriteReadData(DataIn, DataOut, DataLength); 00572 } 00573 00574 /** 00575 * @brief Writes a byte on the SD. 00576 * @param Data: byte to send. 00577 * @retval None 00578 */ 00579 uint8_t SD_IO_WriteByte(uint8_t Data) 00580 { 00581 uint8_t tmp; 00582 /* Send the byte */ 00583 SPIx_WriteReadData(&Data,&tmp,1); 00584 return tmp; 00585 } 00586 00587 /********************************* LINK LCD ***********************************/ 00588 /** 00589 * @brief Initializes the LCD 00590 * @retval None 00591 */ 00592 void LCD_IO_Init(void) 00593 { 00594 GPIO_InitTypeDef GPIO_InitStruct; 00595 00596 /* LCD_CS_GPIO and LCD_DC_GPIO Periph clock enable */ 00597 LCD_CS_GPIO_CLK_ENABLE(); 00598 LCD_DC_GPIO_CLK_ENABLE(); 00599 00600 /* Configure LCD_CS_PIN pin: LCD Card CS pin */ 00601 GPIO_InitStruct.Pin = LCD_CS_PIN; 00602 GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; 00603 GPIO_InitStruct.Pull = GPIO_NOPULL; 00604 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 00605 HAL_GPIO_Init(LCD_CS_GPIO_PORT, &GPIO_InitStruct); 00606 00607 /* Configure LCD_DC_PIN pin: LCD Card DC pin */ 00608 GPIO_InitStruct.Pin = LCD_DC_PIN; 00609 HAL_GPIO_Init(LCD_DC_GPIO_PORT, &GPIO_InitStruct); 00610 00611 /* LCD chip select high */ 00612 LCD_CS_HIGH(); 00613 00614 /* LCD SPI Config */ 00615 SPIx_Init(); 00616 } 00617 00618 /** 00619 * @brief Writes command to select the LCD register. 00620 * @param LCDReg: Address of the selected register. 00621 * @retval None 00622 */ 00623 void LCD_IO_WriteReg(uint8_t LCDReg) 00624 { 00625 /* Reset LCD control line CS */ 00626 LCD_CS_LOW(); 00627 00628 /* Set LCD data/command line DC to Low */ 00629 LCD_DC_LOW(); 00630 00631 /* Send Command */ 00632 SPIx_Write(LCDReg); 00633 00634 /* Deselect : Chip Select high */ 00635 LCD_CS_HIGH(); 00636 } 00637 00638 /** 00639 * @brief Writes data to select the LCD register. 00640 * This function must be used after st7735_WriteReg() function 00641 * @param Data: data to write to the selected register. 00642 * @retval None 00643 */ 00644 void LCD_IO_WriteData(uint8_t Data) 00645 { 00646 /* Reset LCD control line CS */ 00647 LCD_CS_LOW(); 00648 00649 /* Set LCD data/command line DC to High */ 00650 LCD_DC_HIGH(); 00651 00652 /* Send Data */ 00653 SPIx_Write(Data); 00654 00655 /* Deselect : Chip Select high */ 00656 LCD_CS_HIGH(); 00657 } 00658 00659 /** 00660 * @brief Write register value. 00661 * @param pData Pointer on the register value 00662 * @param Size Size of byte to transmit to the register 00663 * @retval None 00664 */ 00665 void LCD_IO_WriteMultipleData(uint8_t *pData, uint32_t Size) 00666 { 00667 uint32_t counter = 0; 00668 __IO uint32_t data = 0; 00669 00670 /* Reset LCD control line CS */ 00671 LCD_CS_LOW(); 00672 00673 /* Set LCD data/command line DC to High */ 00674 LCD_DC_HIGH(); 00675 00676 if (Size == 1) 00677 { 00678 /* Only 1 byte to be sent to LCD - general interface can be used */ 00679 /* Send Data */ 00680 SPIx_Write(*pData); 