STM32F4xx-Nucleo BSP User Manual
|
stm32f4xx_nucleo.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32f4xx_nucleo.h 00004 * @author MCD Application Team 00005 * @version V1.2.6 00006 * @date 31-January-2017 00007 * @brief This file contains definitions for: 00008 * - LEDs and push-button available on STM32F4XX-Nucleo Kit 00009 * from STMicroelectronics 00010 * - LCD, joystick and microSD available on Adafruit 1.8" TFT LCD 00011 * shield (reference ID 802) 00012 ****************************************************************************** 00013 * @attention 00014 * 00015 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00016 * 00017 * Redistribution and use in source and binary forms, with or without modification, 00018 * are permitted provided that the following conditions are met: 00019 * 1. Redistributions of source code must retain the above copyright notice, 00020 * this list of conditions and the following disclaimer. 00021 * 2. Redistributions in binary form must reproduce the above copyright notice, 00022 * this list of conditions and the following disclaimer in the documentation 00023 * and/or other materials provided with the distribution. 00024 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00025 * may be used to endorse or promote products derived from this software 00026 * without specific prior written permission. 00027 * 00028 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00029 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00030 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00031 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00032 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00033 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00034 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00035 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00036 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00037 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00038 * 00039 ****************************************************************************** 00040 */ 00041 00042 /* Define to prevent recursive inclusion -------------------------------------*/ 00043 #ifndef __STM32F4XX_NUCLEO_H 00044 #define __STM32F4XX_NUCLEO_H 00045 00046 #ifdef __cplusplus 00047 extern "C" { 00048 #endif 00049 00050 /* Includes ------------------------------------------------------------------*/ 00051 #include "stm32f4xx_hal.h" 00052 00053 /* To be defined only if the board is provided with the related shield */ 00054 /* https://www.adafruit.com/products/802 */ 00055 #define ADAFRUIT_TFT_JOY_SD_ID802 00056 00057 /** @addtogroup BSP 00058 * @{ 00059 */ 00060 00061 /** @addtogroup STM32F4XX_NUCLEO 00062 * @{ 00063 */ 00064 00065 /** @addtogroup STM32F4XX_NUCLEO_LOW_LEVEL 00066 * @{ 00067 */ 00068 00069 /** @defgroup STM32F4XX_NUCLEO_LOW_LEVEL_Exported_Types STM32F4XX NUCLEO LOW LEVEL Exported Types 00070 * @{ 00071 */ 00072 typedef enum 00073 { 00074 LED2 = 0 00075 }Led_TypeDef; 00076 00077 typedef enum 00078 { 00079 BUTTON_USER = 0, 00080 /* Alias */ 00081 BUTTON_KEY = BUTTON_USER 00082 } Button_TypeDef; 00083 00084 typedef enum 00085 { 00086 BUTTON_MODE_GPIO = 0, 00087 BUTTON_MODE_EXTI = 1 00088 }ButtonMode_TypeDef; 00089 00090 typedef enum 00091 { 00092 JOY_NONE = 0, 00093 JOY_SEL = 1, 00094 JOY_DOWN = 2, 00095 JOY_LEFT = 3, 00096 JOY_RIGHT = 4, 00097 JOY_UP = 5 00098 }JOYState_TypeDef; 00099 00100 /** 00101 * @} 00102 */ 00103 00104 /** @defgroup STM32F4XX_NUCLEO_LOW_LEVEL_Exported_Constants STM32F4XX NUCLEO LOW