STM32F769I-Discovery BSP User Manual
|
stm32f769i_discovery_ts.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32f769i_discovery_ts.c 00004 * @author MCD Application Team 00005 * @version V2.0.0 00006 * @date 30-December-2016 00007 * @brief This file provides a set of functions needed to manage the Touch 00008 * Screen on STM32F769I-DISCOVERY discovery board. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* File Info : ----------------------------------------------------------------- 00040 User NOTES 00041 1. How To use this driver: 00042 -------------------------- 00043 - This driver is used to drive the touch screen module of the STM32F769I-DISCOVERY 00044 discoveryuation board on the K.O.D Optica Technology 480x800 TFT-LCD mounted on 00045 MB1166 daughter board. The touch screen driver IC inside the K.O.D module KM-040TMP-02 00046 is a FT6206 by Focal Tech. 00047 00048 2. Driver description: 00049 --------------------- 00050 + Initialization steps: 00051 o Initialize the TS module using the BSP_TS_Init() function. This 00052 function includes the MSP layer hardware resources initialization and the 00053 communication layer configuration to start the TS use. The LCD size properties 00054 (x and y) are passed as parameters. 00055 o If TS interrupt mode is desired, you must configure the TS interrupt mode 00056 by calling the function BSP_TS_ITConfig(). The TS interrupt mode is generated 00057 as an external interrupt whenever a touch is detected. 00058 The interrupt mode internally uses the IO functionalities driver driven by 00059 the IO expander, to configure the IT line. 00060 00061 + Touch screen use 00062 o The touch screen state is captured whenever the function BSP_TS_GetState() is 00063 used. This function returns information about the last LCD touch occurred 00064 in the TS_StateTypeDef structure. 00065 o The IT is handled using the corresponding external interrupt IRQ handler, 00066 the user IT callback treatment is implemented on the same external interrupt 00067 callback. 00068 00069 ------------------------------------------------------------------------------*/ 00070 00071 /* Includes ------------------------------------------------------------------*/ 00072 #include "stm32f769i_discovery.h" 00073 #include "stm32f769i_discovery_ts.h" 00074 00075 /** @addtogroup BSP 00076 * @{ 00077 */ 00078 00079 /** @addtogroup STM32F769I_DISCOVERY 00080 * @{ 00081 */ 00082 00083 /** @defgroup STM32F769I_DISCOVERY_TS STM32F769I_DISCOVERY TS 00084 * @{ 00085 */ 00086 00087 /** @defgroup STM32F769I_DISCOVERY_TS_Private_Types_Definitions TS Private Types Definitions 00088 * @{ 00089 */ 00090 /** 00091 * @} 00092 */ 00093 00094 /** @defgroup STM32F769I_DISCOVERY_TS_Private_Defines TS Private Types Defines 00095 * @{ 00096 */ 00097 /** 00098 * @} 00099 */ 00100 00101 /** @defgroup STM32F769I_DISCOVERY_TS_Private_Macros TS Private Macros 00102 * @{ 00103 */ 00104 /** 00105 * @} 00106 */ 00107 00108 /** @defgroup STM32F769I_DISCOVERY_TS_Imported_Variables TS Imported Variables 00109 * @{ 00110 */ 00111 /** 00112 * @} 00113 */ 00114 00115 /** @defgroup STM32F769I_DISCOVERY_TS_Private_Variables TS Private Variables 00116 * @{ 00117 */ 00118 static TS_DrvTypeDef *ts_driver; 00119 static uint8_t ts_orientation; 00120 uint8_t I2C_Address = 0; 00121 00122 /* Table for touchscreen event information display on LCD : table indexed on enum @ref TS_TouchEventTypeDef information */ 00123 char * ts_event_string_tab[TOUCH_EVENT_NB_MAX] = { "None", 00124 "Press down", 00125 "Lift up", 00126 "Contact" 00127 }; 00128 00129 /* Table for touchscreen gesture Id information