STM32F769I-Discovery BSP User Manual
|
stm32f769i_discovery_sd.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32f769i_discovery_sd.c 00004 * @author MCD Application Team 00005 * @version V2.0.0 00006 * @date 30-December-2016 00007 * @brief This file includes the uSD card driver mounted on STM32F769I-Discovery 00008 * board. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* File Info : ----------------------------------------------------------------- 00040 User NOTES 00041 1. How To use this driver: 00042 -------------------------- 00043 - This driver is used to drive the micro SD external card mounted on STM32F769I-Discovery 00044 board. 00045 - This driver does not need a specific component driver for the micro SD device 00046 to be included with. 00047 00048 2. Driver description: 00049 --------------------- 00050 + Initialization steps: 00051 o Initialize the micro SD card using the BSP_SD_Init() function. This 00052 function includes the MSP layer hardware resources initialization and the 00053 SDIO interface configuration to interface with the external micro SD. It 00054 also includes the micro SD initialization sequence. 00055 o To check the SD card presence you can use the function BSP_SD_IsDetected() which 00056 returns the detection status 00057 o If SD presence detection interrupt mode is desired, you must configure the 00058 SD detection interrupt mode by calling the function BSP_SD_ITConfig(). The interrupt 00059 is generated as an external interrupt whenever the micro SD card is 00060 plugged/unplugged in/from the discovery board. 00061 o The function BSP_SD_GetCardInfo() is used to get the micro SD card information 00062 which is stored in the structure "HAL_SD_CardInfoTypedef". 00063 00064 + Micro SD card operations 00065 o The micro SD card can be accessed with read/write block(s) operations once 00066 it is reay for access. The access cand be performed whether using the polling 00067 mode by calling the functions BSP_SD_ReadBlocks()/BSP_SD_WriteBlocks(), or by DMA 00068 transfer using the functions BSP_SD_ReadBlocks_DMA()/BSP_SD_WriteBlocks_DMA() 00069 o The DMA transfer complete is used with interrupt mode. Once the SD transfer 00070 is complete, the SD interrupt is handeled using the function BSP_SD_IRQHandler(), 00071 the DMA Tx/Rx transfer complete are handeled using the functions 00072 BSP_SD_DMA_Tx_IRQHandler()/BSP_SD_DMA_Rx_IRQHandler(). The corresponding user callbacks 00073 are implemented by the user at application level. 00074 o The SD erase block(s) is performed using the function BSP_SD_Erase() with specifying 00075 the number of blocks to erase. 00076 o The SD runtime status is returned when calling the function BSP_SD_GetCardState(). 