00681 } 00682 else 00683 { 00684 00685 /* Enable SPI peripheral */ 00686 __HAL_SPI_ENABLE(&hnucleo_Spi); 00687 00688 /* Reset the SPI Data length and data reload*/ 00689 hnucleo_Spi.Instance->CR2 = 0; 00690 00691 /* Master transfer start */ 00692 SET_BIT(hnucleo_Spi.Instance->CR1, SPI_CR1_CSTART); 00693 00694 /* Several data should be sent in a raw */ 00695 /* Direct SPI accesses for optimization */ 00696 for (counter = Size; counter != 0; counter--) 00697 { 00698 while(((hnucleo_Spi.Instance->SR) & SPI_FLAG_TXE) != SPI_FLAG_TXE) 00699 { 00700 } 00701 /* Need to invert bytes for LCD*/ 00702 *((__IO uint8_t*)&hnucleo_Spi.Instance->TXDR) = *(pData+1); 00703 00704 /* Wait until the bus is ready before releasing Chip select */ 00705 while(((hnucleo_Spi.Instance->SR) & SPI_SR_TXC) == RESET) 00706 { 00707 } 00708 __HAL_SPI_CLEAR_EOTFLAG(&hnucleo_Spi); 00709 /* Empty the Rx fifo */ 00710 data = *(&hnucleo_Spi.Instance->RXDR); 00711 UNUSED(data); /* Remove GNU warning */ 00712 00713 while(((hnucleo_Spi.Instance->SR) & SPI_FLAG_TXE) != SPI_FLAG_TXE) 00714 { 00715 } 00716 *((__IO uint8_t*)&hnucleo_Spi.Instance->TXDR) = *pData; 00717 00718 /* Wait until the bus is ready before releasing Chip select */ 00719 while(((hnucleo_Spi.Instance->SR) & SPI_SR_TXC) == RESET) 00720 { 00721 } 00722 __HAL_SPI_CLEAR_EOTFLAG(&hnucleo_Spi); 00723 /* Empty the Rx fifo */ 00724 data = *(&hnucleo_Spi.Instance->RXDR); 00725 UNUSED(data); /* Remove GNU warning */ 00726 00727 counter--; 00728 pData += 2; 00729 } 00730 00731 /* Clear TXTF Flag : Tx FIFO Threshold */ 00732 __HAL_SPI_CLEAR_TXTFFLAG(&hnucleo_Spi); 00733 00734 /* Disable SPI peripheral */ 00735 __HAL_SPI_DISABLE(&hnucleo_Spi); 00736 } 00737 00738 /* Deselect : Chip Select high */ 00739 LCD_CS_HIGH(); 00740 00741 /* add delay after each SPI command to avoid saturating the Adafruit LCD controller 00742 mandatory as the STM32H743I CPU (CM7) is running at 400 MHz */ 00743 for (counter = 256; counter != 0; counter--) 00744 { 00745 __NOP(); 00746 } 00747 00748 } 00749 00750 /** 00751 * @brief Wait for loop in ms. 00752 * @param Delay in ms. 00753 * @retval None 00754 */ 00755 void LCD_Delay(uint32_t Delay) 00756 { 00757 HAL_Delay(Delay); 00758 } 00759 #endif /* HAL_SPI_MODULE_ENABLED */ 00760 00761 /******************************* ADC driver ********************************/ 00762 #ifdef HAL_ADC_MODULE_ENABLED 00763 00764 /** 00765 * @brief Initializes ADC MSP. 00766 * @param hadc: ADC handle 00767 * @retval None 00768 */ 00769 static void ADCx_MspInit(ADC_HandleTypeDef *hadc) 00770 { 00771 GPIO_InitTypeDef GPIO_InitStruct; 00772 static RCC_PeriphCLKInitTypeDef periph_clk_init_struct; 00773 00774 /*** Configure the GPIOs ***/ 00775 /* Enable GPIO clock */ 00776 NUCLEO_ADCx_GPIO_CLK_ENABLE(); 00777 00778 /* Configure the selected ADC Channel as analog input */ 00779 GPIO_InitStruct.Pin = NUCLEO_ADCx_GPIO_PIN ; 00780 GPIO_InitStruct.Mode = GPIO_MODE_ANALOG; 00781 