display on LCD : table indexed on enum @ref TS_GestureIdTypeDef information */ 00130 char * ts_gesture_id_string_tab[GEST_ID_NB_MAX] = { "None", 00131 "Move Up", 00132 "Move Right", 00133 "Move Down", 00134 "Move Left", 00135 "Zoom In", 00136 "Zoom Out" 00137 }; 00138 00139 /** 00140 * @} 00141 */ 00142 00143 /** @defgroup STM32F769I_DISCOVERY_TS_Private_Function_Prototypes TS Private Function Prototypes 00144 * @{ 00145 */ 00146 00147 /** 00148 * @} 00149 */ 00150 00151 /** @defgroup STM32F769I_DISCOVERY_TS_Public_Functions TS Public Functions 00152 * @{ 00153 */ 00154 00155 /** 00156 * @brief Initializes and configures the touch screen functionalities and 00157 * configures all necessary hardware resources (GPIOs, I2C, clocks..). 00158 * @param ts_SizeX : Maximum X size of the TS area on LCD 00159 * @param ts_SizeY : Maximum Y size of the TS area on LCD 00160 * @retval TS_OK if all initializations are OK. Other value if error. 00161 */ 00162 uint8_t BSP_TS_Init(uint16_t ts_SizeX, uint16_t ts_SizeY) 00163 { 00164 uint8_t ts_status = TS_OK; 00165 uint8_t ts_id1, ts_id2 = 0; 00166 /* Note : I2C_Address is un-initialized here, but is not used at all in init function */ 00167 /* but the prototype of Init() is like that in template and should be respected */ 00168 00169 /* Initialize the communication channel to sensor (I2C) if necessary */ 00170 /* that is initialization is done only once after a power up */ 00171 ft6x06_ts_drv.Init(I2C_Address); 00172 00173 ts_id1 = ft6x06_ts_drv.ReadID(TS_I2C_ADDRESS); 00174 if(ts_id1 != FT6206_ID_VALUE) 00175 { 00176 ts_id2 = ft6x06_ts_drv.ReadID(TS_I2C_ADDRESS_A02); 00177 I2C_Address = TS_I2C_ADDRESS_A02; 00178 } 00179 else 00180 { 00181 I2C_Address = TS_I2C_ADDRESS; 00182 } 00183 00184 /* Scan FT6xx6 TouchScreen IC controller ID register by I2C Read */ 00185 /* Verify this is a FT6206 or FT6336G, otherwise this is an error case */ 00186 if((ts_id1 == FT6206_ID_VALUE) || (ts_id2 == FT6206_ID_VALUE)) 00187 { 00188 /* Found FT6206 : Initialize the TS driver structure */ 00189 ts_driver = &ft6x06_ts_drv; 00190 00191 /* Get LCD chosen orientation */ 00192 if(ts_SizeX < ts_SizeY) 00193 { 00194 ts_orientation = TS_SWAP_NONE; 00195 } 00196 else 00197 { 00198 ts_orientation = TS_SWAP_XY | TS_SWAP_Y; 00199 } 00200 00201 if(ts_status == TS_OK) 00202 { 00203 /* Software reset the TouchScreen */ 00204 ts_driver->Reset(I2C_Address); 00205 00206 /* Calibrate, Configure and Start the TouchScreen driver */ 00207 ts_driver->Start(I2C_Address); 00208 00209 } /* of if(ts_status == TS_OK) */ 00210 } 00211 else 00212 { 00213 ts_status = TS_DEVICE_NOT_FOUND; 00214 } 00215 00216 return (ts_status); 00217 } 00218 00219 /** 00220 * @brief Configures and enables the touch screen interrupts. 00221 * @retval TS_OK if all initializations are OK. Other value if error. 00222 */ 00223 uint8_t BSP_TS_ITConfig(void) 00224 { 00225 uint8_t ts_status = TS_OK; 00226 GPIO_InitTypeDef gpio_init_structure; 00227 00228 /* Msp Init of GPIO used for TS_INT pin coming from TouchScreen driver IC FT6x06 */ 00229 /* When touchscreen is operated in interrupt mode */ 00230 BSP_TS_INT_MspInit(); 00231 00232 /* Configure Interrupt mode for TS_INT pin falling edge : when a new touch is available */ 00233 /* TS_INT pin is active on low level on new touch available */ 00234 gpio_init_structure.Pin = TS_INT_PIN; 00235 gpio_init_structure.Pull = GPIO_PULLUP; 00236 gpio_init_structure.Speed = GPIO_SPEED_FAST; 00237 gpio_init_structure.Mode = GPIO_MODE_IT_FALLING; 00238 HAL_GPIO_Init(TS_INT_GPIO_PORT, &gpio_init_structure); 00239 00240 /* Enable and set the TS_INT EXTI Interrupt to an intermediate priority */ 00241 HAL_NVIC_SetPriority((IRQn_Type)(TS_INT_EXTI_IRQn), 0x0F, 0x00); 00242 HAL_NVIC_EnableIRQ((IRQn_Type)(TS_INT_EXTI_IRQn)); 00243 00244 /* Enable the TS in interrupt mode */ 00245 /* In that case the INT output of FT6206 when new touch is available */ 00246 /* is active on low level and directed on EXTI */ 00247 ts_driver->EnableIT(I2C_Address); 00248 00249 return (ts_status); 00250 } 00251 00252 /** 00253 * @brief Returns status and positions of the touch screen. 