00077 00078 ------------------------------------------------------------------------------*/ 00079 00080 /* Includes ------------------------------------------------------------------*/ 00081 #include "stm32f769i_discovery_sd.h" 00082 00083 /** @addtogroup BSP 00084 * @{ 00085 */ 00086 00087 /** @addtogroup STM32F769I_DISCOVERY 00088 * @{ 00089 */ 00090 00091 /** @defgroup STM32F769I_DISCOVERY_SD STM32F769I_DISCOVERY SD 00092 * @{ 00093 */ 00094 00095 00096 /** @defgroup STM32F769I_DISCOVERY_SD_Private_TypesDefinitions STM32F769I Discovery Sd Private TypesDef 00097 * @{ 00098 */ 00099 /** 00100 * @} 00101 */ 00102 00103 /** @defgroup STM32F769I_DISCOVERY_SD_Private_Defines STM32F769I Discovery Sd Private Defines 00104 * @{ 00105 */ 00106 /** 00107 * @} 00108 */ 00109 00110 /** @defgroup STM32F769I_DISCOVERY_SD_Private_Macros STM32F769I Discovery Sd Private Macro 00111 * @{ 00112 */ 00113 /** 00114 * @} 00115 */ 00116 00117 /** @defgroup STM32F769I_DISCOVERY_SD_Private_Variables STM32F769I Discovery Sd Private Variables 00118 * @{ 00119 */ 00120 SD_HandleTypeDef uSdHandle; 00121 00122 /** 00123 * @} 00124 */ 00125 00126 /** @defgroup STM32F769I_DISCOVERY_SD_Private_FunctionPrototypes STM32F769I Discovery Sd Private Prototypes 00127 * @{ 00128 */ 00129 /** 00130 * @} 00131 */ 00132 00133 /** @defgroup STM32F769I_DISCOVERY_SD_Private_Functions STM32F769I Discovery Sd Private Functions 00134 * @{ 00135 */ 00136 00137 /** 00138 * @brief Initializes the SD card device. 00139 * @retval SD status 00140 */ 00141 uint8_t BSP_SD_Init(void) 00142 { 00143 uint8_t sd_state = MSD_OK; 00144 00145 /* PLLSAI is dedicated to LCD periph. Do not use it to get 48MHz*/ 00146 00147 /* uSD device interface configuration */ 00148 uSdHandle.Instance = SDMMC2; 00149 uSdHandle.Init.ClockEdge = SDMMC_CLOCK_EDGE_RISING; 00150 uSdHandle.Init.ClockBypass = SDMMC_CLOCK_BYPASS_DISABLE; 00151 uSdHandle.Init.ClockPowerSave = SDMMC_CLOCK_POWER_SAVE_DISABLE; 00152 uSdHandle.Init.BusWide = SDMMC_BUS_WIDE_1B; 00153 uSdHandle.Init.HardwareFlowControl = SDMMC_HARDWARE_FLOW_CONTROL_DISABLE; 00154 uSdHandle.Init.ClockDiv = SDMMC_TRANSFER_CLK_DIV; 00155 00156 /* Msp SD Detect pin initialization */ 00157 BSP_SD_Detect_MspInit(&uSdHandle, NULL); 00158 if(BSP_SD_IsDetected() != SD_PRESENT) /* Check if SD card is present */ 00159 { 00160 return MSD_ERROR_SD_NOT_PRESENT; 00161 } 00162 00163 /* Msp SD initialization */ 00164 BSP_SD_MspInit(&uSdHandle, NULL); 00165 00166 /* HAL SD initialization */ 00167 if(HAL_SD_Init(&uSdHandle) != HAL_OK) 00168 { 00169 sd_state = MSD_ERROR; 00170 } 00171 00172 /* Configure SD Bus width */ 00173 if(sd_state == MSD_OK) 00174 { 00175 /* Enable wide operation */ 00176 if(HAL_SD_ConfigWideBusOperation(&uSdHandle, SDMMC_BUS_WIDE_4B) != HAL_OK) 00177 { 00178 sd_state = MSD_ERROR; 00179 } 00180 else 00181 { 00182 sd_state = MSD_OK; 00183 } 00184 } 00185 return sd_state; 00186 } 00187 00188 /** 00189 * @brief DeInitializes the SD card device. 00190 * @retval SD status 00191 */ 00192 uint8_t BSP_SD_DeInit(void) 00193 { 00194 uint8_t sd_state = MSD_OK; 00195 00196 uSdHandle.Instance = SDMMC2; 00197 00198 /* HAL SD deinitialization */ 00199 if(HAL_SD_DeInit(&uSdHandle) != HAL_OK) 00200 { 00201 sd_state = MSD_ERROR; 00202 } 00203 00204 /* Msp SD deinitialization */ 00205 uSdHandle.Instance = SDMMC2; 00206 BSP_SD_MspDeInit(&uSdHandle, NULL); 00207 00208 return sd_state; 00209 } 00210 00211 /** 00212 * @brief Configures Interrupt mode for SD detection pin. 00213 * @retval Returns 0 00214 */ 00215 uint8_t BSP_SD_ITConfig(void) 