GPIO_InitStruct.Pull = GPIO_NOPULL; 00782 HAL_GPIO_Init(NUCLEO_ADCx_GPIO_PORT, &GPIO_InitStruct); 00783 00784 /* ADC clock configuration */ 00785 /* PLL2_VCO Input = HSE_VALUE/PLL3M = 8/4 = 2 Mhz */ 00786 /* PLL2_VCO Output = PLL2_VCO Input * PLL2N = 400 Mhz */ 00787 /* ADC Clock = PLL2_VCO Output/PLL2P = 400/6 = 66,6 Mhz */ 00788 periph_clk_init_struct.PeriphClockSelection = RCC_PERIPHCLK_ADC; 00789 periph_clk_init_struct.PLL2.PLL2M = 4; 00790 periph_clk_init_struct.PLL2.PLL2N = 200; 00791 periph_clk_init_struct.PLL2.PLL2P = 6; 00792 periph_clk_init_struct.PLL2.PLL2Q = 2; 00793 periph_clk_init_struct.PLL2.PLL2R = 2; 00794 HAL_RCCEx_PeriphCLKConfig(&periph_clk_init_struct); 00795 00796 /*** Configure the ADC peripheral ***/ 00797 /* Enable ADC clock */ 00798 NUCLEO_ADCx_CLK_ENABLE(); 00799 } 00800 00801 /** 00802 * @brief DeInitializes ADC MSP. 00803 * @param hadc: ADC handle 00804 * @note ADC DeInit does not disable the GPIO clock 00805 * @retval None 00806 */ 00807 static void ADCx_MspDeInit(ADC_HandleTypeDef *hadc) 00808 { 00809 GPIO_InitTypeDef GPIO_InitStruct; 00810 00811 /*** DeInit the ADC peripheral ***/ 00812 /* Disable ADC clock */ 00813 NUCLEO_ADCx_CLK_DISABLE(); 00814 00815 /* Configure the selected ADC Channel as analog input */ 00816 GPIO_InitStruct.Pin = NUCLEO_ADCx_GPIO_PIN ; 00817 HAL_GPIO_DeInit(NUCLEO_ADCx_GPIO_PORT, GPIO_InitStruct.Pin); 00818 00819 /* Disable GPIO clock has to be done by the application*/ 00820 /* NUCLEO_ADCx_GPIO_CLK_DISABLE(); */ 00821 } 00822 00823 /** 00824 * @brief Initializes ADC HAL. 00825 * @retval None 00826 */ 00827 static void ADCx_Init(void) 00828 { 00829 hnucleo_Adc.Instance = NUCLEO_ADCx; 00830 00831 if(HAL_ADC_GetState(&hnucleo_Adc) == HAL_ADC_STATE_RESET) 00832 { 00833 /* ADC Config */ 00834 hnucleo_Adc.Instance = NUCLEO_ADCx; 00835 hnucleo_Adc.Init.ClockPrescaler = ADC_CLOCKPRESCALER_PCLK_DIV4; 00836 hnucleo_Adc.Init.Resolution = ADC_RESOLUTION_12B; 00837 hnucleo_Adc.Init.ContinuousConvMode = DISABLE; 00838 hnucleo_Adc.Init.DiscontinuousConvMode = DISABLE; 00839 hnucleo_Adc.Init.ExternalTrigConvEdge = ADC_EXTERNALTRIGCONVEDGE_NONE; 00840 hnucleo_Adc.Init.EOCSelection = ADC_EOC_SINGLE_CONV; 00841 hnucleo_Adc.Init.NbrOfConversion = 1; 00842 00843 ADCx_MspInit(&hnucleo_Adc); 00844 HAL_ADC_Init(&hnucleo_Adc); 00845 } 00846 } 00847 00848 /** 00849 * @brief Initializes ADC HAL. 00850 * @retval None 00851 */ 00852 static void ADCx_DeInit(void) 00853 { 00854 hnucleo_Adc.Instance = NUCLEO_ADCx; 00855 00856 HAL_ADC_DeInit(&hnucleo_Adc); 00857 ADCx_MspDeInit(&hnucleo_Adc); 00858 } 00859 /** 00860 * @} 00861 */ 00862 00863 /** @addtogroup STM32H7xx_NUCLEO_144_Exported_Functions 00864 * @{ 00865 */ 00866 00867 /******************************* LINK JOYSTICK ********************************/ 00868 00869 /** 00870 * @brief Configures joystick available on adafruit 1.8" TFT shield 00871 * managed through ADC to detect motion. 