00254 * @param TS_State: Pointer to touch screen current state structure 00255 * @retval TS_OK if all initializations are OK. Other value if error. 00256 */ 00257 uint8_t BSP_TS_GetState(TS_StateTypeDef *TS_State) 00258 { 00259 static uint32_t _x[TS_MAX_NB_TOUCH] = {0, 0}; 00260 static uint32_t _y[TS_MAX_NB_TOUCH] = {0, 0}; 00261 uint8_t ts_status = TS_OK; 00262 uint16_t tmp; 00263 uint16_t Raw_x[TS_MAX_NB_TOUCH]; 00264 uint16_t Raw_y[TS_MAX_NB_TOUCH]; 00265 uint16_t xDiff; 00266 uint16_t yDiff; 00267 uint32_t index; 00268 #if (TS_MULTI_TOUCH_SUPPORTED == 1) 00269 uint32_t weight = 0; 00270 uint32_t area = 0; 00271 uint32_t event = 0; 00272 #endif /* TS_MULTI_TOUCH_SUPPORTED == 1 */ 00273 00274 /* Check and update the number of touches active detected */ 00275 TS_State->touchDetected = ts_driver->DetectTouch(I2C_Address); 00276 if(TS_State->touchDetected) 00277 { 00278 for(index=0; index < TS_State->touchDetected; index++) 00279 { 00280 /* Get each touch coordinates */ 00281 ts_driver->GetXY(I2C_Address, &(Raw_x[index]), &(Raw_y[index])); 00282 00283 if(ts_orientation & TS_SWAP_XY) 00284 { 00285 tmp = Raw_x[index]; 00286 Raw_x[index] = Raw_y[index]; 00287 Raw_y[index] = tmp; 00288 } 00289 00290 if(ts_orientation & TS_SWAP_X) 00291 { 00292 Raw_x[index] = FT_6206_MAX_WIDTH - 1 - Raw_x[index]; 00293 } 00294 00295 if(ts_orientation & TS_SWAP_Y) 00296 { 00297 Raw_y[index] = FT_6206_MAX_HEIGHT - 1 - Raw_y[index]; 00298 } 00299 00300 xDiff = Raw_x[index] > _x[index]? (Raw_x[index] - _x[index]): (_x[index] - Raw_x[index]); 00301 yDiff = Raw_y[index] > _y[index]? (Raw_y[index] - _y[index]): (_y[index] - Raw_y[index]); 00302 00303 if ((xDiff + yDiff) > 5) 00304 { 00305 _x[index] = Raw_x[index]; 00306 _y[index] = Raw_y[index]; 00307 } 00308 00309 00310 TS_State->touchX[index] = _x[index]; 00311 TS_State->touchY[index] = _y[index]; 00312 00313 #if (TS_MULTI_TOUCH_SUPPORTED == 1) 00314 00315 /* Get touch info related to the current touch */ 00316 ft6x06_TS_GetTouchInfo(I2C_Address, index, &weight, &area, &event); 00317 00318 /* Update TS_State structure */ 00319 TS_State->touchWeight[index] = weight; 00320 TS_State->touchArea[index] = area; 00321 00322 /* Remap touch event */ 00323 switch(event) 00324 { 00325 case FT6206_TOUCH_EVT_FLAG_PRESS_DOWN : 00326 TS_State->touchEventId[index] = TOUCH_EVENT_PRESS_DOWN; 00327 break; 00328 case FT6206_TOUCH_EVT_FLAG_LIFT_UP : 00329 TS_State->touchEventId[index] = TOUCH_EVENT_LIFT_UP; 00330 break; 00331 case FT6206_TOUCH_EVT_FLAG_CONTACT : 00332 TS_State->touchEventId[index] = TOUCH_EVENT_CONTACT; 00333 break; 00334 case FT6206_TOUCH_EVT_FLAG_NO_EVENT : 00335 TS_State->touchEventId[index] = TOUCH_EVENT_NO_EVT; 00336 break; 00337 default : 00338 ts_status = TS_ERROR; 00339 break; 00340 } /* of switch(event) */ 00341 00342 #endif /* TS_MULTI_TOUCH_SUPPORTED == 1 */ 00343 00344 } /* of for(index=0; index < TS_State->touchDetected; index++) */ 00345 00346 #if (TS_MULTI_TOUCH_SUPPORTED == 1) 00347 /* Get gesture Id */ 00348 ts_status = BSP_TS_Get_GestureId(TS_State); 00349 #endif /* TS_MULTI_TOUCH_SUPPORTED == 1 */ 00350 00351 } /* end of if(TS_State->touchDetected != 0) */ 00352 00353 return (ts_status); 00354 } 00355 00356 #if (TS_MULTI_TOUCH_SUPPORTED == 1) 00357 /** 00358 * @brief Update gesture Id following a touch detected. 