00216 { 00217 GPIO_InitTypeDef gpio_init_structure; 00218 00219 /* Configure Interrupt mode for SD detection pin */ 00220 gpio_init_structure.Pin = SD_DETECT_PIN; 00221 gpio_init_structure.Pull = GPIO_PULLUP; 00222 gpio_init_structure.Speed = GPIO_SPEED_FAST; 00223 gpio_init_structure.Mode = GPIO_MODE_IT_RISING_FALLING; 00224 HAL_GPIO_Init(SD_DETECT_GPIO_PORT, &gpio_init_structure); 00225 00226 /* Enable and set SD detect EXTI Interrupt to the lowest priority */ 00227 HAL_NVIC_SetPriority((IRQn_Type)(SD_DETECT_EXTI_IRQn), 0x0F, 0x00); 00228 HAL_NVIC_EnableIRQ((IRQn_Type)(SD_DETECT_EXTI_IRQn)); 00229 00230 return MSD_OK; 00231 } 00232 00233 /** 00234 * @brief Detects if SD card is correctly plugged in the memory slot or not. 00235 * @retval Returns if SD is detected or not 00236 */ 00237 uint8_t BSP_SD_IsDetected(void) 00238 { 00239 __IO uint8_t status = SD_PRESENT; 00240 00241 /* Check SD card detect pin */ 00242 if (HAL_GPIO_ReadPin(SD_DETECT_GPIO_PORT, SD_DETECT_PIN) == GPIO_PIN_SET) 00243 { 00244 status = SD_NOT_PRESENT; 00245 } 00246 00247 return status; 00248 } 00249 00250 /** 00251 * @brief Reads block(s) from a specified address in an SD card, in polling mode. 00252 * @param pData: Pointer to the buffer that will contain the data to transmit 00253 * @param ReadAddr: Address from where data is to be read 00254 * @param NumOfBlocks: Number of SD blocks to read 00255 * @param Timeout: Timeout for read operation 00256 * @retval SD status 00257 */ 00258 uint8_t BSP_SD_ReadBlocks(uint32_t *pData, uint32_t ReadAddr, uint32_t NumOfBlocks, uint32_t Timeout) 00259 { 00260 if(HAL_SD_ReadBlocks(&uSdHandle, (uint8_t *)pData, ReadAddr, NumOfBlocks, Timeout) != HAL_OK) 00261 { 00262 return MSD_ERROR; 00263 } 00264 else 00265 { 00266 return MSD_OK; 00267 } 00268 } 00269 00270 /** 00271 * @brief Writes block(s) to a specified address in an SD card, in polling mode. 00272 * @param pData: Pointer to the buffer that will contain the data to transmit 00273 * @param WriteAddr: Address from where data is to be written 00274 * @param NumOfBlocks: Number of SD blocks to write 00275 * @param Timeout: Timeout for write operation 00276 * @retval SD status 00277 */ 00278 uint8_t BSP_SD_WriteBlocks(uint32_t *pData, uint32_t WriteAddr, uint32_t NumOfBlocks, uint32_t Timeout) 00279 { 00280 if(HAL_SD_WriteBlocks(&uSdHandle, (uint8_t *)pData, WriteAddr, NumOfBlocks, Timeout) != HAL_OK) 00281 { 00282 return MSD_ERROR; 00283 } 00284 else 00285 { 00286 return MSD_OK; 00287 } 00288 } 00289 00290 /** 00291 * @brief Reads block(s) from a specified address in an SD card, in DMA mode. 00292 * @param pData: Pointer to the buffer that will contain the data to transmit 00293 * @param ReadAddr: Address from where data is to be read 00294 * @param NumOfBlocks: Number of SD blocks to read 00295 * @retval SD status 00296 */ 00297 uint8_t BSP_SD_ReadBlocks_DMA(uint32_t *pData, uint32_t ReadAddr, uint32_t NumOfBlocks) 00298 { 00299 /* Read block(s) in DMA transfer mode */ 00300 if(HAL_SD_ReadBlocks_DMA(&uSdHandle, (uint8_t *)pData, ReadAddr, NumOfBlocks) != HAL_OK) 00301 { 00302 return MSD_ERROR; 00303 } 00304 else 