STM32F469I-Discovery BSP User Manual
|
stm32469i_discovery_ts.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32469i_discovery_ts.h 00004 * @author MCD Application Team 00005 * @version V1.0.2 00006 * @date 13-January-2016 00007 * @brief This file contains the common defines and functions prototypes for 00008 * the stm32469i_discovery_ts.c driver. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* Define to prevent recursive inclusion -------------------------------------*/ 00040 #ifndef __STM32469I_DISCOVERY_TS_H 00041 #define __STM32469I_DISCOVERY_TS_H 00042 00043 #ifdef __cplusplus 00044 extern "C" { 00045 #endif 00046 00047 /* Includes ------------------------------------------------------------------*/ 00048 #include "stm32469i_discovery.h" 00049 #include "stm32469i_discovery_lcd.h" 00050 00051 /* Include TouchScreen component driver */ 00052 #include "../Components/ft6x06/ft6x06.h" 00053 00054 /** @addtogroup BSP 00055 * @{ 00056 */ 00057 00058 /** @addtogroup STM32469I_Discovery 00059 * @{ 00060 */ 00061 00062 /** @addtogroup STM32469I-Discovery_TS 00063 * @{ 00064 */ 00065 00066 /** @defgroup STM32469I-Discovery_TS_Exported_Constants STM32469I Discovery TS Exported Constants 00067 * @{ 00068 */ 00069 /** @brief With FT6206 : maximum 2 touches detected simultaneously 00070 */ 00071 #define TS_MAX_NB_TOUCH ((uint32_t) FT6206_MAX_DETECTABLE_TOUCH) 00072 #define TS_NO_IRQ_PENDING ((uint8_t) 0) 00073 #define TS_IRQ_PENDING ((uint8_t) 1) 00074 00075 #define TS_SWAP_NONE ((uint8_t) 0x01) 00076 #define TS_SWAP_X ((uint8_t) 0x02) 00077 #define TS_SWAP_Y ((uint8_t) 0x04) 00078 #define TS_SWAP_XY ((uint8_t) 0x08) 00079 00080 /** 00081 * @} 00082 */ 00083 00084 /** @defgroup STM32469I-Discovery_TS_Exported_Types STM32469I Discovery TS Exported Types 00085 * @{ 00086 */ 00087 /** 00088 * @brief TS_StateTypeDef 00089 * Define TS State structure 00090 */ 00091 typedef struct 00092 { 00093 uint8_t touchDetected; /*!< Total number of active touches detected at last scan */ 00094 uint16_t touchX[TS_MAX_NB_TOUCH]; /*!< Touch X[0], X[1] coordinates on 12 bits */ 00095 uint16_t touchY[TS_MAX_NB_TOUCH]; /*!< Touch Y[0], Y[1] coordinates on 12 bits */ 00096 00097 #if (TS_MULTI_TOUCH_SUPPORTED == 1) 00098 uint8_t touchWeight[TS_MAX_NB_TOUCH]; /*!< Touch_Weight[0], Touch_Weight[1] : weight property of touches */ 00099 uint8_t touchEventId[TS_MAX_NB_TOUCH]; /*!< Touch_EventId[0], Touch_EventId[1] : take value of type @ref TS_TouchEventTypeDef */ 00100 uint8_t touchArea[TS_MAX_NB_TOUCH]; /*!< Touch_Area[0], Touch_Area[1] : touch area of each touch */ 00101 uint32_t gestureId; /*!< type of gesture detected : take value of type @ref TS_GestureIdTypeDef */ 00102 #endif /* TS_MULTI_TOUCH_SUPPORTED == 1 */ 00103 00104 } TS_StateTypeDef; 00105 00106 /** 00107 * @brief TS_StatusTypeDef 00108 * Define BSP_TS_xxx() functions possible return value, 00109 * when status is returned by those functions. 00110 */ 00111 typedef enum 00112 { 00113 TS_OK = 0x00, /*!< Touch Ok */ 00114 TS_ERROR = 0x01, /*!< Touch Error */ 00115 TS_TIMEOUT = 0x02, /*!< Touch Timeout */ 00116 TS_DEVICE_NOT_FOUND = 0x03 /*!