00359 * @param TS_State: Pointer to touch screen current state structure 00360 * @retval TS_OK if all initializations are OK. Other value if error. 00361 */ 00362 uint8_t BSP_TS_Get_GestureId(TS_StateTypeDef *TS_State) 00363 { 00364 uint32_t gestureId = 0; 00365 uint8_t ts_status = TS_OK; 00366 00367 /* Get gesture Id */ 00368 ft6x06_TS_GetGestureID(I2C_Address, &gestureId); 00369 00370 /* Remap gesture Id to a TS_GestureIdTypeDef value */ 00371 switch(gestureId) 00372 { 00373 case FT6206_GEST_ID_NO_GESTURE : 00374 TS_State->gestureId = GEST_ID_NO_GESTURE; 00375 break; 00376 case FT6206_GEST_ID_MOVE_UP : 00377 TS_State->gestureId = GEST_ID_MOVE_UP; 00378 break; 00379 case FT6206_GEST_ID_MOVE_RIGHT : 00380 TS_State->gestureId = GEST_ID_MOVE_RIGHT; 00381 break; 00382 case FT6206_GEST_ID_MOVE_DOWN : 00383 TS_State->gestureId = GEST_ID_MOVE_DOWN; 00384 break; 00385 case FT6206_GEST_ID_MOVE_LEFT : 00386 TS_State->gestureId = GEST_ID_MOVE_LEFT; 00387 break; 00388 case FT6206_GEST_ID_ZOOM_IN : 00389 TS_State->gestureId = GEST_ID_ZOOM_IN; 00390 break; 00391 case FT6206_GEST_ID_ZOOM_OUT : 00392 TS_State->gestureId = GEST_ID_ZOOM_OUT; 00393 break; 00394 default : 00395 ts_status = TS_ERROR; 00396 break; 00397 } /* of switch(gestureId) */ 00398 00399 return(ts_status); 00400 } 00401 #endif /* TS_MULTI_TOUCH_SUPPORTED == 1 */ 00402 00403 00404 /** @defgroup STM32F769I_DISCOVERY_TS_Private_Functions TS Private Functions 00405 * @{ 00406 */ 00407 00408 #if (TS_MULTI_TOUCH_SUPPORTED == 1) 00409 /** 00410 * @brief Function used to reset all touch data before a new acquisition 00411 * of touch information. 00412 * @param TS_State: Pointer to touch screen current state structure 00413 * @retval TS_OK if OK, TE_ERROR if problem found. 00414 */ 00415 uint8_t BSP_TS_ResetTouchData(TS_StateTypeDef *TS_State) 00416 { 00417 uint8_t ts_status = TS_ERROR; 00418 uint32_t index; 00419 00420 if (TS_State != (TS_StateTypeDef *)NULL) 00421 { 00422 TS_State->gestureId = GEST_ID_NO_GESTURE; 00423 TS_State->touchDetected = 0; 00424 00425 for(index = 0; index < TS_MAX_NB_TOUCH; index++) 00426 { 00427 TS_State->touchX[index] = 0; 00428 TS_State->touchY[index] = 0; 00429 TS_State->touchArea[index] = 0; 00430 TS_State->touchEventId[index] = TOUCH_EVENT_NO_EVT; 00431 TS_State->touchWeight[index] = 0; 00432 } 00433 00434 ts_status = TS_OK; 00435 00436 } /* of if (TS_State != (TS_StateTypeDef *)NULL) */ 00437 00438 return (ts_status); 00439 } 00440 #endif /* TS_MULTI_TOUCH_SUPPORTED == 1 */ 00441 00442 /** 00443 * @brief Initializes the TS_INT pin MSP. 00444 * @retval None 00445 */ 00446 __weak void BSP_TS_INT_MspInit(void) 00447 { 00448 GPIO_InitTypeDef gpio_init_structure; 00449 00450 TS_INT_GPIO_CLK_ENABLE(); 00451 00452 /* GPIO configuration in input for TouchScreen interrupt signal on TS_INT pin */ 00453 gpio_init_structure.Pin = TS_INT_PIN; 00454 00455 gpio_init_structure.Mode = GPIO_MODE_INPUT; 00456 gpio_init_structure.Pull = GPIO_PULLUP; 00457 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00458 HAL_GPIO_Init(TS_INT_GPIO_PORT, &gpio_init_structure); 00459 } 00460 00461 /** 00462 * @} 00463 */ 00464 00465 /** 00466 * @} 00467 */ 00468 00469 /** 00470 * @} 00471 */ 00472 00473 /** 00474 * @} 00475 */ 00476 00477 /** 00478 * @} 00479 */ 00480 00481 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri Dec 30 2016 18:30:07 for STM32F769I-Discovery BSP User Manual by
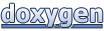