00305 { 00306 return MSD_OK; 00307 } 00308 } 00309 00310 /** 00311 * @brief Writes block(s) to a specified address in an SD card, in DMA mode. 00312 * @param pData: Pointer to the buffer that will contain the data to transmit 00313 * @param WriteAddr: Address from where data is to be written 00314 * @param NumOfBlocks: Number of SD blocks to write 00315 * @retval SD status 00316 */ 00317 uint8_t BSP_SD_WriteBlocks_DMA(uint32_t *pData, uint32_t WriteAddr, uint32_t NumOfBlocks) 00318 { 00319 /* Write block(s) in DMA transfer mode */ 00320 if(HAL_SD_WriteBlocks_DMA(&uSdHandle, (uint8_t *)pData, WriteAddr, NumOfBlocks) != HAL_OK) 00321 { 00322 return MSD_ERROR; 00323 } 00324 else 00325 { 00326 return MSD_OK; 00327 } 00328 } 00329 00330 /** 00331 * @brief Erases the specified memory area of the given SD card. 00332 * @param StartAddr: Start byte address 00333 * @param EndAddr: End byte address 00334 * @retval SD status 00335 */ 00336 uint8_t BSP_SD_Erase(uint32_t StartAddr, uint32_t EndAddr) 00337 { 00338 if(HAL_SD_Erase(&uSdHandle, StartAddr, EndAddr) != HAL_OK) 00339 { 00340 return MSD_ERROR; 00341 } 00342 else 00343 { 00344 return MSD_OK; 00345 } 00346 } 00347 00348 /** 00349 * @brief Initializes the SD MSP. 00350 * @param hsd: SD handle 00351 * @param Params : pointer on additional configuration parameters, can be NULL. 00352 */ 00353 __weak void BSP_SD_MspInit(SD_HandleTypeDef *hsd, void *Params) 00354 { 00355 static DMA_HandleTypeDef dma_rx_handle; 00356 static DMA_HandleTypeDef dma_tx_handle; 00357 GPIO_InitTypeDef gpio_init_structure; 00358 00359 /* Enable SDMMC2 clock */ 00360 __HAL_RCC_SDMMC2_CLK_ENABLE(); 00361 00362 /* Enable DMA2 clocks */ 00363 __DMAx_TxRx_CLK_ENABLE(); 00364 00365 /* Enable GPIOs clock */ 00366 __HAL_RCC_GPIOB_CLK_ENABLE(); 00367 __HAL_RCC_GPIOD_CLK_ENABLE(); 00368 __HAL_RCC_GPIOG_CLK_ENABLE(); 00369 00370 /* Common GPIO configuration */ 00371 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00372 gpio_init_structure.Pull = GPIO_PULLUP; 00373 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00374 00375 /* GPIOB configuration */ 00376 gpio_init_structure.Alternate = GPIO_AF10_SDMMC2; 00377 gpio_init_structure.Pin = GPIO_PIN_3 | GPIO_PIN_4; 00378 HAL_GPIO_Init(GPIOB, &gpio_init_structure); 00379 00380 /* GPIOD configuration */ 00381 gpio_init_structure.Alternate = GPIO_AF11_SDMMC2; 00382 gpio_init_structure.Pin = GPIO_PIN_6 | GPIO_PIN_7; 00383 HAL_GPIO_Init(GPIOD, &gpio_init_structure); 00384 00385 /* GPIOG configuration */ 00386 gpio_init_structure.Pin = GPIO_PIN_9 | GPIO_PIN_10; 00387 HAL_GPIO_Init(GPIOG, &gpio_init_structure); 00388 00389 /* NVIC configuration for SDMMC2 interrupts */ 00390 HAL_NVIC_SetPriority(SDMMC2_IRQn, 0x0E, 0); 00391 HAL_NVIC_EnableIRQ(SDMMC2_IRQn); 00392 00393 /* Configure DMA Rx parameters */ 00394 dma_rx_handle.Init.Channel = SD_DMAx_Rx_CHANNEL; 00395 dma_rx_handle.Init.Direction = DMA_PERIPH_TO_MEMORY; 00396 dma_rx_handle.Init.PeriphInc = DMA_PINC_DISABLE; 00397 dma_rx_handle.Init.MemInc = DMA_MINC_ENABLE; 00398 dma_rx_handle.Init.PeriphDataAlignment = DMA_PDATAALIGN_WORD; 00399 dma_rx_handle.Init.MemDataAlignment = DMA_MDATAALIGN_WORD; 00400 dma_rx_handle.Init.Mode = DMA_PFCTRL; 00401 dma_rx_handle.Init.Priority = DMA_PRIORITY_VERY_HIGH; 00402 dma_rx_handle.Init.FIFOMode = DMA_FIFOMODE_ENABLE; 00403 dma_rx_handle.Init.FIFOThreshold = DMA_FIFO_THRESHOLD_FULL; 00404 dma_rx_handle.Init.MemBurst = DMA_MBURST_INC4; 00405 dma_rx_handle.Init.PeriphBurst = DMA_PBURST_INC4; 00406 00407 dma_rx_handle.Instance = SD_DMAx_Rx_STREAM; 00408 00409 /* Associate the DMA handle */ 00410 __HAL_LINKDMA(hsd, hdmarx, dma_rx_handle); 00411 00412 /* Deinitialize the stream for new transfer */ 00413 HAL_DMA_DeInit(&dma_rx_handle); 00414 00415 /* Configure the DMA stream */ 00416 HAL_DMA_Init(&dma_rx_handle); 00417 00418 /* Configure DMA Tx parameters */ 00419 dma_tx_handle.Init.Channel = SD_DMAx_Tx_CHANNEL; 00420 dma_tx_handle.Init.Direction = DMA_MEMORY_TO_PERIPH; 00421 dma_tx_handle.Init.PeriphInc = DMA_PINC_DISABLE; 00422 dma_tx_handle.Init.MemInc = DMA_MINC_ENABLE; 00423 dma_tx_handle.Init.PeriphDataAlignment = DMA_PDATAALIGN_WORD; 00424 dma_tx_handle.Init.MemDataAlignment = DMA_MDATAALIGN_WORD; 00425 dma_tx_handle.Init.Mode = DMA_PFCTRL; 00426 dma_tx_handle.Init.Priority = DMA_PRIORITY_VERY_HIGH; 00427 dma_tx_handle.Init.FIFOMode = DMA_FIFOMODE_ENABLE; 00428 dma_tx_handle.Init.FIFOThreshold = DMA_FIFO_THRESHOLD_FULL; 00429 dma_tx_handle.Init.MemBurst = DMA_MBURST_INC4; 00430 dma_tx_handle.Init.PeriphBurst = DMA_PBURST_INC4; 00431 00432 dma_tx_handle.Instance = SD_DMAx_Tx_STREAM; 00433 00434 /* Associate the DMA handle */ 00435 __HAL_LINKDMA(hsd, hdmatx, dma_tx_handle); 00436 00437 /* Deinitialize the stream for new transfer */ 00438 HAL_DMA_DeInit(&dma_tx_handle); 00439 00440 /* Configure the DMA stream */ 00441 HAL_DMA_Init(&dma_tx_handle); 00442 00443 /* NVIC configuration for DMA transfer complete interrupt */ 00444 HAL_NVIC_SetPriority(SD_DMAx_Rx_IRQn, 0x0F, 0); 00445 HAL_NVIC_EnableIRQ(SD_DMAx_Rx_IRQn); 00446 00447 /* NVIC configuration for DMA transfer complete interrupt */ 00448 HAL_NVIC_SetPriority(SD_DMAx_Tx_IRQn, 0x0F, 0); 00449 HAL_NVIC_EnableIRQ(SD_DMAx_Tx_IRQn); 00450 } 00451 00452 /** 00453 * @brief Initializes the SD Detect pin MSP. 00454 * @param hsd: SD handle 00455 * @param Params : pointer on additional configuration parameters, can be NULL. 00456 * @retval None 00457 */ 00458 __weak void BSP_SD_Detect_MspInit(SD_HandleTypeDef *hsd, void *Params) 00459 { 00460 GPIO_InitTypeDef gpio_init_structure; 00461 00462 SD_DETECT_GPIO_CLK_ENABLE(); 00463 00464 /* GPIO configuration in input for uSD_Detect signal */ 00465 gpio_init_structure.Pin = SD_DETECT_PIN; 00466 gpio_init_structure.Mode = GPIO_MODE_INPUT; 00467 gpio_init_structure.Pull = GPIO_PULLUP; 00468 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00469 HAL_GPIO_Init(SD_DETECT_GPIO_PORT, &gpio_init_structure); 00470 } 00471 00472 /** 00473 * @brief DeInitializes the SD MSP. 00474 * @param hsd: SD handle 00475 * @param Params : pointer on additional configuration parameters, can be NULL. 00476 */ 00477 __weak void BSP_SD_MspDeInit(SD_HandleTypeDef *hsd, void *Params) 00478 { 00479 static DMA_HandleTypeDef dma_rx_handle; 00480 static