00872 * @retval Joystickstatus (0=> success, 1=> fail) 00873 */ 00874 uint8_t BSP_JOY_Init(void) 00875 { 00876 uint8_t status = HAL_ERROR; 00877 00878 ADCx_Init(); 00879 00880 /* Select the ADC Channel to be converted */ 00881 sConfig.Channel = NUCLEO_ADCx_CHANNEL; 00882 sConfig.SamplingTime = ADC_SAMPLETIME_2CYCLES_5; 00883 sConfig.Rank = 1; 00884 status = HAL_ADC_ConfigChannel(&hnucleo_Adc, &sConfig); 00885 00886 /* Return Joystick initialization status */ 00887 return status; 00888 } 00889 00890 /** 00891 * @brief DeInit joystick GPIOs. 00892 * @note JOY DeInit does not disable the Mfx, just set the Mfx pins in Off mode 00893 * @retval None. 00894 */ 00895 void BSP_JOY_DeInit(void) 00896 { 00897 ADCx_DeInit(); 00898 } 00899 00900 /** 00901 * @brief Returns the Joystick key pressed. 00902 * @note To know which Joystick key is pressed we need to detect the voltage 00903 * level on each key output 00904 * - None : 3.3 V / 4095 00905 * - SEL : 1.055 V / 1308 00906 * - DOWN : 0.71 V / 88 00907 * - LEFT : 3.0 V / 3720 00908 * - RIGHT : 0.595 V / 737 00909 * - UP : 1.65 V / 2046 00910 * @retval JOYState_TypeDef: Code of the Joystick key pressed. 00911 */ 00912 JOYState_TypeDef BSP_JOY_GetState(void) 00913 { 00914 JOYState_TypeDef state; 00915 uint16_t keyconvertedvalue = 0; 00916 00917 /* Start the conversion process */ 00918 HAL_ADC_Start(&hnucleo_Adc); 00919 00920 /* Wait for the end of conversion */ 00921 HAL_ADC_PollForConversion(&hnucleo_Adc, 10); 00922 00923 /* Check if the continuous conversion of regular channel is finished */ 00924 if((HAL_ADC_GetState(&hnucleo_Adc) & HAL_ADC_STATE_REG_EOC) == HAL_ADC_STATE_REG_EOC) 00925 00926 { 00927 /* Get the converted value of regular channel */ 00928 keyconvertedvalue = HAL_ADC_GetValue(&hnucleo_Adc); 00929 } 00930 00931 if((keyconvertedvalue > 50) && (keyconvertedvalue < 200)) 00932 { 00933 state = JOY_DOWN; 00934 } 00935 else if((keyconvertedvalue > 680) && (keyconvertedvalue < 900)) 00936 { 00937 state = JOY_RIGHT; 00938 } 00939 else if((keyconvertedvalue > 1270) && (keyconvertedvalue < 1500)) 00940 { 00941 state = JOY_SEL; 00942 } 00943 else if((keyconvertedvalue > 2010) && (keyconvertedvalue < 2500)) 00944 { 00945 state = JOY_UP; 00946 } 00947 else if((keyconvertedvalue > 3400) && (keyconvertedvalue < 3750)) 00948 { 00949 state = JOY_LEFT; 00950 } 00951 else 00952 { 00953 state = JOY_NONE; 00954 } 00955 00956 /* Loop while a key is pressed */ 00957 if(state != JOY_NONE) 00958 { 00959 keyconvertedvalue = HAL_ADC_GetValue(&hnucleo_Adc); 00960 } 00961 /* Return the code of the Joystick key pressed */ 00962 return state; 00963 } 00964 /** 00965 * @} 00966 */ 00967 #endif /* HAL_ADC_MODULE_ENABLED */ 00968 00969 #endif /* ADAFRUIT_TFT_JOY_SD_ID802 */ 00970 00971 /** 00972 * @} 00973 */ 00974 00975 /** 00976 * @} 00977 */ 00978 00979 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri Apr 21 2017 17:27:08 for STM32H7xx_Nucleo_144 BSP User Manual by
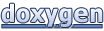