STM32F469I-Discovery BSP User Manual
|
stm32469i_discovery_qspi.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32469i_discovery_qspi.h 00004 * @author MCD Application Team 00005 * @version V1.0.2 00006 * @date 13-January-2016 00007 * @brief This file contains the common defines and functions prototypes for 00008 * the stm32469i_discovery_qspi.c driver. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /** @addtogroup BSP 00040 * @{ 00041 */ 00042 00043 /** @addtogroup STM32469I_Discovery 00044 * @{ 00045 */ 00046 00047 /* Define to prevent recursive inclusion -------------------------------------*/ 00048 #ifndef __STM32469I_DISCOVERY_QSPI_H 00049 #define __STM32469I_DISCOVERY_QSPI_H 00050 00051 #ifdef __cplusplus 00052 extern "C" { 00053 #endif 00054 00055 /* Includes ------------------------------------------------------------------*/ 00056 #include "stm32f4xx_hal.h" 00057 #include "../Components/n25q128a/n25q128a.h" 00058 00059 00060 /** @addtogroup STM32469I_Discovery_QSPI 00061 * @{ 00062 */ 00063 00064 00065 /* Exported constants --------------------------------------------------------*/ 00066 /** @defgroup STM32469I_Discovery_QSPI_Exported_Constants STM32469I Discovery QSPI Exported Constants 00067 * @{ 00068 */ 00069 /* QSPI Error codes */ 00070 #define QSPI_OK ((uint8_t)0x00) 00071 #define QSPI_ERROR ((uint8_t)0x01) 00072 #define QSPI_BUSY ((uint8_t)0x02) 00073 #define QSPI_NOT_SUPPORTED ((uint8_t)0x04) 00074 #define QSPI_SUSPENDED ((uint8_t)0x08) 00075 00076 00077 /* Definition for QSPI clock resources */ 00078 #define QSPI_CLK_ENABLE() __HAL_RCC_QSPI_CLK_ENABLE() 00079 #define QSPI_CLK_DISABLE() __HAL_RCC_QSPI_CLK_DISABLE() 00080 #define QSPI_CS_GPIO_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00081 #define QSPI_CS_GPIO_CLK_DISABLE() __HAL_RCC_GPIOB_CLK_DISABLE() 00082 #define QSPI_DX_CLK_GPIO_CLK_ENABLE() __HAL_RCC_GPIOF_CLK_ENABLE() 00083 #define QSPI_DX_CLK_GPIO_CLK_DISABLE() __HAL_RCC_GPIOF_CLK_DISABLE() 00084 00085 #define QSPI_FORCE_RESET() __HAL_RCC_QSPI_FORCE_RESET() 00086 #define QSPI_RELEASE_RESET() __HAL_RCC_QSPI_RELEASE_RESET() 00087 00088 /* Definition for QSPI Pins */ 00089 #define QSPI_CS_PIN GPIO_PIN_6 00090 #define QSPI_CS_GPIO_PORT GPIOB 00091 #define QSPI_CLK_PIN GPIO_PIN_10 00092 #define QSPI_CLK_GPIO_PORT GPIOF 00093 #define QSPI_D0_PIN GPIO_PIN_8 00094 #define QSPI_D1_PIN GPIO_PIN_9 00095 #define QSPI_D2_PIN GPIO_PIN_7 00096 #define QSPI_D3_PIN GPIO_PIN_6 00097 #define QSPI_DX_GPIO_PORT GPIOF 00098 00099 00100 /** 00101 * @} 00102 */ 00103 00104 /* Exported types ------------------------------------------------------------*/ 00105 /** @defgroup STM32469I_Discovery_QSPI_Exported_Types STM32469I Discovery QSPI Exported Types 00106 * @{ 00107 */ 00108 /** 00109 * @brief QSPI Info 00110 * */ 00111 typedef struct { 00112 uint32_t FlashSize; /*!< Size of the flash */ 00113 uint32_t EraseSectorSize; /*!< Size of sectors for the erase operation */ 00114 uint32_t EraseSectorsNumber; /*!< Number of sectors for the erase operation */ 00115 uint32_t ProgPageSize; /*!< Size of pages for the program operation */ 00116 uint32_t ProgPagesNumber; /*!< Number of pages for the program operation */ 00117 } QSPI_InfoTypeDef; 00118 00119 /** 00120 * @} 00121 */ 00122 00123 00124 /* Exported functions --------------------------------------------------------*/ 00125 /** @addtogroup STM32469I_Discovery_QSPI_Exported_Functions STM32469I Discovery QSPI Exported Functions 00126 * @{ 00127 */ 00128 uint8_t BSP_QSPI_Init (void); 00129 uint8_t BSP_QSPI_DeInit (void); 00130 uint8_t BSP_QSPI_Read (uint8_t* pData, uint32_t ReadAddr, uint32_t Size); 00131 uint8_t BSP_QSPI_Write (uint8_t* pData, uint32_t WriteAddr, uint32_t Size); 00132 uint8_t BSP_QSPI_Erase_Block(uint32_t BlockAddress); 00133 uint8_t BSP_QSPI_Erase_Chip (void); 00134 uint8_t BSP_QSPI_GetStatus (void); 00135 uint8_t BSP_QSPI_GetInfo (QSPI_InfoTypeDef* pInfo); 00136 uint8_t BSP_QSPI_MemoryMappedMode(void); 00137 00138 /* These function can be modified in case the current settings (e.g. DMA stream) 00139 need to be changed for specific application needs */ 00140 void BSP_QSPI_MspInit(QSPI_HandleTypeDef *hqspi, void *Params); 00141 void BSP_QSPI_MspDeInit(QSPI_HandleTypeDef *hqspi, void *Params); 00142 00143 /** 00144 * @} 00145 */ 00146 00147 /** 00148 * @} 00149 */ 00150 00151 #ifdef __cplusplus 00152 } 00153 #endif 00154 00155 #endif /* __STM32469I_DISCOVERY_QSPI_H */ 00156 /** 00157 * @} 00158 */ 00159 00160 /** 00161 * @} 00162 */ 00163 00164 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Wed Jan 13 2016 10:58:45 for STM32F469I-Discovery BSP User Manual by
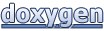