< Touchscreen device not found */ 00117 } TS_StatusTypeDef; 00118 00119 /** 00120 * @brief TS_GestureIdTypeDef 00121 * Define Possible managed gesture identification values returned by touch screen 00122 * driver. 00123 */ 00124 typedef enum 00125 { 00126 GEST_ID_NO_GESTURE = 0x00, /*!< Gesture not defined / recognized */ 00127 GEST_ID_MOVE_UP = 0x01, /*!< Gesture Move Up */ 00128 GEST_ID_MOVE_RIGHT = 0x02, /*!< Gesture Move Right */ 00129 GEST_ID_MOVE_DOWN = 0x03, /*!< Gesture Move Down */ 00130 GEST_ID_MOVE_LEFT = 0x04, /*!< Gesture Move Left */ 00131 GEST_ID_ZOOM_IN = 0x05, /*!< Gesture Zoom In */ 00132 GEST_ID_ZOOM_OUT = 0x06, /*!< Gesture Zoom Out */ 00133 GEST_ID_NB_MAX = 0x07 /*!< max number of gesture id */ 00134 } TS_GestureIdTypeDef; 00135 00136 /** 00137 * @brief TS_TouchEventTypeDef 00138 * Define Possible touch events kind as returned values 00139 * by touch screen IC Driver. 00140 */ 00141 typedef enum 00142 { 00143 TOUCH_EVENT_NO_EVT = 0x00, /*!< Touch Event : undetermined */ 00144 TOUCH_EVENT_PRESS_DOWN = 0x01, /*!< Touch Event Press Down */ 00145 TOUCH_EVENT_LIFT_UP = 0x02, /*!< Touch Event Lift Up */ 00146 TOUCH_EVENT_CONTACT = 0x03, /*!< Touch Event Contact */ 00147 TOUCH_EVENT_NB_MAX = 0x04 /*!< max number of touch events kind */ 00148 } TS_TouchEventTypeDef; 00149 00150 /** 00151 * @} 00152 */ 00153 00154 /** @defgroup STM32469I-Discovery_TS_Imported_Variables STM32469I Discovery TS Imported Variables 00155 * @{ 00156 */ 00157 /** 00158 * @brief Table for touchscreen event information display on LCD : 00159 * table indexed on enum @ref TS_TouchEventTypeDef information 00160 */ 00161 extern char * ts_event_string_tab[TOUCH_EVENT_NB_MAX]; 00162 00163 /** 00164 * @brief Table for touchscreen gesture Id information display on LCD : table indexed 00165 * on enum @ref TS_GestureIdTypeDef information 00166 */ 00167 extern char * ts_gesture_id_string_tab[GEST_ID_NB_MAX]; 00168 /** 00169 * @} 00170 */ 00171 00172 /** @defgroup STM32469I-Discovery_TS_Exported_Functions STM32469I Discovery TS Exported Functions 00173 * @{ 00174 */ 00175 uint8_t BSP_TS_Init(uint16_t ts_SizeX, uint16_t ts_SizeY); 00176 uint8_t BSP_TS_GetState(TS_StateTypeDef *TS_State); 00177 00178 #if (TS_MULTI_TOUCH_SUPPORTED == 1) 00179 uint8_t BSP_TS_Get_GestureId(TS_StateTypeDef *TS_State); 00180 uint8_t BSP_TS_ResetTouchData(TS_StateTypeDef *TS_State); 00181 #endif /* TS_MULTI_TOUCH_SUPPORTED == 1 */ 00182 00183 uint8_t BSP_TS_ITConfig(void); 00184 00185 00186 /* These __weak function can be surcharged by application code in case the current settings 00187 need to be changed for specific (example GPIO allocation) */ 00188 void BSP_TS_INT_MspInit(void); 00189 00190 /** 00191 * @} 00192 */ 00193 00194 /** 00195 * @} 00196 */ 00197 00198 /** 00199 * @} 00200 */ 00201 00202 /** 00203 * @} 00204 */ 00205 00206 00207 #ifdef __cplusplus 00208 } 00209 #endif 00210 00211 #endif /* __STM32469I_DISCOVERY_TS_H */ 00212 00213 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Wed Jan 13 2016 10:58:46 for STM32F469I-Discovery BSP User Manual by
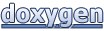