DMA_HandleTypeDef dma_tx_handle; 00481 00482 /* Disable NVIC for DMA transfer complete interrupts */ 00483 HAL_NVIC_DisableIRQ(SD_DMAx_Rx_IRQn); 00484 HAL_NVIC_DisableIRQ(SD_DMAx_Tx_IRQn); 00485 00486 /* Deinitialize the stream for new transfer */ 00487 dma_rx_handle.Instance = SD_DMAx_Rx_STREAM; 00488 HAL_DMA_DeInit(&dma_rx_handle); 00489 00490 /* Deinitialize the stream for new transfer */ 00491 dma_tx_handle.Instance = SD_DMAx_Tx_STREAM; 00492 HAL_DMA_DeInit(&dma_tx_handle); 00493 00494 /* Disable NVIC for SDIO interrupts */ 00495 HAL_NVIC_DisableIRQ(SDIO_IRQn); 00496 00497 /* DeInit GPIO pins can be done in the application 00498 (by surcharging this __weak function) */ 00499 00500 /* Disable SDIO clock */ 00501 __HAL_RCC_SDIO_CLK_DISABLE(); 00502 00503 /* GPOI pins clock and DMA cloks can be shut down in the applic 00504 by surcgarging this __weak function */ 00505 } 00506 00507 /** 00508 * @brief Gets the current SD card data status. 00509 * @retval Data transfer state. 00510 * This value can be one of the following values: 00511 * @arg SD_TRANSFER_OK: No data transfer is acting 00512 * @arg SD_TRANSFER_BUSY: Data transfer is acting 00513 */ 00514 uint8_t BSP_SD_GetCardState(void) 00515 { 00516 return((HAL_SD_GetCardState(&uSdHandle) == HAL_SD_CARD_TRANSFER ) ? SD_TRANSFER_OK : SD_TRANSFER_BUSY); 00517 } 00518 00519 00520 /** 00521 * @brief Get SD information about specific SD card. 00522 * @param CardInfo: Pointer to HAL_SD_CardInfoTypedef structure 00523 * @retval None 00524 */ 00525 void BSP_SD_GetCardInfo(HAL_SD_CardInfoTypeDef *CardInfo) 00526 { 00527 /* Get SD card Information */ 00528 HAL_SD_GetCardInfo(&uSdHandle, CardInfo); 00529 } 00530 00531 /** 00532 * @brief SD Abort callbacks 00533 * @param hsd: SD handle 00534 * @retval None 00535 */ 00536 void HAL_SD_AbortCallback(SD_HandleTypeDef *hsd) 00537 { 00538 BSP_SD_AbortCallback(); 00539 } 00540 00541 /** 00542 * @brief Tx Transfer completed callbacks 00543 * @param hsd: SD handle 00544 * @retval None 00545 */ 00546 void HAL_SD_TxCpltCallback(SD_HandleTypeDef *hsd) 00547 { 00548 BSP_SD_WriteCpltCallback(); 00549 } 00550 00551 /** 00552 * @brief Rx Transfer completed callbacks 00553 * @param hsd: SD handle 00554 * @retval None 00555 */ 00556 void HAL_SD_RxCpltCallback(SD_HandleTypeDef *hsd) 00557 { 00558 BSP_SD_ReadCpltCallback(); 00559 } 00560 00561 /** 00562 * @brief BSP SD Abort callbacks 00563 * @retval None 00564 */ 00565 __weak void BSP_SD_AbortCallback(void) 00566 { 00567 00568 } 00569 00570 /** 00571 * @brief BSP Tx Transfer completed callbacks 00572 * @retval None 00573 */ 00574 __weak void BSP_SD_WriteCpltCallback(void) 00575 { 00576 00577 } 00578 00579 /** 00580 * @brief BSP Rx Transfer completed callbacks 00581 * @retval None 00582 */ 00583 __weak void BSP_SD_ReadCpltCallback(void) 00584 { 00585 00586 } 00587 00588 /** 00589 * @} 00590 */ 00591 00592 /** 00593 * @} 00594 */ 00595 00596 /** 00597 * @} 00598 */ 00599 00600 /** 00601 * @} 00602 */ 00603 00604 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri Dec 30 2016 18:30:07 for STM32F769I-Discovery BSP User Manual by
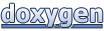