STM32F469I-Discovery BSP User Manual
|
stm32469i_discovery_sdram.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32469i_discovery_sdram.h 00004 * @author MCD Application Team 00005 * @version V1.0.2 00006 * @date 13-January-2016 00007 * @brief This file contains the common defines and functions prototypes for 00008 * the stm32469i_discovery_sdram.c driver. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* Define to prevent recursive inclusion -------------------------------------*/ 00040 #ifndef __STM32469I_DISCOVERY_SDRAM_H 00041 #define __STM32469I_DISCOVERY_SDRAM_H 00042 00043 #ifdef __cplusplus 00044 extern "C" { 00045 #endif 00046 00047 /* Includes ------------------------------------------------------------------*/ 00048 #include "stm32f4xx_hal.h" 00049 00050 /** @addtogroup BSP 00051 * @{ 00052 */ 00053 00054 /** @addtogroup STM32469I_Discovery 00055 * @{ 00056 */ 00057 00058 /** @addtogroup STM32469I-Discovery_SDRAM 00059 * @{ 00060 */ 00061 00062 /** @defgroup STM32469I-Discovery_SDRAM_Exported_Types STM32469I Discovery SDRAM Exported Types 00063 * @{ 00064 */ 00065 00066 /** 00067 * @brief SDRAM status structure definition 00068 */ 00069 #define SDRAM_OK ((uint8_t)0x00) 00070 #define SDRAM_ERROR ((uint8_t)0x01) 00071 00072 /** 00073 * @} 00074 */ 00075 00076 /** @defgroup STM32469I-Discovery_SDRAM_Exported_Constants STM32469I Discovery SDRAM Exported Constants 00077 * @{ 00078 */ 00079 #define SDRAM_DEVICE_ADDR ((uint32_t)0xC0000000) 00080 00081 /* SDRAM device size in MBytes */ 00082 #define SDRAM_DEVICE_SIZE ((uint32_t)0x800000) 00083 00084 #define SDRAM_MEMORY_WIDTH FMC_SDRAM_MEM_BUS_WIDTH_32 00085 #define SDCLOCK_PERIOD FMC_SDRAM_CLOCK_PERIOD_2 00086 00087 /* SDRAM refresh counter (90 MHz SD clock) */ 00088 #define REFRESH_COUNT ((uint32_t)0x0569) 00089 #define SDRAM_TIMEOUT ((uint32_t)0xFFFF) 00090 00091 /* DMA definitions for SDRAM DMA transfer */ 00092 #define __DMAx_CLK_ENABLE __HAL_RCC_DMA2_CLK_ENABLE 00093 #define __DMAx_CLK_DISABLE __HAL_RCC_DMA2_CLK_DISABLE 00094 #define SDRAM_DMAx_CHANNEL DMA_CHANNEL_0 00095 #define SDRAM_DMAx_STREAM DMA2_Stream0 00096 #define SDRAM_DMAx_IRQn DMA2_Stream0_IRQn 00097 #define SDRAM_DMAx_IRQHandler DMA2_Stream0_IRQHandler 00098 00099 00100 /** 00101 * @brief FMC SDRAM Mode definition register defines 00102 */ 00103 #define SDRAM_MODEREG_BURST_LENGTH_1 ((uint16_t)0x0000) 00104 #define SDRAM_MODEREG_BURST_LENGTH_2 ((uint16_t)0x0001) 00105 #define SDRAM_MODEREG_BURST_LENGTH_4 ((uint16_t)0x0002) 00106 #define SDRAM_MODEREG_BURST_LENGTH_8 ((uint16_t)0x0004) 00107 #define SDRAM_MODEREG_BURST_TYPE_SEQUENTIAL ((uint16_t)0x0000) 00108 #define SDRAM_MODEREG_BURST_TYPE_INTERLEAVED ((uint16_t)0x0008) 00109 #define SDRAM_MODEREG_CAS_LATENCY_2 ((uint16_t)0x0020) 00110 #define SDRAM_MODEREG_CAS_LATENCY_3 ((uint16_t)0x0030) 00111 #define SDRAM_MODEREG_OPERATING_MODE_STANDARD ((uint16_t)0x0000) 00112 #define SDRAM_MODEREG_WRITEBURST_MODE_PROGRAMMED ((uint16_t)0x0000) 00113 #define SDRAM_MODEREG_WRITEBURST_MODE_SINGLE ((uint16_t)0x0200) 00114 /** 00115 * @} 00116 */ 00117 00118 /** @defgroup STM32469I-Discovery_SDRAM_Exported_Macro STM32469I Discovery SDRAM Exported Macro 00119 * @{ 00120 */ 00121 /** 00122 * @} 00123 */ 00124 00125 /** @addtogroup STM32469I_Discovery_SDRAM_Exported_Functions 00126 * @{ 00127 */ 00128 uint8_t BSP_SDRAM_Init(void); 00129 uint8_t BSP_SDRAM_DeInit(void); 00130 void BSP_SDRAM_Initialization_sequence(uint32_t RefreshCount); 00131 uint8_t BSP_SDRAM_ReadData(uint32_t uwStartAddress, uint32_t *pData, uint32_t uwDataSize); 00132 uint8_t BSP_SDRAM_ReadData_DMA(uint32_t uwStartAddress, uint32_t *pData, uint32_t uwDataSize); 00133 uint8_t BSP_SDRAM_WriteData(uint32_t uwStartAddress, uint32_t *pData, uint32_t uwDataSize); 00134 uint8_t BSP_SDRAM_WriteData_DMA(uint32_t uwStartAddress, uint32_t *pData, uint32_t uwDataSize); 00135 uint8_t BSP_SDRAM_Sendcmd(FMC_SDRAM_CommandTypeDef *SdramCmd); 00136 void BSP_SDRAM_DMA_IRQHandler(void); 00137 00138 /* These function can be modified in case the current settings (e.g. DMA stream) 00139 need to be changed for specific application needs */ 00140 void BSP_SDRAM_MspInit(SDRAM_HandleTypeDef *hsdram, void *Params); 00141 void BSP_SDRAM_MspDeInit(SDRAM_HandleTypeDef *hsdram, void *Params); 00142 00143 /** 00144 * @} 00145 */ 00146 00147 /** 00148 * @} 00149 */ 00150 00151 /** 00152 * @} 00153 */ 00154 00155 /** 00156 * @} 00157 */ 00158 00159 /** 00160 * @} 00161 */ 00162 00163 #ifdef __cplusplus 00164 } 00165 #endif 00166 00167 #endif /* __STM32469I_DISCOVERY_SDRAM_H */ 00168 00169 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Wed Jan 13 2016 10:58:46 for STM32F469I-Discovery BSP User Manual by
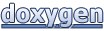