LEVEL Exported Constants 00105 * @{ 00106 */ 00107 00108 /** 00109 * @brief Define for STM32F4XX_NUCLEO board 00110 */ 00111 #if !defined (USE_STM32F4XX_NUCLEO) 00112 #define USE_STM32F4XX_NUCLEO 00113 #endif 00114 00115 /** @defgroup STM32F4XX_NUCLEO_LOW_LEVEL_LED STM32F4XX NUCLEO LOW LEVEL LED 00116 * @{ 00117 */ 00118 #define LEDn 1 00119 00120 #define LED2_PIN GPIO_PIN_5 00121 #define LED2_GPIO_PORT GPIOA 00122 #define LED2_GPIO_CLK_ENABLE() __HAL_RCC_GPIOA_CLK_ENABLE() 00123 #define LED2_GPIO_CLK_DISABLE() __HAL_RCC_GPIOA_CLK_DISABLE() 00124 00125 #define LEDx_GPIO_CLK_ENABLE(__INDEX__) LED2_GPIO_CLK_ENABLE() 00126 #define LEDx_GPIO_CLK_DISABLE(__INDEX__) LED2_GPIO_CLK_DISABLE() 00127 /** 00128 * @} 00129 */ 00130 00131 /** @defgroup STM32F4XX_NUCLEO_LOW_LEVEL_BUTTON STM32F4XX NUCLEO LOW LEVEL BUTTON 00132 * @{ 00133 */ 00134 #define BUTTONn 1 00135 00136 /** 00137 * @brief Key push-button 00138 */ 00139 #define USER_BUTTON_PIN GPIO_PIN_13 00140 #define USER_BUTTON_GPIO_PORT GPIOC 00141 #define USER_BUTTON_GPIO_CLK_ENABLE() __HAL_RCC_GPIOC_CLK_ENABLE() 00142 #define USER_BUTTON_GPIO_CLK_DISABLE() __HAL_RCC_GPIOC_CLK_DISABLE() 00143 #define USER_BUTTON_EXTI_LINE GPIO_PIN_13 00144 #define USER_BUTTON_EXTI_IRQn EXTI15_10_IRQn 00145 00146 #define BUTTONx_GPIO_CLK_ENABLE(__INDEX__) USER_BUTTON_GPIO_CLK_ENABLE() 00147 #define BUTTONx_GPIO_CLK_DISABLE(__INDEX__) USER_BUTTON_GPIO_CLK_DISABLE() 00148 00149 /* Aliases */ 00150 #define KEY_BUTTON_PIN USER_BUTTON_PIN 00151 #define KEY_BUTTON_GPIO_PORT USER_BUTTON_GPIO_PORT 00152 #define KEY_BUTTON_GPIO_CLK_ENABLE() USER_BUTTON_GPIO_CLK_ENABLE() 00153 #define KEY_BUTTON_GPIO_CLK_DISABLE() USER_BUTTON_GPIO_CLK_DISABLE() 00154 #define KEY_BUTTON_EXTI_LINE USER_BUTTON_EXTI_LINE 00155 #define KEY_BUTTON_EXTI_IRQn USER_BUTTON_EXTI_IRQn 00156 00157 /** 00158 * @} 00159 */ 00160 00161 /** @defgroup STM32F4XX_NUCLEO_LOW_LEVEL_BUS STM32F4XX NUCLEO LOW LEVEL BUS 00162 * @{ 00163 */ 00164 /*############################### SPI1 #######################################*/ 00165 #ifdef HAL_SPI_MODULE_ENABLED 00166 00167 #define NUCLEO_SPIx SPI1 00168 #define NUCLEO_SPIx_CLK_ENABLE() __HAL_RCC_SPI1_CLK_ENABLE() 00169 00170 #define NUCLEO_SPIx_SCK_AF GPIO_AF5_SPI1 00171 #define NUCLEO_SPIx_SCK_GPIO_PORT GPIOA 00172 #define NUCLEO_SPIx_SCK_PIN GPIO_PIN_5 00173 #define NUCLEO_SPIx_SCK_GPIO_CLK_ENABLE() __HAL_RCC_GPIOA_CLK_ENABLE() 00174 #define NUCLEO_SPIx_SCK_GPIO_CLK_DISABLE() __HAL_RCC_GPIOA_CLK_DISABLE() 00175 00176 #define NUCLEO_SPIx_MISO_MOSI_AF GPIO_AF5_SPI1 00177 #define NUCLEO_SPIx_MISO_MOSI_GPIO_PORT GPIOA 00178 #define NUCLEO_SPIx_MISO_MOSI_GPIO_CLK_ENABLE() __HAL_RCC_GPIOA_CLK_ENABLE() 00179 #define NUCLEO_SPIx_MISO_MOSI_GPIO_CLK_DISABLE() __HAL_RCC_GPIOA_CLK_DISABLE() 00180 #define NUCLEO_SPIx_MISO_PIN GPIO_PIN_6 00181 #define NUCLEO_SPIx_MOSI_PIN GPIO_PIN_7 00182 /* Maximum Timeout values for flags waiting loops. These timeouts are not based 00183 on accurate values, they just guarantee that the application will not remain 00184 stuck if the SPI communication is corrupted. 00185 You may modify these timeout values depending on CPU frequency and application 00186 conditions (interrupts routines ...). */ 00187 #define NUCLEO_SPIx_TIMEOUT_MAX 1000 00188 00189 /** 00190 * @brief SD Control Lines management 00191 */ 00192 #define SD_CS_LOW() HAL_GPIO_WritePin(SD_CS_GPIO_PORT, SD_CS_PIN, GPIO_PIN_RESET) 00193 #define SD_CS_HIGH() HAL_GPIO_WritePin(SD_CS_GPIO_PORT, SD_CS_PIN, GPIO_PIN_SET) 00194 00195 /** 00196 * @brief LCD Control Lines management 00197 */ 00198 #define LCD_CS_LOW() HAL_GPIO_WritePin(LCD_CS_GPIO_PORT, LCD_CS_PIN, GPIO_PIN_RESET) 00199 #define LCD_CS_HIGH() HAL_GPIO_WritePin(LCD_CS_GPIO_PORT, LCD_CS_PIN, GPIO_PIN_SET) 00200 #define LCD_DC_LOW() HAL_GPIO_WritePin(LCD_DC_GPIO_PORT, LCD_DC_PIN, GPIO_PIN_RESET) 00201 #define LCD_DC_HIGH() HAL_GPIO_WritePin(LCD_DC_GPIO_PORT, LCD_DC_PIN, GPIO_PIN_SET) 00202 00203 /** 00204 * @brief SD Control Interface pins (shield D4) 00205 */ 00206 #define SD_CS_PIN GPIO_PIN_5 00207 #define SD_CS_GPIO_PORT GPIOB 00208 #define SD_CS_GPIO_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00209 #define SD_CS_GPIO_CLK_DISABLE() __HAL_RCC_GPIOB_CLK_DISABLE() 00210 00211 /** 00212 * @brief LCD Control Interface pins (shield D10) 00213 */ 00214 #define LCD_CS_PIN GPIO_PIN_6 00215 #define LCD_CS_GPIO_PORT GPIOB 00216 #define LCD_CS_GPIO_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00217 #define LCD_CS_GPIO_CLK_DISABLE() __HAL_RCC_GPIOB_CLK_DISABLE() 00218 00219 /** 00220 * @brief LCD Data/Command Interface pins (shield D8) 00221 */ 00222 #define LCD_DC_PIN GPIO_PIN_9 00223 #define LCD_DC_GPIO_PORT GPIOA 00224 #define LCD_DC_GPIO_CLK_ENABLE() __HAL_RCC_GPIOA_CLK_ENABLE() 00225 #define LCD_DC_GPIO_CLK_DISABLE() __HAL_RCC_GPIOA_CLK_DISABLE() 00226 00227 #endif /* HAL_SPI_MODULE_ENABLED */ 00228 00229 /*################################ ADC1 ######################################*/ 00230 /** 00231 * @brief ADC Interface pins 00232 * used to detect motion of Joystick available on Adafruit 1.8" TFT shield 00233 */ 00234 00235 #ifdef HAL_ADC_MODULE_ENABLED 00236 00237 #define NUCLEO_ADCx ADC1 00238 #define NUCLEO_ADCx_CLK_ENABLE() __HAL_RCC_ADC1_CLK_ENABLE() 00239 #define NUCLEO_ADCx_CLK_DISABLE() __HAL_RCC_ADC1_CLK_DISABLE() 00240 00241 #define NUCLEO_ADCx_CHANNEL ADC_CHANNEL_8 00242 00243 #define NUCLEO_ADCx_GPIO_PORT GPIOB 00244 #define NUCLEO_ADCx_GPIO_PIN GPIO_PIN_0 00245 #define NUCLEO_ADCx_GPIO_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00246 #define NUCLEO_ADCx_GPIO_CLK_DISABLE() __HAL_RCC_GPIOB_CLK_DISABLE() 00247 #endif /* HAL_ADC_MODULE_ENABLED */ 00248 /** 00249 * @} 00250 */ 00251 00252 /** 00253 * @} 00254 */ 00255 00256 /** @defgroup STM32F4XX_NUCLEO_LOW_LEVEL_Exported_Macros STM32F4XX NUCLEO LOW LEVEL Exported Macros 00257 * @{ 00258 */ 00259 /** 00260 * @} 00261 */ 00262 00263 /** @defgroup STM32F4XX_NUCLEO_LOW_LEVEL_Exported_Functions STM32F4XX NUCLEO LOW LEVEL Exported Functions 00264 * @{ 00265 */ 00266 uint32_t BSP_GetVersion(void); 00267 void BSP_LED_Init(Led_TypeDef Led); 00268 void BSP_LED_DeInit(Led_TypeDef Led); 00269 void BSP_LED_On(Led_TypeDef Led); 00270 void BSP_LED_Off(Led_TypeDef Led); 00271 void BSP_LED_Toggle(Led_TypeDef Led); 00272 void BSP_PB_Init(Button_TypeDef Button, ButtonMode_TypeDef ButtonMode); 00273 void BSP_PB_DeInit(Button_TypeDef Button); 00274 uint32_t BSP_PB_GetState(Button_TypeDef Button); 00275 #ifdef HAL_ADC_MODULE_ENABLED 00276 uint8_t BSP_JOY_Init(void); 00277 JOYState_TypeDef BSP_JOY_GetState(void); 00278 void BSP_JOY_DeInit(void); 00279 #endif /* HAL_ADC_MODULE_ENABLED */ 00280 00281 00282 /** 00283 * @} 00284 */ 00285 00286 /** 00287 * @} 00288 */ 00289 00290 /** 00291 * @} 00292 */ 00293 00294 /** 00295 * @} 00296 */ 00297 00298 #ifdef __cplusplus 00299 } 00300 #endif 00301 00302 #endif /* __STM32F4XX_NUCLEO_H */ 00303 00304 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri Jan 20 2017 15:36:09 for STM32F4xx-Nucleo BSP User Manual by
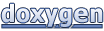